Function Components Cannot Be Given Refs
In React, refs are a powerful feature that allow us to reference and interact with DOM elements or React components. They provide a way to access and manipulate the underlying elements or components. However, when it comes to function components, there is a limitation: function components cannot be given refs directly.
In this article, we will explore the reasons behind this limitation, understand the concept of useRef(), and learn about a workaround called forwardRef that allows us to use refs with function components. We will also discuss how to pass refs from a child to a parent component and explore alternatives such as callback refs and getting the ref of a child function component.
Why can’t function components have refs?
Before we dive into the solution, let’s first understand why this limitation exists in the first place. The reason function components cannot have refs is related to how they differ from class components.
In React, there are two types of components: class components and function components. Class components are defined using the ES6 class syntax, while function components are regular JavaScript functions that accept props and return JSX. Class components have a built-in instance and can be instantiated, whereas function components are stateless. They don’t have instances and can be thought of as being instantiated on every render.
In the past, refs were only supported for class components, as class components had instances and the ref could be directly accessed. However, with the introduction of function components and the increasing popularity of functional programming in React, a new solution was required to enable the use of refs in function components. That’s where the useRef() and forwardRef concepts come into play.
Using useRef() instead
To work around the limitation of function components not being able to have refs, React introduced the useRef() hook. useRef() allows us to create a mutable value that persists across renders. We can then use this ref to reference DOM elements or React components.
Using useRef() is fairly straightforward. We begin by importing it from the ‘react’ package:
“`
import React, { useRef } from ‘react’;
“`
We can then create a ref by calling the useRef() function:
“`
const myRef = useRef();
“`
And finally, we can attach the created ref to an element by using the ref prop:
“`
return ;
“`
With this approach, we can access and manipulate the underlying DOM element or React component using the current property of the ref:
“`
console.log(myRef.current.value); // Accessing value of the input
myRef.current.focus(); // Focusing on the input
“`
ForwardRef: Enabling refs in function components
While useRef() provides a way to create refs in function components, there might be scenarios where we need to pass the ref from a parent component to a child function component. This is where forwardRef comes in handy.
forwardRef is a higher-order function provided by React that allows us to forward a ref from a parent component to a child component. This enables us to use refs in function components just like we would with class components.
To use forwardRef, we start by importing it from the ‘react’ package:
“`
import React, { forwardRef } from ‘react’;
“`
We can then define our child component using the forwardRef function:
“`
const ChildComponent = forwardRef((props, ref) => {
return ;
});
“`
In the above example, the ref argument is automatically passed as the second argument to the forwardRef callback function. We can then attach this ref to an element within our child component, just like we did with class components.
To use this child component within a parent component, we create a ref using useRef() and pass it as a prop to the child component:
“`
const ParentComponent = () => {
const childRef = useRef();
return
};
“`
Now, the parent component has access to the ref of the child component, and we can use it to manipulate the underlying element or component.
Passing ref from child to parent in functional components
In some cases, we might need to pass the ref from a child component to its parent component. This can be achieved by utilizing the forwardRef function along with a callback ref.
A callback ref is a function that gets called with the DOM element or component instance when the ref is attached or detached. This provides us with an opportunity to pass the ref value up to the parent component.
Here’s an example of how this can be done:
“`
const ChildComponent = forwardRef((props, ref) => {
useEffect(() => {
props.passRef(ref);
}, [ref]);
return ;
});
const ParentComponent = () => {
const childRef = useRef();
const handleRef = (ref) => {
childRef.current = ref;
};
return
};
“`
In the above example, the ChildComponent invokes the passRef prop, which is a callback, passing the ref value whenever it changes. The ParentComponent receives and stores the childRef using the handleRef function and can then utilize it as required.
FAQs
Q: Can I use string refs in function components?
A: No, you cannot use string refs in function components. Instead, it is recommended to use useRef() in function components.
Q: Can I use refs with class components?
A: Yes, class components in React support direct use of refs. You can define a ref with the React.createRef() syntax and attach it to elements within the class component.
Q: Are there any performance implications of using forwardRef and refs in function components?
A: While forwardRef and refs in function components are generally performant, it’s important to note that overusing them can lead to unnecessary re-renders. It’s always recommended to consider the performance impact of using refs in function components and optimize as necessary.
Q: Are refs only used to reference DOM elements or can they be used with other React components?
A: Refs can be used to reference and interact with both DOM elements and other React components. They provide a way to access underlying elements or components and perform actions on them.
In conclusion, while function components have a limitation when it comes to using refs directly, React provides us with powerful alternatives such as useRef() and forwardRef to overcome this limitation. By utilizing these techniques, we can effectively use refs with function components, passing them from parent to child and even from child to parent. With a deeper understanding of these concepts, you can confidently implement and leverage refs in your React applications.
Reactjs Tutorial – 30 – Forwarding Refs
Keywords searched by users: function components cannot be given refs forwardRef, React forwardRef, Function components cannot have string refs we recommend using useRef() instead, React ref functional component, Pass ref from child to parent react functional component, forwardRef React functional component, React callback ref, React Get ref of child
Categories: Top 62 Function Components Cannot Be Given Refs
See more here: nhanvietluanvan.com
Forwardref
React has become one of the most popular JavaScript libraries for building user interfaces. It offers developers a declarative and efficient way to design complex applications by dividing them into reusable components. However, when it comes to managing component instances or passing refs between parent and child components, React can present some challenges. That’s where forwardRef comes in.
In this article, we’ll dive deep into the forwardRef feature in React and explore how it can help you overcome these challenges. We’ll cover everything from its basic usage to some advanced concepts and best practices. So, let’s get started!
Understanding forwardRef
The forwardRef is a feature introduced in React 16.3 as a way to pass refs from a parent component to a child component. It allows you to access and manipulate child component instances directly from the parent component. This way, you can avoid common issues related to ref propagation and provide a more flexible and controlled communication between components.
Using forwardRef
To use forwardRef, you need to define a component that accepts a ref from its parent component. This can be done by calling the forwardRef method, which takes a render function as its argument. Inside this render function, you can define the child component and pass the ref to it.
Here’s an example that demonstrates how to use forwardRef in React:
“`jsx
const ChildComponent = React.forwardRef((props, ref) => {
return ;
});
const ParentComponent = () => {
const childRef = React.createRef();
const handleClick = () => {
childRef.current.focus();
};
return (
);
};
“`
In this example, the ParentComponent creates a ref using the createRef() method. It then passes this ref to the ChildComponent using the forwardRef method. Inside the ChildComponent, the ref is attached to an input element, allowing the parent to access and manipulate it.
Handling forwardRef in Higher-Order Components (HOCs)
Forwarding refs can become more complex when you work with Higher-Order Components (HOCs). HOCs are functions that take a component and return a new component with extended functionality. If you want to forward refs through an HOC, you need to be mindful of how the ref is passed along.
To achieve this, you can use the forwardRef method twice: once when defining the HOC and again when rendering the wrapped component. This ensures that the ref is correctly forwarded to the final component.
Here’s an example to illustrate this:
“`jsx
function hoc(WrappedComponent) {
function HOC(props, ref) {
return
}
return React.forwardRef(HOC);
}
“`
In this example, the hoc function takes a WrappedComponent and returns a new HOC component. The ref is passed along from the HOC to the WrappedComponent, allowing refs to be forwarded correctly.
Best Practices and FAQs
Q: When should I use forwardRef?
A: You should consider using forwardRef when you need to access or manipulate child component instances from a parent component, especially when dealing with complex UI components.
Q: Can I use forwardRef with functional components?
A: Yes, forwardRef works with both functional and class components.
Q: Can I forward refs through multiple levels of components?
A: Yes, forwardRef can be used to forward refs through multiple levels of components. However, keep in mind that each component in the chain must support forwarding.
Q: Is there a performance impact when using forwardRef?
A: Using forwardRef itself doesn’t have a significant performance impact. However, make sure to use it when necessary, as unnecessary usage may introduce unnecessary complexity to your codebase.
Q: Can I use forwardRef with Context API?
A: Yes, forwardRef can be used in conjunction with the Context API to pass refs through components wrapped in a context provider.
In conclusion, forwardRef is a powerful feature in React that allows you to pass refs from a parent component to a child component. This helps in managing component instances and ref propagation, providing a more controlled and flexible communication approach. By understanding its usage and best practices, you’ll be better equipped to design complex React applications with ease.
React Forwardref
What is forwardRef?
ForwardRef is a built-in function in React that enables the component to pass down the ref props it receives to one of its child components. This feature is particularly useful when you want to access or interact with the DOM nodes of a child component from its parent. ForwardRef allows you to maintain a direct reference to the underlying element or component, rather than a reference to the component wrapper.
How does forwardRef work?
To understand how forwardRef works, let’s consider an example. Imagine you have a custom input component called `CustomInput`, and you want to focus on the input field programmatically from its parent component. Here’s how you can achieve this using forwardRef:
“`jsx
// CustomInput.js
import React from ‘react’;
const CustomInput = React.forwardRef((props, ref) => {
return (
);
});
export default CustomInput;
“`
In this example, we define the `CustomInput` component using `forwardRef`. The function passed to `forwardRef` receives two parameters: `props`, which contains the component’s props, and `ref`, which represents the forwarded ref.
Now, in the parent component, you can create a ref and pass it to the `CustomInput` component:
“`jsx
// ParentComponent.js
import React, { useRef } from ‘react’;
import CustomInput from ‘./CustomInput’;
const ParentComponent = () => {
const inputRef = useRef();
const focusInput = () => {
inputRef.current.focus();
};
return (
);
};
export default ParentComponent;
“`
Here, by passing the `inputRef` to the `CustomInput` component, the ref is accessible inside the `CustomInput` component, and the `ref` prop is set to the `input` element. This allows the parent component to call `focus()` on the ref, resulting in the input field receiving focus when the `Focus Input` button is clicked.
Using forwardRef enables you to extend the functionality of the child component and use it in various scenarios without having to modify its internals or exposing its implementation details.
FAQs about forwardRef:
Q: Can I use forwardRef with functional components?
A: Yes, forwardRef works perfectly with functional components. It allows you to wrap any functional component and pass down the ref to a child component.
Q: How can I forward refs through multiple levels of components?
A: React supports forwarding refs through multiple levels of components. Simply use `React.forwardRef` at each level to forward the ref to the next level until it reaches the desired child component.
Q: Can I customize the ref name while forwarding it?
A: Yes, you can choose a custom name for the forwarded ref by assigning it to another prop name. For example, you can pass `innerRef` instead of `ref` in the `CustomInput` component and use `innerRef` instead of `ref` inside the component.
Q: Are there any limitations to using forwardRef in React?
A: While forwardRef is a powerful feature, it should be used judiciously. It is important to note that forwardRef breaks encapsulation, as it exposes internal components. Additionally, using too many forwarded refs can make your component hierarchy harder to understand and maintain. Consider using forwardRef sparingly when necessary.
In conclusion, React forwardRef is an essential feature that facilitates the communication between parent and child components by forwarding refs. It allows you to access and manipulate the underlying DOM nodes or components without exposing their implementation details. By leveraging forwardRef, you can extend the functionality of components and create more flexible and interactive React applications.
Function Components Cannot Have String Refs We Recommend Using Useref() Instead
In the realm of React, there are times when we want to refer to a specific element or component using a reference. Traditionally, this was accomplished by providing a string ref, but in recent versions of React, the use of string refs in function components has been deprecated. Instead, React recommends using the useRef() Hook, which provides a more robust and future-proof solution. In this article, we will explore the reasons behind this deprecation, the advantages of using useRef(), and address some frequently asked questions regarding this topic.
Why are string refs deprecated in function components?
React is constantly evolving, striving to improve performance and provide a more intuitive programming experience. One aspect that impeded this progression was the use of string refs in function components. String refs were less reliable and could lead to potential bugs. Moreover, they did not fit well with the React design philosophy of explicitly and declaratively describing the state and behavior of components.
Refactoring function components to use string refs was also a complex and error-prone task. Developers often had to resort to workarounds or third-party libraries to achieve the desired functionality. This introduced unnecessary complexity and made the codebase harder to maintain and reason about.
To address these issues, React 16.13 introduced a warning when using string refs in function components, and starting with React 17, it became invalid to do so. This change not only promotes better coding practices but also prepares the ecosystem for future optimizations and improvements in React.
The useRef() Hook: A better alternative
React’s useRef() Hook provides a safer and more efficient mechanism for accessing and manipulating DOM elements or other entities in function components. The useRef() Hook returns a mutable ref object that persists across re-renders, preserving its value between render cycles.
By employing useRef(), we can achieve the same functionality as string refs while adhering to React’s principles. The useRef() Hook can be used to hold references to DOM elements, previous values, or any other mutable value within a function component. Its syntax is simple:
const refContainer = useRef(initialValue);
To access the current value of the ref, use refContainer.current. Additionally, the ref object returned by useRef() can be passed as a ref prop to a React element, allowing us to access and modify its properties in a more controlled manner.
Benefits of using useRef()
1. Compatibility with hooks: useRef() seamlessly integrates with other hooks, such as useEffect(), useCallback(), or useState(), enabling smooth coordination between different parts of the component.
2. Avoiding unnecessary re-renders: useRef() can be used to store intermediate or derived values that don’t need to trigger re-renders. This optimization can significantly improve the performance of complex components.
3. Reference management: useRef() simplifies managing references by encapsulating all required references within a single object, reducing the cognitive load on the developer and removing potential bugs.
FAQs:
Q1. Can I still use string refs in class components?
Yes, string refs are still valid in class components. However, it is recommended to migrate to using the useRef() Hook to keep your codebase consistent and future-proof.
Q2. What if I need to access a child component’s DOM node?
You can still achieve this by using the useRef() Hook. The ref object returned by useRef() can be passed as a prop to the child component, allowing you to access its properties and methods.
Q3. Are there any performance differences between string refs and useRef()?
The use of useRef() is generally more performant than string refs. This is because useRef() doesn’t cause a re-render when the ref value changes, while string refs do. In complex components, this can lead to substantial performance gains.
Q4. Can useRef() be used for managing state in function components?
No, useRef() is primarily meant for managing references, not state. For state management, you should still use useState() or other appropriate hooks.
In conclusion, React’s deprecation of string refs in function components and the introduction of the useRef() Hook represent a significant step forward in the evolution of the library. By embracing useRef(), developers can adhere to best practices, improve code readability, and prepare their codebase for future React optimizations. So, make the transition and unlock the full potential of function components.
Images related to the topic function components cannot be given refs
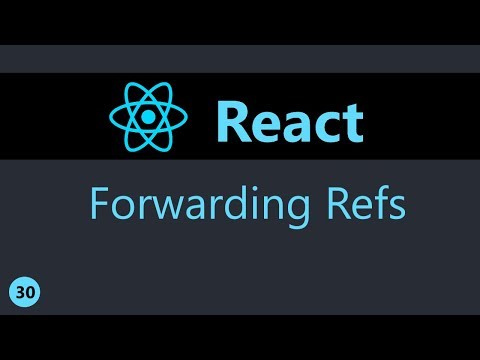
Found 6 images related to function components cannot be given refs theme
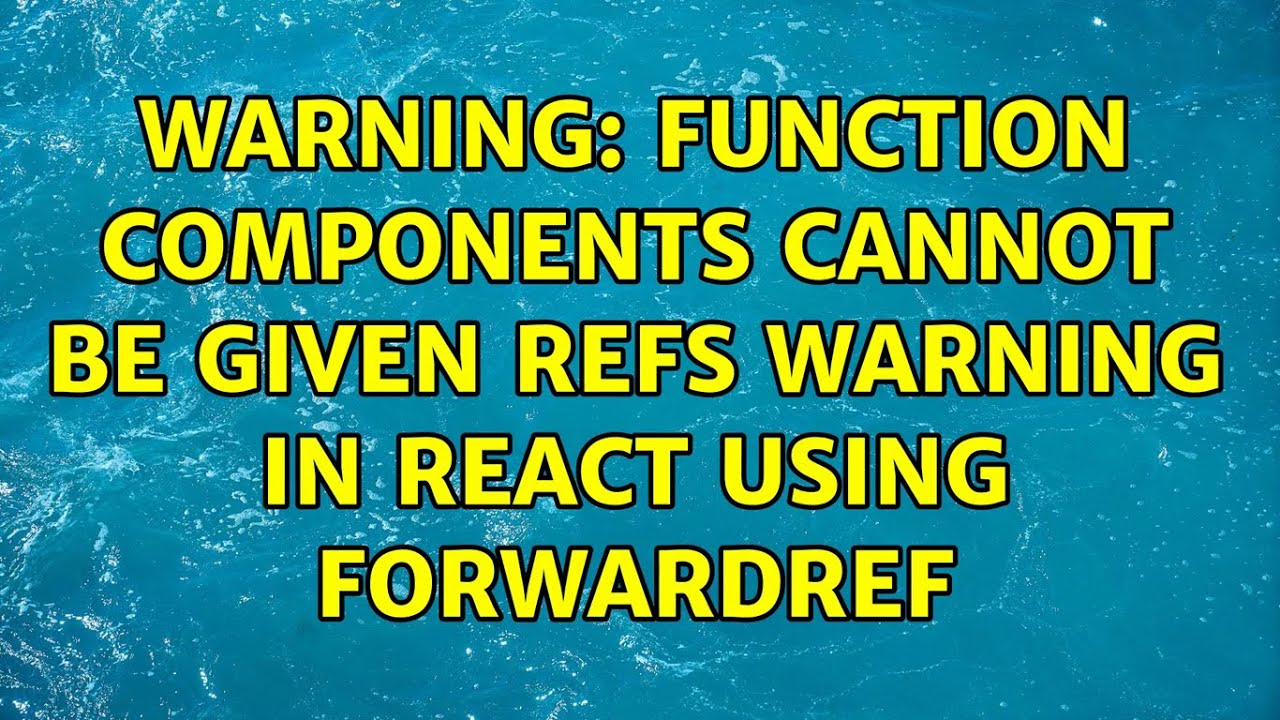



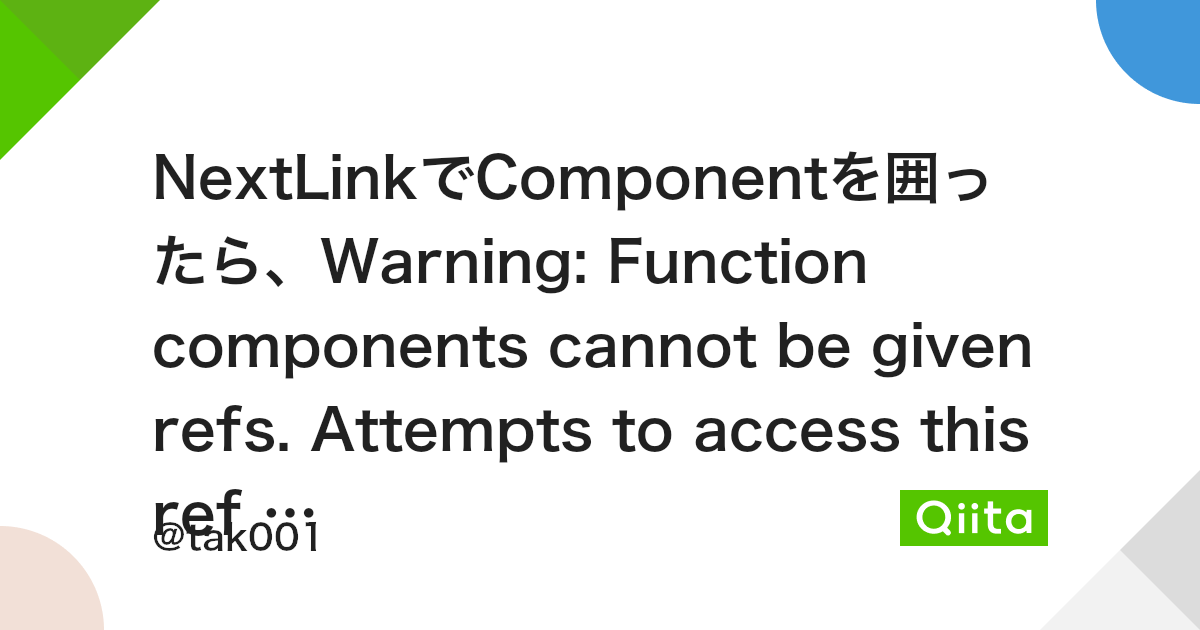
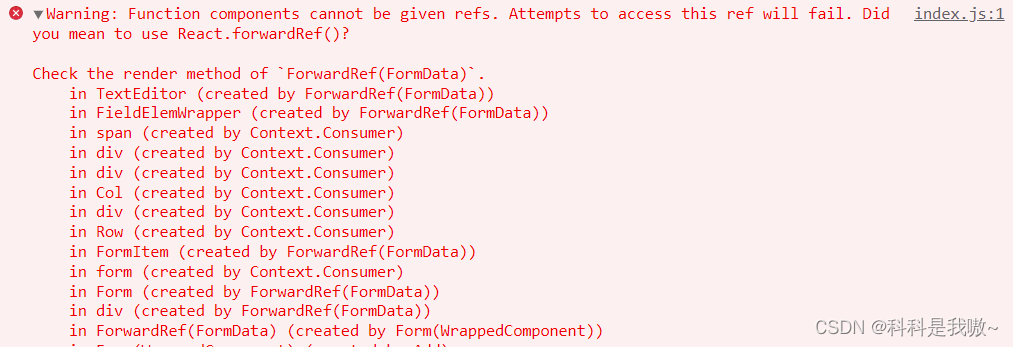
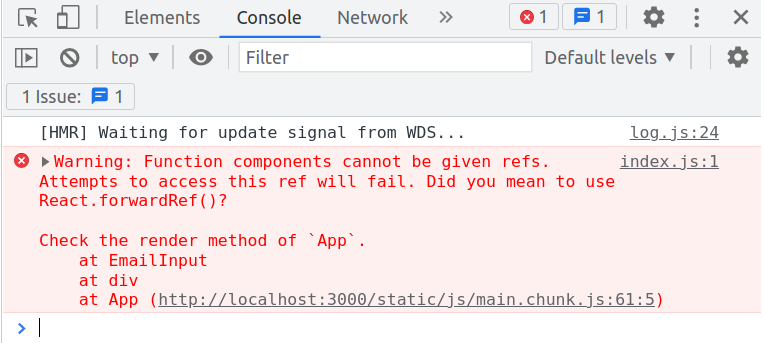
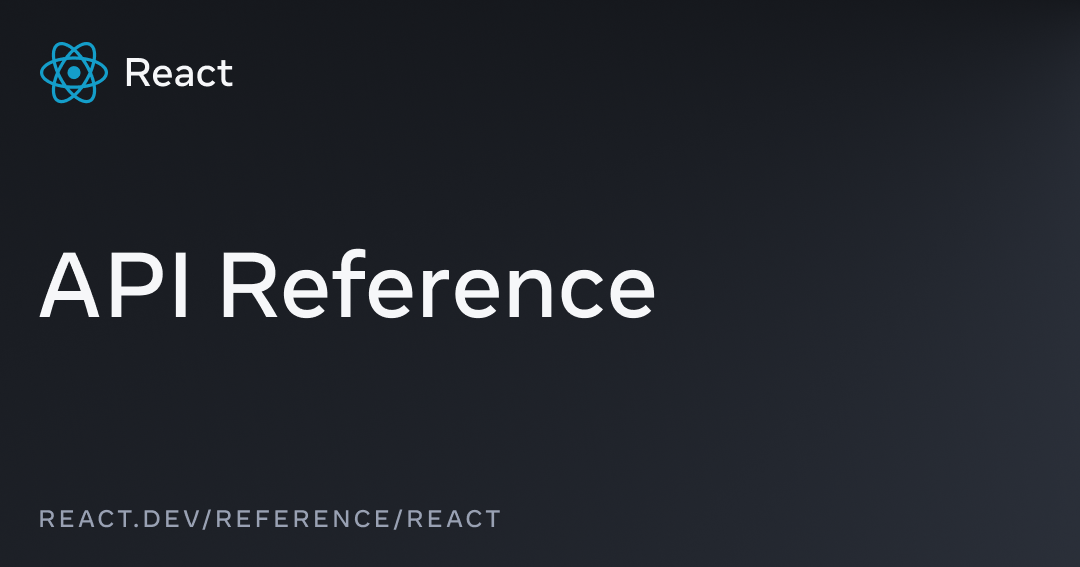
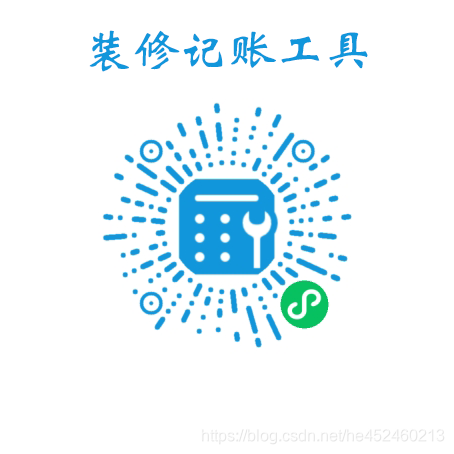
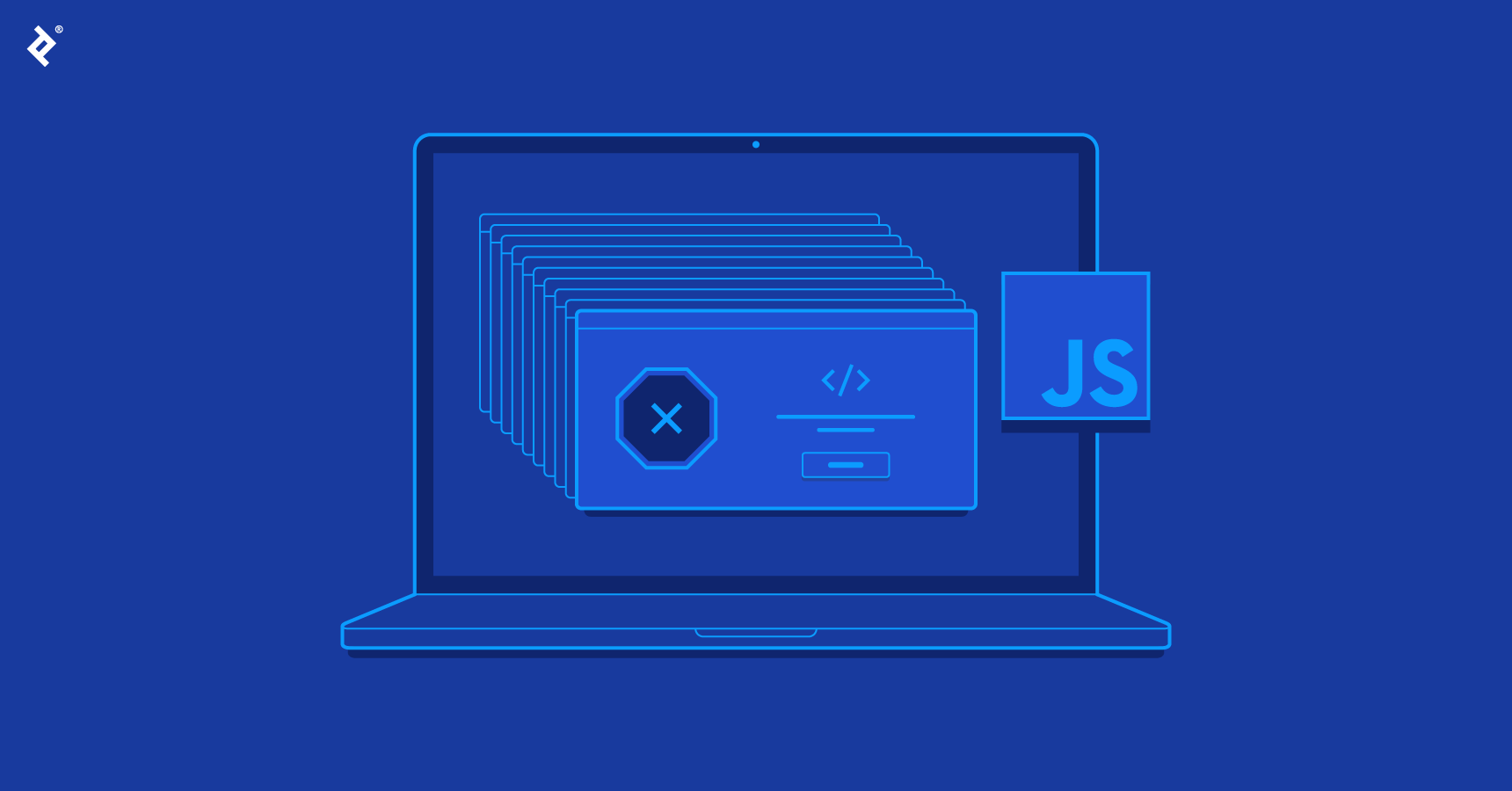
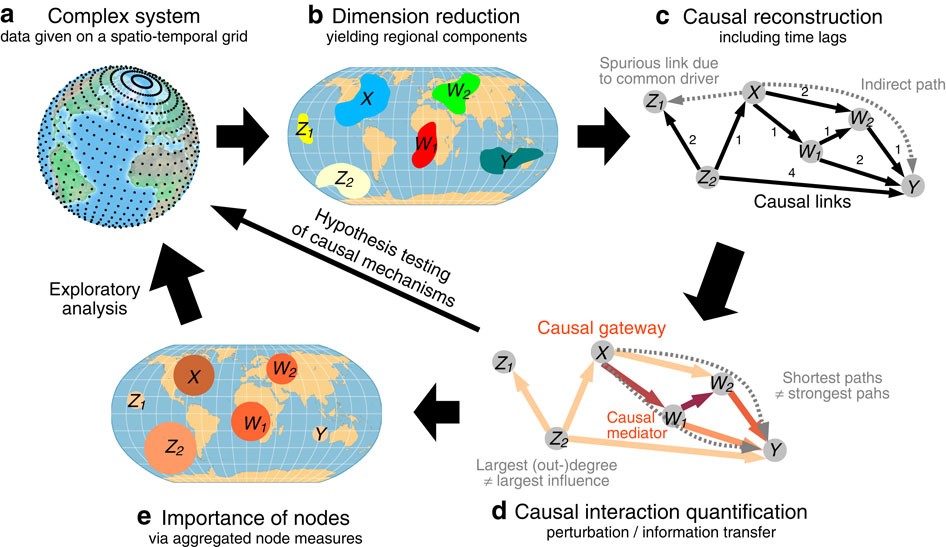
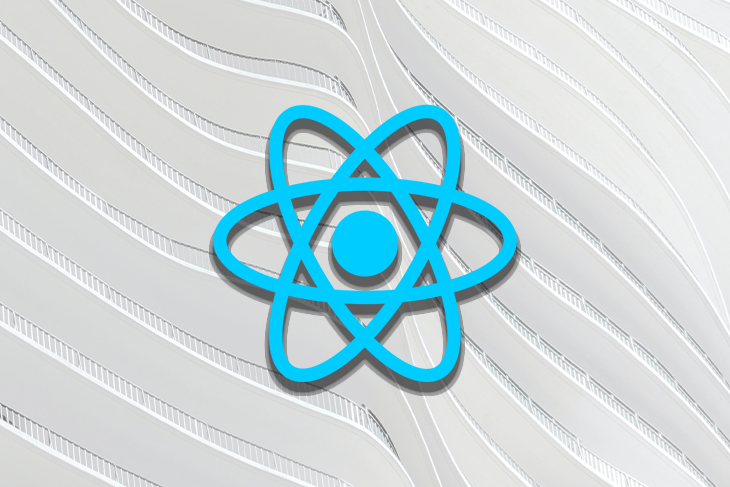

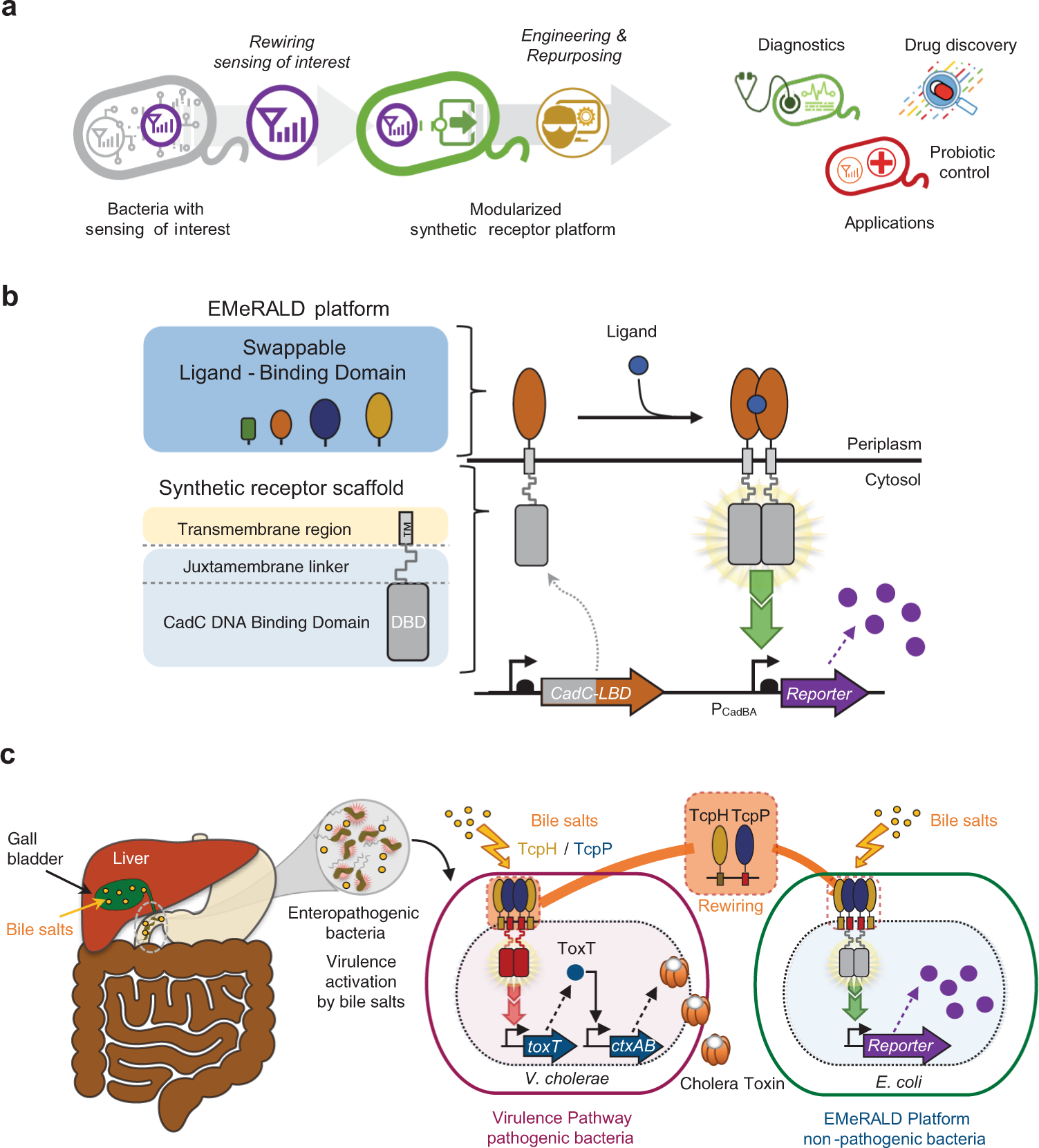

Article link: function components cannot be given refs.
Learn more about the topic function components cannot be given refs.
- How do I avoid ‘Function components cannot be given refs …
- Solved: Function components cannot be given refs … – GitHub
- Function components cannot be given refs … – bobbyhadz
- React forwardRef(): How to Pass Refs to Child Components
- ref prop should not be used on React function components
- UseRef, CreateRef, ForwardRef? What’s up with refs in React?
- Warning: Function components cannot be given refs-Reactjs
- Forwarding Refs – React
- function components cannot be given refs. attempts to access …
See more: nhanvietluanvan.com/luat-hoc