Fetch Is Not Defined
In the world of programming, working with APIs and making HTTP requests is a common task. To simplify this process, many developers rely on the fetch function, which is a built-in JavaScript method for making network requests. However, it’s not uncommon for developers to encounter an error message that says “fetch is not defined.” In this article, we will delve into the possible reasons for this error message and provide troubleshooting techniques to help you resolve it. We will also discuss best practices to prevent encountering this error in the future.
1. Defining the concept of “fetch” in programming
Before we delve into the reasons behind the “fetch is not defined” error, let’s first define the concept of “fetch” in programming. Fetch is a built-in JavaScript method that allows developers to make asynchronous network requests. It offers a simpler and more flexible alternative to traditional methods such as XMLHttpRequest. With fetch, you can easily send HTTP requests and receive responses from web servers.
2. Common reasons for encountering the error message “fetch is not defined”
The “fetch is not defined” error typically occurs when the fetch function is not recognized or accessible in the current scope. There are several common reasons for encountering this error:
a. Lack of appropriate import or initialization of the fetch function
To use the fetch function, you need to ensure that it is properly imported or initialized. If you’re using JavaScript modules, you can import fetch using the following code:
import fetch from ‘node-fetch’;
If you’re working with Node.js, you may need to install the ‘node-fetch’ package and require it in your code:
const fetch = require(‘node-fetch’);
b. Potential issues with dependencies or script loading order
Another common cause of the “fetch is not defined” error is related to dependencies or the order in which scripts are loaded. If you’re using a third-party library or framework that relies on fetch, make sure to include it before your script that uses fetch. In some cases, you may also need to ensure that the required dependencies are properly installed.
c. Compatibility concerns with older browsers or outdated JavaScript versions
Fetch is a relatively new addition to JavaScript and may not be supported in older browsers or outdated JavaScript versions. If you’re targeting a wide range of browsers, you may need to consider using a polyfill or alternative solution to ensure fetch compatibility. The ‘cross-fetch’ library is one such polyfill that can be used to overcome compatibility issues.
d. Errors caused by incorrect usage or misspelling of the fetch function
Misspelling or incorrectly using the fetch function can also lead to the “fetch is not defined” error. Double-check your code for any typographical errors or syntax mistakes. Additionally, make sure that you’re using the fetch function correctly, including providing the required parameters, such as the URL you’re making the request to.
3. Troubleshooting techniques to resolve the “fetch is not defined” error
If you encounter the “fetch is not defined” error, here are some troubleshooting techniques you can try:
a. Double-check the import or initialization
Ensure that the fetch function is correctly imported or initialized in your code. If you’re using JavaScript modules, verify that the import statement is correct, and the required dependencies are installed. If you’re working with Node.js, confirm that you have required the necessary ‘node-fetch’ package.
b. Check script loading order and dependencies
If you’re using external scripts or libraries that rely on fetch, ensure that they are included before the script that uses fetch. Additionally, verify that all the required dependencies are properly installed.
c. Consider using a polyfill or alternative solution
If compatibility with older browsers is a concern, consider using a polyfill like ‘cross-fetch’ to ensure fetch support. Research and explore alternative solutions that provide similar functionality to fetch, but with broader compatibility.
d. Verify correct and accurate usage of fetch
Carefully review your code for any typos or syntax errors. Ensure that you’re using the fetch function correctly and providing the necessary parameters, such as the URL and any additional options or headers required.
4. Best practices to prevent encountering the “fetch is not defined” error in the future
To avoid running into the “fetch is not defined” error in your future projects, consider implementing the following best practices:
a. Always import or initialize fetch correctly
Pay close attention to the correct import or initialization of the fetch function, based on your project requirements and environment.
b. Organize script loading order and dependencies diligently
Be mindful of the order in which your scripts are loaded and ensure that any dependencies are properly included and installed.
c. Stay updated with JavaScript versions and browser compatibility
Keep yourself informed about the latest JavaScript versions and their compatibility with different browsers. Regularly update your code and dependencies to ensure optimal compatibility.
d. Follow best practices for correct usage of fetch
Take the time to understand and follow best practices for using the fetch function. Refer to official documentation and examples to ensure accurate and effective usage.
In conclusion, encountering the “fetch is not defined” error can be frustrating, but it is often caused by common issues that have straightforward solutions. By understanding the potential reasons behind this error and employing the troubleshooting techniques outlined in this article, you’ll be able to resolve the issue and use fetch effectively in your projects. Remember to follow the best practices discussed to prevent encountering this error in the future. Happy coding!
FAQs:
Q1. What is node-fetch, and how is it related to the “fetch is not defined” error?
Node-fetch is a popular package that brings the fetch API to Node.js. If you’re using Node.js and encounter the “fetch is not defined” error, you may need to install and require the node-fetch package in your code to make fetch accessible in your project.
Q2. I’m encountering the “Fetch is not a function” error. How is it related to the “fetch is not defined” error?
The “Fetch is not a function” error is closely related to the “fetch is not defined” error. This error typically occurs when a variable or object named ‘fetch’ is present, but it is not a function. Double-check your code to ensure that there are no conflicting variables or objects named ‘fetch’ that might be causing this error.
Q3. Can the “fetch is not defined” error be specific to certain JavaScript frameworks like React?
While the “fetch is not defined” error is not specific to any particular JavaScript framework, it can occur when using frameworks like React. Ensure that you are correctly importing or initializing fetch in your React components, and consider using a polyfill like ‘isomorphic-fetch’ or ‘cross-fetch’ for broader compatibility if necessary.
Q4. I’ve encountered the “fetch is not defined” error even though I’ve imported or initialized fetch correctly. What should I do?
If you’ve already verified that fetch is properly imported or initialized in your code, double-check for any other potential issues such as script loading order and proper dependency installation. Additionally, consider restarting your local development server or clearing your browser cache, as sometimes caching issues can lead to unexpected errors.
How To Fix Referenceerror: Fetch Is Not Defined
Why Fetch Is Not Defined In Javascript?
JavaScript is a versatile programming language and is widely used in front-end web development. It provides numerous built-in methods and functions that developers utilize to interact with web servers and manipulate data. One commonly used function for making asynchronous network requests is the fetch() function. However, it is crucial to understand that fetch() is not inherently defined in JavaScript but rather in the Web API provided by web browsers. This article will delve into the reasons why fetch() is not a core feature of JavaScript and how it functions within the browser environment.
1. Understanding the Web API:
The Web API is a collection of methods and interfaces provided by web browsers to enhance the functionality of JavaScript. It allows developers to interact with browser features beyond the scope of traditional JavaScript. Fetch() is one such method provided by the Browser API. It can be used to make HTTP requests and receive responses, enabling the retrieval and manipulation of data from external sources.
2. Asynchronous Operations:
JavaScript has always been a single-threaded programming language. It executes code one instruction at a time, typically top to bottom. However, performing synchronous operations, such as making network requests, could block the execution of other code until a response is received. This can cause the user interface to freeze, leading to a poor user experience. As a result, JavaScript introduced the concept of asynchronous operations to allow non-blocking network requests, ultimately leading to more responsive web applications.
3. XMLHttpRequest vs. fetch():
Before fetch() was introduced, the primary method for making asynchronous requests in JavaScript was XMLHttpRequest (XHR). XHR is an older API that has been around since the advent of Ajax. While XMLHttpRequest is still widely used, fetch() offers a more modern and flexible alternative. The fetch() function returns a Promise that resolves to a response, simplifying the handling of asynchronous operations and providing a cleaner syntax.
4. Cross-Origin Resource Sharing (CORS):
CORS is a security mechanism implemented by web browsers to restrict cross-origin requests. It prevents a web page from making requests to a different domain, thus protecting users’ privacy and security. Fetch() automatically handles CORS by adhering to the protocol defined by the browser environment. This eliminates the need for developers to handle the intricacies of CORS manually while making cross-origin requests.
5. Polyfills and Browser Support:
Due to fetch() being a part of the Web API, its availability varies across different browsers and versions. Some legacy browsers may not support fetch() out of the box. However, there are polyfills available, which are JavaScript libraries that emulate the functionality of newer features in older environments. These polyfills can be easily added to ensure fetch() compatibility across a wide range of browsers.
6. FAQs:
Q: Is the fetch() function only used for retrieving data from servers?
A: No, fetch() is not limited to only retrieving data. It can also be used to send data to servers or perform other types of data manipulation.
Q: Can fetch() handle requests to different protocols, such as HTTPS or FTP?
A: Yes, fetch() can handle requests to various protocols, including HTTP, HTTPS, and FTP. This makes it a versatile method for interacting with different types of resources.
Q: Are there any alternatives to fetch() in JavaScript?
A: Yes, apart from fetch(), other HTTP request libraries exist, such as Axios and Superagent. These libraries offer additional features and may have wider browser support compared to fetch().
Q: Can fetch() be used in server-side JavaScript (Node.js)?
A: By default, fetch() is not available in server-side JavaScript environments like Node.js. However, there are packages like node-fetch that provide a similar API for making HTTP requests in Node.js.
In conclusion, fetch() is not inherently defined in JavaScript itself but is part of the Web API provided by web browsers. It offers a modern and versatile way to make asynchronous network requests. Understanding that fetch() is browser-dependent is crucial for handling compatibility issues and utilizing polyfills when necessary. By embracing this web API, developers can elegantly fetch data from remote servers, manipulate it, and create more interactive and responsive web applications.
How To Import Fetch In Typescript?
TypeScript is a strongly typed superset of JavaScript that compiles to plain JavaScript. With its added features and static typing, TypeScript has gained popularity among developers. One of the most common operations in web development is making HTTP requests to fetch data from a server. In JavaScript, the Fetch API is commonly used for this purpose. However, when using TypeScript, there are a few steps you need to follow to import and use Fetch properly. In this article, we will delve into how to import Fetch in TypeScript and go into the details of its usage.
Importing Fetch in TypeScript
To use Fetch in TypeScript, you need to first install the TypeScript definition file for Fetch. This file contains type information that allows the TypeScript compiler to understand and type-check the Fetch API.
To install the Fetch definition file, open your terminal and run the following command:
“`
npm install –save-dev @types/fetch
“`
Once installation is complete, you can start importing Fetch in your TypeScript files.
Import Fetch using the ES6 module syntax:
“`typescript
import fetch from ‘node-fetch’;
“`
If you are using a module bundler like Webpack or Rollup, you can use the default import syntax as shown above. This syntax imports the Fetch implementation from the node-fetch package.
If you are not using a module bundler and running your TypeScript code in a browser environment, you can import Fetch using the following syntax:
“`typescript
import fetch from ‘fetch’;
“`
The ‘fetch’ package provides an implementation of the Fetch API that can be used in browsers.
Using Fetch in TypeScript
After importing Fetch, you can start using it to make HTTP requests in your TypeScript code.
Here’s an example of making a GET request using Fetch in TypeScript:
“`typescript
fetch(‘https://api.example.com/data’)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.log(error));
“`
In the example above, we use the Fetch function to make a GET request to ‘https://api.example.com/data’. The Fetch function returns a Promise that resolves to the server’s response. We can then use the `json()` method on the response to parse the response data. Finally, we handle any possible errors using the `catch()` method.
FAQs
Q: What is Fetch in JavaScript?
A: Fetch is a browser API introduced in ES6 that provides an easy way to make HTTP requests and handle responses.
Q: Why do we need to import Fetch in TypeScript?
A: TypeScript requires type information to properly type-check and compile your code. By importing Fetch, you are telling TypeScript that you want to use the Fetch API and want the compiler to understand and check the types.
Q: What is the difference between using Fetch in JavaScript and TypeScript?
A: The main difference is that TypeScript requires a definition file for Fetch to provide type information. This helps TypeScript to perform static type checks, giving you better tooling support and reducing possible errors.
Q: Can I use Fetch in both Node.js and web browsers?
A: Yes, Fetch can be used in both environments. In Node.js, you need to use the ‘node-fetch’ package, while in browsers, you can use the ‘fetch’ package.
Q: Are there any alternatives to Fetch in TypeScript?
A: Yes, there are alternative libraries like Axios and Superagent that provide more features and various options for making HTTP requests. However, Fetch is widely supported in modern browsers and provides a simple and straightforward API for most use cases.
Conclusion
Importing Fetch in TypeScript is simple, and it enables you to make HTTP requests and handle responses with ease. By following the steps outlined in this article, you can include Fetch in your TypeScript project and benefit from the enhanced type-checking and tooling support provided by TypeScript. Remember to install the Fetch definition file and import the appropriate Fetch implementation based on your environment. Happy coding!
Keywords searched by users: fetch is not defined node-fetch, Fetch is not defined nodejs, Fetch is not a function javascript, Require fetch, Import fetch javascript, React-fetch, Cross fetch, Fetch JS
Categories: Top 10 Fetch Is Not Defined
See more here: nhanvietluanvan.com
Node-Fetch
One of the key advantages of node-fetch is its simplicity and ease of use. As a module, it follows the same API design principles as the Fetch API, which is widely used in web browsers. This means that if you are already familiar with the Fetch API in the browser, you’ll find node-fetch easy to pick up.
To start using node-fetch, you need to install it as a dependency in your Node.js project. You can do this by running the following command:
“`
npm install node-fetch
“`
Once installed, you can require the module in your code as follows:
“`javascript
const fetch = require(‘node-fetch’);
“`
With node-fetch, making HTTP requests is as simple as calling the fetch function and passing the URL of the resource you want to fetch. The function returns a Promise that resolves to the response from the server.
Here’s an example of how to make a GET request to an API and log the response:
“`javascript
fetch(‘https://api.example.com/data’)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
“`
In addition to making GET requests, node-fetch supports other HTTP methods like POST, PUT, DELETE, etc. You can specify the method by passing an options object as the second argument to the fetch function. Let’s see an example of making a POST request:
“`javascript
const postData = {
name: ‘John Doe’,
email: ‘[email protected]’
};
fetch(‘https://api.example.com/create’, {
method: ‘POST’,
headers: { ‘Content-Type’: ‘application/json’ },
body: JSON.stringify(postData)
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
“`
Node-fetch also allows you to set headers, handle cookies, and handle redirects. It provides a lot of flexibility and options for customizing your requests.
Now let’s move on to some frequently asked questions about node-fetch:
**Q: Is node-fetch only for Node.js? Can I use it in the browser?**
A: Node-fetch is primarily designed for use in Node.js. It relies on Node.js modules like ‘http’ and ‘https’ to make requests. However, you can use it in the browser by using tools like Browserify or Webpack to bundle the code along with a set of polyfills.
**Q: Is node-fetch compatible with promises?**
A: Yes, node-fetch is compatible with promises. The fetch function returns a promise that resolves to the response from the server. You can then use the `.then` and `.catch` methods to handle the response or any errors that occur.
**Q: Can I use node-fetch with async/await?**
A: Yes, node-fetch works perfectly fine with async/await syntax. You can use the `await` keyword to wait for the response from the server. Here’s an example:
“`javascript
async function fetchData() {
try {
const response = await fetch(‘https://api.example.com/data’);
const data = await response.json();
console.log(data);
} catch (error) {
console.error(error);
}
}
“`
**Q: Does node-fetch support streaming?**
A: Yes, node-fetch supports streaming. The fetch function returns a readable stream, which you can consume using the `stream.pipe()` method or by iterating over the chunks using the `stream.read()` method.
**Q: Can I cancel a fetch request made with node-fetch?**
A: No, node-fetch does not support request cancellation out of the box. However, you can use third-party libraries like `abort-controller` to achieve this functionality.
In conclusion, node-fetch is a powerful and convenient library for making HTTP requests from Node.js. It simplifies the process of interacting with APIs and fetching resources, providing a modern and intuitive API. Whether you are a beginner or an experienced Node.js developer, node-fetch is definitely worth considering for your projects.
Fetch Is Not Defined Nodejs
Node.js is a powerful and widely-used JavaScript runtime environment that allows developers to build scalable and efficient server-side applications. One of the key features of Node.js is its ability to perform asynchronous operations effectively. However, there are times when developers encounter the error message “fetch is not defined” while working with Node.js. In this article, we will delve deeper into this issue, its causes, and explore potential solutions to overcome it.
Understanding the Error Message
When you encounter the “fetch is not defined” error message while working with Node.js, it typically means that Node.js is unable to recognize the ‘fetch’ function that is used to make HTTP requests. This error is commonly encountered when developers use the ‘fetch’ function in their code without importing it explicitly or without using the appropriate third-party libraries to support it.
Causes of the Issue
There are a few potential causes for the “fetch is not defined” error:
1. Missing ‘fetch’ Function: Node.js does not provide a native implementation of the ‘fetch’ function like modern browsers do. Hence, without explicitly importing and using a library that provides this functionality, the ‘fetch’ function will be undefined, resulting in the error.
2. Different JavaScript Environments: It is important to note that while ‘fetch’ is available in the browser’s JavaScript environment, it is not automatically available in Node.js. The ‘fetch’ function is part of the Window interface, which does not exist in Node.js. Therefore, to use ‘fetch’ in Node.js, you must either polyfill the function or use a third-party library that provides the necessary functionalities.
Solutions to the Issue
To resolve the “fetch is not defined” error in Node.js, you can consider the following solutions:
1. Using a Third-Party Library: Many popular third-party libraries provide a polyfill or wrapper around the ‘fetch’ function, allowing developers to use it in Node.js. One such library is ‘node-fetch,’ which supports the ‘fetch’ API in Node.js by providing a compatible implementation. By installing and importing ‘node-fetch’ in your Node.js project, you can successfully use the ‘fetch’ function.
2. Polyfilling the ‘fetch’ Function: If you prefer not to rely on third-party libraries, you can use a polyfill to add the ‘fetch’ function to the global scope in Node.js. A popular polyfill is ‘isomorphic-fetch,’ which can be installed via npm. By importing ‘isomorphic-fetch’ in your Node.js code, it will add support for the ‘fetch’ function.
3. Using a Fetch Wrapper: Another approach to solve the “fetch is not defined” issue is to use a fetch wrapper library. These libraries generally provide additional functionalities and make the API easier to work with. Some popular fetch wrapper libraries include ‘axios’ and ‘got.’ By using these libraries, you can avoid the “fetch is not defined” error altogether.
FAQs:
Q1. Why does Node.js not have a built-in ‘fetch’ function?
A1. Node.js was primarily designed for server-side JavaScript execution and does not include browser-specific features. The ‘fetch’ function is one of those browser-specific features that are not essential for server-side JavaScript execution in Node.js.
Q2. Why is the ‘fetch’ function necessary in Node.js?
A2. The ‘fetch’ function in Node.js allows developers to make HTTP requests to external APIs, fetch data from other servers, and perform various asynchronous operations, just like in the browser environment. Its absence can limit the capabilities of Node.js applications that require such functionalities.
Q3. Are there any alternative methods to ‘fetch’ in Node.js?
A3. Yes, besides the ‘fetch’ function, various other libraries provide similar functionalities to make HTTP requests in Node.js. Some popular alternatives include ‘axios’ and ‘got,’ which offer additional features and a more user-friendly API.
In conclusion, encountering the “fetch is not defined” error in Node.js can be frustrating, especially when working on projects that require making HTTP requests. However, by understanding the causes and applying suitable solutions, such as using a third-party library like ‘node-fetch’ or polyfilling the ‘fetch’ function with ‘isomorphic-fetch,’ developers can overcome this issue and utilize the power of the ‘fetch’ function in their Node.js applications.
Images related to the topic fetch is not defined
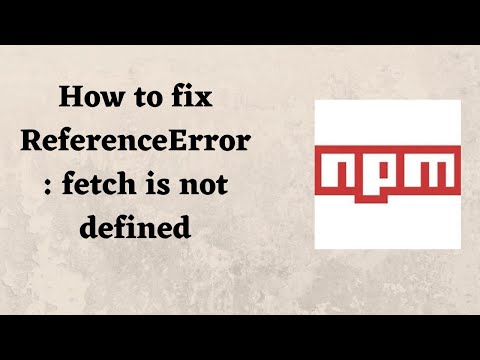
Found 37 images related to fetch is not defined theme
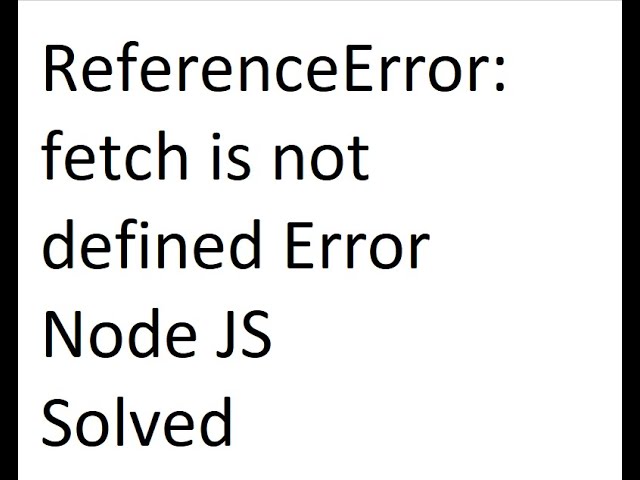
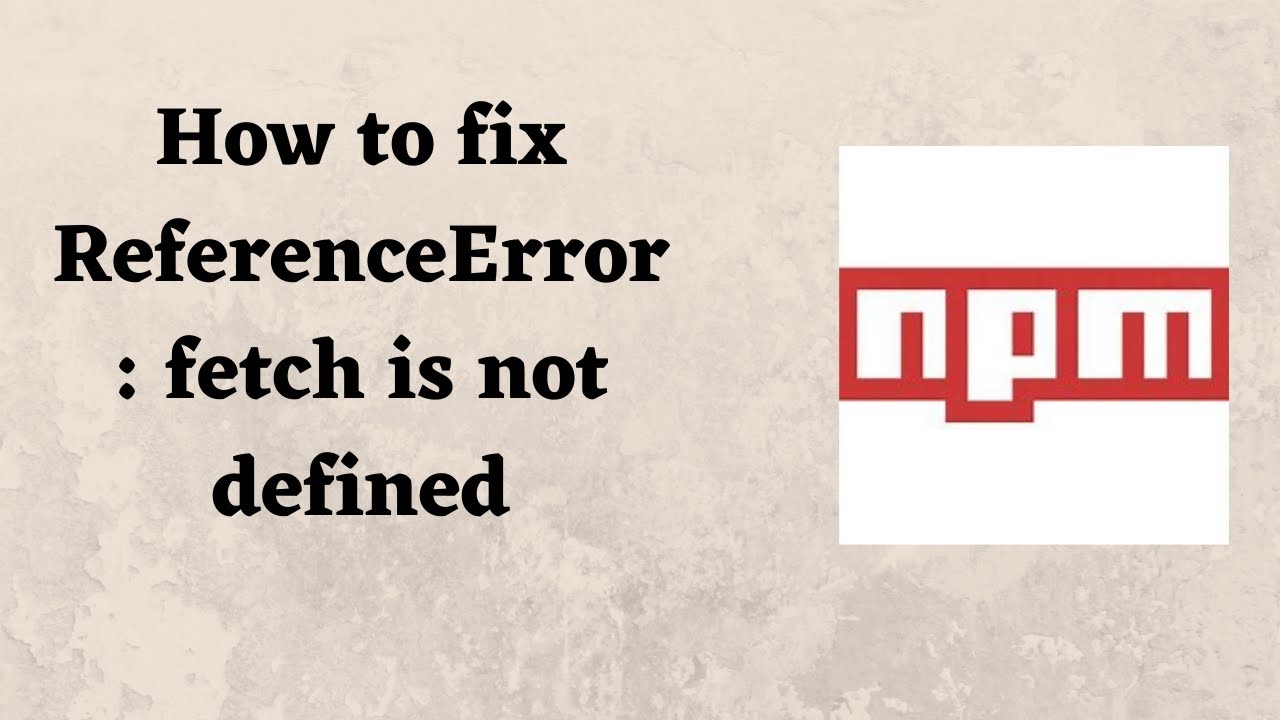

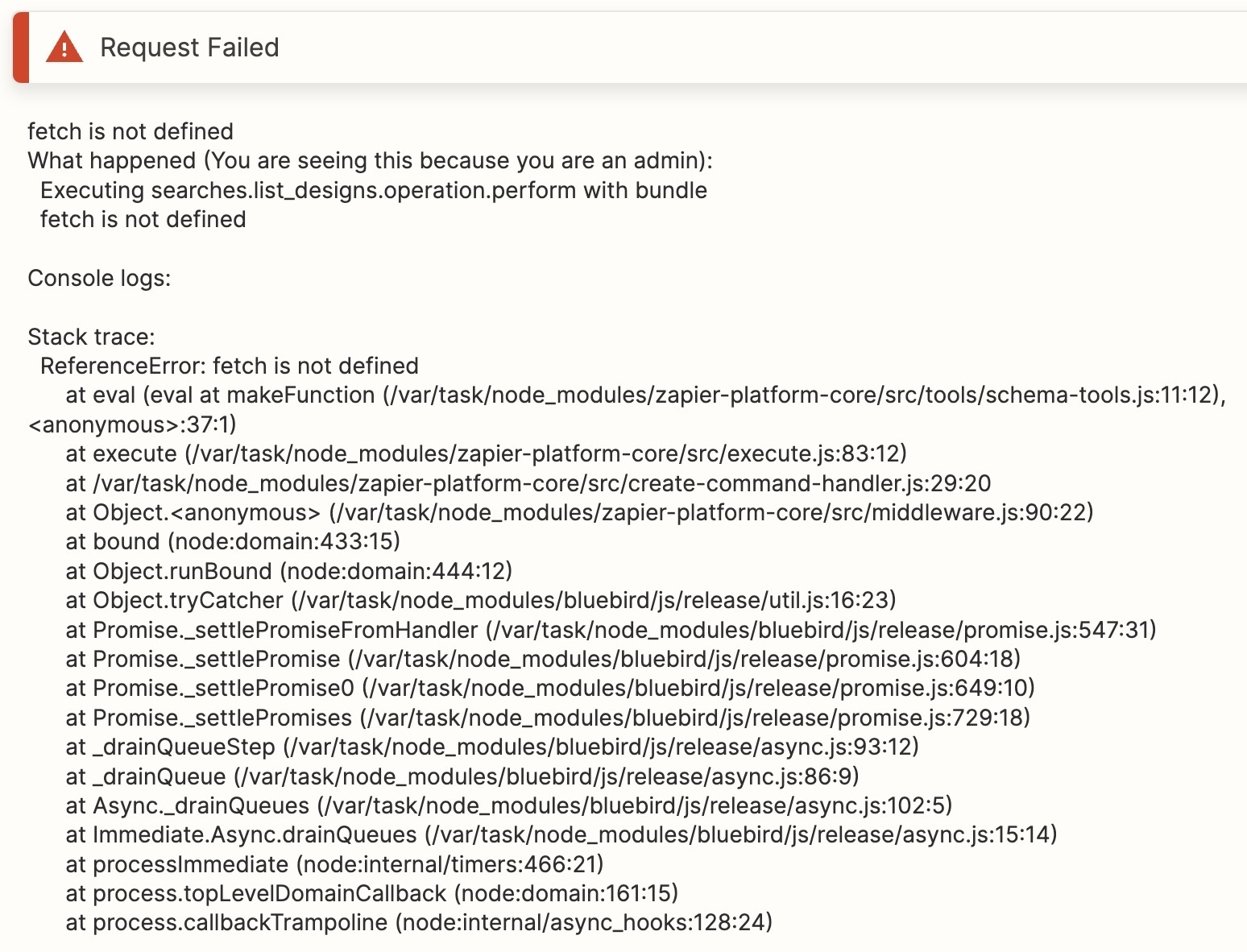

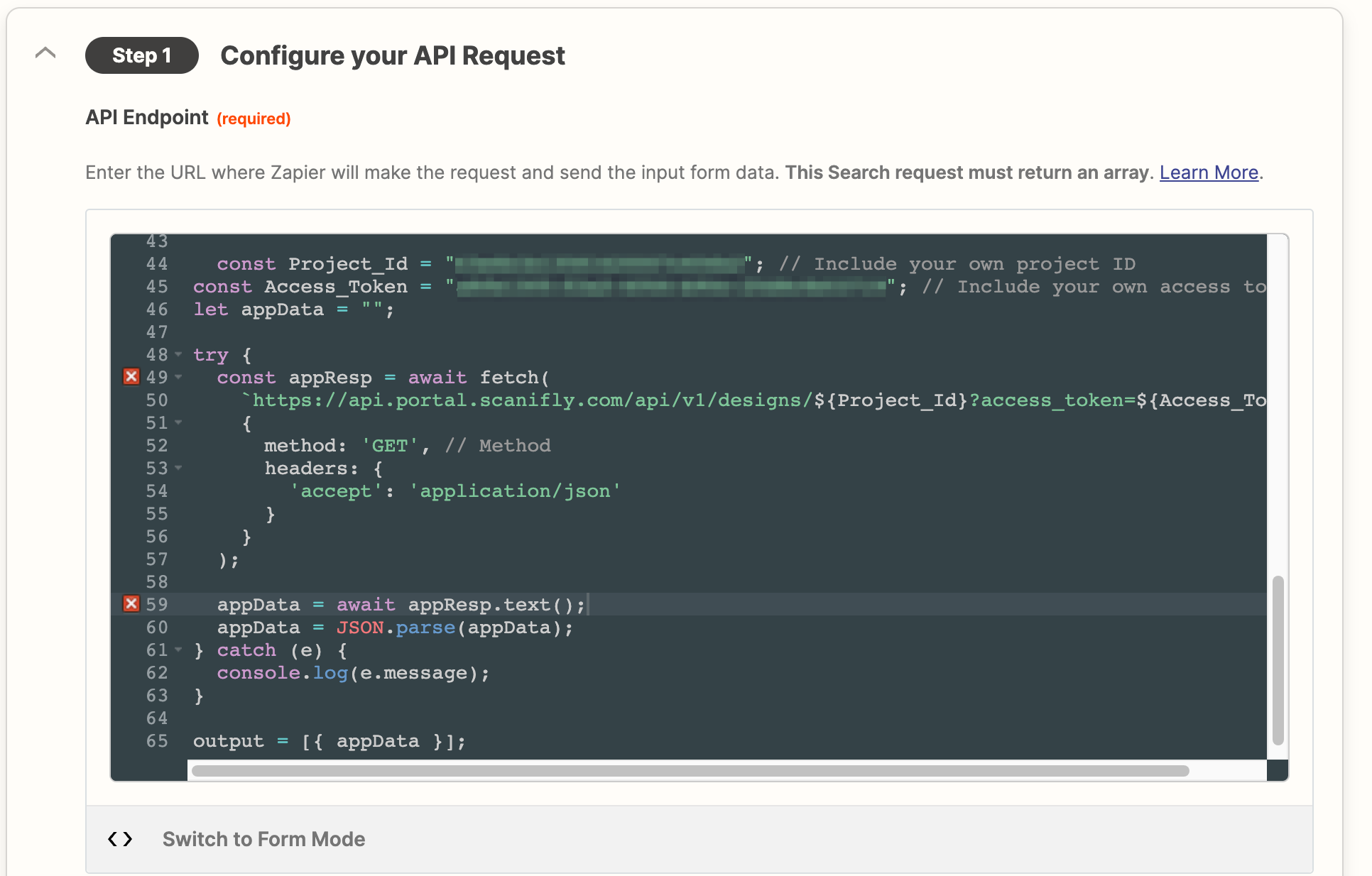
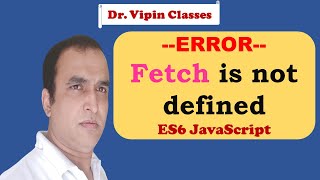
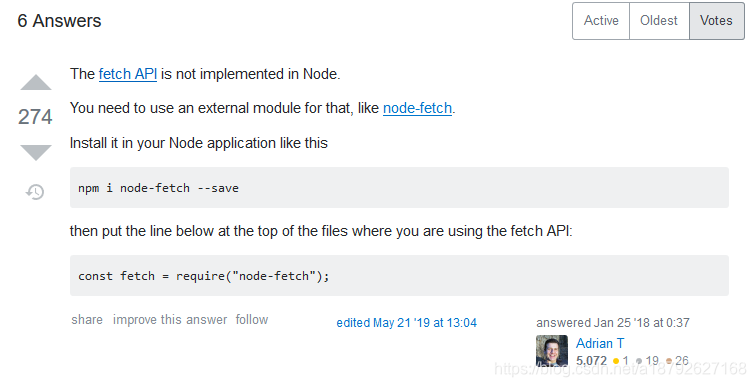



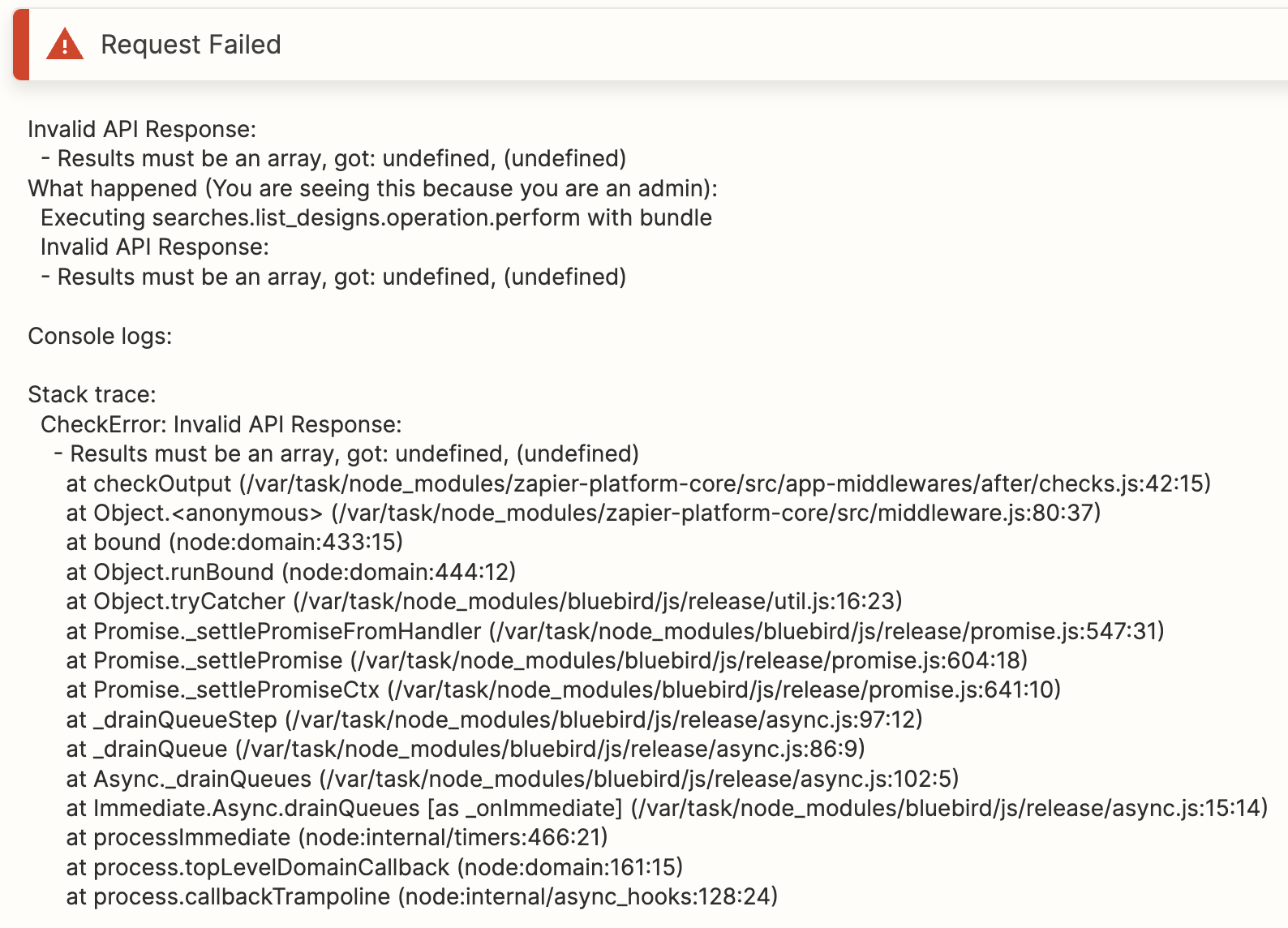

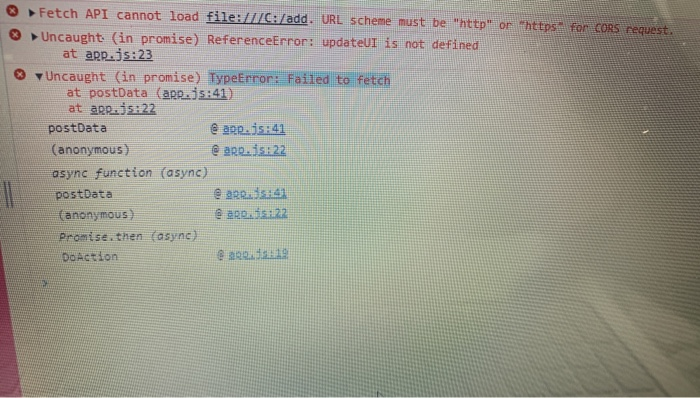
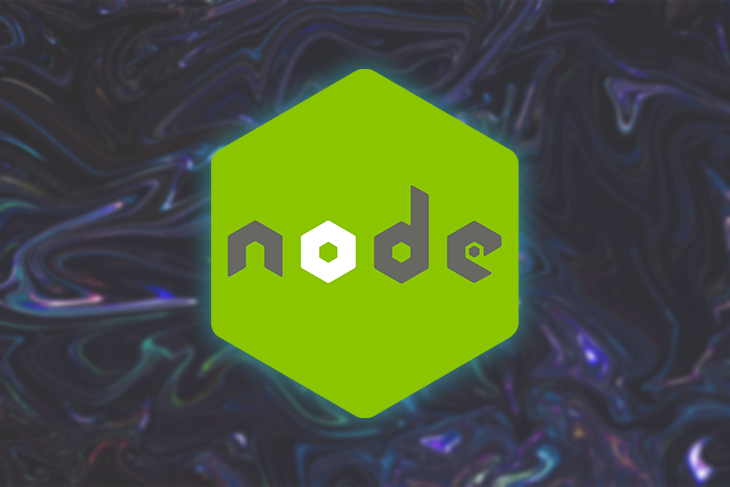
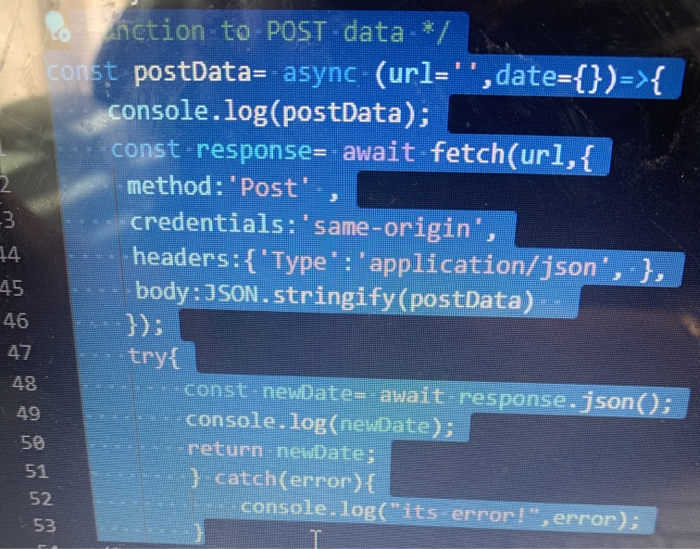
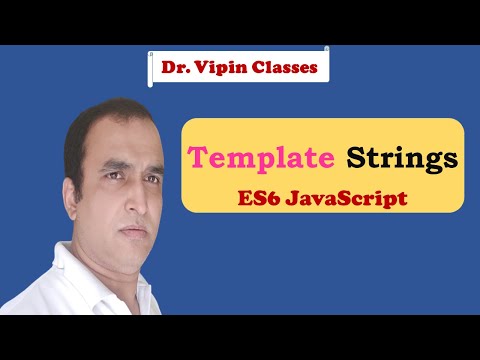
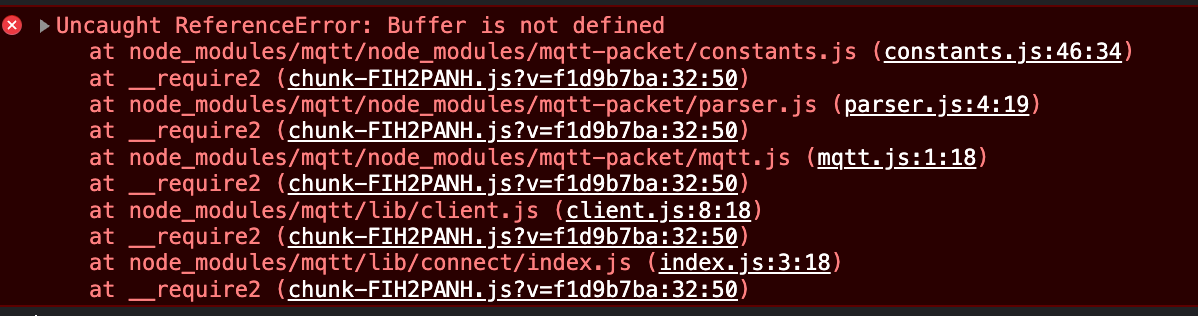
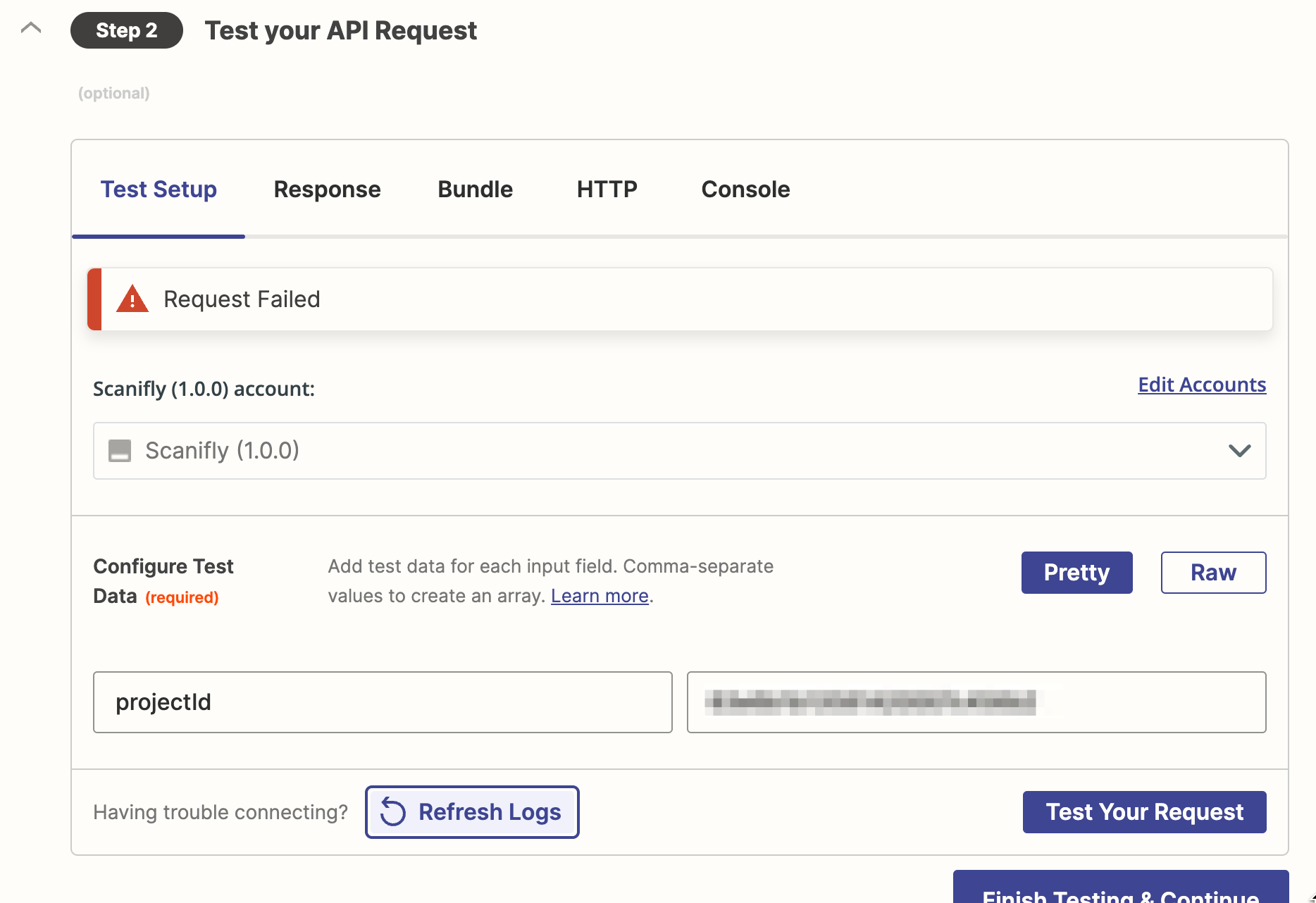



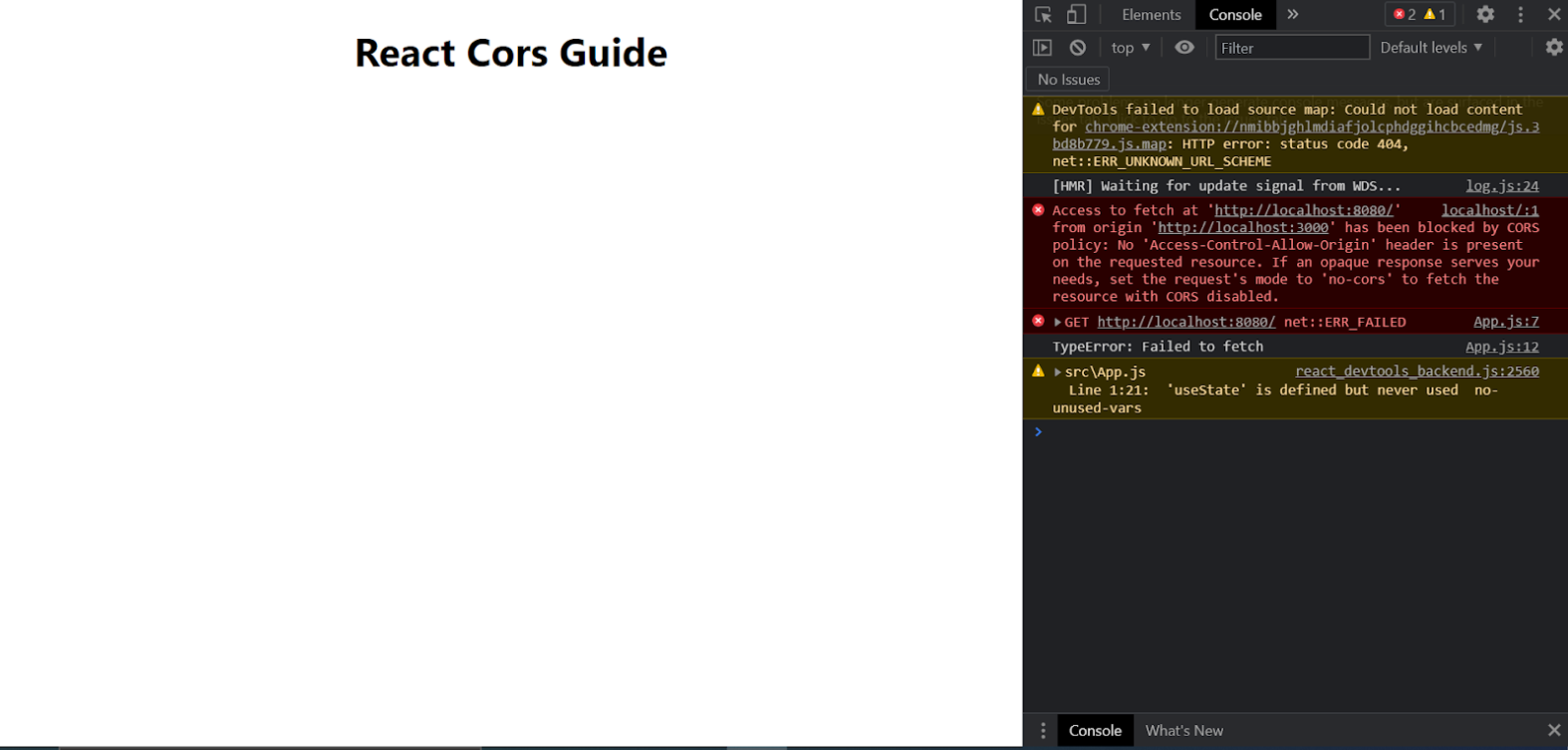
Article link: fetch is not defined.
Learn more about the topic fetch is not defined.
- ReferenceError: fetch is not defined – Stack Overflow
- ReferenceError: fetch is not defined in NodeJs – bobbyhadz
- ReferenceError: fetch is not defined in NodeJs – bobbyhadz
- How to use node-fetch in TypeScript? – Rapid API
- How To Fix ReferenceError: fetch is not defined – Codedamn
- Troubleshooting | Upstash: Documentation
- How to fix ‘ReferenceError: fetch is not defined’ in Node.js
- Fetch Is Not Defined: How To Fix The Referenceerror
- ReferenceError: fetch is not defined Error in Node.js [Solved]
- ReferenceError: fetch is not defined
- How To Fix ReferenceError: fetch is not defined – Codedamn
- ReferenceError: fetch is not defined – Zapier Community
- How to Fix the ‘fetch is not defined’ error in Node.js? – Lightly IDE
See more: https://nhanvietluanvan.com/luat-hoc