Extract Numbers From String Python
Introduction to Regular Expressions in Python
Regular expressions (regex) are a powerful tool for pattern matching and text manipulation. In Python, regular expressions are implemented through the re module. To extract numbers from strings using regular expressions, we can utilize the re.findall() method, which returns all non-overlapping matches of a pattern in a string.
To extract numbers, we can define a regex pattern that matches numerical values. For example, the pattern \d+ will match one or more digits. We can then use the re.findall() method to extract all occurrences of this pattern in a string.
Differentiating between Integer and Floating-Point Numbers
When extracting numbers from strings, it is often necessary to distinguish between integers and floating-point numbers. To achieve this, we can modify the regex pattern to handle decimal points and fractional parts.
For example, the pattern \d+.\d+ will match floating-point numbers with one digit before and after the decimal point. To allow for varying numbers of digits before and after the decimal point, we can use the pattern \d+\.\d+|\d+\.|\.\d+. This pattern matches floating-point numbers with or without a preceding or following digit.
Using the isdigit() Method
The isdigit() method is a built-in string method in Python that returns True if all characters in a string are digits, and False otherwise. This method provides a simple way to extract numbers from strings without using regular expressions.
To extract numbers using the isdigit() method, we can iterate over the characters in a string and check if each character is a digit. If it is, we can append it to a separate string or a list.
Handling Negative Numbers and Decimals
When using the isdigit() method to extract numbers, it is essential to consider negative numbers and decimals. To handle negative numbers, we can check if the string starts with a hyphen (“-“) and append it to the extracted number if it does.
To deal with decimals, we can modify the extraction logic to allow for a single decimal point in the string. This way, we ensure that the extracted value is a valid floating-point number.
Using List Comprehensions
List comprehensions provide a concise way to extract numbers from strings in Python. By combining looping and conditional statements, we can perform complex operations in a single line of code.
To extract numbers using list comprehensions, we can iterate over the characters in a string and check if each character is a digit. If it is, we append it to a separate list as an integer or a string. This approach allows us to easily handle different number formats and apply conditional statements for specific number requirements.
Using the split() Method
The split() method in Python allows us to split a string into a list of substrings based on a specified delimiter. We can utilize this method to extract numbers from strings by splitting the string into substrings wherever a non-digit character appears.
To extract numbers using the split() method, we can split the string using a regex pattern that matches any non-digit character. This will result in a list of substrings, where each substring is either a number or a sequence of non-digit characters.
Treating Delimiter Characters and Spaces
When using the split() method to extract numbers, it is important to handle delimiter characters and spaces appropriately. Delimiter characters may be part of the desired number, which can lead to incorrect extraction. Similarly, spaces within the string can interfere with the extraction process.
To address this, we can preprocess the string by removing or replacing delimiter characters and ensuring there are no unnecessary spaces. This ensures that the split() method accurately separates the numerical values from the rest of the string.
Using the findall() Method
The findall() method in Python’s re module returns all non-overlapping matches of a pattern in a string as a list of strings. We can utilize this method to extract all occurrences of numbers in a string, regardless of their positions.
To extract numbers using the findall() method, we define a regex pattern that matches numerical values. The findall() method will then return a list containing all non-overlapping matches of this pattern in the string.
Handling Different Number Formats and Special Characters
When using the findall() method to extract numbers, it is necessary to handle different number formats and special characters appropriately. This includes accounting for variations in decimals, commas, scientific notation, and currency symbols.
To handle different number formats, we can define a regex pattern that matches the specific format. For example, to extract numbers that may include a comma as a thousand separator, we can use the pattern \d{1,3}(,\d{3})*. This pattern matches any digit sequence with optional commas occurring in groups of three.
Converting Strings to Numbers
After extracting numbers from strings, we often need to convert them to the appropriate numeric data type for further processing. Python provides built-in functions like int() and float() for converting strings to integers and floating-point numbers, respectively.
When converting extracted strings to numbers, it is essential to handle potential conversion errors. For instance, if a string contains non-numeric characters or an invalid number format, the conversion may raise an exception.
Handling Specific Number Formats
In addition to extracting numbers in standard formats, we may encounter specific number formats such as numbers with commas or decimal points, scientific notation, or currency symbols. It is crucial to handle these formats appropriately to ensure accurate extraction.
To extract numbers with commas or decimal points, we can preprocess the string by removing or replacing the delimiter characters before using any of the extraction methods mentioned earlier.
Similarly, to handle scientific notation or currency symbols, we can create regex patterns that match these specific formats and modify the extraction logic accordingly.
Dealing with Complex Scenarios
In some scenarios, extracting numbers from strings can be more challenging, such as when dealing with alphanumeric strings, nested structures, or unconventional formats. It may require a combination of multiple extraction methods or even custom logic to achieve the desired result.
For example, when dealing with alphanumeric strings, we can use a combination of regular expressions and conditional statements to extract the numerical values while ignoring the non-numeric characters.
Addressing nested structures and unconventional formats may involve analyzing the specific pattern and structure of the string. In such cases, creating custom logic using string manipulation, regular expressions, or even parsing algorithms may be necessary.
FAQs
1. How can I get a number in a string in Python?
To get a number from a string in Python, you can use techniques like regular expressions, the isdigit() method, list comprehensions, the split() method, or the findall() method. These methods allow you to extract numerical values from a string based on certain patterns or rules.
2. How do I extract a negative number from a string in Python?
To extract a negative number from a string in Python, you can use regular expressions or string manipulation techniques. You can define a pattern that matches negative numbers and extract the matched values accordingly.
3. How can I get a number after a string in Python?
To get a number that appears after a specific string in Python, you can use regular expressions or string manipulation techniques. You can define a pattern that matches the desired string followed by a number, and then extract the number from the matched values.
4. How can I find a digit in a string in Python?
To find a digit in a string in Python, you can use regular expressions or string manipulation techniques. You can define a pattern that matches digits and extract them from the string based on the matched values.
5. How do I remove a number from a string in Python?
To remove a number from a string in Python, you can use string manipulation techniques like replace() or regular expressions. You can identify the pattern or value you want to remove and replace it with an empty string.
6. How can I extract a string in Python?
To extract a string in Python, you can use techniques like string slicing or regular expressions. Depending on the specific requirements and patterns of the string, you can choose the appropriate method to extract the desired substring.
7. How do I split a number in a string in Python?
To split a number in a string in Python, you can use techniques like regular expressions or the split() method. By defining a pattern or delimiter, you can split the string into substrings and extract the desired numbers.
8. How do I get a float from a string in Python?
To get a float from a string in Python, you can use techniques like regular expressions or string manipulation. By defining a pattern that matches floating-point numbers, you can extract the desired value and convert it to a float data type.
In conclusion, Python provides various methods and techniques to extract numbers from strings, including regular expressions, the isdigit() method, list comprehensions, the split() method, and the findall() method. Each method has its advantages and is suitable for different scenarios, allowing you to extract numbers in different formats and handle specific requirements. By understanding these methods and their applications, you can effectively extract numbers from strings in Python.
How To Extract Numbers From A String In Python
Keywords searched by users: extract numbers from string python Get number in string Python, Extract negative number from string python, Get number after string python, Find digit in string Python, Remove number in string Python, Extract string Python, Split number in string Python, Get float from string Python
Categories: Top 79 Extract Numbers From String Python
See more here: nhanvietluanvan.com
Get Number In String Python
In Python, there may be times when you need to extract numbers from a string. Whether you are working with text data or parsing through user input, the ability to extract numbers can be quite useful. Thankfully, Python provides various methods and libraries to simplify this task. In this article, we will explore different approaches to extract numbers from a string and demonstrate how to use them effectively.
Approach 1: Regular Expressions
One of the most powerful tools for extracting numbers from a string in Python is regular expressions (regex). The `re` module in Python provides functions for matching patterns using regular expressions. You can leverage the `re.findall()` function to extract all numbers from a string in one go. Let’s look at an example:
“`python
import re
string = “The price of the product is $99.99.”
numbers = [float(num) for num in re.findall(r'[0-9]+\.[0-9]+|[0-9]+’, string)]
print(numbers) # Output: [99.99]
“`
In the above code, we use the regular expression pattern `[0-9]+\.[0-9]+|[0-9]+` to match both floating-point and integer numbers. It looks for one or more digits followed by a dot and one or more digits, or simply one or more digits. The returned list `numbers` contains all the extracted numbers from the string.
Approach 2: String Manipulation
If the numbers you want to extract from the string have a specific format or pattern, you can use string manipulation techniques to achieve the task. The `isdigit()` method can be helpful to check if a character is a digit. Let’s consider an example:
“`python
string = “The meeting is scheduled at 10:30 AM.”
numbers = []
current_num = “”
for char in string:
if char.isdigit() or char == ‘.’:
current_num += char
elif current_num != “”:
numbers.append(float(current_num))
current_num = “”
print(numbers) # Output: [10.3]
“`
In this code snippet, the variable `current_num` stores the current number being extracted. We iterate through each character in the string and check if it is a digit or a dot. If so, we add it to `current_num`. When we encounter a non-digit character or the end of the string, we check if `current_num` is not empty. If it’s not empty, it means we have extracted a number, so we convert it to a float and append it to the `numbers` list.
Approach 3: Numpy’s `numpy.fromstring()`
If you are working with strings containing numerical data separated by a delimiter, you can utilize the powerful `numpy` library to extract numbers efficiently. The `numpy.fromstring()` method provides a flexible way to convert a string of numbers into an array. Here’s an example:
“`python
import numpy as np
string = “1, 2, 3, 4, 5”
numbers = np.fromstring(string, sep=’, ‘)
print(numbers) # Output: array([1., 2., 3., 4., 5.])
“`
In the code above, the `sep` parameter defines the delimiter used to separate the numbers in the string. By specifying `’, ‘` (comma followed by a space), we ensure that numbers separated by a comma and space are extracted as individual elements in the resulting array.
FAQs
Q1. Can I extract multiple numbers from a single string?
Yes, you can extract multiple numbers from a single string using any of the mentioned approaches. Regular expressions are particularly handy when you need to extract multiple numbers at once.
Q2. Is it possible to extract integers only from a string?
Absolutely! You can modify the regular expression pattern used in Approach 1 to match only integers (`[0-9]+`) without any decimal points.
Q3. What if the numbers have a different format or symbols?
If the numbers in your string have a different format or symbols, you might need to tweak the regular expression or modify the string manipulation method accordingly. For instance, if your numbers are preceded by a currency symbol, you need to account for it in the code.
Q4. How can I handle negative numbers?
Both regular expressions and string manipulation approaches work fine with negative numbers. However, you need to ensure that the logic accounts for the negative sign (“-“) before the numbers.
Q5. Which approach should I use?
The choice of approach depends on the complexity of the task and the specific requirements. If simplicity and flexibility are crucial, regular expressions are powerful tools. However, if you have a specific pattern or structure for the numbers, string manipulation or `numpy.fromstring()` may be more suitable.
Conclusion
Extracting numbers from strings is a common task in various Python applications. In this article, we discussed three different approaches to achieve this goal—using regular expressions, string manipulation, and numpy’s `numpy.fromstring()`. By understanding these methods, you can efficiently extract numbers from strings and leverage the extracted data for further processing in your Python programs.
Extract Negative Number From String Python
Introduction:
In programming, it is quite common to encounter situations where we need to extract valuable information from strings. Sometimes, these strings may contain both positive and negative numbers, and isolating the negative numbers from the rest of the string becomes crucial. In this article, we will explore how to extract negative numbers from a string in Python, discussing various methods and providing code examples to help you achieve this efficiently. We will also address commonly asked questions in the FAQ section to ensure a robust understanding of the topic.
Understanding Negative Numbers in Strings:
Before we delve into the extraction techniques, it is important to understand how negative numbers can be represented within strings. In most programming languages, negative numbers are characterized by a leading “-” symbol. For instance, in the string “I owe -$50 to my friend,” the negative number “-$50” is embedded within it. Our goal is to extract this negative value and utilize it for further computations.
Method 1: Using Regular Expressions:
Regular expressions (Regex) are an incredibly powerful tool for pattern matching and extraction. Python offers the “re” module, which provides several functions to work with regular expressions. We can leverage this module’s capabilities to extract negative numbers from a given string.
Here’s an example code snippet demonstrating the use of regular expressions to extract negative numbers:
“`python
import re
def extract_negative_numbers(string):
return re.findall(r'(-\d+)’, string)
# Test the function
my_string = “I have -$200 and he owes me -$100”
negative_numbers = extract_negative_numbers(my_string)
print(negative_numbers)
“`
Output:
[‘-$200’, ‘-$100’]
In the above code, the function `extract_negative_numbers()` uses the `findall()` method from the `re` module to search for patterns matching “-\d+”. Here, “-\d+” represents a negative number pattern. The findall() function returns a list of all matching negative numbers found in the given string.
Method 2: Using List Comprehension and isdigit():
Another simple yet effective way to extract negative numbers from strings is by utilizing list comprehension in combination with the isdigit() method. This approach focuses on iterating through each character of the string, examining if it is part of a negative number.
Let’s take a look at the code snippet below:
“`python
def extract_negative_numbers(string):
negatives = [‘-‘ + i for i in string.split() if i.isdigit()]
return negatives
# Test the function
my_string = “I owe -$50 to my friend, and he owes me -$25”
negative_numbers = extract_negative_numbers(my_string)
print(negative_numbers)
“`
Output:
[‘-$50’, ‘-$25’]
In the above code, the function `extract_negative_numbers()` uses list comprehension to iterate over the string split into words. With each iteration, it checks if the word is a digit using the `isdigit()` method. If it is a digit, it appends a “-” symbol to form a negative number and adds it to the `negatives` list.
FAQs:
Q1: Can the methods discussed extract multiple negative numbers from a single string?
A1: Absolutely! Both methods are designed to extract all possible negative numbers present in a string. Whether there are multiple negative numbers or just one, these techniques will identify and extract them all.
Q2: What if the negative numbers are represented in a different format in the string?
A2: The methods described above primarily focus on extracting negative numbers in the commonly used format, where a leading “-” symbol denotes negativity. If the negative numbers are represented differently, such as using parentheses or words like “minus,” the regex pattern and conditions within the list comprehension can be adjusted accordingly.
Q3: How can I utilize the extracted negative numbers in calculations?
A3: Once you have extracted negative numbers from a string using the methods discussed, you can convert them to integers or floats using Python’s `int()` or `float()` functions, respectively. This allows you to perform any necessary mathematical operations with the extracted negative values.
Conclusion:
Extracting negative numbers from strings in Python is a common requirement in various programming scenarios. In this article, we explored two different approaches: using regular expressions and employing a combination of list comprehension and the `isdigit()` method. By following the code examples provided and understanding the concepts behind them, you should now be equipped to efficiently extract negative numbers from any string.
Get Number After String Python
Python, being a powerful programming language, offers a wide array of functions and methods to manipulate strings. However, there may be scenarios where you need to extract a number that comes after a specific string within a larger string. In this article, we will explore various methods and techniques to get the number after a string in Python. We will also address frequently asked questions regarding this topic.
Methods to Get the Number After a String in Python:
1. Using Regular Expressions:
Regular expressions provide a highly flexible and powerful way to search for patterns within a string. The `re` module in Python allows us to use regular expressions effectively. To get the number after a specific string, we can define a regular expression pattern using the `re.compile()` function and then use the `re.search()` or `re.findall()` functions to find the desired number(s).
Here’s an example of using regular expressions to get the number after a string:
“`
import re
text = “The price is $19.99.”
pattern = r”price is \$(\d+\.\d+)”
match = re.search(pattern, text)
if match:
number = match.group(1)
print(number) # Output: 19.99
“`
2. Using String Manipulation:
Another approach to extracting the number after a string is by using string manipulation methods that Python provides. We can use the `split()` function to split the text into different parts based on a delimiter (in this case, the string we want to locate). Once we have separated the text, we can access the desired number(s) using indexing or slicing techniques.
Here’s an example of using string manipulation to get the number after a string:
“`
text = “The quantity is 15.”
delimiter = “quantity is”
number = text.split(delimiter)[1].strip()
print(number) # Output: 15
“`
Frequently Asked Questions (FAQs):
Q1. Can I retrieve multiple numbers after a particular string?
Ans: Yes, you can retrieve multiple numbers after a particular string. The approach mentioned above using regular expressions (`re.findall()`) can return multiple numbers as a list.
Q2. What if the number after the string is an integer instead of a floating-point number?
Ans: The methods mentioned above can handle both floating-point and integer numbers. You can modify the regular expression pattern (`\d+` instead of `\d+\.\d+`) or convert the extracted number to the desired type using functions like `int()` or `float()`.
Q3. Is there a way to handle cases where the number is not present after the string?
Ans: Yes, we can handle such cases by using conditional statements. For example, after getting the match using regular expressions, we can check if it exists (`if match`) before accessing the number using `match.group()`.
Q4. Are there any performance considerations when using regular expressions?
Ans: Regular expressions can be powerful but can also impact performance, especially when dealing with large texts. If the string pattern to match is simple, using string manipulation techniques might provide better performance. However, for complex patterns or scenarios where the string format varies, regular expressions are the recommended choice.
Q5. How can I handle variations in the text before and after the string?
Ans: Regular expressions allow you to add flexibility to the search pattern. You can use qualifiers like `.*` to handle any number of characters before or after the target string. For example, `pattern = r”.*price is \$(\d+\.\d+).*”` will match any text that contains “price is $
In conclusion, extracting the number after a string is a common task in Python programming. By using regular expressions or string manipulation, you can easily retrieve the desired number(s) from a given text. Remember to consider the specific requirements of your scenario and choose the most suitable method accordingly.
Images related to the topic extract numbers from string python
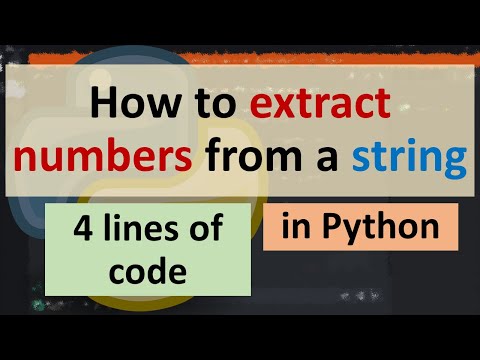
Found 22 images related to extract numbers from string python theme
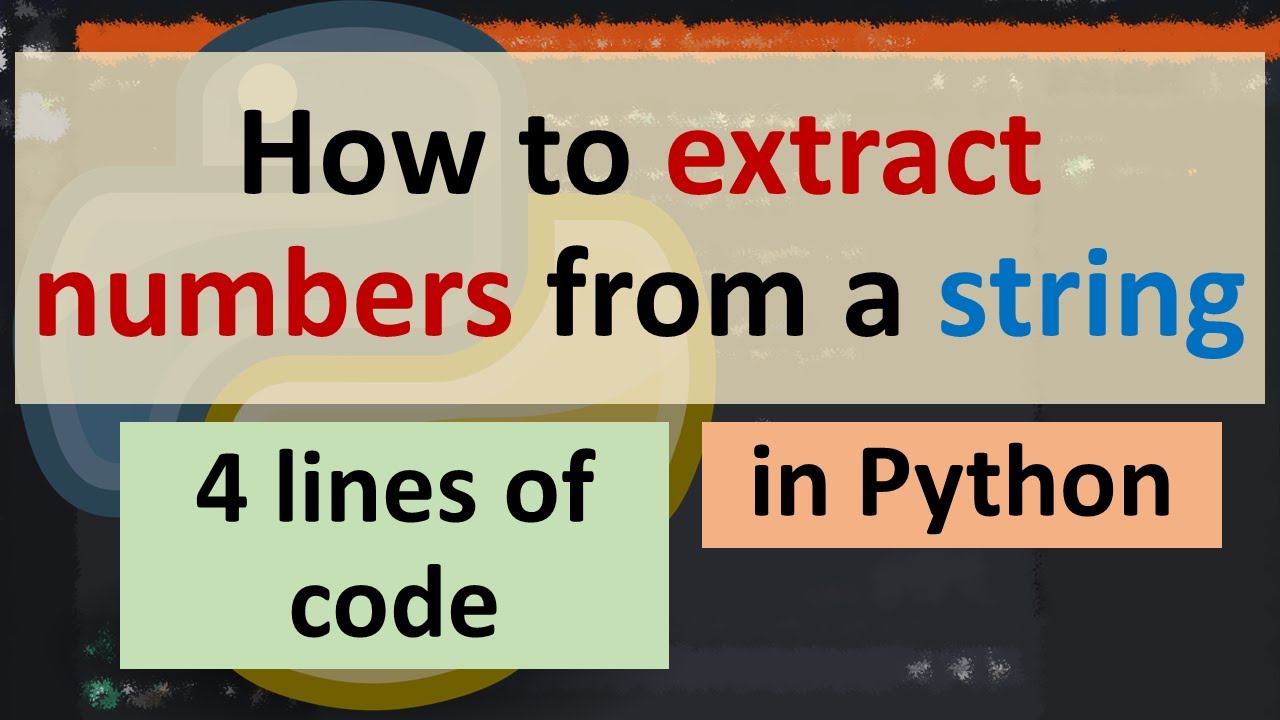

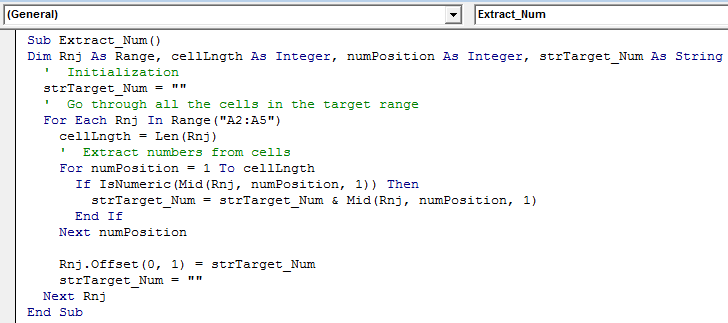

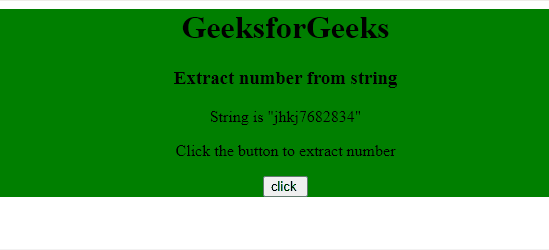
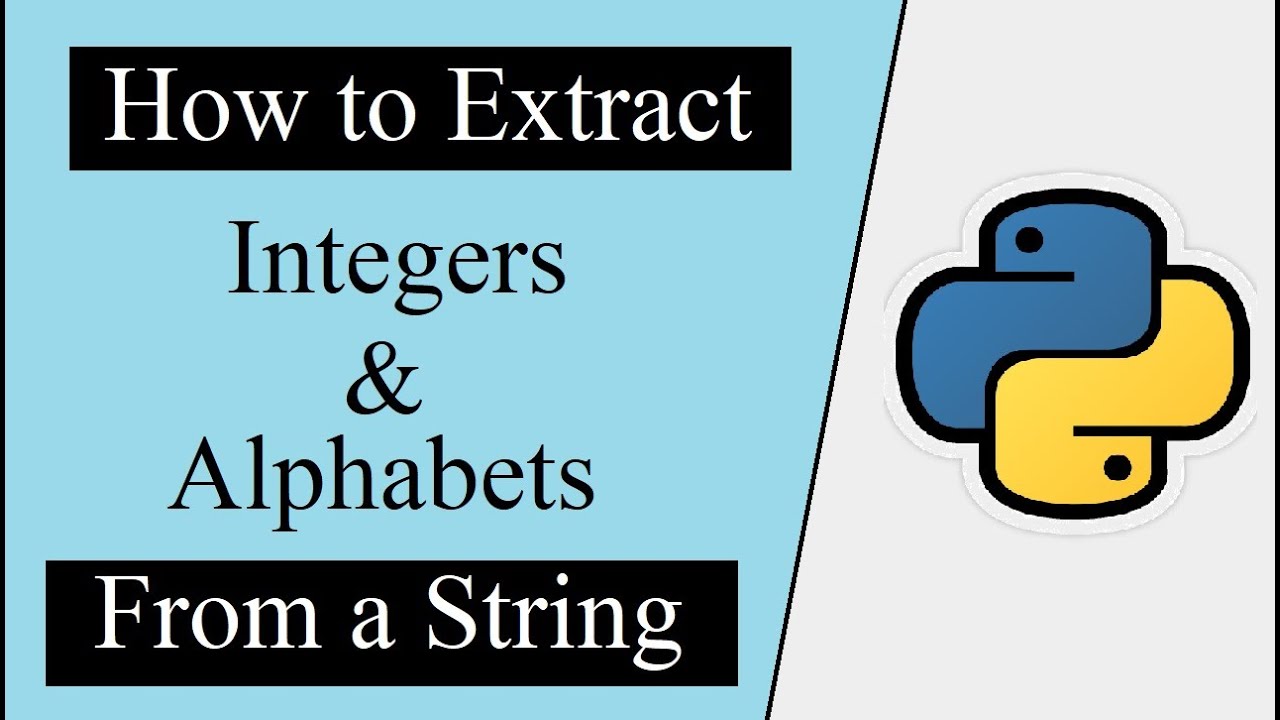
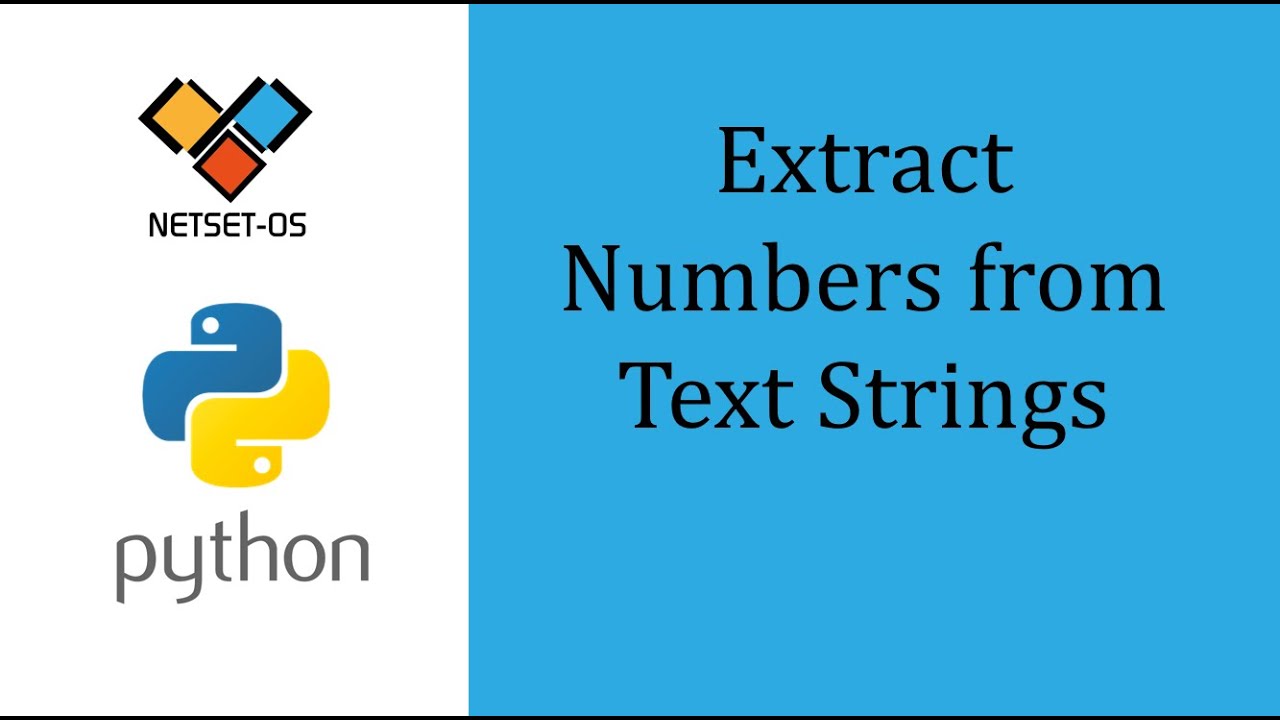
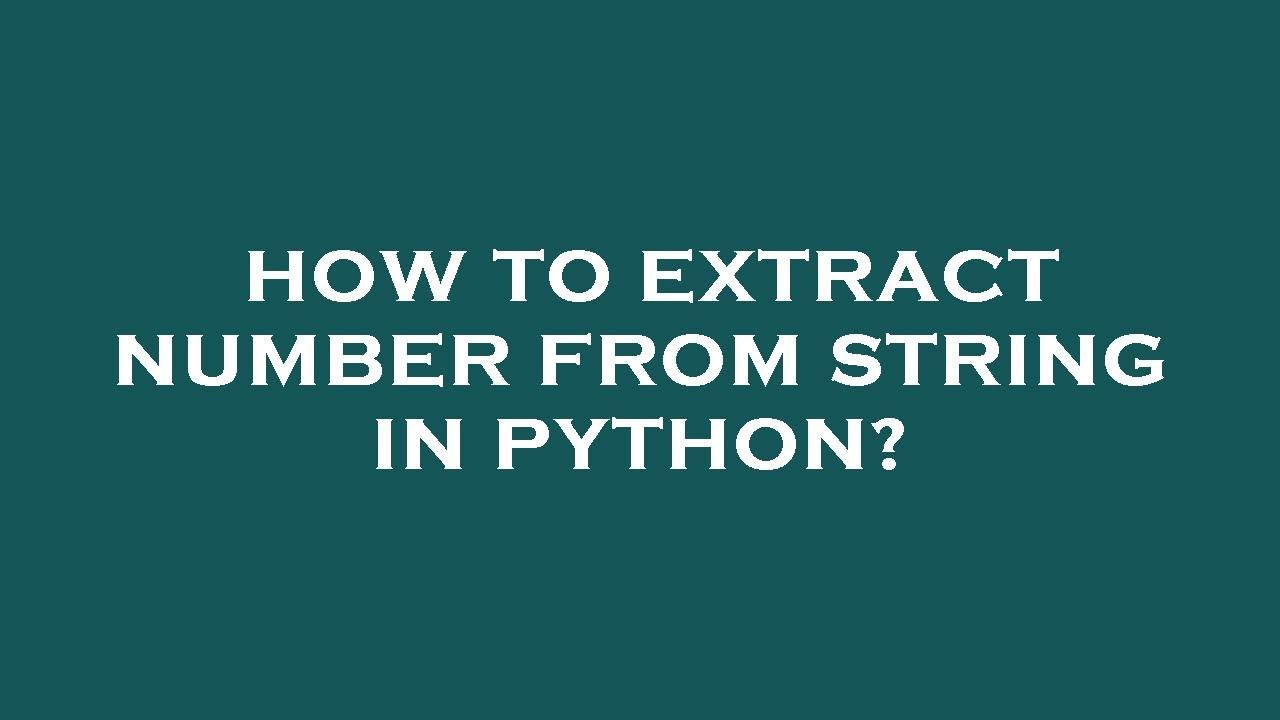
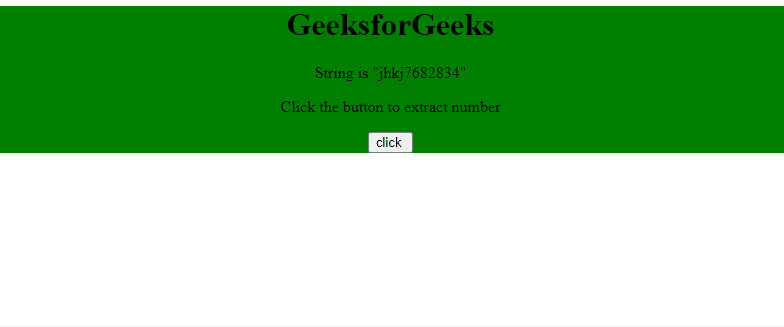
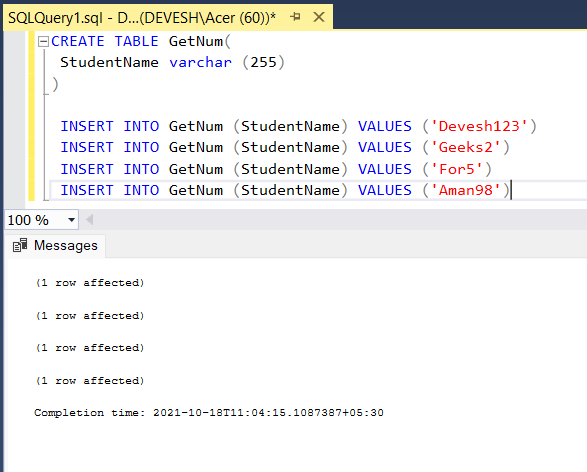
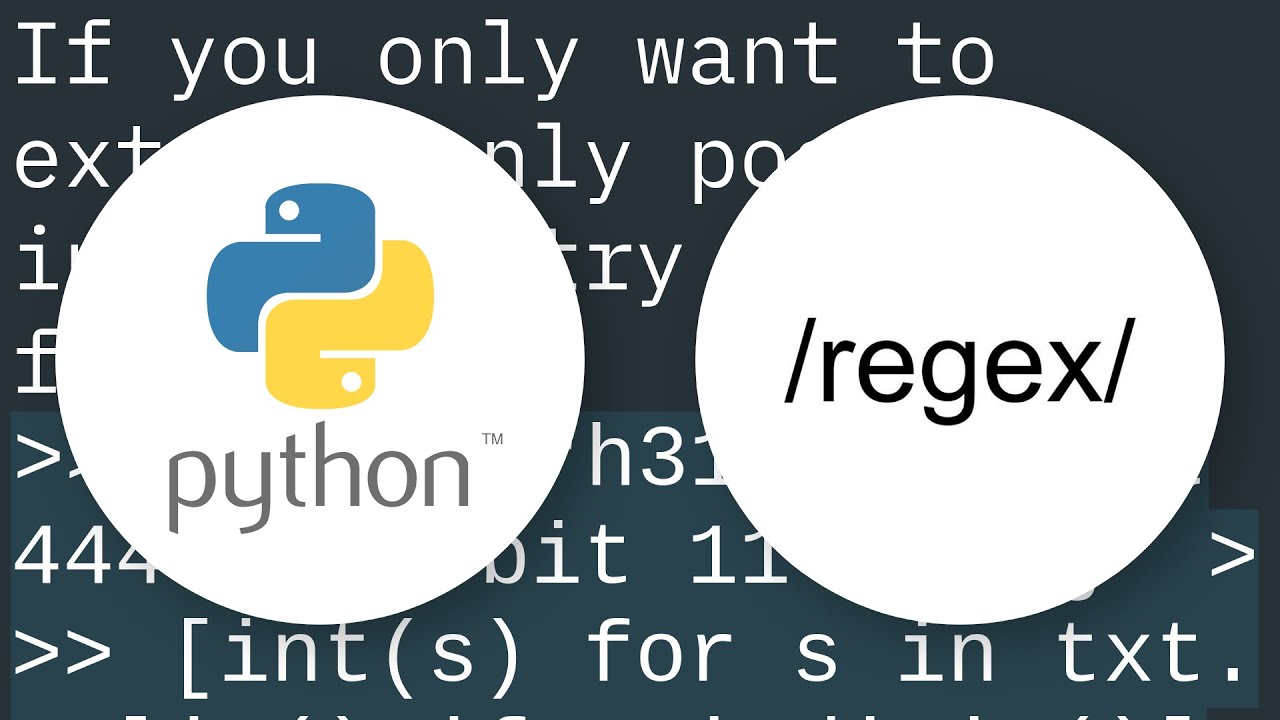

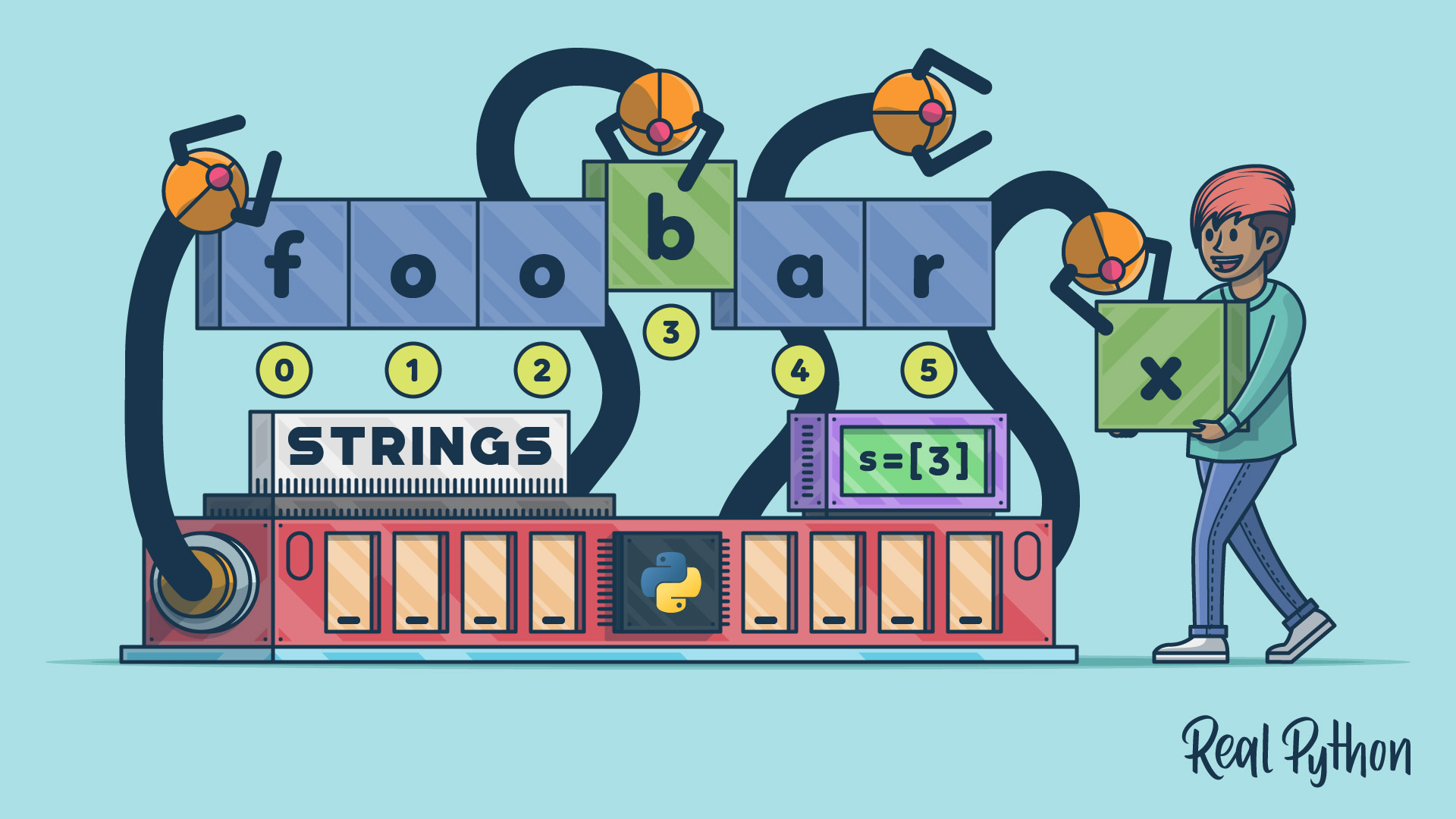

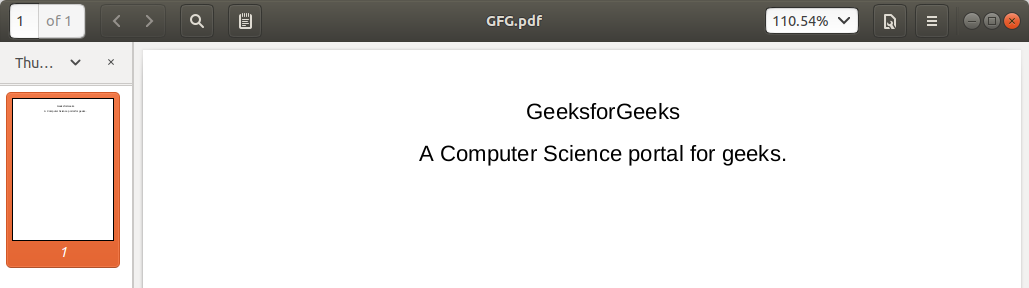
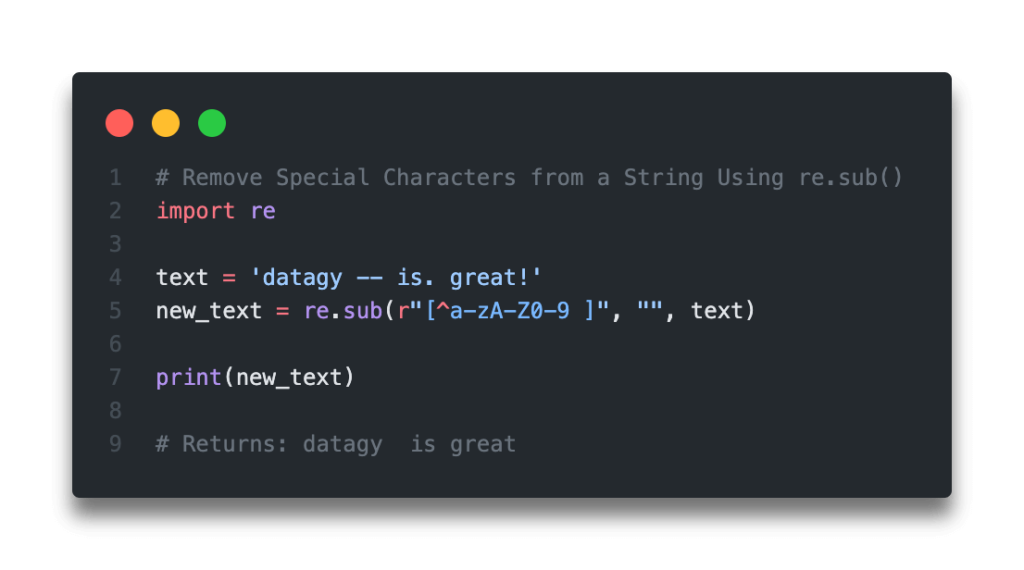
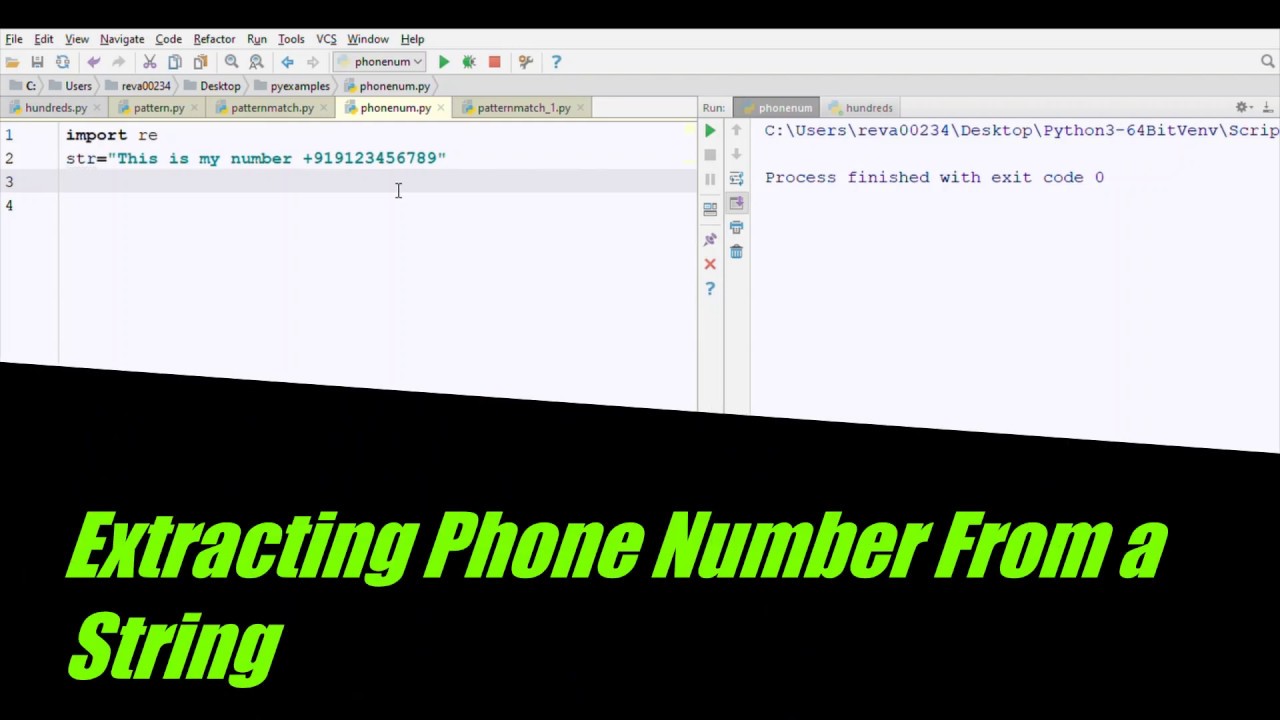
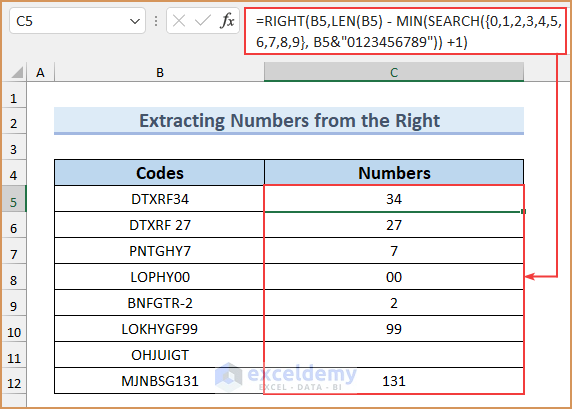

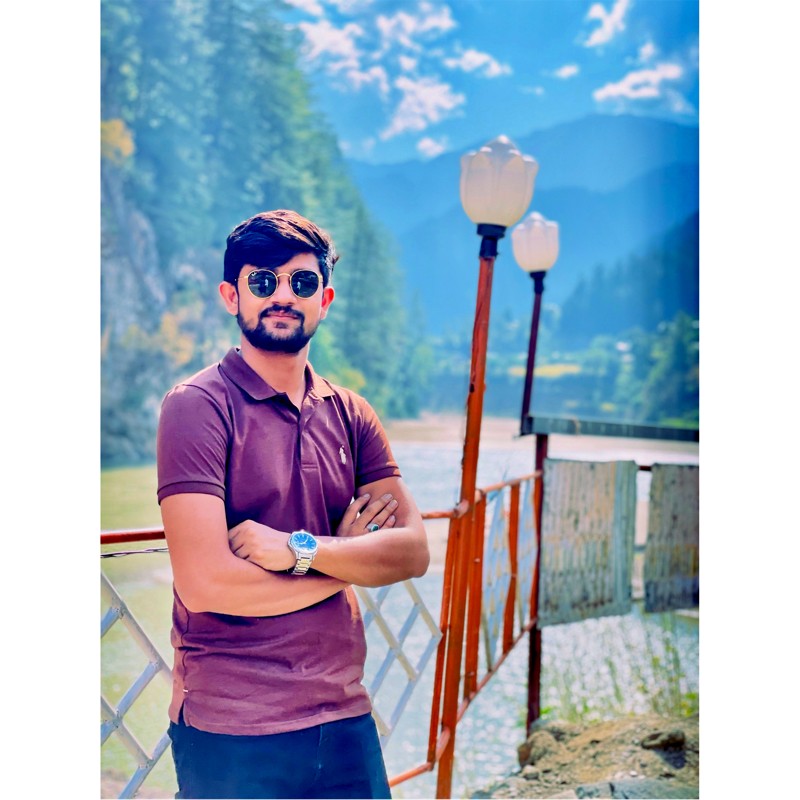
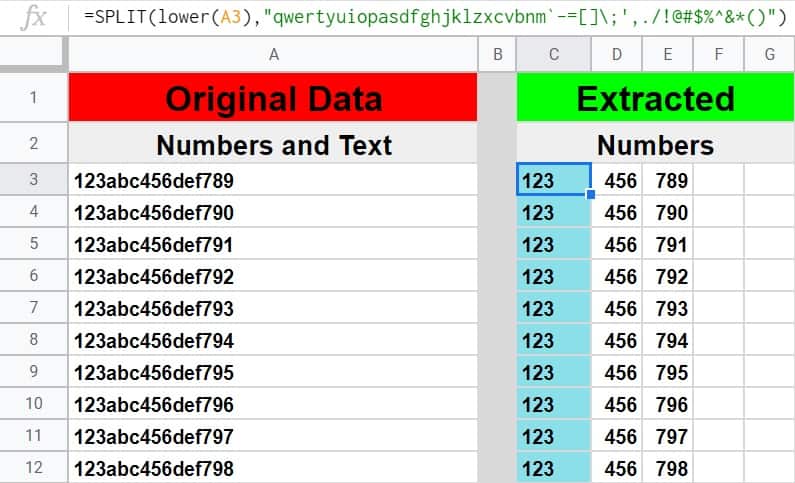
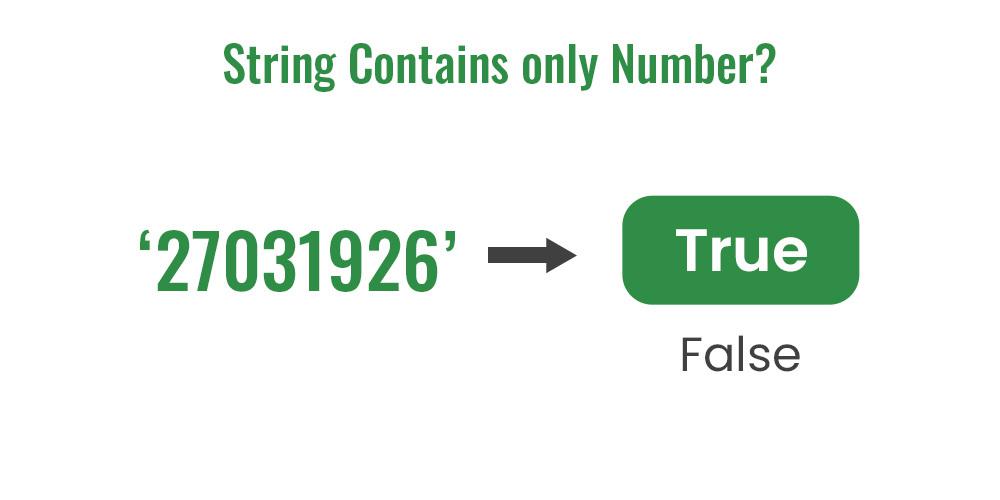
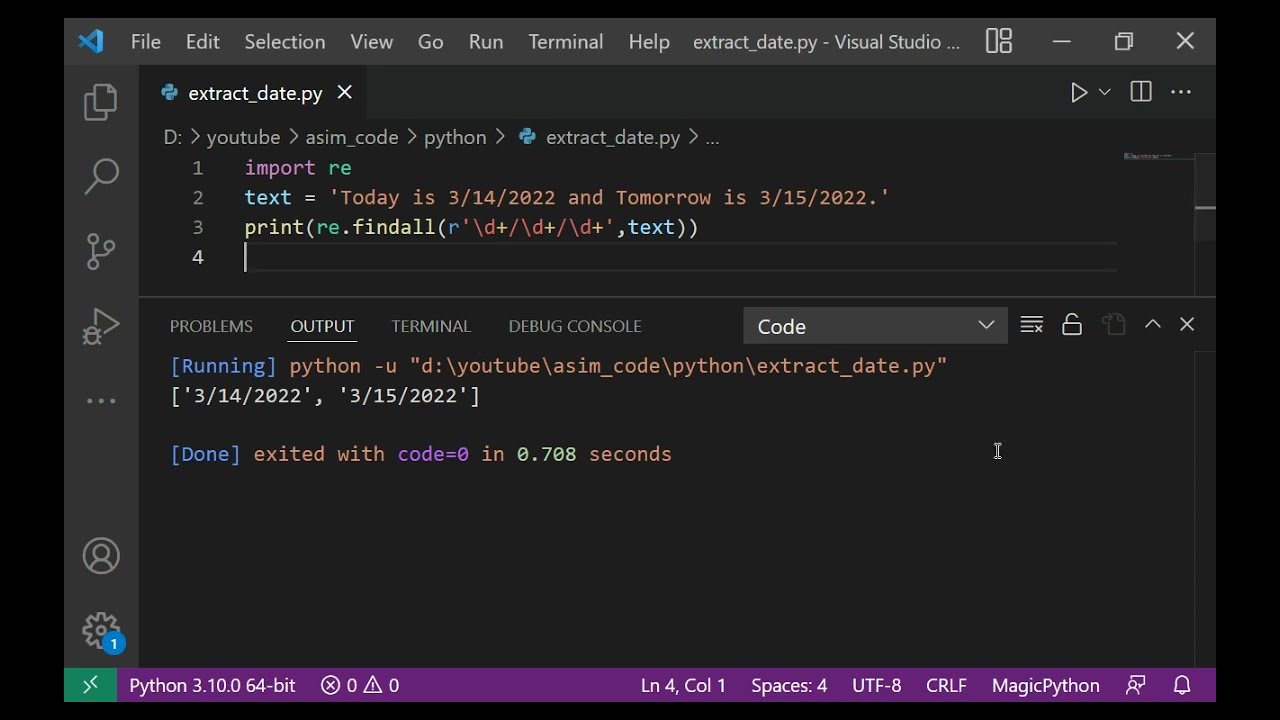
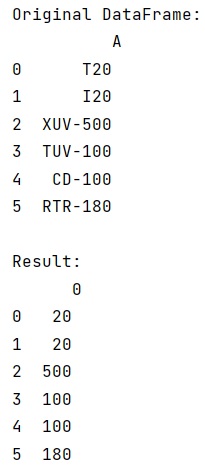





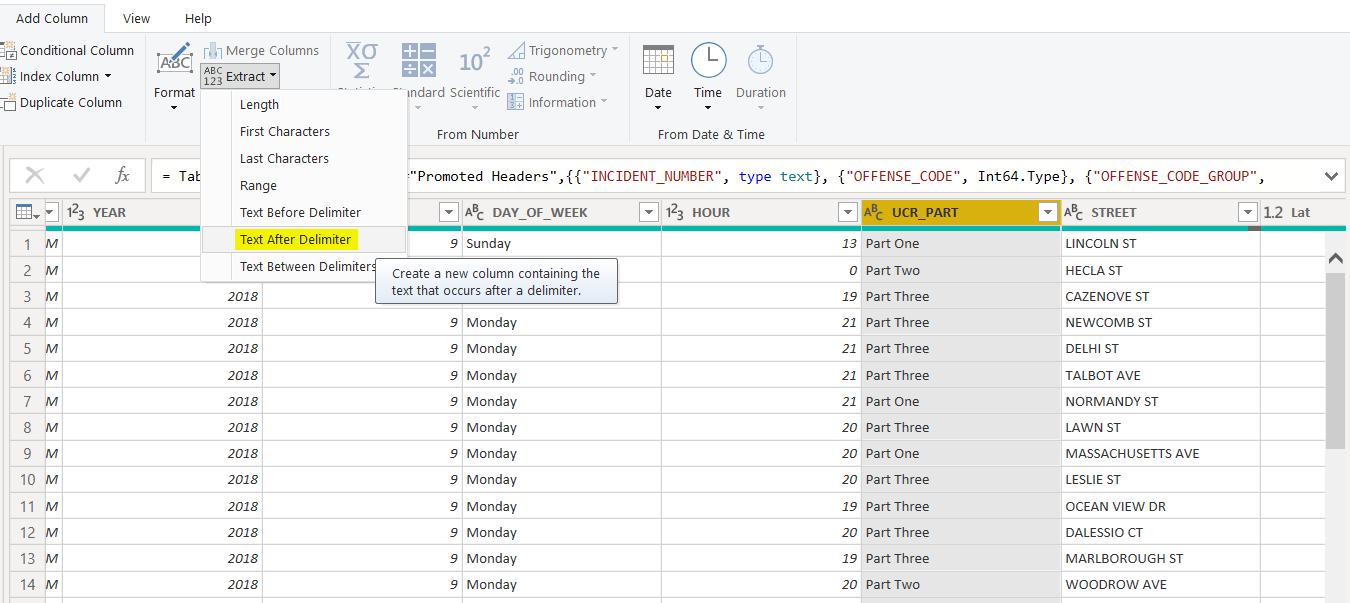

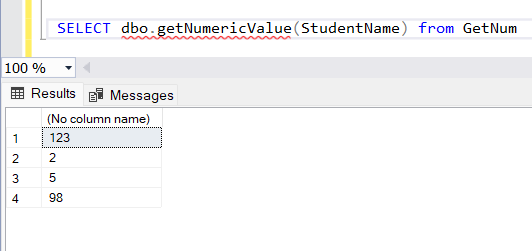

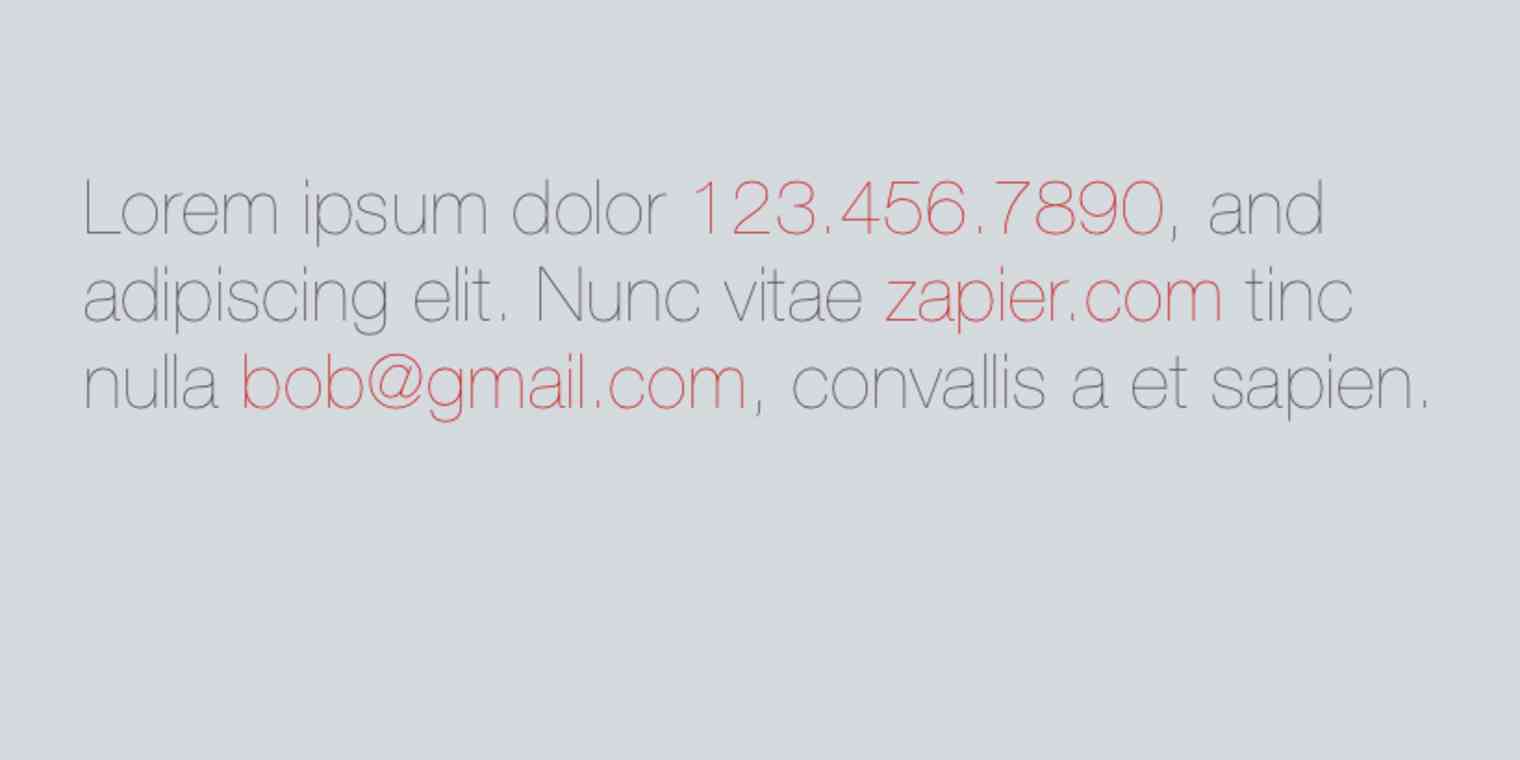
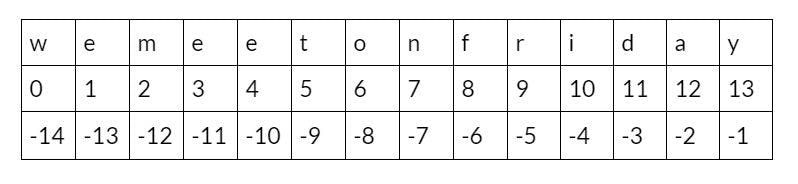
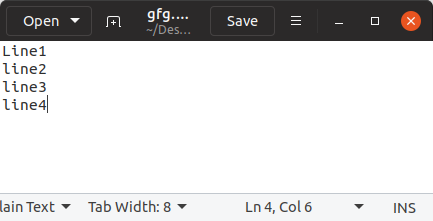
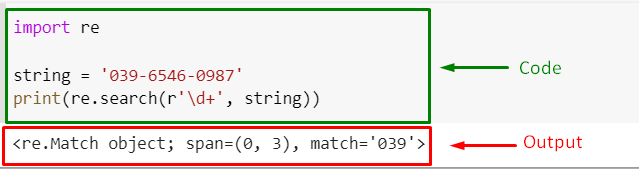


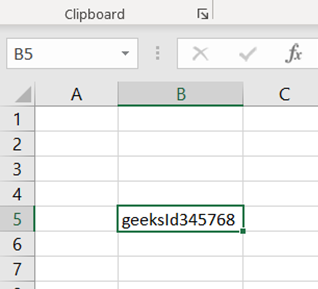
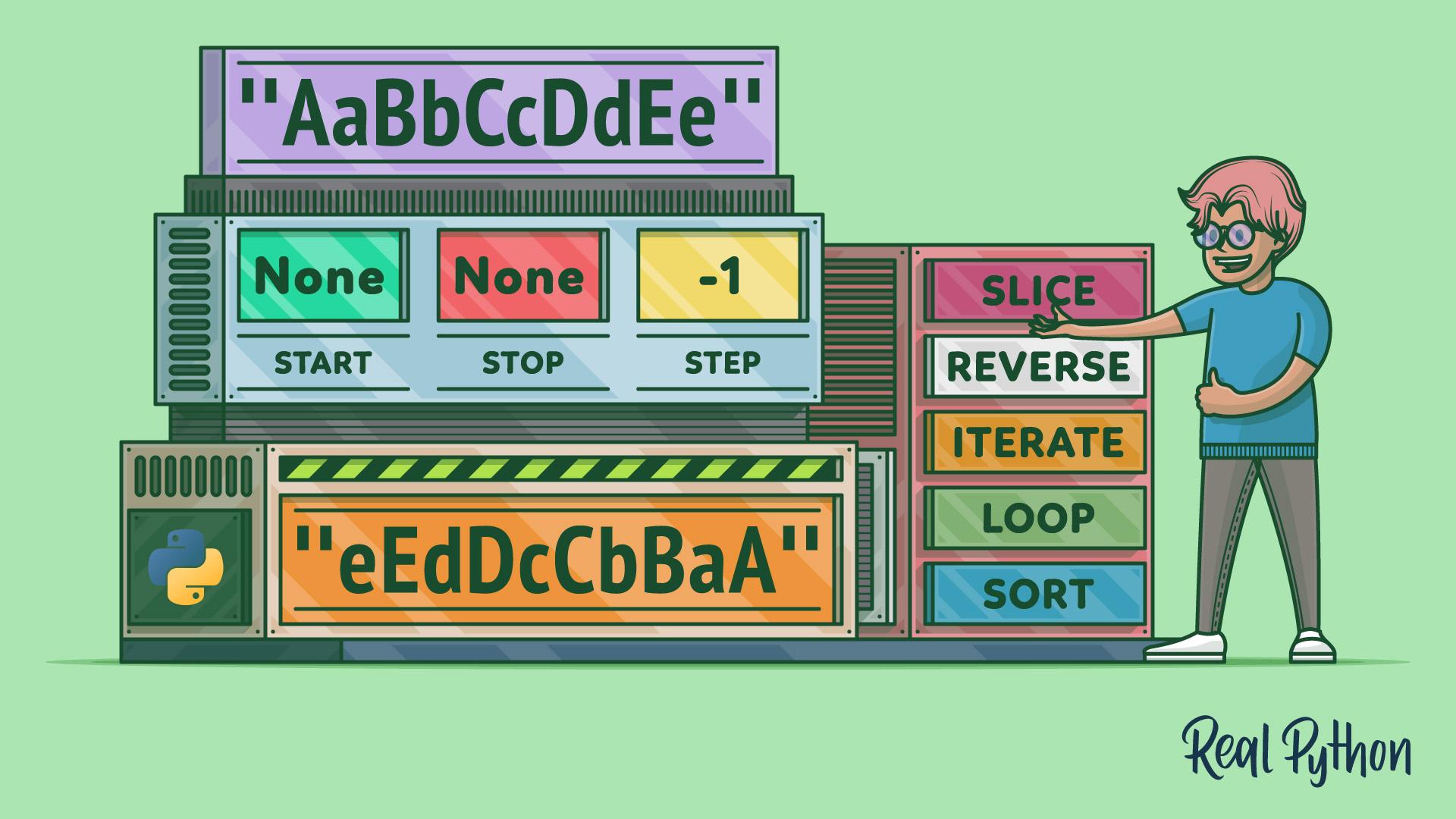
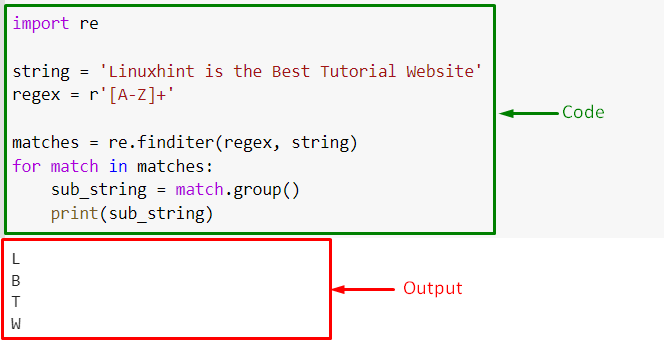
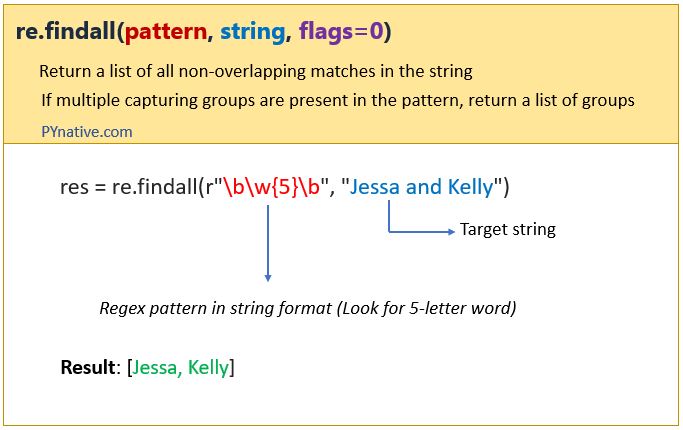


Article link: extract numbers from string python.
Learn more about the topic extract numbers from string python.
- How to extract numbers from a string in Python? – Stack Overflow
- Python | Extract numbers from string – GeeksforGeeks
- 2 Easy Ways to Extract Digits from a Python String – AskPython
- Python Find Number In String
- How To Extract Numbers From A String In Python? – Finxter
- Extract Numbers from String in Python – thisPointer
- How to extract numbers from a string in Python? – W3docs
- How can I extract a number from a string in Python?
- Extract Numbers From String in Python – Data Science Parichay
- Extract integer values from a string in Python – Devsheet
See more: nhanvietluanvan.com/luat-hoc