Except Exception As E
In the world of programming, exceptions play a crucial role in handling unforeseen errors and ensuring that programs run smoothly. An exception is an abnormal condition that arises during the execution of a program and disrupts its normal flow. Python, being a popular programming language, comes with its own set of exception handling mechanisms. One such mechanism is “Except Exception as e”. In this article, we will explore the concept of “Except Exception as e” in Python, its syntax, and various aspects related to exception handling.
Definition of Exception:
An exception is an event that occurs during the execution of a program, which disrupts the normal flow of the program’s instructions. When an exception occurs, it is said to be “raised”. In Python, exceptions are objects that are created when errors occur. These objects contain information about the error and can be used to handle and recover from the error.
Types of Exceptions:
Python provides a wide range of built-in exception classes that can be used to handle different types of errors. Some common types of exceptions include:
1. ValueError: This exception is raised when a function receives an argument of the correct type, but an invalid value.
2. TypeError: This exception is raised when an operation or function is applied to an object of an inappropriate type.
3. IOError: This exception is raised when an input/output operation fails, such as when a file cannot be opened.
4. IndexError: This exception is raised when a sequence subscript is out of range.
5. KeyError: This exception is raised when a dictionary key is not found.
Common Examples of Exceptions:
Exceptions can occur in various scenarios, depending on the nature of the program. Some common examples of exceptions include:
1. DivisionByZeroError: This exception occurs when a program tries to divide a number by zero.
2. FileNotFoundError: This exception occurs when a program tries to open a file that does not exist.
3. TypeError: This exception occurs when incompatible types are used together.
Reasons for Exceptions:
Exceptions can occur due to a wide range of reasons, including user input errors, invalid computations, mismatched data types, network failures, and more. They are designed to handle unexpected situations and prevent program crashes.
Ways to Address Exceptions:
The “Except Exception as e” syntax in Python is used to catch exceptions and handle them gracefully. When an exception is raised, the code within the “except” block is executed. The exception object is assigned to the variable “e”, which can be used to access information about the error.
Example:
“`
try:
# code that may raise an exception
except Exception as e:
# code to handle the exception
“`
Challenges in Dealing with Exceptions:
Handling exceptions efficiently can be challenging, especially when dealing with complex programs. Some common challenges include:
1. Identifying the source of the exception: In large codebases, pinpointing the exact location where an exception is raised can be difficult.
2. Handling multiple exceptions: Programs may encounter multiple exceptions, and handling each one appropriately requires careful planning and organization.
3. Avoiding error masking: When multiple exceptions are caught, it is important to ensure that one exception does not overshadow another, leading to undetected errors.
The Role of Exceptions in Decision-Making:
Exceptions are not only useful for handling errors but also play a vital role in decision-making. By raising exceptions in specific situations, programmers can control the flow of the program and make informed decisions based on the nature of the exception. Exceptions can be used to guide the program’s behavior and recover from errors gracefully.
Conclusion:
In conclusion, the “Except Exception as e” syntax in Python provides a powerful mechanism for handling exceptions effectively. By capturing exception objects and executing specific code blocks, developers can handle errors gracefully and ensure the smooth execution of their programs. Understanding the various types of exceptions, common examples, reasons for exceptions, and challenges in dealing with exceptions is crucial for writing robust and error-free code. By mastering the art of exception handling, programmers can enhance the reliability and stability of their Python applications.
FAQs:
1. What is “Except Exception as e” in Python?
“Except Exception as e” is a syntax used in Python to catch exceptions and handle them gracefully. The exception object is assigned to the variable “e”, which can be used to access information about the error.
2. Can I catch multiple exceptions using “Except Exception as e”?
Yes, you can catch multiple exceptions using multiple “except” blocks. Each “except” block will handle a specific exception type.
3. Can I define my own custom exceptions in Python?
Yes, Python allows you to define your own custom exceptions by creating a new class that inherits from the base “Exception” class.
4. How do I raise an exception in Python?
You can raise an exception using the “raise” statement followed by the exception class or object you want to raise. For example, `raise ValueError(“Invalid input!”)` will raise a ValueError exception with the specified error message.
5. What is the purpose of the “finally” block in exception handling?
The “finally” block is used to specify code that should be executed regardless of whether an exception is raised or not. It is commonly used to clean up resources or perform necessary closing operations.
Keywords: Except Exception as e Python, Python try except print error, Custom exception Python, Exception Python, Try except error Python, Raise error Python, Except exception e syntaxerror invalid syntax, Try except finally Python, except exception as e.
Python Tutorial: Using Try/Except Blocks For Error Handling
Keywords searched by users: except exception as e Except Exception as e Python, Python try except print error, Custom exception Python, Exception Python, Try except error Python, Raise error Python, Except exception e syntaxerror invalid syntax, Try except finally Python
Categories: Top 67 Except Exception As E
See more here: nhanvietluanvan.com
Except Exception As E Python
Python, a widely-used and powerful programming language, offers an extensive collection of built-in functions, modules, and features that make it a popular choice among developers. Two essential concepts in Python programming are the keywords “except” and “exception.” In this article, we will delve into the detailed differences between the two and explore their usage.
Understanding Exceptions:
In the context of programming, an exception is an occurrence that disrupts the normal flow of a program execution. It is often a result of unexpected or erroneous situations. When an exception arises, the program halts its normal execution and jumps to a specific location, known as an exception handler or an exception block. It allows you to handle the exceptional case gracefully and take appropriate actions. Common examples of exceptions might include dividing a number by zero, accessing an index out of range, or attempting to open a file that doesn’t exist.
Using the “except” Statement:
The “except” statement is an integral part of Python’s exception handling mechanism. It allows you to catch and handle specific exceptions that might arise during the execution of your code. The syntax for using “except” is as follows:
“`
try:
# Code that might raise an exception
except ExceptionType:
# Code to handle the exception
“`
In the above code snippet, the code within the “try” block is executed. If an exception of type “ExceptionType” occurs within the “try” block, the execution is immediately transferred to the corresponding “except” block. Here, you can write additional code to handle the exception gracefully.
Differences Between except and Exception:
The terms “except” and “exception” are often used interchangeably when referring to Python’s exception handling mechanism. However, there exists a crucial difference between the two. “Except” is a keyword used to introduce an exception handler block, while “exception” is a commonly used term for the object being raised.
When to Use “except”:
The “except” statement is primarily used when you want to catch a specific exception. For example, you might want to catch a “ZeroDivisionError” while attempting a division operation or a “FileNotFoundError” when dealing with file I/O operations. By utilizing “except,” you can handle these exceptions by writing code that gracefully handles the exceptional case.
When to Use “Exception”:
On the other hand, “Exception” is a generic base class for all exceptions in Python. It serves as a fallback option when you want to catch and handle any exception that might occur within the “try” block. While it is generally not advisable to use “Exception” as a catch-all, it can be useful in certain cases where you want to handle multiple exceptions in a similar manner.
Example Usage:
Consider the following code snippet:
“`
try:
result = x / y
except ZeroDivisionError:
print(“Error: Division by zero”)
except ValueError:
print(“Error: Invalid input”)
except Exception as e:
print(“Error:”, str(e))
“`
Here, we attempt to divide the variable “x” by “y.” If a “ZeroDivisionError” exception occurs, we handle it by printing an error message stating “Division by zero.” Similarly, if a “ValueError” exception occurs, we handle it by printing a message for “Invalid input.” The final “except” block with “Exception as e” ensures that any other exceptions are caught. We print a generic error message that includes the specific exception raised.
FAQs:
Q: Can I have multiple “except” blocks for a single “try” block?
A: Yes, it is possible to have multiple “except” blocks for a single “try” block. This allows you to handle different types of exceptions separately.
Q: What happens if an exception is not caught?
A: If an exception is not caught using an “except” block, it will propagate up the call stack until it is caught in an outer exception handler or until the program terminates, resulting in an error message.
Q: Is there a specific order in which “except” blocks should be defined?
A: Yes, the order of the “except” blocks is significant. Python evaluates them from top to bottom, and the first matching exception block will be executed. It is important to handle exceptions from the specific to the general.
Q: Can I raise my own exceptions?
A: Absolutely! Python allows you to raise your own exceptions using the “raise” statement. This allows you to create custom exceptions that suit your program’s specific needs.
In conclusion, the “except” statement and “exception” play crucial roles in Python’s exception handling mechanism. The former helps catch and handle specific exceptions, while the latter serves as a base class for all exceptions. Understanding their differences and appropriate usage ensures your code handles exceptional cases gracefully, resulting in robust and reliable programs.
Python Try Except Print Error
Introduction
In Python, an exception is an event that occurs during the execution of a program that disrupts the normal flow of instructions. These exceptions can range from syntax errors to runtime errors, such as dividing by zero or accessing an undefined variable. To handle these exceptions effectively, Python provides a powerful mechanism called “try-except.”
The try-except block allows us to catch and handle exceptions in our code, ensuring that our program does not terminate abruptly in response to unexpected errors. In this article, we will explore the Python try-except block and the ways it can be used to identify and gracefully handle errors. Additionally, we will discuss the print error feature and provide a comprehensive understanding of its implementation.
Python Try-Except Block
The try-except block allows us to specify a piece of code that may potentially raise an exception, along with a block of code to handle the exception if it occurs. The general syntax of the try-except block in Python is as follows:
“`
try:
# Code that may raise an exception
…
except ExceptionType:
# Exception handling code
…
“`
When an exception occurs within the try block, the program skips the remaining code inside the try block and proceeds to the except block, where the relevant exception handling code is executed. The “ExceptionType” is the specific type of exception we want to handle. If we don’t specify any exception type, the except block will catch all exceptions.
Print Error in Python
The print error feature in Python is used to output detailed information about an exception occurrence. By printing the error message, we can gain insights into the cause and nature of the error, enabling us to troubleshoot and rectify the issue effectively. To print an error message, we use the built-in “print()” function along with the “sys” module, which provides access to the interpreter’s variables and functions related to the Python system.
Here’s an example of how to print an error message:
“`python
import sys
try:
# Code that may raise an exception
…
except ExceptionType as e:
# Exception handling code
print(“An error occurred:”, e)
“`
In the above example, the variable “e” captures the exception object, allowing us to extract relevant information about the error using the “print()” function. The error message provides details such as the exception type, traceback information, and any additional custom messages we choose to include.
FAQs about Python Try Except Print Error
Q: Can I have multiple except blocks in a try-except statement?
A: Yes, you can have multiple except blocks in a try-except statement to handle different types of exceptions separately. This allows for customized exception handling based on the specific needs of your program.
Q: What happens if an exception is raised that is not caught by any except block?
A: If an exception occurs within the try block and is not caught by any except block, it will propagate up the call stack until it is caught by an outer try-except block or, if not caught at all, ultimately causing the program to terminate prematurely.
Q: Can I raise an exception manually?
A: Yes, you can raise an exception manually using the “raise” statement. This allows you to create custom exceptions or trigger specific error conditions based on your program’s requirements.
Q: How can I handle multiple exceptions in a single except block?
A: To handle multiple exceptions in a single except block, you can enclose the exception types within parentheses, separated by commas. For example:
“`python
try:
# Code that may raise an exception
…
except (ExceptionType1, ExceptionType2) as e:
# Exception handling code
…
“`
Q: Is it necessary to always include a try-except block in my code?
A: No, it is not always necessary to include a try-except block. It is recommended to use try-except only for code sections where you anticipate potential exceptions that could occur. Not all parts of your code will require explicit exception handling.
Conclusion
The Python try-except block is a crucial mechanism for handling exceptions and maintaining program stability. By leveraging the print error feature, developers gain valuable insights into the nature of exceptions, aiding in troubleshooting and resolving issues efficiently. Remember to consider the specific exception types you want to handle and utilize appropriate error messages to provide clarity about the encountered errors. Mastering these techniques will enable you to write robust and fault-tolerant Python programs.
Images related to the topic except exception as e
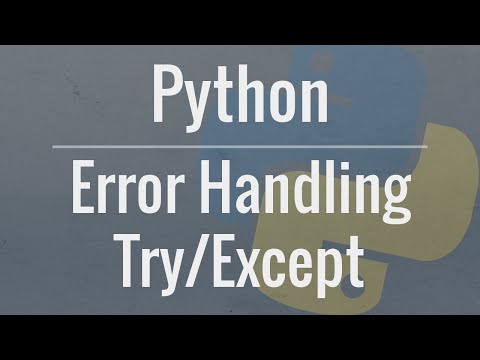
Found 15 images related to except exception as e theme

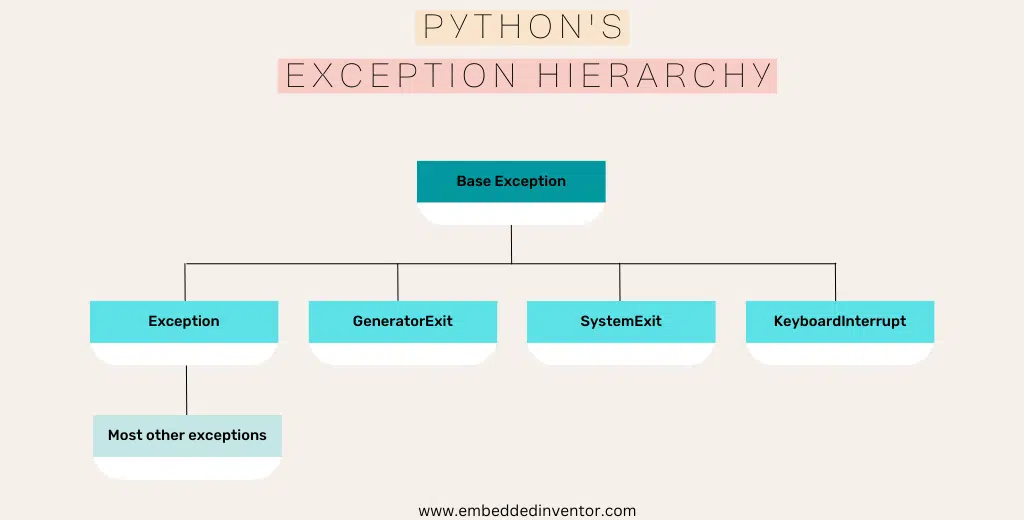
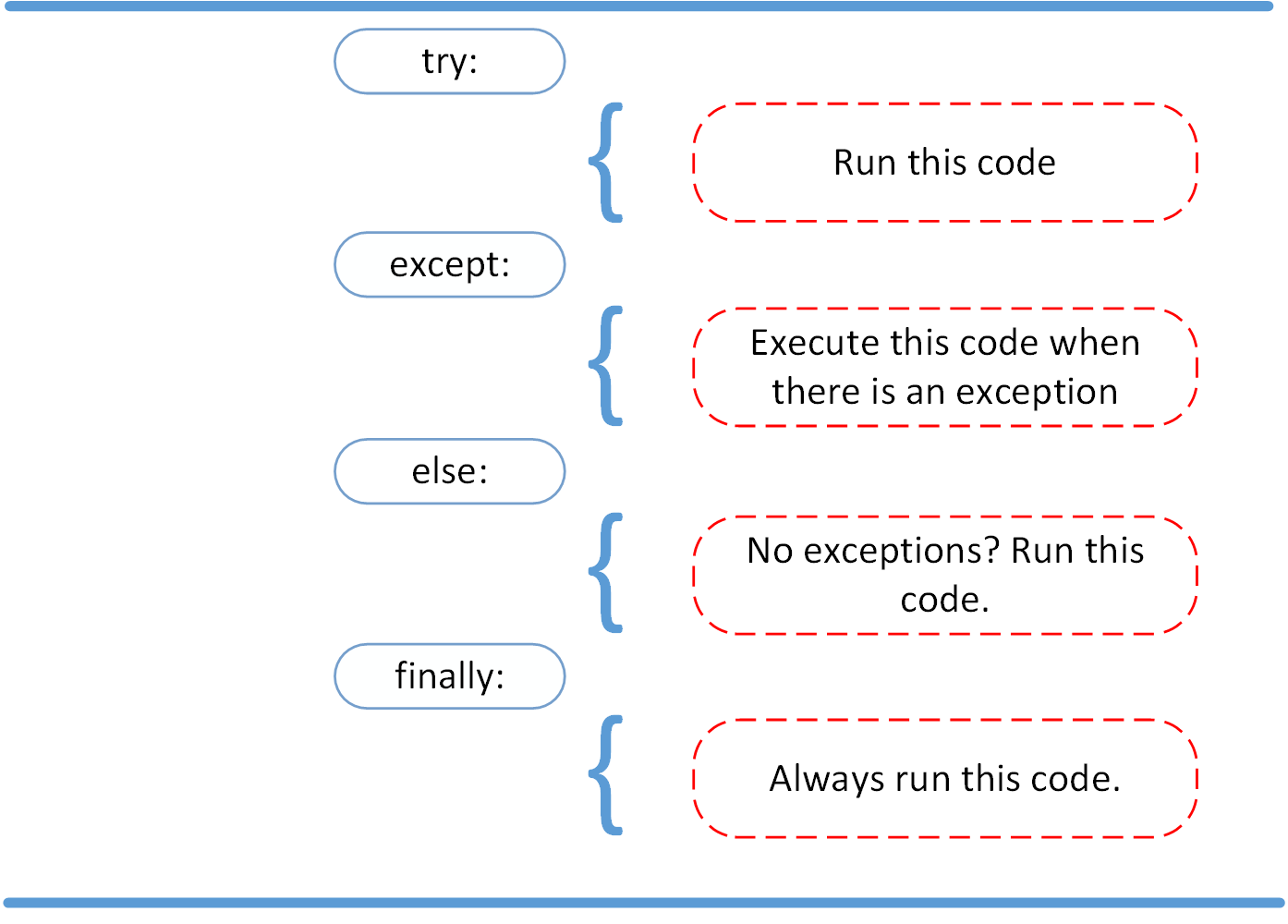
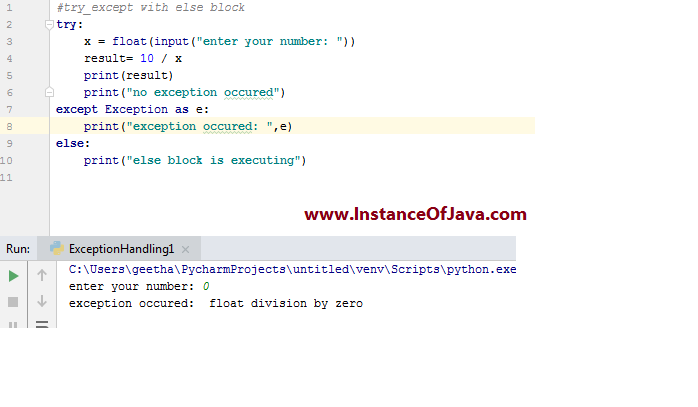
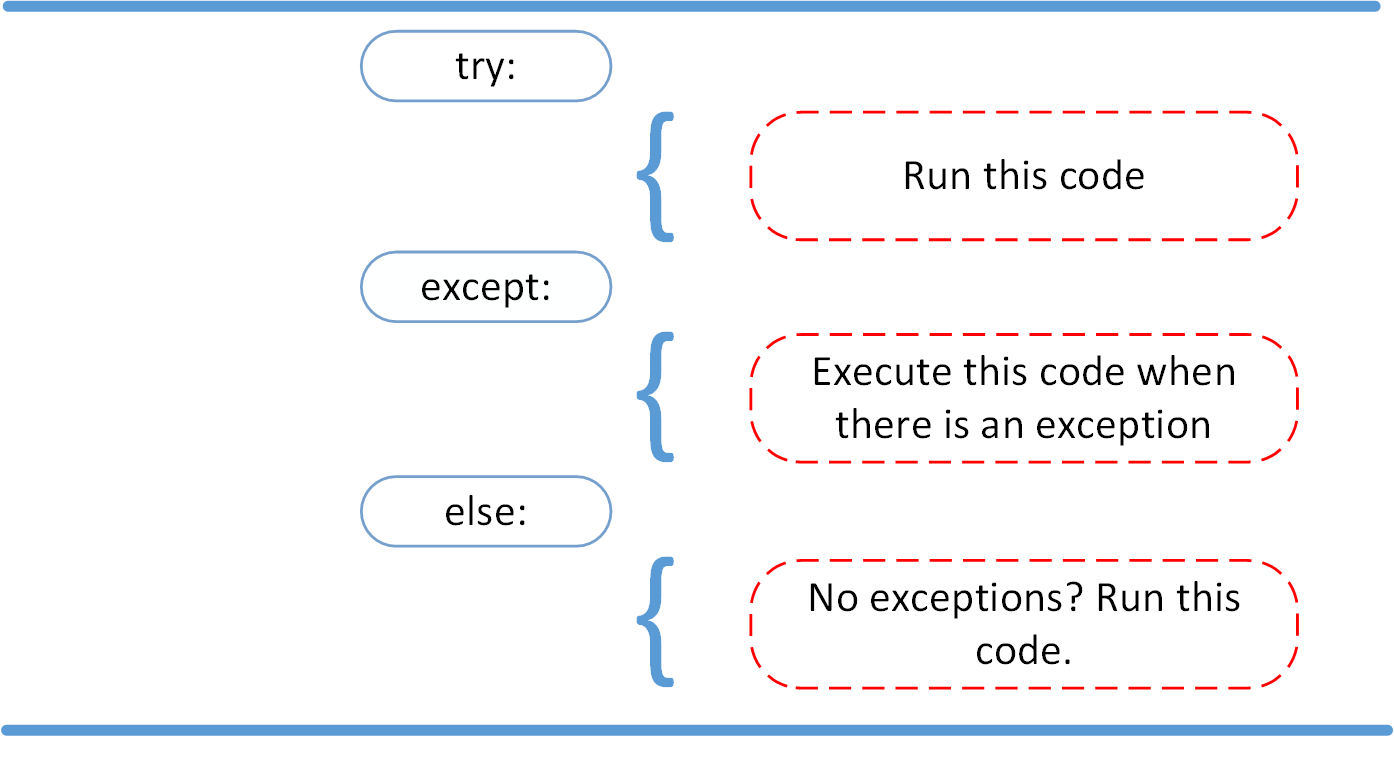
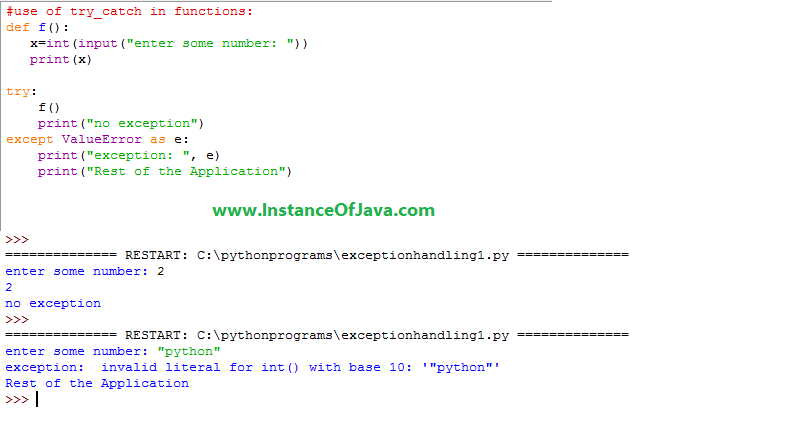


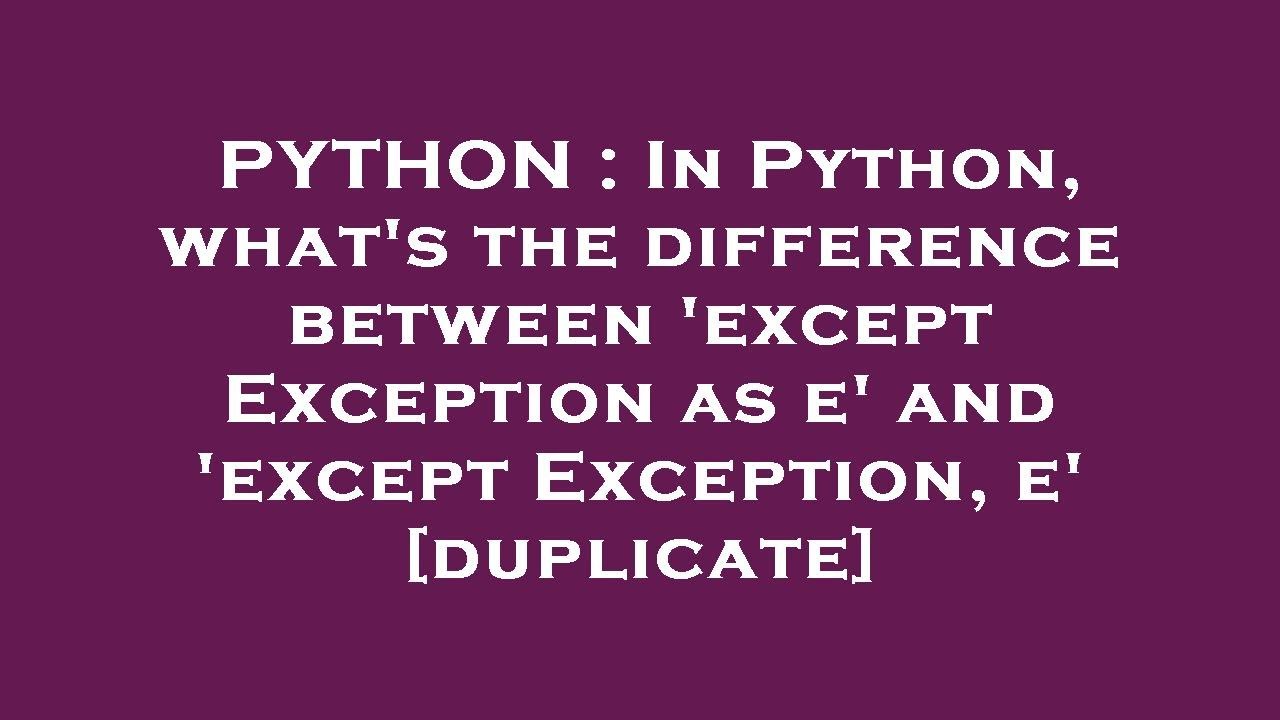

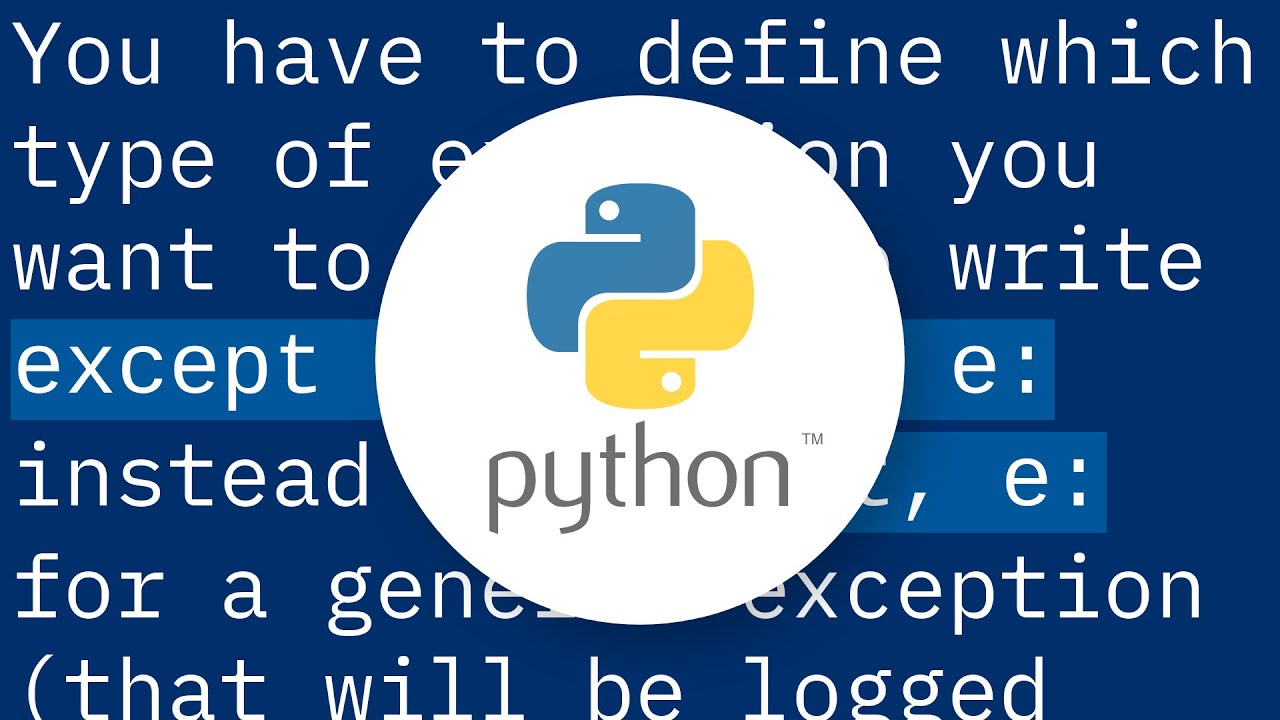

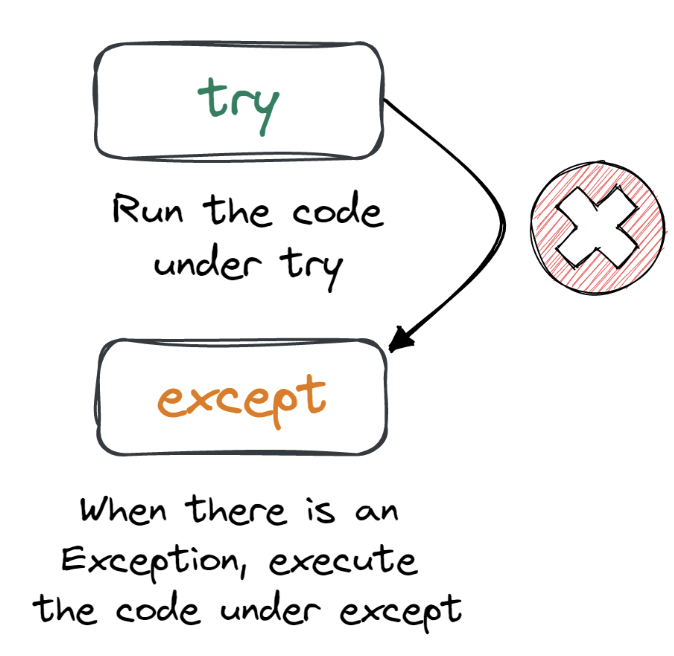
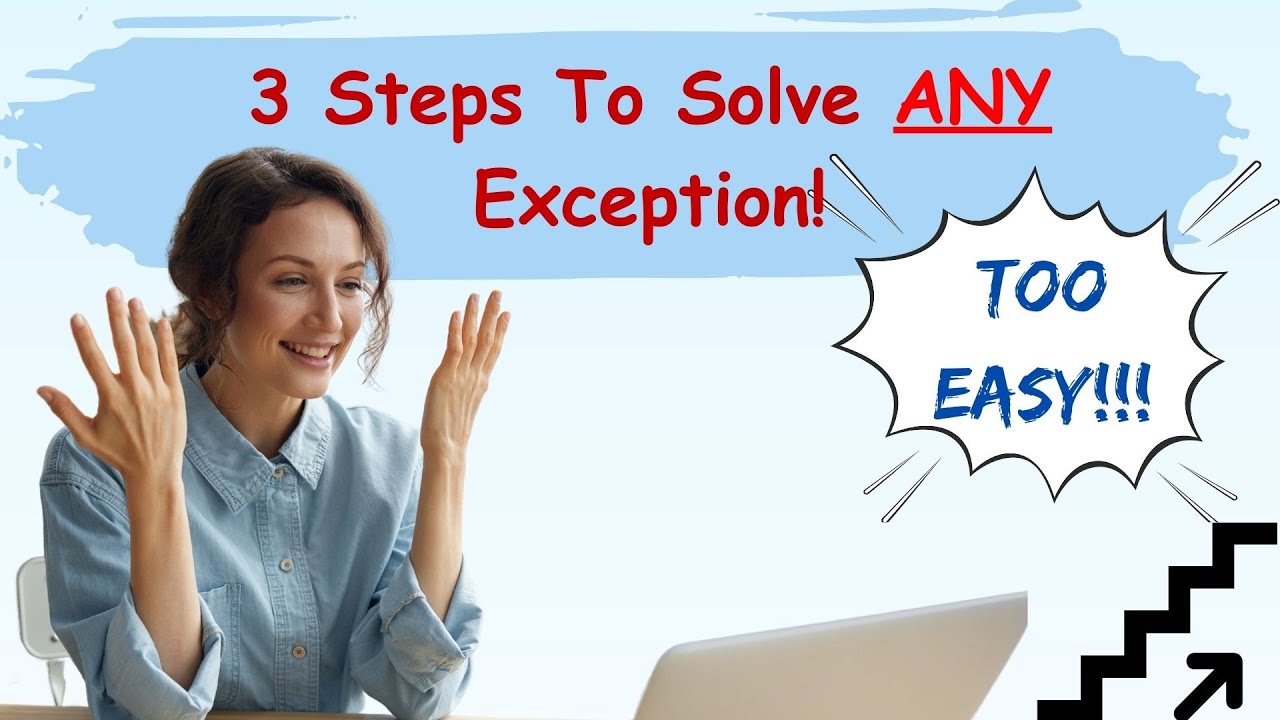

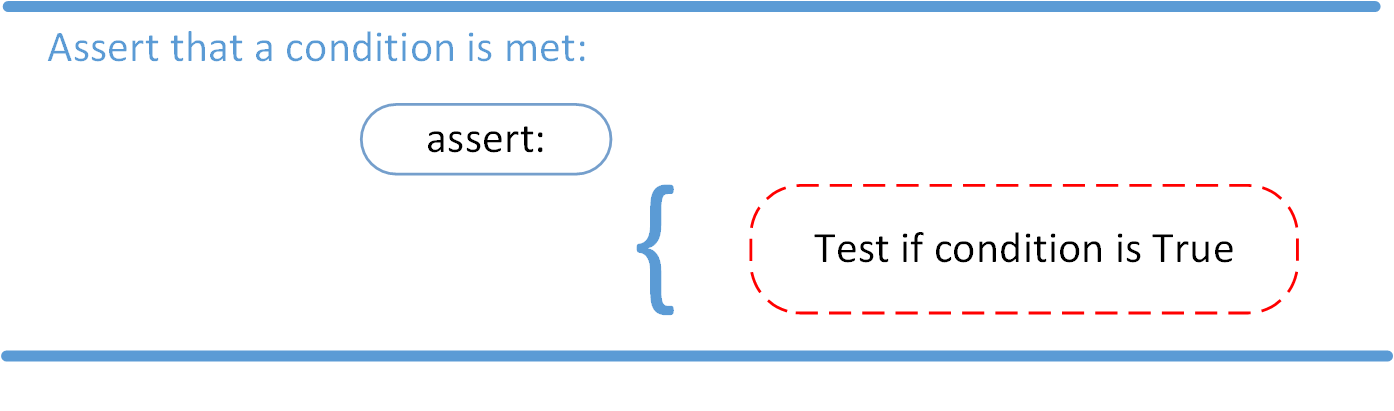

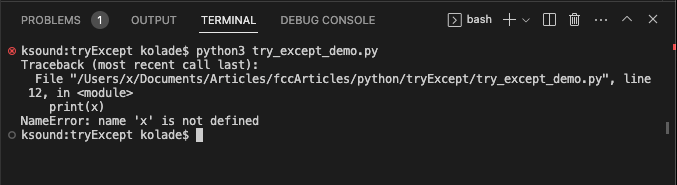
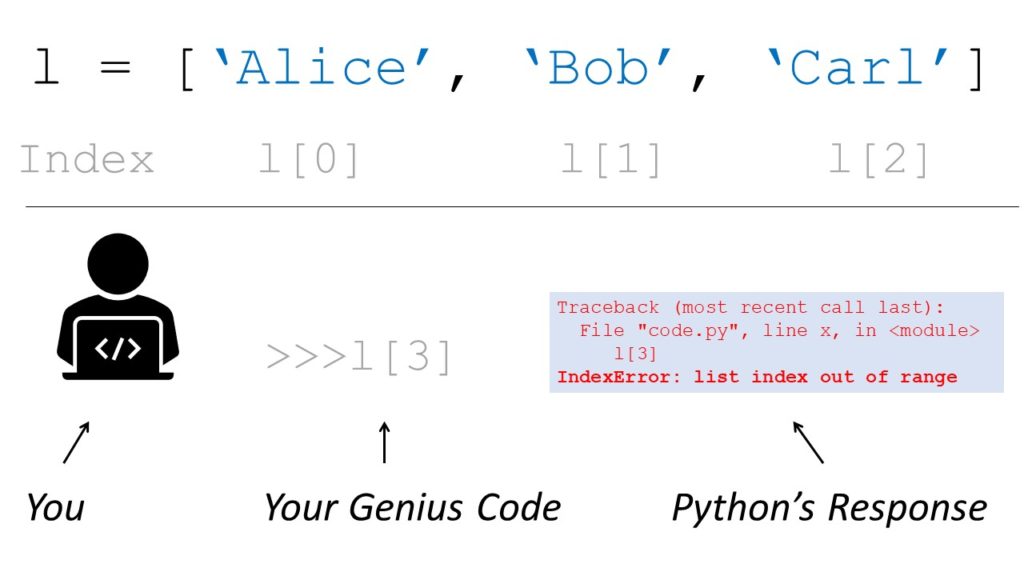


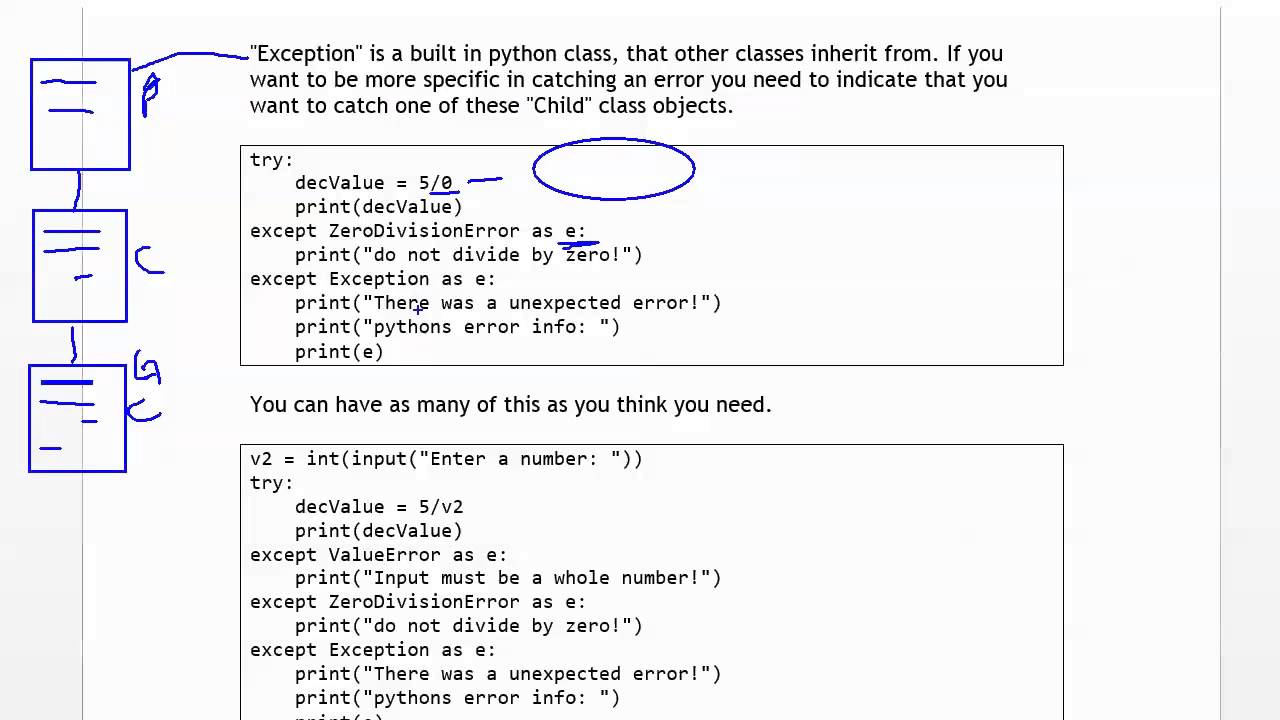



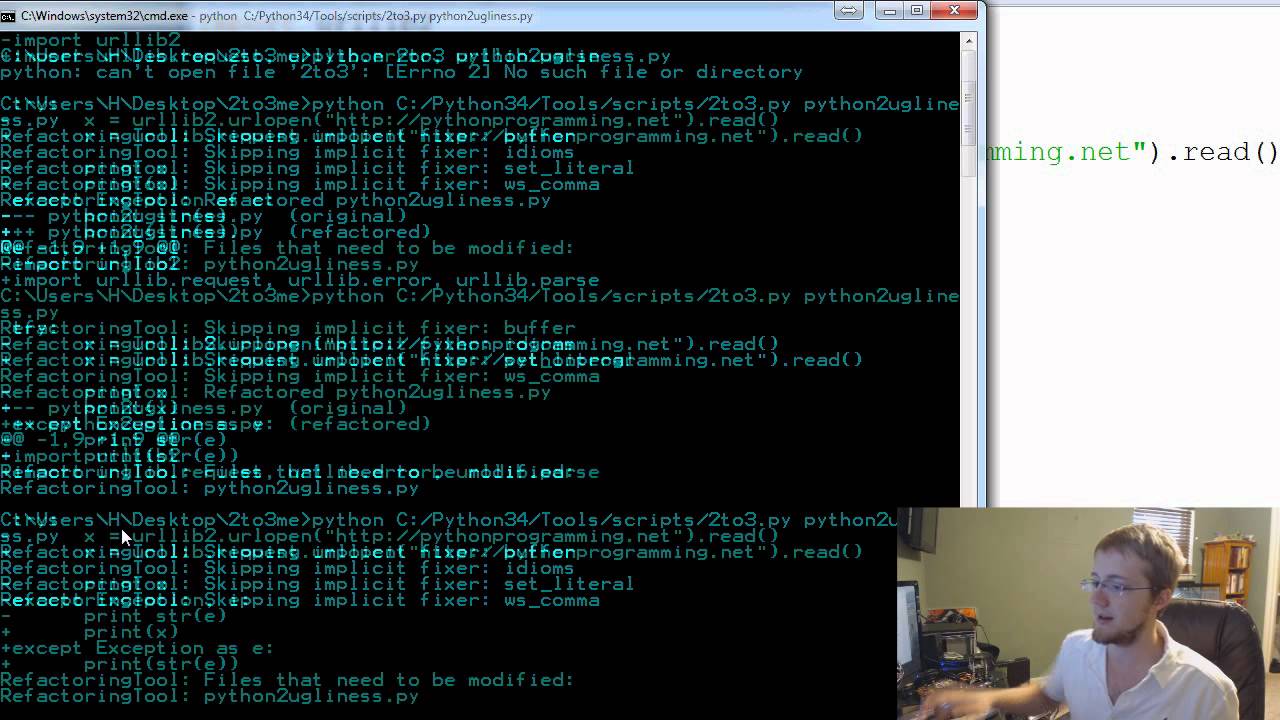

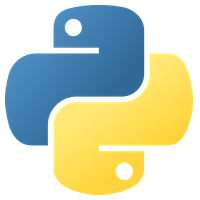
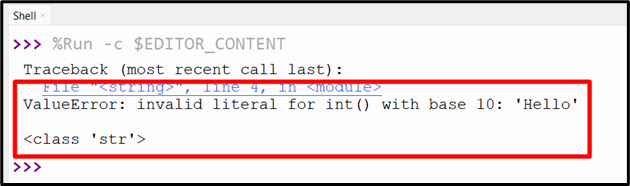
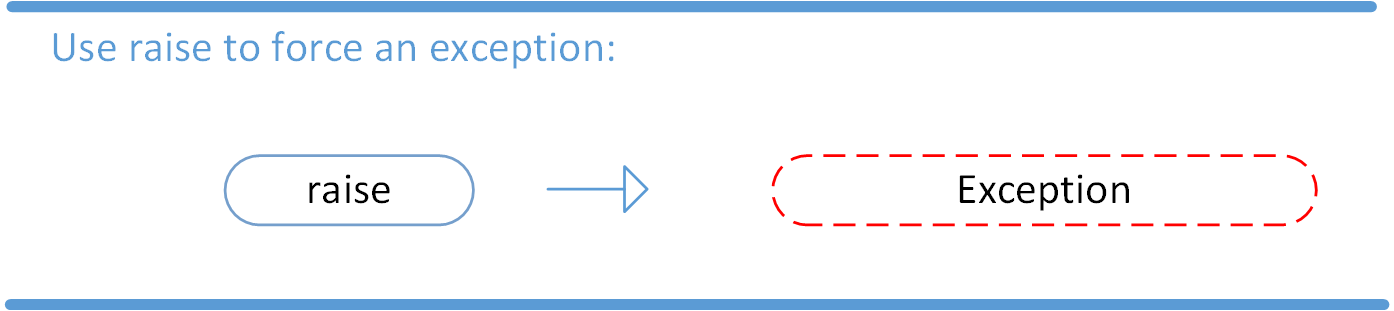
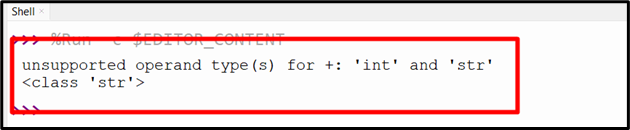

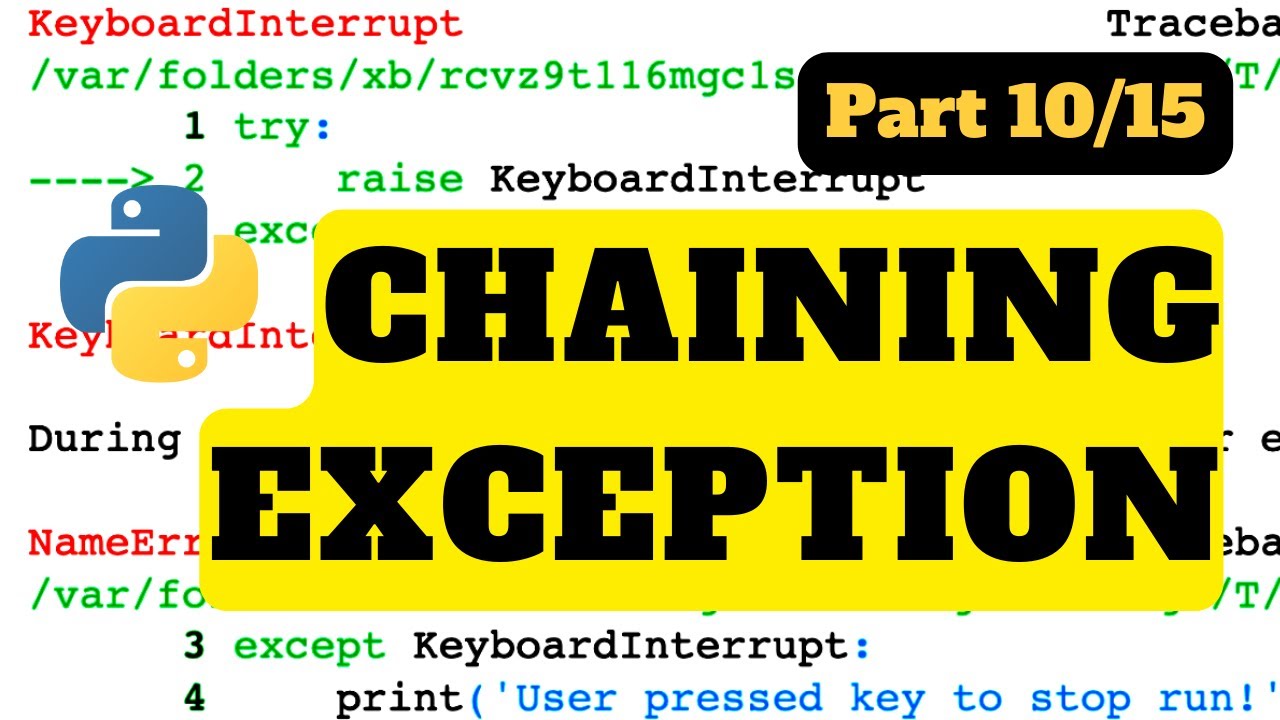

Article link: except exception as e.
Learn more about the topic except exception as e.
- Difference between except: and except Exception as e
- 8. Errors and Exceptions — Python 3.11.4 documentation
- Python except Exception as e
- Python: “except Exception as e” Meaning Explained!
- Try and Except Error handling Python Tutorial
- Python Exception Handling: Try, Except, Else and Finally
- Exception handling in Python (try, except, else, finally)
- Stop Using Exceptions Like This in Python – Jerry Ng’s blog
See more: https://nhanvietluanvan.com/luat-hoc/