Double Question Mark C#
In C#, the double question mark operator (??) is used for handling null values. It provides a concise and readable way to handle null references in a safe and efficient manner. The double question mark operator is part of the C# language and is widely used in various scenarios.
The Nullable Reference Types Feature
One of the major features introduced in C# 8.0 is the Nullable Reference Types. This feature allows developers to specify whether a particular reference type can be assigned null or not. By enabling nullable reference types, the compiler checks for null values at compile-time, reducing the chances of null reference exceptions at runtime.
The double question mark operator plays a crucial role in handling nullable reference types. It can be used to assign default values to variables when they are null. For example, consider the following code snippet:
“`csharp
string name = null;
string validName = name ?? “John Doe”;
“`
In the above code, the double question mark operator checks if the “name” variable is null. If it is null, the value “John Doe” is assigned to the “validName” variable. This ensures that even if the “name” variable is null, the “validName” variable will have a default value.
Conditional Access and Null Propagation Operators
The double question mark operator can also be combined with the conditional access operator (?). The conditional access operator is represented by a question mark followed by a period (?.). It is used to access members of an object only if the object is not null.
By combining the double question mark operator with the conditional access operator, developers can write cleaner and more concise code. Consider the following code snippet:
“`csharp
string? name = null;
int? nameLength = name?.Length ?? 0;
“`
In the above code, the conditional access operator checks if the “name” variable is null. If it is not null, it accesses the “Length” property of the “name” variable. If the “Length” property is also null, the double question mark operator assigns the value 0 to the “nameLength” variable.
Handling Null Coalescing with Double Question Marks
The null coalescing operator (??) is used to assign a default value to a variable if the original value is null. It is commonly used with the double question mark operator to handle null values effectively. Here’s an example:
“`csharp
string name = null;
string validName = name ?? GetNameFromDatabase();
“`
In the above code, if the “name” variable is null, the double question mark operator assigns the value returned by the “GetNameFromDatabase()” method to the “validName” variable. This ensures that the “validName” variable always has a valid non-null value.
Chaining Double Question Marks
Multiple double question marks can be chained together to handle nested null values. Consider the following example:
“`csharp
string? name = null;
string validName = name ?? GetEmployee()?.Department?.Manager?.Name ?? “Unknown”;
“`
In the above code, if the “name” variable is null or any of the nested properties are null, the double question mark operator assigns the value “Unknown” to the “validName” variable. This prevents null reference exceptions and provides a fallback value.
Potential Pitfalls and Best Practices with Double Question Marks
While the double question mark operator is a powerful feature in C#, it is important to understand its limitations and use it judiciously. Here are some best practices to keep in mind:
1. Avoid excessive chaining of double question marks as it can make the code less readable and harder to maintain.
2. Be cautious when using the double question mark operator with value types, as it can lead to unexpected behavior.
3. Ensure that your codebase has proper nullability annotations and enable the nullable reference types feature to take full advantage of the double question mark operator.
Compatibility and Language Versions
The double question mark operator was introduced in C# 2.0 and is supported in all subsequent versions of the C# language. However, the Nullable Reference Types feature was introduced in C# 8.0 and requires the corresponding language version to be enabled.
Summary and Further Resources
The double question mark operator is a powerful tool in C# for handling null values and providing default fallback values. It enables developers to write cleaner and more concise code, reducing the chances of null reference exceptions at runtime. By combining it with the conditional access and null coalescing operators, developers can handle nested null values effectively.
To learn more about the double question mark operator and other features of C#, you can refer to the official Microsoft documentation on C# language features. Additionally, there are numerous online tutorials and resources available for further exploration.
FAQs:
Q: What is the double question mark operator in C#?
A: The double question mark operator (??) is used in C# to handle null values and provide default fallback values.
Q: Does the double question mark operator require a specific language version?
A: The double question mark operator has been available since C# 2.0. However, the Nullable Reference Types feature, which is commonly used with the double question mark operator, requires C# 8.0 or newer.
Q: Can the double question mark operator be used with value types?
A: While the double question mark operator is primarily used with reference types, it can be used with nullable value types. However, caution should be exercised to avoid unexpected behavior.
Q: Are there any best practices for using the double question mark operator?
A: Some best practices include avoiding excessive chaining of double question marks, being cautious when using it with value types, and ensuring proper nullability annotations and enabled nullable reference types feature.
Q: Which versions of C# support the double question mark operator?
A: The double question mark operator has been available since C# 2.0 and is supported in all subsequent versions of the C# language.
C# Double Question Mark Operator
What Is The Double Question Mark In C?
The double question mark (??) is a lesser-known feature in the C programming language. It is known as the “double question mark operator” or the “digraph operator,” and its purpose is to provide a form of conditional compilation in C. This operator allows developers to select between two different values or expressions based on whether or not a specific feature is supported by the compiler.
Conditional compilation is a powerful technique that enables developers to include or exclude certain sections of code during the compilation process. This can be particularly useful when dealing with platform-specific code or when developing libraries that need to adapt to different environments.
The double question mark operator is unique to the C programming language and is not found in other programming languages like C++, Java, or Python. Its usage is relatively rare, but it can be handy in certain situations.
Syntax and Usage:
The syntax for the double question mark operator is as follows:
“`
“`
Here, `
It’s important to note that the double question mark operator works exclusively with predefined macros or symbols. For example, if a compiler supports a specific feature or symbol, it may define a macro to indicate its availability. The double question mark operator allows developers to conditionally include or exclude code based on the presence or absence of such macros.
Let’s consider an example to better understand the usage:
“`
#include
int main() {
#ifdef _WIN32
printf(“Running on Windows\n”);
#else
printf(“Not running on Windows\n”);
#endif
return 0;
}
“`
In this example, the `_WIN32` macro is a predefined macro that is typically defined by compilers when compiling for Microsoft Windows. The `#ifdef` directive checks if `_WIN32` is defined, and if it is, the code inside the corresponding `#ifdef` and `#endif` statements will be included during compilation. Otherwise, it’s excluded.
The double question mark operator can be a handy alternative to the `#ifdef` approach. Here’s how the above example can be modified to use the double question mark operator:
“`
#include
int main() {
printf(“Running on ” __has_include(
return 0;
}
“`
The `__has_include` macro is defined to evaluate whether a specific header file is available or not. It returns either 1 (true) or 0 (false) depending on the availability. In this case, the double question mark operator is used to conditionally print the appropriate message.
FAQs:
Q: Can the double question mark operator be used with non-predefined macros?
A: No, the double question mark operator only works with predefined macros or symbols that indicate feature availability.
Q: Is the double question mark operator widely used in practice?
A: No, it is not a commonly used feature in C. Most developers rely on `#ifdef` and other conditional compilation techniques instead.
Q: Are there any performance implications of using the double question mark operator?
A: No, the double question mark operator is resolved at compile-time and does not affect runtime performance.
Q: How can I check for the availability of a certain feature in C?
A: Typically, you should rely on predefined macros or symbols provided by the compiler to check for feature availability. The double question mark operator can be used in conjunction with these macros.
Q: Can the double question mark operator be used in C++?
A: No, the double question mark operator is a unique feature of the C programming language and is not available in C++.
In conclusion, the double question mark operator is an esoteric feature of the C programming language that allows for conditional compilation based on predefined macros or symbols. While it is not widely used in practice, it can be handy in certain situations where a more concise alternative to `#ifdef` is desired. However, developers should exercise caution and ensure that the macros used are defined by the compiler to avoid potential issues.
What Does A Double Question Mark Mean?
In the vast world of punctuation, the double question mark (?) is a lesser-known symbol. Often, we encounter single question marks to denote a question or inquiry. However, the use of a double question mark can lend an additional layer of significance to a sentence, adding emphasis, curiosity, or even incredulity. In this article, we will explore the meaning and usage of a double question mark, as well as provide some examples to clarify its purpose.
The use of a double question mark is not as standardized as other punctuation marks; it is considered more informal and less commonly used. As such, its meaning can vary depending on the context and the writer’s intention. Generally, a double question mark is used to denote a higher level of surprise, skepticism, or incredulity than what a single question mark would convey.
For instance, consider the sentence, “You really thought that would work??” In this case, the double question mark adds an element of disbelief or astonishment. It emphasizes the speaker’s incredulity at the other person’s misguided belief or action. Similarly, the double question mark can also underscore a sense of urgency or importance in a question. For example, “Are you seriously going to miss the deadline??”
The double question mark can also be used to indicate a rhetorical question. Rhetorical questions are posed for effect, often expecting no direct response. They are used to make a point or to emphasize a particular idea. The use of a double question mark adds extra emphasis to these rhetorical questions, making them more powerful and thought-provoking. Consider the sentence, “How can we expect to find happiness if we keep dwelling on negativity??” The double question mark intensifies the reflective and introspective nature of the question.
Additionally, the double question mark can be used to express heightened doubt or uncertainty. It can indicate that the speaker is truly puzzled or perplexed by the situation. For example, “You’re saying you didn’t know anything about it??”
FAQs:
Q: Can the double question mark be used interchangeably with a single question mark?
A: While the double question mark and the single question mark share the same purpose of indicating a question, the double question mark adds an extra layer of emphasis or incredulity beyond what a single question mark accomplishes. Therefore, they cannot be used interchangeably in all instances.
Q: Is the double question mark grammatically correct?
A: The double question mark is not a universally recognized punctuation mark, and its usage is considered informal. However, in informal contexts, such as casual writing or online communication, it can be used to emphasize strong feelings or incredulity.
Q: Does the double question mark have any other names?
A: The double question mark is sometimes referred to informally as a “doubt point” or even as an “incredulous question mark.” However, these terms are not widely recognized or formally accepted.
Q: Can the double question mark be used in formal writing?
A: Because the double question mark is considered informal, it is generally best to avoid using it in formal writing, such as academic essays, business correspondence, or professional documents. It is more commonly used in casual conversations, texts, or social media platforms.
Q: Are there any other punctuation marks that serve a similar purpose to the double question mark?
A: While the double question mark is the most commonly used symbol to convey heightened emphasis or incredulity, other punctuation marks, such as exclamation points or ellipses, can also be utilized to achieve similar effects. However, each of these punctuation marks conveys a distinct tone or emotion, and the choice may depend on the specific intention of the writer.
In conclusion, the double question mark is a rarely used punctuation mark that adds an extra layer of emphasis, incredulity, or urgency to a sentence. Its informal nature and versatility allow writers to convey surprise, skepticism, or rhetorical questions more vividly. While it may not have universal recognition or formal acceptance, it can be a useful tool in informal writing and digital communication to convey strong emotions or heighten the impact of a question.
Keywords searched by users: double question mark c# C# double question mark operator, C question mark, Double question mark java, C# question mark, What is double question mark in JavaScript, C# question mark after type, Operator, C# double quote in string
Categories: Top 74 Double Question Mark C#
See more here: nhanvietluanvan.com
C# Double Question Mark Operator
Introduction:
In the world of software development, programming languages play a crucial role in providing developers with powerful tools to create robust and efficient software solutions. One such language is C#, which is widely used by developers due to its versatility and extensive range of features. Among these features, the double question mark operator stands out as one of the most fascinating and useful operators in C#. In this article, we will delve into the depths of the C# double question mark operator, exploring its syntax, purpose, and practical examples, while also addressing frequently asked questions.
Understanding the Double Question Mark Operator:
The double question mark operator, also known as the null-coalescing operator, is represented by “??”. Its primary purpose is to simplify null value handling and conditional logic, a common challenge faced by developers. When used, this operator returns the value on its left-hand side if it is not null, otherwise, it returns the value on its right-hand side.
Syntax:
The syntax of the double question mark operator is straightforward and concise. It can be used in the following format:
variableName = value1 ?? value2;
In this syntax, value1 represents the left-hand side value, while value2 represents the right-hand side value. If value1 is not null, the operator will return value1. However, if value1 is null, the operator will return value2. This allows developers to replace lengthy if-else statements with a single line of code, resulting in cleaner and more concise code.
Practical Examples:
To better understand the application of the double question mark operator, let’s explore some practical examples.
Example 1:
int? number1 = null;
int number2 = 10;
int result = number1 ?? number2;
In this example, we declare a nullable integer variable called “number1” and assign null to it. We then assign the integer value “10” to “number2”. Using the double question mark operator, we assign the value of number1 to the “result” variable. Since number1 is null, the operator returns the value on the right-hand side, which is “10”. Therefore, the “result” variable will have a value of “10”.
Example 2:
string name = null;
string defaultName = “John Doe”;
string result = name ?? defaultName;
In this example, we declare a string variable called “name” and assign null to it. We also declare another string variable named “defaultName” and assign the value “John Doe” to it. Using the double question mark operator, we assign the value of name to the “result” variable. Since name is null, the operator returns the value on the right-hand side, which is “John Doe”. Therefore, the “result” variable will have a value of “John Doe”.
Benefits of the Double Question Mark Operator:
The double question mark operator offers several benefits to developers, including:
1. Improved code readability: By replacing lengthy if-else statements with the double question mark operator, developers can simplify their code and improve its readability.
2. Streamlined null value handling: The operator provides a concise way to handle null values, reducing the complexity of null checks in code.
3. Increased code efficiency: The operator helps to write efficient code by avoiding unnecessary conditional logic, resulting in faster execution.
FAQs:
Q1. Can the double question mark operator be used with any data type?
Yes, the double question mark operator can be used with any nullable data type, such as integer, string, DateTime, etc. It simplifies null handling across various data types.
Q2. Can the double question mark operator be used in nested operations?
Yes, the double question mark operator can be used in nested operations to handle multiple null values. However, it is important to ensure clarity and maintainability when using complex nested operations.
Q3. What happens when both the left-hand side and the right-hand side values are null?
If both the left-hand side and the right-hand side values are null, the operator will still return null.
Q4. Can the double question mark operator be used with custom types?
No, the double question mark operator cannot be used directly with custom types. However, custom types can be made nullable by using the “?” modifier, allowing the operator to be used.
Conclusion:
The C# double question mark operator is a powerful tool that simplifies null value handling and conditional logic in code. Its concise syntax and ability to handle different data types make it one of the most valuable operators in the C# language. By leveraging this operator, developers can write cleaner, more readable, and more efficient code. So, next time you encounter a null value challenge in your C# development, consider using the double question mark operator to streamline your code and enhance its performance.
C Question Mark
In the world of programming languages, the C language stands out as one of the most influential and widely used. Known for its simplicity and efficiency, C provides programmers with the tools to develop powerful and versatile applications. However, within the realm of C, there are several features and quirks that may puzzle even seasoned developers. One such intriguing feature is the C question mark.
The C question mark, denoted as “?”, is an operator that, when used in conjunction with the colon, forms what is known as the conditional operator or ternary operator. This unique operator allows developers to write concise and expressive code, enhancing readability and conciseness. In this article, we will explore the C question mark in depth, discussing its syntax, applications, and addressing frequently asked questions to provide a comprehensive understanding of this powerful operator.
Syntax:
The conditional operator follows a specific syntax pattern:
result = condition ? expression1 : expression2;
Here, “condition” is an expression that evaluates to either true or false. If the condition evaluates to true, the value of “expression1” is assigned to “result.” Conversely, if the condition evaluates to false, the value of “expression2” is assigned to “result.” This ternary operator condenses the functionality of a simple if-else statement into a single line of code.
Applications:
1. Conditional Assignments:
The conditional operator allows for quick and elegant conditional assignments. For example, consider the following code:
maximum = (a > b) ? a : b;
In this code snippet, if the value of “a” is greater than “b,” the maximum is assigned to “a”; otherwise, it is assigned to “b.” This avoids the need for an explicit if-else statement, making the code more concise and readable.
2. Replacements for Short if-else Statements:
The conditional operator is often used as a replacement for simple if-else statements. For instance:
grade = (score >= 80) ? ‘A’ : ‘B’;
Here, if the value of “score” is greater than or equal to 80, the grade is assigned ‘A’; otherwise, it is assigned ‘B’. This usage simplifies the code and improves its readability.
3. Inline Conditions:
The conditional operator is also useful in cases where a condition needs to be evaluated in-place, without the need for a separate variable assignment. For example:
printf(“The number is %s”, (number % 2 == 0) ? “even” : “odd”);
In this code, the condition checks whether the number is even or odd, and based on the result, the corresponding text is printed. The conditional operator allows for concise and efficient decision making within a single line of code.
Frequently Asked Questions:
Q1: Can the conditional operator be nested?
Yes, the conditional operator can be nested within each other to handle multiple conditions. However, excessive nesting may reduce code readability and should be avoided whenever possible.
Q2: Are there any limitations to the expressions used in the conditional operator?
No, the expressions used in the conditional operator can be of any valid C expression type. These can include literals, variables, arithmetic expressions, or even function calls.
Q3: Are there any performance considerations for using the conditional operator?
While the conditional operator offers an expressive and concise way to write code, it is essential to keep in mind that excessive use of this operator can negatively impact code performance. Complex expressions within the conditional operator can make code harder to read and maintain, and they might also hinder optimizations performed by the compiler. Therefore, it is recommended to use the conditional operator judiciously and prioritize code readability and maintainability.
Q4: Can the conditional operator be used without the second expression?
No, the conditional operator requires both expression1 and expression2 to be present. If either expression is omitted, a syntax error would occur.
In conclusion, the C question mark, or the conditional operator, is a powerful tool for concise and expressive coding in the C language. By leveraging this operator, developers can write code that is not only efficient but also readable. However, it is crucial to strike a balance between code brevity and code maintainability. The conditional operator should be used judiciously, keeping in mind the potential impact on code readability and performance.
Images related to the topic double question mark c#
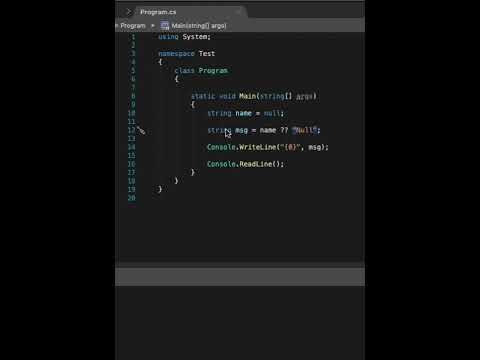
Article link: double question mark c#.
Learn more about the topic double question mark c#.
- What do two question marks together mean in C#? – Stack …
- The Double Question mark in C# — ?? | Geek Culture – Medium
- What does the two question marks together () mean in C
- How are double question marks used in a sentence? – Vedantu
- C/C++ data types, basic operators, and control structures
- How To Use The Double Question Mark Operator In TypeScript?
- C# Double Question Mark Operator (??) – Josip Miskovic
- The c# double question mark operator – Chubby Developer
- What does the two question marks together () mean in C
- C# Double Question Mark – Linux Hint
- C# Double Question Mark Operator – Null-Coalescing …
- and ??= operators – null-coalescing operators – Microsoft Learn
- How To Use The Double Question Mark Operator In TypeScript?