Document Body Contenteditable True Document Designmode On Void 0
The Document Object Model (DOM) is a programming interface for HTML, XML, and SVG documents. It represents the page so that programs can change the document structure, style, and content. The DOM is crucial for dynamic web development as it allows developers to interact with and modify the elements and contents of a web page.
What is the Document Object Model (DOM)?
The Document Object Model (DOM) is a model representation of an HTML or XML document. It defines the structure of the document and provides methods and properties to access, manipulate, and update its elements. The DOM treats an HTML or XML document as a tree structure, where each element is a node in the tree with parent-child relationships.
Understanding the structure of the DOM
The DOM structure is based on the hierarchical arrangement of HTML or XML elements. Each element is represented by a node in the DOM tree. The DOM structure includes the root node, which represents the entire document, and various child nodes representing different elements in the document. These nodes can also have attributes, such as id, class, or style, which further define their properties.
How does the DOM represent an HTML document?
The DOM represents an HTML document as a structured tree of elements. The root of the tree is the document object itself, which represents the entire document. The document object contains properties and methods to access and manipulate the document’s elements, attributes, and content. Each element in the HTML document is represented by its own node in the DOM tree, with parent-child relationships linking them together.
Introduction to document.body
What is the document.body property?
The document.body property is a reference to the body element of an HTML document. It represents the main content area of the document, where most of the visible content is displayed. The document.body property provides access to the body element’s properties, attributes, and methods, allowing developers to manipulate its contents dynamically.
Accessing and manipulating the body element using JavaScript
To access and manipulate the body element using JavaScript, you can use the document.body property. For example, you can change the background color of the body element by setting its style property:
“`
document.body.style.backgroundColor = ‘red’;
“`
You can also add or modify HTML content within the body element using the innerHTML property:
“`
document.body.innerHTML = ‘
Hello, World!
‘;
“`
Use cases of document.body
The document.body property is commonly used in JavaScript to dynamically modify the content and appearance of a web page. Some use cases of document.body include:
1. Changing the background or text color of the body element.
2. Appending or removing elements within the body element.
3. Dynamically updating the content of the body element based on user interactions.
4. Adding event listeners to the body element for capturing user actions.
Understanding contenteditable attribute
Exploring the contenteditable attribute and its purpose
The contenteditable attribute is an HTML attribute that allows the user to edit the content of an element directly in the browser. When applied to an element, such as a div or a paragraph, it enables the element to behave like a rich-text editor, allowing users to make changes to the text, apply formatting, and even add or remove elements.
Enabling editing within HTML elements
To enable editing within HTML elements, you can add the contenteditable attribute to the desired element:
“`
“`
With the contenteditable attribute set to “true,” the div element becomes editable, and the user can modify its content directly in the browser.
Controlling the behavior of contenteditable elements
Contenteditable elements can have different behaviors based on their attribute values. The attribute values can be:
– “true”: The element is editable, and the user can modify its content.
– “false”: The element is not editable, and the user cannot modify its content.
– “inherit”: The element inherits the editability from its parent element.
Additionally, contenteditable elements can have different levels of editability, allowing developers to define which parts of the content are editable or read-only.
Exploring the designMode property
Introduction to the designMode property
The designMode property is a feature of the DOM that allows the entire document to be put into an editable mode. When the designMode property is enabled, the entire document becomes editable, providing a similar editing experience as contenteditable elements but on a global scale.
Enabling the design mode to edit the entire document
To enable the designMode property and make the whole document editable, you can use the following JavaScript code:
“`
document.designMode = ‘on’;
“`
Once the designMode property is set to “on,” the entire document becomes editable, and the user can modify any part of the content directly.
Limitations and considerations of using designMode
While designMode offers a powerful way to enable editing on the entire document, there are some limitations and considerations to keep in mind:
1. Browser compatibility: Not all browsers support the designMode property. It’s essential to check the browser compatibility before relying on this feature.
2. Security concerns: Enabling designMode gives users full control over the content of the document, which can pose security risks in certain scenarios. It’s crucial to validate and sanitize user-generated content before saving it to prevent potential security vulnerabilities.
3. Performance impact: Enabling designMode can have a performance impact, especially on complex documents or pages with a large amount of content. It’s important to optimize the code and minimize unnecessary editing operations to maintain good performance.
Leveraging document.designMode vs. contenteditable
Comparing document.designMode and contenteditable in terms of usage
Both document.designMode and contenteditable provide ways to make web content editable. However, they differ in terms of their scope and application.
– document.designMode enables editing on the entire document, allowing global changes to the content.
– contenteditable applies to specific HTML elements, giving more control over which parts of the content are editable.
Benefits and drawbacks of each approach
The benefits of using document.designMode include:
1. Global editing: It allows users to edit the entire document, making it suitable for scenarios where comprehensive content editing is required.
2. Uniform experience: Enabling designMode provides a consistent editing experience across different parts of the document.
The benefits of using contenteditable include:
1. Granular control: With contenteditable, developers can define which specific elements are editable, allowing for more precise control over the editing capabilities.
2. Flexibility: contenteditable can be applied to different elements, making it suitable for various scenarios, such as inline editing or creating custom text editors within specific sections of a page.
The drawbacks of using both approaches include:
1. Browser compatibility: Both document.designMode and contenteditable may not be supported in all browsers, requiring fallback options or alternative solutions.
2. Security concerns: Allowing users to edit the content of a web page can introduce potential security risks, and proper content validation and sanitization are crucial to prevent attacks.
Determining the appropriate choice for specific scenarios
The choice between document.designMode and contenteditable depends on the specific requirements and goals of the project. If global editing on the entire document is desired, document.designMode may be the appropriate choice. On the other hand, if more granular control over editing capabilities is needed, contenteditable may be the better option.
A practical example: Making a text editor
Step-by-step guide to creating a simple text editor using document.body and contenteditable
1. Create an HTML file with a textarea element and a button:
“`html
“`
2. Add JavaScript code to handle the toggle button:
“`javascript
let editorTextarea = document.getElementById(‘editorTextarea’);
let toggleEditorBtn = document.getElementById(‘toggleEditorBtn’);
toggleEditorBtn.addEventListener(‘click’, function() {
if (editorTextarea.style.display === ‘none’) {
editorTextarea.style.display = ‘block’;
document.body.contentEditable = ‘true’;
} else {
editorTextarea.style.display = ‘none’;
document.body.contentEditable = ‘false’;
}
});
“`
3. Style the textarea and button as desired using CSS.
Implementing basic editing functionalities
To implement basic editing functionalities, you can use event listeners and manipulate the content of the editorTextarea or document.body based on user actions. For example, you can listen for a keydown event to capture user input and update the content accordingly.
Enhancing the editor with additional features
To enhance the editor with additional features, you can extend the JavaScript code to handle various events and implement specific functionalities. For example, you can add options for text formatting, insert images, or even integrate with external libraries or APIs to provide a more comprehensive editing experience.
Browser compatibility and considerations
Understanding browser support for document.body, contenteditable, and designMode
The browser support for document.body, contenteditable, and designMode may vary. It’s essential to verify the compatibility of these features across different browsers before implementing them. Popular modern browsers, such as Chrome, Firefox, Safari, and Edge, generally support these features, but it’s important to check for any limitations or specific behavior differences.
Handling cross-browser issues and fallbacks
To handle cross-browser issues and ensure consistent behavior, it’s recommended to test the implementation on different browsers and versions. You can use feature detection techniques to determine if the browser supports document.body, contenteditable, or designMode and provide fallback options or alternative approaches for unsupported browsers.
Best practices for ensuring consistent behavior across browsers
To ensure consistent behavior across browsers, follow these best practices:
1. Use feature detection: Instead of relying on browser-specific checks, use feature detection techniques to determine if a certain functionality is supported before applying it. This ensures that unsupported features are gracefully handled.
2. Test on multiple browsers: Test your implementation on popular browsers to identify any compatibility issues or unexpected behavior.
3. Update browser compatibility information: Regularly check for updates on browser compatibility for the features you are using and update your code or fallback options accordingly.
By following these best practices, you can create a more robust and reliable implementation that works consistently across different browsers.
How To Use Javascript:Document.Contenteditable=’True Document.Designmode=’On’ Void 0
Keywords searched by users: document body contenteditable true document designmode on void 0 javascript:document.body.contenteditable=’true’; document.designmode=’on’; void 0, javascript.document.body.contenteditable =’true’ document.designmode = ‘on’, https://m.facebook.com javascript:document.body.contenteditable =’true’; documen.designmode = ‘on’;, Javascript document body contenteditable false document designmode off void 0, F12 trên điện thoại, JavaScript chỉnh sửa trang web, Code F12, F12 trên điện thoại iPhone
Categories: Top 30 Document Body Contenteditable True Document Designmode On Void 0
See more here: nhanvietluanvan.com
Javascript:Document.Body.Contenteditable=’True’; Document.Designmode=’On’; Void 0
JavaScript is a powerful programming language that allows developers to enhance the functionality and interactivity of websites. One interesting feature of JavaScript is the ability to make elements of a webpage editable, enabling users to modify the content directly on the page. In this article, we will explore the JavaScript code: document.body.contenteditable=’true’; document.designmode=’on’; void 0, and discuss its applications and potential use cases.
What does document.body.contenteditable=’true’; document.designmode=’on’; void 0 do?
The code snippet document.body.contenteditable=’true’; document.designmode=’on’; void 0 sets the contenteditable attribute of the body element to true, and enables design mode for the entire document.
The contenteditable attribute allows users to directly edit the content of an HTML element, such as a text paragraph or a heading, by simply clicking on that element and modifying the text. When applied to the body element, it allows users to edit the entire page’s content.
On the other hand, design mode is a feature that makes the entire document editable, including not only the text but also other HTML elements, such as images, tables, or forms. It provides a more comprehensive editing environment where users can modify various aspects of a webpage.
By combining contenteditable=’true’ and design mode, users gain the ability to make changes to the webpage’s content as if they were using a basic text editor. This feature can be particularly useful when developing and testing webpages or when building web-based applications that require live content modification.
Applications and Use Cases:
1. Content Creation and Editing: The primary application of this JavaScript code is in content creation and editing systems. Developers can integrate it into their CMS (Content Management System) to facilitate easy content editing for end-users. It provides a simplified and intuitive interface that saves users from the hassle of navigating complex editing environments.
2. Collaborative Editing: With this feature, multiple users can collaborate and edit a webpage simultaneously. Think of a situation where a team is working on a shared document and all members need to have real-time editing capabilities. This JavaScript snippet enables a simple yet effective way for teams to work together in a live editing environment.
3. Prototyping and Development: Designers and developers often need to quickly test and tweak the layout and content of a webpage during the prototyping phase. The ability to edit the content of a page directly on the browser eliminates the need for a dedicated text editor or an external tool. JavaScript code like document.designMode offers an easy solution for rapid prototyping.
4. User Feedback and Reviews: By integrating this JavaScript code into feedback or review forms, users can provide direct comments on a webpage’s content. This eliminates the need for users to copy, paste, or take screenshots of the page, streamlining the feedback process.
5. HTML Editing and Debugging: When developing web applications, it can be helpful to examine and edit the HTML structure of a page in real-time to spot issues or improve elements. The contenteditable attribute combined with design mode allows developers to quickly assess and modify the HTML, helping them identify and rectify problems more efficiently.
FAQs:
Q: Can this JavaScript code be used for malicious purposes?
A: Although this code snippet provides powerful editing capabilities, it can be turned off by the end-user simply by refreshing the page or closing the browser tab. It does not permanently alter the webpage or impact the server. However, caution should be exercised when embedding this code on public websites, as it may enable unauthorized modifications if applied without proper security measures.
Q: Is this feature supported by all web browsers?
A: Most modern web browsers support the contenteditable and design mode features. However, it is always a good practice to test the functionality across different browsers to ensure cross-compatibility.
Q: Can this code be applied to specific HTML elements instead of the body element?
A: Absolutely! The contenteditable attribute can be applied to any HTML element, allowing users to edit specific sections of a webpage instead of the entire body. It provides a more tailored editing experience based on the specific requirements of the application.
In summary, the JavaScript code document.body.contenteditable=’true’; document.designmode=’on’; void 0 opens up a world of possibilities for content editing, collaboration, prototyping, and debugging. It provides a simple yet effective way to make webpages editable directly on the browser. When used responsibly, this feature offers an enhanced user experience and unlocks new creative solutions for content creation and web development.
Javascript.Document.Body.Contenteditable =’True’ Document.Designmode = ‘On’
#### Understanding `document.body.contenteditable =’true’`:
The `document.body.contenteditable` property allows us to make the entire content within the body of the webpage editable. By setting this property to `’true’`, all the elements present within the `
` tag become editable, including text, images, and even the layout.This capability proves to be extremely useful for creating web-based applications such as content management systems, collaborative editing platforms, or any platform where users need to modify the content directly on the webpage.
#### Understanding `document.designmode = ‘on’`:
While the `document.body.contenteditable =’true’` property allows users to edit the content, the `document.designmode = ‘on’` command takes it a step further, enabling users to manipulate the design aspects of the webpage as well. With this property, users can edit not just the text but also the layout, styles, and formatting of the content.
With `document.designmode = ‘on’`, the browser turns into a rich text editor, providing users an interactive environment to tweak and customize the webpage according to their preferences. This feature finds its application in web development, as it allows developers to make live changes to the design during the development process.
#### Benefits of using these JavaScript commands:
1. **Enhanced User Experience:** Allowing users to edit content directly on a webpage boosts interactivity and engagement, leading to an enhanced user experience. This feature eliminates the need for users to switch between multiple interfaces, making the editing process smooth and seamless.
2. **Efficiency and Collaboration:** With the ability to edit the content directly on a webpage, users can make changes quickly and efficiently. This feature is particularly beneficial for collaborative work, as it enables users to work on the same document simultaneously, reducing the time consumed in sending and receiving multiple versions of the document.
3. **Simplified Development Process:** Developers can take advantage of `document.body.contenteditable =’true’` and `document.designmode =’on’` to build web-based applications that offer live content editing or customizable interfaces. This saves time and effort, as developers can instantly preview and evaluate their changes directly in the browser without needing to deploy the website repeatedly.
4. **Promote Accessibility:** Enabling users to edit content and design aspects directly within a webpage can be particularly helpful for individuals with physical or cognitive impairments. It simplifies the editing process, allowing a wider range of users to engage with and contribute to web content.
#### FAQs:
**Q1. Can any webpage be edited using `document.body.contenteditable =’true’`?**
The ability to edit a webpage’s content using `document.body.contenteditable =’true’` depends on the webpage’s implementation. By default, most websites do not allow editing of their content. However, it is possible to build web applications or components with editable content areas by implementing this feature.
**Q2. Is it safe to use `document.body.contenteditable =’true’` and `document.designmode =’on’?**
It is generally safe to use these JavaScript commands. However, since users can modify the content and design of a webpage when these properties are enabled, caution should be exercised to prevent unauthorized or malicious modifications. Implementing proper security measures, such as restricting editing rights or incorporating user authentication, can help mitigate the risk.
**Q3. Can the changes made using `document.body.contenteditable =’true’` and `document.designmode =’on’` be saved?**
These JavaScript commands do not inherently provide the capability to save user modifications. However, developers can incorporate additional functionality to save changes, such as using backend technologies like databases, APIs, or local storage to persist the modifications made by users.
**Q4. Can these JavaScript commands be used on mobile devices?**
Yes, both `document.body.contenteditable =’true’` and `document.designmode =’on’` are supported on mobile devices that have JavaScript capabilities. Mobile browsers provide touch-based editing features to allow users to interact with editable web content efficiently.
In conclusion, using `document.body.contenteditable =’true’` and `document.designmode =’on’` in JavaScript empowers users to directly edit content and design aspects on a webpage. This functionality not only enhances the user experience but also promotes collaboration and makes the development process more efficient. However, it is crucial to implement appropriate security measures and additional functionality to ensure the safe and effective usage of these commands.
Images related to the topic document body contenteditable true document designmode on void 0
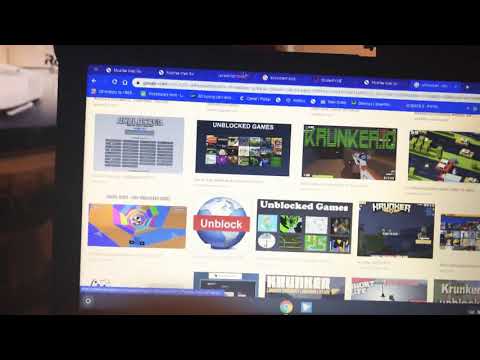
Found 6 images related to document body contenteditable true document designmode on void 0 theme
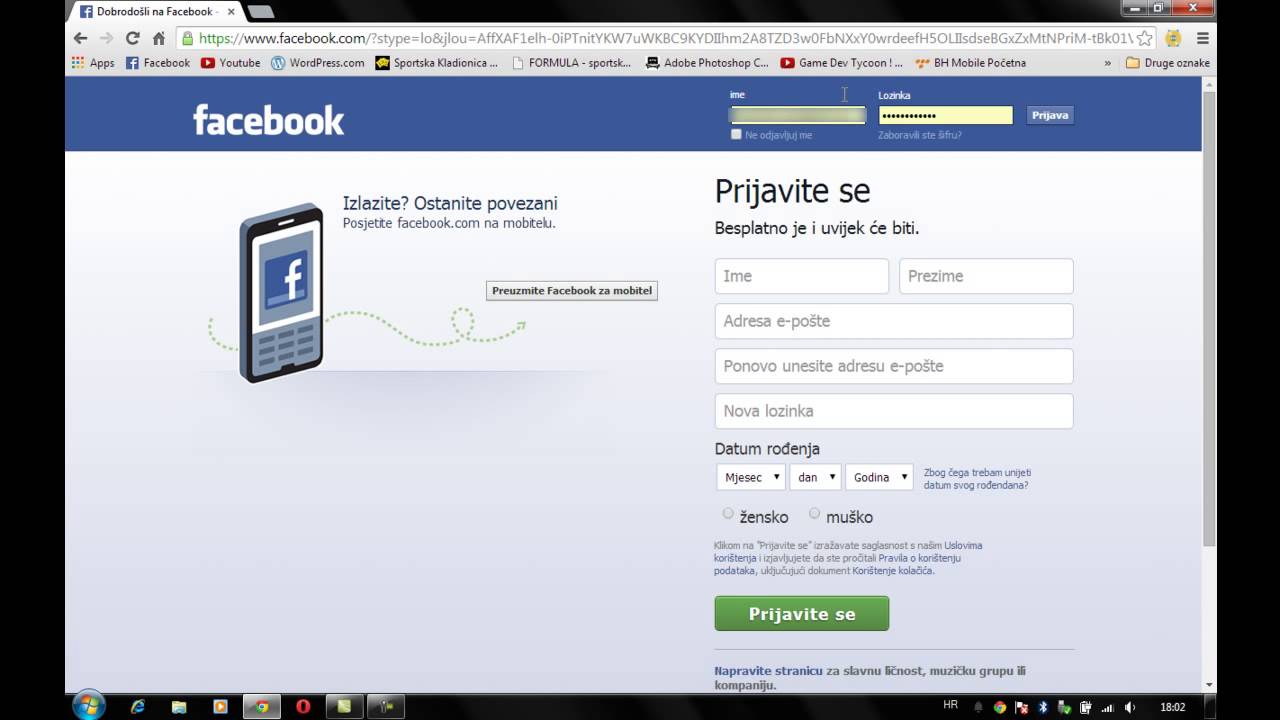


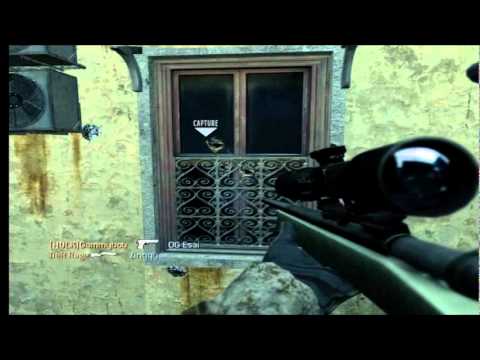
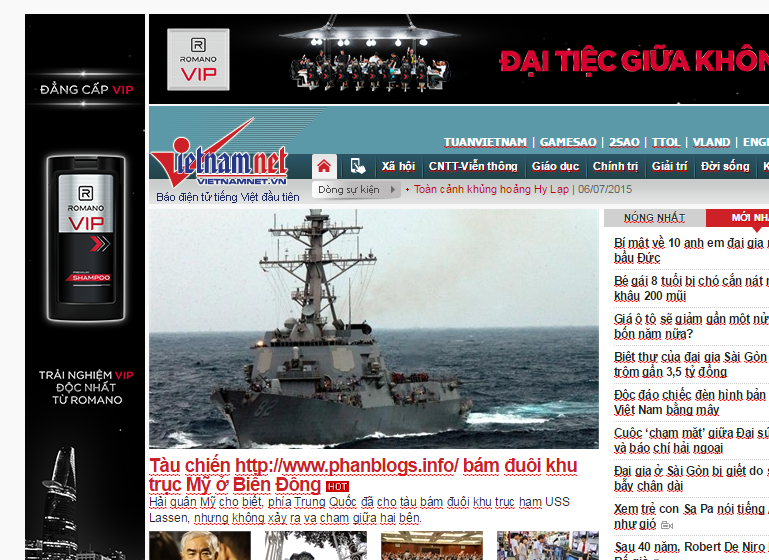

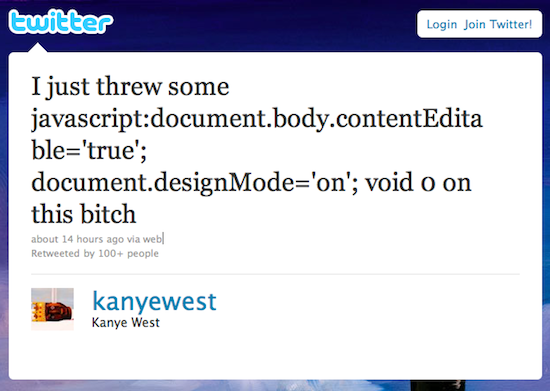
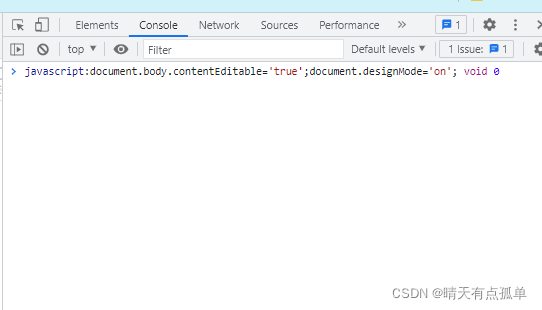
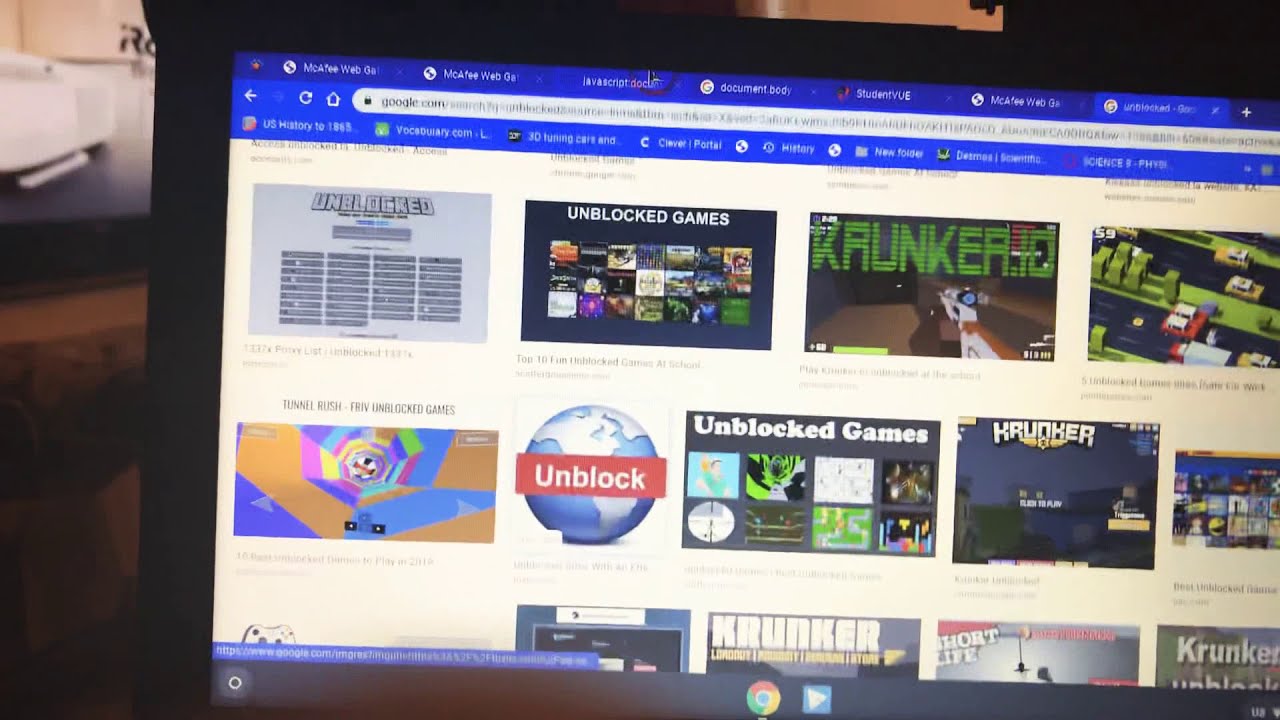
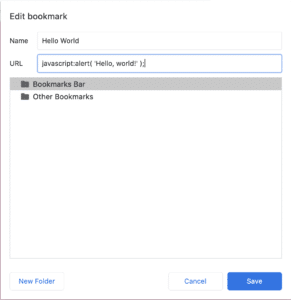
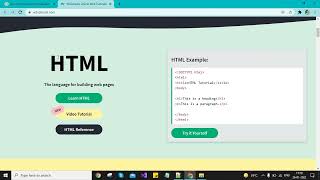
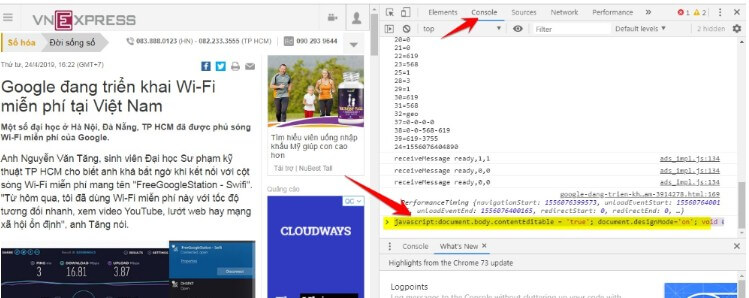
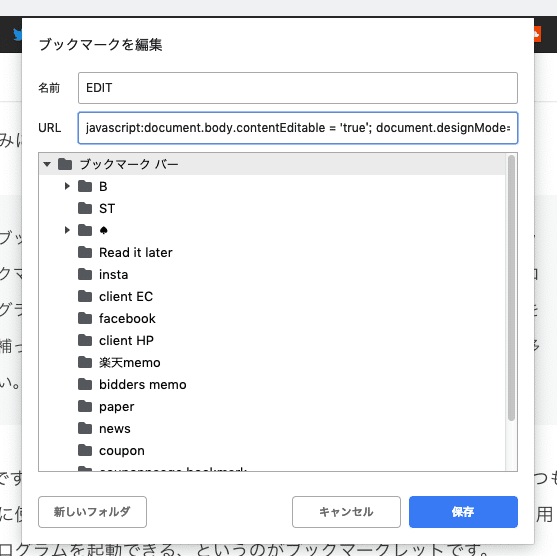
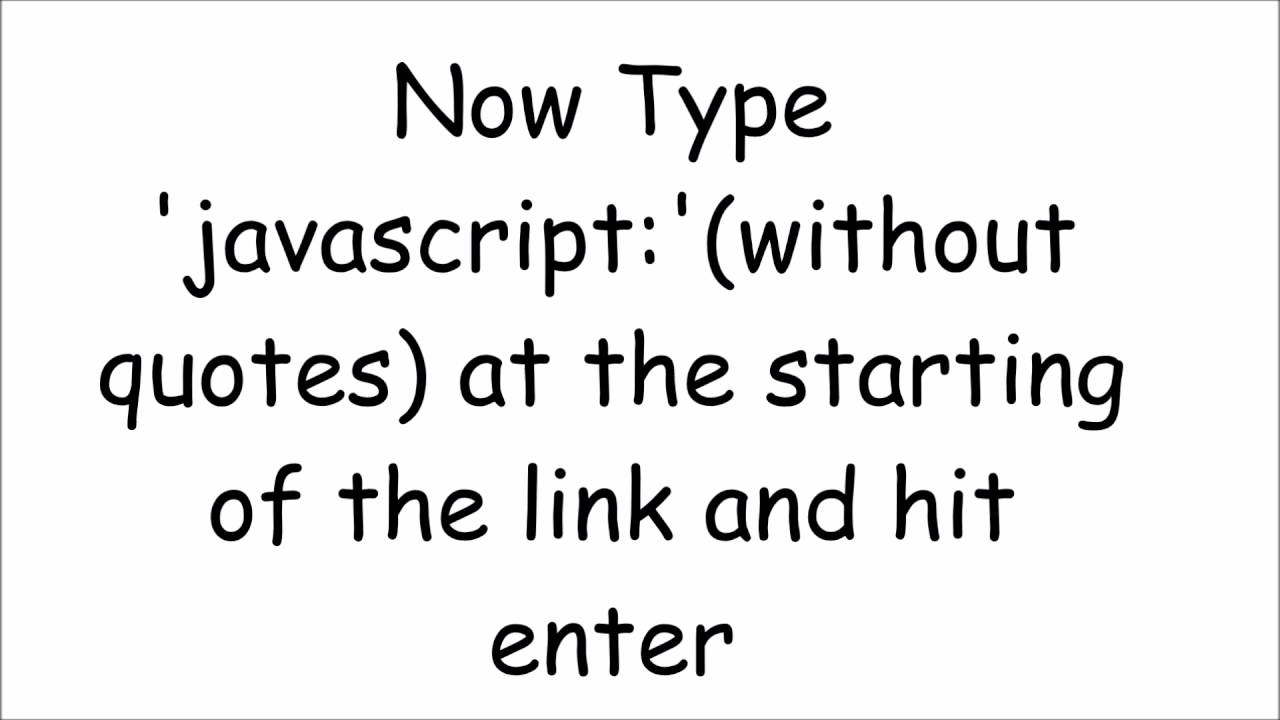
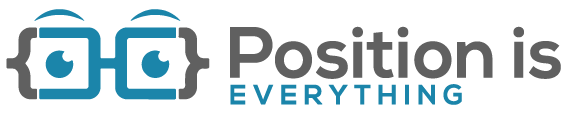


Article link: document body contenteditable true document designmode on void 0.
Learn more about the topic document body contenteditable true document designmode on void 0.
- thủ thuật với javascript để chỉnh sửa các trang web như fac và …
- Chỉnh sửa văn bản trực tiếp trong trang web
- web – javascript:document.body.contentEditable=’true …
- javascript:document.body.contentEditable=’true’ – void 0 – Quizlet
- document.body.contenteditable=’true’; document.designmode …
- Document.body.contenteditable = ‘true’ – Eyelight.vn
- JavaScript trick to EDIT any website in a Browser
- Document.designmode=’On’; Void 0 – Position Is Everything
- Edit any website like it’s a word doc, directly from your browser
- Các bác cho em hỏi về javascrip [Archive] – Diễn Đàn Tin Học
See more: nhanvietluanvan.com/luat-hoc