Do Not Access Object.Prototype Method ‘Hasownproperty’ From Target Object
In JavaScript, the “hasOwnProperty” method is used to determine whether an object has a specific property of its own. This method is inherited from the Object.prototype and returns a boolean value indicating whether the object has the specified property.
The purpose and usage of the “hasOwnProperty” method in JavaScript
The “hasOwnProperty” method is commonly used when iterating over the properties of an object to ensure that only the object’s own properties are considered. This is important because when you iterate over an object using a “for…in” loop, it not only iterates over the object’s own properties but also over any properties inherited from its prototype chain.
By using the “hasOwnProperty” method, you can avoid unintended consequences or conflicts that may arise from iterating over inherited properties. It allows you to check if a property is directly defined on the object itself, rather than being inherited from its prototype.
Beware of accessing “hasOwnProperty” through the Object.prototype
An important point to remember is that it is generally not recommended to access the “hasOwnProperty” method directly from an object’s prototype, i.e., Object.prototype.hasOwnProperty. Accessing “hasOwnProperty” from the Object.prototype can lead to unexpected behavior and potential pitfalls.
The potential pitfalls of accessing “hasOwnProperty” from the target object
When an object’s prototype chain is modified, accessing “hasOwnProperty” from the target object can produce incorrect results. This is because the method may be overridden or modified higher up the prototype chain.
Accessing “hasOwnProperty” directly on the target object also creates the risk of collision if an object property has the same name as the “hasOwnProperty” method. This can lead to errors or unexpected behavior.
Avoiding conflicts and unintended consequences when using “hasOwnProperty”
To avoid conflicts and unintended consequences when using the “hasOwnProperty” method, it is recommended to wrap the method call in a conditional statement or use the “in” operator instead. The “in” operator checks if a property exists anywhere in the prototype chain, including the object’s own properties.
Alternative approaches for checking object property existence
In addition to using the “hasOwnProperty” method or the “in” operator, there are other approaches to check for object property existence. One popular alternative is to use the “Object.keys()” method, which returns an array of an object’s own enumerable property names. By checking if the desired property exists in the array, you can determine its existence.
Another approach is to use the “Object.getOwnPropertyNames()” method, which returns an array of an object’s own property names, regardless of their enumerability. This method can be useful when you need to check for non-enumerable properties as well.
Best practices for property checking without using “hasOwnProperty”
To ensure robust and error-free property checking, it is recommended to follow these best practices:
1. Use the “hasOwnProperty” method or the “in” operator when iterating over an object’s properties, to differentiate between own and inherited properties.
2. Avoid accessing “hasOwnProperty” directly from Object.prototype to mitigate potential pitfalls and conflicts.
3. Use alternative methods like “Object.keys()” or “Object.getOwnPropertyNames()” for checking property existence in a more flexible way.
4. When working with third-party libraries or frameworks, review their documentation to understand their recommended practices for object property checking.
Conclusion: A cautionary reminder of the implications of using “hasOwnProperty” from object.prototype
In conclusion, while the “hasOwnProperty” method is a useful tool for checking an object’s own properties, it is important to exercise caution when accessing it from the Object.prototype. By understanding the potential pitfalls and alternative approaches for property checking, developers can avoid conflicts, unintended consequences, and ensure more reliable code.
FAQs:
Q1: What happens if I access “hasOwnProperty” from the Object.prototype?
Accessing “hasOwnProperty” directly from the Object.prototype can lead to unexpected behavior and potential pitfalls. Modifications to the prototype chain can affect the behavior of the method, and there is a risk of collision if an object property has the same name as the method.
Q2: Why should I use the “hasOwnProperty” method?
The “hasOwnProperty” method is often used when iterating over object properties to ensure that only the object’s own properties are considered. It helps differentiate between own and inherited properties, avoiding conflicts and unintended consequences.
Q3: Are there any alternative methods for checking property existence?
Yes, there are alternative methods like “Object.keys()” and “Object.getOwnPropertyNames()” that can be used to check for property existence. These methods provide more flexibility and can handle non-enumerable properties as well.
Q4: What are the best practices for property checking without using “hasOwnProperty”?
To ensure robust property checking, it is recommended to use the “hasOwnProperty” method or the “in” operator when iterating over properties. Additionally, using alternative methods like “Object.keys()” or “Object.getOwnPropertyNames()” can provide more flexibility in property checking. Always refer to the documentation of third-party libraries or frameworks for recommended practices.
Newer Object Hasown Method
Keywords searched by users: do not access object.prototype method ‘hasownproperty’ from target object Check object js, Property hasOwn does not exist on type ‘ObjectConstructor, Check exist in object js, Check property in object JavaScript, Property does not exist on type, hasOwnProperty trong JavaScript, Object has key js, Module’ is not defined eslintno undef
Categories: Top 54 Do Not Access Object.Prototype Method ‘Hasownproperty’ From Target Object
See more here: nhanvietluanvan.com
Check Object Js
In the world of JavaScript programming, the Check object plays a crucial role in facilitating data validation, improving application performance, and ensuring code reliability. This powerful utility allows developers to easily validate input data and check the presence, type, and content of values within their JavaScript programs. In this article, we will delve into the intricacies of the Check object in JS, discussing its features, usage, and exploring some frequently asked questions about this fundamental JavaScript tool.
Features of Check Object JS
The Check object offers a variety of features that enhance application development and improve code reliability. Let’s take a closer look at some of its most important features:
1. Type Checking: The Check object allows developers to perform type checks on variables, ensuring that the expected data type is being used. This feature helps catch potential bugs and ensures data consistency within the program.
2. Value Presence Check: Through the Check object, it is easy to determine whether a value is present or if it is undefined, null, or empty. This capability helps developers handle potential errors or exceptions and prevents unexpected behavior in the program.
3. Regular Expression Validation: The Check object also provides an efficient way to validate data using regular expressions. With its RegExp method, developers can easily verify whether the input matches the desired pattern.
4. Data Consistency: By utilizing the Check object, developers can enforce data consistency throughout their programs. For example, they can ensure that all strings are written in a specific format or that dates adhere to a particular structure.
Using the Check Object JS
Now, let’s dive into some practical examples to better understand how to employ the Check object in JS:
1. Type Check:
“`javascript
Check.isNumber(42); // Returns true
Check.isString(“Hello”); // Returns true
Check.isObject({}); // Returns true
Check.isArray([]); // Returns true
“`
2. Value Presence Check:
“`javascript
Check.isDefined(42); // Returns true
Check.isNull(null); // Returns true
Check.isEmpty(“”); // Returns true
“`
3. Regular Expression Validation:
“`javascript
Check.matchRegExp(“Hello, World!”, /^Hello/i); // Returns true
Check.matchRegExp(“12345”, /^\d+$/); // Returns true
“`
4. Data Consistency:
“`javascript
function formatName(firstName, lastName) {
if (!Check.isString(firstName) || !Check.isString(lastName)) {
throw new Error(“Invalid input”);
}
return `${Check.capitalize(firstName)} ${Check.capitalize(lastName)}`;
}
formatName(“john”, “doe”); // Returns “John Doe”
“`
FAQs about Check Object JS
Q1. What is the Check object used for in JavaScript?
The Check object in JavaScript is used for data validation, type checking, value presence verification, regular expression evaluation, and ensuring data consistency within programs.
Q2. Can I use the Check object in all JavaScript environments?
Yes, the Check object is compatible with all environments that support JavaScript, including web browsers, server-side environments, and Node.js.
Q3. How can I include the Check object in my JavaScript project?
You can include the Check object by importing it from a dedicated utility library like Lodash or by writing your custom implementation of the Check object.
Q4. Is the Check object suitable for large-scale applications?
Certainly! The Check object is highly suitable for large-scale applications as it helps ensure data integrity, prevents common errors, and enhances overall code reliability.
Q5. Can I extend the functionality of the Check object?
Yes, you can easily extend the functionality of the Check object by incorporating additional validation methods specific to your project’s requirements.
In conclusion, the Check object in JavaScript serves as an essential tool for data validation, type checking, and ensuring the integrity of values within JavaScript applications. By leveraging its features, developers can enhance program reliability and minimize potential bugs. So, make sure to utilize the Check object in your next JavaScript project and say goodbye to unexpected errors!
Property Hasown Does Not Exist On Type ‘Objectconstructor
One common issue that developers using TypeScript often encounter is the “Property hasOwn does not exist on type ‘ObjectConstructor'” error. This error typically occurs when attempting to use the hasOwnProperty method on an object in TypeScript. In this article, we will explore this issue in depth, understand its causes, and discuss possible solutions.
Understanding the Error
Before diving into the error itself, it is important to understand the concept of hasOwnProperty. The hasOwnProperty method is a built-in method in JavaScript that allows you to check if an object has a particular property. It returns a boolean value indicating whether the object has the specified property as a direct property (not inherited from its prototype chain).
TypeScript, being a strongly-typed and statically-typed language, provides type checking and additional features on top of JavaScript. However, TypeScript does not recognize the hasOwnProperty method as a valid method on the ObjectConstructor, leading to the “Property hasOwn does not exist on type ‘ObjectConstructor'” error.
Causes of the Error
The main cause of this error is related to the type definition of the ObjectConstructor in TypeScript. The ObjectConstructor is a type that represents the object constructor function, Object. However, the default type definition for ObjectConstructor in TypeScript does not include the hasOwnProperty method. Consequently, when developers try to use hasOwnProperty on an object in TypeScript, the compiler throws an error, as it does not recognize this method on the ObjectConstructor type.
Possible Solutions
There are several potential solutions to resolve this error and ensure type safety in TypeScript.
1. Casting the object to any:
One straightforward solution is to explicitly cast the object to the ‘any’ type. Casting an object to ‘any’ essentially tells TypeScript to bypass type checking for that object, allowing you to use any method or property you want. While this solution might solve the immediate error, it sacrifices the benefits of type checking and may lead to potential bugs or issues down the line.
Example:
const myObject: any = { /* object properties */ };
myObject.hasOwnProperty(‘property’);
2. Extending the Object prototype:
Another solution is to extend the Object prototype in TypeScript and explicitly define the hasOwnProperty method as part of the ObjectConstructor type. This approach ensures type safety and allows you to use hasOwnProperty on any object without any compilation errors.
Example:
interface ObjectConstructor {
hasOwnProperty(prop: PropertyKey): boolean;
}
const myObject: Object = { /* object properties */ };
myObject.hasOwnProperty(‘property’);
By extending the Object prototype, you can augment the existing type definition of ObjectConstructor in TypeScript, enhancing it with the appropriate method.
FAQs
Q: Why does TypeScript not include hasOwnProperty in the default ObjectConstructor type definition?
A: The default type definition for ObjectConstructor in TypeScript is intentionally kept minimal to prevent method name clashes and to provide a lightweight and flexible base for developers to extend as per their requirements.
Q: Are there any downsides to casting an object to ‘any’ to bypass the error?
A: While casting an object to ‘any’ allows you to bypass the error, it undermines the benefits of type checking in TypeScript. This approach can potentially lead to bugs and make the codebase less maintainable and robust.
Q: Is there a way to solve the error without modifying the ObjectConstructor type?
A: Yes, instead of using the hasOwnProperty method, you can use the in operator to check if a property exists on an object. The in operator works on any type and is type-safe.
Example:
const myObject: object = { /* object properties */ };
‘property’ in myObject;
By using the in operator, you can perform property existence checks without encountering the aforementioned error.
Conclusion
The “Property hasOwn does not exist on type ‘ObjectConstructor'” error is a common issue encountered by TypeScript developers. It occurs due to the absence of the hasOwnProperty method in the default ObjectConstructor type definition. By applying the suggested solutions, such as casting the object to ‘any’ or extending the Object prototype, you can resolve the error and ensure type safety in your TypeScript projects. Remember to weigh the pros and cons of each solution and choose the one that best suits your specific requirements.
Check Exist In Object Js
Introduction:
In JavaScript, there are multiple ways to determine if a specific key or property exists within an object. This ability is crucial, especially when dealing with complex data structures or performing various operations on objects. In this article, we will explore different techniques and methods to check for the existence of a key or property in JavaScript objects. Additionally, we will address common questions regarding these checks.
Ways to check if a key or property exists in an object:
1. Using the ‘in’ operator:
The ‘in’ operator allows us to check if a key or property exists in an object. It returns a boolean value – true if the key exists, and false if it does not. Here’s an example:
“`javascript
const obj = { name: “John”, age: 25 };
console.log(“name” in obj); // Output: true
console.log(“address” in obj); // Output: false
“`
2. Using the ‘hasOwnProperty’ method:
The ‘hasOwnProperty’ method checks whether an object has a specific property. It returns a boolean value indicating whether the object has the property directly (not inherited from its prototype chain). Here’s an example:
“`javascript
const obj = { name: “John”, age: 25 };
console.log(obj.hasOwnProperty(“name”)); // Output: true
console.log(obj.hasOwnProperty(“address”)); // Output: false
“`
3. Using the ‘!== undefined’ comparison:
Another way to determine if a property exists in an object is by comparing it to ‘undefined’. If the property is undefined, it means it does not exist in the object. Here’s an example:
“`javascript
const obj = { name: “John”, age: 25 };
console.log(obj.name !== undefined); // Output: true
console.log(obj.address !== undefined); // Output: false
“`
4. Using the ‘Object.keys’ method:
The ‘Object.keys’ method returns an array containing all the enumerable property names of an object. By checking if a specific key exists within this array, we can determine if it exists in the object. Here’s an example:
“`javascript
const obj = { name: “John”, age: 25 };
console.log(Object.keys(obj).includes(“name”)); // Output: true
console.log(Object.keys(obj).includes(“address”)); // Output: false
“`
Frequently Asked Questions (FAQs):
Q1. What is the difference between ‘in’ operator and ‘hasOwnProperty’ method?
A1. The ‘in’ operator checks for the existence of a key or property in an object, including those inherited from its prototype chain. On the other hand, the ‘hasOwnProperty’ method only checks for properties that are directly present in the object and are not inherited.
Q2. Which method is the most efficient one to check property existence?
A2. The most efficient method to check property existence would be using the ‘in’ operator. It provides a concise way to determine if a key exists in an object.
Q3. Can we use the ‘in’ operator to check if a nested property exists in an object?
A3. Yes, the ‘in’ operator can be used to check for existence of nested properties as well. For example:
“`javascript
const obj = { person: { name: “John”, age: 25 } };
console.log(“name” in obj.person); // Output: true
console.log(“address” in obj.person); // Output: false
“`
Q4. Is it possible to check for property existence in objects with a null prototype?
A4. Yes, we can use the ‘hasOwnProperty’ method to check property existence in objects that have a null prototype. However, the ‘in’ operator will not work in such cases, as it only checks for properties inherited from the prototype chain.
Q5. Should we always check for property existence before accessing it?
A5. It is generally good practice to check for property existence before accessing it, especially when dealing with user input or data from external sources. This helps prevent errors and ensure the code behaves as expected.
Conclusion:
Checking for the existence of a key or property in JavaScript objects is crucial for performing various operations and handling data efficiently. This article discussed several methods to perform this check, including the ‘in’ operator, ‘hasOwnProperty’ method, comparison with ‘undefined’, and the ‘Object.keys’ method. Understanding these techniques will enable developers to write more robust and error-free code when dealing with objects in JavaScript.
Remember to consider the nature of your object and requirements when choosing the appropriate method to check for property existence. Stay vigilant and ensure you handle possible scenarios to avoid any unexpected errors.
Images related to the topic do not access object.prototype method ‘hasownproperty’ from target object
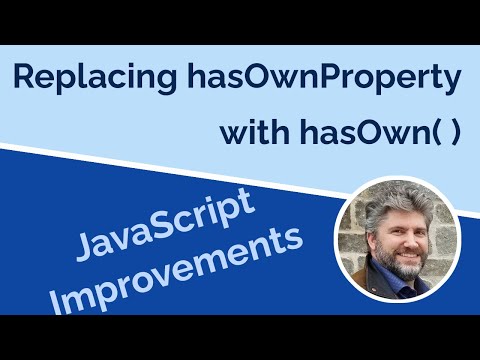
Found 48 images related to do not access object.prototype method ‘hasownproperty’ from target object theme
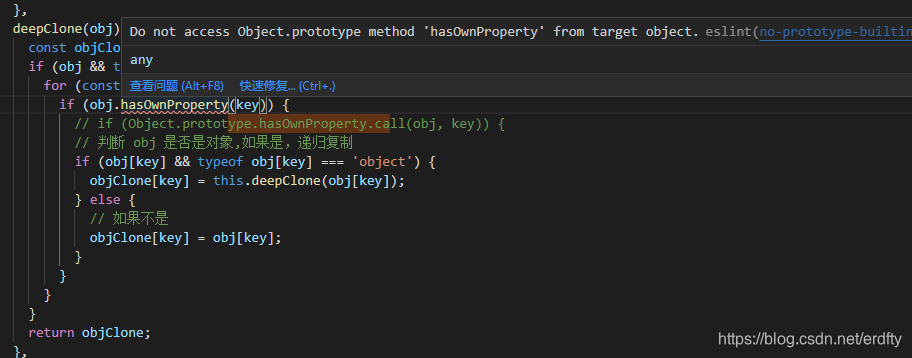
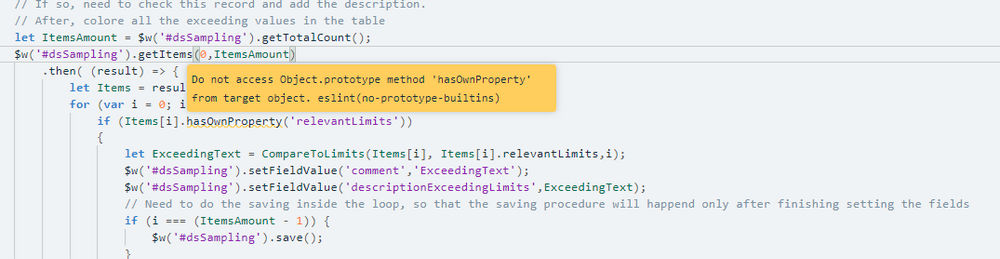
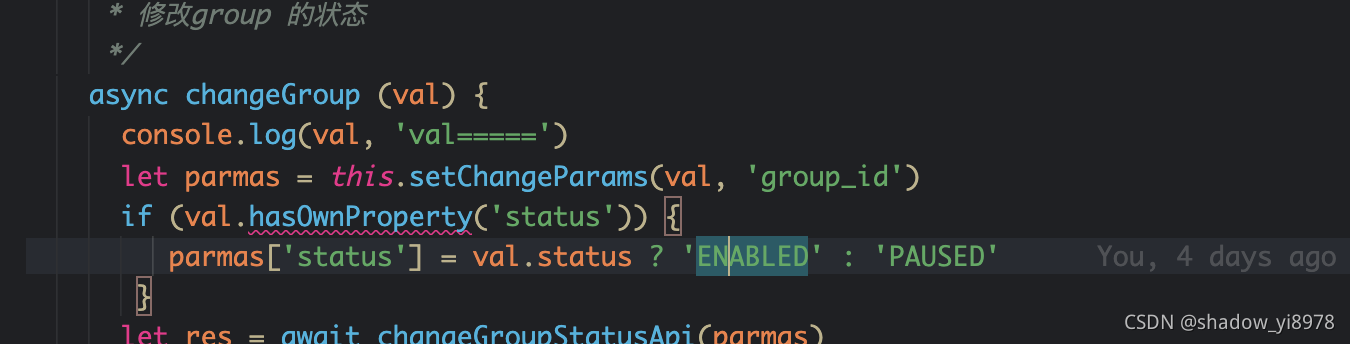
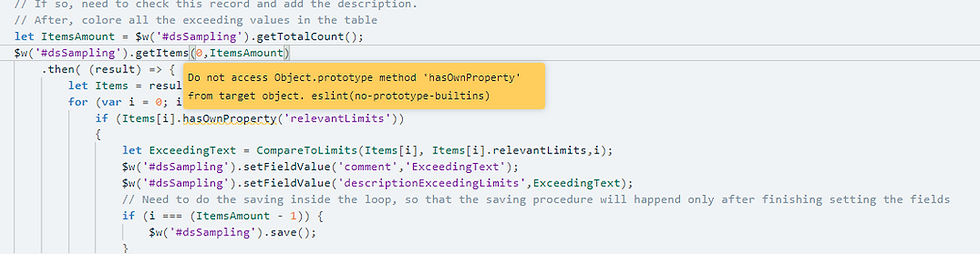
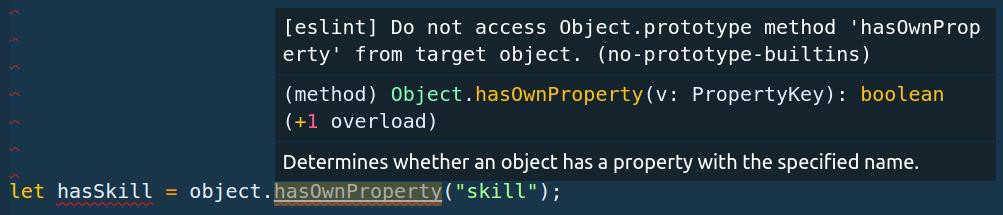
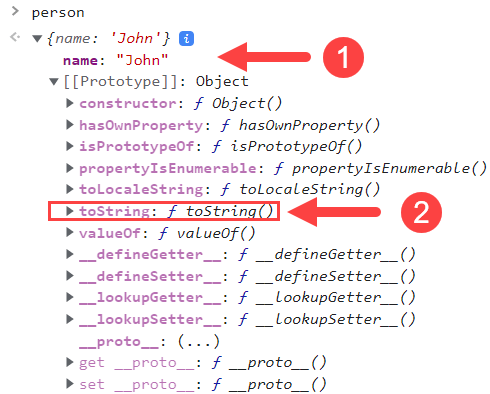
Article link: do not access object.prototype method ‘hasownproperty’ from target object.
Learn more about the topic do not access object.prototype method ‘hasownproperty’ from target object.
- Object.hasOwnProperty() yields the ESLint ‘no-prototype …
- How to fix ESLint error: Do not access Object.prototype …
- no-prototype-builtins – ESLint – Pluggable JavaScript Linter
- Error: Do not access Object.prototype method · Issue #138
- Object.prototype.hasOwnProperty() – JavaScript | MDN
- Accessible Object.prototype.hasOwnProperty details
- Do not access Object.prototype method ‘hasOwnProperty’ from …
- Lint Rule noPrototypeBuiltins – Rome Tools
See more: nhanvietluanvan.com/luat-hoc