Django Read Only Database
1. Understanding Django’s Read-Only Database Configuration
Django, a popular Python web framework, offers the ability to configure read-only databases for applications. A read-only database is a database that can only be read from, and not written to. This means that while data can be retrieved and displayed, no modifications or updates can be made to the data stored in the database.
The purpose of utilizing read-only databases in Django is to separate the responsibilities of read operations and write operations, improving performance and security. By having a separate database for read operations, the load on the read-write database can be reduced, resulting in improved performance. Additionally, read-only databases can provide an additional layer of protection against unauthorized modifications to data.
Scenarios where read-only databases may be useful include cases where data is rarely modified but frequently read, such as static content, reference data, or data that is rarely updated, such as historical data. By utilizing a read-only database for these scenarios, the read-write database can be primarily used for storing and accessing frequently updated data, resulting in a more efficient system.
2. Configuring a Read-Only Database in Django
To configure a read-only database in Django, the database connection settings need to be modified in the application’s settings.py file. Django supports multiple databases, allowing for separate configurations for read-only and read-write databases.
First, define a new database in the DATABASES setting, specifying the necessary connection details such as engine, host, port, and credentials. After defining the database, mark it as read-only by setting the OPTIONS attribute to contain {‘readOnly’: True}. This tells Django that this database connection should only be used for read operations.
3. Implementing Read-Only Models in Django
Read-only models in Django are used to map database tables or views that should be treated as read-only. To define a read-only model, create a new Python class that inherits from the django.db.models.Model class. Instead of defining fields, the model should use the Meta class to specify the database table or view name and the managed attribute set to False.
By setting managed to False, Django will not create or alter the database schema for this model and will assume that the table or view already exists in the database. This allows read-only models to be used for mapping existing database schemas.
4. Reading Data from a Read-Only Database in Django
To retrieve data from a read-only database in Django, utilize the Django ORM (Object-Relational Mapping) and its querying mechanisms. The ORM provides a high-level abstraction for interacting with databases.
When querying a read-only database, the ORM will automatically generate the appropriate SQL queries for reading data, ensuring that no write operations are performed. This provides a seamless experience for developers, allowing them to retrieve data in the same way as they would from a read-write database.
To distinguish between read-only and read-write queries, developers can refer to the documentation and use appropriate methods such as filter(), values(), or raw SQL queries with SELECT statements.
5. Improving Performance and Scalability with Read-Only Databases
Utilizing read-only databases can improve performance by offloading read operations from the read-write database. This separation allows for dedicated resources and optimizations for read operations, resulting in faster response times and improved scalability.
To further enhance performance, caching techniques can be implemented. By caching frequently accessed read-only data in memory, the need to query the read-only database for every request is reduced, resulting in faster response times. Django provides built-in caching mechanisms that can be easily integrated into applications.
In distributed systems where scalability is a concern, read-only databases can be utilized to provide horizontal scaling capabilities. By having multiple read-only database replicas, the read workload can be distributed across multiple servers, improving performance and handling larger traffic loads.
6. Tips and Best Practices for Working with Django Read-Only Databases
When working with Django read-only databases, some practical tips and best practices should be followed:
– Regularly backup the read-only database, even though modifications cannot be made, data can still be lost due to various reasons such as hardware failures or accidental deletions.
– Monitor the read-only database for performance issues or bottlenecks. It is essential to ensure that the read-only database can handle the required read workload without sacrificing performance.
– Consider using read replicas for read-only databases to distribute the load and improve scalability.
– Carefully design and plan the use of read-only databases. Analyze the data requirements and usage patterns to identify which data is suitable for read-only databases.
– Consider using materialized views or denormalized schemas in the read-only database to optimize read performance.
– Use appropriate indexing on the read-only database to enhance query performance.
– Regularly test and benchmark the application to ensure that the read-only database configuration is effectively improving performance and scalability.
FAQs:
Q1: Can I use multiple read-only databases in Django?
A1: Yes, Django supports multiple databases, so you can configure and use multiple read-only databases in your application.
Q2: Can I switch between read-only and read-write databases dynamically in Django?
A2: Yes, Django allows you to switch between different databases dynamically. You can specify the database to use for each query or change the default database dynamically.
Q3: Can I create Django models from an existing read-only database schema?
A3: Yes, you can create Django models from an existing read-only database schema. By using the inspectdb command, Django can automatically generate model definitions based on the existing database schema.
Q4: Can I save a model instance to a different read-only database in Django?
A4: No, saving a model instance to a different database is not possible in Django. The write operations are strictly limited to the read-write database.
Q5: Are read-only databases suitable for all types of applications?
A5: No, read-only databases are most suitable for scenarios where data is rarely modified but frequently read. For applications with heavy write operations, a traditional read-write database is more appropriate.
Q6: Can I use the Django admin interface with a read-only database?
A6: Yes, you can use the Django admin interface with a read-only database. However, modifications or updates made through the admin interface will not be reflected in the read-only database.
Django Multiple Database Setup Ex1
How To Read File From Database In Django?
Django is a high-level Python web framework that simplifies web application development. It provides various powerful tools and features to manage and retrieve data from databases. In this article, we will focus on how to read a file from a database in Django.
When it comes to reading files from a database in Django, there are a few steps you need to follow. Let’s dive into the process in detail:
Step 1: Set Up Database Models
To read files from a database, you first need to define a model that represents the data structure. In this case, we will include a FileField or ImageField in the model to store the file.
Here’s an example of a model named Document:
“`python
from django.db import models
class Document(models.Model):
file = models.FileField(upload_to=’documents/’)
uploaded_at = models.DateTimeField(auto_now_add=True)
“`
In this model, the ‘file’ field represents the file itself, and the ‘uploaded_at’ field will store the timestamp of when the file was uploaded. The ‘upload_to’ parameter specifies the directory where the file will be stored.
Step 2: Add FileField to Form
Next, you need to create a form that allows users to upload files. You can use Django’s built-in Form class for this purpose.
“`python
from django import forms
class DocumentForm(forms.Form):
file = forms.FileField()
“`
This form includes a ‘file’ field of type FileField, which enables users to select and upload files. You can customize the form further according to your requirements.
Step 3: Store Uploaded File in the Database
Once the user uploads a file, you need to handle the file and store it in the database. This can be done using the views in Django.
First, you would need to import the necessary modules in the views.py file:
“`python
from django.shortcuts import render, redirect
from .forms import DocumentForm
from .models import Document
“`
Then, you can define a view function to handle the file upload:
“`python
def upload_file(request):
if request.method == ‘POST’:
form = DocumentForm(request.POST, request.FILES)
if form.is_valid():
document = Document(file=request.FILES[‘file’])
document.save()
return redirect(‘success_page’) # Redirect to a success page
else:
form = DocumentForm()
return render(request, ‘upload.html’, {‘form’: form})
“`
In this code snippet, we first check if the request method is ‘POST’. If it is, that means the form has been submitted, and we can proceed to save the file. The ‘is_valid()’ method checks if the form input is valid.
The file is saved as a Document object in the database using the ‘Document’ model we defined earlier. The ‘request.FILES’ attribute allows access to the uploaded file. Finally, we redirect the user to the success page.
Step 4: Read and Display the File
To read and display the uploaded file from the database, you need to retrieve it from the database and render it in a view.
First, import the necessary modules:
“`python
from django.http import HttpResponse
from .models import Document
“`
Next, define a view function to retrieve and display the file:
“`python
def display_file(request, file_id):
document = Document.objects.get(pk=file_id)
file_content = document.file.read()
response = HttpResponse(content_type=’application/pdf’) # Set the appropriate content type
response.write(file_content)
return response
“`
In this code snippet, we retrieve the Document object from the database using its primary key (‘file_id’). The ‘file_content’ variable stores the content of the file retrieved from the database. Finally, we create an HttpResponse object with the appropriate content type (e.g., ‘application/pdf’) and write the file content to the response.
To display the file, you need to define a URL pattern and connect it to the view in your URL configuration.
“`python
from .views import display_file
urlpatterns = [
# Other URL patterns…
path(‘display_file/
]
“`
Now you can access the file by visiting the URL ‘/display_file/
FAQs:
Q: Can I read and display files other than PDFs?
A: Yes, you can. The example provided uses the ‘application/pdf’ content type, but you can adjust it according to the file type you want to display. For example, if you want to display an image file, you can set ‘image/png’ or ‘image/jpeg’ as the content type.
Q: How can I add additional fields to the Document model?
A: You can customize the Document model by adding any additional fields you need. For example, you might consider adding fields like ‘title’, ‘description’, or ‘owner’ to provide more information about the uploaded file.
Q: How can I secure file uploads?
A: Django provides various security measures to protect file uploads, including validation, file type checking, and controlling file size. You can refer to Django’s documentation on file uploads for a comprehensive understanding of the security measures you can implement.
Q: Can I read files stored outside the Django application’s filesystem?
A: Yes, Django provides support for reading files from external storage systems such as Amazon S3, Google Cloud Storage, or a remote file server. You can configure Django to use these external storage systems by modifying your settings file.
Q: Can I download the file instead of displaying it in the browser?
A: Yes, you can replace the ‘HttpResponse’ object with a ‘FileResponse’ object and use the ‘attachment’ option to prompt the user to download the file instead of displaying it in the browser.
Conclusion:
Reading files from a database in Django involves defining a model with a ‘FileField’ or ‘ImageField’, creating a form for file uploads, storing the files in the database, and finally retrieving and rendering them in a view. By following these steps, you can effectively read files from a database in your Django application, providing flexibility and convenience to your users.
How To Read Mysql Database In Django?
Django is a powerful web framework that allows developers to efficiently create web applications, and one of its key features is its seamless integration with various database systems, including MySQL. In this article, we will explore the different methods of reading data from a MySQL database using Django and provide a step-by-step guide to help you get started.
1. Configure database settings:
Before we dive into reading data, it’s essential to set up the database connection in Django’s settings file. Open the ‘settings.py’ file in your Django project and locate the ‘DATABASES’ dictionary. Replace the default SQLite settings with MySQL settings by specifying the ‘ENGINE’, ‘NAME’, ‘USER’, ‘PASSWORD’, ‘HOST’, and ‘PORT’ parameters accordingly.
2. Create models:
Models in Django represent the structure and logic of your database tables. To read data from a MySQL database, you need to define models that mirror the tables you want to access. Open your Django app’s ‘models.py’ file and create a new class for each table, inheriting from the ‘django.db.models.Model’ superclass. Within each class, define the fields and their respective types using Django’s built-in field classes, such as ‘CharField’, ‘IntegerField’, ‘DateField’, and more.
3. Generate migrations:
After defining the models, it’s time to create the necessary database tables and columns based on the models you’ve created. Django provides a set of management commands that help with this process. Run the command ‘python manage.py makemigrations’ followed by ‘python manage.py migrate’ to generate and apply the migrations respectively. This will create the necessary database schema for your models.
4. Read data from the database:
Now that your database is set up, you can start reading data from it. Django provides a powerful Object-Relational Mapping (ORM) system that abstracts away the underlying SQL queries and enables you to work with models as Python objects. In your views or any other desired section of your code, you can import the models you created and query the database using Django’s ORM methods such as ‘objects.all()’, which returns all the objects of a model, or ‘objects.filter()’, which allows you to apply various filters to narrow down the result set.
5. Example usage:
Let’s consider a simple example where we have a ‘Book’ model representing a table in the database. To read all the books from the database and display their titles, you can follow these steps:
“`python
from .models import Book
def display_book_titles():
books = Book.objects.all()
for book in books:
print(book.title)
“`
In this example, we import the ‘Book’ model from our models file, then use the ‘objects.all()’ method to retrieve all the book objects from the database. Finally, we iterate through the ‘books’ queryset and print each book’s title.
Frequently Asked Questions (FAQs):
Q1. Can Django read data from a MySQL database hosted on a remote server?
A1. Yes, Django can connect to a remote MySQL database server. You need to provide the proper host and port parameters in the database settings to establish the connection.
Q2. How can I optimize MySQL database queries in Django?
A2. Django provides optimization techniques such as selecting only the required fields, using ‘select_related()’ and ‘prefetch_related()’ to minimize database hits, and leveraging the ‘QuerySet.only()’ method to limit the number of retrieved fields.
Q3. Can I read data from multiple MySQL databases within a single Django project?
A3. Yes, Django supports working with multiple databases. You need to define separate database configurations under the ‘DATABASES’ dictionary in the settings file and specify the database alias when querying data from a specific database.
Q4. Is it possible to execute complex SQL queries directly instead of using Django’s ORM?
A4. Yes, Django provides the ‘raw()’ method, which allows you to execute raw SQL queries and retrieve the results as model objects or plain dictionaries.
Q5. What happens if a query retrieves a large number of records from the database?
A5. Django includes pagination support that allows you to efficiently handle large result sets by fetching only a subset of the results at a time.
In conclusion, Django offers a seamless way to read data from a MySQL database. By following the steps outlined in this article and utilizing Django’s ORM, you can easily retrieve and manipulate data from your MySQL database within your Django web application.
Keywords searched by users: django read only database django multiple database readonly, django read-only model, django multiple database example, django save model to different database, django switch database, django db connection, create django models from existing database, multiple django apps one database
Categories: Top 38 Django Read Only Database
See more here: nhanvietluanvan.com
Django Multiple Database Readonly
Introduction
When it comes to building web applications, Django is a popular choice among developers due to its simplicity, versatility, and powerful features. In many cases, applications require large amounts of data that can put a strain on the main database and affect overall performance. To tackle this challenge, Django provides a feature called multiple databases, which allows developers to distribute the workload across multiple databases. In this article, we will delve into the concept of multiple database read-only in Django, exploring its benefits, implementation, and provide answers to commonly asked questions.
Understanding Multiple Database in Django
In Django, the multiple database functionality can be leveraged to split the database load for better performance or to integrate with various types of databases. By default, Django works with a single database, but when the need arises for additional databases, Django’s support for multiple databases proves invaluable.
The primary use case of multiple databases is often to improve application performance and scalability. By distributing the read and write operations across multiple databases, the load is balanced, resulting in faster response times and increased capacity. However, while multiple databases offer significant advantages for read-heavy applications, it’s crucial to differentiate between read-only and read-write databases.
Multiple Database ReadOnly for Performance Optimization
When a web application has a high volume of read requests compared to writes, it becomes beneficial to configure some databases as read-only. By designating certain databases as read-only, developers can ensure that only read operations are directed to them, further optimizing the performance and scalability of the application.
To configure read-only databases in Django, you need to define multiple database settings in your project’s settings.py file. Each database setting includes the necessary configuration details such as name, engine, host, port, and credentials. Additionally, you can specify the `PRIMARY_REPLICA` setting to define the primary database and its replicas for load balancing. By specifying the desired database as read-only, Django will automatically direct read queries to these databases, ensuring the read workload is evenly distributed.
Implementing Multiple Database ReadOnly in Django
To implement multiple database read-only in Django, follow these steps:
1. Define the database settings: In your project’s settings.py file, define multiple database settings by specifying the name, engine, host, port, and credentials for each database, along with the `PRIMARY_REPLICA` setting.
2. Create router for read-only databases: Create a database router that defines the behavior for read and write operations. The router should direct read operations to the designated read-only databases and write operations to the primary database.
3. Define Read-Only Database Models: For read-only databases, define the required models in your Django application. These models will represent the read-only database’s tables and allow you to perform read operations effectively.
4. Use QuerySets and Managers: To interact with the read-only databases, use Django’s QuerySets and Managers. QuerySets provide convenient methods for retrieving data from the database, while Managers allow you to define custom methods for specific operations.
Benefits of Multiple Database ReadOnly
1. Enhanced Performance: Distributing the read workload across multiple databases improves performance and reduces response times. By segregating reads from writes, the overall database performance can be significantly improved.
2. Scalability: With multiple read-only databases, the application can handle a higher load by allowing more concurrent read requests. This scalability is especially useful for applications with high read traffic.
3. Load Balancing: By balancing the read workload across multiple read-only databases, the overall system load is distributed evenly, preventing any single database from becoming a bottleneck.
4. Improved Availability: Read-only replicas can serve as hot standbys, providing increased availability and data redundancy. If the primary database fails, the read-only replicas can quickly take over the read operations, ensuring business continuity.
5. Cost Optimization: Leveraging read-only replicas reduces the need for expensive hardware upgrades for the primary database. It allows for more cost-effective scaling by utilizing multiple less powerful read-only databases.
FAQs
Q1. Can I perform write operations on read-only databases?
No, read-only databases are explicitly designated for read operations only. Attempting to write data to a read-only database will result in an exception/error.
Q2. How can I ensure data consistency between read-only and read-write databases?
To ensure data consistency, Django provides a mechanism called database routing. By configuring a specific database router, you can direct write operations to the designated read-write database while performing read operations on the read-only databases.
Q3. Can I use multiple databases with different database engines?
Yes, Django supports using different database engines for various databases. For example, you can have one MySQL database as the primary database and multiple PostgreSQL databases as read-only replicas.
Q4. How can I control which database to use for a specific query or operation?
Django provides a database abstraction API that allows developers to explicitly specify which database to use for a specific query or operation. By utilizing the `using` statement, you can ensure the query is directed to the appropriate database.
Conclusion
Implementing multiple database read-only functionality in Django can significantly enhance the performance, scalability, and availability of web applications. By distributing the read workload among multiple databases, developers can achieve better response times and optimize resource utilization. While read-only databases offer numerous benefits, it’s essential to carefully consider data consistency and ensure proper routing mechanisms for a seamless experience. With proper implementation and configuration, multiple database read-only in Django can be a game-changer for high-traffic applications.
Django Read-Only Model
Django is a high-level Python web framework that facilitates rapid development and clean design. One of the powerful features of Django is its model system, which allows developers to define the structure and behavior of their application’s data. In this article, we will explore the concept of a Django read-only model, its benefits, and how to implement it in your Django project.
Understanding Django Read-Only Model:
A read-only model in Django is a model that cannot be modified or saved directly by end-users or even developers. It primarily serves as a container for data that remains constant throughout the lifespan of an application, such as reference data, configuration settings, or read-only views of complex data.
Why use Django Read-Only Model?
1. Data Integrity: By marking certain models as read-only, you ensure that the data remains constant and accurate. This prevents accidental modifications or deletions, ultimately enhancing data reliability.
2. Security: If a model contains sensitive or critical information that should never be altered, making it read-only can add an extra layer of security. It prevents unauthorized modifications and reduces the risk of data tampering.
3. Performance Optimization: Read-only models are lighter on system resources as they do not have to worry about write operations. This can result in improved application performance and scalability.
Implementing Django Read-Only Model:
Implementing a read-only model in Django is a straightforward process. Let’s explore the steps involved:
Step 1: Define the Model:
Start by defining a regular Django model class, just like any other model. Specify the fields, relationships, and any other attributes required for your read-only data.
“`python
from django.db import models
class ReadOnlyModel(models.Model):
name = models.CharField(max_length=100)
# other fields…
class Meta:
# Specify that this model is read-only
managed = False
# Optionally, define the database table name or use an existing view
db_table = ‘read_only_model’
“`
Note the `managed = False` attribute in the `Meta` class. This is the key step that makes this model read-only and prevents migration management for this model.
Step 2: Register the Model:
To make the read-only model visible in the Django admin interface or accessible via the Django ORM, register it with admin.site.register() or add it to your project’s list of installed apps.
“`python
from django.contrib import admin
admin.site.register(ReadOnlyModel)
“`
Step 3: Set Permissions:
To enforce the read-only behavior globally, you can add custom permission checks at the model or view level. For example, you can create a custom Django permission and assign it to the read-only model.
“`python
from django.contrib.auth.models import Permission
from django.contrib.contenttypes.models import ContentType
from django.db.models.signals import post_migrate
def add_readonly_permission(sender, **kwargs):
readonly_model = ContentType.objects.get(app_label=’your_app’, model=’readonlymodel’)
permission = Permission.objects.create(
codename=’view_readonlymodel’,
name=’Can view readonly model’,
content_type=readonly_model,
)
post_migrate.connect(add_readonly_permission)
“`
This ensures that only users with the “Can view readonly model” permission can access and view the read-only model’s data.
Step 4: Update Views:
If you want to display the read-only data in your application’s views, you can override the default Django CRUD views and modify them to only allow GET requests. This way, any attempts to modify the data will result in permission denied errors.
Frequently Asked Questions (FAQs):
Q1. Can I still modify a read-only model using Django’s management commands?
A: No, read-only models cannot be modified using Django’s management commands, such as `makemigrations` or `migrate`. The `managed = False` attribute prevents database modifications for this model.
Q2. Can I still access a read-only model’s data using the Django ORM?
A: Yes, read-only models can still be accessed using the Django ORM, allowing you to fetch and use the data within your application.
Q3. What if I need to modify the data within a read-only model?
A: If you have a requirement to modify the data within a read-only model, you would need to remove the `managed = False` attribute and handle the necessary migration management yourself. However, it is strongly recommended to carefully consider the implications before making such a change.
Q4. Can I convert an existing model to a read-only model?
A: Yes, you can convert an existing model to a read-only model by adding the `managed = False` attribute and ensuring migration management is handled accordingly.
Conclusion:
Implementing a read-only model in Django allows developers to safeguard critical data, enhance data integrity, and optimize application performance. By combining the `managed = False` attribute, custom permissions, and view modifications, you can easily create and work with read-only models in your Django projects. However, it is crucial to carefully assess the need for read-only models and their potential impact on your application’s functionality before implementation.
Images related to the topic django read only database
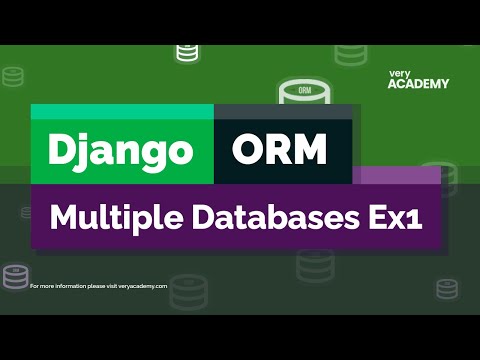
Found 18 images related to django read only database theme

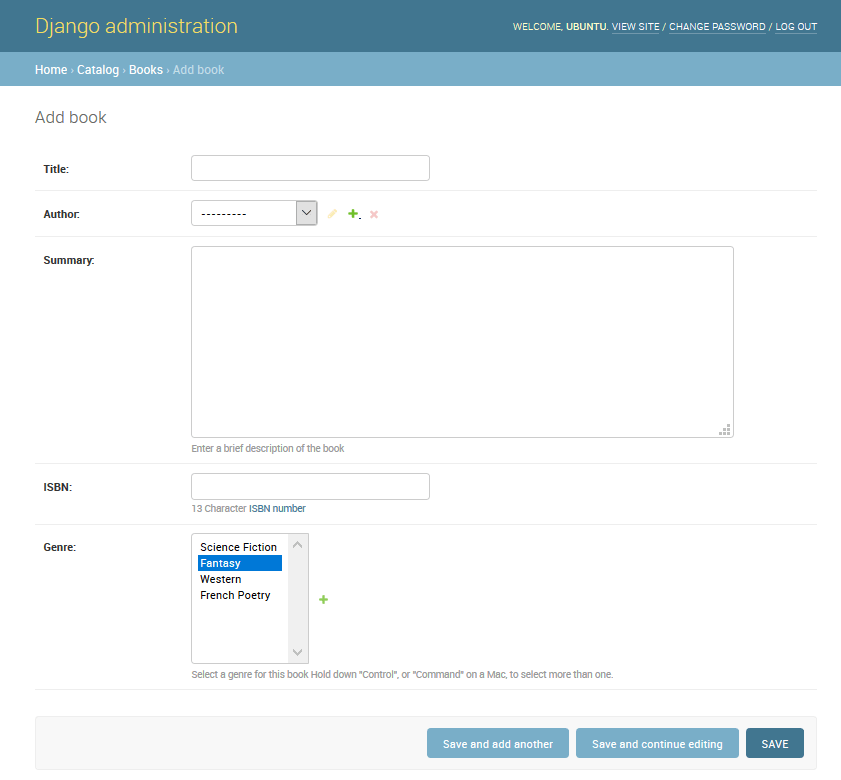
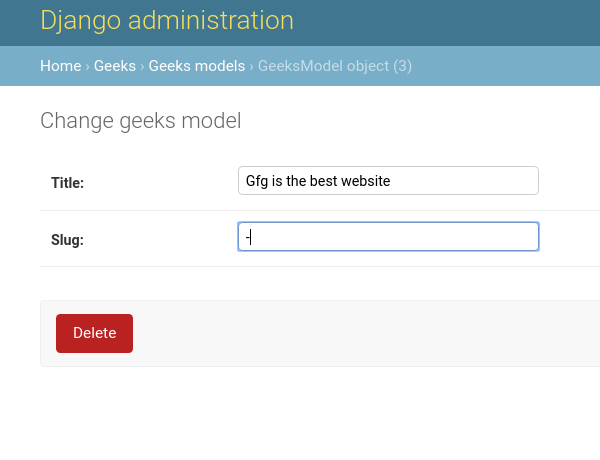
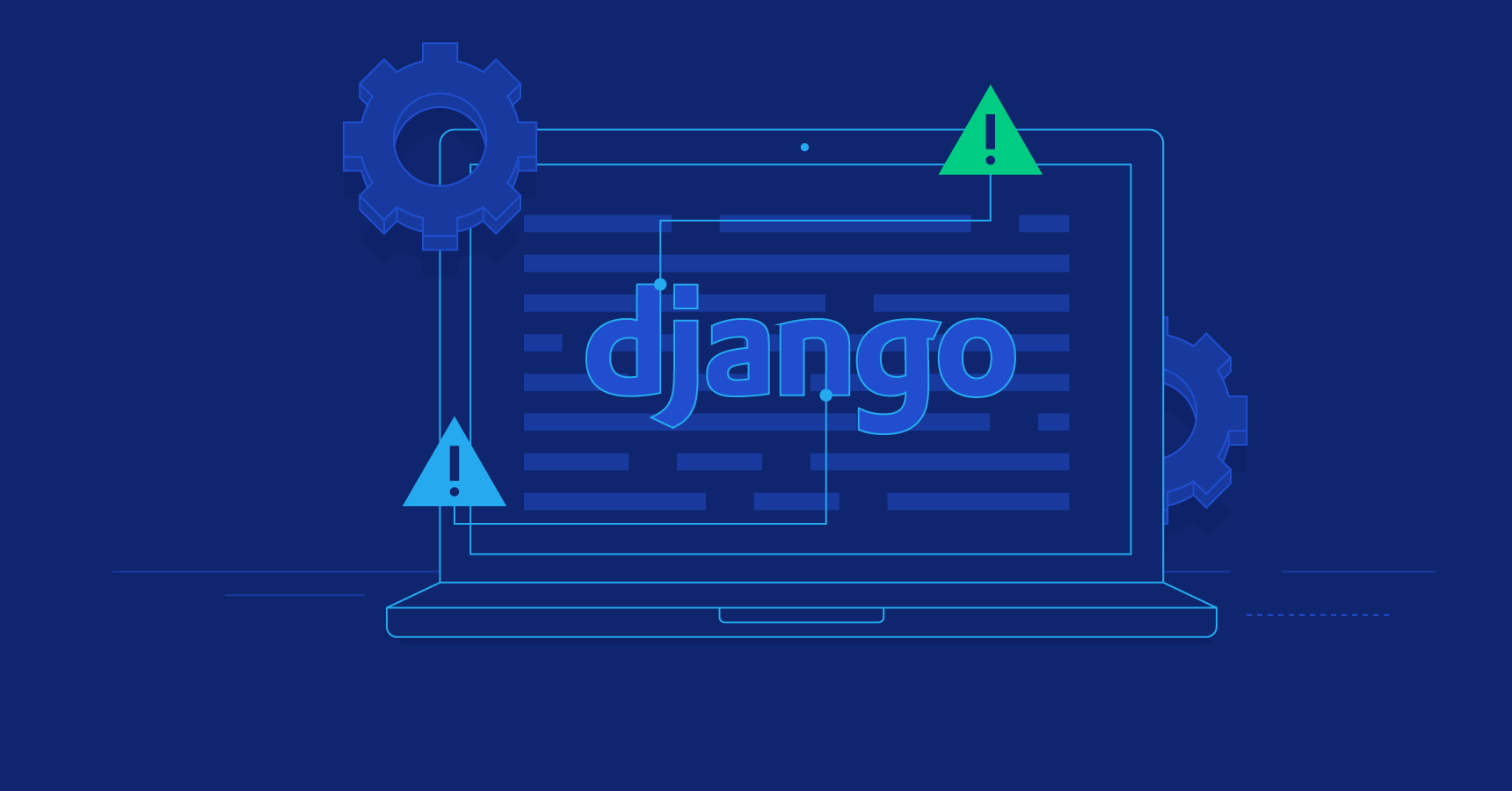

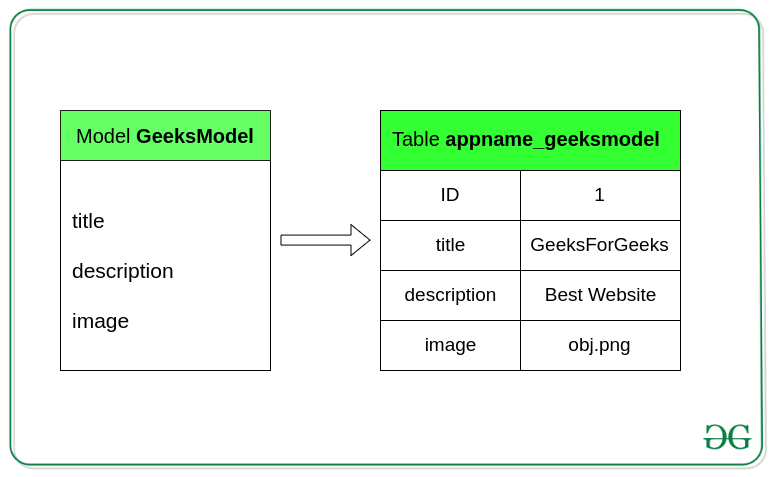
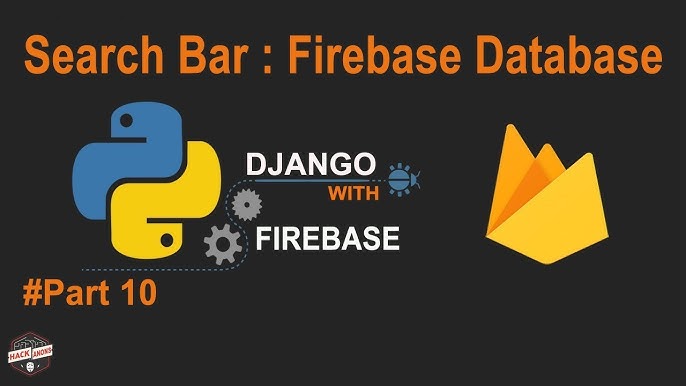

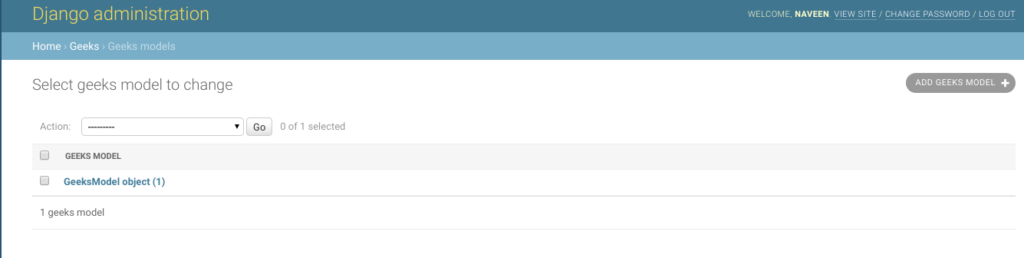
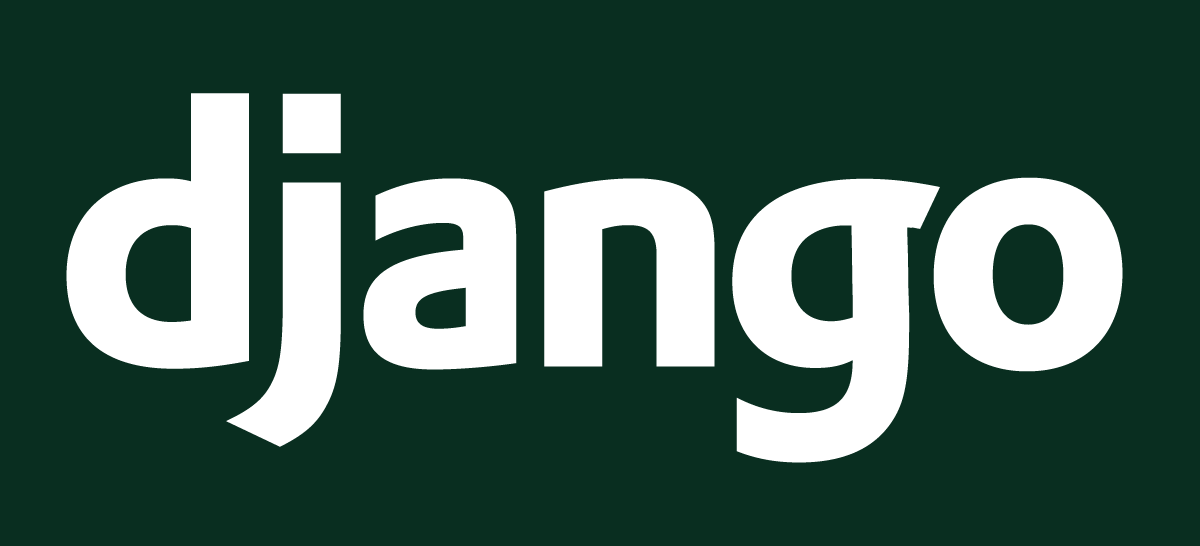
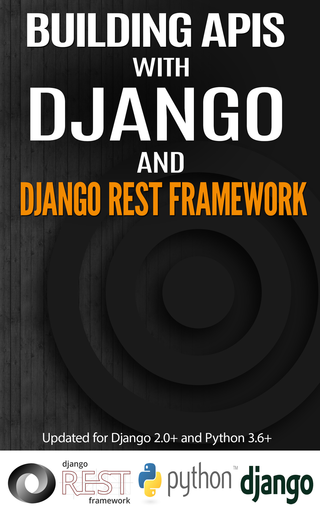

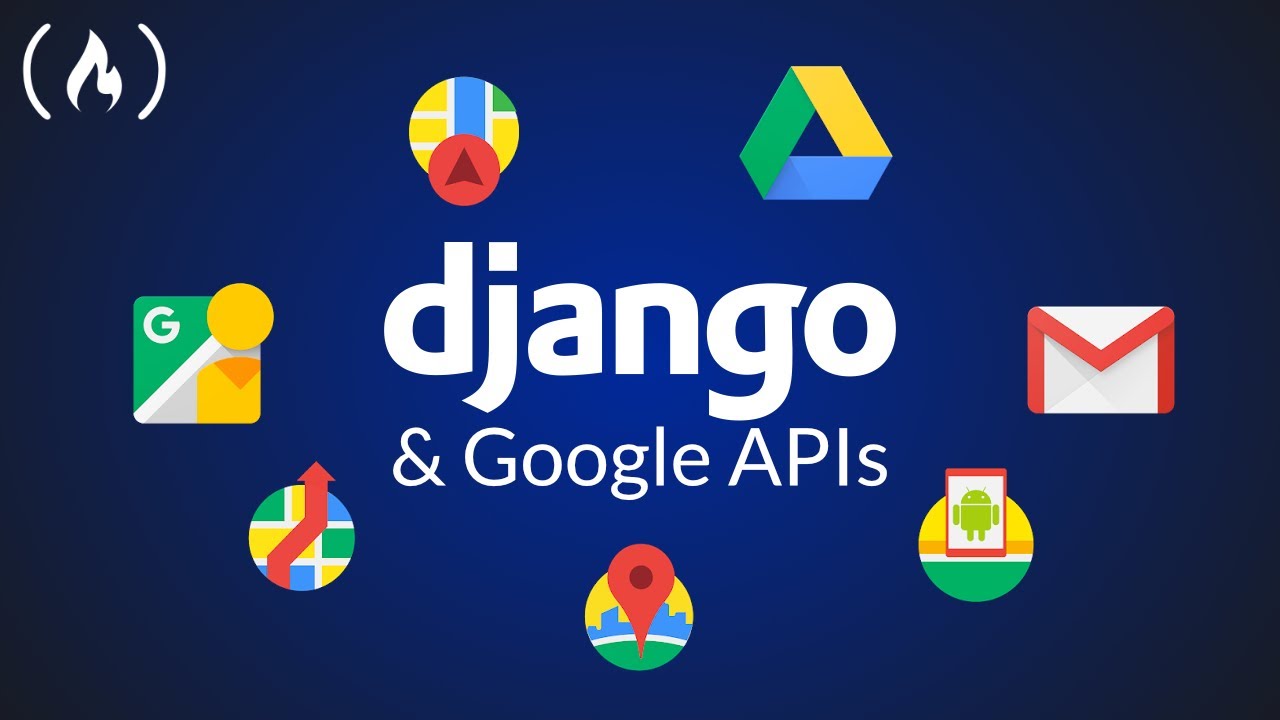
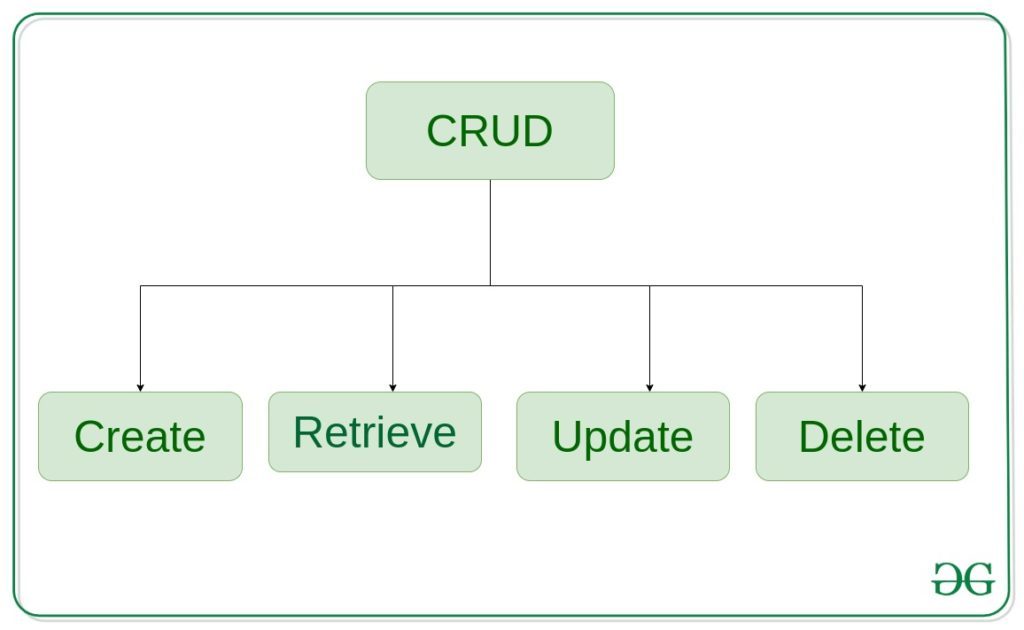
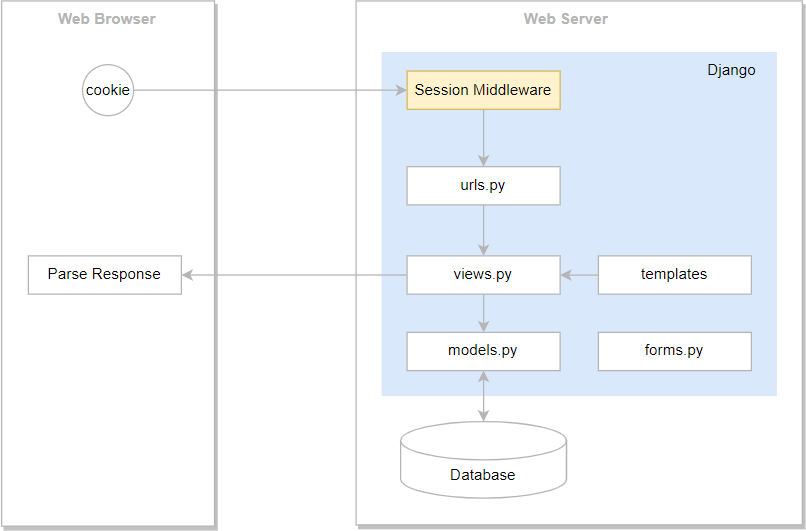


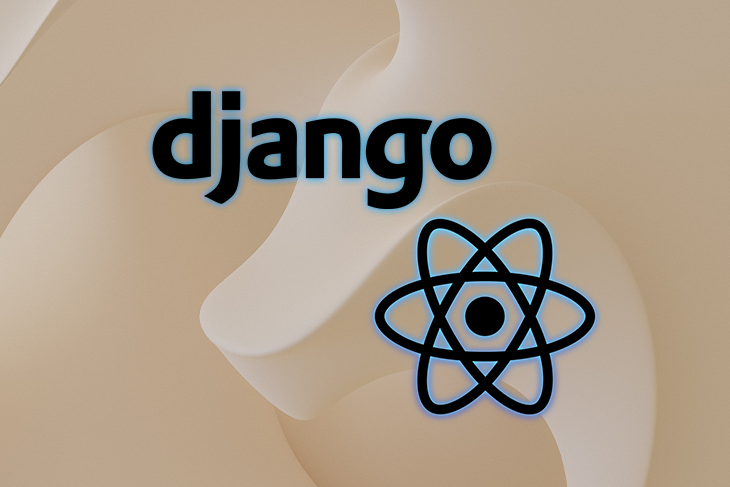
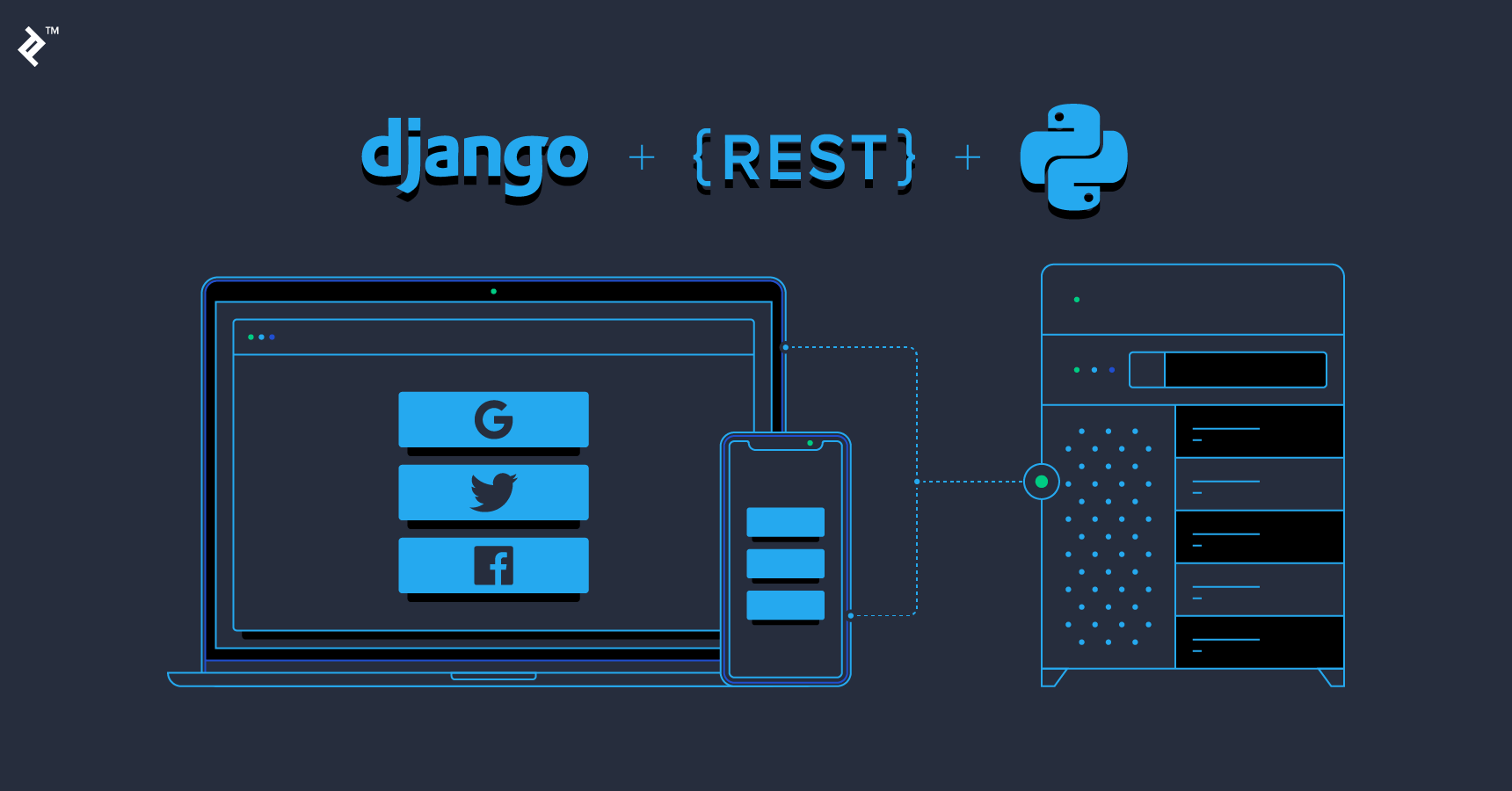
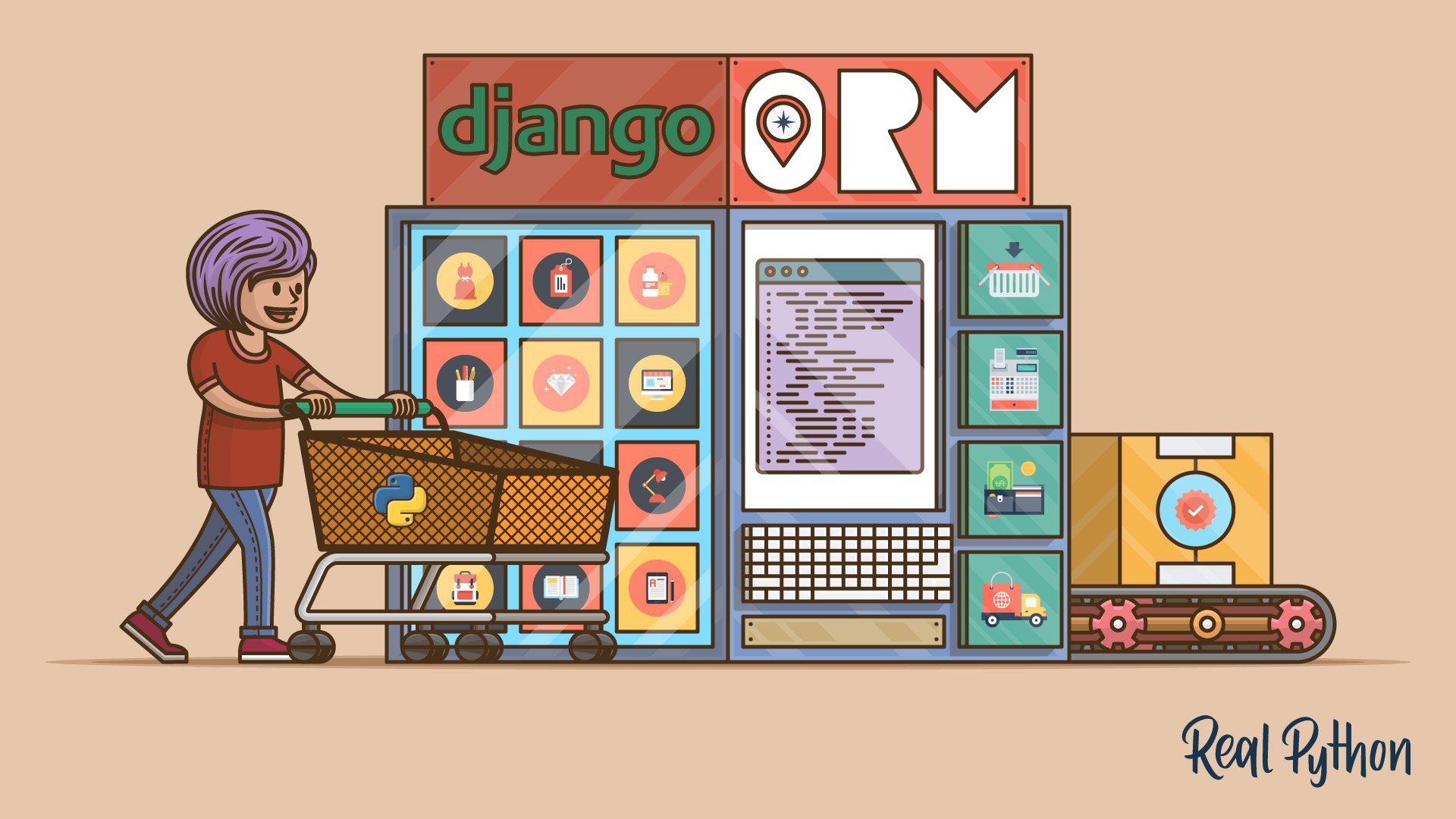
![how to fix SQL Error- attempt to write a read only database | unable to open database file [SOLVED] - YouTube How To Fix Sql Error- Attempt To Write A Read Only Database | Unable To Open Database File [Solved] - Youtube](https://i.ytimg.com/vi/AHj0zDifHzE/maxresdefault.jpg)

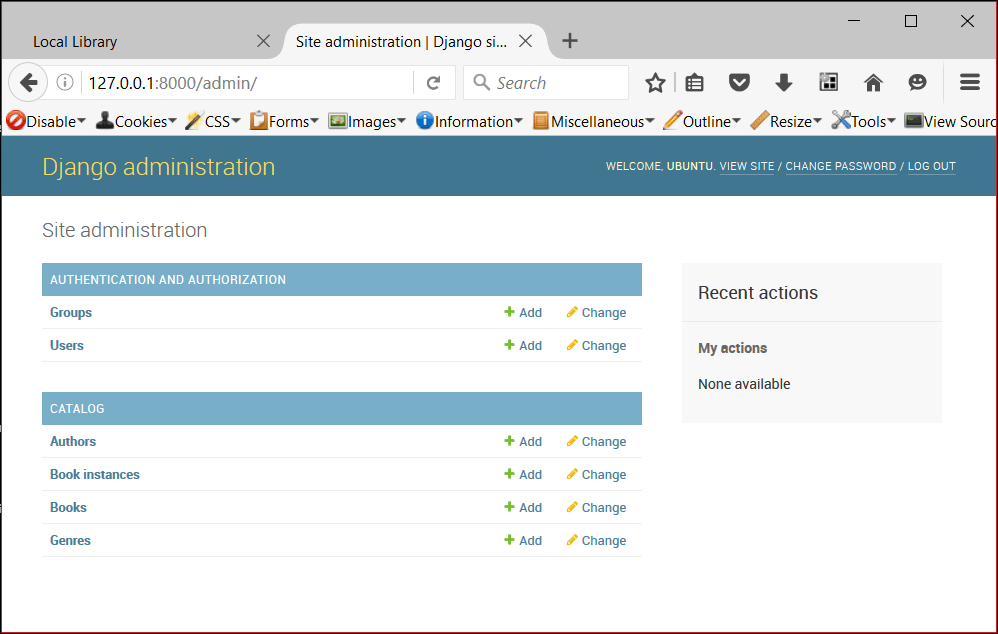



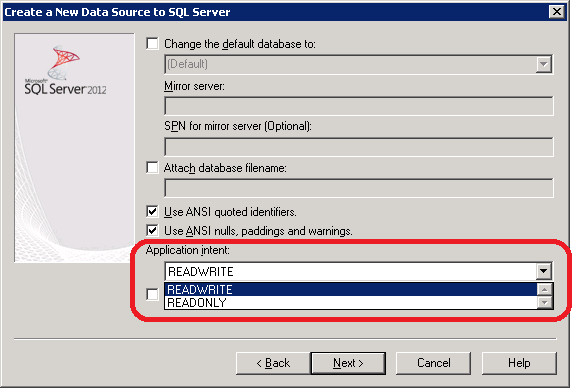
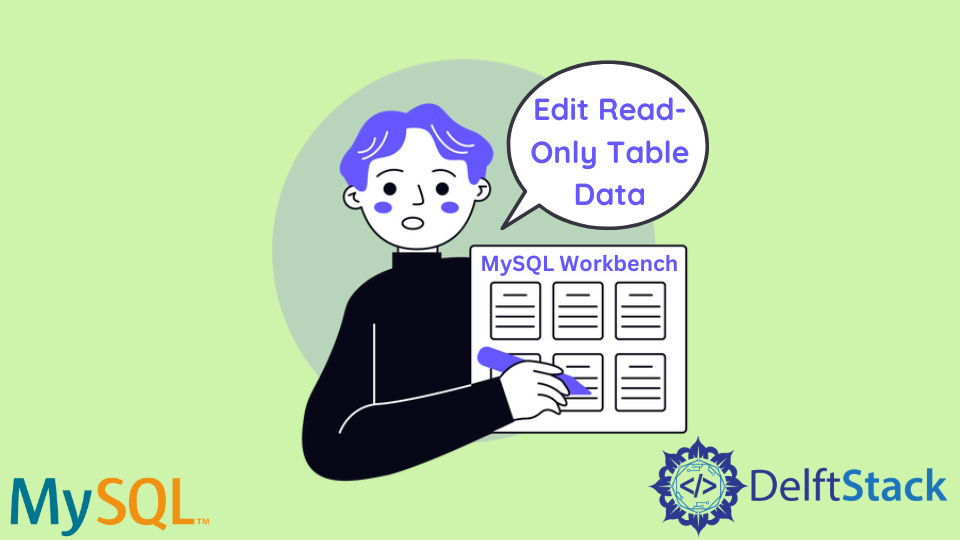

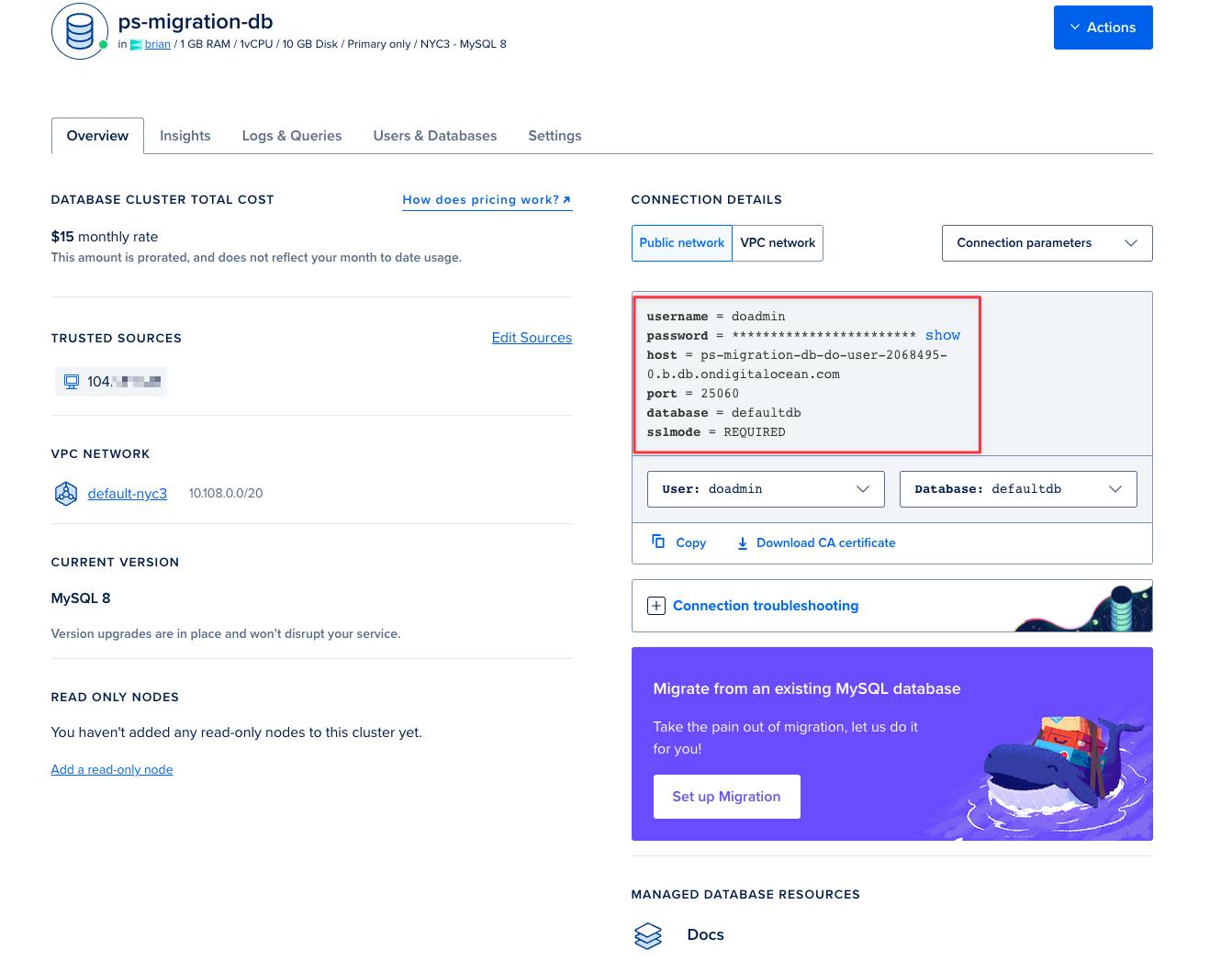

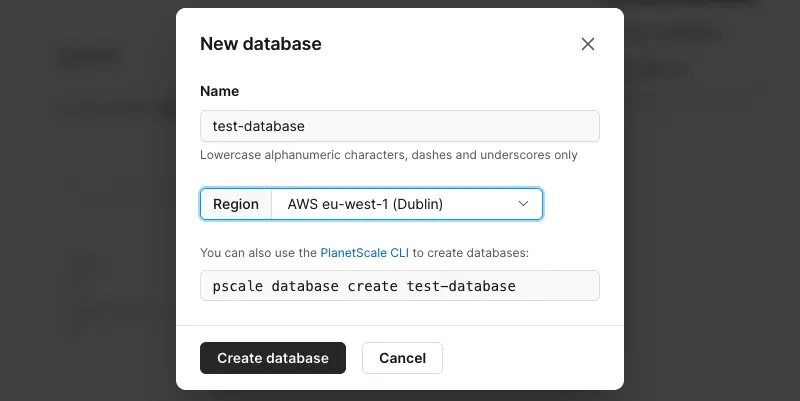
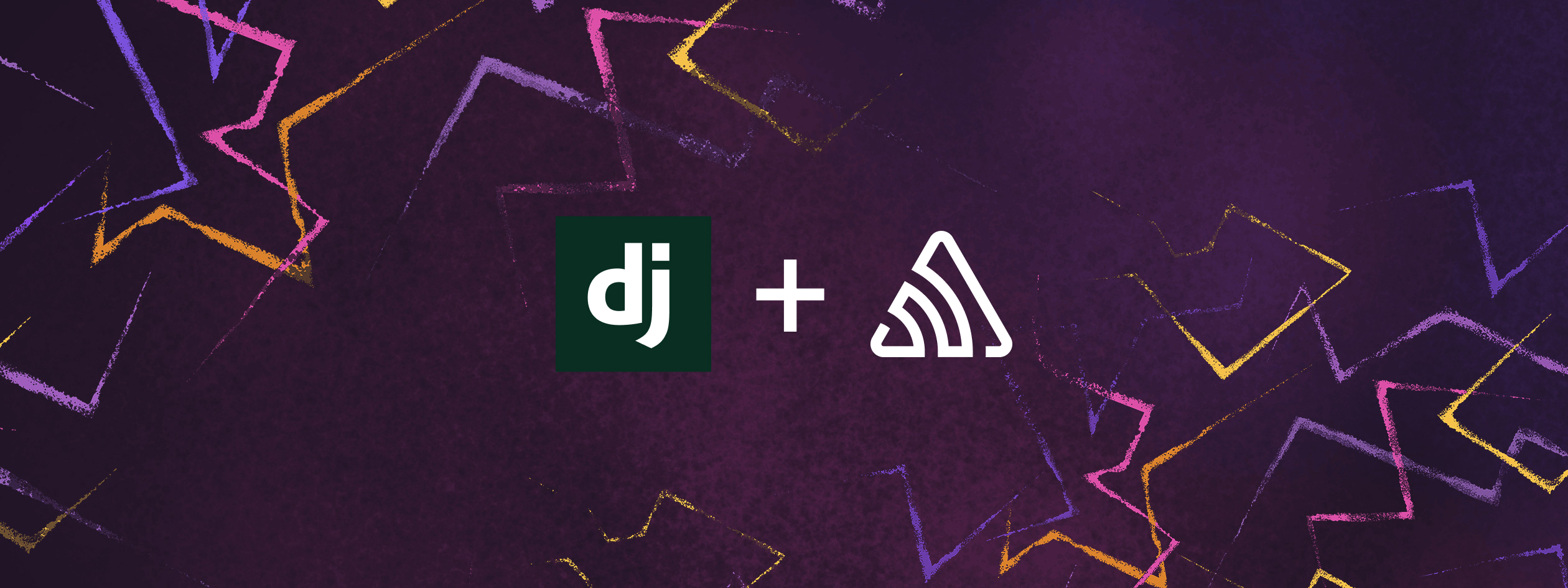
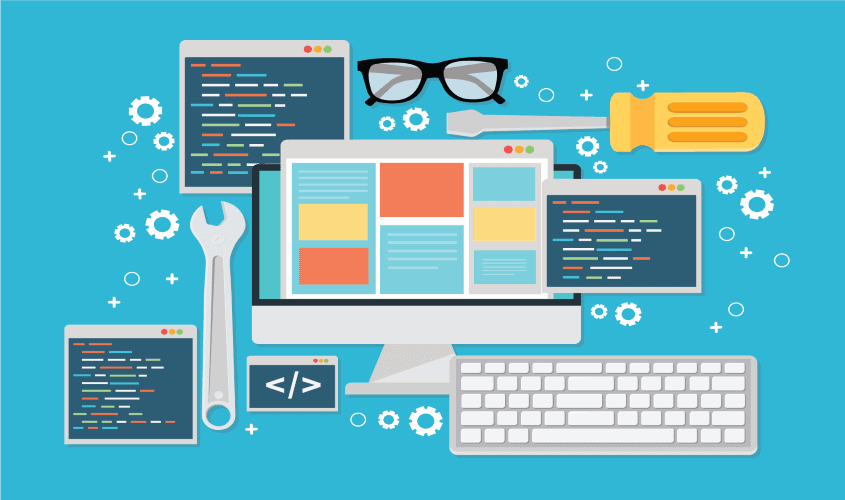
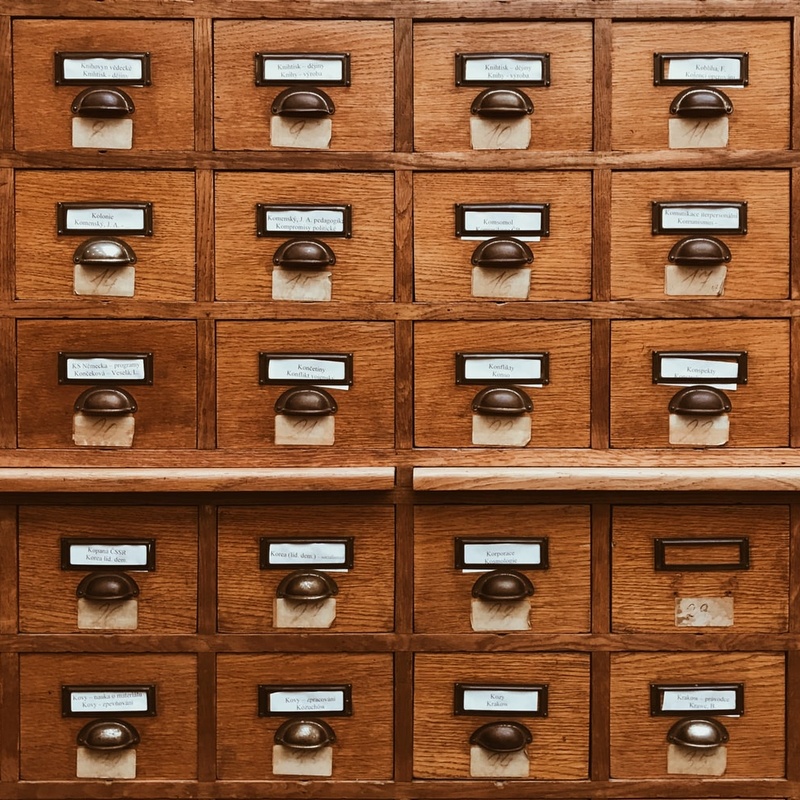
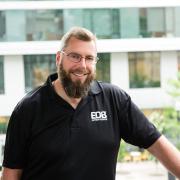
Article link: django read only database.
Learn more about the topic django read only database.
- Django and read-only database connections – Stack Overflow
- Multiple databases | Django documentation
- Working with an existing read-only database? : r/django – Reddit
- Prevent Unintended Data Modification With django-read-only
- Read-only connection to database. How to? – Google Groups
- Taking advantage of write-only and read-only connections …
- How to read and write a file using Django – etutorialspoint
- How to connect MySQL to Django – Javatpoint
- How to integrate Mysql database with Django? – GeeksforGeeks
- Multiple databases | Django documentation
- attempt to write a readonly database in django app hosting on …
See more: https://nhanvietluanvan.com/luat-hoc