Django Override Save Method
When working with Django models, we often come across situations where we need to customize the way objects are saved or updated in the database. This is where the save method comes into play. In this article, we will explore what the save method in Django is, how it works, and why you may need to override it. We will also discuss how to override the save method, common use cases, best practices, and alternatives available. So, let’s dive in!
What is the save method in Django?
——————————
In Django, the save method is a built-in function that allows you to save or update an object’s data in the database. It is defined in the base Model class, which provides a convenient interface for interacting with the database.
How does the save method work in Django models?
——————————————
The save method is automatically called whenever you create a new instance of a model or modify an existing one. It performs various operations behind the scenes, such as validating the data, generating SQL queries, and executing them to persist the changes in the database. By default, the save method handles all these tasks for you.
Why would you need to override the save method in Django?
—————————————————
While the default save method suffices for most scenarios, there are cases where you may want to customize the saving behavior. Some common reasons for overriding the save method include:
1. Modifying data before saving: You might need to manipulate or sanitize the data before it gets saved. For example, you could automatically generate a unique slug based on the object’s title or convert certain values to a specific format.
2. Implementing complex business logic: If your application has complex requirements for saving objects, such as updating related models or performing additional calculations, you can encapsulate this logic within the save method.
3. Auditing or logging purposes: Overriding the save method allows you to keep track of changes made to objects. You could record the user who made the modification, the timestamp of the change, or any other relevant information.
How to override the save method in Django models?
——————————————–
To override the save method, you simply need to define a new method with the same name in your model class. This method should accept two parameters: self and **kwargs. The self parameter refers to the current instance of the model, while **kwargs allows you to receive any additional keyword arguments that are passed by Django.
Let’s take a look at a simple example of overriding the save method:
“`python
from django.db import models
class MyModel(models.Model):
title = models.CharField(max_length=100)
def save(self, *args, **kwargs):
# Custom logic before saving
self.title = self.title.upper()
super().save(*args, **kwargs) # Call the original save method
“`
In this example, we are capitalizing the title field before saving it. We achieve this by converting the value to uppercase within the save method. After that, we call the original save method using the super() function to ensure that all the necessary database operations are performed.
Common use cases for overriding the save method in Django models
—————————————————————-
Let’s explore some real-world use cases where overriding the save method proves to be beneficial:
1. Slug generation: Instead of manually specifying a slug field, you can override the save method to automatically generate a slug based on the object’s title or any other relevant field. This can save developers from repetitive tasks and maintain data consistency.
2. File upload: When uploading files, you might want to save them to a specific directory or apply certain naming conventions. By overriding the save method, you can easily achieve this and customize the file-saving behavior according to your needs.
3. Logging changes: If you need to track changes made to models, such as historical records or auditing purposes, you can override the save method to create new log entries or update existing ones.
Best practices and considerations when overriding the save method in Django
———————————————————————–
Here are some best practices and considerations to keep in mind when overriding the save method in Django:
1. Consider the performance impact: Overriding the save method can introduce additional processing time, especially if your custom logic involves complex computations or database queries. Make sure to test the performance of your implementation and optimize it if necessary.
2. Preserve atomicity and data integrity: Ensure that your custom logic is designed in a way that it doesn’t compromise the atomicity of saving operations. You should also handle any potential failures gracefully to maintain data integrity.
3. Document your code: Overriding the save method can make your codebase more intricate. It’s essential to document your custom logic and provide clear comments explaining why you made those changes. This will help other developers understand your implementation and avoid confusion in the future.
Alternatives to overriding the save method in Django
————————————————
While overriding the save method is a powerful tool, it might not always be the best approach for your specific requirements. Here are some alternatives worth considering:
1. Override serializer save method: If you are using Django REST Framework and need to customize the saving behavior of your serializers, you can override the save method within the serializer class. This gives you more control over the serialization process without modifying the model itself.
2. Override form save method: When working with Django forms, you can override the save method of the form class to implement custom saving logic. This allows you to handle form data validation, modify the data, or perform additional operations before saving it.
Conclusion
———
In this article, we’ve explored the save method in Django, how it works, and why you may need to override it. We’ve discussed the process of overriding the save method and provided common use cases, best practices, and alternatives available. By understanding the power of customizing data saving, you can unlock new possibilities and tailor Django models to fit your unique application requirements.
How To Override Save Method In Django Model.
Keywords searched by users: django override save method Override save method Django, Override serializer save method Django, Override save form django, Save Django, Django admin custom save, Django model save get old value, Django model save update, Override form django
Categories: Top 85 Django Override Save Method
See more here: nhanvietluanvan.com
Override Save Method Django
Django, a popular Python web framework, provides a range of powerful features to help developers build robust and efficient web applications. One such feature is the ability to override the save method, allowing developers to add custom logic before or after saving an object to the database. In this article, we will delve into the details of overriding the save method in Django, explore its benefits, and address some common questions developers might have.
What is the save method in Django?
In Django, every model class inherits from the base models.Model class, which provides a save method. This save method is responsible for persisting the object’s state to the database. By default, the save method handles the insertion or update of the model instance, depending on whether it already exists in the database.
Why would you want to override the save method?
While the default save method in Django is sufficient for most use cases, there are scenarios where developers may want to customize the saving behavior. Here are a few common reasons:
1. Data validation: By overriding the save method, developers can perform additional data validation before saving an object. This ensures that data integrity is maintained and prevents invalid or inconsistent data from being stored.
2. Pre-processing or post-processing: The save method can be used to perform pre-processing or post-processing tasks on the object’s data. For example, you might want to automatically generate a slug based on the object’s title or update a timestamp field before saving.
3. Signals: Django signals allow decoupled applications to get notified when certain actions occur. By overriding the save method, developers can emit custom signals before or after saving an object, enabling other parts of the application to respond to these events.
How to override the save method in Django?
To override the save method, you need to define a new save method in your model class that incorporates your desired custom logic. Let’s take a look at a simple example:
“`python
from django.db import models
class MyModel(models.Model):
title = models.CharField(max_length=100)
slug = models.SlugField(unique=True)
def save(self, *args, **kwargs):
# Perform custom logic before saving
self.slug = self.title.replace(” “, “-“)
# Call the parent class’s save method
super().save(*args, **kwargs)
“`
In this example, we override the save method to automatically generate a slug based on the object’s title. We replace any spaces in the title with hyphens and assign the result to the slug field. After performing our custom logic, we call the parent class’s save method to persist the object to the database.
It’s important to note that if you override the save method, you’re responsible for ensuring that the object gets saved. Calling the parent class’s save method is crucial to avoid losing data.
Are there any considerations when overriding the save method?
While overriding the save method can add valuable functionality to your Django models, there are a few considerations to keep in mind:
1. Performance impact: Overriding the save method might introduce additional computations or database operations, which could affect the overall performance of your application. It’s essential to carefully consider the impact on performance and conduct appropriate testing, especially for large-scale applications.
2. Conditional saving: In some cases, you might want to conditionally save an object. For instance, you might only want to save an object if a specific field meets a certain condition. To achieve this, you can add conditional logic within the overridden save method.
3. Relationships and foreign keys: When overriding the save method, be cautious when dealing with relationships and foreign keys. Saving related objects within the overridden save method can lead to unexpected behavior or circular dependencies. It’s recommended to carefully consider the impact on your database schema and application design.
Frequently Asked Questions
Q1: Can I access the object’s old values when overriding the save method?
A: Yes, you can access the object’s old values using the `self._state.fields_cache` dictionary within the overridden save method. This dictionary contains the object’s fields and their previous values.
Q2: Can I prevent the save method from being called during specific operations?
A: Yes, you can prevent the save method from being called in certain scenarios. For example, you can use `self.pk` to check if the object has already been saved, and conditionally skip saving.
Q3: Can I override the save method for related objects?
A: Yes, you can override the save method for related objects, such as foreign keys or many-to-many relationships. However, you should be cautious when saving related objects within the save method to avoid potential issues.
Conclusion
Overriding the save method in Django allows developers to add custom logic before or after persisting an object to the database. It provides the flexibility to perform data validation, pre-processing, post-processing, and emit signals during the saving process. However, it’s essential to consider performance impact, handle conditional saving, and be cautious with relationships and foreign keys. By understanding how to override the save method and its considerations, developers can leverage Django’s flexibility to tailor the persistence behavior of their models to their specific needs.
Override Serializer Save Method Django
Django, an open-source Python framework, provides powerful tools for building web applications. One of the key features of Django is its built-in serialization framework, which allows developers to convert complex data types, such as Django models, into Python native datatypes. This enables data interchange between different systems and languages.
In many cases, the default behavior of Django’s serializer works perfectly fine. However, there are situations where you may need to customize the serialization process to meet your specific requirements. This is where the ability to override the serializer save method comes in handy. In this article, we will explore how to override the serializer save method in Django and discuss some common FAQs related to this topic.
What is the serializer save method?
Before diving into the specifics of overriding the serializer save method, let’s first understand what it does. When you use a serializer to create or update an object, the serializer’s save method is called. This method is responsible for saving the validated data as a new object or updating an existing object.
By default, when you call the serializer’s save method, it creates or updates an instance of the model associated with the serializer, based on the validated data. However, in some cases, you might want to perform additional operations before or after saving the data. This is where the ability to override the serializer save method becomes useful.
How to override the serializer save method?
To override the serializer save method, follow these steps:
1. Create a serializer class that extends the Django REST Framework’s Serializer class.
2. Define a save method within your serializer class.
3. Inside the save method, you can perform any additional operations you need before or after saving the data.
4. If you need to call the default implementation of the save method, make sure to include the super() call.
Here’s an example of how to override the serializer save method:
“`python
from rest_framework import serializers
class MySerializer(serializers.Serializer):
field1 = serializers.CharField()
field2 = serializers.IntegerField()
def save(self):
# Perform any additional operations here
# You can access validated data using self.validated_data
# For example:
custom_data = self.validated_data[‘field1’] + str(self.validated_data[‘field2’])
# Call the default implementation of the save method
super().save()
“`
In this example, we created a custom serializer named MySerializer that has two fields: field1 and field2. Inside the save method, we accessed the validated data and performed some additional operations. Finally, we called the default implementation of the save method using super().
FAQs about overriding the serializer save method in Django
Q: Why would I need to override the serializer save method?
A: There are various scenarios where overriding the serializer save method can be useful. For example, you may want to perform additional database operations, interact with external APIs, or customize the way data is saved.
Q: Can I override the save method for a specific serializer only?
A: Yes, you can override the save method for a specific serializer by creating a custom serializer class that extends the Serializer you want to modify.
Q: How can I access the validated data within the save method?
A: The validated data can be accessed using the self.validated_data attribute. It contains a dictionary with the validated field values.
Q: Can I perform additional validation within the save method?
A: Yes, you can perform any additional validation you need within the save method. You can raise ValidationError if the data is not valid.
Q: What happens if I don’t call the default implementation of the save method?
A: If you don’t call the default implementation of the save method, the object will not be saved to the database. It’s important to include the super() call to ensure the data is properly saved.
In conclusion, Django’s serializer save method provides a way to customize the serialization process according to your specific needs. By overriding this method, you can perform additional operations before or after saving the data, enabling you to create more flexible and tailored serialization logic. Remember to always include the super() call when overriding the save method to ensure the default behavior is preserved.
Hopefully, this article has provided you with a comprehensive understanding of how to override the serializer save method in Django. If you have any further questions or concerns, don’t hesitate to refer to the FAQs section or consult the official Django documentation for more detailed information. Happy coding!
Override Save Form Django
Django, one of the most popular Python frameworks for web development, offers a convenient way to handle form submissions with its built-in Form class. However, there are times when we need to go beyond the default behavior and customize the save process. In this article, we will explore the ways to override the save method in Django forms and provide a detailed explanation of each step. Additionally, we will address some frequently asked questions regarding this topic.
Before we dive into the details, it is important to understand the save method in Django forms. By default, the save method persists the form data into the database. When we create a new instance of a ModelForm, it automatically generates a save method behind the scenes. This default implementation saves the form data as a new record in the corresponding database table.
However, in certain scenarios, we may want to perform additional operations before or after saving the form data. For example, we might need to modify some fields, perform data validation, or trigger certain actions. Django allows us to override the save method in our form class to cater to these specific needs.
To begin with, let’s take a look at a simple example of overriding the save method in a Django form:
“`python
from django import forms
class MyForm(forms.Form):
name = forms.CharField(max_length=100)
email = forms.EmailField()
def save(self):
# Perform additional operations before saving
# …
# Call the super save method to save the form data
super().save()
# Perform additional operations after saving
# …
“`
In the above code snippet, we have created a basic form called `MyForm`, which has two fields: `name` and `email`. By overriding the save method, we can add any custom logic before and after saving the form.
When we override the save method, it is important to ensure that we call the super save method using `super().save()`. This ensures that the default behavior of saving the form data is preserved. If we omit this line, the data won’t be saved to the database.
Now that we have a basic understanding of how to override the save method, let’s dive deeper into the process and explore some common scenarios.
1. Modifying Field Values:
In some cases, we might need to modify the values of certain fields before saving the form data. We can do this by accessing the field values using the `.cleaned_data` attribute. For example:
“`python
def save(self):
# Modify the name field value
self.cleaned_data[‘name’] = self.cleaned_data[‘name’].upper()
super().save()
“`
In the above code snippet, we convert the `name` field value to uppercase before saving it.
2. Performing Validation:
Django provides a built-in validation mechanism that helps us ensure the data submitted through a form is valid. We can perform additional validation in the save method by raising a `ValidationError` if the data is not valid. For example:
“`python
from django.core.exceptions import ValidationError
def save(self):
if not self.cleaned_data[‘name’].startswith(‘A’):
raise ValidationError(‘Name must start with A.’)
super().save()
“`
In this example, we check if the `name` field value starts with ‘A’ and raise a validation error if not.
3. Triggering Actions:
Sometimes, we may need to trigger certain actions after saving the form data. For instance, we might want to send an email notification or update related records. In these cases, we can call the required actions within the save method. For example:
“`python
def save(self):
super().save()
# Send an email notification
send_email(self.cleaned_data[’email’], ‘Form submitted successfully.’)
“`
In the above code snippet, we call a hypothetical `send_email` function to send a notification email after saving the form.
Now, let’s address some frequently asked questions about overriding the save method in Django forms.
#### FAQs
1. Can I override the save method in a ModelForm?
Yes, the process of overriding the save method in a ModelForm is very similar to overriding a regular form. However, since ModelForms are tightly coupled with database models, it provides additional hooks to customize the saving process. For instance, we can override the `save()` method of the related model to perform additional logic before or after saving the form data.
2. How do I access the instance being saved within the save method?
To access the instance being saved within the save method, we can capture the returned instance by assigning the super save method call to a variable. For example:
“`python
def save(self):
instance = super().save()
# Access the saved instance
print(instance.id)
“`
In this example, we capture the returned instance and print its ID after saving.
3. Can I prevent the form from saving using the save method?
Yes, we can prevent the form from saving by overriding the save method and not calling the super save method. This effectively cancels the saving process. However, it is important to handle the consequences of not saving the form data in such cases.
In conclusion, overriding the save method in Django forms allows us to extend the default behavior and perform additional operations while saving form data. Whether modifying field values, performing validation, or triggering actions, this customization offers flexibility and control in handling form submissions. By following the examples and guidelines provided in this article, you can effectively override the save method in your Django forms and enhance your web applications.
Images related to the topic django override save method
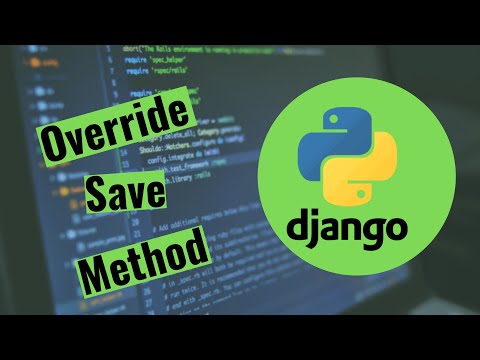
Found 36 images related to django override save method theme
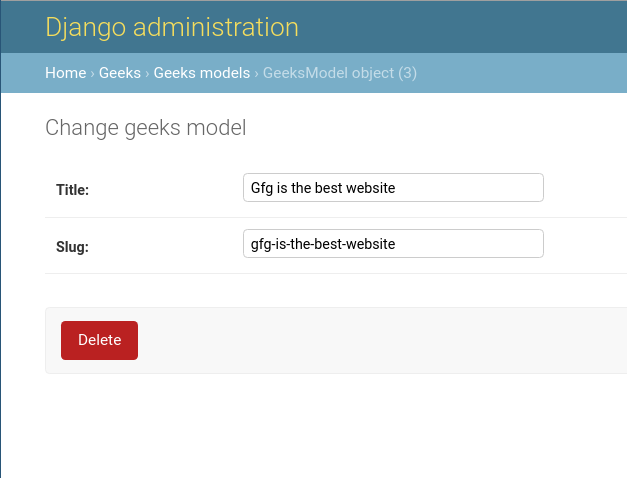

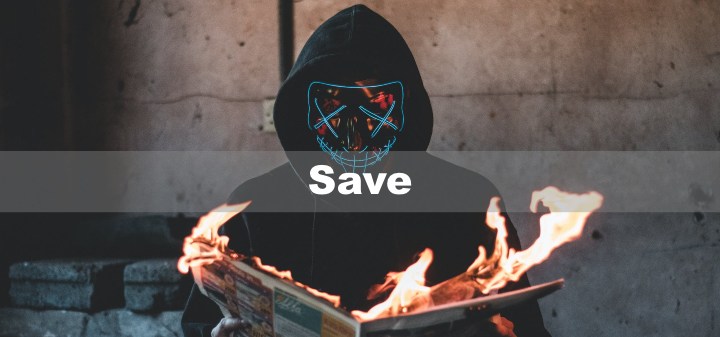
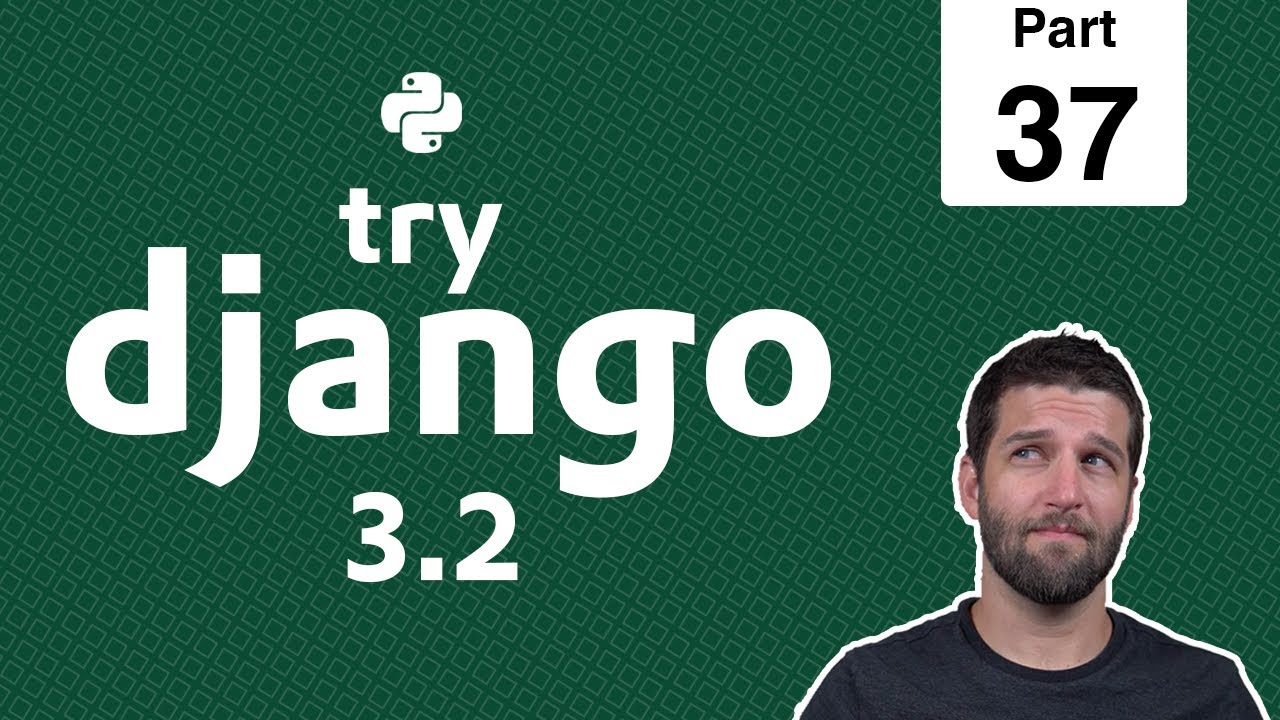
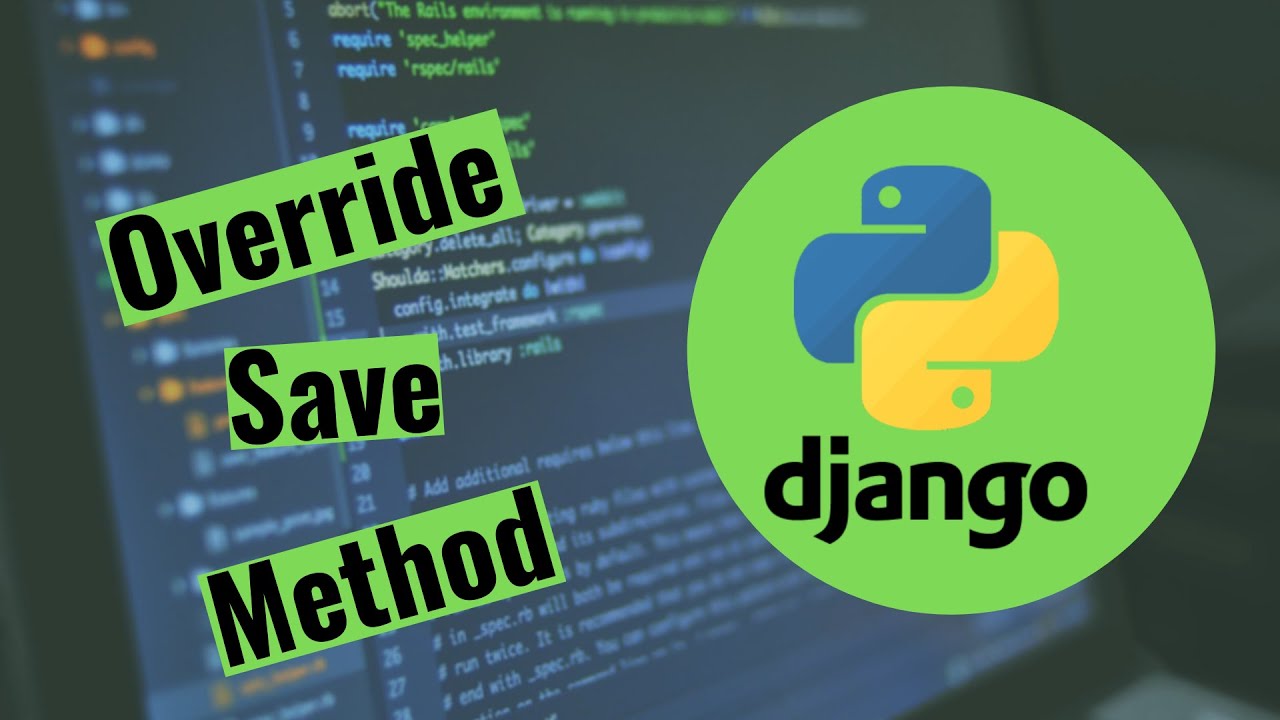




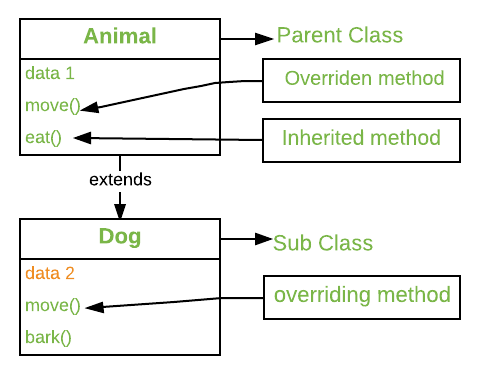
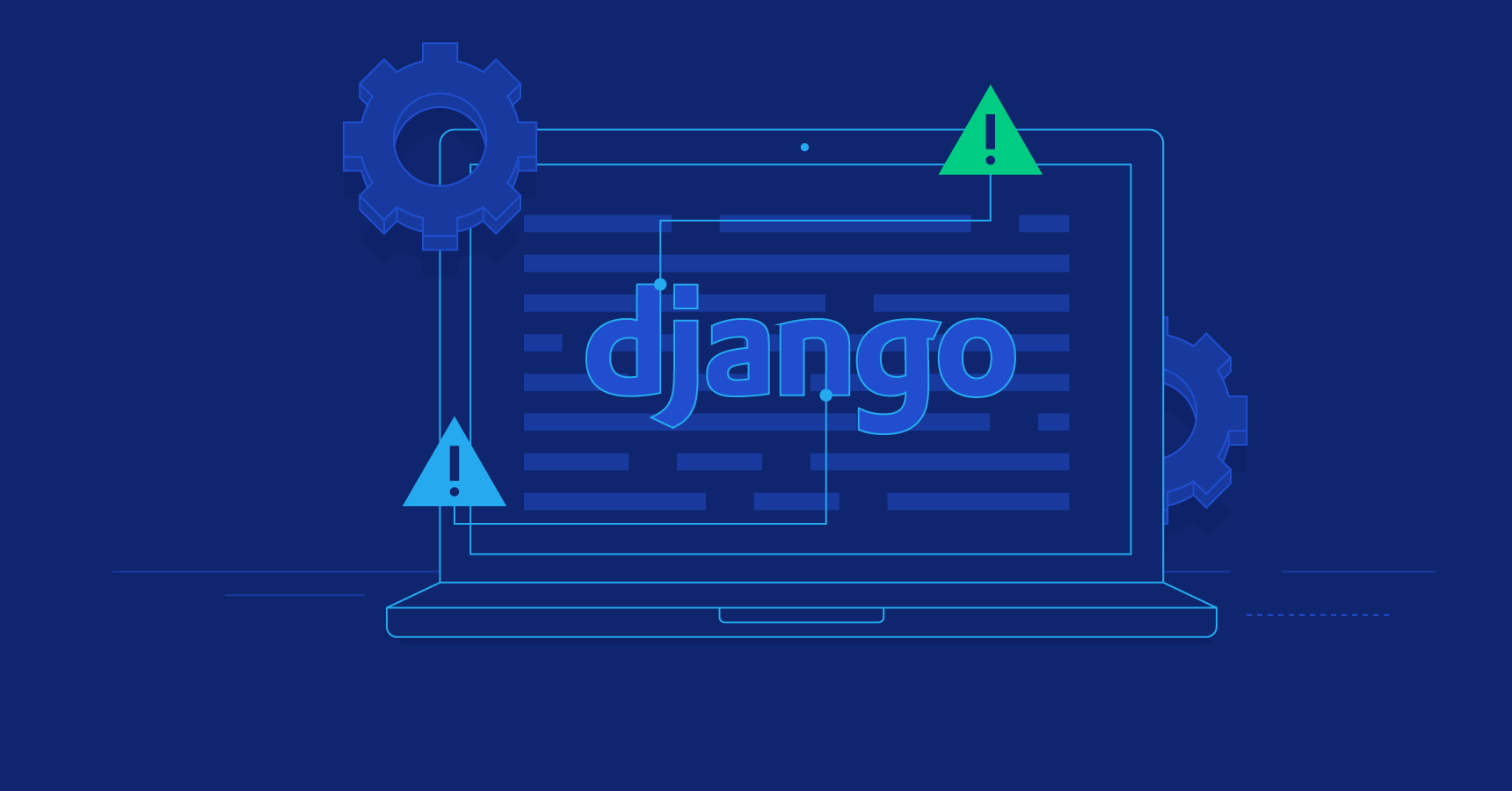
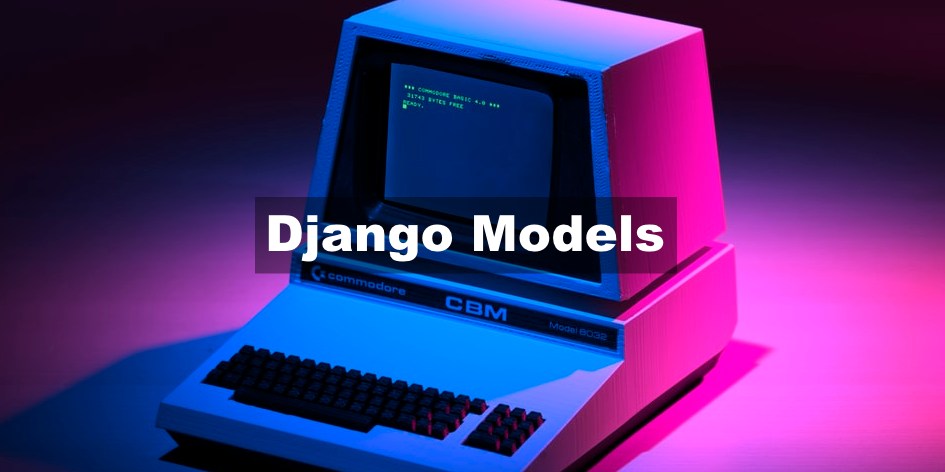

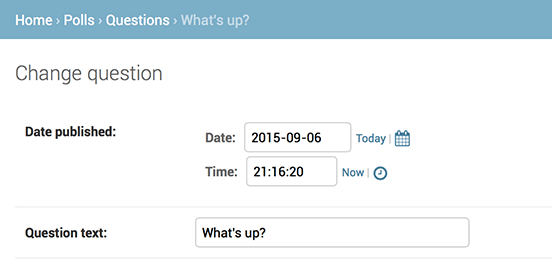
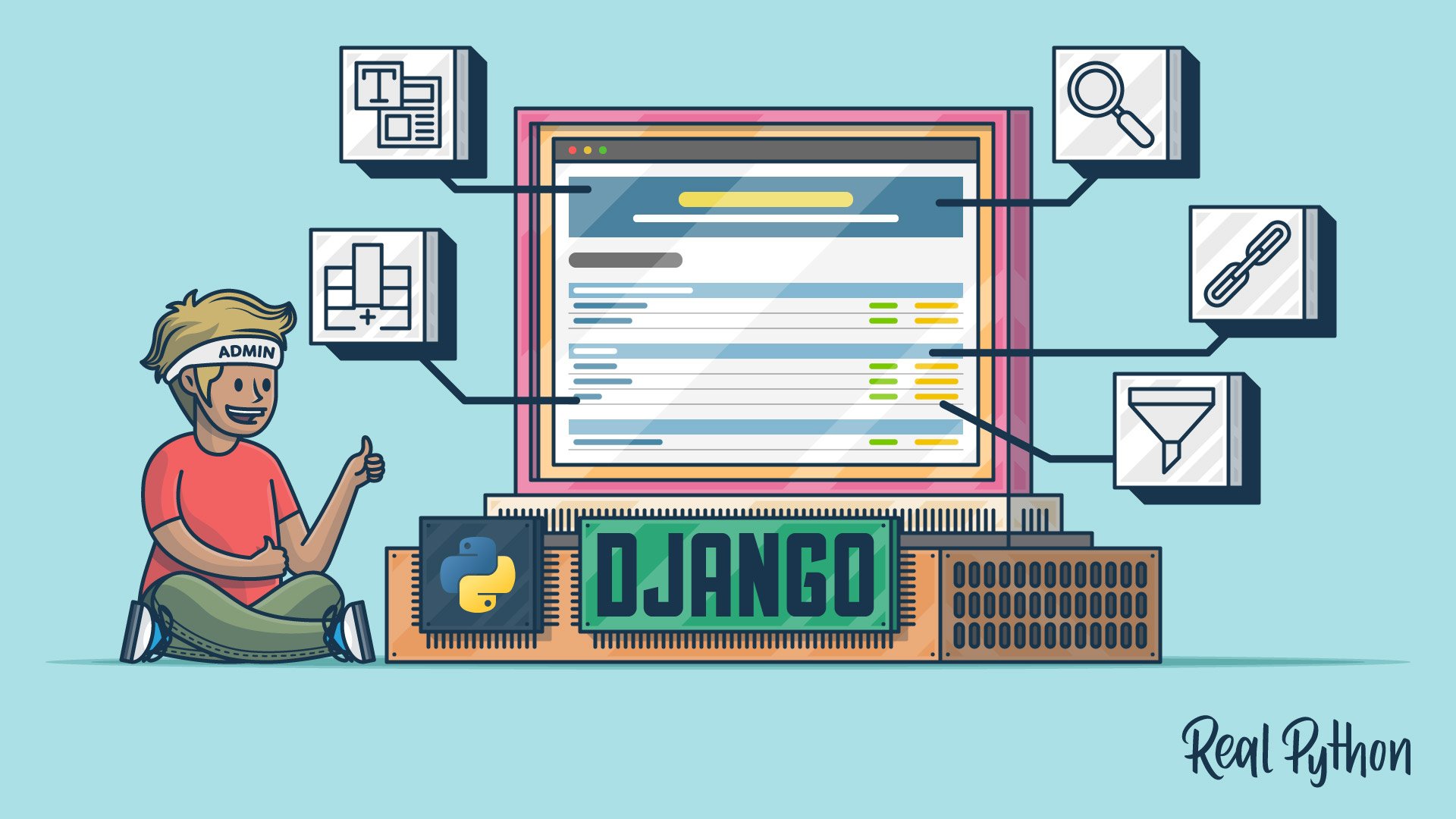
Article link: django override save method.
Learn more about the topic django override save method.
- Overriding the save method – Django Models
- Django override save for model only in some cases?
- Override Save Method Django Models
- How to override the save method in your Django models
- override model save method – Using the ORM
- 3. How to override save behaviour for Django admin?
- override save in model
See more: nhanvietluanvan.com/luat-hoc