Dereference Of A Possibly Null Reference
Introduction
In programming, a null reference refers to a variable or object that does not point to any value or memory location. Dereferencing, on the other hand, is the act of accessing the value or data stored at a particular memory location. However, when dereferencing a possibly null reference, there is a potential risk of encountering a null pointer exception. This article aims to explore the concept of dereferencing a possibly null reference, discuss the implications of null references, and provide best practices for handling such situations.
Possible Null References and Their Implications
When dealing with programming languages that allow null references, it is essential to understand the implications of such references. A null reference can lead to null pointer exceptions, which occur when a program attempts to access data or invoke a method on a null reference. This commonly results in runtime errors and crashes, making it a significant issue to address.
Null references can occur in various scenarios. For example, when a reference type variable is declared but not initialized or when a method returns a reference that may or may not be null. Additionally, when accessing elements of a collection or querying databases using LINQ, there is a possibility of encountering null references.
The consequences of null references can be severe. They can disrupt program flow, cause unexpected behaviors, and lead to frustration for both developers and end-users. Therefore, it is crucial to adopt techniques and practices that mitigate the risks associated with dereferencing null references.
Null-Safe Dereferencing Techniques
To avoid null pointer exceptions and safely dereference possibly null references, developers can employ several techniques. One such technique is null-safe dereferencing, which allows accessing properties or methods only if the reference is not null. Common examples of null-safe dereferencing include the null-conditional operator (?.) in C# and the safe navigation operator (?.) in languages like Kotlin and Groovy.
By utilizing null-safe dereferencing, developers can write concise and readable code while eliminating the risk of null pointer exceptions. For instance, instead of writing cumbersome null-checking code, null-safe dereferencing allows writing cleaner expressions like `myObj?.MyProperty` or `myObj?.MyMethod()`.
Conditional Dereferencing
Conditional dereferencing is another approach for handling null references. It involves explicitly checking if a reference is null before proceeding with dereferencing. This can be achieved using an “if” statement or a conditional operator like the ternary operator (?:). By performing the null check before accessing the reference, developers can prevent null pointer exceptions.
For example, in C#, one can write:
“`csharp
if (myObj != null)
{
// Dereference myObj safely here
}
“`
Or using the ternary operator:
“`csharp
var result = (myObj != null) ? myObj.SomeProperty : defaultValue;
“`
Using the Null-Coalescing Operator
The null-coalescing operator (??) is a powerful tool for handling null references. It allows assigning a default value to a variable if the reference is null. The operator works by evaluating the expression on the left side and returning its result if it is not null, or the value on the right side if the expression is null.
Here’s an example in C#:
“`csharp
string name = possiblyNullName ?? “Unknown”;
“`
In the above code, if `possiblyNullName` is null, the variable `name` will be assigned the default value “Unknown.” Otherwise, the value of `possiblyNullName` will be assigned to `name`. This provides a concise and effective way of handling null references while providing meaningful fallback values.
Best Practices for Handling Null References
When dealing with possibly null references, it is essential to follow best practices to minimize the risk of null pointer exceptions and improve code quality. Some recommendations include:
1. Initialize reference variables when declaring them.
2. Apply null-safe dereferencing techniques when accessing properties or invoking methods.
3. Utilize conditional dereferencing when necessary to explicitly check null references.
4. Use the null-coalescing operator to assign default values when dealing with null references.
5. Thoroughly test code that interacts with external systems such as databases, APIs, or file systems to ensure it handles null references correctly.
6. Enable null reference warnings and resolve any potential null reference issues flagged by the compiler.
7. Adopt defensive programming practices by validating user input and implementing appropriate error handling mechanisms to handle unexpected null references gracefully.
FAQs
Q: What is the dereference of a possibly null reference?
A: Dereferencing a possibly null reference refers to accessing the value or data stored at a memory location that may or may not be null. It is a potential risk that can lead to null pointer exceptions if not handled properly.
Q: How can I avoid null pointer exceptions when dereferencing null references?
A: To avoid null pointer exceptions, you can employ techniques such as null-safe dereferencing using operators like ?. (C#) or ?. (Kotlin), conditional dereferencing through null checks, and the use of the null-coalescing operator (??) to assign default values.
Q: What are the implications of null references?
A: Null references can lead to null pointer exceptions, which cause runtime errors and crashes in programs. They can disrupt program flow, result in unexpected behaviors, and affect the overall stability of software applications.
Q: What are best practices for handling null references?
A: Best practices include initializing reference variables when declaring them, utilizing null-safe dereferencing techniques, employing conditional dereferencing when necessary, using the null-coalescing operator to assign default values, enabling null reference warnings, thoroughly testing code, and adopting defensive programming practices.
Conclusion
Dereferencing a possibly null reference can be a significant concern for developers as it poses the risk of null pointer exceptions and runtime errors. By understanding the implications of null references, adopting null-safe dereferencing techniques, and following best practices, developers can ensure their code handles null references gracefully and maintains stability. By implementing these practices, developers can create more reliable and robust software, reducing the occurrence of null pointer exceptions and enhancing the overall user experience.
C# Nullable Reference Types – No More Null Reference Exceptions!
What Is Null Reference Dereference In C#?
In the world of programming, null reference dereference refers to an attempt to access or manipulate an object that has not been initialized or has been set to null. In C#, null is a special value that represents the absence of an object reference. When a null reference is dereferenced, it leads to a runtime exception, commonly known as a NullReferenceException.
Null reference dereference can be a common source of bugs and crashes in software applications. It occurs when a developer tries to perform an operation on an object that is null, expecting it to have a valid reference. Since null represents the absence of an object, attempting to access or modify its attributes or invoke its methods will result in an exception being thrown.
Understanding the NullReferenceException
A NullReferenceException is an unchecked exception in C# that is thrown when a null reference is accessed or dereferenced. When this exception occurs, it is typically an indication that the programmer made an error by assuming an object reference is valid when in fact it is null.
Let’s take a closer look at an example to better understand how null reference dereference and NullReferenceExceptions work in C#:
“`csharp
class Program
{
static void Main()
{
string message = null;
Console.WriteLine(message.Length);
}
}
“`
In this code snippet, we declare a string variable named “message” and set it to null. Then, we try to access the “Length” property of the string, assuming it to be a valid object reference. However, since “message” is null, a NullReferenceException will be thrown at runtime.
To avoid null reference dereference issues and NullReferenceExceptions, it is essential to ensure that object references are properly initialized before using them. One common practice is to perform null checks to verify if an object reference is null before accessing its members.
Handling Null References Safely
To prevent null reference dereferences and handle null references safely, several techniques can be employed in C#. Let’s explore these techniques:
1. Null Checking: Before accessing an object’s members, it is crucial to validate if the object reference is null. This can be done using an if statement or the null-coalescing operator (??).
“`csharp
if (message != null)
{
Console.WriteLine(message.Length);
}
“`
Alternatively, we can use the null-conditional operator (?.) introduced in C# 6 to handle null references more concisely:
“`csharp
Console.WriteLine(message?.Length);
“`
2. Conditional Statements: Using the conditional (ternary) operator can be useful when assigning a default value in case of a null reference.
“`csharp
int length = message != null ? message.Length : 0;
“`
3. Null Object Pattern: Applying the Null Object Pattern involves creating a specific class representing a null object that provides default behavior instead of throwing exceptions. This can help eliminate null reference dereference issues in some cases.
“`csharp
class NullString
{
public int Length => 0;
}
// Usage
string message = null;
var safeMessage = message ?? new NullString();
Console.WriteLine(safeMessage.Length); // Outputs 0
“`
FAQs about Null Reference Dereference in C#
Q: Is null a valid value in C#?
A: Yes, null is a valid value in C#. It represents the absence of an object reference.
Q: What is a NullReferenceException?
A: A NullReferenceException is a runtime exception in C# that occurs when attempting to access or dereference a null reference.
Q: How can I prevent null reference dereference issues?
A: To prevent null reference dereference, you can perform null checks before accessing an object’s members, use conditional statements, or implement the Null Object Pattern.
Q: Can I catch and handle a NullReferenceException?
A: Yes, you can catch and handle NullReferenceExceptions using try-catch blocks to gracefully handle null reference dereference issues in your code.
Q: Are there any best practices to avoid null reference dereferences?
A: Yes, some best practices include initializing object references properly, performing null checks, and following a defensive programming approach by validating user inputs to prevent null reference issues.
Conclusion
Null reference dereference is a common issue in C# programming that occurs when trying to access or manipulate an object that is null. This leads to a NullReferenceException being thrown at runtime. By understanding the concepts behind null reference dereference and employing techniques such as null checks, conditional statements, and the Null Object Pattern, programmers can prevent such issues and create more robust and reliable software applications.
How To Avoid Possible Null Reference C#?
Null reference errors can be frustrating and time-consuming to debug in any programming language, including C#. These errors occur when you try to access a member or property of an object that is null, resulting in a runtime exception. In this article, we will explore various techniques and best practices to avoid possible null reference errors in C#.
Understanding Null References
In C#, null is a special value that indicates the absence of an object reference. It is often assigned to variables to denote that they do not currently refer to any object. When you attempt to access a member or property of a null reference, the system raises a NullReferenceException.
The following example demonstrates how a null reference error can occur:
“`csharp
string name = null;
int length = name.Length; // NullReferenceException will be thrown here
“`
To avoid null reference errors, it is important to follow some guidelines and defensive programming techniques.
1. Check for null before accessing members or properties
Before accessing any member or property of an object, it is crucial to ensure that the object reference is not null. This can be done using a simple null check:
“`csharp
if (name != null)
{
int length = name.Length;
}
“`
By validating the object reference before accessing its members, you can prevent null reference exceptions.
2. Use the null-conditional (`?.`) operator
C# 6 introduced the null-conditional (`?.`) operator, which simplifies null checks and member access. It allows you to directly access members or properties of an object reference, while implicitly checking for null:
“`csharp
int? length = name?.Length;
“`
In this example, if `name` is null, the result will be null rather than throwing an exception.
3. Leverage the null-coalescing (`??`) operator
The null-coalescing (`??`) operator provides a concise way to assign a default value when a null reference is encountered. It allows you to specify an alternative value if the object reference is null:
“`csharp
int nonNullLength = name?.Length ?? 0;
“`
In this case, if `name` is null, the length will be assigned as 0.
4. Initialize variables properly
To avoid null references, it is important to initialize variables appropriately. When declaring reference-type variables, ensure they are assigned valid objects before using them. If the variable cannot be assigned a value immediately, consider using nullable reference types or explicitly declaring them as nullable:
“`csharp
string? name = null; // Nullable reference type
string? name; // Explicitly declared nullable
“`
By using nullable reference types, you are forced to handle null cases explicitly, reducing the chances of null reference exceptions.
5. Use debugging tools to detect null references
Modern Integrated Development Environments (IDEs) provide powerful debugging tools to identify null references. Utilize features like breakpoints and watch windows to observe variables’ values during runtime. This helps you identify possible sources of null references and allows you to fix them before any runtime issues occur.
6. Write unit tests to catch null reference errors
Incorporating unit tests in your development process can help catch null reference errors upfront. By writing tests that check for null references in critical areas of your code, you can ensure that your application remains stable and reliable.
Frequently Asked Questions (FAQs):
Q: What causes a null reference error?
A: A null reference error occurs when you try to access a member or property of an object that is null.
Q: How can I avoid null reference errors in C#?
A: To avoid null reference errors in C#, you can follow several techniques, including proper null checks, using the null-conditional and null-coalescing operators, initializing variables properly, leveraging debugging tools, and writing unit tests to catch null references.
Q: Can nullable reference types help prevent null reference errors?
A: Yes, by using nullable reference types, you are forced to handle null cases explicitly, reducing the chances of null reference errors.
Q: Is there any potential performance impact from using null checks in C#?
A: Null checks in C# have negligible performance impact. The C# compiler and runtime optimize null checks efficiently, ensuring minimal overhead.
Q: Are there any code analysis tools available to detect null references in C#?
A: Yes, there are various code analysis tools available, such as ReSharper and SonarQube, that can detect null references and offer suggestions for improvement in your code.
In conclusion, null reference errors can be a source of frustration in C#. By following the techniques mentioned in this article, such as proper null checks, utilizing null-conditional and null-coalescing operators, initializing variables correctly, leveraging debugging tools, and writing unit tests, you can significantly reduce the likelihood of encountering null reference errors. Remember, prevention is better than debugging!
Keywords searched by users: dereference of a possibly null reference Dereference of a possibly null reference, Dereference of a possibly null reference linq, converting null literal or possible null value to non-nullable type, possible null reference return warning, CS8604, Non nullable property must contain a non null value when exiting constructor, Cannot convert null literal to non nullable reference type, Warning cs8604 Possible null reference argument for parameter ‘s in int int parse string ‘s
Categories: Top 97 Dereference Of A Possibly Null Reference
See more here: nhanvietluanvan.com
Dereference Of A Possibly Null Reference
Introduction
In the realm of computer programming and software development, the concept of dereferencing a pointer or reference is a critical operation. Dereferencing refers to accessing the information stored at the memory address pointed to by a reference or pointer variable. However, when dealing with a possibly null reference, this operation can be a source of potential bugs and crashes if not handled correctly. In this article, we will delve into the intricacies of dereferencing null references, discussing the dangers they pose and the best practices to mitigate these risks.
Understanding Null References
A null reference, also known as a null pointer, is a special value that is assigned to a pointer or reference variable when it doesn’t point to any valid memory address. Instead, it indicates the absence of an object or the variable not being assigned to any memory location. Dereference of a null reference occurs when attempting to access or retrieve a value from a null pointer, leading to undefined behavior.
Dangers of Dereferencing Null References
Dereferencing a possibly null reference can introduce various hazards to a software system. Some of the potential consequences include:
1. Null Pointer Exceptions: When attempting to dereference a null pointer, a Null Pointer Exception (NPE) is thrown. This exception can halt the execution of the program and cause crashes that impact user experience. Tracking down the specific location where the NPE is thrown can be challenging, especially in large codebases, making debugging a time-consuming and complex process.
2. Impacted System Stability: Dereferencing a null reference can destabilize the entire system. A crash caused by a null reference can lead to the loss of unsaved data and disrupt the normal flow of operations. Moreover, if the null reference occurs in a critical part of the code, it may render the entire system unusable until the bug is identified and fixed.
3. Security Vulnerabilities: Null reference bugs have been exploited by attackers to gain unauthorized access, manipulate data, or execute arbitrary code. These vulnerabilities can give rise to security breaches. Therefore, it is crucial to implement proper null reference checks and handle them securely.
Best Practices for Handling Null References
To tackle the risks associated with dereferencing null pointers, developers should adhere to the following best practices:
1. Null Checking: Prior to dereferencing any reference or pointer, perform a null check to ensure it is not null. By incorporating such protective measures, developers can prevent Null Pointer Exceptions. For instance, using conditional statements like “if (reference != null)” can help intercept null references and avoid potential crashes.
2. Defensive Programming: Employing a defensive programming approach can significantly reduce the impact of null reference bugs. By validating inputs, documenting assumptions, and incorporating exception handling, the code can be fortified against unintended null pointer dereferences.
3. Use Null Object Pattern: In certain scenarios, using the Null Object Pattern can provide a graceful solution. Rather than using null references, objects representing the absence of a value are created and used instead. These null objects ensure consistency and eliminate the need for numerous null checks throughout the codebase.
4. Clear Error Messages: When a null value is detected, it is essential to provide clear and informative error messages. Such messages can simplify the debugging process, making it easier for developers to locate and fix the issue quickly.
Frequently Asked Questions (FAQs)
Q1. Why do null references exist?
Null references exist to represent a scenario where a variable or reference has not been assigned to any memory location or does not point to any valid object. They allow developers to handle cases where an expected object does not exist or is not yet available.
Q2. Are null references only a concern in specific programming languages?
Null references can be a concern in many programming languages, including Java, C++, C#, and others. However, some modern languages, like Rust, Kotlin, and Swift, employ alternative strategies to null references, reducing their potential dangers.
Q3. Can all null references be avoided?
While efforts can be made to minimize the presence of null references, completely avoiding them in large codebases can be challenging. Due to the complexity and dynamics of software systems, certain cases may necessitate handling null references. Careful null checking and robust exception handling can help mitigate risks associated with null dereferencing.
Q4. How to effectively debug null reference issues?
Debugging null reference issues can be challenging. Using debugging tools provided by the integrated development environment (IDE) can help identify the exact location where the null reference exception is thrown. Additionally, logging frameworks can be utilized to capture relevant information, making it easier to analyze the issue.
Conclusion
Dereferencing a possibly null reference can be a perilous operation if not handled scrupulously. Null pointer exceptions, system instability, and security vulnerabilities are some of the risks associated with dereferencing null references. By following best practices such as null checking, leveraging defensive programming techniques, and using the Null Object Pattern, developers can significantly reduce the likelihood and impact of null reference bugs. With proper handling and precautions, software systems can be safeguarded against the dangers posed by dereferencing null references.
Dereference Of A Possibly Null Reference Linq
Introduction:
In software development, the term “dereference” refers to the act of accessing the value of a memory address, typically through a pointer or a reference. Dereferencing becomes particularly crucial when dealing with object-oriented programming languages like C# and using LINQ (Language Integrated Query) to query data collections. One critical challenge developers face is handling possibly null references during LINQ operations. In this article, we will delve into the importance of dereferencing null references in LINQ and discuss the best practices to ensure robust and error-free code.
Understanding Null References in LINQ:
In LINQ, queries are performed on data collections using the built-in extension methods and lambda expressions. However, when these collections potentially contain null references, executing LINQ operations without checking for null values can lead to runtime exceptions like NullReferenceException.
Null references occur when a reference-type variable doesn’t refer to an object in memory but instead points to a null value. Thus, attempting to access members or properties of such null references directly can pose a significant risk. To mitigate this, developers must take necessary precautions to dereference possibly null references.
Best Practices for Dereferencing Null References in LINQ:
1. Null Checking:
Before executing any LINQ operations, it is essential to check for null references. A simple if condition on the collection or object can prevent unnecessary errors. For example:
“`
if (collection != null)
{
// Perform LINQ operations here
}
“`
By ensuring the collection is not null, we protect subsequent LINQ operations from encountering a NullReferenceException.
2. Null Conditional Operator:
Introduced in C# 6.0, the null conditional operator (?.) simplifies null checking and dereferencing. It allows accessing members or properties only if the reference is not null. For instance:
“`
var result = collection?.Where(item => item.Property == value).ToList();
“`
This approach eliminates the need for explicit null checks and provides a concise way of performing LINQ operations safely.
3. DefaultIfEmpty() Method:
When performing LINQ operations, a common requirement is to handle empty collections or null results gracefully. The DefaultIfEmpty() method can be used to provide a default value if the collection is empty or null. Example:
“`
var result = collection.DefaultIfEmpty(defaultValue).Where(item => item.Property == value).ToList();
“`
By using this method, developers can ensure that LINQ operations continue smoothly even when encountering empty collections or null references.
4. Safe Navigation Operator:
In C# 6.0, the safe navigation operator (?.) can be extended by using the null coalescing operator (??) to provide a default value instead of null. This technique is particularly useful when working with nullable reference types to ensure safe and predictable results.
5. Defensive Programming:
To further enhance code robustness, consider adopting defensive programming techniques. This includes rigorous parameter and input validation, utilizing exception handling mechanisms, and implementing proper error logging. By actively preventing errors and handling exceptional cases, developers can mitigate the risks associated with null references in LINQ.
FAQs:
Q: What is the significance of dereferencing null references in LINQ?
A: Dereferencing null references is crucial to avoid runtime exceptions like NullReferenceException during LINQ operations. By properly handling null references, developers ensure robust code execution.
Q: What are the potential risks of ignoring null references in LINQ?
A: Ignoring null references can result in runtime exceptions, causing application crashes and unexpected behavior. Furthermore, if null references are inadvertently used in calculations or comparisons, it may lead to invalid results.
Q: Are there any performance implications associated with null reference handling in LINQ?
A: While null reference handling introduces additional code, the performance impact is generally negligible. Modern optimizing compilers and efficient LINQ query execution minimize any potential overhead.
Q: Can null reference handling be applied to LINQ expressions involving multiple collections?
A: Yes, developers can apply null reference handling techniques to LINQ expressions involving multiple collections. By checking null references on all involved collections and applying appropriate null-safe operators, developers can ensure correctness and avoid exceptions.
Q: Are there any built-in methods in LINQ for handling null references?
A: LINQ provides several built-in methods like DefaultIfEmpty() that help handle null references and empty collections more gracefully. These methods are specifically designed to handle such scenarios.
Conclusion:
Dereferencing possibly null references in LINQ is an essential practice to ensure safe and reliable software development. By following the best practices outlined in this article, such as null checking, using the null conditional operator, employing the default value mechanism, and implementing defensive programming, developers can mitigate the risks associated with null references. With proper null reference handling, the code becomes more robust, improves user experience, and avoids potential runtime exceptions in LINQ operations.
Converting Null Literal Or Possible Null Value To Non-Nullable Type
In programming, handling null values can often be a challenge, especially when working with non-nullable types. In this article, we will explore the concept of converting null literals or possible null values to non-nullable types. We will delve deeper into the topic, discussing its significance and providing insights on how to effectively handle this scenario. Additionally, we will address some frequently asked questions to provide a comprehensive understanding.
What are Non-Nullable Types?
Non-nullable types, also known as value types, are variables that cannot hold null values. These types are explicitly declared to never be null, ensuring that they always contain a valid value. Examples of non-nullable types in many programming languages include integers, floats, and booleans.
The Challenge of Null Values
Null values represent the absence of a value or an uninitialized object reference. They can cause serious issues when used inappropriately with non-nullable types, as they may lead to runtime errors. These errors occur when a null value is assigned to a non-nullable type, resulting in a null reference exception or similar error.
Converting Null Literal to Non-Nullable Type
To convert a null literal to a non-nullable type, a developer needs to handle this situation proactively. One common approach is to use conditional statements, such as if-else or ternary operators. By checking whether the value is null before assigning it to a non-nullable type, developers can prevent unwanted exceptions.
Let’s consider an example using C#:
“`csharp
string nullableString = null;
string nonNullableString = nullableString ?? “default value”;
“`
In the above code snippet, we assign a null value to a nullable string. However, before assigning it to a non-nullable string, we utilize the null-coalescing operator (??). This operator allows us to choose a default value if the nullable string is null. In this case, “default value” will be assigned to the non-nullable string.
Converting Possible Null Value to Non-Nullable Type
Sometimes, a value may be nullable based on certain conditions or input from external sources. To convert such possible null values to non-nullable types, developers can adopt defensive programming techniques. Defensive programming involves validating and sanitizing the input before assigning it to non-nullable variables.
For instance, consider a scenario where a user enters their age, and the input could be null if they choose not to provide it. We can use conditional statements to ensure the value is not null before assigning it to a non-nullable integer. We can also display an appropriate error message if the input is invalid.
“`csharp
int? userInputAge = GetAgeInput(); // Assume GetAgeInput() returns nullable int
int nonNullableAge;
if (userInputAge.HasValue)
{
nonNullableAge = userInputAge.Value;
}
else
{
Console.WriteLine(“Age cannot be null, please provide a valid input.”);
return;
}
“`
In the above example, we use the `.HasValue` property provided by nullable types to check if the userInputAge contains a value. If it does, we use the `.Value` property to extract the value and assign it to the non-nullable variable. If the userInputAge is null, we display an error message and exit the function.
Frequently Asked Questions
Q: What are the benefits of using non-nullable types?
A: Non-nullable types provide a way to enforce the existence of a valid value, reducing the likelihood of null reference exceptions at runtime. They make code more robust, as developers can rely on the presence of a value when working with these types.
Q: Can I convert a non-nullable type to a nullable type?
A: Yes, it is possible to convert a non-nullable type to a nullable type. This conversion is referred to as “boxing” or “wrapping.” However, it is important to note that the process may involve potential loss of performance or increased memory usage, as the value needs to be boxed within a nullable container.
Q: How can I handle null values efficiently in a large codebase?
A: In a large codebase, it is crucial to establish a solid error-handling strategy. Consider using defensive programming techniques mentioned earlier, such as verifying inputs for null values, to ensure the presence of valid data. Additionally, adopting design patterns like null object patterns or Option types can be helpful for dealing with null values more effectively.
Q: Are there any language-specific tools or features that aid in handling null values?
A: Yes, several programming languages offer tools and features that assist in handling null values. For instance, C# provides nullable value types, null coalescing operator (??), and null conditional operator (?.), all of which are designed to simplify null value handling.
In conclusion, converting null literals or possible null values to non-nullable types is an essential aspect of robust programming. By employing conditional statements, defensive programming techniques, and adopting language-specific features, developers can effectively handle null values, significantly reducing runtime exceptions.
Images related to the topic dereference of a possibly null reference
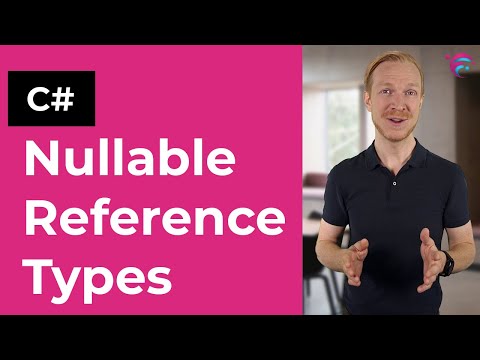
Found 12 images related to dereference of a possibly null reference theme



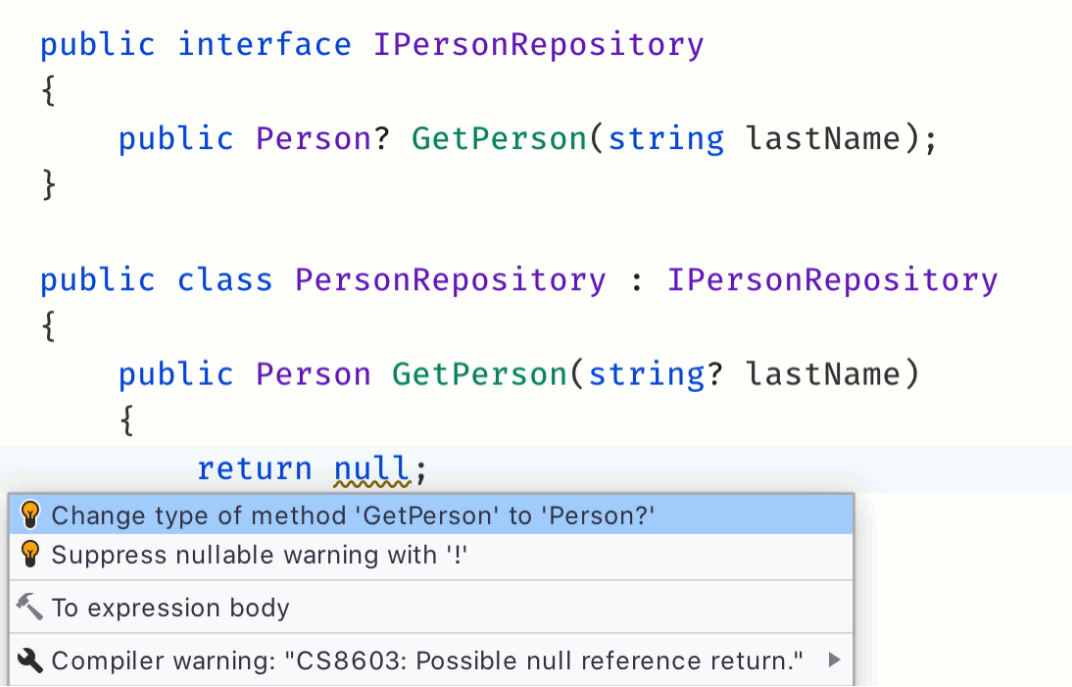
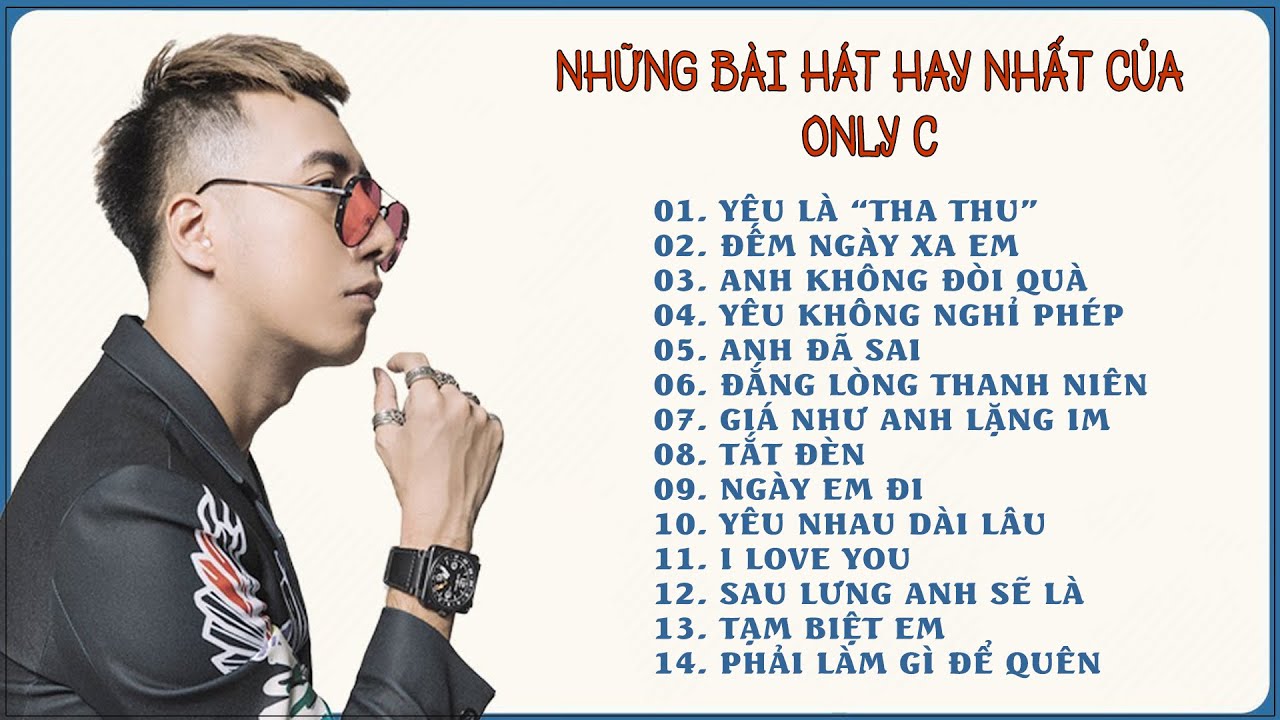
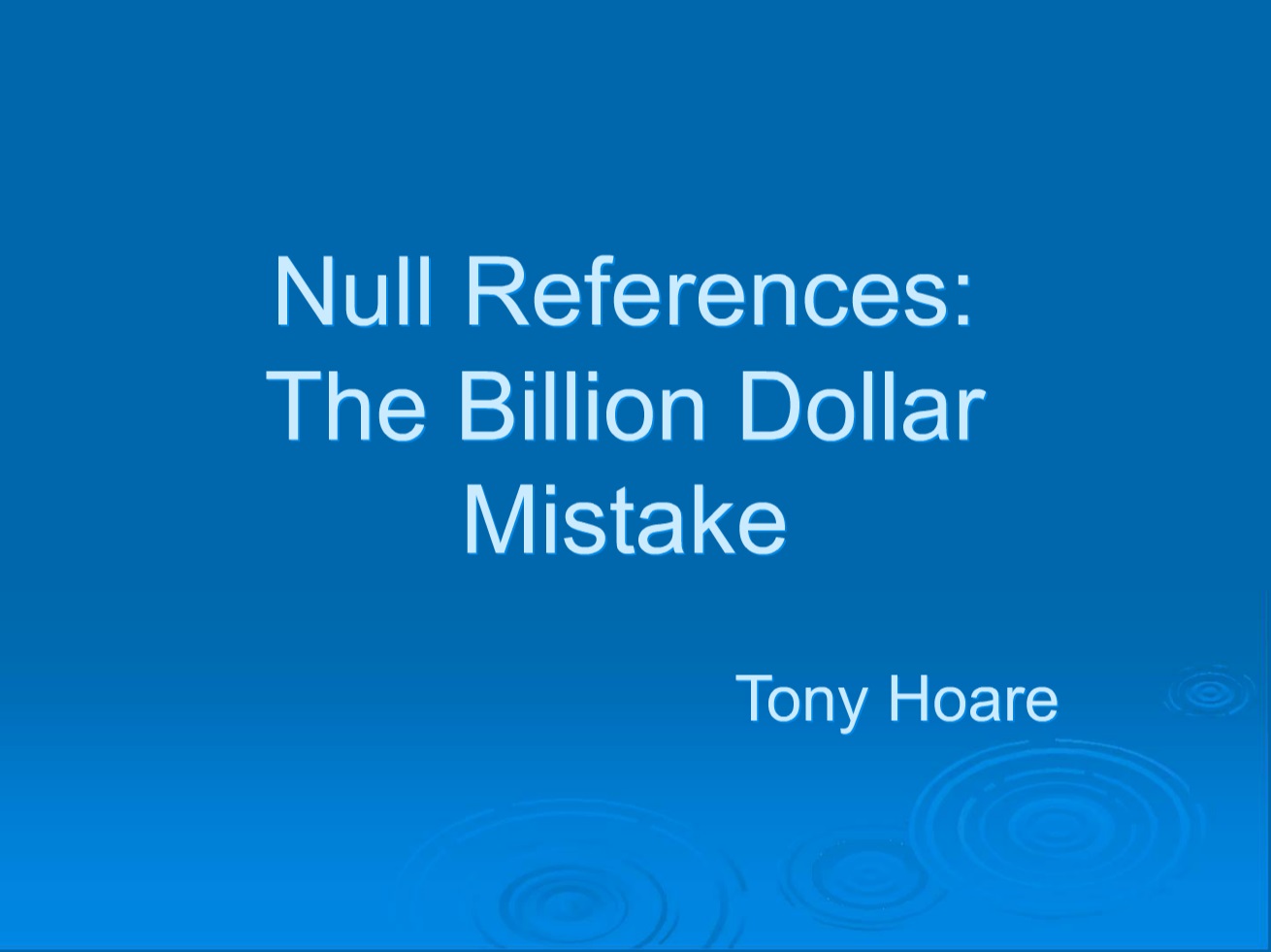

Article link: dereference of a possibly null reference.
Learn more about the topic dereference of a possibly null reference.
- Resolve nullable warnings | Microsoft Learn
- Why do we get possible dereference null reference warning …
- Approaches for Resolving Nullable Warnings on Existing …
- Avoid NULL Pointer Dereference (C#, VB.NET) | CAST Appmarq
- C# NullReferenceException | How to Avoid … – eduCBA
- What is NullReferenceException in C#? – Code Maze
- Is null reference possible? – c++ – Stack Overflow
- Unexpected Dereference of a possibly null reference warning
- Possible null reference assignment error on FirstOrDefault
- Nullable reference types in C#
- Dereference of a possibly null reference in Entity Framework 6 …
- NullReferenceException in C#. What is it and how to fix it?
See more: https://nhanvietluanvan.com/luat-hoc