Delete File In C#
Deleting files is a common operation in programming, and being able to do so efficiently and reliably is crucial. In this article, we will explore how to delete files using C# and discuss best practices for handling this task. We will cover topics such as checking if a file exists, deleting single and multiple files, handling read-only files, distinguishing between permanent deletion and sending files to the Recycle Bin, performing deletion safely, and following best practices for file deletion. Let’s dive in!
Checking if a File Exists
Before attempting to delete a file, it is wise to check if the file actually exists. This prevents unwanted errors and ensures that only existing files are targeted for deletion. C# provides a convenient method called `File.Exists()` that allows us to check if a file exists in the given file path. Let’s see how it can be used:
“`
string filePath = “C:\\path\\to\\file.txt”;
if (File.Exists(filePath))
{
// File exists, proceed with deletion
}
else
{
// File does not exist, provide appropriate feedback to the user
}
“`
Utilizing this approach helps us avoid exceptions and provides a way to handle situations where a file does not exist.
Deleting a File
Once we have confirmed that the file exists, we can proceed with deleting it. In C#, the `File.Delete()` method is used to remove a file from the file system. Here’s an example of how to delete a file:
“`
string filePath = “C:\\path\\to\\file.txt”;
if (File.Exists(filePath))
{
File.Delete(filePath);
Console.WriteLine(“File deleted successfully”);
}
else
{
Console.WriteLine(“File does not exist”);
}
“`
It is important to note that the `File.Delete()` method throws an exception if the file is read-only, locked by another process, or if the user does not have sufficient permissions to delete the file, among other scenarios. We will discuss how to handle these exceptions in the next section.
Handling Exceptions and Potential Errors
When attempting to delete a file, various exceptions can occur. For example, if a file is read-only, the `UnauthorizedAccessException` exception is thrown. Similarly, a `FileNotFoundException` is thrown if the file does not exist. It is crucial to handle these exceptions appropriately and provide meaningful feedback to the user.
Consider the following example, which handles some common exceptions:
“`
try
{
string filePath = “C:\\path\\to\\file.txt”;
if (File.Exists(filePath))
{
File.Delete(filePath);
Console.WriteLine(“File deleted successfully”);
}
else
{
Console.WriteLine(“File does not exist”);
}
}
catch (UnauthorizedAccessException)
{
Console.WriteLine(“You do not have permission to delete this file”);
}
catch (FileNotFoundException)
{
Console.WriteLine(“File not found”);
}
catch (Exception ex)
{
Console.WriteLine($”An error occurred: {ex.Message}”);
}
“`
By using try-catch blocks, we can gracefully handle various exceptions and provide helpful feedback to the user.
Understanding the Permissions Required for File Deletion
To delete a file, the user must have appropriate permissions. If a file is read-only, locked by another process, or the user does not have write access to the file, deleting it will result in an exception. It is important to consider these scenarios and handle them gracefully.
To delete read-only files, we can modify the file attributes to allow deletion programmatically. Here’s an example:
“`
string filePath = “C:\\path\\to\\file.txt”;
if (File.Exists(filePath))
{
// Check if the file is read-only
if ((File.GetAttributes(filePath) & FileAttributes.ReadOnly) == FileAttributes.ReadOnly)
{
// Remove the read-only attribute
File.SetAttributes(filePath, File.GetAttributes(filePath) & ~FileAttributes.ReadOnly);
}
// Proceed with deletion
File.Delete(filePath);
Console.WriteLine(“File deleted successfully”);
}
else
{
Console.WriteLine(“File does not exist”);
}
“`
By removing the read-only attribute, we ensure that the file can be deleted successfully. However, it is crucial to inform the user about this modification and provide any necessary warnings or instructions.
Confirming Successful Deletion with Appropriate Feedback
After deleting a file, it is essential to provide appropriate feedback to the user. This helps them understand whether the deletion was successful or if any errors occurred. By using the appropriate try-catch blocks and handling exceptions, we can display meaningful messages for different scenarios.
For example:
“`
try
{
string filePath = “C:\\path\\to\\file.txt”;
if (File.Exists(filePath))
{
File.Delete(filePath);
Console.WriteLine(“File deleted successfully”);
}
else
{
Console.WriteLine(“File does not exist”);
}
}
catch (UnauthorizedAccessException)
{
Console.WriteLine(“You do not have permission to delete this file”);
}
catch (FileNotFoundException)
{
Console.WriteLine(“File not found”);
}
catch (Exception ex)
{
Console.WriteLine($”An error occurred: {ex.Message}”);
}
“`
By providing clear and concise feedback, users can easily understand the outcome of the deletion operation.
Deleting Multiple Files
In some cases, we may need to delete multiple files simultaneously. A common approach is to use a loop to iterate over the files and delete them one by one. Let’s see how we can achieve this:
“`
string[] filePaths = { “C:\\path\\to\\file1.txt”, “C:\\path\\to\\file2.txt”, “C:\\path\\to\\file3.txt” };
foreach (string filePath in filePaths)
{
if (File.Exists(filePath))
{
File.Delete(filePath);
Console.WriteLine($”{filePath} deleted successfully”);
}
else
{
Console.WriteLine($”{filePath} does not exist”);
}
}
“`
By using a loop, we can handle multiple file deletions efficiently and provide feedback for each file.
Differentiating Between Files and Directories
When dealing with file deletions, it is important to differentiate between regular files and directories. Attempting to delete a directory using the `File.Delete()` method will result in an exception. To delete a directory, we need to use the appropriate method, such as `Directory.Delete()`. Here’s an example:
“`
string directoryPath = “C:\\path\\to\\directory”;
if (Directory.Exists(directoryPath))
{
Directory.Delete(directoryPath);
Console.WriteLine(“Directory deleted successfully”);
}
else
{
Console.WriteLine(“Directory does not exist”);
}
“`
By using the correct method based on the type of file (regular file or directory), we can handle file deletions effectively.
Considering Efficient Ways of Filtering and Selecting Files
When deleting multiple files, it is important to filter and select the files appropriately. C# provides several methods to filter files based on criteria such as file extension, file name, or creation date. By utilizing methods like `Directory.GetFiles()` or LINQ queries, we can efficiently select the files we want to delete.
For example, to delete all text files in a directory, we can use the following code:
“`
string[] filePaths = Directory.GetFiles(“C:\\path\\to\\directory”, “*.txt”);
foreach (string filePath in filePaths)
{
if (File.Exists(filePath))
{
File.Delete(filePath);
Console.WriteLine($”{filePath} deleted successfully”);
}
}
“`
By using appropriate filtering methods, we can ensure that only the desired files are selected for deletion.
Ensuring File List Accuracy and Thoroughness
When dealing with multiple file deletions, it is essential to ensure that the file list is accurate and thorough. This means that all relevant files are included for deletion and no files are missed.
To achieve this, it is important to thoroughly test the file selection logic and consider different scenarios. Performing extensive testing helps uncover any potential issues and ensures that file deletions are accurate and thorough.
Handling Read-Only Files
When dealing with read-only files, additional considerations are necessary. As mentioned earlier, attempting to delete a read-only file using the `File.Delete()` method will result in an exception. To delete read-only files, we need to modify the file attributes.
It is important to inform the user about these modifications and provide them with appropriate warnings and instructions. Additionally, it is crucial to ensure that the user has the necessary permissions or credentials to modify file attributes.
Recycle Bin vs. Permanent Deletion
Deleting a file can either send it to the Recycle Bin or permanently delete it from the file system. It is important to clarify the distinction between these two methods and provide options for the user to choose their desired outcome.
Deleting a file to the Recycle Bin allows for easy recovery in case of accidental deletion. On the other hand, permanently deleting a file cannot be undone and requires caution.
When implementing file deletion functionality, it is important to take user preferences into account and provide the appropriate methods for the desired outcome.
Performing File Deletion Safely
To ensure the safety of file deletions, several practices can be followed. Implementing proper error handling and exception management is crucial for handling unexpected scenarios. Confirming user intentions before deletion helps prevent accidental file deletions. Displaying warning messages for critical files ensures that users are aware of the potential consequences of deleting them. Finally, providing an undo or recovery option adds an extra layer of safety when dealing with critical files.
By following these practices, we can minimize the risk of data loss and handle file deletions safely.
Best Practices for File Deletion
When deleting files, it is important to follow best practices to protect data integrity and ensure reliable operations. Here are some best practices to consider:
1. Follow secure coding practices: Validate user input and sanitize file paths to prevent any potential security vulnerabilities.
2. Ensure necessary backups are in place: Before deleting files, ensure that necessary backups are available to restore data if needed.
3. Thoroughly test deletion functionality: Test file deletion functionality extensively to uncover any potential issues or edge cases.
4. Document file deletion procedures: Document the procedures for file deletion for maintenance or troubleshooting purposes. This documentation helps in case of future modifications or error analysis.
By following these best practices, we can perform file deletions efficiently and safely, minimizing the risk of data loss and ensuring reliable operations.
In conclusion, deleting files in C# requires careful consideration of various factors such as file existence, permissions, error handling, file type differentiation, and safety precautions. By leveraging the appropriate methods and following best practices, we can confidently perform file deletions and provide reliable functionality to users.
File Handling In C – Insert, Update, Delete, Sort, Search Of Student Record – In File With Structure
How To Delete A File In C Terminal?
The C terminal, also known as the command line or the command prompt, provides a powerful interface for performing various tasks on a computer. Deleting files using the C terminal is a simple yet essential task that every user should know. In this article, we will guide you through the process of deleting a file using the C terminal, along with some additional tips and frequently asked questions (FAQs) to enhance your understanding.
Before we begin, it is important to note that deleting a file using the C terminal is a permanent action. Once a file is deleted, it cannot be easily recovered unless you have a backup. Therefore, exercise caution when deleting files and ensure that you delete the right file.
Now, let’s dive into the step-by-step process of deleting a file in the C terminal:
Step 1: Launch the C Terminal
To delete a file, you need to open the C terminal. This can be done by searching for “Command Prompt” in the Start menu or by pressing the Windows key + R and typing “cmd” in the Run dialog box for Windows users. For Mac users, it can be accessed through the Terminal application, which can be found in the Utilities folder within the Applications directory.
Step 2: Navigate to the File’s Location
Once the C terminal is open, you need to navigate to the directory where the file you want to delete is located. This can be achieved by using the “cd” command followed by the directory path. For example, if the file is located in the Documents folder, the command would be “cd Documents.”
Step 3: Delete the File
To delete the file, you need to use the “del” command (delete) for Windows or the “rm” command (remove) for Mac and Linux. Following the command, specify the name of the file you want to delete, including the file extension. For example, to delete a file named “example.txt,” the command on Windows would be “del example.txt” or “rm example.txt” for Mac and Linux.
Step 4: Confirm the Deletion
After executing the delete command, you will be prompted to confirm the deletion. On Windows, you will be asked to confirm by pressing Y (yes) or N (no) and then pressing Enter. On Mac and Linux, no confirmation message will appear, and the file will be deleted immediately.
Step 5: Verify the Deletion
To ensure that the file has been successfully deleted, you can navigate to the file’s location using the file explorer (Windows) or the Finder (Mac), and check that the file is no longer present.
Frequently Asked Questions (FAQs):
Q1: Can I recover a file after deleting it in the C terminal?
A1: Generally, files deleted using the C terminal are permanently removed and cannot be easily recovered. However, there are specialized data recovery tools that may offer a chance of recovering deleted files, but the success rate is not guaranteed.
Q2: Can I delete multiple files at once using the C terminal?
A2: Yes, you can delete multiple files at once by specifying their names separated by spaces. For example, “del file1.txt file2.jpg” or “rm file1.txt file2.jpg”.
Q3: How can I delete a directory/folder using the C terminal?
A3: To delete a directory, you can use the “rmdir” command on Windows or the “rm -r” command on Mac and Linux. The directory must be empty before deletion, so make sure to remove all files and subdirectories within it first.
Q4: Can I delete read-only files using the C terminal?
A4: No, read-only files cannot be deleted using the standard “del” or “rm” commands. To delete such files, you will need to change their attributes to allow deletion or use the appropriate command-line option to force deletion.
In conclusion, deleting a file using the C terminal is a quick and effective way to remove unwanted files from your computer. By following the step-by-step process outlined in this article and being cautious about the actions performed, you can confidently manage and delete files through the command line. Remember to be careful, as deletion is permanent, and always double-check before executing any commands.
How To Use Remove () In C?
C is a powerful programming language that allows developers to create efficient and robust programs. When it comes to file handling, the remove() function in C is a useful tool to delete files from the system. In this article, we will take an in-depth look at how to utilize the remove() function effectively, along with its various aspects, and address some frequently asked questions. So, let’s dive in!
Understanding the remove() Function:
The remove() function, defined in the stdio.h header file, allows programmers to delete files from the file system permanently. Its syntax is simple: int remove(const char *filename). The return value for the function is zero if the deletion is successful; otherwise, it returns a non-zero value.
Using the remove() Function:
To use the remove() function effectively, you need to follow these steps:
Step 1. Include the necessary header file:
First and foremost, the stdio.h header file must be included in your program. This file contains the necessary functions and definitions required for I/O operations.
Step 2. Open the file:
Before you can delete a file, it must be opened in the appropriate mode. This can be done using the fopen() function, which returns a FILE pointer.
Step 3. Close the file:
After performing the necessary file operations, it is essential to close the file using fclose() before attempting to remove it. This ensures proper handling of resources and avoids any potential issues.
Step 4. Use the remove() function:
Finally, once the file is closed, you can use the remove() function to delete the file. Pass the filename as a parameter to the function. It is important to note that the function does not support wildcards. Instead, it only works with specific filenames or a path to the file.
Error Handling:
Errors can occur while using the remove() function, and it is crucial to handle them gracefully. Some common issues include:
1. Permissions: If a file is read-only or protected, the remove() function will fail to delete it. Ensure that the necessary file permissions are set correctly.
2. File in Use: If a file is currently being accessed or used by another program, it may not be deleted using remove(). Ensure that the file is not open or being used by any other application.
FAQs Section:
Q1. Can I use wildcards with the remove() function in C?
A1. No, the remove() function does not support wildcards. It can only delete specific files or files specified by their path.
Q2. What happens if the remove() function fails to delete a file?
A2. If the remove() function fails, it returns a non-zero value. You can utilize this return value to handle the failure gracefully and display an appropriate error message to the user.
Q3. What precautions should I take while using the remove() function?
A3. When using the remove() function, make sure the file you intend to delete is closed, properly handled, and not being used by any other process. Additionally, check the file permissions before attempting deletion.
Q4. Are there any limitations to the remove() function?
A4. The remove() function can only delete files; it cannot delete directories. To delete a directory, you must use the rmdir() function.
Q5. Can I undo a deletion performed by the remove() function?
A5. No, the remove() function permanently deletes the file from the file system. There is no built-in mechanism to undo the deletion.
Q6. Is the remove() function platform-independent?
A6. Yes, the remove() function is part of the standard C library and is available on all major platforms. It can be used universally without the need for any platform-specific modifications.
Conclusion:
The remove() function in C provides a simple and effective way to delete files from the system. By understanding its usage and error handling, you can confidently remove files while developing your C programs. Remember to handle any potential errors gracefully and check for proper permissions before attempting deletion. With this knowledge, you are now equipped to utilize the remove() function effectively in your C programming endeavors.
Keywords searched by users: delete file in c# Delete file C++, Delete in C, Delete folder in c, Delete file C++ fstream, Create file in C, Write a program in C to delete a specific line from a file, Remove file c linux, Read and write file in C
Categories: Top 70 Delete File In C#
See more here: nhanvietluanvan.com
Delete File C++
When writing a C++ program, it is common to come across situations where you need to delete files. Deleting a file in C++ involves a few steps and requires the use of certain functions and libraries. In this article, we will explore how to delete files in C++ and discuss some frequently asked questions related to this topic.
Deleting a File in C++:
To delete a file in C++, you need to perform the following steps:
Step 1: Include the necessary libraries:
To manipulate files in C++, you need to include the `
“`cpp
#include
#include
“`
Step 2: Declare the file name:
Next, declare a string variable that holds the name of the file you want to delete.
“`cpp
std::string fileName = “example.txt”;
“`
Step 3: Delete the file:
To delete the file, you need to call the `remove()` function from the `
“`cpp
std::remove(fileName.c_str());
“`
This function permanently deletes the specified file from the filesystem. However, be cautious when using this function, as it does not prompt for confirmation and directly removes the file. Therefore, double-check the file name and ensure that you have the necessary permissions before executing this command.
Step 4: Check if the file was deleted:
After calling the `remove()` function, you can check if the file was deleted successfully by using the `std::ifstream` class. Create an instance of the `std::ifstream` class, passing the file name as an argument.
“`cpp
std::ifstream file(fileName);
“`
Then, use the `is_open()` function to check if the file was opened successfully. If the file does not exist, it means it was deleted.
“`cpp
if (file.is_open()) {
std::cout << "File deletion failed!" << std::endl;
} else {
std::cout << "File successfully deleted!" << std::endl;
}
```
This step ensures that the file was successfully deleted and prevents any unintended consequences.
Frequently Asked Questions (FAQs):
Q1: Can I delete multiple files using the `remove()` function in C++?
A1: No, the `remove()` function can only delete one file at a time. If you want to delete multiple files, you need to call the function multiple times with each file name.
Q2: How can I delete a file if it is opened or in use by another program?
A2: If a file is currently open or in use by another program, you cannot directly delete it. You must wait until the file is closed or no longer in use before attempting to delete it.
Q3: Is there a way to move the deleted file to the recycle bin instead of permanently deleting it?
A3: Unfortunately, there is no built-in function in C++ to move the deleted file to the recycle bin. If you want to achieve this behavior, you would need to use operating system-specific APIs or libraries.
Q4: What happens if I try to delete a file that does not exist?
A4: If you try to delete a file that does not exist, the `remove()` function will return an error. It is good practice to check for errors and handle them appropriately in your program.
Q5: Do I need special permissions to delete a file in C++?
A5: Yes, you need the necessary permissions to delete a file. If you are running your program with administrative privileges, you should have no problems deleting any file. However, if you do not have the required permissions, the deletion operation will fail.
Conclusion:
Deleting files in C++ is a relatively straightforward process. By using the `
Through this article, we covered the steps to delete a file in C++, along with answering some frequently asked questions related to this topic. By following these guidelines, you will be able to incorporate file deletion capabilities into your C++ programs effectively.
Delete In C
Introduction
In the C programming language, “delete” is a critical keyword that enables developers to effectively manage memory allocation. By deallocating memory that is no longer needed, the delete keyword helps prevent memory leaks and improve overall program efficiency. In this article, we will delve into the various uses of delete in C, explore the syntax and functionality associated with this keyword, and discuss some best practices to ensure proper memory management. We will also address frequently asked questions to provide a thorough understanding of the topic.
Understanding the delete Keyword
The delete keyword in C is primarily used to deallocate memory that was previously allocated dynamically using the ‘new’ keyword. When memory is no longer needed or has served its purpose, deleting it ensures that the memory space is returned to the system, making it available for other parts of the application or other programs running on the operating system.
Syntax and Usage of delete in C
To understand how the delete keyword is used, let’s take a look at the syntax:
“`c
delete pointer;
“`
Here, ‘pointer’ refers to the memory address that needs to be deallocated. It is important to note that delete should only be used with dynamically allocated memory, such as objects created with new. If used incorrectly, like with statically allocated memory or variables without dynamic allocation, it can lead to undefined behavior or program crashes.
It is worth mentioning that calling delete on a null pointer has no effect, avoiding any potential crashes or issues arising from attempting to delete uninitialized or unallocated memory. Therefore, it is generally considered a good practice to assign a null value to a pointer after deleting it, preventing accidental reuse of the memory.
Best Practices for Using delete
To ensure memory is deallocated correctly and to avoid common pitfalls, consider the following best practices:
1. Pair new with delete: Always use delete for every new, as failing to do so can lead to memory leaks. As a general practice, delete any dynamically allocated memory right before it goes out of scope or is no longer needed.
2. Avoid double deletion: Deleting the same memory address multiple times can result in undefined behavior as the memory will no longer be valid. It is important to track and maintain a clear and accurate record of allocated and deallocated memory to avoid double deletions.
3. Use delete[] for arrays: When working with dynamic arrays, it is crucial to employ delete[] instead of delete. Using delete on an array can lead to undefined behavior. Deleting a dynamic array using delete[] ensures that all elements are properly deallocated without any memory leaks.
4. Delete memory in destructors: If objects contain dynamically allocated memory, ensure they are properly deallocated within the destructor to avoid memory leaks. This practice is particularly crucial for classes, as failing to delete memory in the destructor can result in resource leaks.
5. Refresh pointers after deletion: Setting a pointer to null after deleting its allocated memory helps prevent inadvertent access to invalidated or deallocated memory. This simple practice can help avoid potential segmentation faults and improve program safety.
Frequently Asked Questions
Q1: What happens if I call delete on a pointer that was not allocated with new?
A1: Calling delete on a pointer that was not allocated with new will result in undefined behavior. It may lead to program crashes, memory corruption, or other unexpected issues. Therefore, it is crucial to only use delete with dynamically allocated memory.
Q2: Can I use delete in C++ code as well?
A2: Yes, delete is a valid keyword in both C and C++ languages. However, note that in C++, there is another keyword called delete[] specifically designed for deallocating dynamic arrays, ensuring every element is correctly deallocated.
Q3: Can I use delete to deallocate memory allocated with malloc?
A3: No, delete and malloc do not go hand in hand. In C, the use of delete with dynamically allocated memory using malloc is not only incorrect but also leads to undefined behavior. Use free() to deallocate memory allocated with malloc.
Q4: What is the consequence of forgetting to delete dynamically allocated memory?
A4: Failing to delete dynamically allocated memory can result in memory leaks. In the case of long-running or resource-intensive programs, the accumulation of memory leaks can diminish system performance and eventually lead to a program crash or system instability.
Q5: How does delete differ from free() in C?
A5: delete is used to deallocate memory when working with objects and memory allocated using new, while free() is used with memory allocated using malloc in C. delete ensures that objects’ destructors are properly called, providing additional functionality specific to object-oriented programming in C++.
Conclusion
Understanding the importance of memory management and the various uses of the delete keyword in C is crucial for writing reliable and efficient programs. By following best practices, such as appropriately pairing new with delete, avoiding double deletions, and refreshing pointers after deletion, developers can prevent memory leaks, improve program stability, and ensure efficient resource utilization. Remember to use delete solely on dynamically allocated memory, and when working with dynamic arrays, employ delete[] instead of delete. By adhering to these guidelines, developers can harness the power of the delete keyword to produce robust and high-performing C programs.
Images related to the topic delete file in c#
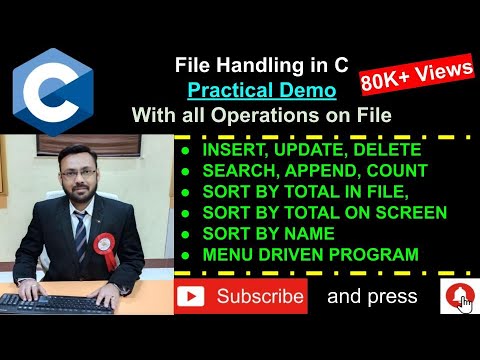
Article link: delete file in c#.
Learn more about the topic delete file in c#.
- C program to delete a file – GeeksforGeeks
- How to Delete File in C? – Tutorial Kart
- Deleting files (rm command) – IBM
- C Language: remove function (Remove File) – TechOnTheNet
- C program to delete a file – Tutorialspoint
- DELETE keyword – LIX-polytechnique
- Different ways to delete file in C – OpenGenus IQ
- Deleting Files (The GNU C Library)
- C Program to Delete a File – W3schools
- How to Delete a File in C#
- How to Use the C remove() function to Delete a File