Delete A File In C#
When working with files in a C# program, it is sometimes necessary to delete a file. Whether you want to clean up unnecessary files, remove temporary files, or simply delete a file as part of a larger task, knowing how to perform this operation is essential. In this article, we will explore various methods of deleting a file in C#, including handling exceptions and deleting files permanently.
Preparation
Before deleting a file, it is important to check if the file actually exists. Attempting to delete a file that does not exist can lead to unnecessary errors and can disrupt the flow of your program. To avoid this, you can use the File.Exists() method to check if the file exists before attempting to delete it.
Checking if the File Exists
To check if a file exists, you can use the File.Exists() method from the System.IO namespace. This method accepts a file path as a parameter and returns a boolean value indicating whether the file exists or not. Here’s an example:
“`csharp
string filePath = “path/to/file.txt”;
if (File.Exists(filePath))
{
// File exists, proceed with deletion
}
“`
Deleting a File
Once you have verified that the file exists, deleting it is a straightforward task. You can use the File.Delete() method, also from the System.IO namespace, which accepts a file path as a parameter and deletes the file at that location. Here’s an example:
“`csharp
string filePath = “path/to/file.txt”;
if (File.Exists(filePath))
{
File.Delete(filePath);
Console.WriteLine(“File deleted successfully.”);
}
“`
Handling Exceptions
When deleting a file, it is important to handle any potential exceptions that may occur. For example, if the file is currently being used by another process or if the user does not have the necessary permissions to delete the file, an exception will be thrown. To handle these exceptions, you can use a try-catch block. Here’s an example:
“`csharp
try
{
string filePath = “path/to/file.txt”;
if (File.Exists(filePath))
{
File.Delete(filePath);
Console.WriteLine(“File deleted successfully.”);
}
}
catch (Exception ex)
{
Console.WriteLine(“An error occurred while deleting the file: ” + ex.Message);
}
“`
Deleting a File Permanently
By default, when you delete a file using the File.Delete() method, it is moved to the Recycle Bin rather than being permanently deleted. However, if you want to delete the file permanently without moving it to the Recycle Bin, you can use the Win32 API function DeleteFile(). To use this function, you need to import it from the kernel32.dll library using the DllImport attribute. Here’s an example:
“`csharp
using System.Runtime.InteropServices;
// …
[DllImport(“kernel32.dll”, SetLastError = true, CharSet = CharSet.Unicode)]
static extern bool DeleteFile(string lpFileName);
string filePath = “path/to/file.txt”;
if (File.Exists(filePath))
{
DeleteFile(filePath);
Console.WriteLine(“File deleted permanently.”);
}
“`
Deleting a Directory
In addition to deleting individual files, you may also need to delete entire directories. To delete a directory and all its contents, including subdirectories and files, you can use the Directory.Delete() method from the System.IO namespace. Here’s an example:
“`csharp
string directoryPath = “path/to/directory”;
if (Directory.Exists(directoryPath))
{
Directory.Delete(directoryPath, true);
Console.WriteLine(“Directory deleted successfully.”);
}
“`
Deleting Read-Only Files
If a file is marked as read-only, you will not be able to delete it directly using the File.Delete() method. To delete a read-only file, you can first remove the read-only attribute and then delete the file. Here’s an example:
“`csharp
string filePath = “path/to/read-only/file.txt”;
if (File.Exists(filePath))
{
File.SetAttributes(filePath, FileAttributes.Normal);
File.Delete(filePath);
Console.WriteLine(“Read-only file deleted successfully.”);
}
“`
FAQs
Q: Can I delete a file in C++ using similar methods?
A: Yes, you can delete a file in C++ using the remove() function from the
“`c++
std::remove(“path/to/file.txt”);
“`
Q: How can I delete a folder in C?
A: To delete a directory in C, you can use the rmdir() function from the
“`c
#include
// …
char* directoryPath = “path/to/directory”;
rmdir(directoryPath);
“`
Q: How can I delete a specific line from a file in C?
A: To delete a specific line from a file in C, you can read the contents of the file line by line, skipping the line you want to delete, and then write the remaining lines back to the file. Here’s an example:
“`c
#include
// …
int lineToRemove = 2; // Line number to remove
FILE* inputFile = fopen(“path/to/file.txt”, “r”);
FILE* outputFile = fopen(“path/to/temp.txt”, “w”);
char line[100];
int currentLine = 1;
while (fgets(line, sizeof(line), inputFile))
{
if (currentLine != lineToRemove)
{
fputs(line, outputFile);
}
currentLine++;
}
fclose(inputFile);
fclose(outputFile);
// Rename the temporary file to the original file
remove(“path/to/file.txt”);
rename(“path/to/temp.txt”, “path/to/file.txt”);
“`
Q: How can I remove a file from the disk in C?
A: To remove a file from the disk in C, you can use the remove() function from the
“`c
#include
// …
remove(“path/to/file.txt”);
“`
In conclusion, deleting a file in C# can be accomplished using the File.Delete() method, with proper checks for file existence and exception handling. Additionally, you can delete directories, handle read-only files, and even delete files permanently. Remember to use the appropriate methods and techniques depending on your specific use case.
Deleting A Record From A File|| File Handling In C
How To Delete A File In C?
Deleting files is a fundamental operation in any programming language, including C. Whether you need to clean up unused files, manage disk space, or simply remove temporary files, understanding how to delete files in C is crucial. In this article, we will explore different methods to delete files using C programming language, covering the topic in-depth.
Before we dive into the code, it is important to grasp some basic concepts. In modern operating systems, files consist of a combination of metadata and actual file content. The metadata includes information such as the file name, size, creation and modification timestamps, and permissions. Meanwhile, the file content represents the actual data stored within the file. When you delete a file, these two components need to be considered.
Now, let’s explore different methods to delete a file in C.
Method 1: Using the remove() Function
The simplest and most commonly used method to delete a file in C is by using the remove() function. This function is defined in the `stdio.h` library and accepts a file name as an argument. Here’s an example that demonstrates how to delete a file using the remove() function:
“`c
#include
int main() {
char filename[] = “example.txt”;
if (remove(filename) == 0) {
printf(“%s file deleted successfully.\n”, filename);
} else {
printf(“Unable to delete the file %s.\n”, filename);
}
return 0;
}
“`
In the above code, we pass the filename “example.txt” to the remove() function. If the removal is successful, remove() returns 0, and a success message is printed. Otherwise, if the removal fails, a corresponding error message is displayed.
Method 2: Using the unlink() Function
Similar to remove(), the unlink() function can be used to delete a file in C. The unlink() function is part of the `unistd.h` library, which provides access to various operating system functionalities. Here’s an example that demonstrates how to use the unlink() function:
“`c
#include
#include
int main() {
char filename[] = “example.txt”;
if (unlink(filename) == 0) {
printf(“%s file deleted successfully.\n”, filename);
} else {
printf(“Unable to delete the file %s.\n”, filename);
}
return 0;
}
“`
As shown in the code snippet above, the unlink() function is used similar to remove(). It returns 0 upon successful deletion, and an appropriate message is printed accordingly.
Method 3: Using the remove_directory() Function in Windows
In Windows systems, deleting a directory requires additional steps beyond simply calling remove() or unlink(). Microsoft provides the `_rmdir()` function for deleting directories. Here’s an example that demonstrates how to delete a directory in C on a Windows system:
“`c
#include
#include
int main() {
char dirname[] = “example_dir”;
if (_rmdir(dirname) == 0) {
printf(“%s directory deleted successfully.\n”, dirname);
} else {
printf(“Unable to delete the directory %s.\n”, dirname);
}
return 0;
}
“`
Note that on non-Windows systems, `_rmdir()` is not available, and `rmdir()` should be used instead.
FAQs:
Q1: Can I delete multiple files at once in C?
Yes, you can delete multiple files at once by utilizing loops and iterating through a list of file names. Within the loop, you can call the remove() or unlink() function with each file name.
Q2: What precautions should I take before deleting a file?
Before deleting a file, make sure to handle any critical data that may be lost. Also, double-check the path and file name to avoid accidentally deleting the wrong file.
Q3: How can I check if a file exists before deleting it?
You can use the access() function from the `unistd.h` library to check if a file exists. If access() returns 0, the file exists, and you can proceed with its deletion.
Q4: Are there any file deletion permissions or restrictions?
Yes, file deletion permissions and restrictions can vary depending on the operating system and file system. Ensure that you have the necessary permissions to delete a file.
Q5: Can I recover a deleted file in C?
No, once a file is deleted using C or any programming language, it is generally not recoverable through programmatic means. Specialized data recovery software may be able to recover deleted files in certain scenarios.
In conclusion, deleting files in C can be achieved using various methods, such as remove(), unlink(), and _rmdir(). Each method serves a specific purpose and should be chosen based on the operating system and desired functionality. It is important to handle file deletion with caution, considering potential data loss and the necessary permissions. By following the information provided in this article, you will be well-equipped to delete files in C effectively.
How To Delete A File In C Terminal?
File management is an essential aspect of any operating system, and deleting files is a common task in this realm. The C terminal provides a powerful command-line interface for performing various file operations, including deletion. In this article, we will explore how to delete a file using the C terminal, step by step, and provide additional tips and information to help you better understand the process.
Before we proceed, it’s important to mention that deleting a file is a permanent action. Once a file is deleted, it cannot be easily recovered unless you have a backup. Therefore, exercise caution and double-check your actions before deleting any files.
Step 1: Opening the C Terminal
To begin, launch the C terminal on your operating system. Depending on your system, you might find the terminal under different names such as Command Prompt (Windows) or Terminal (macOS/Linux).
Step 2: Navigating to the Directory
Use the command “cd” followed by the directory path to navigate to the folder where the file you want to delete is located. For instance, if the file is located on the desktop, use the command:
“`
cd Desktop
“`
This will change the current directory to the Desktop.
Step 3: Checking the Contents of the Directory
You can verify the files present in the current directory using the “ls” command, which lists the files and folders. This step allows you to ensure you’re operating in the correct location and confirms the presence of the file you wish to delete.
Step 4: Deleting the File
To delete the file, utilize the “rm” command followed by the file’s name. For example, if the file is named “example.txt,” enter the following command:
“`
rm example.txt
“`
This command will permanently remove the file from the directory. If you want to delete multiple files, you can provide multiple file names as arguments after the “rm” command.
Step 5: Confirmation
By default, the terminal doesn’t prompt for confirmation when deleting files. Therefore, be extremely careful while using the “rm” command, as there is no way to recover files once they are deleted. To add an extra layer of confirmation, you can utilize the “-i” option as follows:
“`
rm -i example.txt
“`
This prompts you to confirm the deletion of each file individually before proceeding.
Step 6: Deleting Files in Subdirectories
The steps aforementioned are applicable for files within the current directory. If you need to delete a file located in a subdirectory, you must ensure that you navigate to that specific directory using the “cd” command before executing the “rm” command.
FAQs
Q1: Can I recover a file once it is deleted using the C terminal?
A1: No, once a file is deleted in the C terminal, it cannot be easily recovered. It is crucial to ensure you have backed up any important files before performing any delete operations.
Q2: How can I delete a directory and all of its contents?
A2: To delete a directory and its contents, the “rm” command is used with the “-r” option, which stands for recursive. For example, to delete a directory named “folder” and all its contents, use the following command:
“`
rm -r folder
“`
Q3: What happens if I accidentally delete a file?
A3: Unfortunately, accidental deletion can occur. If you accidentally delete a file, you might want to consider using specialized file recovery tools or seeking professional assistance to attempt file retrieval. However, success in recovering a deleted file is not guaranteed.
Q4: Is there any way to delete files without permanently removing them?
A4: Yes, some systems provide a “Trash” or “Recycle Bin” functionality, which allows files to be temporarily moved to a separate location before permanent deletion. However, this functionality might not be available in the C terminal unless integrated into specific commands or scripts.
In conclusion, deleting a file in the C terminal involves navigating to the desired directory and using the “rm” command followed by the file’s name. It is essential to exercise caution and double-check your actions before confirming the deletion, as it is a permanent operation. Always ensure the correct file is selected before executing the “rm” command.
Keywords searched by users: delete a file in c# Delete in C, Delete file C++, Remove file c linux, Std::remove, Delete folder in c, Delete file C++ fstream, Write a program in C to delete a specific line from a file, Write a program in c to remove a file from the disk
Categories: Top 87 Delete A File In C#
See more here: nhanvietluanvan.com
Delete In C
In the C programming language, the delete keyword is used to deallocate dynamically allocated memory in the heap. Memory deallocation is a crucial aspect of memory management as it allows developers to free up memory that is no longer needed, preventing memory leaks and optimizing resource usage. In this article, we will dive deep into the concept of delete in C, its syntax, usage, and best practices. So, let’s get started!
Understanding Dynamic Memory Allocation in C:
Before we delve into the specifics of delete, it’s essential to understand dynamic memory allocation in C. Unlike static allocation, where memory is allocated at compile time, dynamic allocation allows for memory allocation at runtime. This feature enables flexibility and efficient memory utilization.
The Dynamic Memory Allocation functions in C:
C offers several functions to dynamically allocate memory, such as malloc(), calloc(), realloc(), and new (in C++). The new operator returns a pointer to the dynamically allocated memory block. Once the memory is no longer required, it must be explicitly deallocated using the delete keyword.
The delete Keyword:
The delete keyword in C is used to deallocate memory allocated using the new operator. Its syntax is simple:
delete pointer;
Here, “pointer” refers to the pointer variable that holds the address of the dynamically allocated memory block. Deleting a pointer frees the memory it points to, making it available for other operations.
Frequently Asked Questions (FAQs):
Q1. What happens if delete is called on a null pointer?
If delete is called on a null pointer, it has no effect. The null pointer is a special value that indicates the absence of a valid memory address. Calling delete on a null pointer is considered safe and does nothing.
Q2. Can delete be used with statically allocated memory?
No, delete is used only with memory allocated dynamically using new. Statically allocated memory is allocated at compile time and typically resides in the stack. Such memory is released automatically when the corresponding scope ends. Delete should be used only on dynamically allocated memory, preventing access to deallocated or invalid memory.
Q3. What happens if delete is called multiple times on the same pointer?
Calling delete multiple times on the same pointer results in undefined behavior. It can lead to memory corruption, crashes, or other unexpected program behavior. To avoid this, it’s crucial to set the pointer to null after deletion, indicating that it no longer points to valid memory.
Q4. What are the consequences of forgetting to delete dynamically allocated memory?
Forgetting to delete dynamically allocated memory leads to memory leaks. Memory leaks occur when memory is allocated but not freed, resulting in a gradual reduction in available memory in the heap. Over time, this can cause performance issues and eventually lead to system instability or crashes. It’s crucial to pay attention to proper memory deallocation to prevent memory leaks.
Q5. Can delete be used with arrays?
Yes, delete can be used with arrays allocated using the new[] operator. The correct syntax would be:
delete[] arrayPointer;
Here, “arrayPointer” refers to the pointer variable holding the address of the dynamically allocated array. Using delete instead of delete[] or vice versa results in undefined behavior. Arrays allocated using new[] must be deallocated using delete[].
Q6. Is it necessary to delete dynamically allocated memory manually?
Yes, it is essential to delete dynamically allocated memory manually to prevent memory leaks and optimize resource usage. C does not have automatic garbage collection like some other programming languages, making manual memory management a responsibility of the programmer. By explicitly freeing dynamically allocated memory using delete, you ensure efficient memory utilization and prevent the accumulation of unused memory blocks.
In conclusion, delete in C is a powerful keyword that allows for the deallocation of dynamically allocated memory. Proper use of delete ensures efficient memory management, preventing memory leaks and optimizing resource usage. Understanding how to allocate and deallocate memory dynamically is crucial for developing robust and efficient C programs. By adhering to the best practices for memory deallocation and addressing the frequently asked questions, you can avoid common pitfalls and ensure your programs run smoothly.
Delete File C++
Introduction:
In computer programming, file handling is an essential aspect of software development. While creating, modifying, and reading files are often the primary focus, deleting files is also a critical operation for managing data effectively. In this article, we will delve into the process of deleting files in C++. We will explore various methods and techniques, highlighting their advantages, possible challenges, and best practices. So let’s dive in!
Delete File with remove() Function:
The most basic and widely used method to delete a file in C++ is by employing the remove() function. This function is defined in the
“`cpp
#include
int main() {
const char* filename = “example.txt”;
int result = remove(filename);
if (result == 0) {
printf(“File %s deleted successfully.”, filename);
} else {
printf(“Failed to delete file %s.”, filename);
}
return 0;
}
“`
However, it is worth noting that the remove() function may not always provide detailed error messages when it fails to delete a file. Therefore, it is advisable to utilize additional techniques to handle error scenarios more effectively.
Delete File with std::filesystem:
Starting from C++17, the Standard Template Library (STL) introduced the
“`cpp
#include
#include
int main() {
const std::filesystem::path filename = “example.txt”;
try {
if (std::filesystem::exists(filename)) {
std::filesystem::remove(filename);
std::cout << "File " << filename << " deleted successfully." << std::endl;
} else {
std::cout << "File " << filename << " not found." << std::endl;
}
} catch (const std::filesystem::filesystem_error& e) {
std::cout << "Failed to delete file " << filename << ". Error: " << e.what() << std::endl;
}
return 0;
}
```
This implementation demonstrates error handling using exceptions, making it easier to identify the cause of the deletion failure. Additionally, the std::filesystem library provides a more platform-independent approach to file system operations.
Delete File vs. Delete Directory:
It is important to differentiate between deleting a file and deleting a directory (folder). While deleting a file does not typically present many challenges, dealing with a directory requires careful attention. Deleting a directory usually involves recursively deleting all its contents, including subdirectories and files. Therefore, to delete a directory efficiently, it is recommended to use specialized functions like std::filesystem::remove_all(). Here's an example to illustrate deleting a directory using std::filesystem:
```cpp
#include
#include
int main() {
const std::filesystem::path dir = “example_dir”;
try {
if (std::filesystem::exists(dir) && std::filesystem::is_directory(dir)) {
std::filesystem::remove_all(dir);
std::cout << "Directory " << dir << " deleted successfully." << std::endl;
} else {
std::cout << "Directory " << dir << " doesn't exist." << std::endl;
}
} catch (const std::filesystem::filesystem_error& e) {
std::cout << "Failed to delete directory " << dir << ". Error: " << e.what() << std::endl;
}
return 0;
}
```
FAQs:
Q1. What precautions should I take before deleting a file?
A1. Before deleting a file, ensure that it is not open by any other program or not in use within your own code. Closing any file streams or associated program instances is essential to avoid potential errors during deletion.
Q2. Is there a method to recover deleted files easily?
A2. In general, files deleted programmatically cannot be easily recovered. However, various file recovery tools and techniques exist, depending on the operating system and usage scenario. It's crucial to seek professional assistance if file recovery is necessary.
Q3. What is the recommended approach for error handling during file deletion?
A3. Error handling during file deletion should be an integral part of your code. Consider using functions like remove(), std::filesystem::remove(), or std::filesystem::remove_all(), and include proper exception handling to identify and handle deletion failures effectively.
Q4. Can the removed file be restored?
A4. Once a file is removed, it is typically permanently deleted from the file system, and its contents might not be recoverable. Therefore, it is advisable to ensure the deletion operation is intended and take necessary backup precautions beforehand.
Q5. Are there any platform compatibility issues when using std::filesystem?
A5. While std::filesystem provides a more platform-independent approach, there might be compatibility issues with older compilers or operating systems. Ensure that your development environment supports C++17 or later and refer to the compiler documentation for specific details.
Conclusion:
Deleting files is an integral part of file management in C++. By utilizing the remove() function or the robust std::filesystem library, you can easily delete files and directories, ensuring efficient file handling within your applications. Always remember to handle errors and exceptions appropriately, and consider the potential consequences of file deletion before proceeding. Happy file handling!
Remove File C Linux
In the world of C programming on the Linux system, file manipulation is an essential task that programmers encounter on a regular basis. One such task is removing files. In this article, we will explore the various methods and functions available in the C programming language to remove files on a Linux operating system. We will cover this topic in depth, providing step-by-step instructions and code examples to help you effectively remove files in your C programs.
I. Introduction to File Removal
Before diving into the technicalities of removing files in C programming, let’s first understand the concept of file removal. In Linux, files are organized in a hierarchical filesystem structure. Each file is associated with an inode, which contains relevant metadata such as the file’s permissions, size, and location. The removal of a file involves deleting its associated inode and freeing up the disk space it occupied.
II. The remove() Function
The standard C library provides the remove() function, which allows us to delete a file using its filename as the input parameter. The syntax of the remove() function is as follows:
“`c
int remove(const char *filename);
“`
To remove a file using the remove() function, we need to include the stdio.h header file in our C program. Let’s take a look at an example:
“`c
#include
int main() {
if (remove(“example.txt”) == 0)
printf(“File removed successfully.\n”);
else
printf(“Unable to remove the file.\n”);
return 0;
}
“`
In the above example, the remove() function is used to delete a file named “example.txt”. If the file is successfully removed, the program will print “File removed successfully.” Otherwise, it will print “Unable to remove the file.”
III. Dealing with Error Conditions
While using the remove() function, it is crucial to handle potential error conditions. The remove() function returns an integer value as its output, which can be used to determine the success or failure of file removal. A return value of 0 indicates successful removal, while a value of -1 denotes an error.
To obtain additional information about the error, we can use the perror() function, which prints a descriptive error message to the standard error stream. Let’s modify the previous example to include error handling:
“`c
#include
int main() {
if (remove(“example.txt”) == 0)
printf(“File removed successfully.\n”);
else {
perror(“Error”);
printf(“Unable to remove the file.\n”);
}
return 0;
}
“`
In this updated example, if an error occurs during file removal, the perror() function will provide a descriptive error message, such as “No such file or directory” or “Permission denied.”
IV. Frequently Asked Questions (FAQs)
Q1. Can I remove multiple files using the remove() function?
A1. No, the remove() function only allows the removal of one file at a time. If you want to remove multiple files, you need to call the remove() function for each file individually.
Q2. What happens if I try to remove a file that doesn’t exist?
A2. If you attempt to remove a file that doesn’t exist, the remove() function will return -1 and set the errno variable to indicate the error. You can use the perror() function to display the corresponding error message.
Q3. Can I use the remove() function to remove directories?
A3. No, the remove() function is designed to delete regular files, not directories. To remove a directory, you can use the rmdir() function, which is specifically designed for this purpose.
Q4. Is there any alternative to the remove() function for file removal?
A4. Yes, the unlink() function is another standard C library function that can be used to delete a file. It has similar functionality to remove(), but many programmers prefer remove() for its simplicity and versatility.
V. Conclusion
In this article, we explored various methods to remove files in C programming on a Linux system. We learned about the remove() function and how to handle error conditions using perror(). Additionally, we provided answers to frequently asked questions regarding file removal. Armed with this knowledge, you can efficiently manipulate files in your C programs on Linux, adding a robust file management component to your applications.
Images related to the topic delete a file in c#
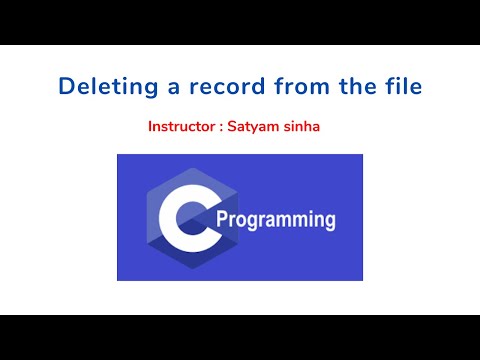
Article link: delete a file in c#.
Learn more about the topic delete a file in c#.
- C program to delete a file – GeeksforGeeks
- How to Delete File in C? – Tutorial Kart
- C program to delete a file – GeeksforGeeks
- Deleting files (rm command) – IBM
- C Language: remove function (Remove File) – TechOnTheNet
- C program to delete a file – Tutorialspoint
- Different ways to delete file in C – OpenGenus IQ
- Deleting Files (The GNU C Library)
- How to Use the C remove() function to Delete a File
- C Program to Delete a File – W3schools
- How to Delete a File in C#