Decorator Pattern In C#
The decorator pattern is a design pattern that allows for the dynamic addition of new functionalities to an existing object by wrapping it with a decorator class. This pattern is known for its ability to enhance flexibility and extensibility in object-oriented programming, making it a valuable tool for developers.
Basic Concepts of the Decorator Pattern:
The decorator pattern is based on the principle of composition over inheritance. Instead of creating subclasses to add functionalities to an object, the decorator pattern utilizes a wrapping approach, where new functionalities are added by wrapping the original object in one or more decorator classes.
The problems it aims to solve:
The decorator pattern solves the problem of adding new functionalities to an object dynamically without the need for creating a large number of subclasses. It also allows for the selective addition of functionalities to an object, enabling the creation of flexible and modular code.
Understanding the Structure of the Decorator Pattern:
The decorator pattern consists of four main components: the component interface, the concrete component, the decorator class, and the concrete decorator.
1. Component Interface: This defines the interface that the decorator and concrete component classes must implement. It provides a common interface for both the component and the decorators.
2. Concrete Component: This is the original object to which new functionalities will be added. It implements the component interface and represents the core functionality of the object.
3. Decorator Class: This is an abstract class that implements the component interface. It acts as a base class for concrete decorators and provides a default implementation for the component interface. It also contains a reference to the component object that is being decorated.
4. Concrete Decorator: This is a subclass of the decorator class and represents the additional functionalities that will be added to the component object. It overrides methods from the decorator class and adds new behaviors to the component object.
How the Pattern is Implemented in C#:
In C#, the decorator pattern can be implemented using interfaces and inheritance. The component interface is defined as an interface, and the concrete component and decorator classes implement this interface. The concrete decorator class extends the decorator class and overrides its methods to add new functionalities.
Benefits and Advantages of Using the Decorator Pattern:
1. Flexibility: The decorator pattern allows for the dynamic addition of functionalities to an object at runtime. This flexibility enables developers to modify the behavior of an object without affecting other objects in the system.
2. Extensibility: By using the decorator pattern, new functionalities can be added to an object without the need for modifying its underlying structure. This makes it easier to extend the functionality of an existing object and promotes code reuse.
3. Open-Closed Principle: The decorator pattern follows the Open-Closed Principle, which states that classes should be open for extension but closed for modification. With the decorator pattern, new functionalities can be added without modifying the existing code, preserving the stability of the system.
Real-World Examples of the Decorator Pattern in C#:
1. Logging: Imagine a logging system where different types of loggers are used to log messages. The decorator pattern can be used to dynamically add additional logging functionalities, such as timestamping or filtering, to the basic logger object.
2. UI Customization: In a user interface, the decorator pattern can be used to add different visual decorations to a basic component, such as borders, shadows, or tooltips. Each decorator class adds a specific behavior to the component, allowing for customizable and flexible UI components.
Applying the Decorator Pattern to Extend Functionality:
To apply the decorator pattern to extend functionality, start by creating a component interface that defines the methods that all components must implement. Then, create a concrete component class that implements the component interface and represents the core functionality of the object.
Next, create an abstract decorator class that implements the component interface. This class should contain a reference to the component object and provide default implementations for the interface methods.
Finally, create concrete decorator classes by extending the abstract decorator class. Each concrete decorator class should override the interface methods and add additional functionalities to the component object.
Incorporating the Decorator Pattern with Other Design Patterns:
The decorator pattern can be combined with other design patterns to create more complex and flexible systems. One common combination is the Strategy pattern, where the decorator uses different strategies to add functionalities to the component object at runtime.
Another combination is the Composite pattern, where the component object can be a composite object that contains other objects. The decorator classes can then be used to add functionalities to both the composite object and its children.
Potential Drawbacks and Considerations when using the Decorator Pattern:
1. Complexity: The decorator pattern can add complexity to the codebase, especially when multiple decorators are involved. Developers need to carefully manage the relationships between decorators and ensure that the decorators don’t introduce conflicts or unnecessary overhead.
2. Overuse: While the decorator pattern provides a flexible and extensible approach to add functionalities, it’s important not to overuse it. Adding too many decorators can lead to a convoluted design and make the code difficult to understand and maintain.
Best Practices and Tips for Implementing the Decorator Pattern in C#:
1. Keep Decorators Small: To maintain code readability and manage complexity, it’s advisable to keep individual decorators small and focused on a specific functionality.
2. Use Interfaces: Implementing the component interface as an interface allows for easier extensibility and promotes loose coupling between components.
FAQs:
Q: What is the Decorator Pattern?
A: The decorator pattern is a design pattern that allows for the dynamic addition of new functionalities to an existing object by wrapping it with a decorator class.
Q: How is the Decorator Pattern implemented in C#?
A: The decorator pattern can be implemented in C# using interfaces and inheritance. The component interface is defined as an interface, and the concrete component and decorator classes implement this interface.
Q: What are the benefits of using the Decorator Pattern?
A: The decorator pattern provides flexibility, extensibility, and follows the Open-Closed Principle. It allows for dynamic addition of functionalities, easy extension of existing objects, and preserves the stability of the system.
Q: Can the Decorator Pattern be used with other design patterns?
A: Yes, the decorator pattern can be combined with other design patterns such as the Strategy pattern and Composite pattern to create more complex systems.
In conclusion, the decorator pattern is a powerful design pattern that enhances flexibility and extensibility in object-oriented programming. By allowing the dynamic addition of new functionalities to an existing object, it provides a modular and reusable approach to code design. With its ability to seamlessly extend the functionality of an object without modifying its underlying structure, the decorator pattern is a must-have tool in a developer’s toolkit.
Embedded C Programming Design Patterns | Clean Code | Coding Standards |
What Is The Decorator Pattern In Cs?
In computer science, the decorator pattern is a design pattern that belongs to the family of structural patterns. It allows us to dynamically add new functionality to an existing object without modifying its structure. The decorator pattern is based on the principle of composition rather than inheritance, making it a flexible alternative to subclassing.
To understand the decorator pattern, let’s consider a real-world example. Imagine a bakery that offers a variety of flavored cakes. Each cake is made from a basic recipe, but customers can choose to add different toppings or decorations to customize their cake. The decorator pattern is similar to this scenario, where the basic object (cake) can be enhanced with additional features (toppings).
Key Concepts:
1. Components: Components are the objects that we want to enhance by adding extra features. In our previous example, the basic cake is the component.
2. Decorators: Decorators are responsible for adding new functionality to the components. They wrap the component objects and provide additional behavior without changing the underlying structure.
3. Interface: A common interface is used by both the components and decorators. This ensures that decorators can be used interchangeably with the original components.
How does the decorator pattern work?
The decorator pattern works by creating a hierarchy of decorator classes that mirror the class hierarchy of the components. Each decorator derives from the same interface as the components, allowing both components and decorators to be treated uniformly. This design enables an unlimited number of combinations since decorators can be stacked and wrapped around components.
To illustrate, let’s consider a scenario where we have a basic coffee object. We want to enhance the coffee object with additional functionalities such as milk, sugar, and flavor. First, we define the common interface for the coffee component, which includes methods like getCost() and getDescription(). Then we create concrete implementations of this interface, representing the basic coffee and its variants.
Next, we define the decorator class, which also implements the same interface. The decorator class is responsible for wrapping a coffee object and adding behavior. For example, the milk decorator can extend the decorator class and add the cost and description of milk to the coffee. Similarly, the sugar and flavor decorators can extend the decorator class and add the cost and description of sugar and flavor respectively.
Using the decorator pattern, we can combine these decorators in different configurations and create a customized coffee object at runtime. This allows us to add or remove functionalities dynamically, providing flexibility and extensibility.
Benefits of using the decorator pattern:
1. Flexibility: The decorator pattern allows for the dynamic addition of functionalities to objects at runtime, providing flexibility without modifying their structure. It allows us to create new combinations of functionalities by stacking decorators.
2. Extensibility: As decorators wrap the original objects, new decorator classes can be easily added without affecting the existing code. This enhances the extensibility of the system by enabling the addition of new features.
3. Preservation of interface: Both components and decorators implement the same interface, ensuring that they can be used interchangeably. This preserves the original interface, allowing clients to interact with components and decorators uniformly.
FAQs:
Q1. What is the difference between the decorator pattern and inheritance?
The decorator pattern allows for the addition of functionalities at runtime without modifying the object’s structure. It favors composition over inheritance, offering flexibility and extensibility. In contrast, inheritance modifies the structure of the object by creating subclasses. While inheritance can also provide additional functionalities, it is less flexible and may result in a complex class hierarchy.
Q2. When should I use the decorator pattern?
The decorator pattern is useful when you want to add functionalities dynamically to objects without modifying their structure. It is beneficial in scenarios where a large number of combinations are possible, and the functionalities may change at runtime. The decorator pattern is especially valuable when you need to customize an object’s behavior at runtime without affecting other instances of the same class.
Q3. Are there any drawbacks to using the decorator pattern?
One potential drawback of the decorator pattern is that it can lead to the creation of numerous small objects, as every decorator wraps the component object. This can impact performance and memory usage, especially in systems with a large number of decorators and components. However, this drawback can be mitigated by careful design and optimization techniques.
Q4. Can I remove functionalities using the decorator pattern?
Yes, the decorator pattern allows for the addition and removal of functionalities dynamically. By simply not using a particular decorator, the functionality associated with it is excluded from the object’s behavior.
In conclusion, the decorator pattern is a powerful design pattern in computer science that allows for the dynamic addition of functionalities without modifying the object’s structure. It favors composition over inheritance and provides flexibility, extensibility, and the preservation of interface. By understanding and applying this pattern effectively, developers can create more modular and flexible systems.
What Is Decorator In C?
In the realm of programming, decorators play a vital role in enhancing the functionality and flexibility of code. In the C programming language, a decorator is a design pattern that allows the addition of new functionality to an existing object dynamically, at runtime.
C, being a procedural language, lacks built-in support for decorators. However, by understanding the concept of function pointers and the principles of the decorator design pattern, developers can effectively implement decorators in their C code.
How Does the Decorator Design Pattern Work?
The decorator design pattern consists of two key components: the base component (also known as the concrete component) and the decorator component. The base component provides the core functionality, while the decorator component adds new features to the base component.
The decorator component is implemented as a wrapper class or structure that takes an instance of the base component as its parameter. This allows the decorator to inherit the base component’s functionality while adding additional behavior.
To make use of the decorator pattern in C, developers commonly utilize function pointers. These function pointers, combined with the power of the decorator design pattern, enable the dynamic addition of new functionality to an existing object.
Implementing Decorators in C
To understand how decorators are implemented in C, let’s consider an example of a simple text processor. We have a base text processor component that performs basic operations such as reading, writing, and formatting text. Now, we want to extend its functionality by adding a decorator that will perform spell checking.
First, we define the base text processor component:
“`
typedef struct {
void (*read)(char* text);
void (*write)(char* text);
void (*format)(char* text);
} TextProcessor;
“`
Next, we define the spell checker decorator component:
“`
typedef struct {
TextProcessor* textProcessor;
} SpellCheckerDecorator;
“`
The above code snippets demonstrate the declarations of the base text processor and spell checker decorator. The TextProcessor structure contains function pointers for reading, writing, and formatting text.
Now, to implement the decorator, we define functions for each operation:
“`
void readText(char* text) {
// Custom logic for reading text
}
void writeText(char* text) {
// Custom logic for writing text
}
void formatText(char* text) {
// Custom logic for formatting text
}
void spellCheck(TextProcessor* textProcessor, char* text) {
// Custom logic for spell checking
}
“`
With the necessary functions defined, we can now create the decorator by linking the appropriate functions:
“`
void decorateWithSpellChecker(TextProcessor* textProcessor) {
textProcessor->read = readText;
textProcessor->write = writeText;
textProcessor->format = formatText;
// Additional functionality of spell checking
SpellCheckerDecorator* spellChecker = malloc(sizeof(SpellCheckerDecorator));
spellChecker->textProcessor = textProcessor;
spellChecker->textProcessor->read = readText;
spellChecker->textProcessor->write = writeText;
spellChecker->textProcessor->format = formatText;
spellChecker->spellCheck = spellCheck;
}
“`
FAQs
Q: Can decorators be applied to any object in the C programming language?
A: In C, decorators can be implemented on any object or component as long as it follows the structure and function pointers required by the decorator design pattern.
Q: What are the advantages of using decorators in C?
A: Decorators enhance code flexibility by allowing dynamic addition of features to existing objects. This reduces the need for modifying and recompiling existing code, thereby promoting code reusability and maintainability.
Q: Are decorators limited to a single functionality addition?
A: No, decorators can be chained together to add multiple functionalities to an object. Each decorator can add a specific feature without affecting the others.
Q: Are decorators used exclusively in C programming?
A: No, decorators are a design pattern that can be implemented in various programming languages, including C++. However, the implementation details might differ based on language-specific features.
Q: Are decorators commonly used in real-world applications?
A: Yes, decorators are frequently used in various software applications where the flexibility and scalability of code are crucial. They are especially useful in scenarios where multiple features need to be added dynamically without modifying existing code.
In conclusion, decorators in C facilitate the dynamic addition of new functionality to an existing object. By leveraging the decorator design pattern alongside function pointers, developers can enhance the flexibility and scalability of their C programs. Understanding the principles and implementation details of decorators is vital for creating robust and maintainable code.
Keywords searched by users: decorator pattern in c# Decorator pattern, Decorator Pattern la gì, Decorator design pattern wiki, Decorator pattern Python, Decorator pattern c++ pizza, Strategy pattern, Composite design pattern example, Composite pattern
Categories: Top 96 Decorator Pattern In C#
See more here: nhanvietluanvan.com
Decorator Pattern
When it comes to developing software applications, ensuring flexibility, code reusability, and maintainability are key considerations. One design pattern that helps achieve these objectives is the Decorator pattern. This article will delve into the Decorator pattern, exploring its essence, use cases, implementation details, and benefits. By the end, you’ll have a clear understanding of how to apply this powerful pattern in your own software projects.
1. Understanding the Decorator Pattern:
The Decorator pattern is a structural design pattern that allows behavior and functionality to be dynamically added to an individual object, without the need to modify the underlying class structure. It involves creating a decorator class that wraps the original object and provides additional functionality by extending or modifying its behavior.
2. Use Cases:
The Decorator pattern is particularly beneficial when we want to add functionality to an object at runtime, dynamically and transparently. This eliminates the need to predict all possible combinations of features at compile-time and provides a way to extend a class’s functionality without modifying its structure. Some common use cases for the Decorator pattern include:
– Adding new features to a software component without modifying its original code.
– Modifying the behavior of an object temporarily or conditionally.
– Adding responsibilities to objects without creating numerous subclasses.
– Ensuring a class’s core functionality remains unchanged while adding optional features.
3. Implementation Details:
To implement the Decorator pattern, we typically follow these steps:
– Create an interface or abstract class defining the core functionality.
– Implement the concrete class representing the base or core object.
– Create a decorator class that implements the same interface/abstract class and maintains a reference to the core object.
– In the decorator class, wrap the core object and add additional functionality either before or after delegating to the core object.
4. Example:
To illustrate the Decorator pattern, let’s consider a simple coffee ordering system. We have a base Coffee class representing a basic cup of coffee. We extend this class to create various types of coffee, such as Espresso, Cappuccino, and Decaf. Now, suppose we want to add additional features like adding flavors (vanilla, caramel, etc.) or extra toppings (whipped cream, chocolate sprinkles, etc.) to our coffee dynamically. Instead of modifying the core Coffee class, we create decorators for each additional feature and wrap the core coffee object with them. This way, we can dynamically add any combination of flavors and toppings to a specific coffee order without modifying its original implementation.
5. Benefits of Using the Decorator Pattern:
Implementing the Decorator pattern brings several benefits to your software development process:
– Flexibility: With the Decorator pattern, you can easily add or remove features from an object at runtime, providing a high degree of flexibility.
– Scalability: The pattern allows easy extension of existing codebase, avoiding the creation of numerous subclasses.
– Reusability: Decorators can be reused across multiple objects or projects.
– Transparent functionality enhancement: Decorators wrap the original object and add functionality seamlessly without affecting the client’s code, thus maintaining code coherence.
– Open-Closed Principle: By using decorators, you can extend functionality without modifying the existing code, adhering to the Open-Closed Principle.
– Clear separation of concerns: The Decorator pattern helps separate core functionality from optional features, promoting modular and organized code.
FAQs:
Q1. How does the Decorator pattern differ from subclassing?
A1. In subclassing, additional functionality is added by creating new derived classes, leading to the proliferation of class hierarchies and making code management complex. In contrast, the Decorator pattern allows us to extend functionality dynamically by wrapping the original object with decorators, eliminating the need for excessive subclasses.
Q2. Can the Decorator pattern be combined with other design patterns?
A2. Yes, the Decorator pattern can be combined with other patterns like the Factory, Singleton, or Composite patterns to enhance its functionality. For example, a Singleton Object Factory can be used to create and manage decorated objects.
Q3. Can decorators be stacked indefinitely?
A3. Yes, decorators can be stacked indefinitely to add as many features as desired. Each decorator enhances the functionality while still maintaining reference to the original object.
In conclusion, the Decorator pattern is a valuable tool for enhancing flexibility, maintainability, and code reusability in software development. By allowing dynamic addition of features to objects at runtime, the Decorator pattern empowers developers to extend functionality without modifying core classes. By implementing this pattern, you can create modular and scalable code that adapts to changing requirements effortlessly.
Decorator Pattern La Gì
Design patterns are an essential part of software development, offering reusable solutions to common problems. One such pattern is the Decorator pattern, which allows for dynamic adding of behaviors or functionalities to objects at runtime. In this article, we will explore the Decorator pattern in depth, discussing its purpose, structure, implementation, and benefits.
What is the Decorator Pattern?
The Decorator pattern, also known as the Wrapper pattern, is a structural design pattern that provides a flexible way to allow object composition and enhance the functionality of individual objects without modifying their structure. It is employed when we want to add new features or behaviors to objects dynamically at runtime.
Understanding the Structure of the Decorator Pattern
At the core of the Decorator pattern is the notion of wrapping one object with another. This is achieved through the use of a common interface or abstract class that both the original object and the decorators implement. The decorators have the same base class as the object they decorate, ensuring they maintain the same type.
The basic components of the Decorator pattern include:
1. Component: This represents the common interface or abstract class that defines the core functionality for both the original object and the decorators.
2. Concrete Component: This is the original object to which we want to add additional functionality at runtime.
3. Decorator: This is the base class for all decorators and implements the common interface. It contains a reference to the component being decorated.
4. Concrete Decorators: These are the actual decorators that extend the Decorator base class and add specific functionalities by wrapping the component reference.
How is the Decorator Pattern Implemented?
To implement the Decorator pattern, we follow a few key steps:
1. Create an interface or abstract class for the component and define the core functionality that all objects, including the decorators, will implement.
2. Implement the concrete component class that provides the base functionality.
3. Create a base decorator class that extends the component’s interface or abstract class and includes a reference to the component.
4. Implement concrete decorators that extend the base decorator class and add additional functionalities by wrapping the component.
Benefits of the Decorator Pattern
The Decorator pattern offers numerous benefits, making it a valuable tool for designing flexible and extensible systems. Here are some key advantages:
1. Enhanced flexibility: The Decorator pattern allows for the dynamic addition and removal of functionalities at runtime. This flexibility makes it easier to modify individual objects without impacting the overall structure.
2. Open-Closed Principle compliance: The Decorator pattern follows the Open-Closed Principle, as it allows for extension without modifying the existing code. New functionalities can be added by simply creating new decorators, rather than modifying existing classes.
3. Single Responsibility Principle adherence: By separating functionalities into decorators, each decorator can have a single responsibility, promoting better code organization and maintainability.
4. Easy combination of functionalities: The Decorator pattern enables the flexible combination of different decorators, allowing for the creation of various combinations of functionalities based on specific requirements.
Frequently Asked Questions (FAQs):
Q: What is the difference between the Decorator pattern and inheritance?
A: Inheritance is a mechanism that defines an “is-a” relationship between classes. It focuses on extending the behavior of classes by inheriting and refining existing functionalities. On the other hand, the Decorator pattern creates a “has-a” relationship, allowing objects to dynamically add or modify behaviors at runtime without changing their structure.
Q: Why should I use the Decorator pattern instead of subclassing?
A: Subclassing can quickly lead to a complex class hierarchy, making it harder to maintain and extend. The Decorator pattern, however, provides a more flexible and scalable solution. It allows for the dynamic composition and combination of functionalities at runtime, ensuring a cleaner and more maintainable codebase.
Q: When should I use the Decorator pattern?
A: The Decorator pattern is particularly useful in scenarios where you need to add or modify an object’s behavior dynamically and where you want to avoid bloating the class hierarchy with numerous subclasses. It is also helpful when you want to adhere to the Open-Closed Principle and promote code reuse.
Q: Can I use the Decorator pattern in combination with other design patterns?
A: Yes, the Decorator pattern can be combined with other design patterns to solve more complex problems. For example, it can work well with the Composite pattern to create a recursive structure of decorators. Additionally, it can be used in conjunction with the Factory pattern to dynamically create decorated objects.
In conclusion, the Decorator pattern offers an elegant solution for enhancing the functionalities of objects at runtime. Its flexibility, adherence to design principles, and ease of combination make it an invaluable tool for creating scalable and maintainable software systems. By understanding the structure and implementation details of the Decorator pattern, developers can leverage its benefits to design robust and extensible applications.
Images related to the topic decorator pattern in c#
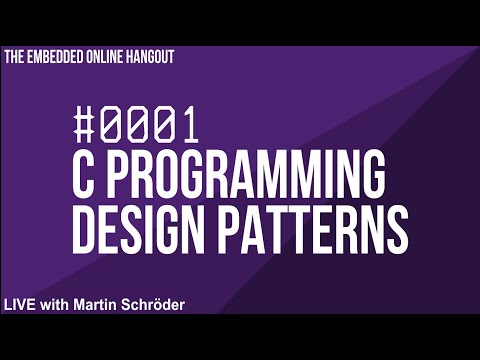
Article link: decorator pattern in c#.
Learn more about the topic decorator pattern in c#.
- C decorator design pattern (using the pizza example)
- C# Decorator Design Pattern – Dofactory
- Decorator pattern – Wikipedia
- Decorator in C++ / Design Patterns – Refactoring.Guru
- C Programming Design Patterns For Embedded Development
- Decorator Design Pattern – Scaler Topics
- Design Patterns – Decorator Pattern – Tutorialspoint
- Design Patterns: Decorator Pattern – 2020 – BogoToBogo
- Decorator Pattern | Set 3 (Coding the Design) – GeeksforGeeks
- The Decorator Pattern – ModernesCpp.com
- Hiểu biết cơ bản về Decorator pattern – Viblo
- Design patterns in C# – The Decorator Pattern – Endjin