Cypress Test Input Value
Cypress is a popular end-to-end testing framework that allows developers to write reliable and efficient tests for web applications. One crucial aspect of testing web applications is validating and manipulating input values. Cypress provides a variety of commands and assertions to facilitate input value testing.
In this article, we will explore the importance of input value testing in Cypress and how to effectively validate input values using Cypress commands and assertions. We will also discuss how to handle different types of input fields and deal with dynamic input values. Finally, we will share some best practices for testing input values with Cypress.
Introduction to Cypress Test Input Value
Testing input values is essential to ensure that web applications function correctly and provide the expected results. Input values can come from various sources, including user input, API responses, or dynamic changes in the application. Ensuring that the input values are correctly handled and processed is crucial to maintain the integrity and functionality of the application.
Cypress provides a comprehensive set of tools and utilities to test input values in web applications. These tools allow developers to input values into various form fields, validate the entered values, and handle different scenarios like empty inputs or dynamically changing values.
Understanding the Importance of Input Value Testing in Cypress
Testing input values in Cypress is vital for several reasons. Firstly, it helps uncover any bugs or issues related to input handling and processing. By simulating different input scenarios, developers can identify and fix potential problems proactively.
Secondly, input value testing ensures that the user interface correctly reflects the entered values. It is common for web applications to display entered values in various parts of the UI, such as confirmation messages or user profiles. By validating the displayed values against the input values, developers can ensure data consistency and accuracy.
Implementing Cypress Commands to Input Values in Tests
Cypress provides a straightforward and intuitive way to input values into form fields. The `type` command is commonly used to simulate user input in text fields, text areas, and other input elements. For example, to input a value into a text field with the attribute `name=”username”`, we can use the following Cypress command:
“`
cy.get(‘input[name=”username”]’).type(‘john.doe’)
“`
This command locates the input field with the specified name attribute and types the value `’john.doe’` into it.
Validating Input Values Using Cypress Assertions
After inputting values into form fields, it is essential to validate that the entered values are correctly processed. Cypress provides a range of assertions to validate the input values. The `should` command is commonly used in combination with assertions to verify the expected values.
For instance, to assert that a text field with the attribute `id=”email”` contains a non-empty value, we can use the following Cypress command:
“`
cy.get(‘#email’).should(‘not.be.empty’)
“`
This command checks if the specified input field is not empty and ensures that the entered value is successfully processed.
Handling Different Types of Input Fields in Cypress Tests
Web applications often contain various types of input fields, such as checkboxes, radio buttons, drop-down selects, and more. Cypress provides specific commands and assertions to handle different types of input fields effectively.
For example, to select a checkbox with the attribute `name=”agree”`, we can use the `check` command:
“`
cy.get(‘input[name=”agree”]’).check()
“`
Similarly, to select an option from a drop-down select with the attribute `id=”country”`, we can use the `select` command:
“`
cy.get(‘#country’).select(‘United States’)
“`
Dealing with Dynamic Input Values in Cypress Tests
Web applications often have dynamic input values that change based on user actions or API responses. To test such scenarios, Cypress provides commands like `invoke` and `within`.
The `invoke` command allows developers to invoke a function or method on the selected element. This is particularly useful when the input value depends on an external factor, such as a time-based calculation or an API response.
Additionally, the `within` command is used to target specific elements within a parent element. It enables developers to scope their assertions and commands to a specific section of the UI.
Best Practices for Testing Input Values with Cypress
To ensure effective and reliable input value testing with Cypress, consider these best practices:
1. Cypress recommends using the `force` option when interacting with disabled or read-only input fields. This option allows bypassing any built-in validation or restrictions on the input elements.
2. Make use of assertions to verify the input values at different stages of the testing process. Assertions provide clear feedback on whether the input values are correctly processed or displayed in the UI.
3. Use attribute-based selectors, like `id` or `name`, to locate input fields. These selectors offer better stability compared to other selectors like class names or element types, as attributes are less likely to change.
4. Leverage Cypress commands like `invoke` and `within` to handle dynamic input values effectively. These commands provide the flexibility to test scenarios where input values change based on user interactions or API responses.
FAQs
1. How can I get the value of an input field using Cypress?
To get the value of an input field, you can use the `invoke` command with the `’val’` argument. For example:
“`
cy.get(‘#myInput’).invoke(‘val’).should(‘equal’, ‘example value’)
“`
2. How can I check if an input field is not empty using Cypress?
To check if an input field is not empty, you can use the `should` command with the `’not.be.empty’` assertion. For example:
“`
cy.get(‘#myInput’).should(‘not.be.empty’)
“`
3. How can I get the attribute value of an element using Cypress?
To get the attribute value of an element, you can use the `invoke` command with the attribute name. For example, to get the `href` attribute value of a link element:
“`
cy.get(‘a’).invoke(‘attr’, ‘href’).should(‘equal’, ‘https://example.com’)
“`
4. How can I get the text of an element using Cypress?
To get the text of an element, you can use the `invoke` command with the `’text’` argument. For example:
“`
cy.get(‘#myElement’).invoke(‘text’).should(‘equal’, ‘example text’)
“`
5. How can I get an input field by name using Cypress?
To get an input field by its name attribute, you can use the `get` command with the `[name=””]` selector. For example:
“`
cy.get(‘input[name=”myInput”]’).type(‘example value’)
“`
6. How can I clear the value of an input field using Cypress?
To clear the value of an input field, you can use the `clear` command. For example:
“`
cy.get(‘#myInput’).clear()
“`
7. What does the `force: true` option do in Cypress input value testing?
The `force: true` option in Cypress allows bypassing any built-in validation or restrictions on the input elements. It is useful when interacting with disabled or read-only input fields that would otherwise prevent user input.
How To Handle Inputs Or Textbox Or Textarea In Cypress
How To Get Input Text Value In Cypress?
Cypress is a powerful end-to-end testing framework for web applications. It provides a seamless and efficient way to write tests that simulate user interactions within a web browser. One common scenario in testing is to retrieve the value of an input text field. In this article, we will explore various methods to get the input text value in Cypress and discuss their usage in detail.
Getting the input text value with Cypress is relatively easy. Let’s start by understanding the basic structure of an input element. An input element typically consists of an opening tag, a value attribute, and a closing tag. The value attribute holds the text entered by the user into the input field.
To access the value of an input field in Cypress, we can utilize the cy.get() command. This command allows us to target specific elements on the page using CSS selectors. By specifying the selector for the input field, we can retrieve the input value using the .invoke() method. The .invoke() method is used to execute a function on a given subject and retrieve its return value.
Here is an example of how we can get the input text value using Cypress:
“`javascript
cy.get(‘input[name=”username”]’).invoke(‘val’).then((text) => {
// `text` holds the input value
cy.log(text);
});
“`
In the example above, we first use the cy.get() command to select the input field with the name attribute equal to ‘username’. Then we chain the .invoke() method to execute the ‘val’ function, which returns the value of the input field. Finally, we use the .then() method to handle the retrieved value and log it to the Cypress command log.
Another way to get the input text value is by using the .type() command in Cypress. The .type() command simulates user keystrokes and can populate input fields. By chaining the .type() command with a blank string, we can overwrite the input field’s current value and retrieve it at the same time.
Here is an example of how we can use the .type() command to get the input text value:
“`javascript
cy.get(‘input[name=”username”]’).type(”).then((text) => {
// `text` holds the input value
cy.log(text);
});
“`
In the above example, we again use the cy.get() command to select the input field, followed by the .type() command with a blank string. This action triggers the clearing of the input field’s value, but the current value is returned and can be handled using the .then() method. We can then log the retrieved value to the Cypress command log.
It’s important to note that using the .type() command to retrieve the input text value should only be considered when we also need to clear the input field’s value. Otherwise, the .invoke(‘val’) method is more appropriate and explicit.
FAQs about Getting Input Text Value in Cypress:
Q: Can I get the input text value without clearing it?
A: Yes, you can use the .invoke(‘val’) method to retrieve the input text value without clearing it. This method specifically retrieves the value of the input field without modifying its current state.
Q: How do I handle input fields with dynamic IDs or attributes?
A: Cypress provides a wide range of powerful selectors, including CSS and XPath selectors. To handle input fields with dynamic IDs or attributes, you can use wildcard characters or other attribute matching techniques. For example, you can use the `[attribute^=”value”]` selector to match elements with attributes beginning with a specific value.
Q: What if the input field is inside an iframe?
A: If the input field is located within an iframe, you can use the cy.iframe() command to switch to the iframe context and then access the input field using cy.get() or other relevant commands. The .invoke() and .type() methods can be used as usual to retrieve the input text value.
Q: How can I verify the retrieved input text value in my test?
A: Cypress provides multiple ways to assert and verify values. You can use the .should() command along with appropriate matchers, such as .should(‘have.value’, expectedValue), to validate the retrieved input text value against an expected value. Additionally, you can chain assertions and perform more complex checks by using Cypress’s built-in commands and utilities.
In conclusion, retrieving the input text value in Cypress is a common requirement in testing scenarios. By using the cy.get() command along with the .invoke(‘val’) or .type() methods, we can easily access and handle the value of an input field. It’s important to consider the context and purpose of the test when choosing the appropriate method. It’s also crucial to validate the retrieved value using Cypress’s built-in assertions or custom assertions for robust and reliable tests.
How To Enter Input In Cypress?
Cypress is a modern JavaScript-based end-to-end testing framework that allows developers to write tests directly in the browser. With its powerful features and simple syntax, Cypress has gained popularity among developers for testing web applications. One of the crucial aspects of writing effective tests is the ability to accurately enter input into web pages and test various user interactions. In this article, we will explore how to enter input in Cypress, covering different scenarios and techniques to make your tests more robust.
1. Entering Text Input:
Entering text into input fields is a common scenario in web applications. Cypress provides a convenient way to simulate user input using the `type` command. To use this command, first target the input element using a Cypress selector, and then invoke the `type` command, passing the desired input as an argument. For example, to enter the text “Hello, World!” into the input field with the id “textInput”, you can use the following code:
“`
cy.get(‘#textInput’).type(‘Hello, World!’);
“`
Cypress will automatically trigger the necessary events to simulate a real user typing into the input field.
2. Entering Special Characters:
Sometimes, you may need to simulate entering special characters or key combinations like enter or tab. Cypress provides the flexibility to handle such scenarios using the `type` command’s additional options. To enter special characters, pass them as separate strings after the input text. For example, to enter the text “Hello, Cypress!” followed by pressing the enter key, you can use the following code:
“`
cy.get(‘#textInput’).type(‘Hello, Cypress!{enter}’);
“`
Similarly, you can enter other special characters like “{tab}”, “{backspace}”, or even multiple keys such as “{ctrl}{a}” for selecting all text.
3. Selecting Options in Dropdowns:
Dropdown menus are widely used in web forms. To test selecting options in dropdowns, first, use the `get` command to target the select element. Then, use the `select` command to choose the desired option. For example, to select the option with the value “option2” in a select element with the id “mySelect”, you can use the following code:
“`
cy.get(‘#mySelect’).select(‘option2’);
“`
Cypress will automatically handle the dropdown and select the specified option.
4. Checking Checkboxes and Radio Buttons:
Testing checkboxes and radio buttons is essential to ensure correct user interactions. To check a checkbox or select a radio button, use the `check` or `click` command, respectively. First, target the element using a Cypress selector, and then use the appropriate command. For example, to check a checkbox with the id “myCheckbox”, you can use the following code:
“`
cy.get(‘#myCheckbox’).check();
“`
To select a radio button, replace the `check()` command with the `click()` command.
5. Frequently Asked Questions (FAQs):
Q1. How can I clear an input field before entering new text?
– To clear an input field before entering new text, you can use the `clear` command provided by Cypress. Target the input element using a Cypress selector, and then invoke the `clear` command. For example:
“`
cy.get(‘#textInput’).clear().type(‘New Text’);
“`
Q2. Can I simulate user interactions like hovering or dragging using Cypress?
– Yes, Cypress provides APIs to simulate user interactions like hovering, dragging, and more. You can use commands like `trigger`, `drag`, and `should` in combination with Cypress selectors to achieve these interactions. Refer to Cypress documentation for detailed examples and usage.
Q3. How do I handle input field auto-suggestions or dropdown autosuggestions in Cypress?
– Cypress allows you to stub network requests and intercept responses. If the input field auto-suggestions or dropdown values are populated dynamically based on external data sources, you can stub the network requests to simulate different scenarios and test how the application behaves. Cypress provides methods like `cy.server`, `cy.route`, and `cy.request` to help you intercept and modify network requests.
In conclusion, Cypress offers a powerful and intuitive way to enter input in web pages during testing. Whether it’s entering text, selecting options, checking checkboxes, or interacting with different elements, Cypress provides a comprehensive set of commands to handle various scenarios. By using the techniques outlined in this article, you can write reliable and efficient tests to ensure your web applications are functioning as expected.
Keywords searched by users: cypress test input value Cypress get value of input, Cypress check input value not empty, Cypress get attribute value, Cypress get text of element, Cypress get input by name, Get text Cypress, Cypress clear input, Force: true Cypress
Categories: Top 34 Cypress Test Input Value
See more here: nhanvietluanvan.com
Cypress Get Value Of Input
Getting the value of an input field is fairly straightforward in Cypress. The framework provides several methods that allow us to access and manipulate input elements on a webpage. One of the most commonly used methods is the `.get()` command, which retrieves an element on the page that matches a specific selector. Once we have selected the input element, we can use the `.invoke()` command to retrieve its value.
To better understand how this works, let’s consider an example. Imagine we have a simple login form with two inputs: one for the username and another for the password. To get the value of the username input, we would write the following Cypress code:
“`javascript
cy.get(‘#usernameInput’).invoke(‘val’).then((value) => {
const username = value;
// Use the username value for further assertions or actions
});
“`
In the above code, `cy.get(‘#usernameInput’)` retrieves the input element with the id “usernameInput”. We then chain the `.invoke(‘val’)` command to get the value of that input. The `.then()` command is used to handle the retrieved value and store it in a variable called `username` for further use in our test.
Similarly, we can obtain the value of the password input using the following code:
“`javascript
cy.get(‘#passwordInput’).invoke(‘val’).then((value) => {
const password = value;
// Use the password value for further assertions or actions
});
“`
Once we have retrieved the input values, we can perform additional actions or assertions. For example, we can check if the input values are correct or if we successfully logged in using the respective credentials.
Now, let’s address some frequently asked questions related to getting the value of an input using Cypress:
**Q: Can I directly access the input value without using `.invoke(‘val’)`?**
A: Yes, Cypress provides an alternative method called `.type()` that not only sets the value of an input but also returns its value. However, using `.type()` solely for getting the input value is discouraged, as it simulates key presses and might cause unnecessary side effects during testing.
**Q: What if the input value is dynamically generated by JavaScript or AJAX calls?**
A: Cypress automatically waits for these dynamic changes, so you can use the same `.get().invoke(‘val’)` method to retrieve the updated input value.
**Q: How can I get the value of multiple inputs on a page?**
A: You can simply repeat the code snippet for each input field and modify the selector accordingly. For example, if you have three inputs with ids “input1,” “input2,” and “input3,” you can retrieve their values as follows:
“`javascript
cy.get(‘#input1’).invoke(‘val’).then((value) => {
const input1Value = value;
});
cy.get(‘#input2’).invoke(‘val’).then((value) => {
const input2Value = value;
});
cy.get(‘#input3’).invoke(‘val’).then((value) => {
const input3Value = value;
});
“`
**Q: Are there any alternatives to using `.invoke(‘val’)` to get an input value?**
A: Yes, Cypress offers other commands like `.should()`, `.invoke(‘prop’, ‘value’)`, or even custom commands to retrieve an input’s value. However, `.invoke(‘val’)` is the most commonly used and recommended method for obtaining an input value.
**Q: Can I chain multiple commands after retrieving the input value?**
A: Yes, after retrieving the input value, you can chain other Cypress commands like `.click()`, `.select()`, or `.type()` to perform additional actions or assertions based on the retrieved value.
In conclusion, retrieving the value of an input using Cypress is a simple task. The framework provides convenient commands like `.get()` and `.invoke(‘val’)` to make this process smooth and effortless. By employing these commands, we can easily retrieve input values and utilize them for various assertions and actions. Remember, Cypress offers several other commands to retrieve input values, but `.invoke(‘val’)` remains the most common and preferred method.
Cypress Check Input Value Not Empty
Introduction:
In web development, ensuring proper user input is crucial for creating a seamless user experience. One common requirement is to check if an input value is empty or not before performing any action. Cypress, the popular JavaScript end-to-end testing framework, provides a powerful set of tools to handle this task efficiently. In this article, we will explore different approaches to check input values using Cypress and delve deep into its implementation details.
Section 1: Understanding Cypress Commands for Input Handling
Before diving into the specifics of checking input values, it is important to familiarize yourself with Cypress commands related to input handling. Cypress provides a variety of commands such as ‘type’, ‘clear’, and ‘invoke’ that allow you to interact with input elements easily.
The ‘type’ command is commonly used to set input values programmatically. It simulates user typing by sending a series of key events to the specified input element. This command is useful for setting input values to test different scenarios.
The ‘clear’ command, on the other hand, clears the value of an input element. It ensures that the input field is empty before performing any actions.
Lastly, the ‘invoke’ command is used to invoke various methods on an element. For input elements, the ‘invoke’ command can be used to trigger events like onFocus, onBlur, or any custom event handlers.
Section 2: Checking Input Values Using Cypress
Now that we have a clear understanding of the Cypress commands related to input handling, let’s explore different approaches to check if an input value is empty or not.
Approach 1: Using the ‘should’ Assertion
Cypress provides the ‘should’ assertion to perform various assertions on DOM elements, including input elements. To check if an input value is empty, you can simply use the ‘should’ assertion with the ‘have.value’ method.
Here’s an example:
“`javascript
cy.get(‘input[type=”text”]’).should(‘have.value’, ”)
“`
Approach 2: Validating the ‘value’ property
Another approach to check if an input value is empty or not is by directly accessing the ‘value’ property of the input element. Cypress allows you to access element properties using the ‘invoke’ command.
Here’s an example:
“`javascript
cy.get(‘input[type=”text”]’).invoke(‘prop’, ‘value’).should(‘eq’, ”)
“`
Both approaches mentioned above are equally valid and serve the same purpose. You can choose the one that suits your preference or specific testing scenario.
Section 3: Dealing with Dynamic Input Values
In some cases, input values might be dynamically populated, making it challenging to check their emptiness. Cypress provides robust solutions to handle dynamic input values efficiently.
Approach 1: Waiting for a Specific Value
If an input value is dynamically populated and you need to wait for a specific value before proceeding further, you can use the ‘should’ assertion with a custom function. This function can evaluate the input value and return a boolean value indicating if the value meets your desired condition.
Here’s an example:
“`javascript
cy.get(‘input[type=”text”]’).should($input => {
const value = $input.val();
return value !== ”;
});
“`
Approach 2: Timing Assertions
Cypress allows you to set custom timeouts for commands and assertions. This feature is especially useful when dealing with input values that might take some time to load or change. By extending the timeout, you can ensure that Cypress waits until the input value is updated before checking for emptiness.
Here’s an example:
“`javascript
cy.get(‘input[type=”text”]’, { timeout: 10000 }).should(‘have.value’, ”);
“`
Section 4: FAQs
Q1. Can Cypress check input values for different input types?
A1. Yes, Cypress can check input values for various input types such as text, number, email, password, and more. You can use the appropriate selector to target specific input types and perform the necessary checks.
Q2. Is it possible to check if an input value is not empty?
A2. Absolutely! By slightly modifying the ‘should’ assertions, you can check if an input value is not empty. For instance, you can use the ‘not.eq’ assertion to check if the value is not equal to an empty string.
Q3. Can Cypress handle inputs within iframes?
A3. Yes, Cypress has excellent support for handling iframes. You can use the ‘cy.iframe()’ command to target specific iframes and then interact with the input elements within them.
Q4. What should I do if an input value changes dynamically but Cypress fails to detect it?
A4. In such cases, you can try using the ‘force’ option with Cypress commands like ‘type’ or ‘invoke’ to bypass any event handling that might prevent Cypress from detecting the value changes. However, exercise caution when using the ‘force’ option, as it may affect the accuracy of your tests.
Conclusion:
Checking if an input value is empty or not is a crucial aspect of web development, and Cypress provides an array of powerful tools to accomplish this task efficiently. By utilizing Cypress commands like ‘should’, ‘invoke’, and custom waiting techniques, you can seamlessly validate input values and ensure the smooth functioning of your web applications. So, leverage the power of Cypress and make input value validation an integral part of your testing strategy.
Cypress Get Attribute Value
Cypress is a popular JavaScript end-to-end testing framework that provides developers with an efficient and reliable way to automate browser-based tests. It offers a wide range of useful features, including the ability to interact with DOM elements and retrieve their attribute values. In this article, we will explore how to use Cypress to get attribute values, discuss its importance, and provide some frequently asked questions to help you maximize your testing capabilities.
Why is getting attribute values important?
Retrieving attribute values is a critical requirement in web application testing. It allows developers to verify that the attributes set on elements are correct based on their expected behavior. By checking attribute values, testers can validate that the correct data or state is being displayed, ensuring the integrity and accuracy of the tested application.
How to get attribute values using Cypress?
Cypress provides several reliable and easy-to-use methods to retrieve attribute values from DOM elements. Let’s explore the most common ways to achieve this:
1. Using the “invoke” method:
The “invoke” method in Cypress allows developers to invoke functions on elements. To get an attribute value using this approach, you can call the “invoke” method with the attribute name as the argument. For example, to retrieve the value of the “data-id” attribute, you can write:
“`javascript
cy.get(‘selector’).invoke(‘attr’, ‘data-id’);
“`
2. Using the “attr” method:
Cypress also provides the “attr” method, which directly retrieves the value of an attribute from an element. This method takes the attribute name as its argument and returns the attribute value. Here’s an example of how this can be done:
“`javascript
cy.get(‘selector’).invoke(‘attr’, ‘data-id’).then((attributeValue) => {
// Attribute value is stored in ‘attributeValue’ variable
});
“`
3. Using the “invoke” method with jQuery:
Cypress uses jQuery under the hood, giving developers access to its rich set of methods. To get attribute values using jQuery syntax, you can chain the “.attr()” method after selecting the element using Cypress. Here’s an example:
“`javascript
cy.get(‘selector’).invoke(‘attr’, ‘data-attribute’).then((attributeValue) => {
// Attribute value is stored in ‘attributeValue’ variable
});
“`
These are just a few examples of the approaches that Cypress offers to retrieve attribute values. Depending on your specific requirements and the structure of your application, you may find other methods more suitable. It’s always a good idea to consult Cypress documentation and experiment with different approaches to find the one that works best for you.
Frequently Asked Questions (FAQs):
Q1. How can I assert the value of an attribute using Cypress?
To assert the value of an attribute, you can use the “should” assertion method provided by Cypress. Here’s an example:
“`javascript
cy.get(‘selector’).invoke(‘attr’, ‘data-attribute’).should(‘equal’, ‘expectedValue’);
“`
Q2. Can I retrieve multiple attribute values at once?
Yes, Cypress allows you to retrieve multiple attribute values simultaneously using the “invoke” method. Simply provide an array of attribute names as arguments. Here’s an example:
“`javascript
cy.get(‘selector’).invoke(‘attr’, [‘attribute1’, ‘attribute2’]).then((attributeValues) => {
// Attribute values are stored in the ‘attributeValues’ array
});
“`
Q3. How can I check if an attribute exists on an element?
To verify if an attribute exists on an element, you can use the “should” assertion method with the “have.attr” property. Here’s an example:
“`javascript
cy.get(‘selector’).should(‘have.attr’, ‘data-attribute’);
“`
Q4. Can I retrieve the attribute of a specific element in a list?
Yes, Cypress allows you to select a specific element from a list using the “.eq(index)” method. Combined with the attribute retrieval methods discussed earlier, you can easily retrieve the attribute of a specific element. Here’s an example:
“`javascript
cy.get(‘selector’).eq(index).invoke(‘attr’, ‘data-attribute’);
“`
In conclusion, Cypress offers various methods to retrieve attribute values from DOM elements in an efficient and straightforward manner. By leveraging these methods, developers can ensure the accuracy and integrity of their web application tests. Remember to consult the official Cypress documentation for detailed usage instructions and explore the various possibilities that Cypress provides.
Images related to the topic cypress test input value
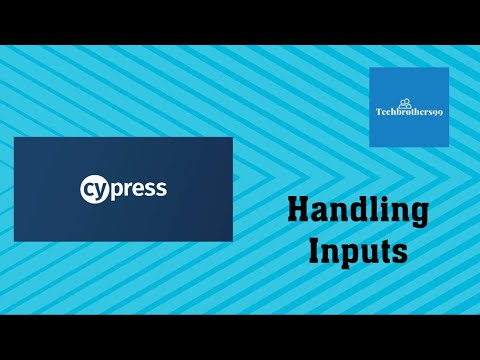
Found 13 images related to cypress test input value theme
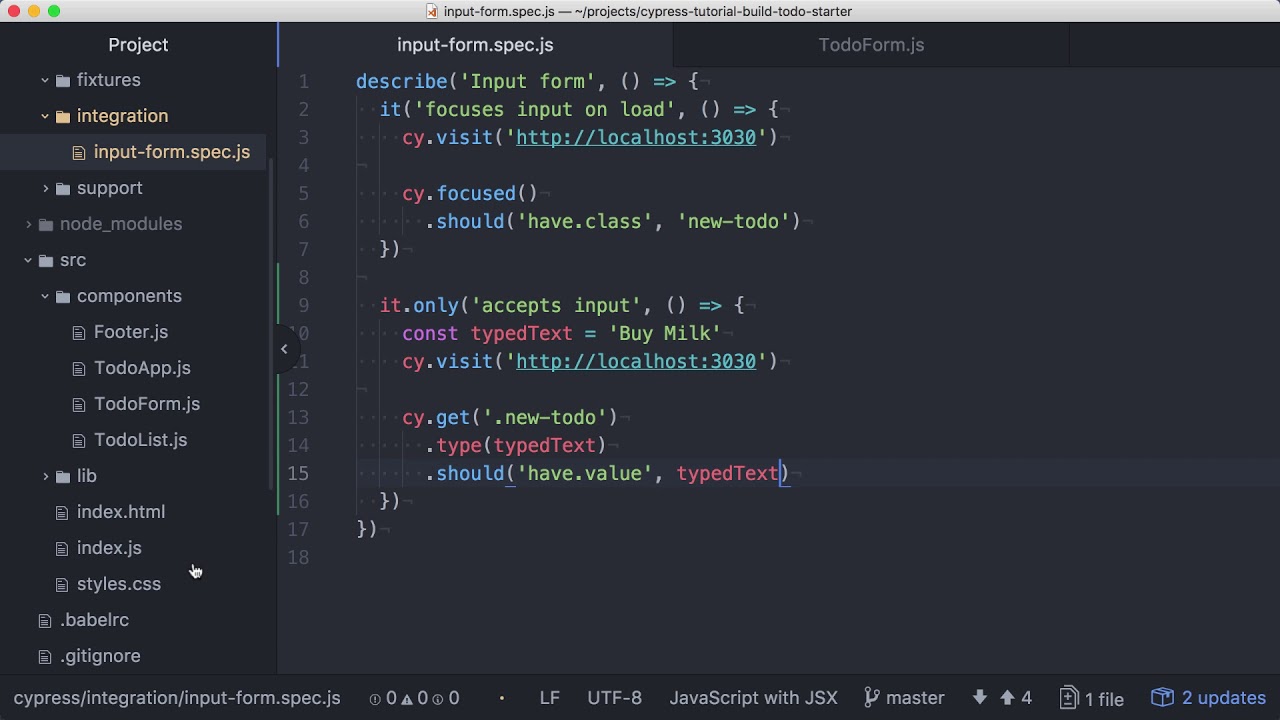
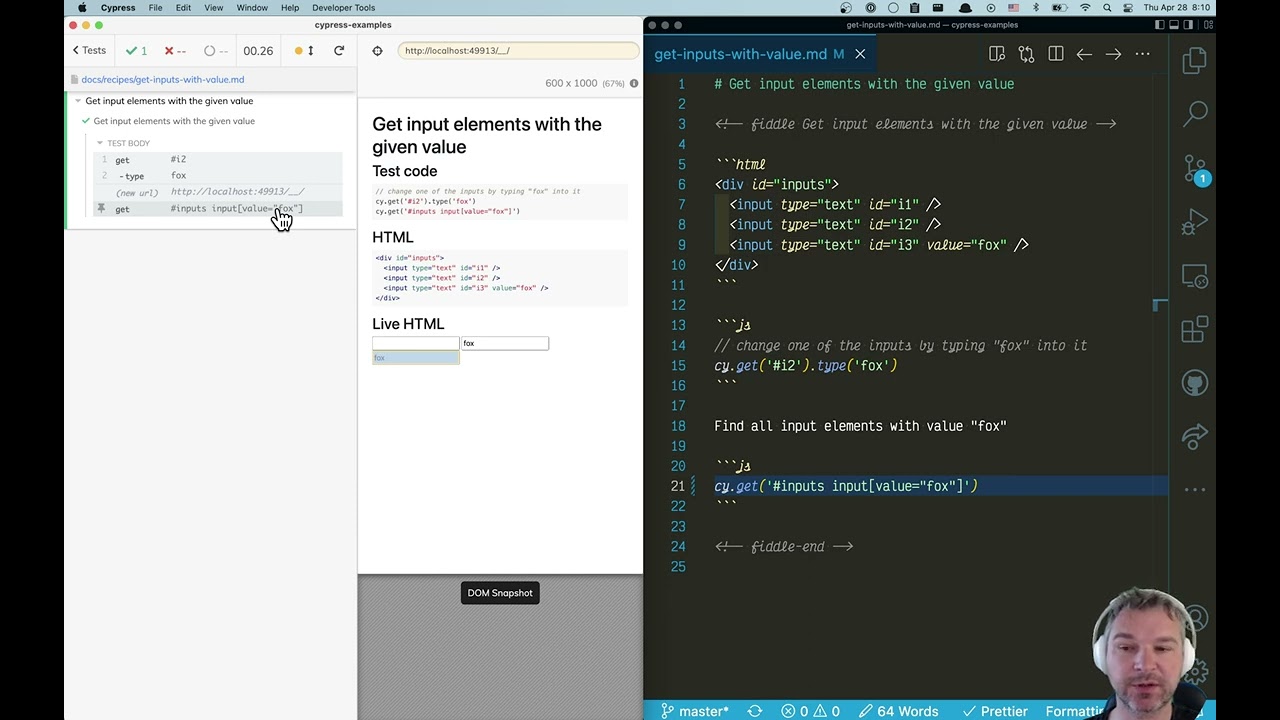
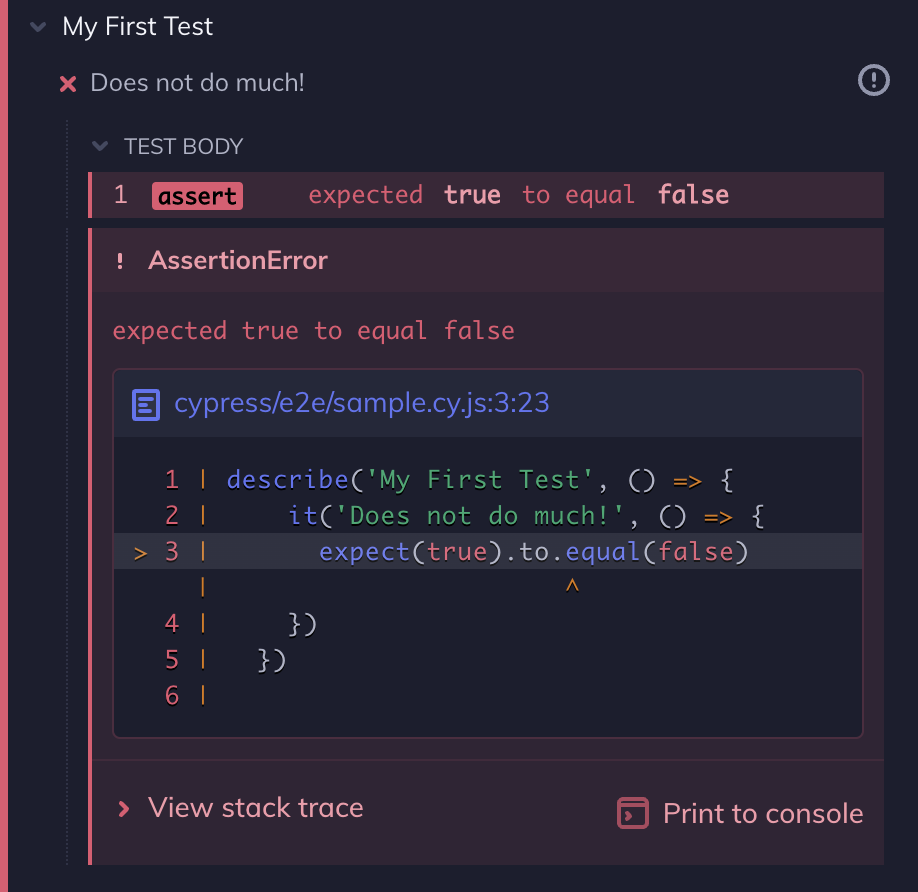








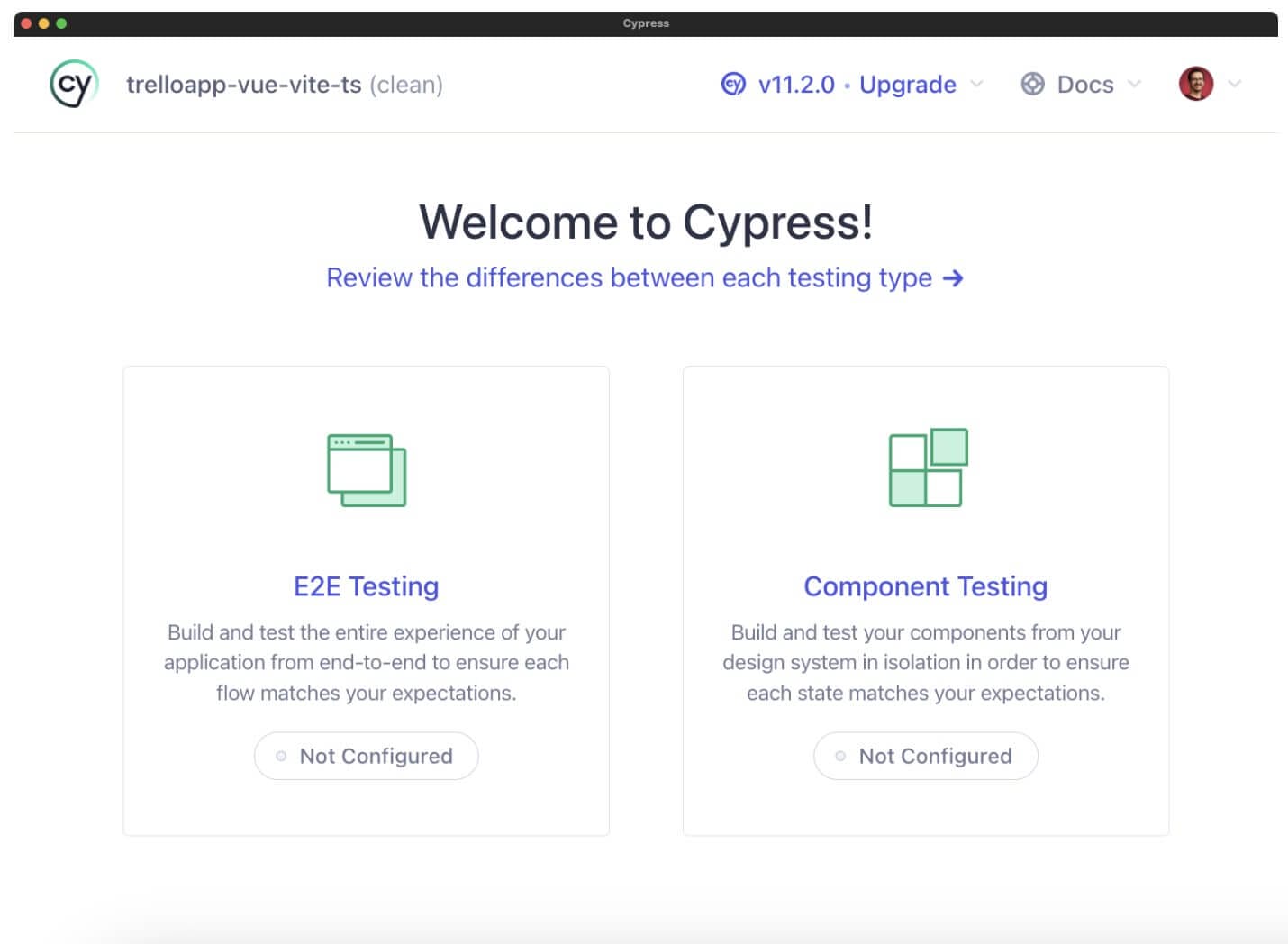
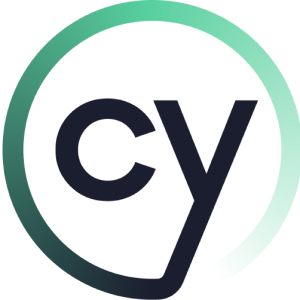

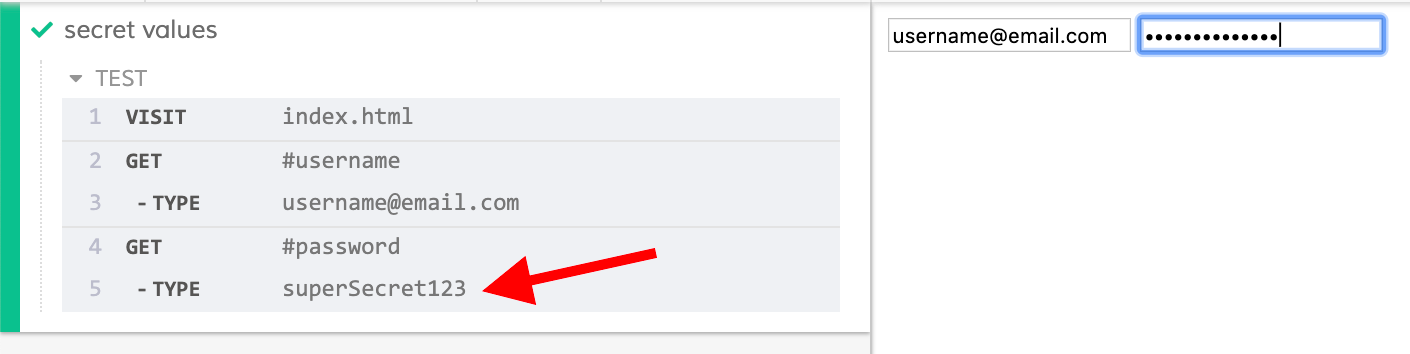



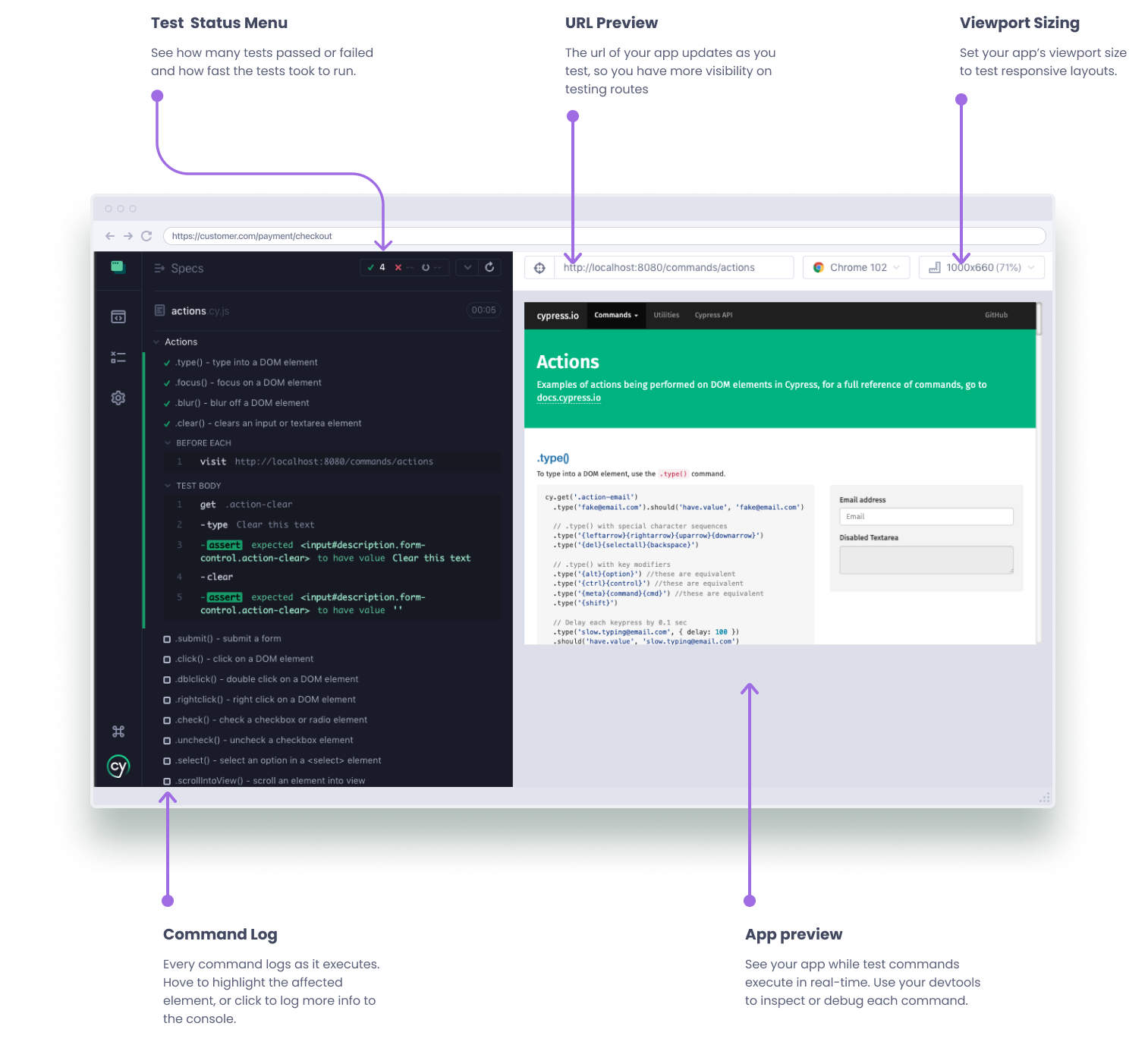




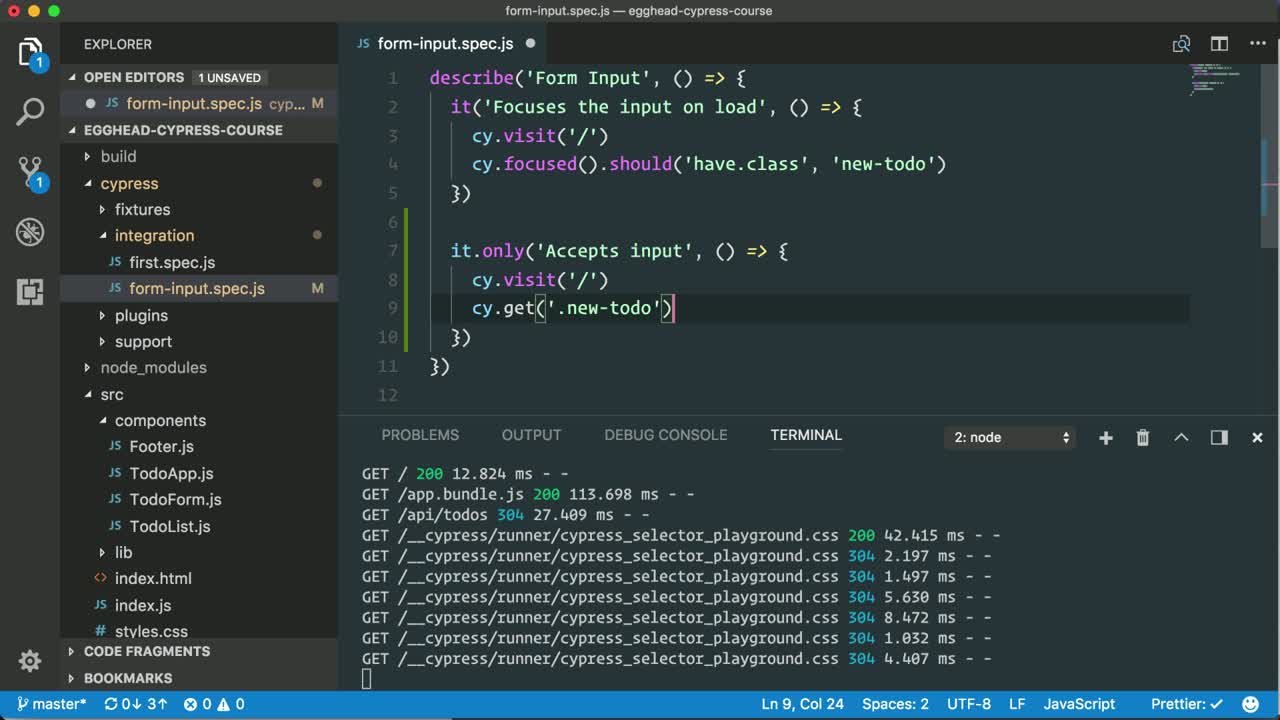
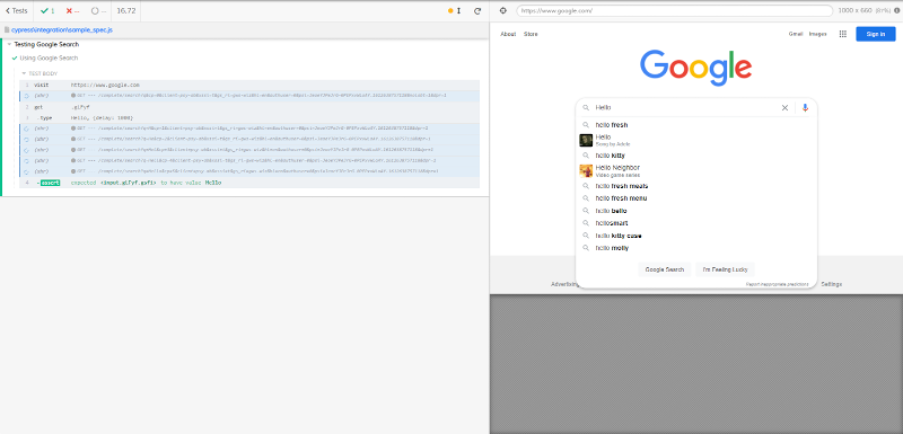
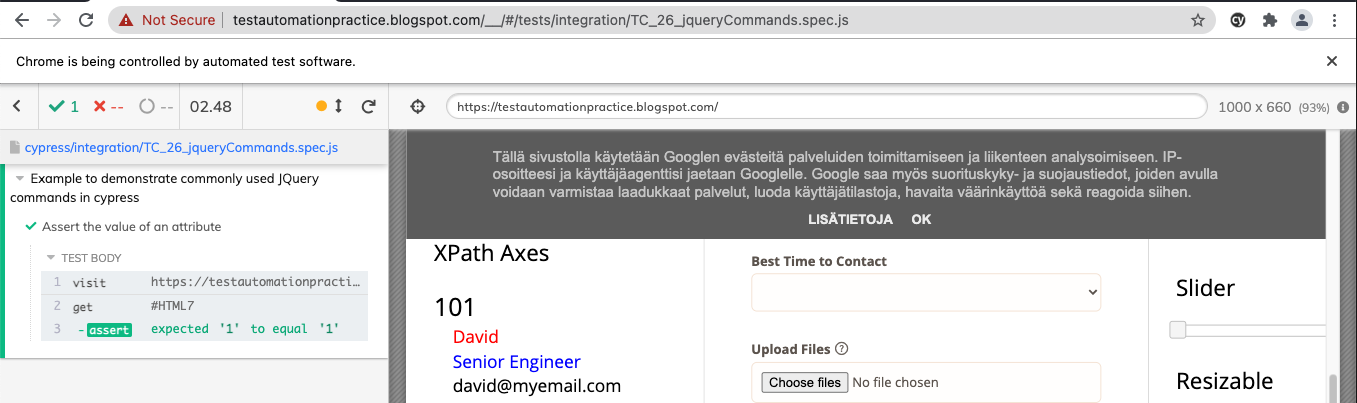

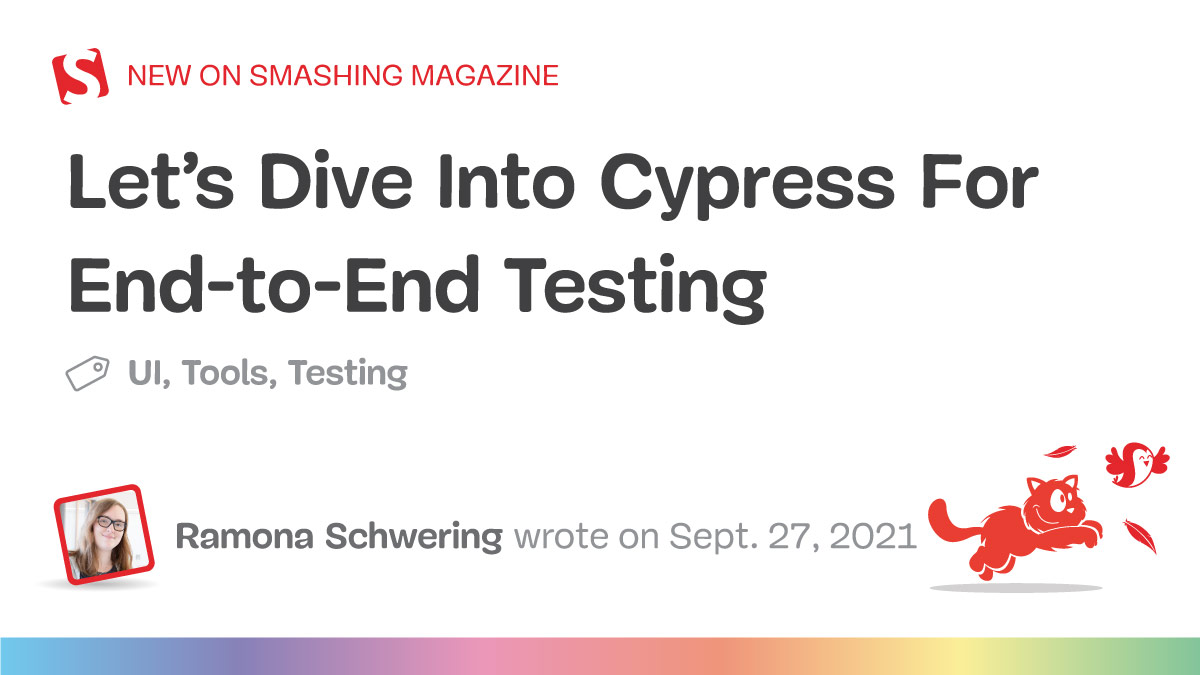
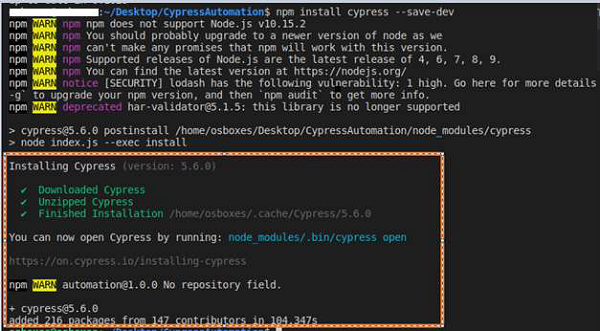


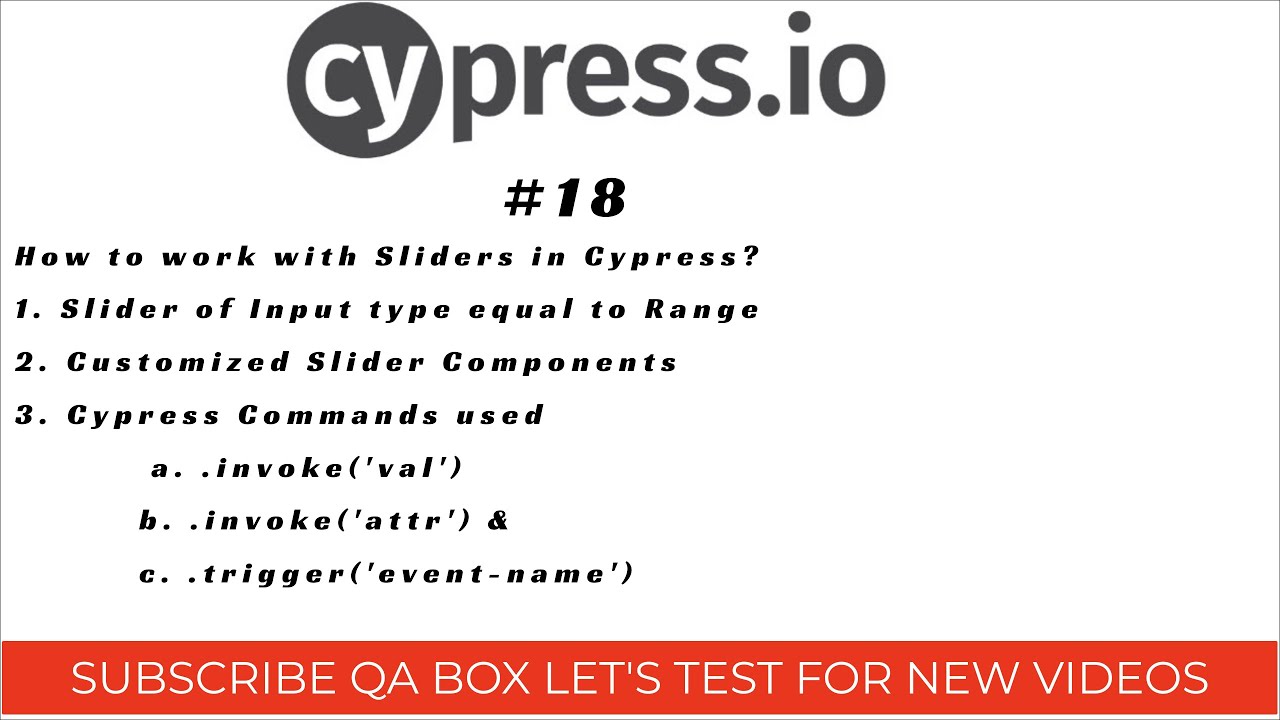
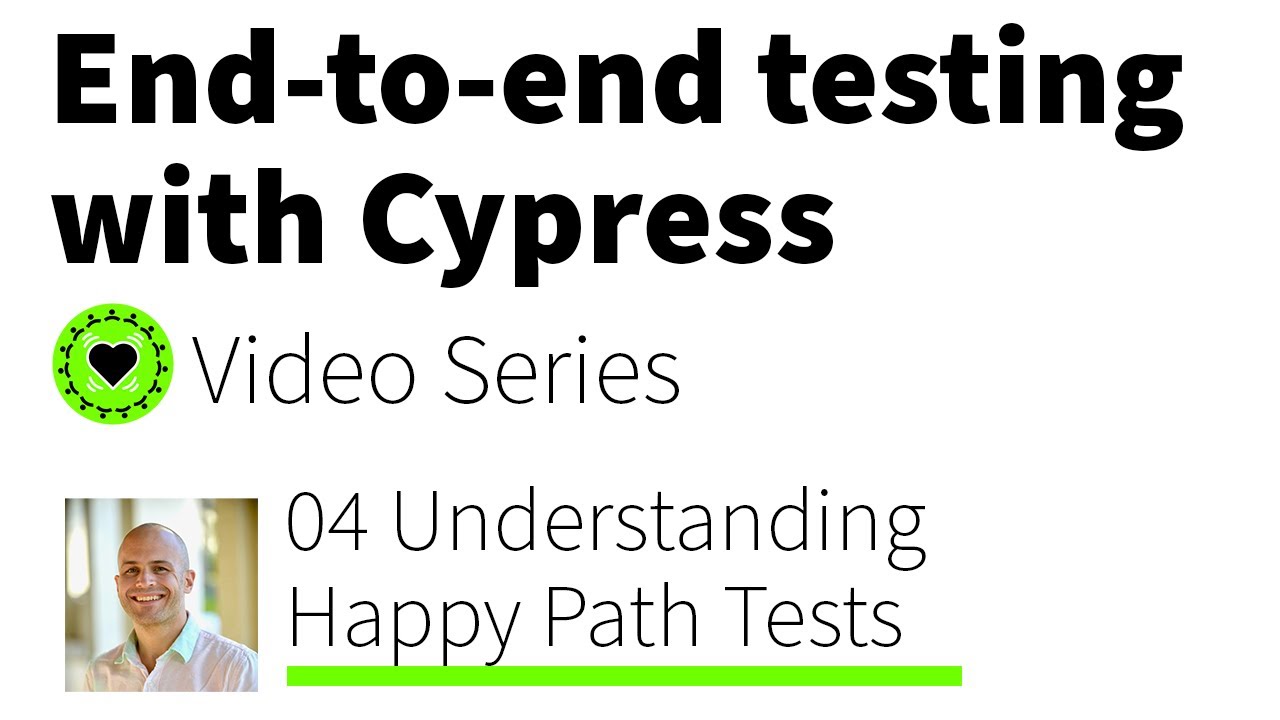




![Uploading a JSON File using API - Cypress.io [shorts] Uploading A Json File Using Api - Cypress.Io [Shorts]](https://digitalpress.fra1.cdn.digitaloceanspaces.com/omtmgjm/2021/07/sda.jpg)
Article link: cypress test input value.
Learn more about the topic cypress test input value.
- Asserting that an input element contains a specific value in …
- Two Ways to get a Form Input’s Value in Cypress
- Cypress Tips And Tricks: A Comprehensive Guide With …
- How to check for Attribute Values in Cypress? – BrowserStack
- How to check if an Element exists using Cypress? – BrowserStack
- Two Ways to get a Form Input’s Value in Cypress
- How to Work with Input Fields in Cypress | by Dilpreet Johal
- Cypress basics: Check attributes, value and text – Filip Hric
- Cypress Assertions Cheat Sheet – by Anshita Bhasin – Medium
- How to Fill and Submit Forms in Cypress | BrowserStack
See more: blog https://nhanvietluanvan.com/luat-hoc