Convert String To Byte Array C#
In the world of programming, there are various scenarios where you might need to convert a string into a byte array. This conversion process is essential when you want to work with binary data or when you need to transfer data over a network. In C#, there are multiple ways to achieve this conversion, depending on the encoding requirements and the purpose of the byte array. In this article, we will explore different methods to convert a string to a byte array in C# and also discuss best practices and considerations for this task.
What is a Byte Array?
A byte array is an object that represents a sequence of bytes. Each byte in the array can hold a value ranging from 0 to 255, giving a wide range of possible combinations. Byte arrays are commonly used in scenarios where binary data needs to be stored or transferred.
Why Convert a String to a Byte Array in C#?
Strings in C# are stored as Unicode characters, which means each character is represented by one or more bytes. There might be cases where you need to work with individual characters or modify the byte-level representation of a string. In such cases, converting the string to a byte array becomes necessary.
Converting a String to a Byte Array using Encoding.ASCII.GetBytes()
The Encoding.ASCII.GetBytes() method allows you to convert a string into a byte array using the ASCII encoding standard. ASCII encoding uses 7 bits to represent characters, allowing a maximum of 128 characters. This method is sufficient when you are dealing with English alphabets, digits, and a limited set of special characters.
Example usage:
“`
string str = “Hello World”;
byte[] byteArray = Encoding.ASCII.GetBytes(str);
“`
Converting a String to a Byte Array using Encoding.UTF8.GetBytes()
The Encoding.UTF8.GetBytes() method is used to convert a string into a byte array using the UTF-8 encoding standard. UTF-8 is a variable-width encoding scheme that can represent almost all characters in the Unicode character set. It is widely used in modern applications since it provides compatibility with multiple languages and scripts.
Example usage:
“`
string str = “你好世界”;
byte[] byteArray = Encoding.UTF8.GetBytes(str);
“`
Converting a String to a Byte Array using Encoding.Unicode.GetBytes()
Encoding.Unicode.GetBytes() method is used to convert a string to a byte array using the UTF-16 encoding standard. UTF-16 is a variable-width encoding scheme, similar to UTF-8, but it can handle characters outside the Basic Multilingual Plane (BMP) more efficiently. This encoding scheme is commonly used in Windows-based systems.
Example usage:
“`
string str = “こんにちは世界”;
byte[] byteArray = Encoding.Unicode.GetBytes(str);
“`
Converting a String to a Byte Array with Custom Encodings in C#
In addition to the built-in encoding schemes, you can also use custom encodings to convert a string to a byte array in C#. Custom encodings are useful when you want to work with specific character sets or when you need to comply with legacy systems.
Example usage:
“`
string str = “Custom Encoding”;
Encoding customEncoding = Encoding.GetEncoding(“ISO-8859-1”);
byte[] byteArray = customEncoding.GetBytes(str);
“`
Handling Exceptions and Errors during String to Byte Array Conversion
During the conversion process, certain situations can arise that may result in exceptions or errors. It is essential to handle these cases gracefully to avoid program crashes or undesired behavior. Common exceptions that can occur during string to byte array conversion include ArgumentException (when the provided string is null), EncoderFallbackException (when an error occurs during encoding), and ArgumentNullException (when the encoding is null).
To handle these exceptions, it is recommended to use try-catch blocks and handle each exception accordingly. You can also use Encoding.GetBytes() method overload that provides error handling options.
Best Practices and Considerations for Converting Strings to Byte Arrays in C#
1. Specify the correct encoding: Ensure that you choose the appropriate encoding based on the characters present in your string and the requirements of your application.
2. Handle exceptions: Always handle exceptions carefully and ensure you have proper error handling mechanisms in place.
3. Validate input strings: Validate the input string before converting it to a byte array to prevent errors due to invalid characters or null strings.
4. Use appropriate encoding standards: Be aware of the differences between ASCII, UTF-8, and UTF-16 encoding standards and choose the one that suits your application’s needs.
5. Consider performance implications: Converting strings to byte arrays can be resource-intensive, so it is important to consider performance implications and optimize your code if necessary.
6. Test with different inputs: Test your code with different input strings to ensure reliable and accurate conversions.
FAQs
Q1. Can I convert a hexadecimal string to a byte array in C#?
Yes, you can convert a hexadecimal string to a byte array in C#. One way to achieve this is by using the `BitConverter` class. Here’s an example:
“`
string hexString = “48656C6C6F20576F726C64”;
byte[] byteArray = Enumerable.Range(0, hexString.Length / 2)
.Select(x => Convert.ToByte(hexString.Substring(x * 2, 2), 16))
.ToArray();
“`
Q2. Is there an online tool to convert a string to a byte array?
Yes, there are several online tools available that can convert a string to a byte array. These tools typically provide a user interface where you can input your string, choose the desired encoding, and obtain the resulting byte array.
Q3. How can I convert a string to a 32-bit byte array in C#?
To convert a string to a 32-bit byte array in C#, you can use the `BitConverter.GetBytes()` method. Here’s an example:
“`
string str = “Hello”;
byte[] byteArray = BitConverter.GetBytes(str.GetHashCode());
“`
In conclusion, converting a string to a byte array is a common task in C# programming, especially when working with binary data or transferring data over a network. By understanding the different methods available and following best practices, you can ensure efficient and accurate conversions while handling exceptions and considering performance implications. So, choose the encoding standard wisely, validate inputs, and test your code thoroughly to achieve reliable and secure string to byte array conversions in your C# applications.
C# Programmers Faq – 008 String To Byte Array Ascii #Programming #Developer #Csharp #Dotnet #Shorts
How To Convert String To Byte Array In C?
When working with data in C programming language, it is common to find situations where you need to convert a string to a byte array. This conversion is necessary for various purposes, such as encryption, network communication, or storing data in a byte-oriented format. In this article, we will explore the process of converting a string to a byte array in C, providing step-by-step guidance and addressing common concerns along the way.
To begin, let’s first understand what a byte array and a string mean in the context of C programming. A string is a sequence of characters terminated by a null character (‘\0’). On the other hand, a byte array is an ordered collection of bytes, often used to represent binary data. Therefore, converting a string to a byte array implies transforming the characters of the string into their underlying byte representation.
1. Using the strlcpy() function:
One approach to convert a string to a byte array is by using the strlcpy() function. This function is part of the string.h library and copies a string into a character array while ensuring null termination. To use this function, follow these steps:
a. Declare a character array that will hold the byte array.
“`c
char byteArray[MAX_SIZE];
“`
b. Initialize the string that you wish to convert.
“`c
char* myString = “Hello, World!”;
“`
c. Call the strlcpy() function to copy the string into the byteArray.
“`c
strlcpy(byteArray, myString, sizeof(byteArray));
“`
Now, the byteArray variable contains the byte representation of the string.
2. Using a loop:
Another method to convert a string to a byte array is by iterating over the characters of the string and storing their byte representation in a byte array. This process can be accomplished using the following steps:
a. Allocate memory for the byte array.
“`c
unsigned char* byteArray = (unsigned char*)malloc(strlen(myString));
“`
b. Iterate over each character of the string and store its byte representation in the byte array.
“`c
int i;
for (i = 0; i < strlen(myString); i++) {
byteArray[i] = (unsigned char)myString[i];
}
```
It is important to note that the byte array should be of type unsigned char to ensure proper storage of the byte values.
Now that you are familiar with two common methods of converting a string to a byte array, let's address some frequently asked questions regarding this topic:
FAQs:
Q: Can the byte array be larger than the size of the string?
A: Yes, the byte array can be larger than the size of the string. However, it is important to ensure that the byte array size is allocated correctly to avoid buffer overflows or memory allocation issues.
Q: What if the string contains non-textual or special characters?
A: When converting a string to a byte array, each character in the string will be converted to its corresponding byte value. Non-textual or special characters will be converted to their ASCII or Unicode representation, depending on the character encoding used.
Q: How can I convert a byte array back to a string?
A: To convert a byte array back to a string, you can iterate over each byte and convert it to its corresponding character representation using ASCII or Unicode values.
Q: Are there any memory-related concerns when converting the string to a byte array?
A: Yes, it is important to allocate sufficient memory for the byte array to hold all the byte representations of the string characters. Failure to allocate proper memory can cause data corruption or memory access errors.
Q: What other methods can be used to convert a string to a byte array in C?
A: Besides the strlcpy() function and using a loop, you can also explore other methods, such as explicitly casting the string to a (unsigned) char array or using libraries, such as OpenSSL, if available in your project.
In conclusion, converting a string to a byte array in C is a fundamental task in various programming scenarios. By following the steps outlined in this article, you can successfully convert a string to its byte representation. Remember to consider memory allocation, character encoding, and other specific requirements of your project while implementing the conversion process.
How To Convert A String Into Byte Array?
In programming, there are scenarios where you may need to convert a string into a byte array. This conversion is especially useful when you want to read and write binary data, encryption, or when dealing with network protocols that require byte-level communication. This article aims to provide a comprehensive guide on how to convert a string into a byte array, explaining different approaches and highlighting their advantages and drawbacks.
Methods to Convert a String into a Byte Array
There are various methods available to convert a string into a byte array, depending on the programming language you are using. Let’s explore three commonly used methods:
1. Using the Encoding Class:
Most programming languages provide an Encoding class that offers built-in methods to convert strings into byte arrays. For instance, in C#, the Encoding class allows you to call the GetBytes() method, which takes a string parameter and returns a byte array.
Example in C#:
“`csharp
string str = “Hello world!”;
Encoding encoding = Encoding.ASCII; // Select the desired encoding
byte[] byteArray = encoding.GetBytes(str); // Convert the string to a byte array
“`
2. Utilizing the getBytes() Method:
Another common approach is to use the getBytes() method provided by the standard library. This method takes the character set as an argument and returns a byte array representation of the string. However, this method may throw an exception if there are any unsupported characters in the string.
Example in Java:
“`java
String str = “Hello world!”;
byte[] byteArray = str.getBytes(); // Convert the string to a byte array
“`
3. Manual Conversion:
In some cases, you may need to manually convert each character of the string into its corresponding byte representation. This approach allows you to have more control over the conversion process. However, it requires additional coding efforts compared to the previous two methods.
Example in Python:
“`python
str = “Hello world!”
byteArray = [ord(char) for char in str] # Convert each character to its ASCII representation
“`
Frequently Asked Questions (FAQs)
Q1: Why would I need to convert a string into a byte array?
A1: Converting a string into a byte array becomes imperative in scenarios like reading and writing binary data, encryption algorithms, or while working with network protocols that require byte-level communication.
Q2: Can I convert a string into a byte array without considering the character encoding?
A2: No, character encoding is crucial for converting a string into a byte array. Different character encodings (e.g., ASCII, UTF-8) represent characters differently in bytes.
Q3: What are the advantages of using the Encoding class for string-to-byte array conversion?
A3: The Encoding class provides a convenient and standardized way to convert strings to byte arrays. It supports various character encodings, allowing you to choose the most suitable one for your needs.
Q4: Why might manual conversion be preferred over built-in methods?
A4: Manual conversion can be beneficial when you need fine-grained control over the conversion process. For example, you may want to apply custom transformations during the conversion or handle unsupported characters in a specific way.
Q5: Is there a performance difference between the different methods?
A5: Performance differences may exist between methods, but they are usually negligible unless you are dealing with very large strings or performing conversions at a very high frequency. It’s advisable to measure and evaluate the performance impact in specific cases.
Q6: How can I ensure the integrity of the converted byte array?
A6: To ensure the integrity of the converted byte array, it’s crucial to use a reliable character encoding and handle any potential exceptions or errors that may arise during the conversion process.
Conclusion
Converting a string into a byte array is a common requirement in programming, particularly when dealing with binary data, encryption, or networking. This article provided a comprehensive guide on various methods to accomplish this task. Whether you choose to use the Encoding class, the getBytes() method, or manually convert each character, understanding the different approaches and their implications will empower you to handle string-to-byte array conversion effectively in your programming endeavors.
Keywords searched by users: convert string to byte array c# Convert string to byte array c#, Hex string to byte array in C, Convert string to byte C#, convert string to byte[] c#, String to byte c, Hex string to byte array C#, Convert String to byte array online, Convert string to byte 32 c#
Categories: Top 64 Convert String To Byte Array C#
See more here: nhanvietluanvan.com
Convert String To Byte Array C#
In many programming scenarios, it is often necessary to convert string data into a byte array for various purposes. The C# programming language provides a straightforward way to accomplish this task through its robust string manipulation and byte array handling capabilities. In this article, we will discuss the process of converting a string to a byte array in C# and explore different scenarios where this conversion is commonly used. Additionally, we will address some frequently asked questions regarding this topic.
Converting a string to a byte array in C# can be accomplished using the Encoding class, which is part of the System.Text namespace. The Encoding class provides a collection of static methods that make it easy to encode and decode strings in different character encoding formats, including UTF-8, UTF-16, and ASCII. To convert a string to a byte array, the GetBytes method of the Encoding class can be used, which returns an array of bytes representing the characters in the input string.
Here is an example of converting a string to a byte array using UTF-8 encoding:
“`csharp
string inputString = “Hello, world!”;
byte[] byteArray = Encoding.UTF8.GetBytes(inputString);
“`
In the above example, the string “Hello, world!” is converted to a byte array using the UTF-8 encoding. The resulting byteArray variable will contain the byte representation of each character in the string.
It is important to note that the encoding used for the conversion should be consistent with the expected encoding format at the destination. For instance, if you are sending the byte array over a network or storing it in a file, you should consider the encoding format used by the target system to properly interpret the data.
Besides the aforementioned method, there are other approaches to converting a string to a byte array in C#. For instance, the BitConverter class provides a convenient way to convert numeric data types to and from byte arrays. By leveraging the GetBytes method of the BitConverter class, you can easily convert a string representation of a numeric value to a byte array. However, this approach is limited to numeric conversions and may not serve the purpose for arbitrary strings.
Frequently Asked Questions:
Q: What happens if the string contains non-ASCII characters?
A: The byte array generated from a non-ASCII string will depend on the encoding used. For instance, if UTF-8 encoding is used, characters outside the ASCII range will be encoded using multiple bytes. It is crucial to ensure the receiver understands the encoding format to correctly interpret the byte array.
Q: Can I convert the byte array back to a string?
A: Yes, you can convert a byte array back to a string using the GetString method of the Encoding class. The GetString method decodes a sequence of bytes in a specific encoding and returns the corresponding string representation.
Q: How can I determine the size of the resulting byte array?
A: The size of the byte array can be obtained using the Length property of the byte array. For example, `byteArray.Length` will return the size of the resulting byte array.
Q: Can I convert a string to a byte array using a specific encoding other than UTF-8?
A: Yes, you can replace `Encoding.UTF8.GetBytes` with the appropriate encoding provided by the Encoding class. For example, to use UTF-16 encoding, use `Encoding.Unicode.GetBytes`.
Q: Are there any performance considerations when converting large strings to byte arrays?
A: Converting large strings to byte arrays may consume significant memory resources, especially with encoding formats that result in a larger byte representation. It is important to evaluate the memory requirements and optimize the process accordingly.
In conclusion, converting a string to a byte array in C# is a common task with various use cases. Through the Encoding class and its GetBytes method, developers can easily convert strings to byte arrays in different encoding formats. It is important to ensure consistent encoding usage between the conversion and interpretation of the byte array to avoid any misinterpretation of the data. Remember to consider the encoding requirements of the destination system or medium where the byte array will be used. By understanding the conversion process and its nuances, developers can efficiently manipulate string and byte array data in their C# applications.
Hex String To Byte Array In C
In computer programming, a hexadecimal (hex) string is a representation of numerical values using the base-16 numbering system. A byte array, on the other hand, is a collection of consecutive bytes stored in memory. Converting a hex string to a byte array is a common task when working with binary data or performing cryptographic operations. In this article, we will explore various methods and techniques to convert a hex string to a byte array in the C programming language.
I. Converting Hex String to Byte Array
1. Method 1: Direct Conversion
One of the simplest approaches is to directly convert each pair of hex characters to their corresponding byte value. For instance, the hex string “A3B7” would be converted into the byte array {0xA3, 0xB7}. This method is straightforward but requires careful handling of the input string to ensure it contains valid hexadecimal digits.
2. Method 2: Standard Library Functions
C provides standard library functions to convert strings to integers. The `strtol` function can be used to convert individual hex substrings to their integer values, which can then be stored in a byte array. This method allows for error handling by indicating invalid substrings within the hex string.
3. Method 3: Bitwise Operations
In C, bitwise operations can be leveraged to extract the individual byte values from the hex string. By shifting and masking the hexadecimal characters, we can isolate the lower and upper nibbles (4-bit segments) and combine them to obtain the corresponding byte values. This method requires a deeper understanding of binary operations but provides efficient conversion.
II. Implementation Example
Let’s consider an implementation example using Method 2, which utilizes the standard library functions `strtol` and `sscanf`. The code snippet below demonstrates how to convert a hex string to a byte array:
“`c
#include
#include
#define MAX_HEX_STRING_SIZE 100
size_t hexStringToByteArray(const char* hexString, unsigned char* byteArray) {
size_t len = strlen(hexString);
size_t byteLen = len / 2;
for (size_t i = 0; i < byteLen; i++) { sscanf(hexString + 2 * i, "%2hhx", &byteArray[i]); } return byteLen; } int main() { const char* hexString = "A3B7D12F"; unsigned char byteArray[MAX_HEX_STRING_SIZE] = {0}; // Ensure sufficient size size_t byteLen = hexStringToByteArray(hexString, byteArray); printf("Converted Byte Array: "); for (size_t i = 0; i < byteLen; i++) { printf("%02x ", byteArray[i]); } printf("\n"); return 0; } ``` The `hexStringToByteArray` function takes a hex string and a byte array as parameters. It iterates through the hex string, converting each pair of hex characters to a byte value using `sscanf`. The resulting byte array is then returned along with the length of the byte array. The main function demonstrates the conversion by printing the resulting byte array. III. FAQs Q1. Can the method handle different byte orders (endianess)? A1. The byte order is not directly addressed in the conversion process. It relies on the platform's byte order. If byte order manipulation is required, bitwise operations or platform-specific functions must be used. Q2. How can I handle invalid hex strings or non-hex characters? A2. The methods mentioned above do not inherently handle invalid hex strings. Custom error handling can be implemented by validating the input string or checking for invalid substrings during conversion. Q3. Are there any limitations on the size of the hex string or resulting byte array? A3. The size of the hex string and byte array depends on various factors, such as memory availability and system limitations. Ensure sufficient memory is allocated to prevent buffer overflows. Q4. Can the conversion process be reversed (byte array to hex string)? A4. Yes, the reverse conversion is possible by converting each byte value back to a hex string. This can be achieved using standard library functions or by implementing custom algorithms. IV. Conclusion Converting a hex string to a byte array is a fundamental operation in various programming scenarios, such as dealing with binary data or cryptographic processing. This article explored different methods to accomplish this task in the C programming language. Whether you choose the direct conversion, standard library functions, or bitwise operations, each approach has its advantages and considerations. By understanding these techniques, you can confidently handle hex string to byte array conversions in your C programs.
Convert String To Byte C#
There are multiple ways to convert a string to a byte array in C#. We will discuss the most commonly used approaches and their advantages and limitations.
Method 1: Encoding.GetBytes()
The Encoding class in C# provides the GetBytes() method, which converts a string into a byte array using a specified encoding. Here’s an example of how to use it:
“`csharp
string str = “Hello World!”;
byte[] byteArray = Encoding.UTF8.GetBytes(str);
“`
In this method, we use the UTF8 encoding to convert the string. UTF8 is a widely used encoding that supports a wide range of characters. Other available encodings include ASCII, Unicode, and more.
Advantages:
– Supports various encodings.
– Straightforward and simple to use.
Limitations:
– May produce different byte arrays for the same string, depending on the encoding used.
– The resulting byte array may contain additional bytes for encoding specific information.
Method 2: BitConverter.GetBytes()
Another method to convert a string to a byte array is by using the BitConverter class. This method treats each character of the string as a Unicode character and converts it into a byte array. Here’s an example:
“`csharp
string str = “Hello World!”;
byte[] byteArray = BitConverter.GetBytes(str);
“`
Advantages:
– Easy to use and understand.
– Suitable for simple string to byte array conversions.
Limitations:
– Works only for ASCII or Unicode characters.
– Does not support encoding-specific conversions.
Method 3: ASCII.GetBytes()
If you are working with ASCII characters only, you can use the ASCII encoding’s GetBytes() method. This method converts each character of the string to its ASCII representation. Here’s an example:
“`csharp
string str = “Hello World!”;
byte[] byteArray = Encoding.ASCII.GetBytes(str);
“`
Advantages:
– Suitable for ASCII characters.
– Simple and efficient.
Limitations:
– Does not support non-ASCII characters.
– Limited to a specific character set.
Method 4: Stream-based Conversion
If you are working with large files or streams, using the Stream class and its derived classes can be more efficient. The MemoryStream class allows you to read and write a byte array to and from a stream. Here’s an example:
“`csharp
string str = “Hello World!”;
byte[] byteArray;
using (MemoryStream stream = new MemoryStream())
{
using (StreamWriter writer = new StreamWriter(stream))
{
writer.Write(str);
writer.Flush();
byteArray = stream.ToArray();
}
}
“`
In this method, we create a MemoryStream and a StreamWriter to write the string to the stream. Finally, we convert the stream contents to a byte array using the ToArray() method.
Advantages:
– Suitable for large files or streams.
– Can handle encoding-specific conversions.
Limitations:
– Requires additional memory for the MemoryStream.
– Relatively complex compared to other methods.
FAQs:
Q1: Can I convert a byte array back to a string?
A1: Yes, you can convert a byte array back to a string using the appropriate encoding. For example, to convert a UTF8 encoded byte array back to a string, you can use Encoding.UTF8.GetString(byteArray).
Q2: How can I convert a string representation of a number to a byte array?
A2: To convert a string representation of a number to a byte array, you can use the appropriate BitConverter class method based on the number type. For example, BitConverter.GetBytes(int.Parse(str)) for converting a string representation of an integer.
Q3: Can I use these methods to convert a string to a byte array in other programming languages?
A3: The methods mentioned in this article are specific to the C# programming language. However, most programming languages provide similar functionality to convert strings to byte arrays, although the implementation details may vary.
In conclusion, converting a string to a byte array in C# can be done using various methods and techniques. Depending on your requirements and the nature of the string data, you can choose the appropriate method. Understanding the advantages and limitations of each method will help you select the most suitable approach for your specific scenario.
Images related to the topic convert string to byte array c#
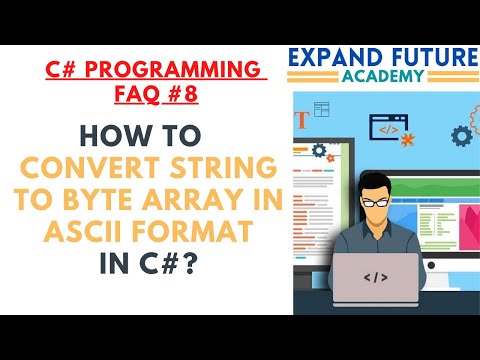
Article link: convert string to byte array c#.
Learn more about the topic convert string to byte array c#.
- Convert ASCII string (char[]) to BYTE array in C
- Converting string to byte array in C# – Stack Overflow
- How to Convert String To Byte Array in C# – C# Corner
- How to convert a string to a byte array in C# – Dofactory
- Convert string to byte array in C++ – Techie Delight
- String to byte array, byte array to String in Java | DigitalOcean
- Convert a String to Integer Array in C/C++ – GeeksforGeeks
- How to convert strings to bytes in Python – Educative.io
- HELP PLEASE – How to convert string to byte array – C / C++
- How to convert a string into bytes in C – Quora
- Convert String to Byte Array and Reverse in Java | Baeldung
- Convert char * String to System::Byte Array – Microsoft Learn
- String to byte array, byte array to String in Java | DigitalOcean
- Converting strings to bytes and vice versa – CryptoSys
See more: https://nhanvietluanvan.com/luat-hoc/