Check String Empty Javascript
In JavaScript, an empty string refers to a string that does not contain any characters. It is represented by a pair of double quotes (“”) or single quotes (”). Understanding how to check for an empty string in JavaScript is essential for efficient coding and error handling.
Checking for an Empty String using the Length Property
One common way to check for an empty string in JavaScript is by utilizing the length property. The length property returns the number of characters in a string. When the length of a string is 0, it means the string is empty.
Here’s an example:
“`
let str = “”;
if (str.length === 0) {
console.log(“The string is empty”);
} else {
console.log(“The string is not empty”);
}
“`
Using the Comparison Operator to Check if a String is Empty
The comparison operator can also be used to check if a string is empty. By comparing the string to an empty string, we can determine if it is empty or not.
“`
let str = “”;
if (str === “”) {
console.log(“The string is empty”);
} else {
console.log(“The string is not empty”);
}
“`
Employing the trim() Method to Check for an Empty String
The trim() method in JavaScript removes leading and trailing whitespace from a string. By using the trim() method, we can check if a string is empty or only contains whitespace characters.
“`
let str = ” “;
if (str.trim() === “”) {
console.log(“The string is empty or contains only whitespace”);
} else {
console.log(“The string is not empty”);
}
“`
Checking for an Empty String using Regular Expressions
Regular expressions provide a more advanced way to check for an empty string in JavaScript. By using a regular expression pattern that matches an empty string, we can determine if a string is empty.
“`
let str = “”;
if (/^\s*$/.test(str)) {
console.log(“The string is empty”);
} else {
console.log(“The string is not empty”);
}
“`
Utilizing the isEmpty() Function to Check for an Empty String
In some cases, it can be useful to create a reusable function to check for an empty string. We can define a custom isEmpty() function that checks if a string is empty.
“`
function isEmpty(str) {
return str === “”;
}
let myString = “”;
if (isEmpty(myString)) {
console.log(“The string is empty”);
} else {
console.log(“The string is not empty”);
}
“`
Distinguishing between an Empty String and a String with Whitespace
It is important to note the difference between an empty string and a string that contains only whitespace. An empty string has zero characters, while a string with whitespace has one or more whitespace characters.
To distinguish between the two, it is recommended to utilize the trim() method to remove any leading and trailing whitespace before performing the empty string check.
Checking for an Empty String in Different JavaScript Data Types
In addition to checking for an empty string in a regular string variable, it may be necessary to check for an empty string in different JavaScript data types, such as objects or arrays.
For objects, we can check if the object’s keys length is 0:
“`
let obj = {};
if (Object.keys(obj).length === 0) {
console.log(“The object is empty”);
} else {
console.log(“The object is not empty”);
}
“`
For arrays, we can check if the array’s length is 0:
“`
let arr = [];
if (arr.length === 0) {
console.log(“The array is empty”);
} else {
console.log(“The array is not empty”);
}
“`
Dealing with Edge Cases and Considering Null or Undefined Values
When handling empty strings in JavaScript, it is essential to consider edge cases such as null or undefined values. These values are different from empty strings and need to be handled separately.
To check for null or undefined values, we can use the following approaches:
“`
let value = null;
if (value === null || value === undefined) {
console.log(“The value is null or undefined”);
} else if (value === “”) {
console.log(“The value is an empty string”);
} else {
console.log(“The value is not null, undefined, or an empty string”);
}
“`
Best Practices for Handling Empty Strings in JavaScript
When working with empty strings in JavaScript, it is recommended to use a combination of techniques to cover different scenarios. Here are some best practices:
1. Always use the trim() method to remove leading and trailing whitespace when checking for an empty string.
2. Utilize the length property or the comparison operator to check for an empty string in regular strings.
3. When dealing with objects, use the Object.keys() method to check if the object is empty.
4. For arrays, check the length property to determine if the array is empty.
5. Consider edge cases such as null or undefined values and handle them separately.
6. Create reusable functions, like isEmpty(), to simplify the code and improve readability.
By employing these best practices, you can ensure accurate and effective handling of empty strings in your JavaScript code.
FAQs
Q: How do I check if a string is empty in Java?
A: To check for an empty string in Java, you can use the isEmpty() method of the String class. Here’s an example:
“`
String myString = “”;
if (myString.isEmpty()) {
System.out.println(“The string is empty”);
} else {
System.out.println(“The string is not empty”);
}
“`
Q: How can I check if a string is empty in JavaScript?
A: In JavaScript, there are multiple ways to check if a string is empty. Some of the common methods include using the length property, the comparison operator, the trim() method, or regular expressions. Choose the method that suits your requirements and coding style.
Q: How do I check if a JavaScript object is empty?
A: You can use the Object.keys() method to check if a JavaScript object is empty. If the length of the array returned by Object.keys() is 0, it means the object is empty. Here’s an example:
“`
let myObject = {};
if (Object.keys(myObject).length === 0) {
console.log(“The object is empty”);
} else {
console.log(“The object is not empty”);
}
“`
Q: What is the isEmpty() function in JavaScript?
A: The isEmpty() function is not a built-in JavaScript function. It is a custom function that can be created to check if a particular value, such as a string, is empty. The implementation can vary depending on the specific requirements of your code.
Q: How do I check if an array is empty in JavaScript?
A: To check if an array is empty in JavaScript, you can use the length property of the array. If the length is 0, it means the array is empty. Here’s an example:
“`
let myArray = [];
if (myArray.length === 0) {
console.log(“The array is empty”);
} else {
console.log(“The array is not empty”);
}
“`
Q: How do I check if an object is empty in JavaScript ES6?
A: In JavaScript ES6, you can use the Object.keys() method to check if an object is empty. If the length of the array returned by Object.keys() is 0, it means the object is empty. Here’s an example:
“`
let myObject = {};
if (Object.keys(myObject).length === 0) {
console.log(“The object is empty”);
} else {
console.log(“The object is not empty”);
}
“`
Q: How do I check if a string is empty or whitespace in JavaScript?
A: To check if a string is empty or consists only of whitespace characters in JavaScript, you can use the trim() method in combination with the length property. Here’s an example:
“`
let myString = ” “;
if (myString.trim().length === 0) {
console.log(“The string is empty or contains only whitespace”);
} else {
console.log(“The string is not empty”);
}
“`
How To Check For Empty String In Javascript (Best Way)
Keywords searched by users: check string empty javascript Check empty string Java, Check empty js, JavaScript check string empty or whitespace, Check empty object JavaScript, Check empty array js, jQuery check empty string, Check if object is empty JavaScript ES6, isEmpty JS
Categories: Top 70 Check String Empty Javascript
See more here: nhanvietluanvan.com
Check Empty String Java
—————————————————–
Introduction:
————–
In Java programming, checking whether a string is empty or not is a common and essential task. An empty string is defined as a string that contains no characters or only contains whitespace characters. In this article, we will explore various methods to check for an empty string in Java and provide thorough explanations with examples. So, let’s dive in!
Methods to Check for an Empty String:
————————————–
Here are some popular methods in Java that can be used to check for an empty string:
1. Using the length() Method:
The length() method returns the number of characters present in a string. To check if a string is empty, you can simply compare its length with zero. If the length is zero, it means the string is empty. Here’s an example:
“`java
String str = “”; // Empty string
if (str.length() == 0) {
System.out.println(“The string is empty”);
}
“`
Output: The string is empty
2. Using the isEmpty() Method:
The isEmpty() method is a convenient way to check if a string is empty or not. It returns true if the length of the string is zero; otherwise, it returns false. Here’s an example:
“`java
String str = “”; // Empty string
if (str.isEmpty()) {
System.out.println(“The string is empty”);
}
“`
Output: The string is empty
3. Using the trim() Method:
The trim() method is used to remove leading and trailing whitespace from a string. By combining trim() with isEmpty() or length(), you can check for an empty string while ignoring whitespace characters. Here’s an example:
“`java
String str = ” “; // String containing only whitespace characters
if (str.trim().isEmpty()) {
System.out.println(“The string is empty”);
}
“`
Output: The string is empty
Frequently Asked Questions (FAQs):
———————————–
Q: What is the difference between length() and isEmpty() methods?
A: The length() method returns the number of characters in a string, whereas the isEmpty() method checks if the length is zero without explicitly accessing it. In terms of checking for an empty string, both methods can be used interchangeably.
Q: Does isEmpty() method only check for whitespace characters?
A: No, the isEmpty() method checks if the length of the string is zero. It considers any string without characters, including whitespace characters, as empty.
Q: Why do we need to use the trim() method in conjunction with isEmpty() or length() methods?
A: The trim() method eliminates any leading or trailing whitespace from a string. By using it, you can accurately determine if a string is empty, even if it contains only whitespace characters.
Q: Can we use the == operator to check for an empty string?
A: Technically, yes, you can use the == operator to check if a string is empty by comparing it to an empty string literal (“”). However, it is not recommended because the equals() method is more robust and considered best practice for string comparison in Java.
Q: Is it possible to have a null string instead of an empty string?
A: Yes, in Java, a string can have a null value, which means it does not point to any memory location. To avoid null pointer exceptions, it is essential to check for null values before performing any operations on a string.
Conclusion:
————–
Checking for an empty string is a crucial task in Java programming. In this article, we explored various methods like length(), isEmpty(), and trim() that can be utilized to determine if a string is empty or not. Additionally, we provided detailed explanations and examples to help you understand the concepts better. By applying these techniques, you can effectively handle empty strings in your Java programs.
Check Empty Js
When it comes to JavaScript programming, one of the most important aspects is data validation. Ensuring that variables are not empty or null is crucial in order to prevent silent errors that can easily creep into your code. In this article, we will dive deep into the concept of checking empty JS, exploring various techniques and best practices to ensure data validity.
Understanding the Importance of Data Validation
Data validation is the process of ensuring that the data being used in a program is correct, feasible, and useful. It helps in maintaining data integrity and preventing issues caused by invalid or empty data. JavaScript, being a loosely typed language, requires extra caution when it comes to variable assignment and usage. A small oversight in handling empty data can lead to significant issues such as unexpected behavior, crashes, or even security vulnerabilities.
The Need to Check Empty JS
When working with JavaScript, there are numerous scenarios where you need to check if a variable is empty or null before using it. For instance, when receiving user input, it is crucial to ensure that the input fields are not left blank. Moreover, when fetching data from APIs or databases, it is necessary to validate the received data before processing it further. By checking empty JS, you can prevent potential issues and provide better user experiences within your applications.
Techniques to Check Empty JS
Several techniques can be employed to check empty JS, each with its own advantages and use cases. Here are some commonly used approaches:
1. Using the typeof Operator:
The typeof operator in JavaScript returns the type of a variable. By comparing the typeof value of a variable with “undefined” or “null”, you can check if it is empty or null. For example:
“`javascript
if (typeof variable === “undefined” || variable === null) {
// handle empty or null variable
}
“`
2. Using the length Property:
JavaScript provides the length property for arrays and strings. By checking if the length of a variable is zero, you can determine if it is empty. This works well for strings and arrays that should have at least one element. For example:
“`javascript
if (str.length === 0) {
// handle empty string
}
if (arr.length === 0) {
// handle empty array
}
“`
3. Using the isEmpty Function:
Frameworks like lodash or Underscore.js provide an `isEmpty` function that checks whether a value is empty. This function covers various data types including strings, arrays, objects, and even JavaScript Map or Set. Example:
“`javascript
if (_.isEmpty(object)) {
// handle empty object
}
“`
4. Regular Expression Testing:
Regular expressions can also be utilized to check if a variable contains only whitespace or special characters. This approach is especially useful when you want to validate user input. Example:
“`javascript
if (/^\s*$/.test(str)) {
// handle empty or whitespace string
}
“`
Best Practices for Checking Empty JS
While checking empty JS, it’s important to follow some best practices to ensure optimal performance and maintainable code:
1. Use descriptive variable names: By using meaningful variable names, you can easily understand the purpose of the variable and improve code readability.
2. Handle potential errors: Instead of relying solely on checking for empty or null values, it is good practice to implement error handling mechanisms. This way, issues can be properly identified and handled.
3. Consider type coercion: JavaScript implicitly performs type coercion, which can sometimes lead to unexpected results. To avoid such scenarios, use strict equality (===) when comparing variables.
4. Utilize unit tests: To ensure the correctness and effectiveness of your empty JS checks, write unit tests that cover various use cases. This will help identify any potential flaws or edge cases.
FAQs
Q: How can I handle empty form submissions?
A: To handle empty form submissions, you can add client-side validation using JavaScript before sending the data to the server. Check if the required fields have been filled out and display an error message if they are empty.
Q: What should I do if I encounter empty or null variables in my code?
A: Depending on the context, you can choose to display default values, prompt the user for input, or handle the error gracefully by showing appropriate error messages.
Q: Are there any performance differences between the different techniques for checking empty JS?
A: The performance differences between the techniques for checking empty JS are negligible. However, using the most appropriate technique for a specific use case can lead to cleaner and more readable code.
In conclusion, checking empty JS is an essential practice to ensure data validity and prevent silent errors in JavaScript programming. By employing the right techniques and adhering to best practices, you can greatly improve the reliability and usability of your applications. Remember to handle empty variables appropriately, perform thorough testing, and continuously update your code to address potential issues.
Images related to the topic check string empty javascript
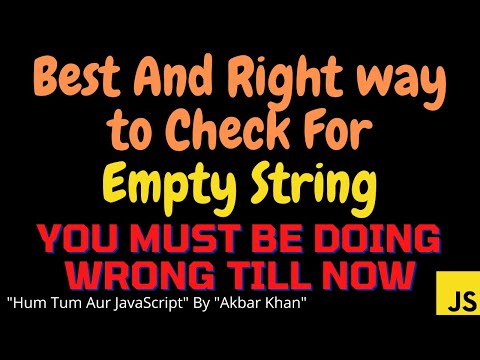
Found 14 images related to check string empty javascript theme
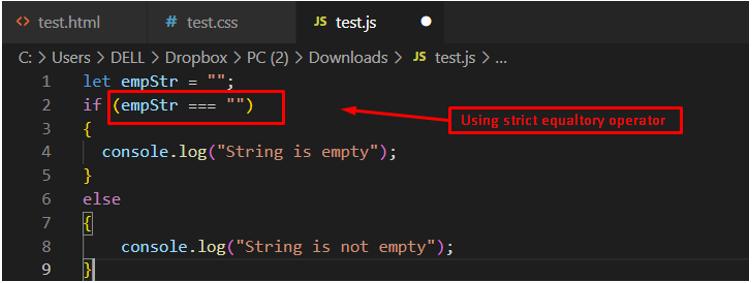

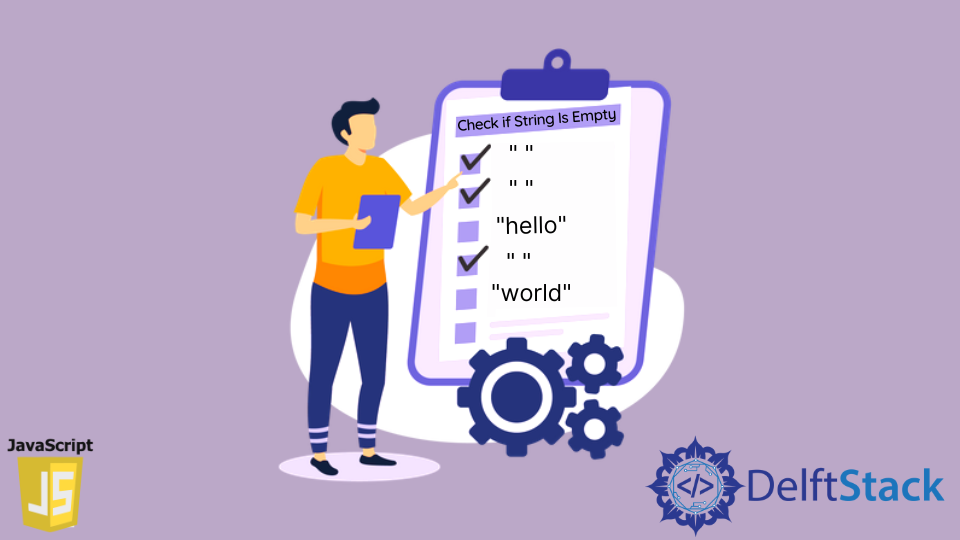
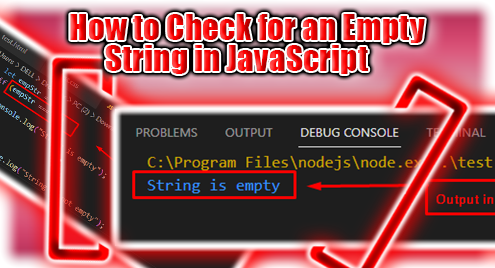
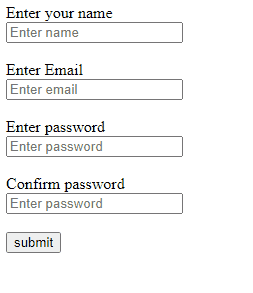
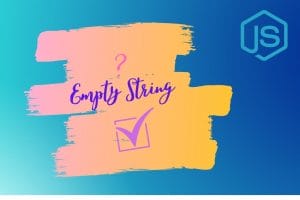


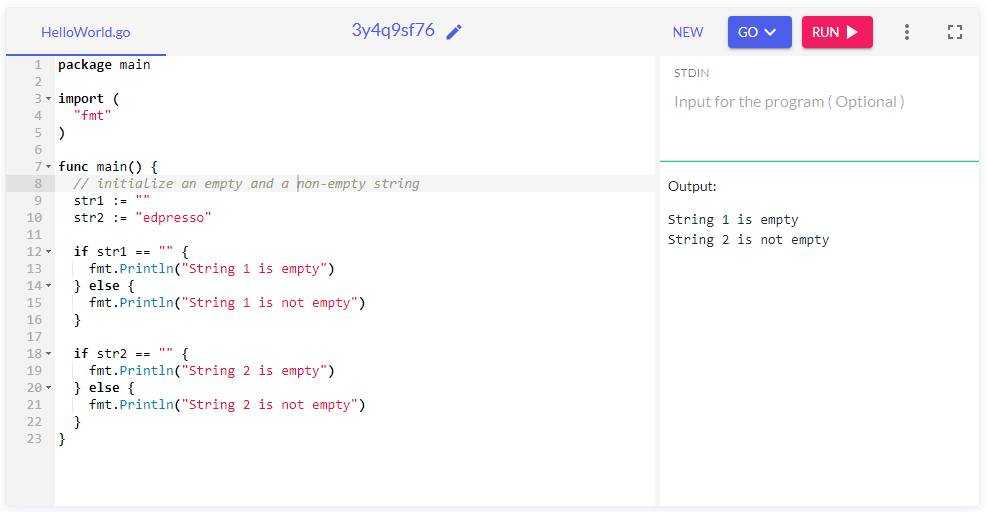

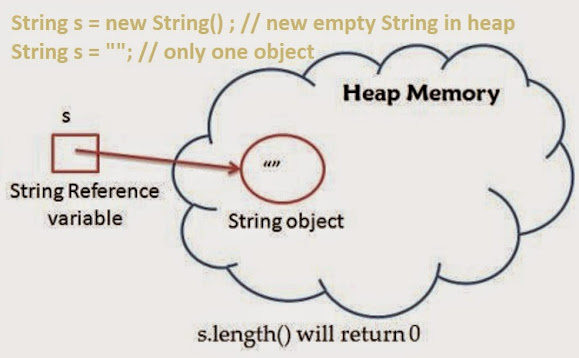
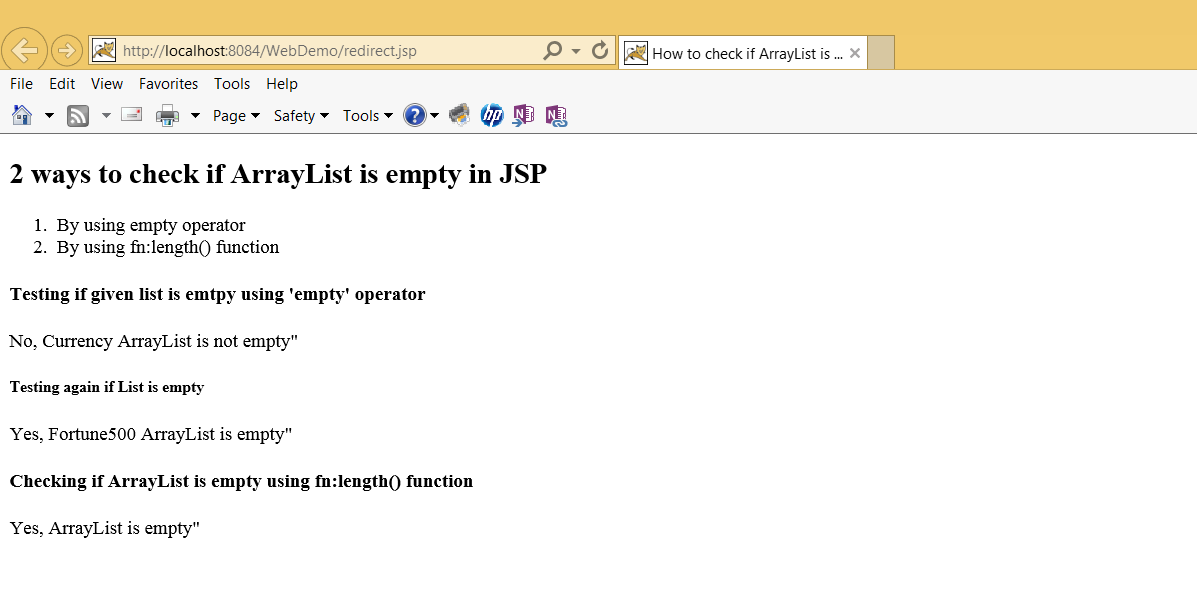
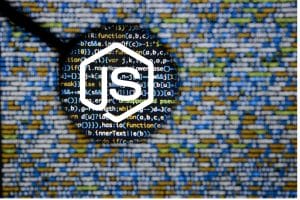


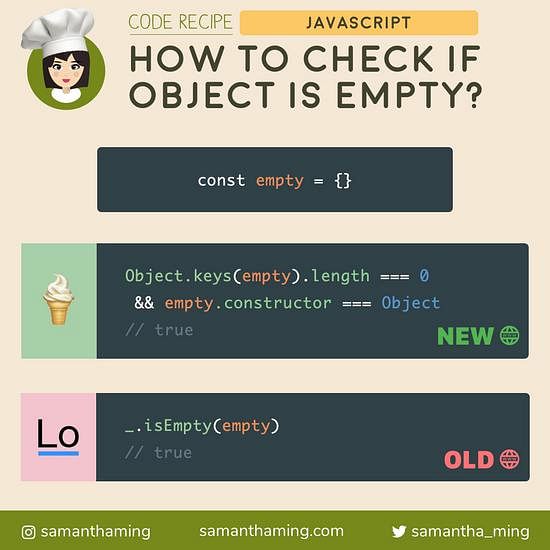

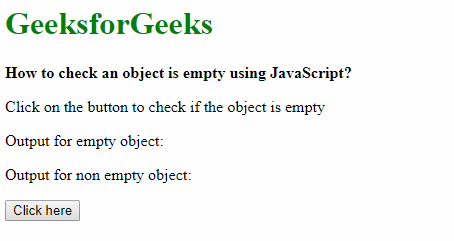


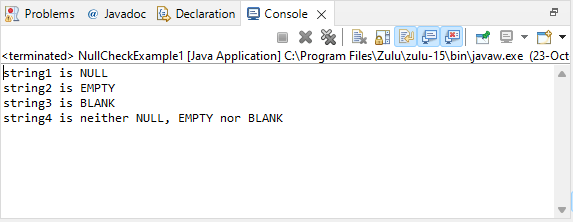
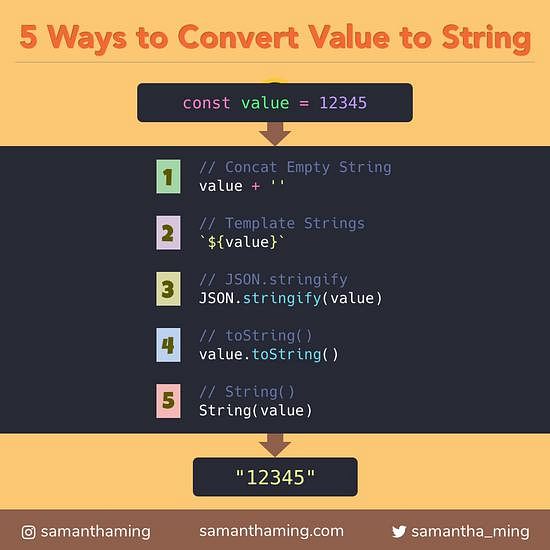

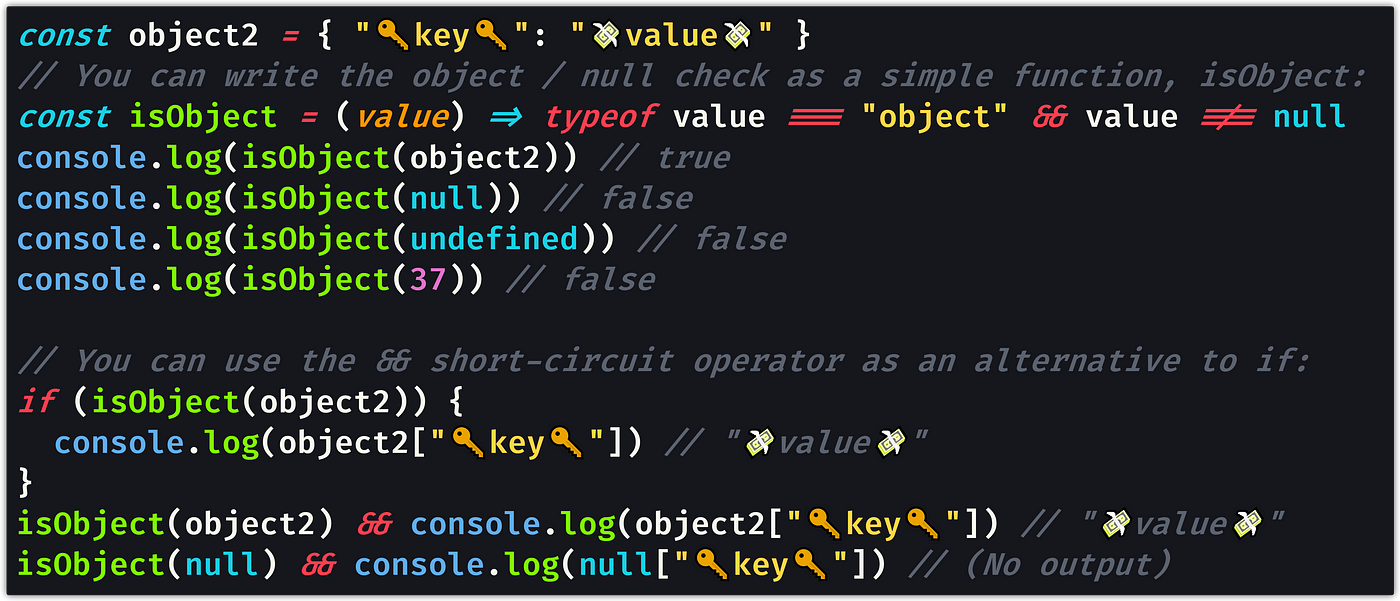
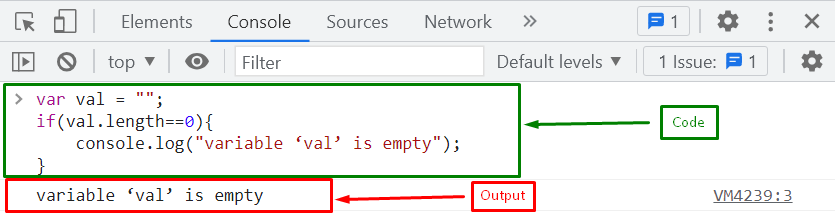

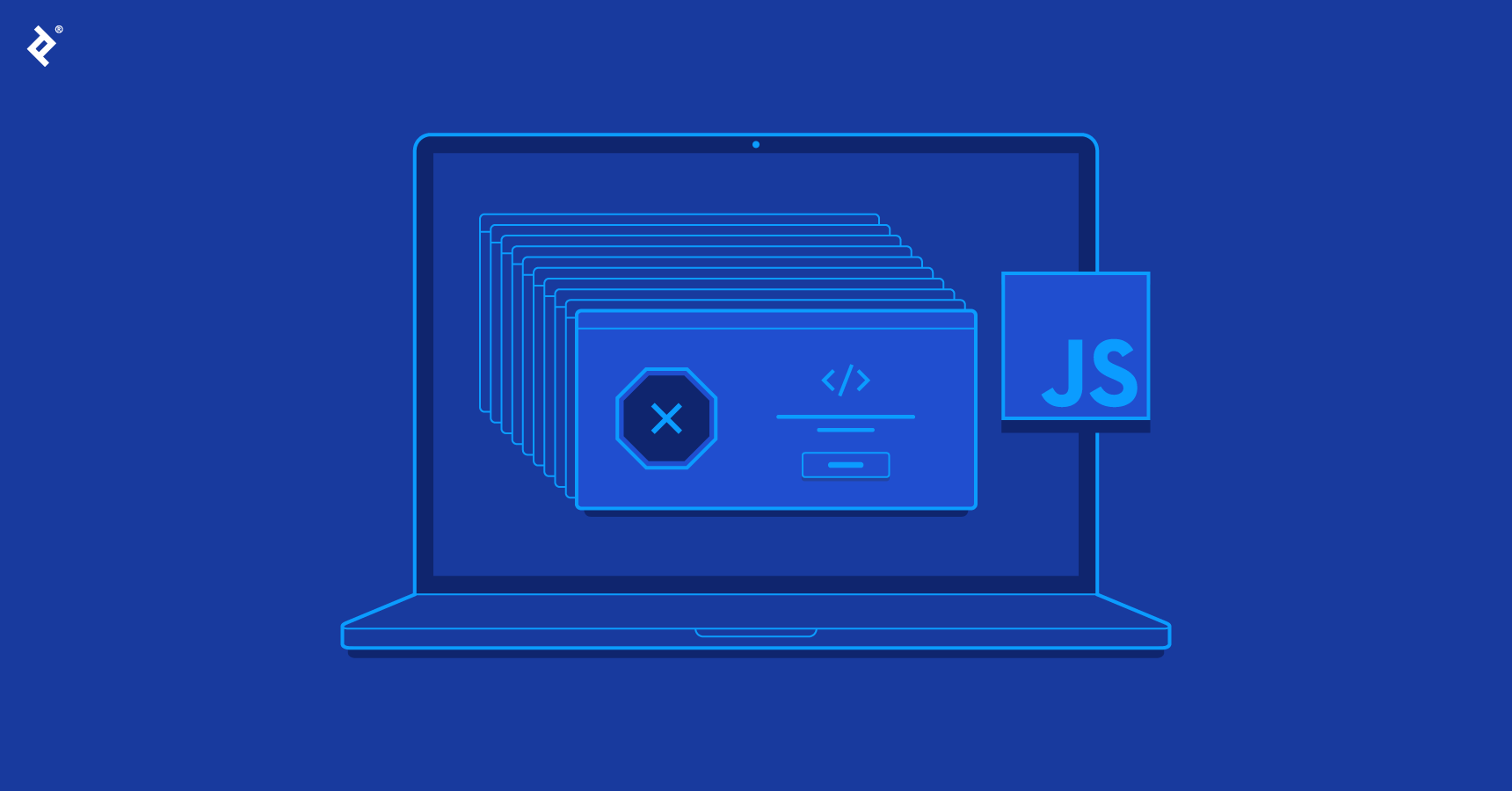
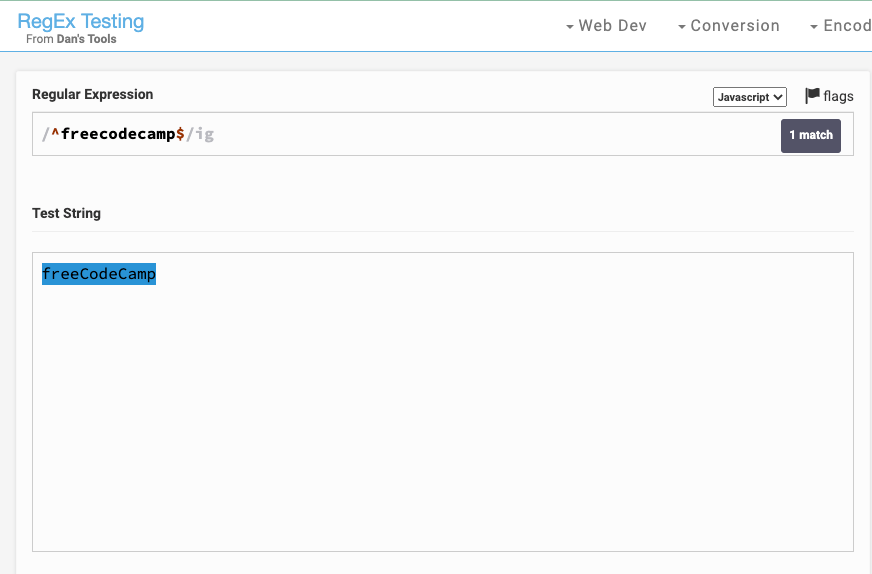
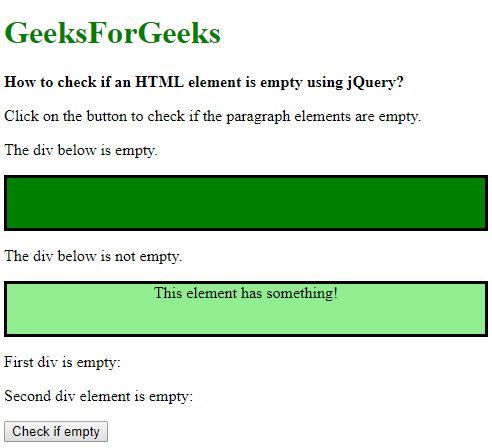

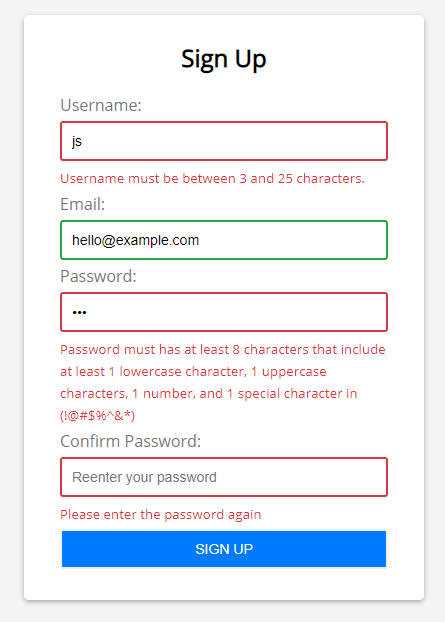
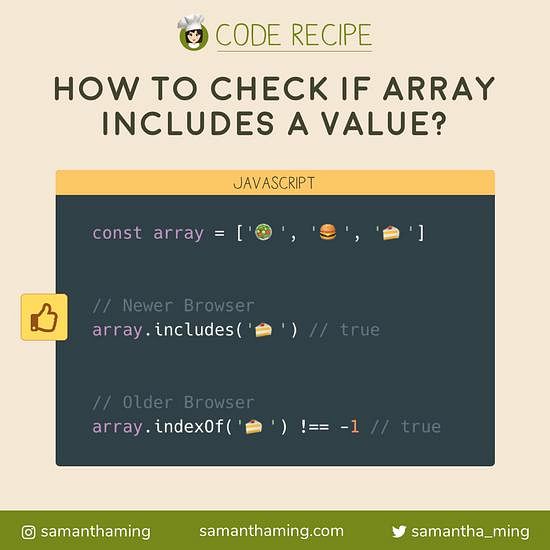

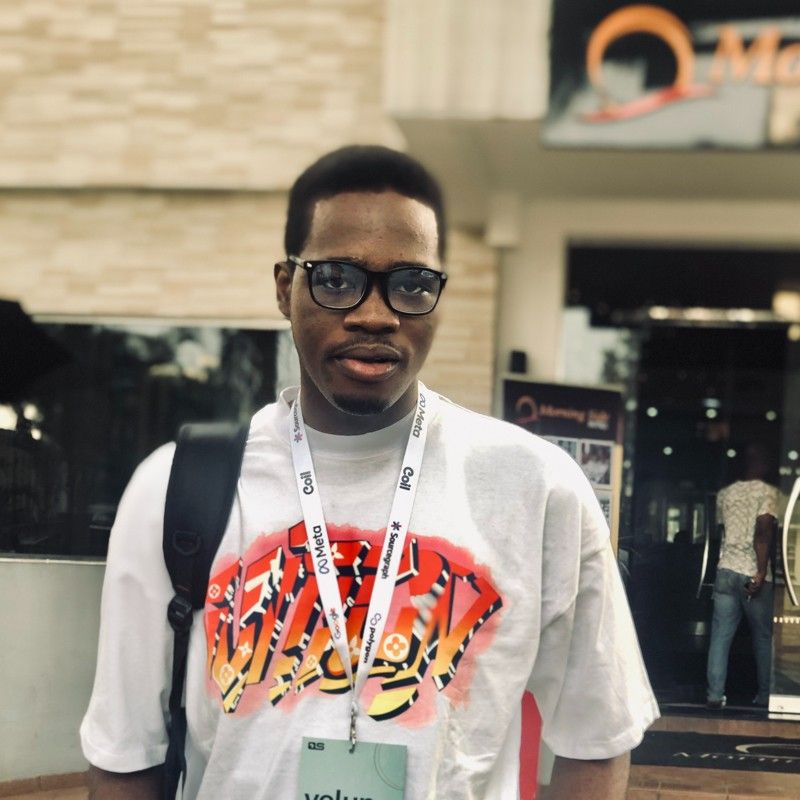
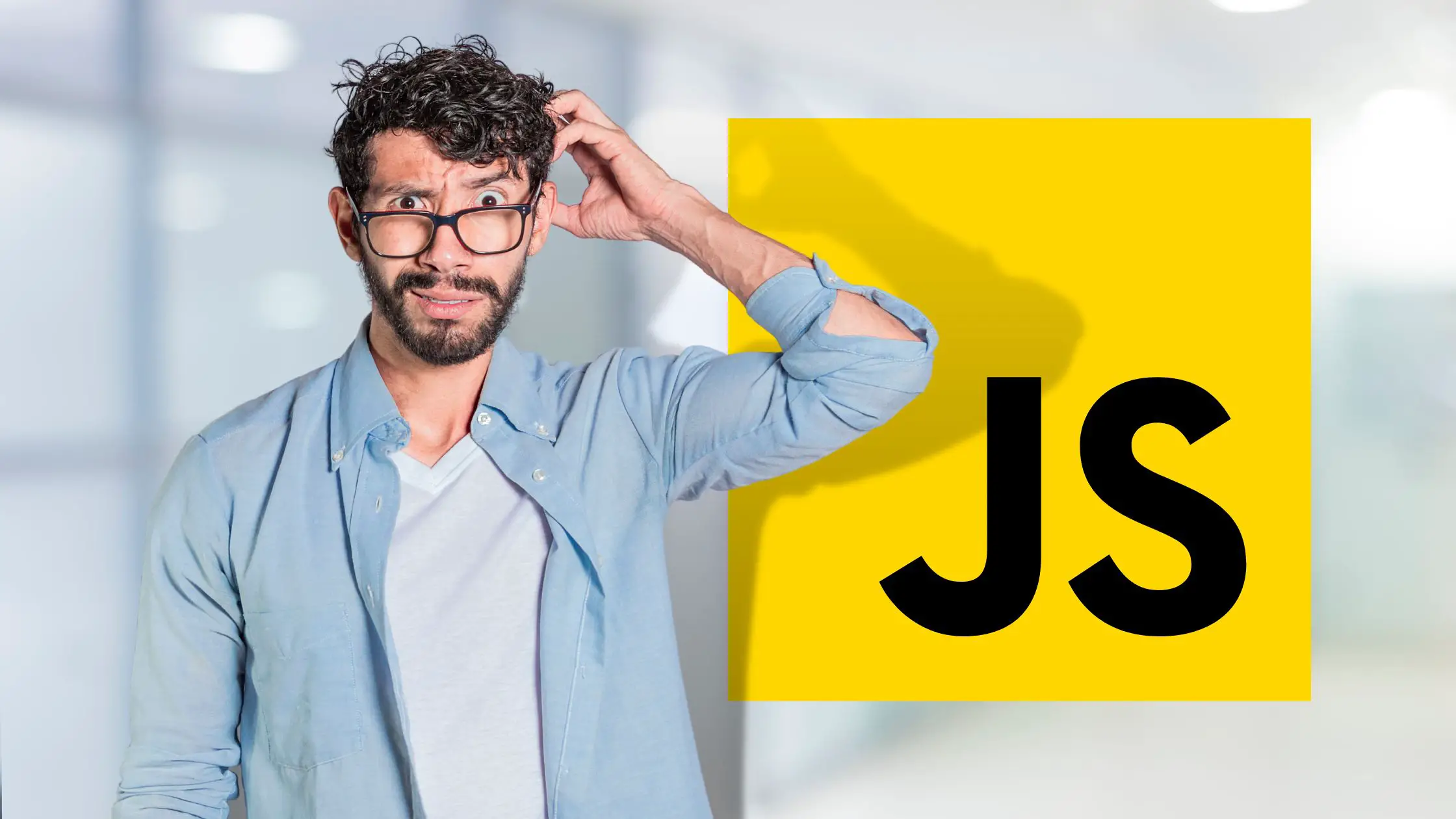
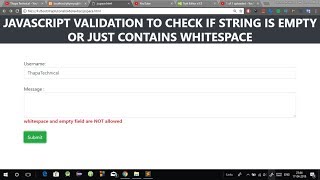
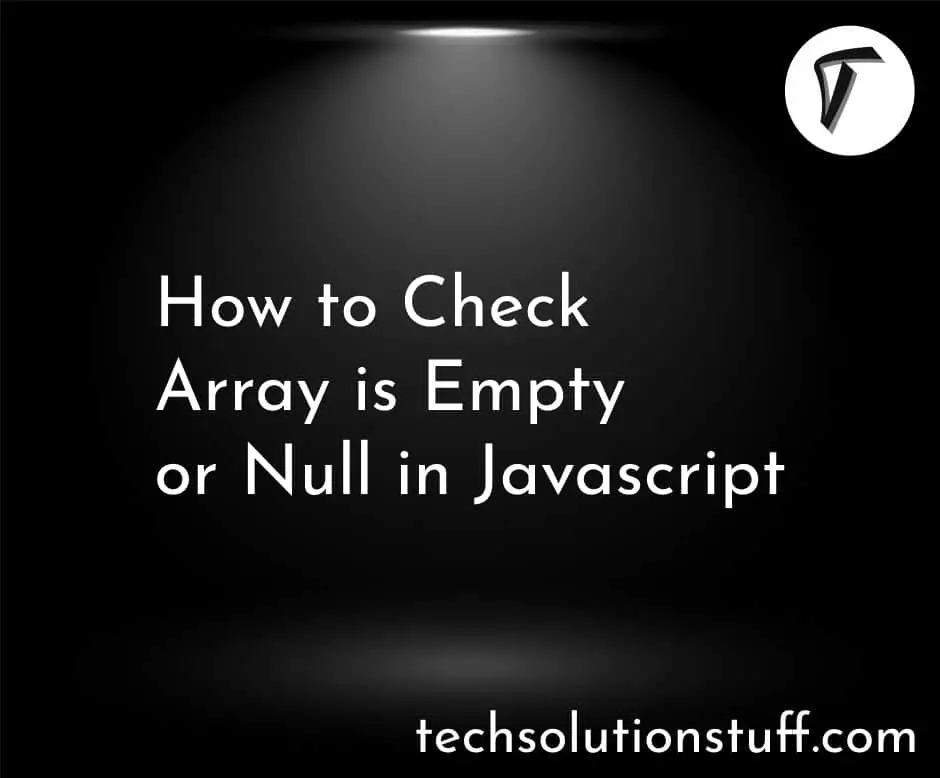

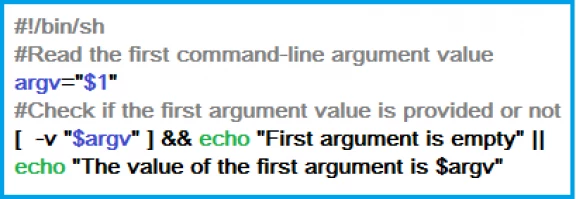
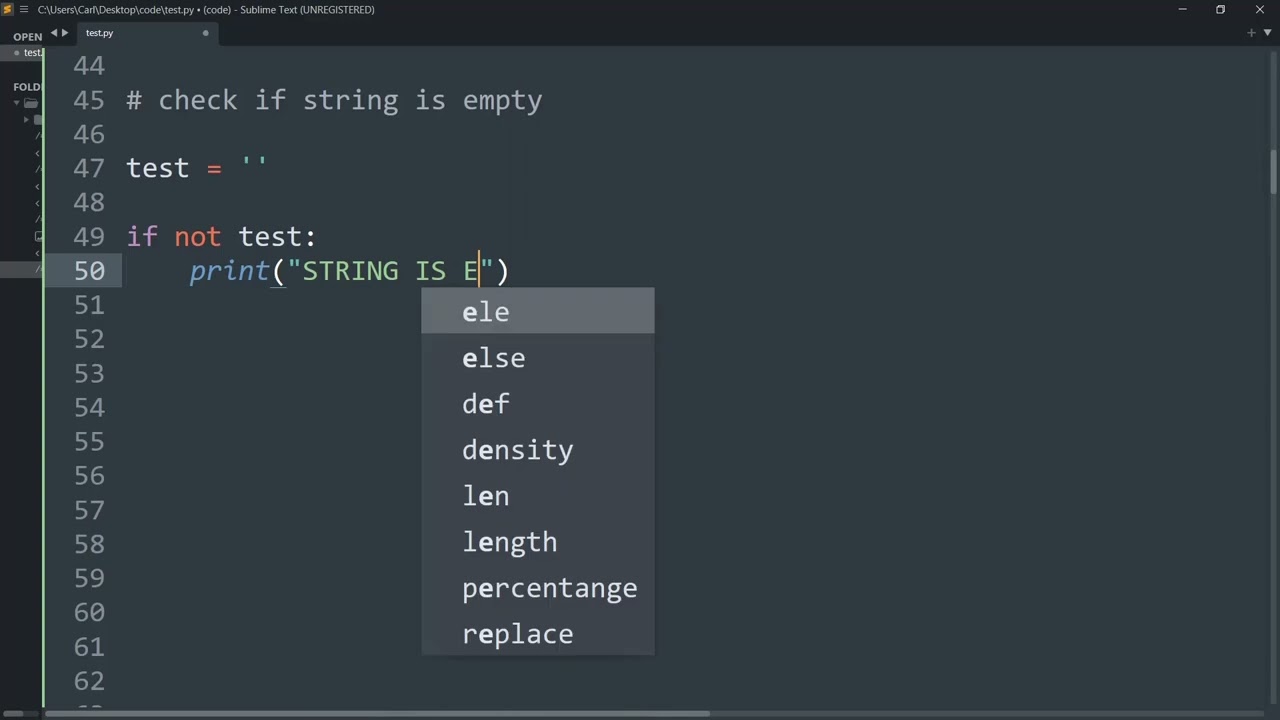

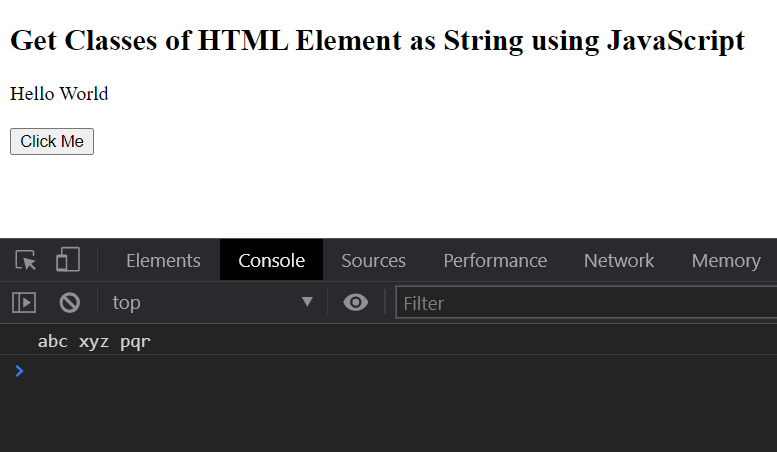

Article link: check string empty javascript.
Learn more about the topic check string empty javascript.
- How do I check for an empty/undefined/null string in JavaScript?
- How to check if a String is Empty in JavaScript – bobbyhadz
- How to Check for an Empty String in JavaScript
- How to Check if a String is Empty in JavaScript – Coding Beauty
- How do I Check for an Empty/Undefined/Null String in … – Sentry
- How to check empty undefined null strings in JavaScript
See more: nhanvietluanvan.com/luat-hoc