Check List Empty Python
An empty list can be very useful in programming when we need a container to store elements dynamically. In Python, creating and checking an empty list can be done in different ways. This article will explore various methods to create an empty list, check if a list is empty, append elements to an empty list, clear an existing list, and check if a list is empty after clearing. Additionally, we will cover frequently asked questions related to empty lists in Python.
Creating an Empty List
There are two common methods to create an empty list in Python:
1. Using square brackets: The simplest way to create an empty list is by using square brackets. Here is an example:
“`python
my_list = []
“`
2. Using the list() function: Another way to create an empty list is by using the list() function. Here is an example:
“`python
my_list = list()
“`
Checking if a List is Empty
To check if a list is empty or not, we can use various methods. Let’s explore three common approaches:
1. Using the if statement and len() function: This method checks if the length of the list is equal to zero. Here is an example:
“`python
if len(my_list) == 0:
print(“The list is empty.”)
else:
print(“The list is not empty.”)
“`
2. Using the if statement and not operator: This method directly checks if the list is not True (i.e., not empty). Here is an example:
“`python
if not my_list:
print(“The list is empty.”)
else:
print(“The list is not empty.”)
“`
3. Using the if statement and comparison with an empty list: This method compares the list with an empty list. Here is an example:
“`python
if my_list == []:
print(“The list is empty.”)
else:
print(“The list is not empty.”)
“`
Appending Elements to an Empty List
Once we have an empty list, we may need to append elements to it. There are multiple methods to achieve this:
1. Using the append() method: We can use the append() method to add elements to the end of the list. Here is an example:
“`python
my_list.append(10)
“`
2. Using the extend() method: The extend() method allows us to add multiple elements to the end of the list. Here is an example:
“`python
my_list.extend([20, 30, 40])
“`
3. Using the insert() method: The insert() method can be used to add an element at a specific index in the list. Here is an example:
“`python
my_list.insert(0, 50) # Adds 50 at index 0
“`
Clearing an Existing List
If we have an existing list and want to remove all its elements, we can use the following methods:
1. Using the clear() method: The clear() method removes all the elements from the list. Here is an example:
“`python
my_list.clear()
“`
2. Using slicing and assignment to an empty list: By assigning an empty list to the existing list, we can effectively clear it. Here is an example:
“`python
my_list = []
“`
3. Using the pop() method in a loop: By repeatedly using the pop() method, we can remove all the elements from the list. Here is an example:
“`python
while my_list:
my_list.pop()
“`
Checking if a List is Empty after Clearing
After clearing a list, we may want to check if it is empty. We can use similar methods as described earlier:
1. Using the if statement and len() function: This method checks if the length of the list is equal to zero. Here is an example:
“`python
if len(my_list) == 0:
print(“The list is empty.”)
else:
print(“The list is not empty.”)
“`
2. Using the if statement and not operator: This method directly checks if the list is not True (i.e., not empty). Here is an example:
“`python
if not my_list:
print(“The list is empty.”)
else:
print(“The list is not empty.”)
“`
3. Using the if statement and comparison with an empty list: This method compares the list with an empty list. Here is an example:
“`python
if my_list == []:
print(“The list is empty.”)
else:
print(“The list is not empty.”)
“`
Frequently Asked Questions:
1. How can I check if an object is empty in Python?
– To check if an object is empty, you can use the `not` operator. For example: `if not my_object:`.
2. What is the best way to check if a list is empty in Python?
– The recommended way is to use the `not` operator: `if not my_list:`.
3. How can I check if a variable is empty in Python?
– You can use the `not` operator to check if a variable is empty. For example: `if not my_variable:`.
4. How do I check if a NumPy array is empty in Python?
– You can use the `size` attribute to check if a NumPy array is empty. For example: `if my_array.size == 0:`.
5. How can I check if a string is empty in Python?
– You can use the `not` operator to check if a string is empty. For example: `if not my_string:`.
6. How can I check if a list contains another list in Python?
– To check if a list contains another list, you can use the `in` operator along with the list. For example: `if sublist in my_list:`.
In conclusion, creating and managing empty lists is a common task in Python programming. By understanding the different methods to create, check, append, and clear empty lists, you can efficiently handle various scenarios. Remember to use the appropriate method based on your specific requirements and preferences.
#Python For Check A List Empty Or Not
Keywords searched by users: check list empty python Check object empty Python, Empty list Python, If variable is empty Python, Check numpy array empty, Check empty string Python, Check list contains list python, Write a Python program to check a list is empty or not, Check element in list Python
Categories: Top 14 Check List Empty Python
See more here: nhanvietluanvan.com
Check Object Empty Python
In Python, checking whether an object is empty or not can be a common task in various programming scenarios. Whether you are working with lists, dictionaries, strings, or custom objects, determining if they are empty or contain data can significantly impact the logic flow and help avoid errors. In this article, we will explore different methods to check object emptiness in Python, along with detailed examples and FAQs to provide you with a complete understanding of the topic.
Understanding Object Emptiness:
Before diving into the methods to check for object emptiness, it is essential to have a clear understanding of what it means for an object to be empty in Python. An object is considered empty if it does not contain any elements or has a length of zero.
Python provides various built-in data structures that we commonly use, such as lists, dictionaries, and strings. We will discuss how to check for emptiness in these common data structures, along with how to handle emptiness for custom objects as well.
Checking for Emptiness in Built-in Data Structures:
1. Checking Empty Lists:
To check if a list is empty, we can utilize the fact that an empty list evaluates to `False` in a boolean context. We can use this property to write a simple condition. Here’s an example:
“`python
my_list = []
if not my_list:
print(“List is empty”)
else:
print(“List is not empty”)
“`
2. Checking Empty Dictionaries:
Similar to lists, we can use a boolean condition to check if a dictionary is empty. An empty dictionary evaluates to `False` as well. Here’s an example:
“`python
my_dict = {}
if not my_dict:
print(“Dictionary is empty”)
else:
print(“Dictionary is not empty”)
“`
3. Checking Empty Strings:
To check if a string is empty, we can simply use the `len()` function and compare it with zero. If the length is zero, the string is empty. Here’s an example:
“`python
my_string = “”
if len(my_string) == 0:
print(“String is empty”)
else:
print(“String is not empty”)
“`
Checking Emptiness of Custom Objects:
When dealing with custom objects, the process of checking for emptiness depends on how the object’s emptiness or non-emptiness is defined. In general, you can utilize the same approach as mentioned above, by customizing the class’s `__bool__` or `__len__` dunder methods.
For instance, let’s consider a custom object called `Person`:
“`python
class Person:
def __init__(self, name):
self.name = name
def __bool__(self):
return bool(self.name)
person1 = Person(“John”)
person2 = Person(“”)
print(bool(person1)) # Output: True
print(bool(person2)) # Output: False
“`
In the above example, the `Person` object’s emptiness is based on whether the `name` attribute has a value or not. By defining the `__bool__` method, we can override the default behavior and determine whether the object should be considered empty or not.
FAQs:
Q1. Can the `len()` function be used universally to check for object emptiness?
While the `len()` function works well for data structures like lists, dictionaries, and strings, it may not be suitable for all objects. It relies on the object having the `__len__` method defined. If the object lacks this method, a `TypeError` will occur. Therefore, it is always a good practice to check the object’s documentation or implement appropriate dunder methods as needed.
Q2. Are there any other methods apart from `__bool__` and `__len__` to check object emptiness?
The `__bool__` method and the `__len__` method are the most commonly used to check object emptiness. However, for specific custom objects, additional methods can be implemented based on their requirements. These additional methods might include checking attributes or specific conditions, depending on the object’s internal logic.
Q3. Can we use the `not` operator to check object emptiness directly?
Yes, the `not` operator can be used directly with objects. As discussed earlier, a blank object or an object with a length of zero is considered `False` in a boolean context. So, using `not` can provide a direct way to check for emptiness.
Conclusion:
Checking object emptiness is a crucial aspect of programming in Python. We explored various methods to check for emptiness, covering built-in data structures like lists, dictionaries, and strings, as well as custom objects. By understanding the concepts discussed in this article, you will be equipped with the knowledge needed to handle object emptiness effectively and write more robust Python code.
Empty List Python
In Python, a list is a versatile and widely-used data structure that allows you to store and manage collections of items. An empty list, as the name suggests, is a list that contains no items. While it may seem trivial at first glance, understanding empty lists in Python is crucial in programming, especially when it comes to initializing lists, handling edge cases, and optimizing memory consumption. In this article, we will explore the concept of empty lists in Python, dive into their fundamentals, cover common use cases, and discuss frequently asked questions.
Understanding Empty Lists:
– To create an empty list in Python, you can simply use a pair of square brackets with no elements inside.
Example: empty_list = []
– Empty lists can be assigned to variables and manipulated just like any other list in Python.
Initializing Lists:
– One common use case for empty lists is initializing a list before adding elements dynamically.
Example: my_list = [] # an empty list to store user inputs later
– Empty lists provide a clean slate to build upon as you start adding elements in your program’s logic.
Manipulating Lists:
– Empty lists can be populated by various means such as appending, extending, or inserting elements.
Example: my_list.append(“element”) # adds “element” at the end of the list
my_list.extend([1, 2, 3]) # extends the list by adding [1, 2, 3] at the end
my_list.insert(0, “start”) # inserts “start” at the beginning of the list
– You can also create a new list with specific elements and assign it to the empty list using slicing.
Example: my_list = [“element1”, “element2”] # initialize the list with specific elements
Handling Edge Cases:
– When working with lists, it’s essential to consider edge cases, such as dealing with empty input.
– You can check if a list is empty by using the `len()` function or by using a simple truthiness test.
Example: if len(my_list) == 0: # checks if list is empty using `len()`
print(“List is empty.”)
if not my_list: # checks if list is empty using truthiness
print(“List is empty.”)
– Handling empty lists appropriately can help prevent errors and make your code more robust.
Memory Optimization:
– Empty lists consume a small, constant amount of memory compared to lists with elements.
– If memory optimization is a concern, using an empty list to initialize and build upon can be advantageous.
Example: Instead of initializing a list with default elements, you can start with an empty list and add elements when needed.
Frequently Asked Questions:
Q1: What is the difference between an empty list and a None value in Python?
A1: An empty list is a valid list object that contains no elements, while None represents the absence of a value or an uninitialized object. In Python, an empty list is a mutable object that can be modified, while None is immutable.
Q2: Can I add elements to an empty list using the index operator?
A2: No, the index operator `[]` is used for accessing elements in a list, not for adding new elements. To append or insert elements, you can use methods like `.append()` or `.insert()`.
Q3: How can I remove elements from an empty list?
A3: Attempting to remove elements from an empty list will result in an error. Before removing elements, ensure that the list has elements using a conditional statement or try-except block to handle the edge case.
Q4: Are empty lists commonly used in Python programming?
A4: Yes, empty lists are frequently used as placeholders, starting points for dynamic input, or as accumulator variables in loops. They provide flexibility and allow for efficient manipulation and storage of data.
Q5: Can I compare an empty list with other data types using logical operators?
A5: Yes, you can compare an empty list with other data types using logical operators. However, the result of the comparison will depend on the specific logic and purpose of your code.
In conclusion, understanding the concept and applications of empty lists in Python is crucial for effective programming. Empty lists serve as a foundation for building dynamic lists and offer memory optimization advantages. By leveraging the power of empty lists, you can better handle edge cases, manage collections of data, and enhance the overall efficiency of your code.
If Variable Is Empty Python
Python, being a versatile and powerful programming language, offers numerous ways to manage and manipulate data. One crucial aspect of any programming language is handling empty or uninitialized variables. In Python, dealing with empty variables involves understanding the concept of variable emptiness and employing appropriate techniques to check and handle them. In this article, we will explore the intricacies of checking if a variable is empty in Python and discuss some common scenarios where this knowledge proves beneficial.
Understanding Variable Emptiness in Python
Before diving into the specifics, let’s establish a basic understanding of empty variables in Python. An empty variable refers to a variable that hasn’t been assigned a value or doesn’t contain any data. In such cases, Python treats the variable as None, a special object representing the absence of a value.
Assigning a variable to None is different from initializing it with an empty value, such as an empty string or an empty list. The variable None explicitly signifies the absence of a value, while an empty value suggests the presence of a value but with no content.
Checking if a Variable is Empty
Python offers several approaches to check if a variable is empty. Each method caters to different scenarios and provides flexibility based on the expected variable type.
1. Using ‘if’ Statement:
The most straightforward method involves using the ‘if’ statement along with the ‘is’ keyword to check if the variable is None. Here’s an example:
“`python
if my_var is None:
print(“Variable is empty”)
“`
This approach is ideal for checking if a variable has not been assigned any value yet.
2. Using ‘not’ Operator:
Another popular method is to use the ‘not’ operator to check if a variable is None. This approach is useful if you want to invert the condition for applying subsequent logic. Here’s an example:
“`python
if not my_var:
print(“Variable is empty”)
“`
This method works well when you only want to check if the variable is empty, irrespective of its type.
3. Using ‘len()’ Function:
If you are dealing with containers like strings, lists, or dictionaries, you can utilize the ‘len()’ function to determine if the container is empty. Let’s consider a list as an example:
“`python
my_list = []
if not len(my_list):
print(“List is empty”)
“`
By checking the length, this method allows you to verify if a container is empty, regardless of its type. However, it may not work as intended for other variable types.
Handling Empty Variables
Once you identify an empty variable, you might need to handle it differently depending on its usage and the desired outcome. Here are a few common techniques to effectively deal with empty variables in Python:
1. Assigning a Default Value:
If a variable is empty, you can assign it a default value to ensure predictable behavior throughout the program. This approach is particularly useful when dealing with user inputs or optional data. Here’s an example:
“`python
my_var = my_var or “Default Value”
“`
By using the ‘or’ operator, the variable can be assigned a default value when it is empty. This technique saves you from potential errors caused by using an uninitialized variable.
2. Raising an Exception:
In some cases, an empty variable might indicate an error or unexpected behavior. Thus, raising an exception can help identify and handle such situations. Here’s a hypothetical scenario:
“`python
if my_var is None:
raise ValueError(“Variable cannot be empty”)
“`
By raising an exception, your code can halt execution and provide an informative error message to help identify the issue.
Frequently Asked Questions (FAQs)
Q1. Can a variable be empty without being assigned None?
Yes, a variable can be empty without being explicitly assigned None. For example, if you initialize a variable as an empty string or an empty list, it would not be equal to None, but still considered empty.
Q2. How can I handle multiple variables to check if any of them are empty?
To handle multiple variables simultaneously, you can combine the conditions using logical operators like ‘or’ or ‘and’. For example:
“`python
if var1 is None or var2 is None or var3 is None:
print(“At least one of the variables is empty”)
“`
Q3. What is the difference between an empty variable and an uninitialized variable?
An uninitialized variable refers to a variable that has not been assigned any value yet. In Python, uninitialized variables will throw a NameError when used. Conversely, an empty variable generally refers to a variable explicitly assigned None or a container with no elements.
Conclusion
Effectively dealing with empty variables is vital for writing robust and error-free Python code. By understanding the concept of variable emptiness and employing appropriate techniques, you can ensure your code behaves as intended even when encountering empty or uninitialized variables. Whether it is assigning default values, raising exceptions, or simply checking for None, Python provides versatile methods to tackle empty variables efficiently and effectively.
Images related to the topic check list empty python
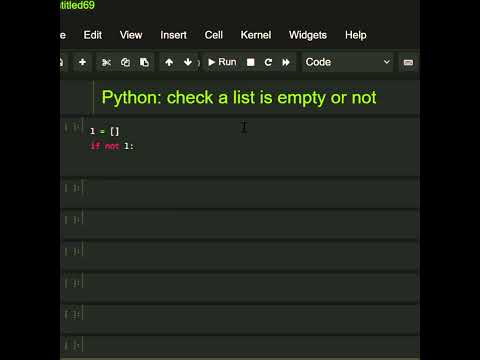
Found 11 images related to check list empty python theme

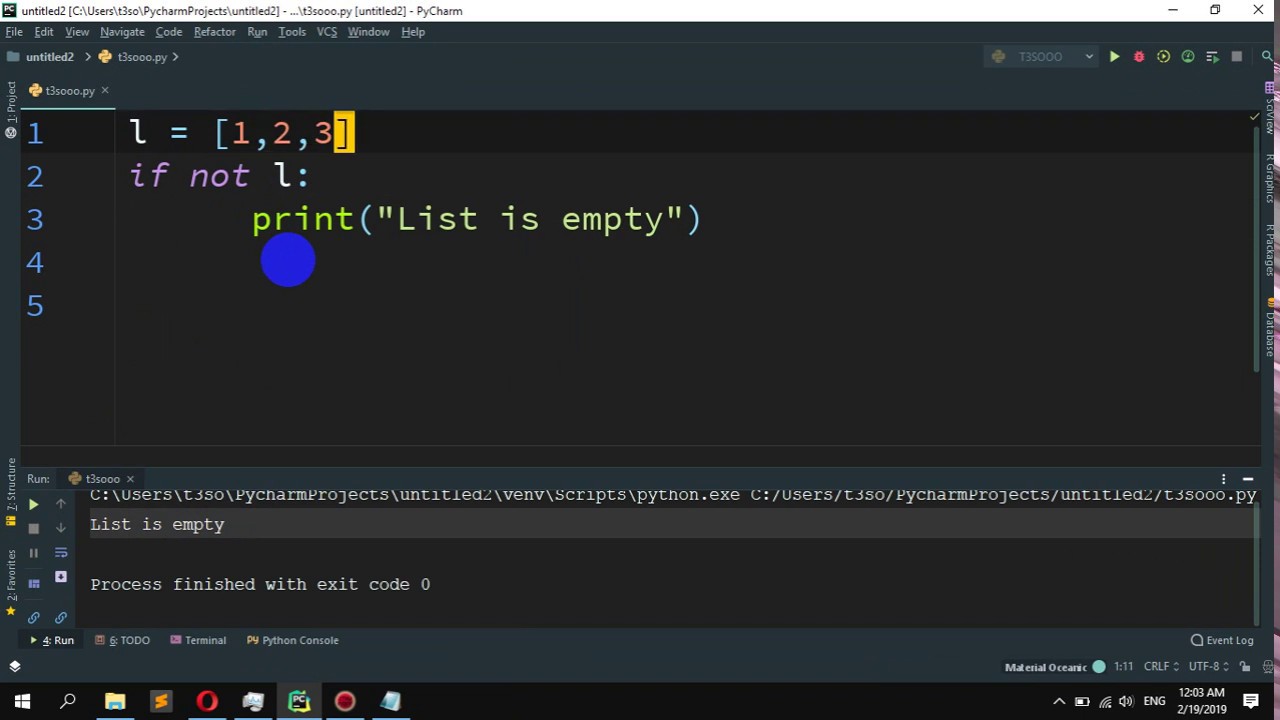
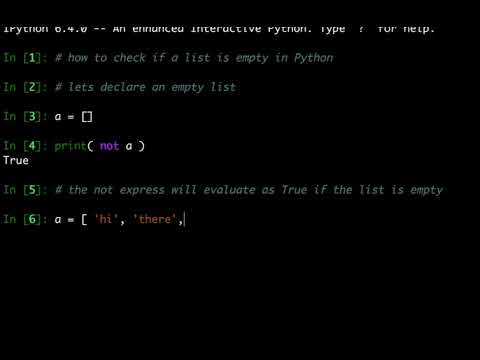







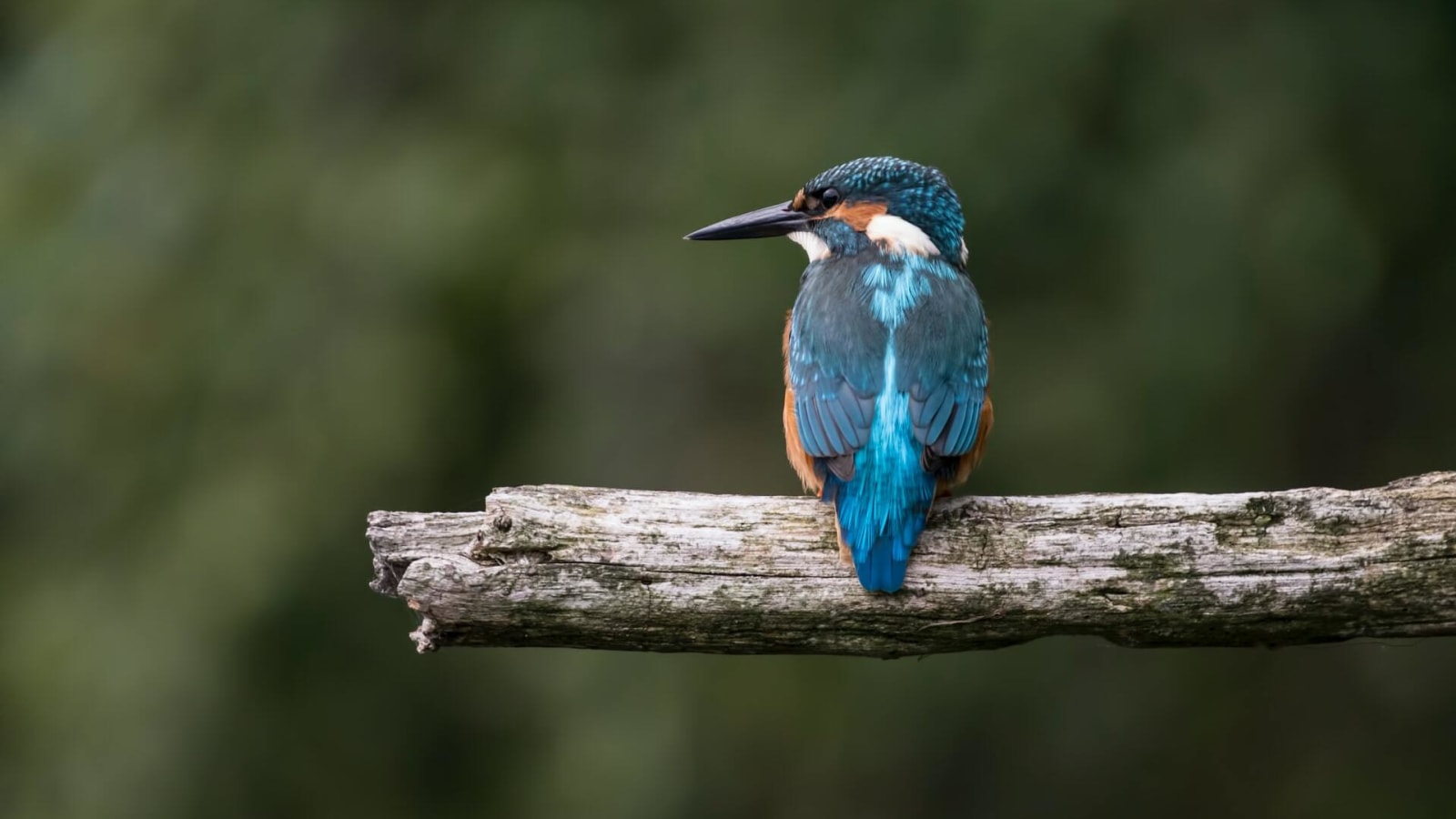
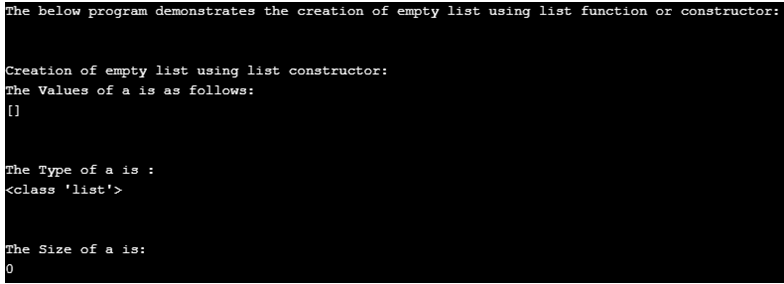


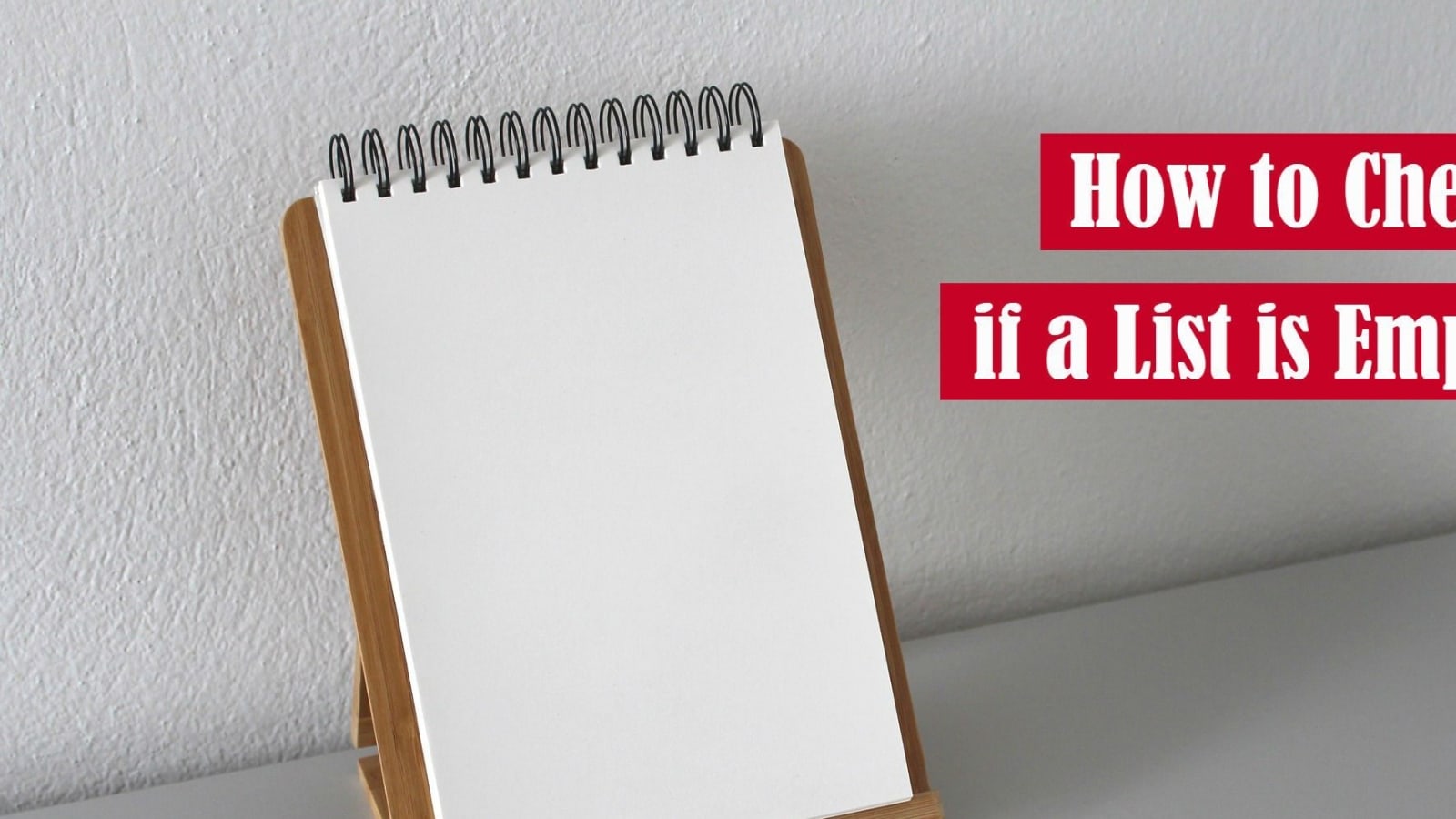
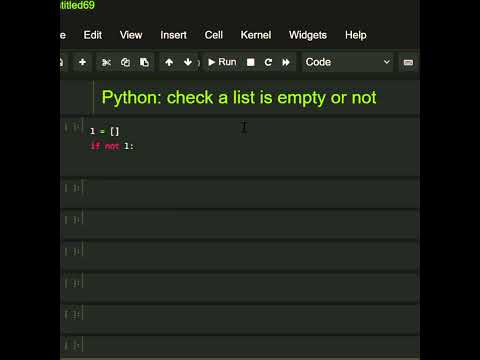
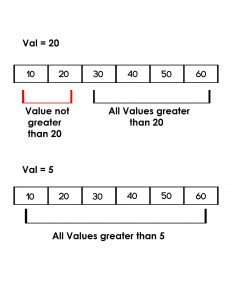

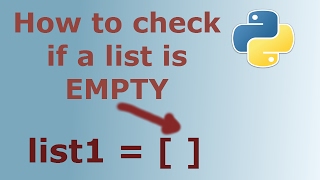
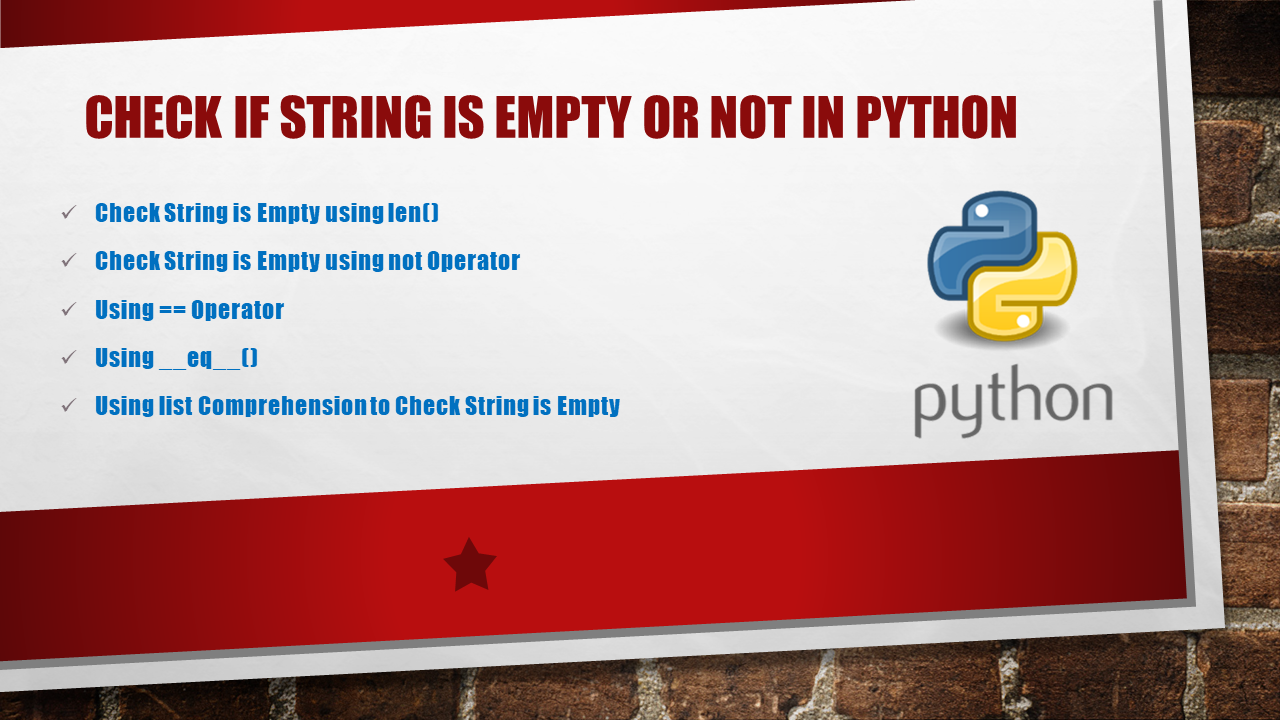

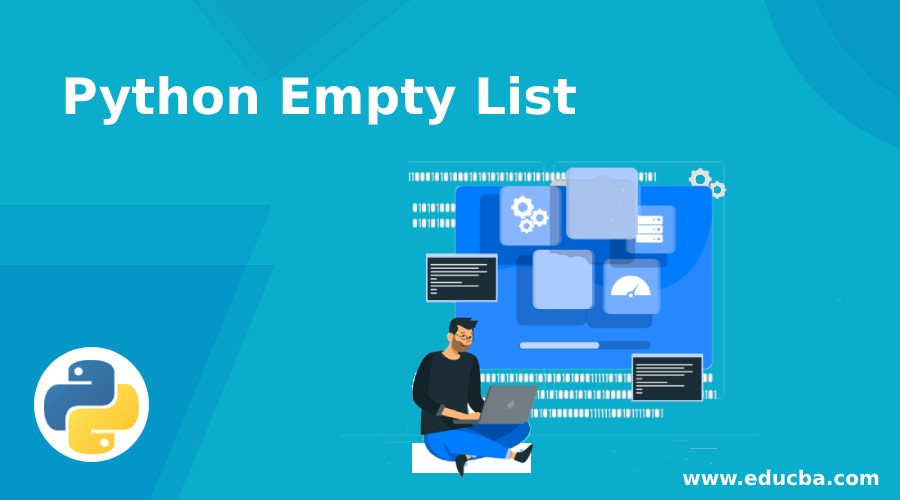
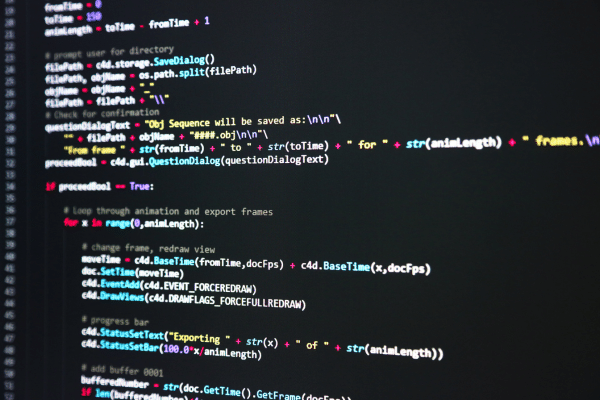
![Sample Paper] Kabir is a Python programmer working in a school. Sample Paper] Kabir Is A Python Programmer Working In A School.](https://d1avenlh0i1xmr.cloudfront.net/6447e807-9ecc-4410-9e08-0392f6f8ecab/python-program-(with-comments)-to-update-and-display-the-records-in-a-csv-file---teachoo.png)



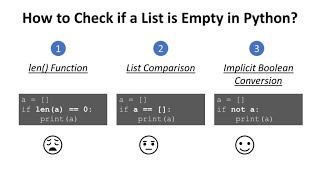
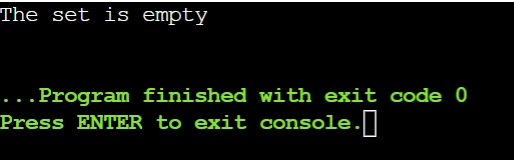
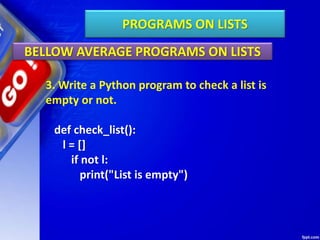
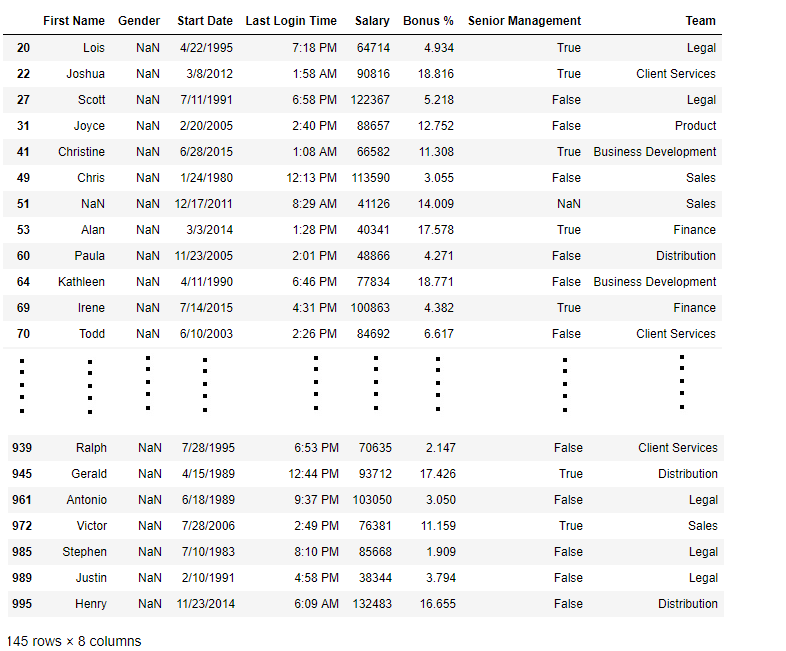


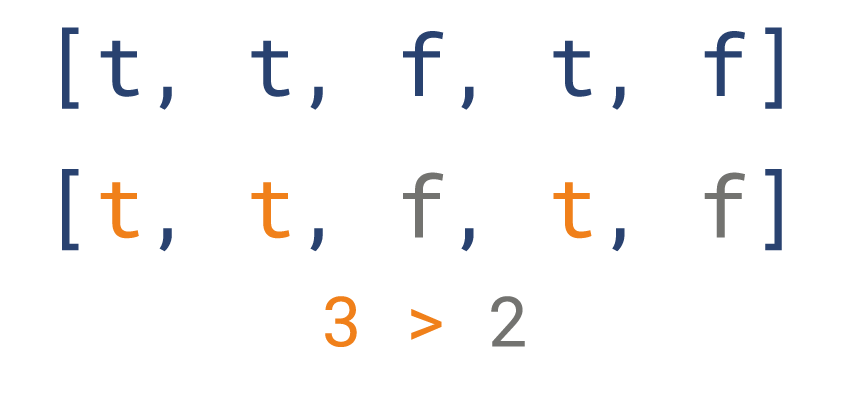


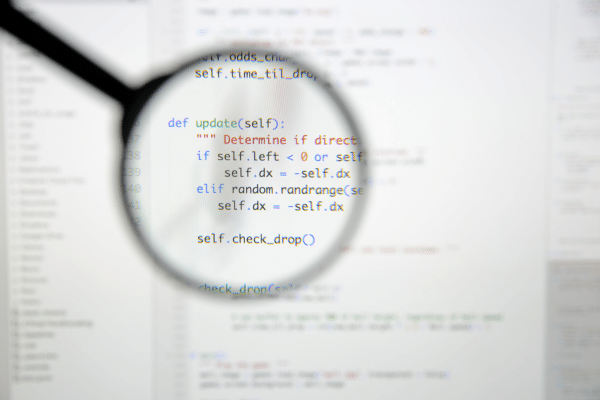


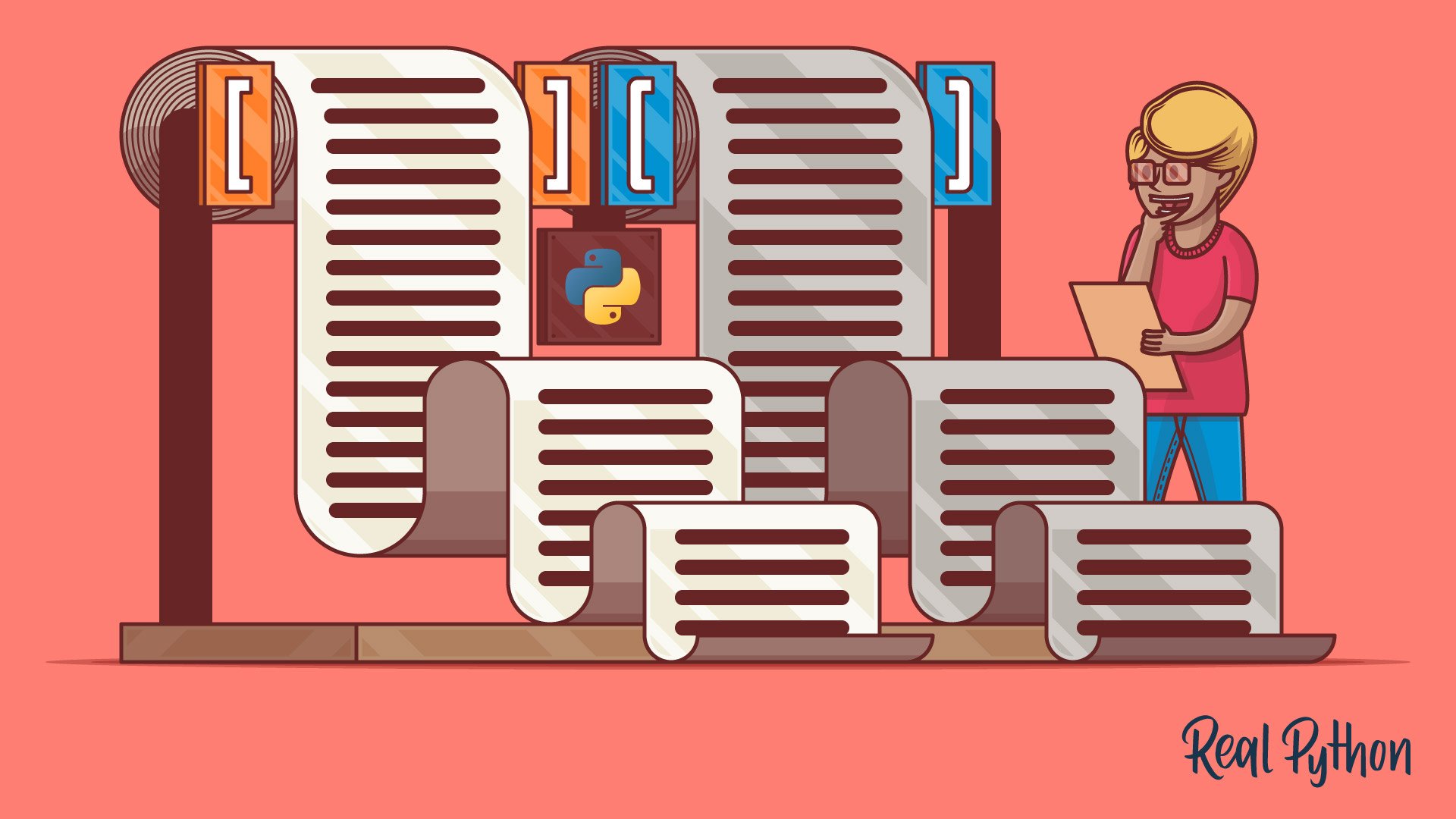

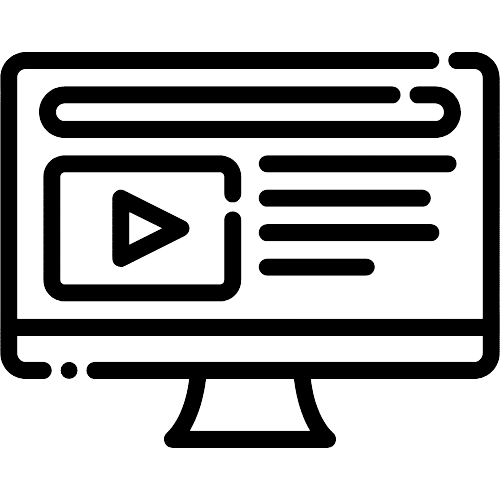

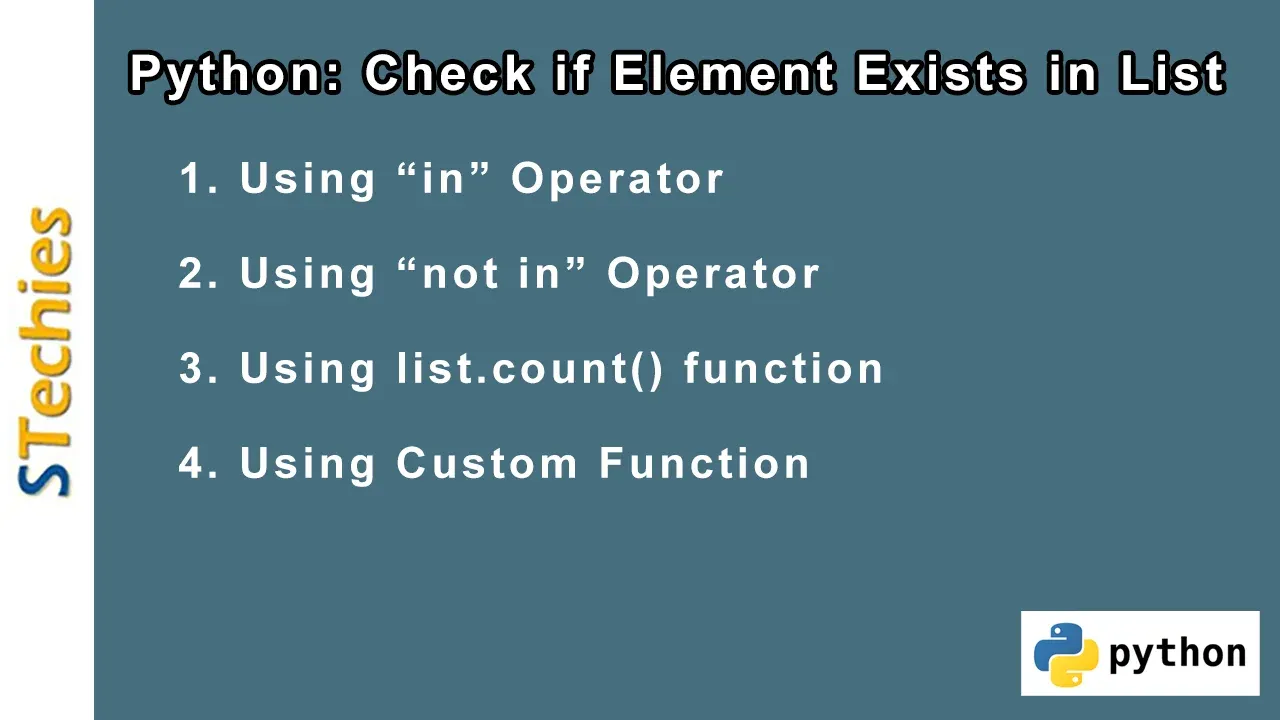

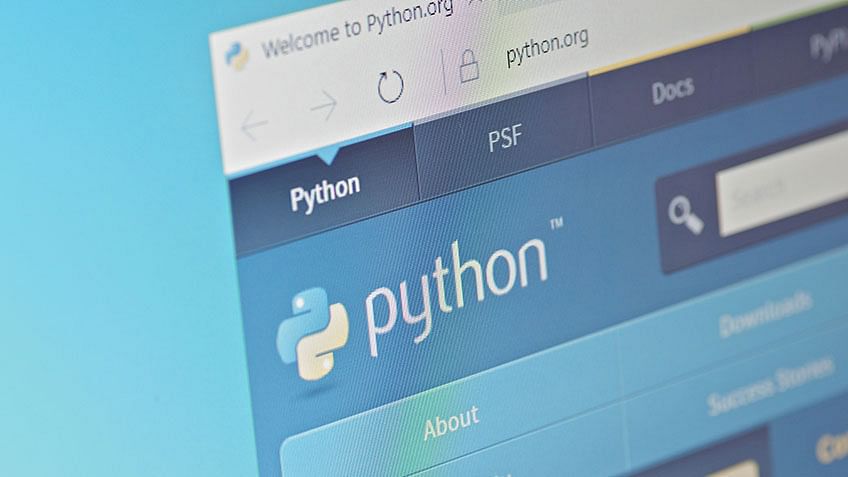
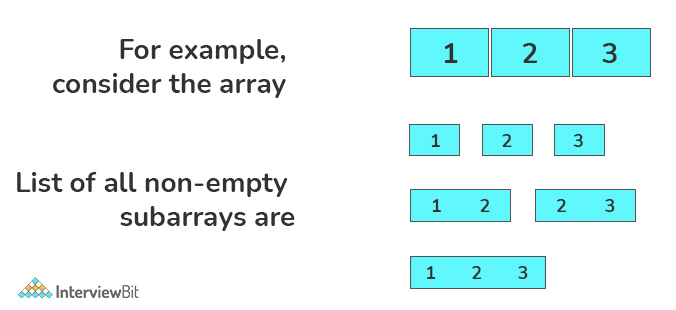
Article link: check list empty python.
Learn more about the topic check list empty python.
- How to check if a list is empty in Python? – Flexiple Tutorials
- python – How do I check if a list is empty? – Stack Overflow
- Python isEmpty() equivalent – How to Check if a List is Empty …
- How to check if a list is empty in Python – Tutorialspoint
- Python – Check if a list is empty or not – GeeksforGeeks
- How to Check if List is Empty in Python – Stack Abuse
- How To Check If A List Is Empty In Python?
- Here is how to check if a list is empty in Python – PythonHow
- Check if the List is Empty in Python – Spark By {Examples}
- Check if a list is empty in Python | Techie Delight
See more: blog https://nhanvietluanvan.com/luat-hoc