Check If String Empty Python
In Python, it is often necessary to check if a string is empty before performing certain operations or making decisions in the code. Empty strings can arise when a user does not provide any input or when a string variable is not assigned a value. This article will explore different methods and techniques to check if a string is empty in Python, along with some important concepts related to handling empty strings.
Using the len() Function to Check for an Empty String
One of the simplest ways to check if a string is empty in Python is by using the len() function. The len() function returns the length of a string, and if the length is zero, it means the string is empty. Here’s an example:
“`python
my_string = “”
if len(my_string) == 0:
print(“The string is empty.”)
“`
In this code snippet, we create an empty string and then use the len() function to check its length. If the length is zero, we print a message indicating that the string is empty. This method works efficiently and is widely used in Python code.
Applying the bool() Function to Determine If a String is Empty
Another approach to check if a string is empty in Python is by applying the bool() function. The bool() function converts a value to its corresponding Boolean value, which is either True or False. When applied to a string, if the string is empty, the bool() function returns False. Here’s an example:
“`python
my_string = “”
if not bool(my_string):
print(“The string is empty.”)
“`
In this code snippet, we pass the string variable to the bool() function and use the not keyword to check if the Boolean value is False. If it is, we print a message indicating that the string is empty. This method provides a concise way to check for empty strings in Python.
Utilizing If Statements to Check for an Empty String
If statements are commonly used in Python to perform logical checks and make decisions based on certain conditions. To check if a string is empty, we can utilize if statements along with the comparison operator ==. Here’s an example:
“`python
my_string = “”
if my_string == “”:
print(“The string is empty.”)
“`
In this code snippet, we directly compare the string variable with an empty string using the == operator. If they are equal, we print a message indicating that the string is empty. This method is straightforward and easy to understand.
Using Regular Expressions to Verify If a String is Empty
Regular expressions are powerful tools in Python for pattern matching and string manipulation. Though not the most common method for checking empty strings, regular expressions can also be used. Here’s an example:
“`python
import re
my_string = “”
if re.match(“^\s*$”, my_string):
print(“The string is empty.”)
“`
In this code snippet, we use the re.match() function to match the entire string with a regular expression pattern. The pattern “^\s*$” checks if the string consists only of whitespace characters or is completely empty. If it matches, we print a message indicating that the string is empty. Regular expressions provide flexibility to handle various patterns but may not be necessary for simple empty string checks.
Understanding the Importance of Handling Empty Strings in Python
Handling empty strings is crucial in Python as they can lead to unexpected behavior or errors in code execution. If a variable is empty, attempting to perform operations on it may result in runtime errors. Similarly, if user inputs are not validated for emptiness, it can cause issues in the program’s logic. It is essential to account for these scenarios and handle them appropriately.
FAQs
Q1. How can I check if a variable is empty in Python?
Ans. To check if a variable is empty in Python, you can use multiple methods. One common approach is to use the len() function. If len(variable) == 0, it means the variable is empty.
Q2. How can I check if the user input is empty in Python?
Ans. To check if user input is empty in Python, you can utilize the len() function or if statements. For example, if len(user_input) == 0 or if user_input == “”, it means the input is empty.
Q3. How can I check if a string contains only spaces in Python?
Ans. To check if a string contains only spaces in Python, you can use regular expressions or the isspace() method. For example, if my_string.isspace() returns True, it means the string contains only spaces.
Q4. How can I check if an object is empty in Python?
Ans. The concept of emptiness for objects may vary depending on the object’s type and purpose. For custom objects, you can define a method or property to check emptiness. For built-in objects, you can typically use the len() function or if statements to check emptiness.
Q5. How can I check if an object is null in Python?
Ans. In Python, the concept of null values is typically represented as None. To check if an object is null, you can directly compare it with None using if object_name is None.
Conclusion
Checking if a string is empty in Python is a fundamental task in programming. By using methods such as the len() function, bool() function, if statements, or regular expressions, you can efficiently handle empty strings and prevent potential errors. Remember to consider the importance of handling empty strings in your code to ensure robustness and reliability.
Checking For An Empty, Null, Or Undefined String
How To Detect Empty String Python?
Dealing with strings is a fundamental part of programming, and there are situations when we need to determine whether a string is empty or not. In Python, detecting empty strings is a straightforward task, but it’s essential to know the various approaches available to achieve this. In this article, we will explore different methods to detect empty strings in Python and discuss their pros and cons.
Before diving into the solutions, let’s clarify what an empty string actually means. In programming, an empty string refers to a string variable that contains no characters. It is represented by “”, which is simply two double quotes with nothing in between. Now, let’s get started!
Method 1: Using len() function
The simplest way to check if a string is empty is by using the len() function. This function returns the length of a given object, and if the length of the string is zero, it means the string is empty. Here’s an example that demonstrates its usage:
“`python
string1 = “”
if len(string1) == 0:
print(“Empty string”)
else:
print(“Non-empty string”)
“`
This method works perfectly fine and is widely used. However, keep in mind that it may not be the most efficient approach when dealing with large strings since it calculates the length of the entire string.
Method 2: Using the not operator
Another intuitive way to detect an empty string is by utilizing the not operator. By applying the not operator to a string variable, we can check if it evaluates to False, indicating an empty string. Here’s an example:
“`python
string2 = “”
if not string2:
print(“Empty string”)
else:
print(“Non-empty string”)
“`
This method is more concise and often preferred due to its simplicity. It is also efficient as it does not perform any additional calculations like the len() function. However, be cautious when using this approach as it will consider any string that evaluates to False (e.g., “”, None, False) as empty.
Method 3: Comparing with an empty string
In Python, we can compare strings with an empty string using the equality operator (==). If the given string is equal to “”, it means the string is empty. Here’s an example to illustrate this method:
“`python
string3 = “”
if string3 == “”:
print(“Empty string”)
else:
print(“Non-empty string”)
“`
This method is straightforward and easy to understand. However, keep in mind that it may not be the most efficient approach in terms of execution time when dealing with large strings.
Frequently Asked Questions:
Q1: What is the difference between using len() and the not operator?
Both methods are used to detect empty strings, but their approach differs. The len() function calculates the length of the string, and if it is zero, it indicates an empty string. On the other hand, the not operator checks if the variable evaluates to False, which includes empty strings. The not operator method is usually preferred due to its simplicity and efficiency.
Q2: Are there any alternative approaches to detect empty strings?
Yes, you can use various other methods to determine if a string is empty. Some alternative methods include using the string’s Boolean evaluation, checking if the string is None, using regular expressions, or using the built-in string methods like str.isspace() or str.strip(). Each method has its own advantages and operands, so choose the one that suits your specific needs.
Q3: Can I combine multiple methods to detect empty strings?
Certainly! You can combine multiple detection methods to strengthen your condition. For example, you can use the not operator along with checking the length of the string, such as using `if not string and len(string) == 0:`. However, keep in mind that redundancy in such scenarios can be avoided, and it is generally recommended to choose the most appropriate method based on your requirement.
Q4: What should I do if I want to handle empty strings differently?
If you want to perform specific actions based on whether a string is empty or not, you can use conditionals such as if-else statements. By applying the desired actions inside the respective blocks, you can easily differentiate the handling of empty and non-empty strings.
In conclusion, detecting empty strings in Python is a common task and can be accomplished using several methods. While each approach has its own advantages and disadvantages, the choice depends on factors such as simplicity, efficiency, and the specific requirements of your program. By understanding and using the appropriate method, you can ensure smooth and effective string handling in your Python programs.
How To Check If String Is Empty?
In programming, data validation is a critical aspect, and checking if a string is empty is an essential part of it. Whether you are a beginner or an experienced developer, this article will cover everything you need to know about verifying the emptiness of a string in different programming languages.
1. Introduction to Empty Strings
An empty string, also known as a null string, is a string that contains no characters. It is represented by a pair of double quotes (“”) or a null reference. Checking for an empty string is significant as it helps avoid errors and ensures that the code functions as intended.
2. How to Check if a String is Empty
2.1. Languages without Built-in Functions (C, C++, Java pre-11)
In programming languages like C, C++, and Java versions before 11, there is no built-in function to check for an empty string. However, it can be achieved by comparing the string’s length with zero using the `strlen` function.
Here is an example in C++:
“`cpp
#include
#include
int main() {
std::string str = “”;
if (str.length() == 0) {
std::cout << "String is empty." << std::endl;
} else {
std::cout << "String is not empty." << std::endl;
}
return 0;
}
```
2.2. Python
Python is known for its simplicity, and checking if a string is empty is no exception. The most straightforward method is to use the logical operator `not` to verify if the string evaluates to False.
```python
str = ""
if not str:
print("String is empty.")
else:
print("String is not empty.")
```
2.3. JavaScript
In JavaScript, there are several ways to check for an empty string. One common approach is using the conditional `if` statement with the `length` property.
```javascript
var str = "";
if (str.length === 0) {
console.log("String is empty.");
} else {
console.log("String is not empty.");
}
```
2.4. PHP
PHP offers multiple ways to determine if a string is empty. One way is to use the `empty` function, which evaluates the variable as a boolean value.
```php
$str = "";
if (empty($str)) {
echo "String is empty.";
} else {
echo "String is not empty.";
}
```
3. Best Practices and Considerations
3.1. Trim the String
Before checking for emptiness, it is a good practice to trim the string to remove leading or trailing whitespace. This prevents false positives or negatives, ensuring accurate validation.
3.2. Be Aware of Null Values
When dealing with databases or user input, it's important to consider the possibility of null values. Check for null values before checking for emptiness to avoid errors.
3.3. Understand String Comparison
In some languages, comparing strings can be different from comparing integers or other data types. Be familiar with the specific rules for string comparison in your chosen programming language.
4. FAQs
Q1. Is there a difference between an empty string and a null string?
A1. Yes, there is a distinction between an empty string, which contains no characters, and a null string, which is a null reference. An empty string has a length of zero, while a null string has no value at all.
Q2. Can I use the `==` operator to check if a string is empty?
A2. It depends on the programming language. Some languages, like JavaScript, allow the use of the `==` operator for this purpose, while others, like Python, require the use of the `not` operator.
Q3. Why should I trim the string before checking for emptiness?
A3. Trimming the string removes leading and trailing whitespace, ensuring that spaces before or after the actual content do not interfere with the emptiness check. This improves the accuracy of your validation.
Q4. Is it necessary to check for null values before checking for emptiness?
A4. Yes, it is essential to check for null values, especially when dealing with user input or data from databases. Neglecting to do so can lead to errors or unexpected behavior in your code.
Q5. Can I use regular expressions to check for an empty string?
A5. While it is technically possible to use regular expressions, it is not recommended for such a simple task. Using a built-in function or logical operators is a more efficient and readable approach.
In conclusion, checking if a string is empty is a fundamental programming task that requires attention to detail and consideration of language-specific functions or techniques. By following the examples and best practices outlined in this article, you'll be able to precisely determine the emptiness of a string in various programming languages with ease. Remember to trim the string and handle null values appropriately to ensure accurate validation in your applications.
Keywords searched by users: check if string empty python If variable is empty Python, Python check if input is empty, Check if string contains only spaces python, Check object empty Python, Python check null, Check if object is null python, Python check string empty or whitespace, Python how to check null value
Categories: Top 30 Check If String Empty Python
See more here: nhanvietluanvan.com
If Variable Is Empty Python
Python is a versatile programming language that offers various functionalities suitable for different applications. One common task in programming is checking whether a variable is empty or not. In this article, we will delve into different methods to determine if a variable is empty in Python and explore some frequently asked questions on the topic.
Understanding Empty Variables in Python
Before diving into ways to check if a variable is empty, let’s first understand what an empty variable means in Python. In Python, an empty variable refers to a variable that does not contain any value or has a value equal to None. It is important to note that an empty variable is different from a variable with a value of zero or an empty string.
Methods to Check if a Variable is Empty in Python
1. Using if statement
The simplest way to check if a variable is empty is by using the if statement. This method involves evaluating the variable within the condition of an if statement. If the variable is empty, the condition will be true, and you can perform the desired actions accordingly. Here’s an example:
“`python
variable = None
if variable:
# code to execute if variable is not empty
else:
# code to execute if variable is empty
“`
2. Using the len() function
The len() function in Python is primarily used to get the length of an object, such as a string, list, or tuple. However, it can also be utilized to check if a variable is empty. By passing the variable as a parameter to the len() function, we can determine if it contains any elements. If the length is zero, the variable is empty. Here’s an example:
“`python
variable = “”
if len(variable) == 0:
# code to execute if variable is empty
else:
# code to execute if variable is not empty
“`
3. Using the not operator
The not operator in Python can be used to reverse the truth value of a condition. By combining the not operator with the if statement, we can check if a variable is empty. For example:
“`python
variable = []
if not variable:
# code to execute if variable is empty
else:
# code to execute if variable is not empty
“`
4. Using the isinstance() function
The isinstance() function in Python is used to check if an object belongs to a specific class or data type. It can also be utilized to determine if a variable is empty. By verifying if the variable is an instance of the desired data type (such as list, dict, or string), we can establish if it is empty. Here’s an example:
“`python
variable = []
if isinstance(variable, list) and not variable:
# code to execute if variable is an empty list
else:
# code to execute if variable is a non-empty list or of a different data type
“`
Frequently Asked Questions (FAQs)
Q1: How can I check if a variable is empty or contains only whitespace?
To check if a variable is empty or contains only whitespace, you can use the strip() method along with the len() function. For example:
“`python
variable = ” ”
if len(variable.strip()) == 0:
# code to execute if variable is empty or contains only whitespace
else:
# code to execute if variable is not empty or contains non-whitespace characters
“`
Q2: What happens if I try to access an empty variable?
If you try to access an empty variable, you may encounter an error. For example, if you try to access an empty list, you may receive an “IndexError: list index out of range” error. It is crucial to ensure that variables are not empty before accessing their elements.
Q3: Can I check if a variable is empty without using any conditionals or functions?
No, it is not possible to check if a variable is empty without using any conditionals or functions. Conditionals and functions are essential tools to evaluate a variable’s state and determine if it is empty or not.
Q4: What should I do if a variable is empty?
If a variable is empty, you can handle it according to your program’s requirements. You may decide to assign a default value or prompt the user to enter a valid value. Handling empty variables is crucial to prevent unexpected errors and ensure the smooth execution of your program.
Conclusion
Checking if a variable is empty is an essential task in Python programming. There are various methods available, including using if statements, the len() function, the not operator, and the isinstance() function. By understanding these techniques, you can effectively determine if a variable is empty and take appropriate actions based on the result. Remember to handle empty variables appropriately to avoid errors and unexpected behaviors in your Python programs.
Python Check If Input Is Empty
When it comes to user input, Python provides us with the `input()` function, which allows us to receive input from the user via the command line. However, sometimes users may accidentally hit the enter key without providing any input. In such cases, it becomes necessary to check whether the input is empty or contains some value. Let’s explore a few approaches to handle this scenario.
1. Using Conditional Statements:
The most straightforward way to check for empty input is to use a conditional statement such as an `if` statement. We can evaluate if the length of the user input is zero, indicating an empty input. Here’s an example:
“`python
user_input = input(“Please enter something: “)
if len(user_input) == 0:
print(“Input is empty”)
else:
print(“Input is not empty”)
“`
2. Using the `strip()` method:
Another handy method is `strip()`, which removes leading and trailing whitespace characters from a string. By applying `strip()` to the user input, we can check if the stripped input is empty or not. Here is an example:
“`python
user_input = input(“Please enter something: “)
if user_input.strip() == ”:
print(“Input is empty”)
else:
print(“Input is not empty”)
“`
3. Using the `isspace()` method:
The `isspace()` method checks whether all characters in a string are whitespace characters. By using this method, we can determine if the user input consists only of whitespaces. Let’s take a look at an example:
“`python
user_input = input(“Please enter something: “)
if user_input.isspace():
print(“Input is empty”)
else:
print(“Input is not empty”)
“`
These are a few simple approaches to check for empty user input in Python. However, there are some notable considerations to keep in mind while implementing these methods.
– Case sensitivity: The above methods do not differentiate between empty input and input consisting of whitespace characters. If you need to distinguish between these cases, you can modify the condition accordingly.
– Error handling: When handling user input, it is important to consider potential errors that may arise. For instance, if the user interrupts the input process using a keyboard interruption signal (e.g., Ctrl+C), Python may raise a `KeyboardInterrupt` exception. Adequate error handling mechanisms should be in place to handle such scenarios.
Now, let’s address some frequently asked questions related to checking for empty input in Python:
### FAQs:
Q1. What happens if the user enters only a space character?
A1. If the user enters only a space character, the methods discussed above will consider it as empty input since they remove leading and trailing whitespace characters.
Q2. How can I handle cases where I want to consider spaces as valid input?
A2. To consider leading and trailing spaces as valid input, you can modify the condition to check if the stripped input length is zero while keeping the original input intact.
Q3. What if I want to check for multiple inputs and exit only when all inputs are empty?
A3. In such cases, you can utilize a loop to repeatedly ask for input and accumulate the results. Then, you can check if all the inputs are empty and terminate the loop accordingly.
Q4. Are there any Python libraries that simplify empty input checking?
A4. While there are no specific libraries just for checking empty input, Python provides other libraries like `argparse` for handling command-line arguments in a more structured manner.
Wrapping Up:
In this article, we delved into multiple techniques to check if user input is empty in Python. We explored conditional statements, the `strip()` method, and the `isspace()` method. Additionally, a FAQs section addressed common queries on this topic. By following these approaches and considering the mentioned considerations, you can effectively handle empty user input scenarios in your Python programs.
Check If String Contains Only Spaces Python
Python is a versatile programming language that provides several built-in functions and methods to perform various tasks efficiently. One common requirement is to check whether a given string contains only spaces. There are different approaches to achieve this, depending on the complexity of the task and the specific needs. In this article, we will explore multiple methods to check if a string contains only spaces using Python. We will also address some frequently asked questions related to this topic.
Method 1: Using the isspace() method
Python strings come with a built-in isspace() method which returns True if a string consists of only whitespace characters, such as spaces, tabs, or newlines. Using this method, we can easily solve our problem with just a single line of code.
“`python
def contains_only_spaces(string):
return string.isspace()
“`
This function takes a string as input and returns True if the string contains only spaces. Otherwise, it returns False. Let’s test this function on a few examples:
“`python
print(contains_only_spaces(” “)) # Output: True
print(contains_only_spaces(” Hello “)) # Output: False
print(contains_only_spaces(“”)) # Output: False
“`
Method 2: Using the replace() method
Another approach to check if a string contains only spaces is by utilizing the replace() method. In this method, we can replace all whitespace characters with an empty string and check if the resulting string is empty.
“`python
def contains_only_spaces(string):
return string.replace(‘ ‘, ”) == ”
“`
This function takes a string as input, replaces all spaces with an empty string, and checks if the resulting string is empty. If the result is empty, it means that the input string only contained spaces. Otherwise, it contained other characters as well.
Method 3: Using a regular expression
Regular expressions are powerful tools for pattern matching, and they can be used to solve complex string operations. In this method, we can use a regular expression pattern to check if a string contains only spaces.
“`python
import re
def contains_only_spaces(string):
return re.match(r’^\s*$’, string) is not None
“`
This function takes a string as input and uses the re.match() function to match the pattern. The pattern ‘^’ matches the start of the string, ‘\s*’ matches zero or more whitespace characters, and ‘$’ matches the end of the string. If the match is successful, it means that the input string contains only spaces.
FAQs
Q1: Can the methods mentioned above be used for strings containing non-ASCII whitespace characters?
A1: Yes, both the isspace() and regular expression methods can handle non-ASCII whitespace characters, such as tabs or newlines, as they are considered whitespace.
Q2: Are leading and trailing spaces considered valid in the given string?
A2: Yes, all the methods discussed above consider leading and trailing spaces in the string. If a string contains only leading or trailing spaces, the methods will still return True.
Q3: Can the methods handle strings with multiple whitespace characters in between?
A3: Yes, all the methods can handle strings with multiple whitespace characters in between. They treat any consecutive whitespace characters as a single space and check if the entire string consists of such spaces.
Q4: Are there any performance differences between the three methods?
A4: The isspace() method is generally the fastest, followed by the replace() method. However, the performance differences are negligible unless working with extremely large strings or using the methods in a computationally intensive loop.
Q5: Can the methods handle Unicode strings?
A5: Yes, all the methods described above can handle Unicode strings in Python. Python’s string handling is Unicode-aware, so the methods will work correctly with Unicode strings.
In conclusion, Python provides multiple approaches to check if a string contains only spaces. Depending on the specific requirements and complexity, you can choose the method that best suits your needs. Whether it’s using built-in functions like isspace(), utilizing the replace() method, or using regular expressions with re.match(), Python ensures that you have the tools to tackle this task efficiently.
Images related to the topic check if string empty python
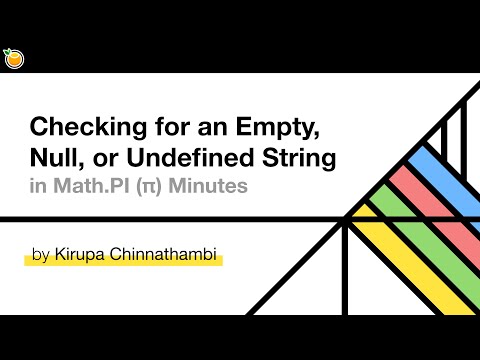
Found 34 images related to check if string empty python theme
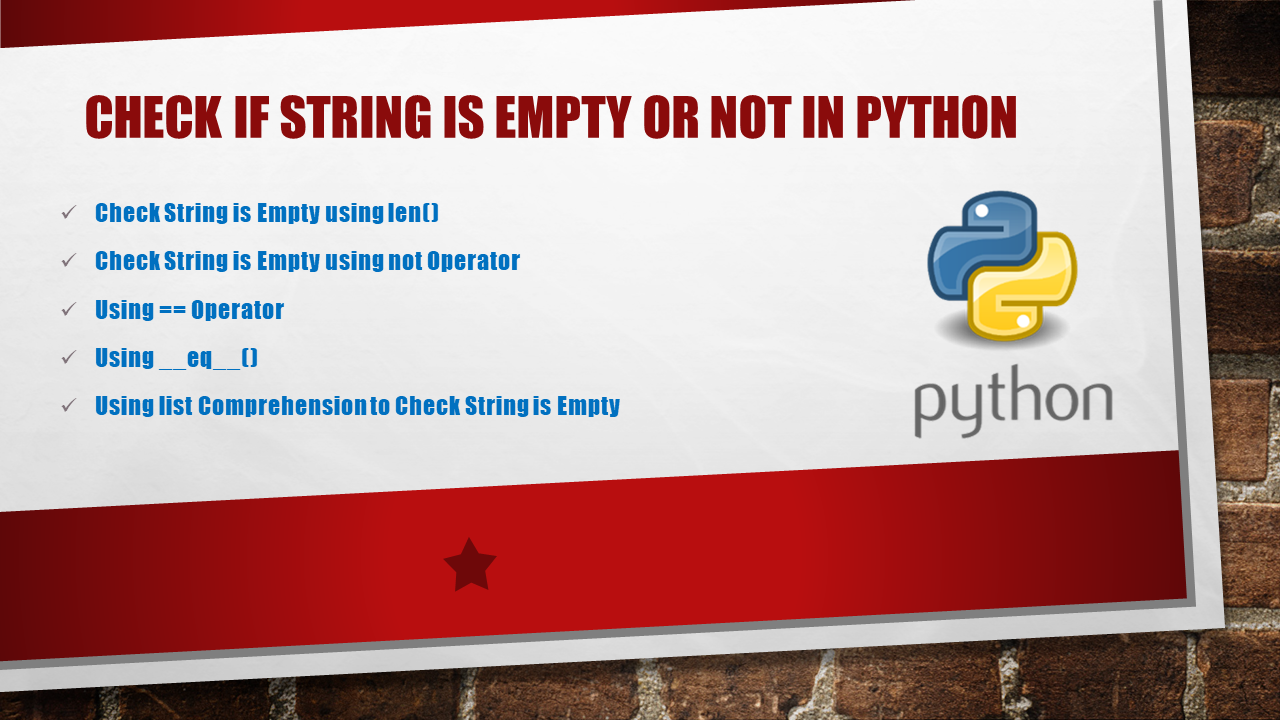
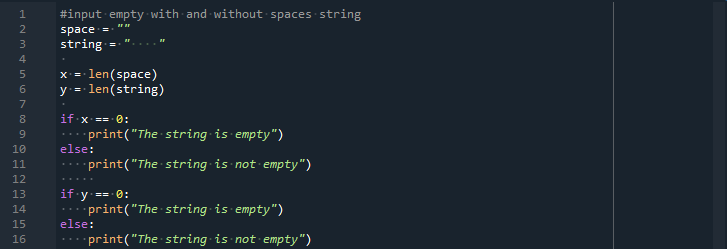

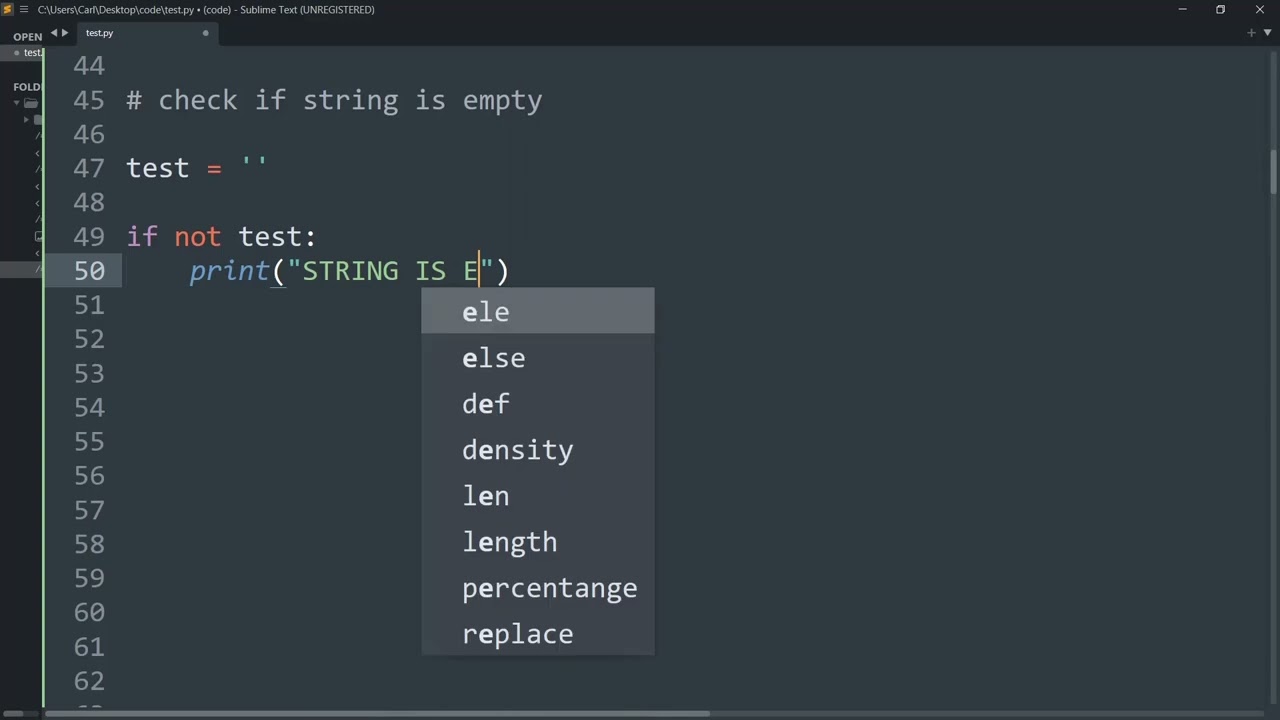

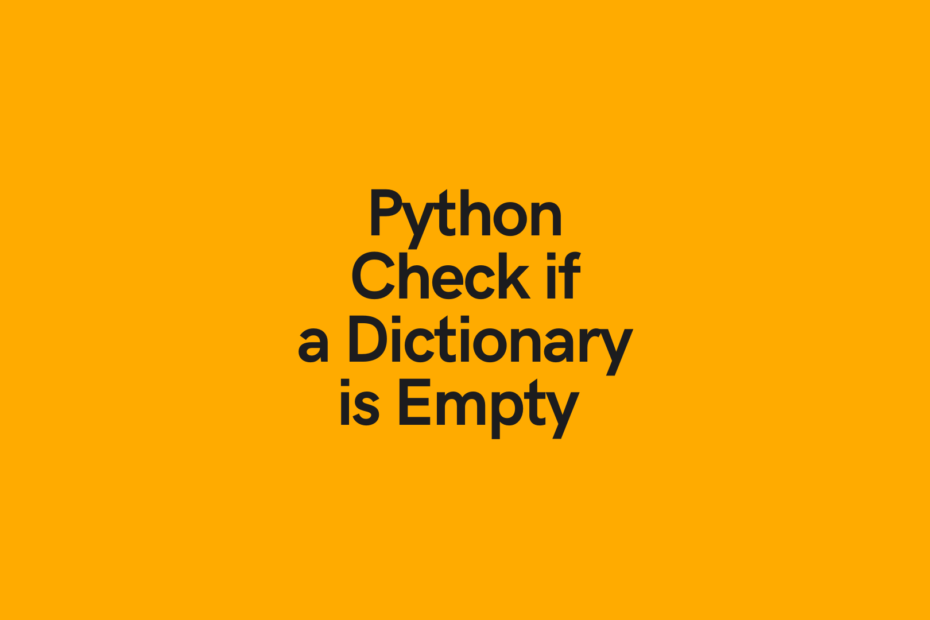




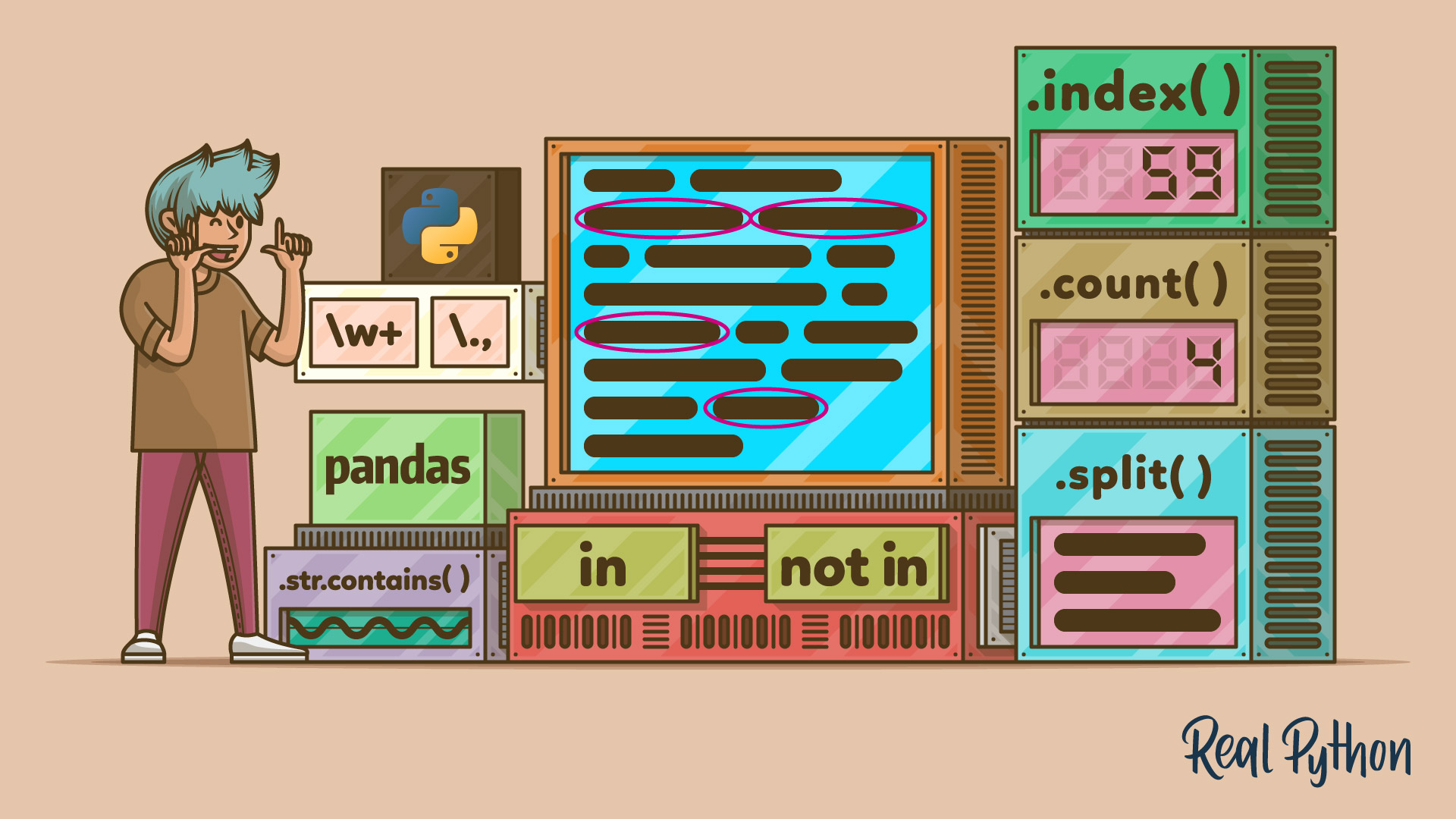
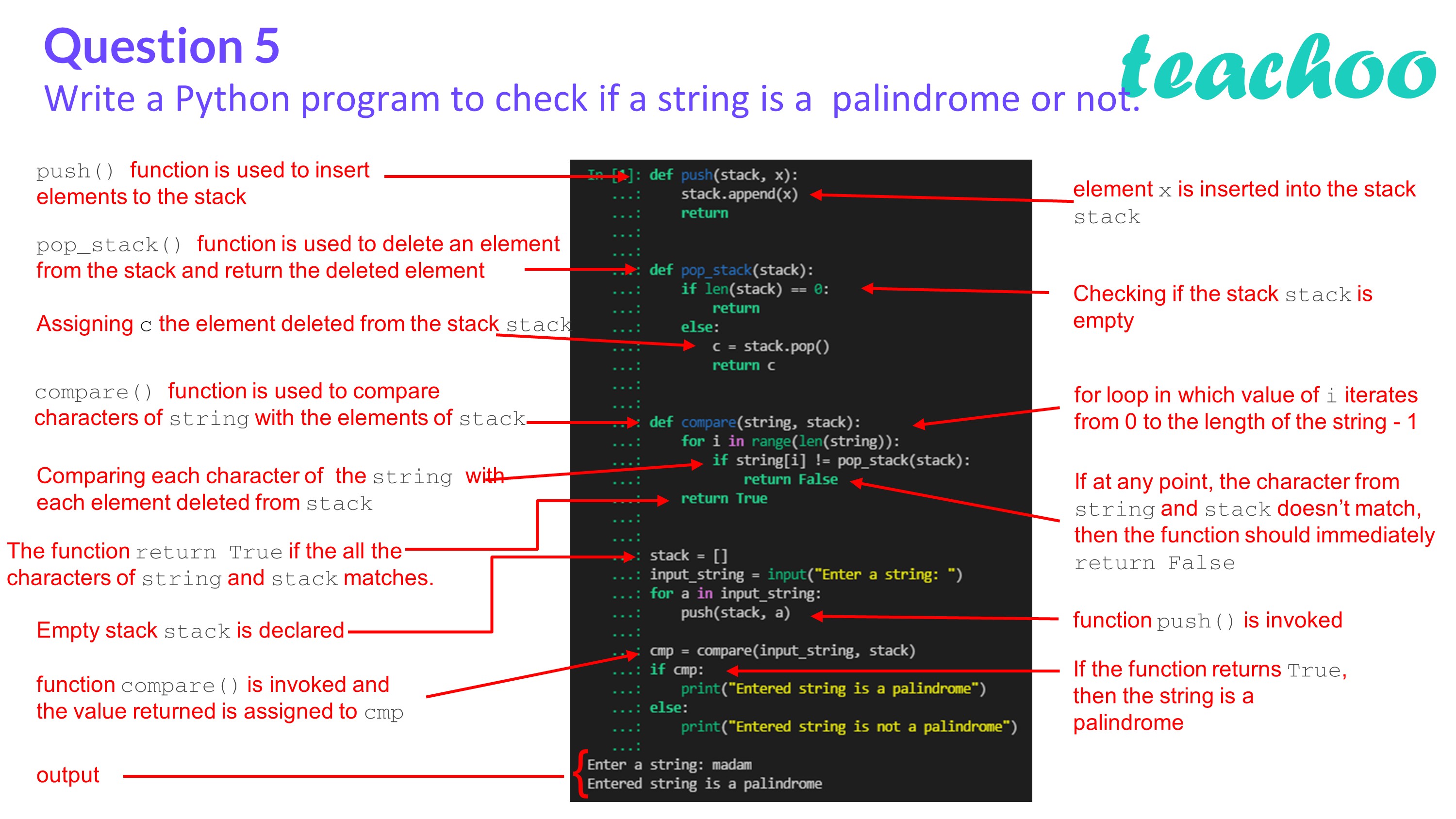



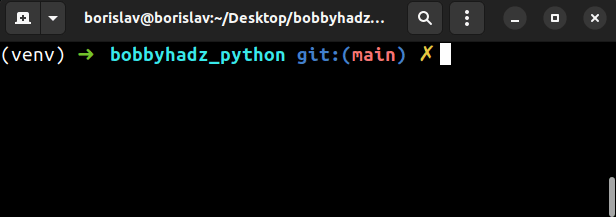



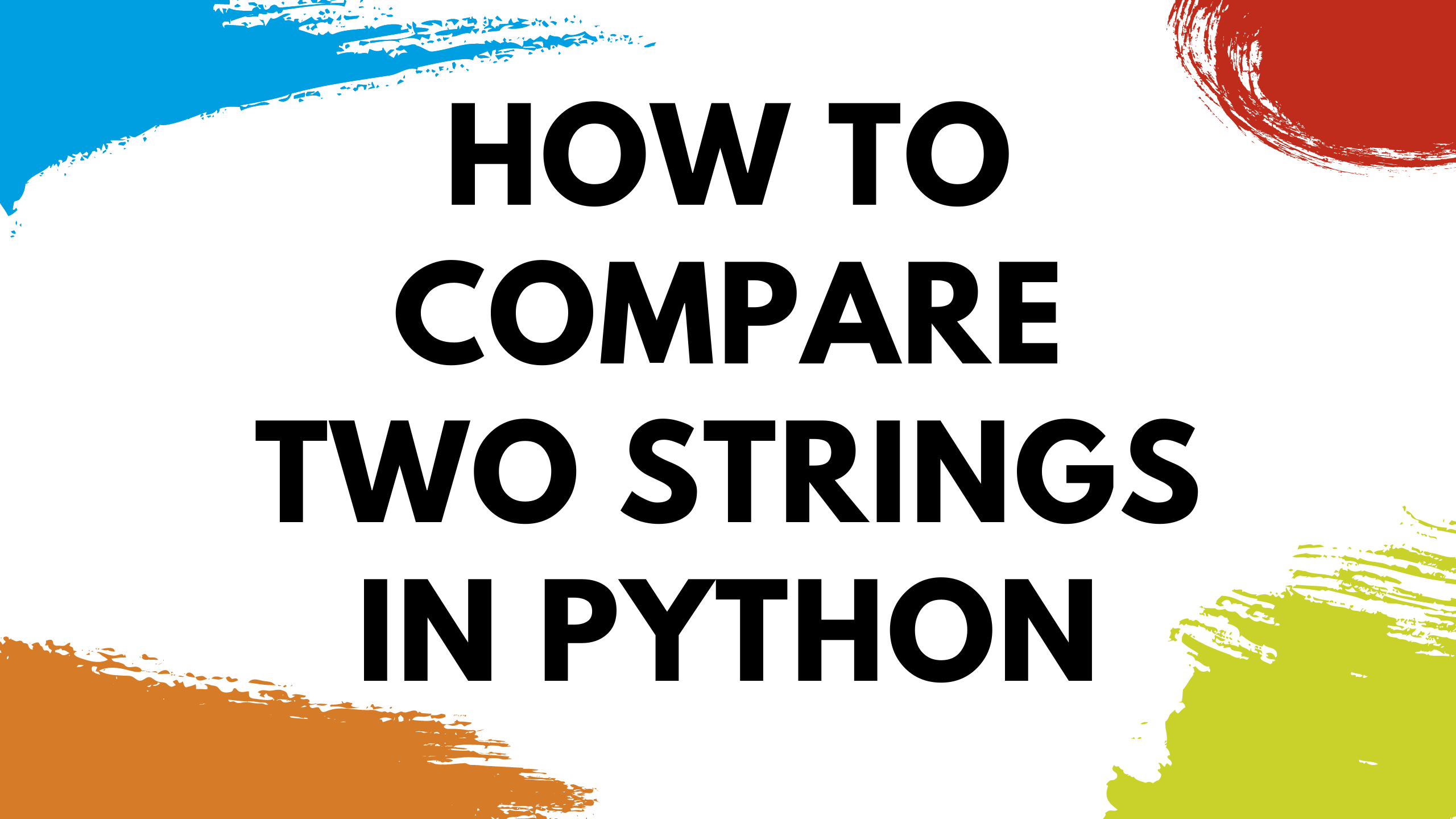
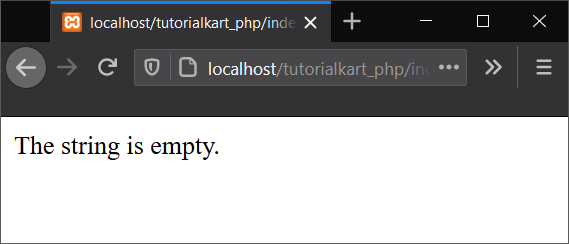

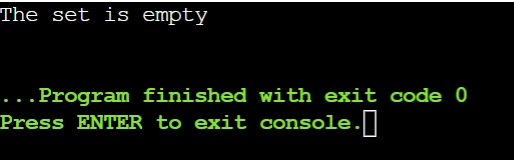
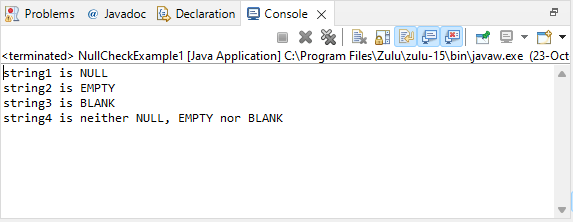
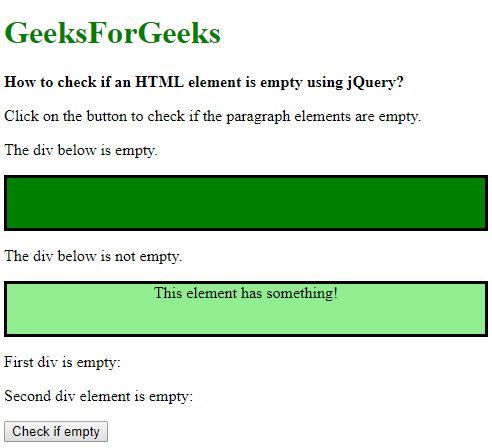

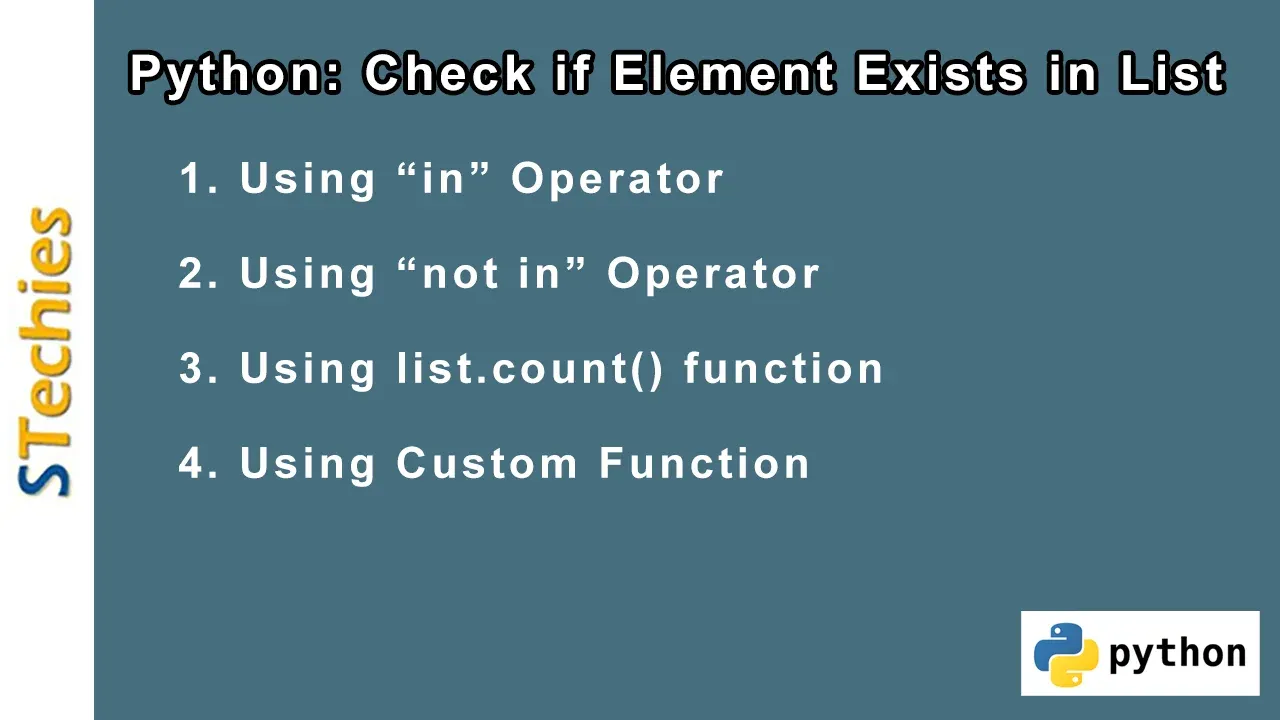


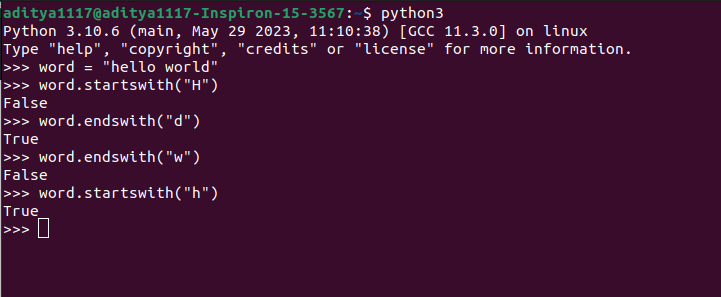
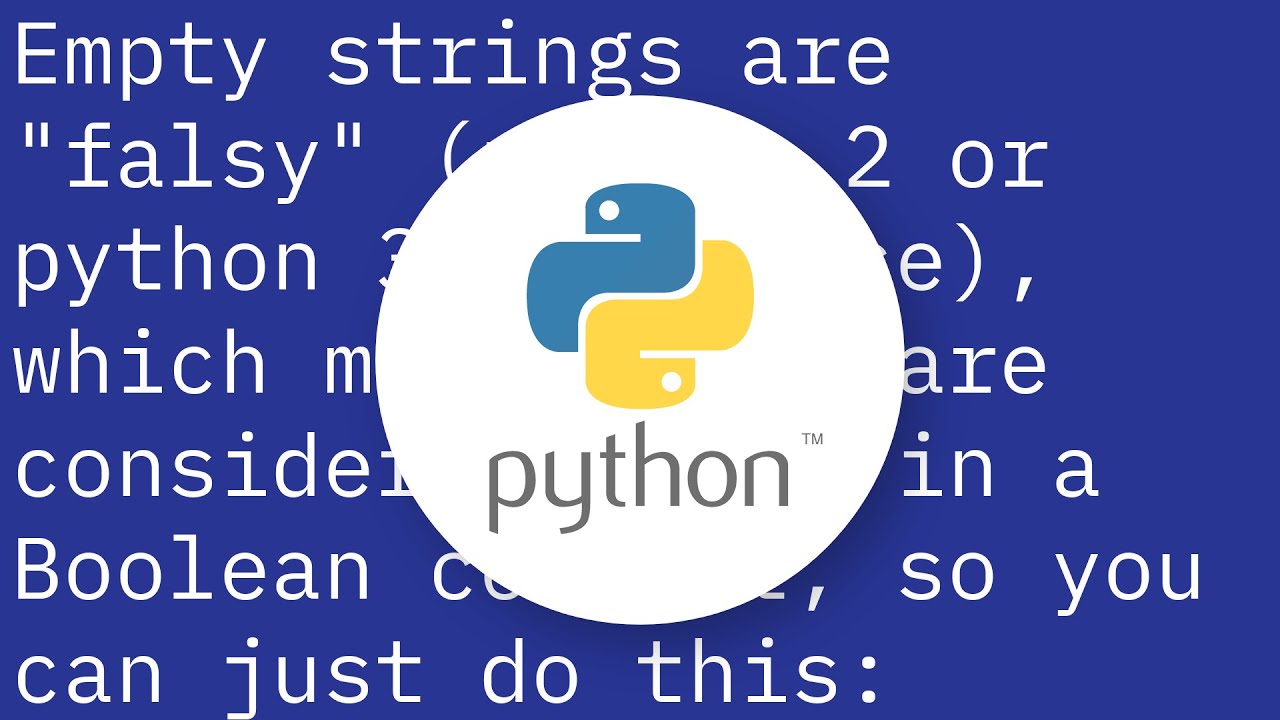

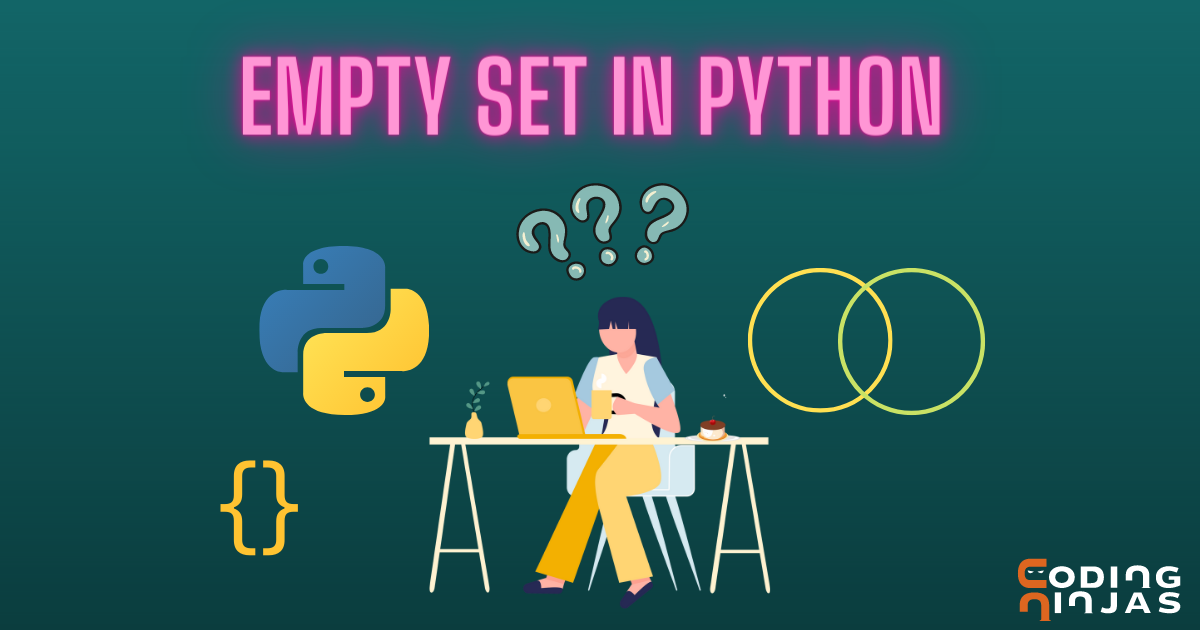


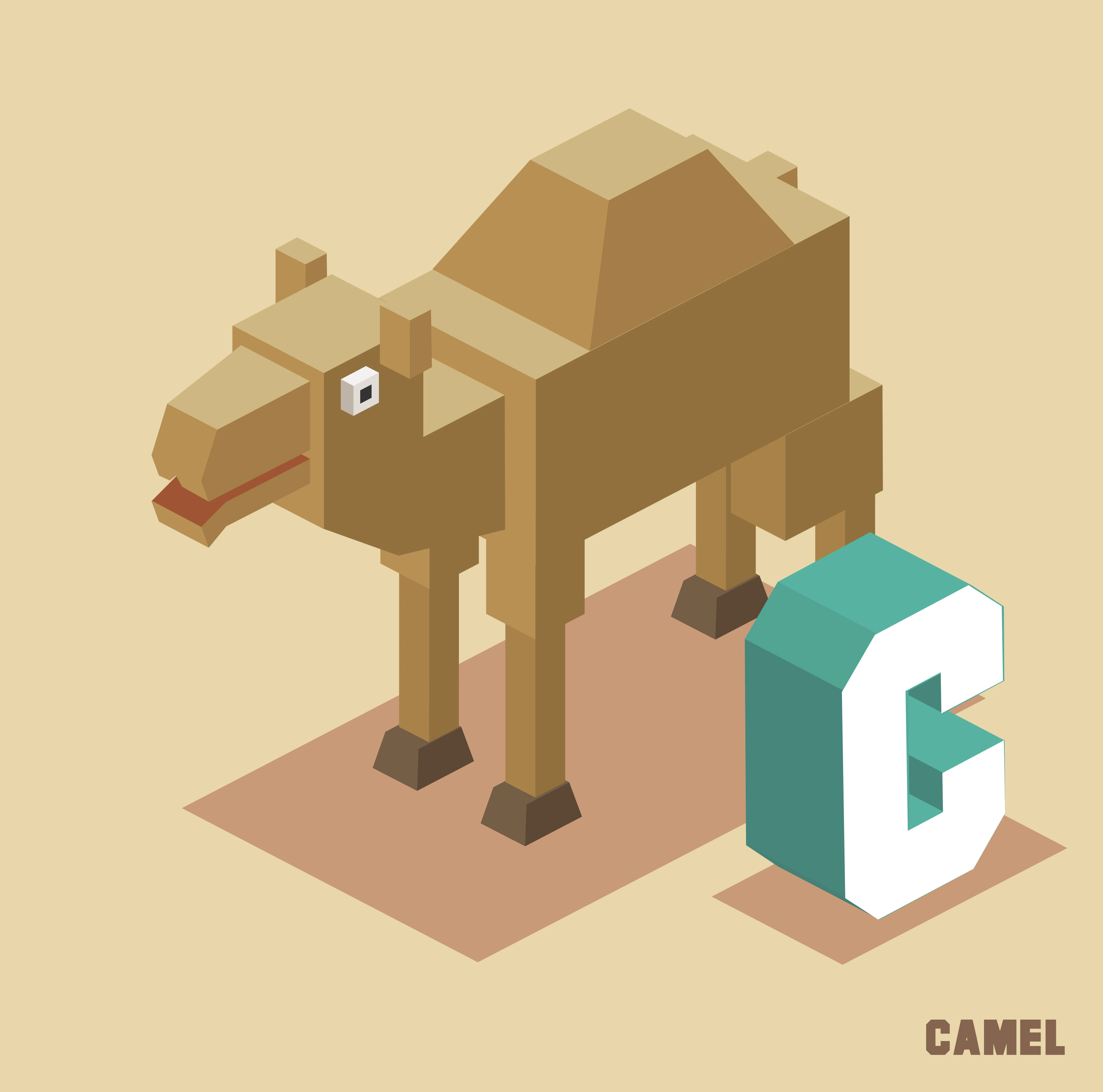
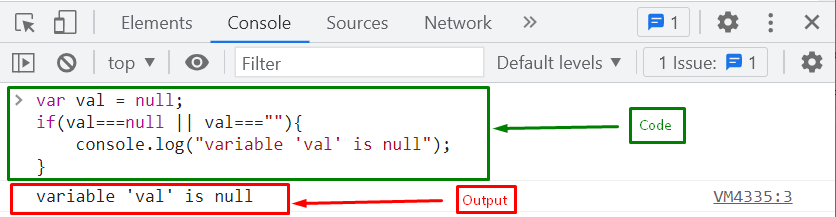

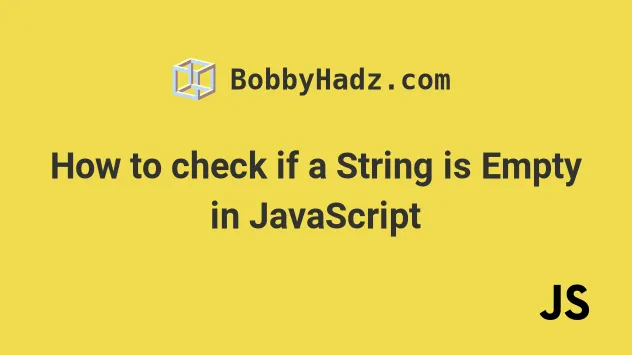
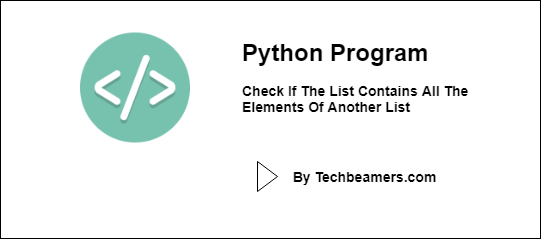
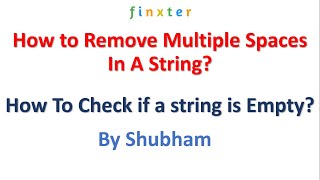
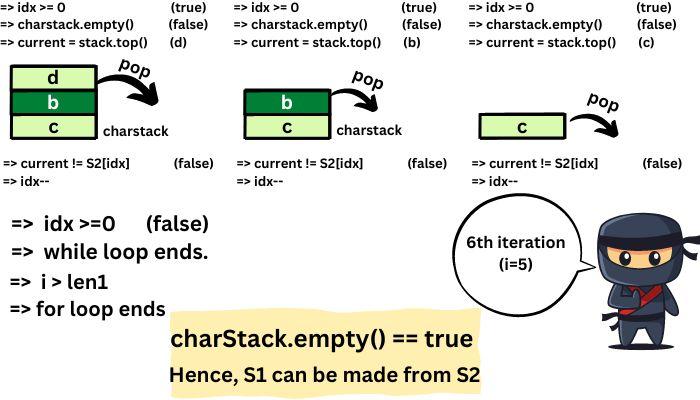
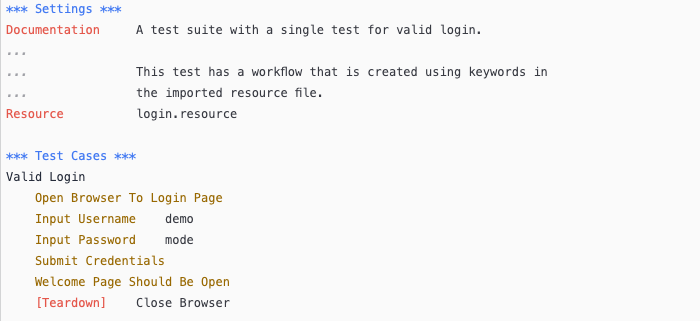
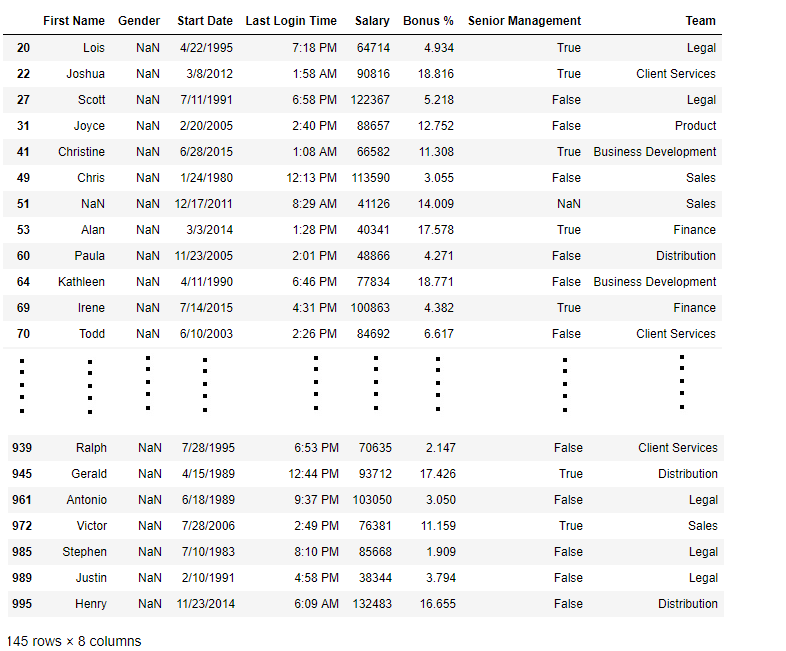


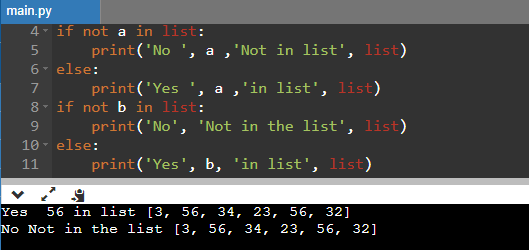
Article link: check if string empty python.
Learn more about the topic check if string empty python.
- How to check if the string is empty? – Stack Overflow
- Python Program to Check if String is Empty or Not
- How to Check if a String is Empty in Python – Datagy
- Here is how to check if a string is empty in Python
- Java String isEmpty() Method – W3Schools
- Check if String is Empty or Not in Python – Spark By {Examples}
- How to Check if a String is Empty or None in Python
- Here is how to check if a string is empty in Python
- How to Check if a String is Empty in Python – Javatpoint
- Program to Check if String is Empty in Python – Scaler Topics
- Python: Check if String is Empty – STechies
- How To Check if a string is Empty in Python? – Finxter