Check If Key In Dict Python
In Python, dictionaries are commonly used data structures that store key-value pairs. It is often necessary to check if a specific key exists in a dictionary before performing certain operations. Luckily, Python provides several methods to accomplish this task. In this article, we will explore these methods in detail and also discuss related topics such as checking if a key does not exist, accessing key-value pairs, checking values, changing values, and more.
Using the ‘in’ Operator
The simplest and most common way to check if a key exists in a dictionary is to use the ‘in’ operator. This operator returns True if the key is present in the dictionary, and False otherwise. Here is an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
if ‘age’ in my_dict:
print(“Key ‘age’ exists”)
else:
print(“Key ‘age’ does not exist”)
# Output: Key ‘age’ exists
“`
Using the ‘not in’ Operator
Similar to the ‘in’ operator, the ‘not in’ operator can be used to check if a key does not exist in a dictionary. This operator returns True if the key is not present in the dictionary, and False otherwise. Here is an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
if ‘height’ not in my_dict:
print(“Key ‘height’ does not exist”)
else:
print(“Key ‘height’ exists”)
# Output: Key ‘height’ does not exist
“`
Using the get() Method
The get() method is another way to check if a key exists in a dictionary. This method returns the value associated with the key if it exists, and a default value (which is None by default) if the key does not exist. We can take advantage of this behavior to check the existence of a key. Here is an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
age = my_dict.get(‘age’)
if age is not None:
print(“Key ‘age’ exists”)
else:
print(“Key ‘age’ does not exist”)
# Output: Key ‘age’ exists
“`
Using the keys() Method
The keys() method returns a view object that contains all the keys in a dictionary. We can convert this view object to a list and then use the ‘in’ operator to check if a key exists. Here is an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
keys_list = list(my_dict.keys())
if ‘city’ in keys_list:
print(“Key ‘city’ exists”)
else:
print(“Key ‘city’ does not exist”)
# Output: Key ‘city’ exists
“`
Using the has_key() Method (Deprecated)
The has_key() method was available in older versions of Python but has been deprecated since version 2.3. It is not recommended to use this method in modern Python code.
Using the try-except Block
We can also check if a key exists in a dictionary by using a try-except block. We can try to access the value associated with the key, and if an exception is raised, it means the key does not exist. Here is an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
try:
age = my_dict[‘age’]
print(“Key ‘age’ exists”)
except KeyError:
print(“Key ‘age’ does not exist”)
# Output: Key ‘age’ exists
“`
Using the __contains__() Method
The __contains__() method allows us to define our own logic for checking the existence of a key in a dictionary. By default, it uses the ‘in’ operator to perform the check. Here is an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
if my_dict.__contains__(‘age’):
print(“Key ‘age’ exists”)
else:
print(“Key ‘age’ does not exist”)
# Output: Key ‘age’ exists
“`
Using the if-else Statement
We can also utilize the if-else statement along with the ‘in’ operator to check if a key exists in a dictionary. Here is an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
if ‘age’ in my_dict.keys():
print(“Key ‘age’ exists”)
else:
print(“Key ‘age’ does not exist”)
# Output: Key ‘age’ exists
“`
Using the any() Function
The any() function returns True if at least one element in the iterable is true, and False otherwise. We can apply this function to the result of a generator expression that checks if a key exists in a dictionary. Here is an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
if any(key == ‘age’ for key in my_dict.keys()):
print(“Key ‘age’ exists”)
else:
print(“Key ‘age’ does not exist”)
# Output: Key ‘age’ exists
“`
Using the all() Function
The all() function returns True if all elements in the iterable are true, and False otherwise. We can apply this function to the result of a generator expression that checks if a key exists in a dictionary. Here is an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
if all(key != ‘height’ for key in my_dict.keys()):
print(“Key ‘height’ does not exist”)
else:
print(“Key ‘height’ exists”)
# Output: Key ‘height’ does not exist
“`
Check if Key not Exists in Dictionary Python
To check if a key does not exist in a dictionary, we can use the ‘not in’ operator. This operator returns True if the key is not present in the dictionary, and False otherwise. Here is an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
if ‘height’ not in my_dict:
print(“Key ‘height’ does not exist”)
else:
print(“Key ‘height’ exists”)
# Output: Key ‘height’ does not exist
“`
For Key-Value in Dict Python
To iterate over the key-value pairs in a dictionary, we can use a for loop. The items() method returns a view object that contains the key-value pairs, which can be looped over. Here is an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
for key, value in my_dict.items():
print(key, value)
# Output:
# name John
# age 25
# city New York
“`
Check Value in Dictionary Python
To check if a value exists in a dictionary, we can use the ‘in’ operator along with the values() method. The values() method returns a view object that contains all the values in a dictionary. Here is an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
if ‘John’ in my_dict.values():
print(“Value ‘John’ exists”)
else:
print(“Value ‘John’ does not exist”)
# Output: Value ‘John’ exists
“`
Change Value in Dictionary Python
To change the value associated with a key in a dictionary, we can simply assign a new value to that key. Here is an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
my_dict[‘age’] = 30
print(my_dict)
# Output: {‘name’: ‘John’, ‘age’: 30, ‘city’: ‘New York’}
“`
How to Check if Value Exists in Python
To check if a value exists in a list, tuple, or dictionary in Python, we can use the ‘in’ operator. This operator returns True if the value is present in the collection, and False otherwise. Here are some examples:
“`python
my_list = [1, 2, 3, 4, 5]
if 3 in my_list:
print(“Value 3 exists”)
else:
print(“Value 3 does not exist”)
# Output: Value 3 exists
my_tuple = (1, 2, 3, 4, 5)
if 6 in my_tuple:
print(“Value 6 exists”)
else:
print(“Value 6 does not exist”)
# Output: Value 6 does not exist
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
if ‘John’ in my_dict.values():
print(“Value ‘John’ exists”)
else:
print(“Value ‘John’ does not exist”)
# Output: Value ‘John’ exists
“`
Python Check Key in Array
To check if a key exists in an array in Python, we can use the ‘in’ operator. This operator returns True if the key is present in the array, and False otherwise. Here is an example:
“`python
my_array = [‘name’, ‘age’, ‘city’]
if ‘age’ in my_array:
print(“Key ‘age’ exists”)
else:
print(“Key ‘age’ does not exist”)
# Output: Key ‘age’ exists
“`
Add Value to Key Dictionary Python
To add a value to a key in a dictionary, we can simply assign a value to that key. If the key already exists, the value will be updated. Otherwise, a new key-value pair will be added. Here is an example:
“`python
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
my_dict[‘height’] = 180
print(my_dict)
# Output: {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’, ‘height’: 180}
“`
Order Dict Python
In Python versions prior to 3.7, dictionaries are unordered. This means that the order in which key-value pairs are stored may vary. However, starting from Python 3.7, dictionaries are guaranteed to maintain the insertion order of the keys. If you want to maintain a specific order in a dictionary, you can use the OrderedDict class from the collections module. Here is an example:
“`python
from collections import OrderedDict
my_dict = OrderedDict()
my_dict[‘name’] = ‘John’
my_dict[‘age’] = 25
my_dict[‘city’] = ‘New York’
print(my_dict)
# Output: OrderedDict([(‘name’, ‘John’), (‘age’, 25), (‘city’, ‘New York’)])
“`
FAQs
Q: What is the most common method to check if a key exists in a Python dictionary?
A: The most common method is to use the ‘in’ operator. It returns True if the key is present in the dictionary, and False otherwise.
Q: How to check if a key does not exist in a dictionary?
A: To check if a key does not exist in a dictionary, you can use the ‘not in’ operator. It returns True if the key is not present in the dictionary, and False otherwise.
Q: How can I change the value associated with a key in a dictionary?
A: To change the value associated with a key in a dictionary, you can simply assign a new value to that key.
Q: How can I check if a value exists in a list, tuple, or dictionary in Python?
A: To check if a value exists in a list, tuple, or dictionary in Python, you can use the ‘in’ operator. It returns True if the value is present in the collection, and False otherwise.
Q: Are dictionaries in Python ordered?
A: In Python versions prior to 3.7, dictionaries are unordered. Starting from Python 3.7, dictionaries are guaranteed to maintain the insertion order of the keys.
How To Check If A Key Is In A Dictionary In Python
Keywords searched by users: check if key in dict python Check if key not exists in dictionary Python, For key-value in dict Python, Check value in dictionary Python, Change value in dictionary Python, How to check if value exists in python, Python check key in array, Add value to key dictionary Python, Order dict Python
Categories: Top 13 Check If Key In Dict Python
See more here: nhanvietluanvan.com
Check If Key Not Exists In Dictionary Python
Python dictionaries are versatile data structures used to store key-value pairs. These pairs allow for efficient and fast data retrieval based on a specific key. However, when working with dictionaries in Python, it is often necessary to check if a particular key does not exist in the dictionary. In this article, we will explore various ways to perform this task and discuss their advantages and disadvantages.
Checking if a key does not exist in a dictionary can be accomplished using the `in` keyword. This keyword allows us to check if a specific key is present in the dictionary or not. If the key is not present, it returns `False`; otherwise, it returns `True`. Here’s an example to illustrate this:
“`python
my_dict = {“apple”: 1, “banana”: 2, “orange”: 3}
if “mango” not in my_dict:
print(“Key does not exist in the dictionary.”)
“`
In this example, the key “mango” is not present in the dictionary `my_dict`, so the corresponding message “Key does not exist in the dictionary.” is printed.
Another way to check if a key does not exist in a dictionary is by using the `get()` method. This method returns the value associated with the given key if it exists in the dictionary. However, if the key does not exist, it returns a default value (which can be specified as an argument). By using this method, we can easily determine whether a key exists or not. Here’s an example:
“`python
my_dict = {“apple”: 1, “banana”: 2, “orange”: 3}
if my_dict.get(“mango”) is None:
print(“Key does not exist in the dictionary.”)
“`
In this example, the `get()` method is used to retrieve the value associated with the key “mango”. Since this key does not exist, `None` is returned, and the corresponding message is printed.
One important thing to note is that using the `in` keyword is generally more efficient than using the `get()` method, especially when the dictionary is large. The `in` keyword performs a direct lookup of the key, which has a time complexity of O(1) on average. On the other hand, the `get()` method performs a lookup and returns the value if found, which has a time complexity of O(1) as well.
Additionally, we can make use of the `try-except` block to handle the case when a key does not exist in a dictionary. By trying to access the dictionary with the given key and catching the `KeyError` exception, we can determine whether the key exists or not. Here’s an example:
“`python
my_dict = {“apple”: 1, “banana”: 2, “orange”: 3}
try:
value = my_dict[“mango”]
except KeyError:
print(“Key does not exist in the dictionary.”)
“`
In this example, the `try` block attempts to access the dictionary using the key “mango”. Since this key does not exist, a `KeyError` is raised, and the corresponding message is printed.
Lastly, it is also possible to use the `not` operator along with the `in` keyword to check if a key does not exist in a dictionary. Here’s an example:
“`python
my_dict = {“apple”: 1, “banana”: 2, “orange”: 3}
if not “mango” in my_dict:
print(“Key does not exist in the dictionary.”)
“`
This example achieves the same result as the previous examples, but with a slightly different syntax.
In summary, there are several ways to check if a key does not exist in a dictionary in Python. The `in` keyword, the `get()` method, the `try-except` block, and the combination of the `not` operator with the `in` keyword all provide effective means of performing this task.
—
FAQs
Q1. How do I check if a key exists in a dictionary?
A1. You can use the `in` keyword or the `get()` method to check if a key exists in a dictionary.
Q2. What is the difference between using the `in` keyword and the `get()` method?
A2. The `in` keyword directly checks if a key exists in a dictionary and returns a boolean value. The `get()` method checks if a key exists and returns the value associated with it if found.
Q3. Which method is more efficient for checking if a key does not exist in a dictionary?
A3. Using the `in` keyword is generally more efficient as it performs a direct lookup of the key with a time complexity of O(1) on average.
Q4. Can I use the `try-except` block to check if a key exists in a dictionary?
A4. Yes, you can use the `try-except` block to handle the `KeyError` exception when trying to access a key that does not exist in a dictionary.
Q5. Is there any difference between `None` and `KeyError` when checking if a key does not exist?
A5. `None` is returned by the `get()` method when a key does not exist, while a `KeyError` is raised when trying to access a non-existent key directly. Both can be used to determine if a key does not exist.
For Key-Value In Dict Python
Python, being a versatile and powerful programming language, provides numerous built-in data structures for effective manipulation and storage of data. One such data structure is a dictionary, also known as “dict” in Python. The dict data type allows us to store data in the form of key-value pairs, providing fast and efficient access based on the provided keys. In this article, we will delve into the concept of key-value pairs in dict and explore various operations that can be performed on them.
Understanding Key-Value Pairs in Dict
A key-value pair in a dictionary refers to a combination of two elements: a unique key and its corresponding value. The key acts as an identifier for the value and plays a crucial role in accessing and manipulating the associated data. In Python, keys within a dictionary must be unique, while values can be either duplicates or distinct.
Creating a Dictionary
To create a dictionary, we enclose key-value pairs within curly braces ‘{‘ and ‘}’. The key-value pairs are separated by colons ‘:’ and individual elements are separated by commas ‘,’. Here’s an example that demonstrates the creation of a dictionary:
“`
my_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
“`
In this example, we have created a dict called `my_dict` with three key-value pairs. The keys are ‘name’, ‘age’, and ‘city’, while the corresponding values are ‘John’, 25, and ‘New York’.
Accessing Values in a Dictionary
Once a dictionary is created, accessing values becomes a simple task. We can retrieve the value associated with a particular key by using the key as an index within square brackets ‘[ ]’. Check out the following example:
“`
print(my_dict[‘name’]) # Output: John
“`
In this case, the value ‘John’ is accessed by providing the ‘name’ key. Similarly, other values can be accessed by providing their respective keys.
Modifying Values in a Dictionary
Python allows us to update the values in a dictionary by reassigning new values to the existing keys. Here’s how it can be done:
“`
my_dict[‘age’] = 30
print(my_dict) # Output: {‘name’: ‘John’, ‘age’: 30, ‘city’: ‘New York’}
“`
In this example, the value associated with the key ‘age’ is modified from 25 to 30. By overwriting the old value, the dictionary is updated to reflect the new value.
Iterating Over a Dictionary
Python provides several methods to iterate over the contents of a dictionary. We can use either the keys, values, or both to accomplish this. Consider the following code snippet:
“`
for key in my_dict:
print(key, my_dict[key])
“`
This loop iterates over each key in the dictionary and prints both the key and its corresponding value. If we only want to iterate over the values, we can use the `.values()` method:
“`
for value in my_dict.values():
print(value)
“`
Similarly, if we only want to iterate over the keys, we can use the `.keys()` method:
“`
for key in my_dict.keys():
print(key)
“`
Deleting an Element from a Dictionary
Python allows us to delete a key-value pair from a dictionary using the `del` keyword. Here’s an example that demonstrates its usage:
“`
del my_dict[‘age’]
print(my_dict) # Output: {‘name’: ‘John’, ‘city’: ‘New York’}
“`
In this case, the key-value pair with the key ‘age’ is removed from the dictionary, resulting in an updated dictionary.
FAQs:
Q: Can keys in a dictionary be of any data type?
A: In Python, dictionary keys can be of any immutable data type. This includes strings, integers, floats, and tuples. However, mutable data types like lists and dictionaries cannot be used as keys.
Q: Can a dictionary have duplicate values?
A: Yes, a dictionary can have duplicate values. While the keys must be unique, the values can be repeated.
Q: How can I check if a key exists in a dictionary?
A: Python provides the `in` and `not in` operators to check the existence of a key within a dictionary. For example, `if ‘name’ in my_dict` will return `True` if the key ‘name’ is present in `my_dict`.
Q: Can I sort a dictionary based on its keys or values?
A: Yes, dictionaries can be sorted using the `sorted()` function or the `sorted()` method along with the `key` parameter. For example, `sorted(my_dict.items(), key=lambda x: x[0])` will sort the dictionary based on its keys.
Q: Are dictionaries ordered in Python?
A: Starting from Python 3.7, dictionaries are guaranteed to maintain the order of insertion. This means that the order of keys and values will be preserved when iterating or accessing elements in a dictionary.
In conclusion, the key-value pairs in a dict in Python provide a flexible and efficient way to store and manipulate data. They allow for quick access based on unique keys, enabling a wide range of data processing operations. By understanding the fundamentals and various operations associated with key-value pairs, one can harness the power of dictionaries in Python programming effectively.
Check Value In Dictionary Python
In Python, dictionaries are a powerful data structure that allows you to store and retrieve values using keys. While working with dictionaries, it is often necessary to check if a particular value exists in the dictionary. In this article, we will explore different techniques and methods to check the value in a dictionary in Python.
Checking Value using the “in” Keyword:
The simplest way to check if a value exists in a dictionary is by using the “in” keyword. The “in” keyword allows you to check if a value is present in the dictionary or not. Here’s an example:
“`python
# Defining a dictionary
person = {
“name”: “John”,
“age”: 25,
“gender”: “male”
}
# Checking if a value exists
if “John” in person.values():
print(“Value found!”)
else:
print(“Value not found!”)
“`
In the above example, we have a dictionary called “person” with three key-value pairs. We check if the value “John” exists in the dictionary using the “in” keyword followed by the `values()` method. If the value is found, it prints “Value found!” otherwise it prints “Value not found!”.
Checking Value using `dict.get()` Method:
Python dictionaries provide a built-in method called `get()` which allows you to check if a value exists in the dictionary. The `get()` method takes two parameters: the key for which you want to retrieve the value, and an optional default value to be returned if the key is not found. Here’s an example:
“`python
# Checking if a value exists using get() method
if person.get(“name”) == “John”:
print(“Value found!”)
else:
print(“Value not found!”)
“`
In the above example, we use the `get()` method to retrieve the value associated with the key “name”. If the value is equal to “John”, it prints “Value found!” otherwise it prints “Value not found!”.
Using a Loop to Check Values:
Another way to check if a value exists in a dictionary is by iterating over the dictionary’s values using a loop. We can use a `for` loop to iterate through each value and check if it matches the desired value. Here’s an example:
“`python
# Defining a dictionary
fruits = {
“apple”: 10,
“banana”: 5,
“orange”: 2
}
# Checking if a value exists using a loop
value_to_check = 5
value_found = False
for value in fruits.values():
if value == value_to_check:
value_found = True
break
if value_found:
print(“Value found!”)
else:
print(“Value not found!”)
“`
In the above example, we define a dictionary called “fruits” with three key-value pairs. We iterate over each value using a `for` loop and check if it matches the desired value (in this case, 5). If a matching value is found, we set the `value_found` variable to True and break out of the loop. Finally, we check the value of `value_found` and print the appropriate message.
FAQs:
Q1. Is it possible to check if a value exists in a dictionary using the “in” keyword with keys?
A1. Yes, the “in” keyword can be used to check if a value exists in a dictionary by iterating over the keys instead of the values. Here’s an example:
“`python
if “name” in person.keys():
print(“Value found!”)
else:
print(“Value not found!”)
“`
Q2. What is the difference between using the “in” keyword and `dict.get()` method to check values in a dictionary?
A2. The “in” keyword checks if a value exists in a dictionary by searching through all the values, whereas the `get()` method checks if a value exists by providing the key. The `get()` method allows you to specify a default value if the key is not found, while the “in” keyword does not provide this functionality.
Q3. Can I use a combination of methods to check if a value exists in a dictionary?
A3. Yes, you can combine multiple methods to check if a value exists in a dictionary. For example, you can use the “in” keyword to check if the value is present in the dictionary’s values and then use `dict.get()` method to check if the value is present for a specific key.
In conclusion, checking the value in a dictionary is an essential task while working with Python dictionaries. In this article, we explored various techniques, including the “in” keyword, `dict.get()` method, and using loops to check if a value exists in a dictionary. Each method provides its own advantages and can be used depending on the requirements of the problem at hand.
Images related to the topic check if key in dict python
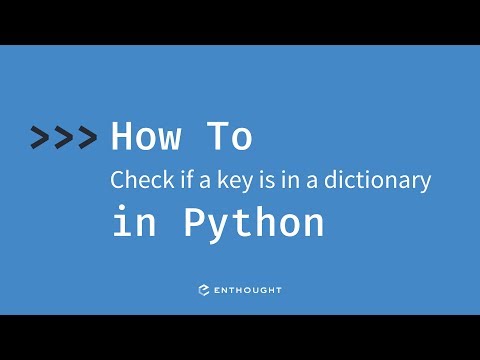
Found 6 images related to check if key in dict python theme

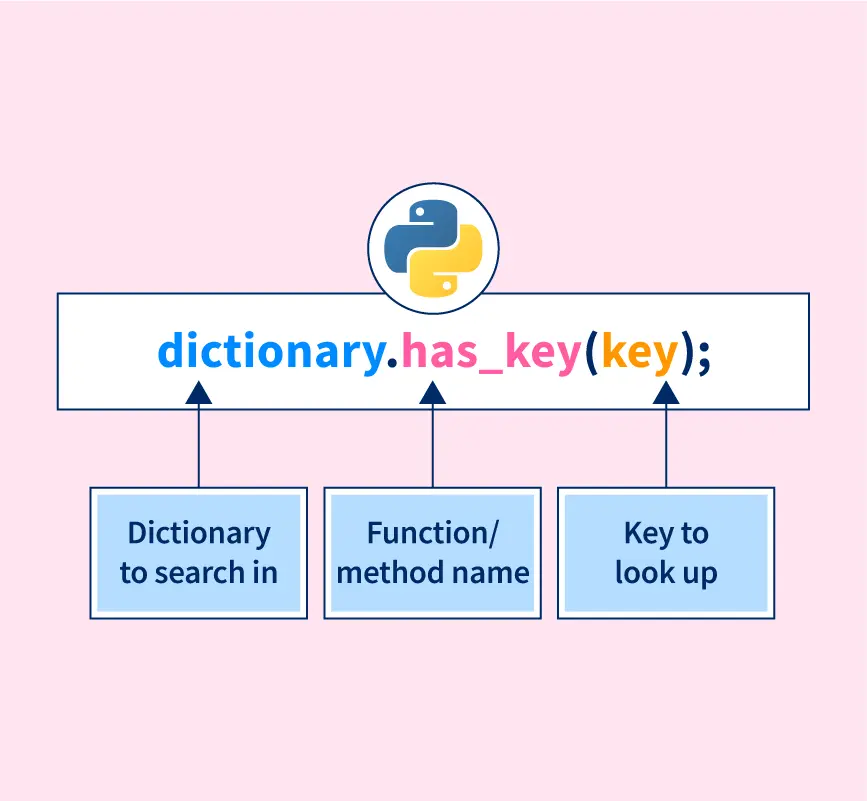

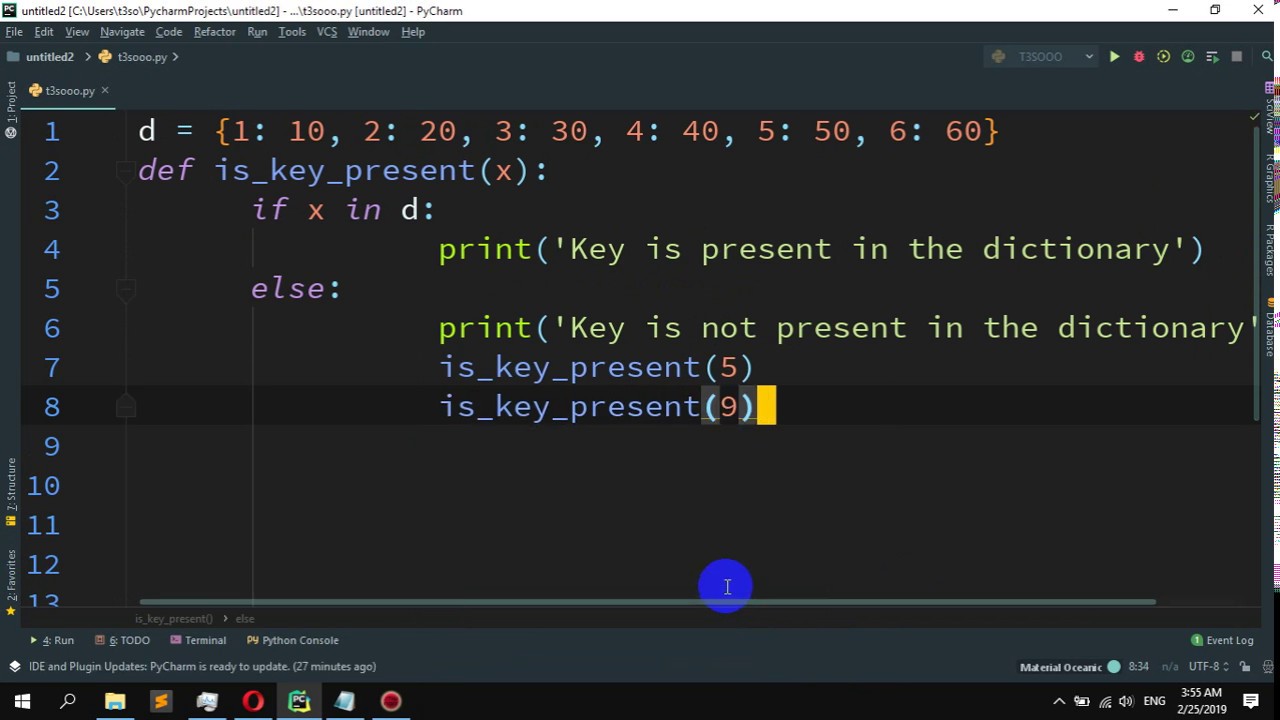
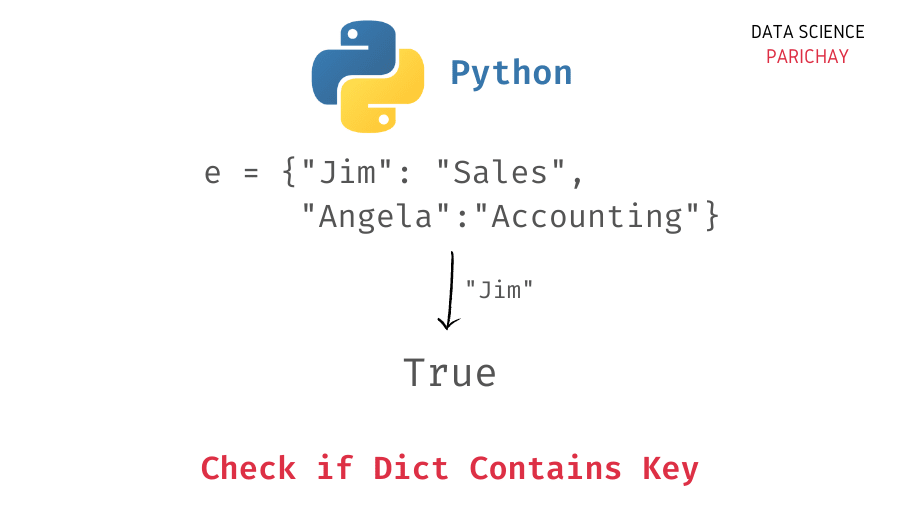
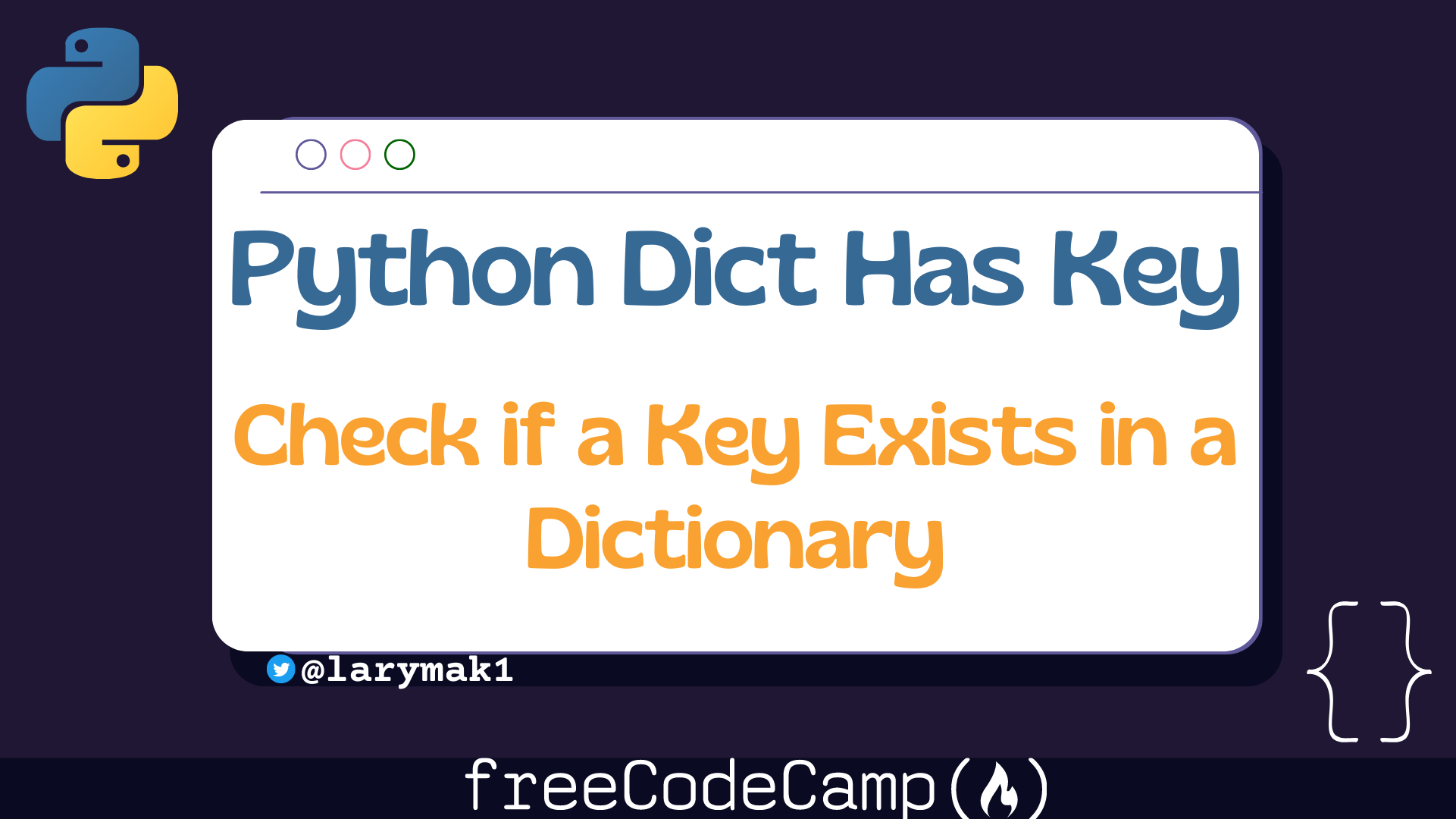
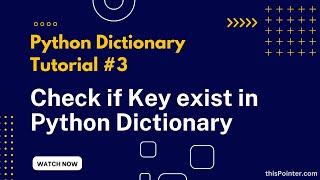
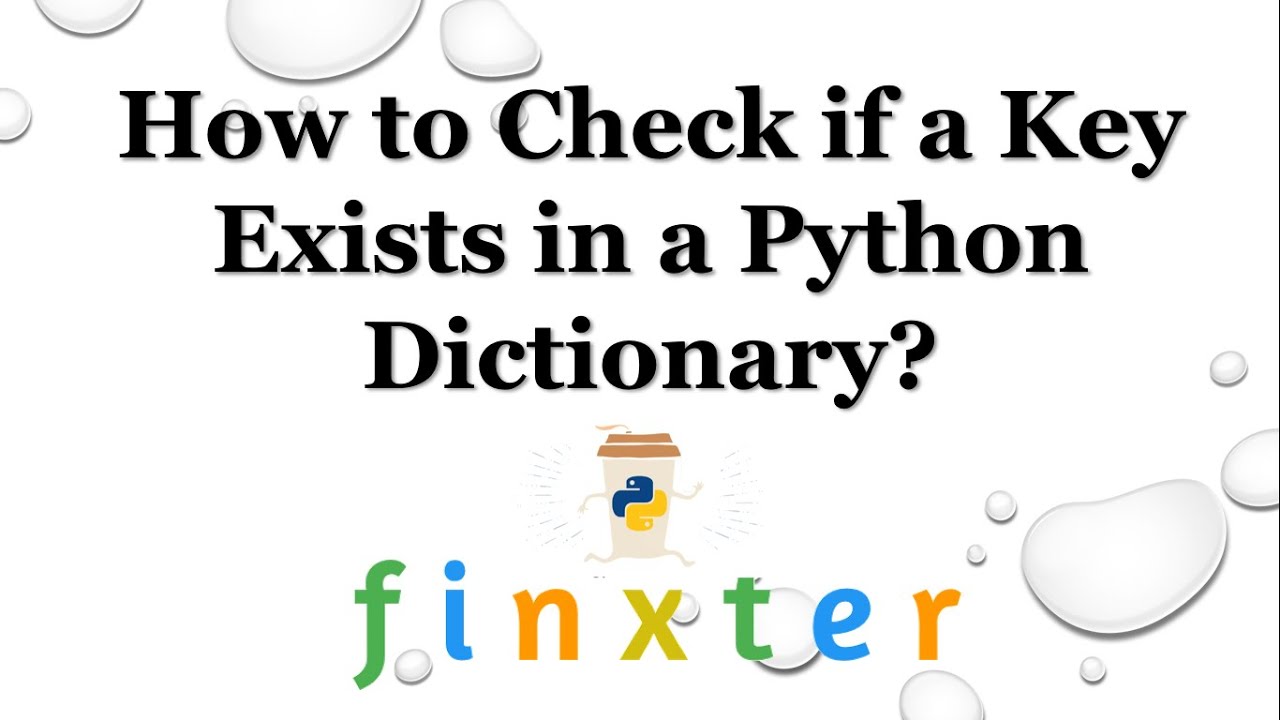
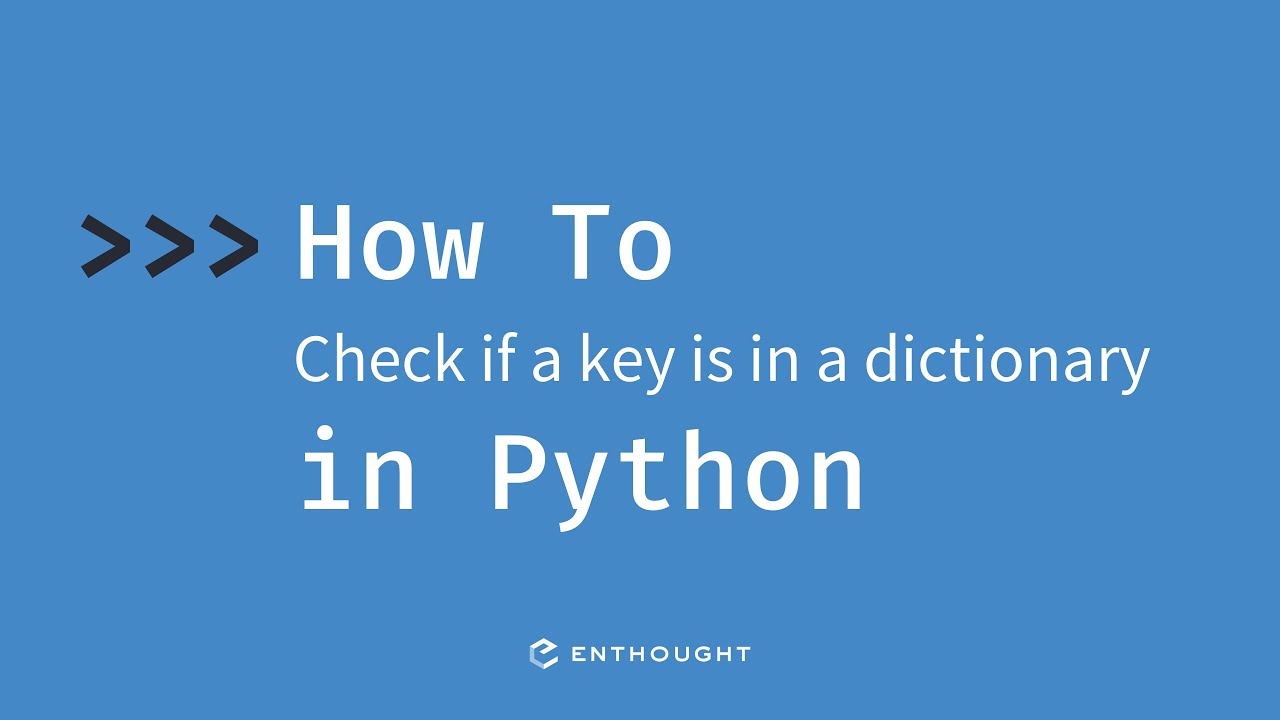
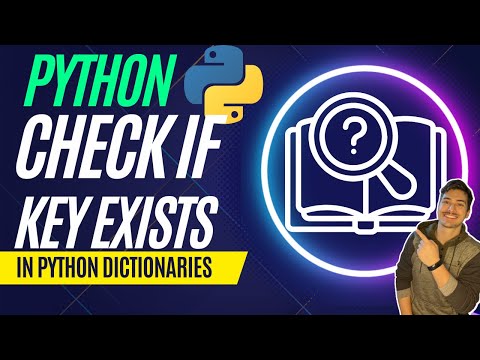





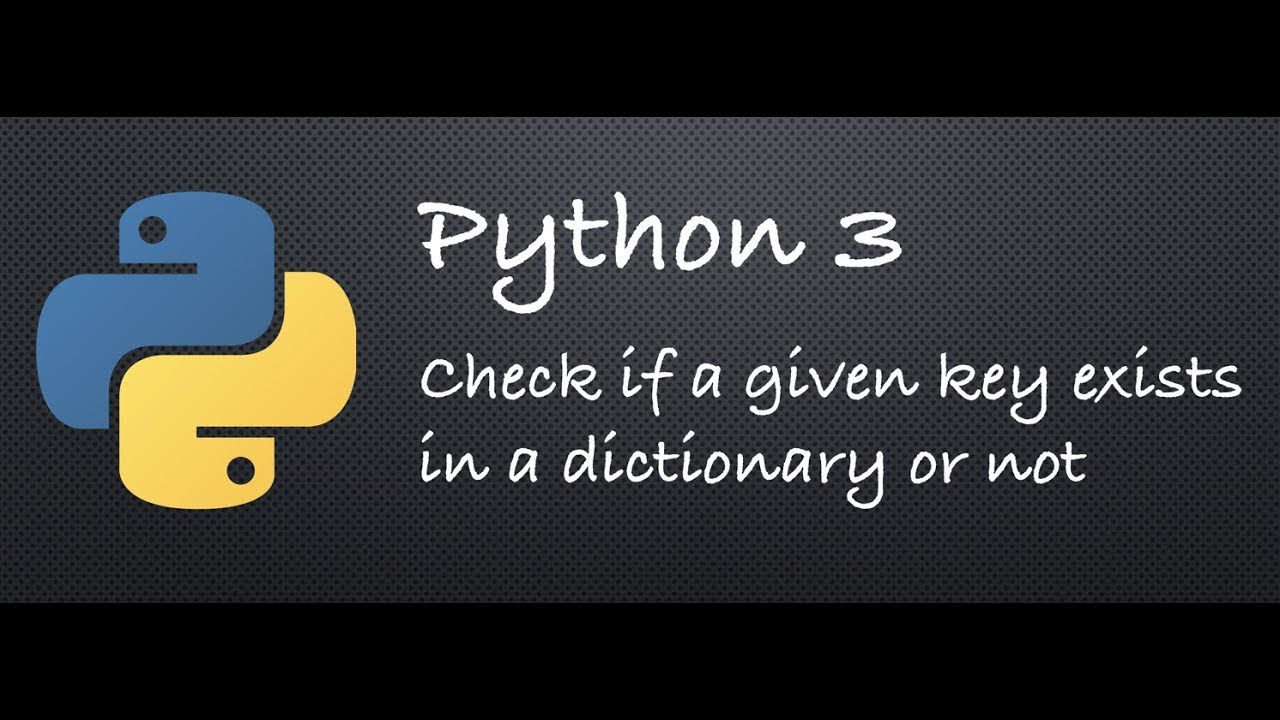
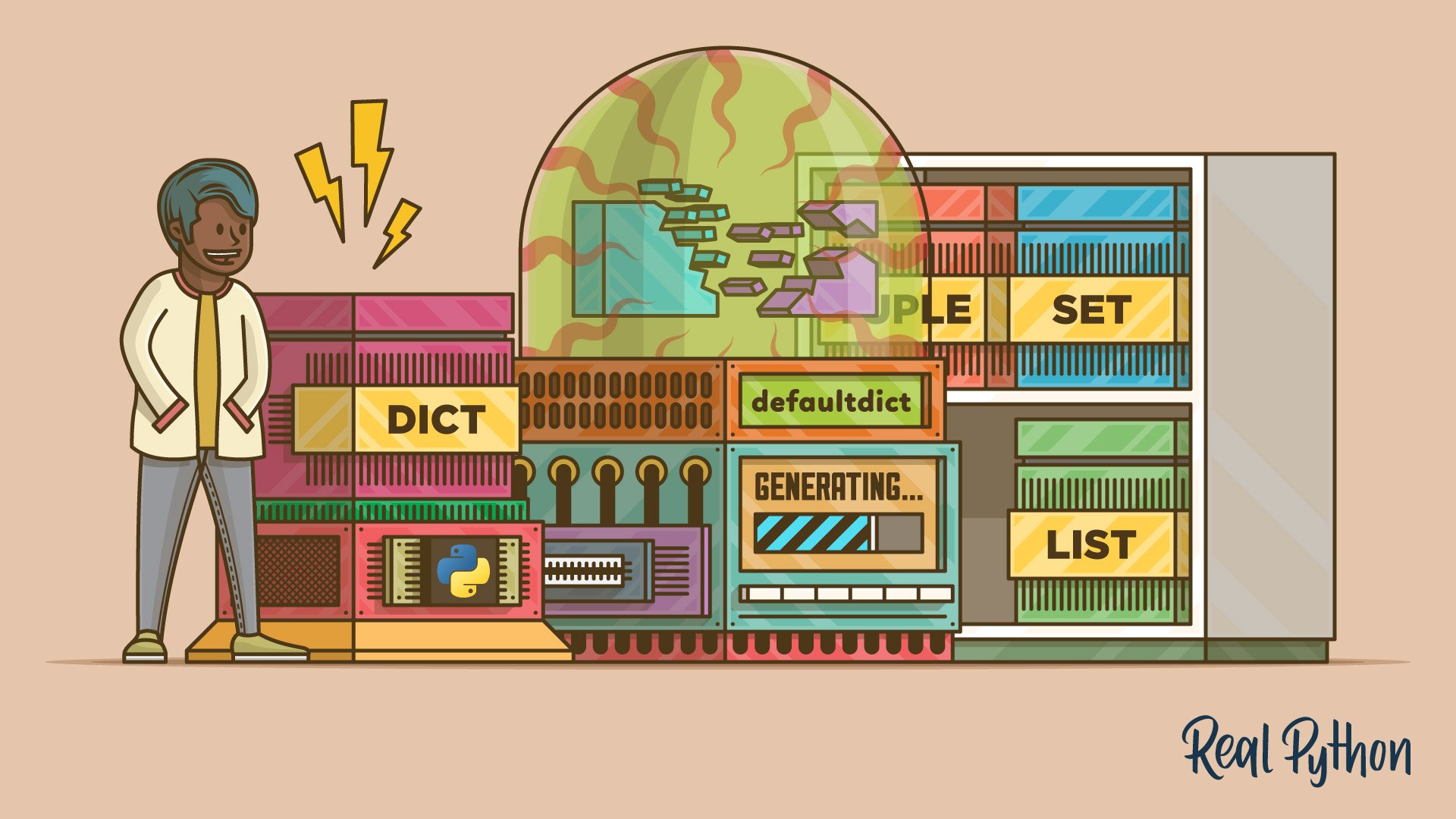
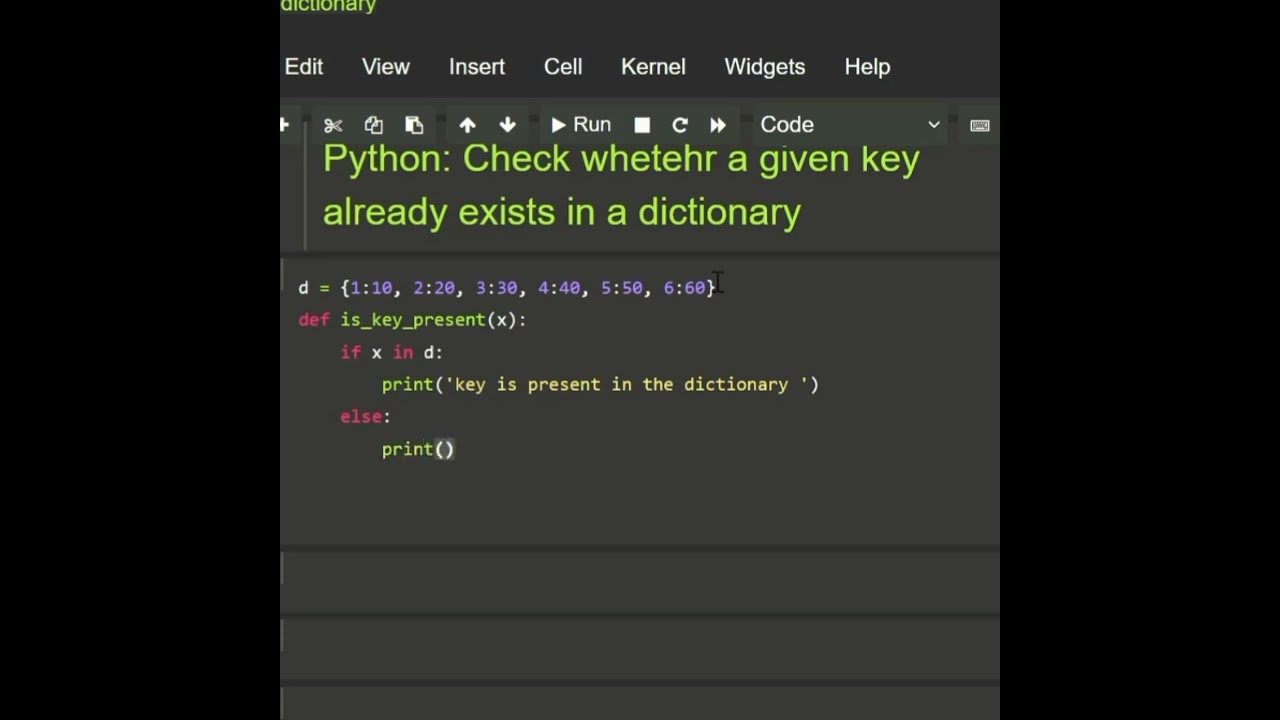
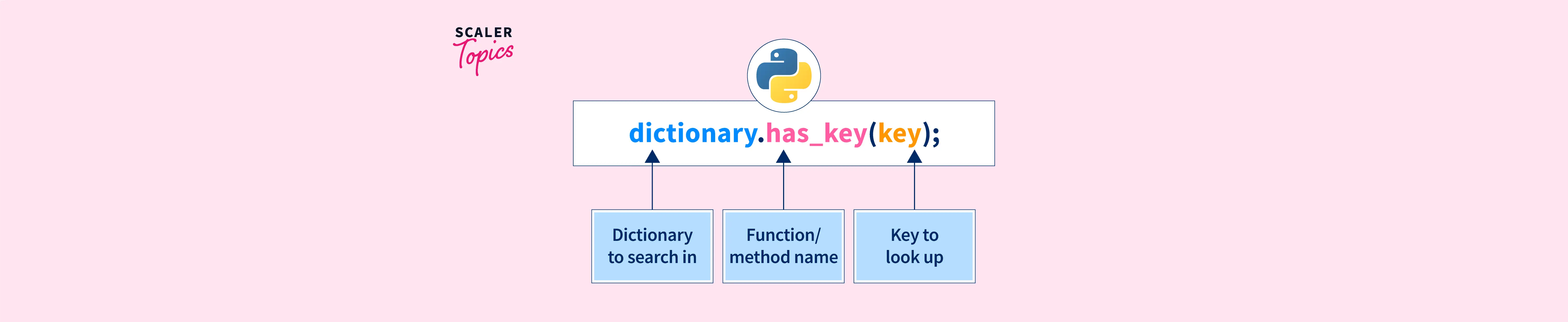
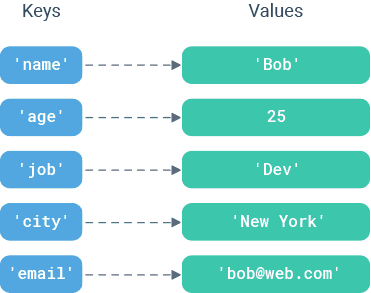
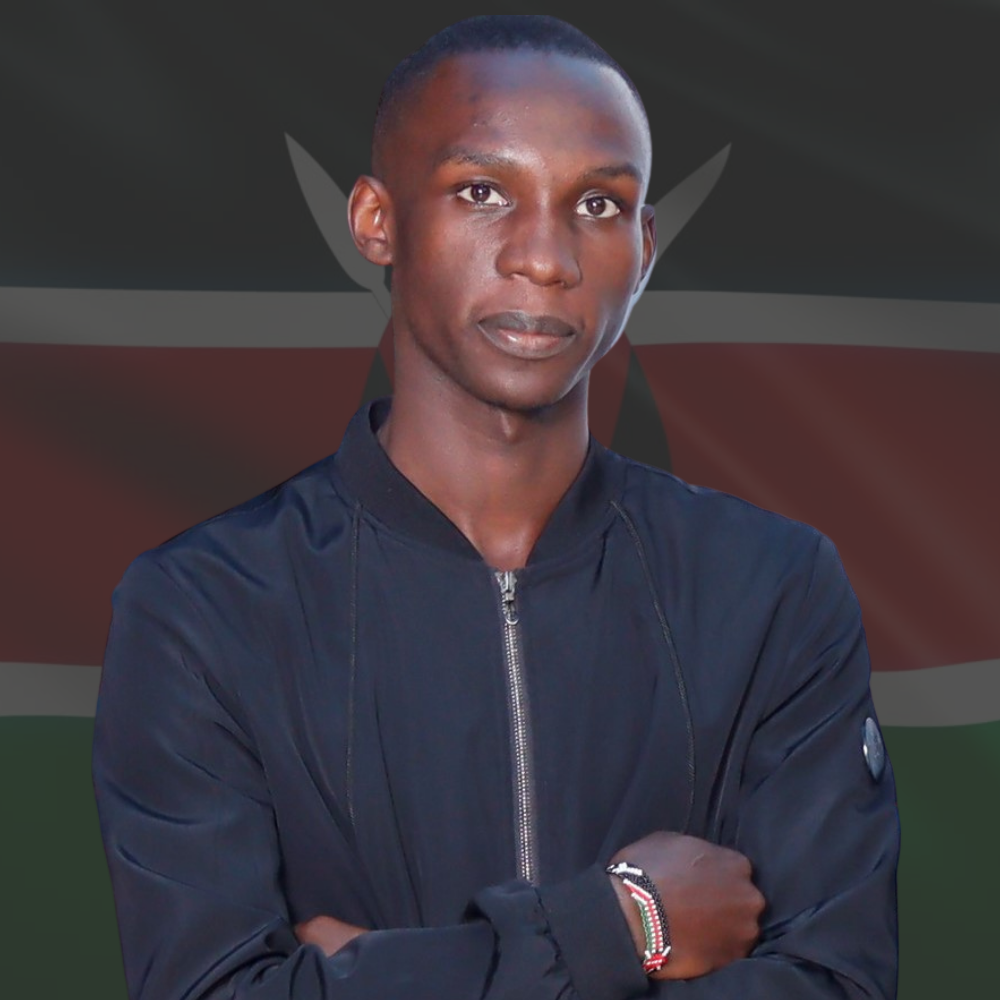

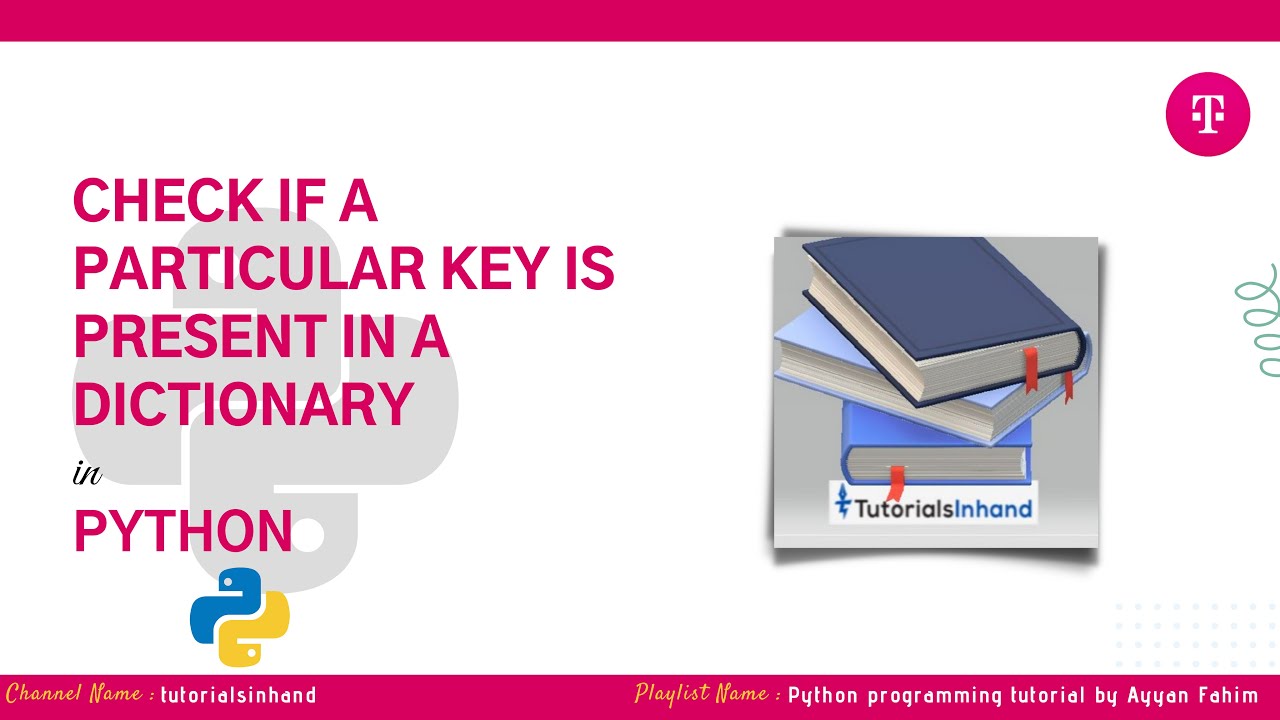

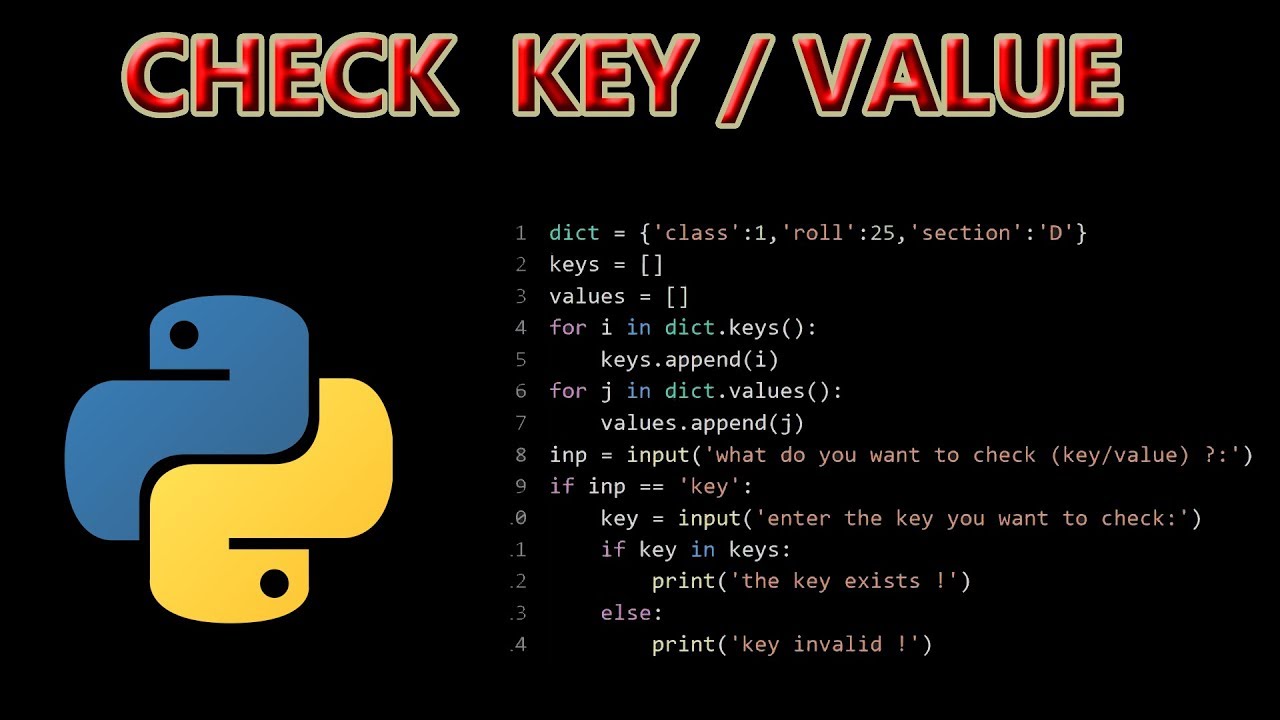



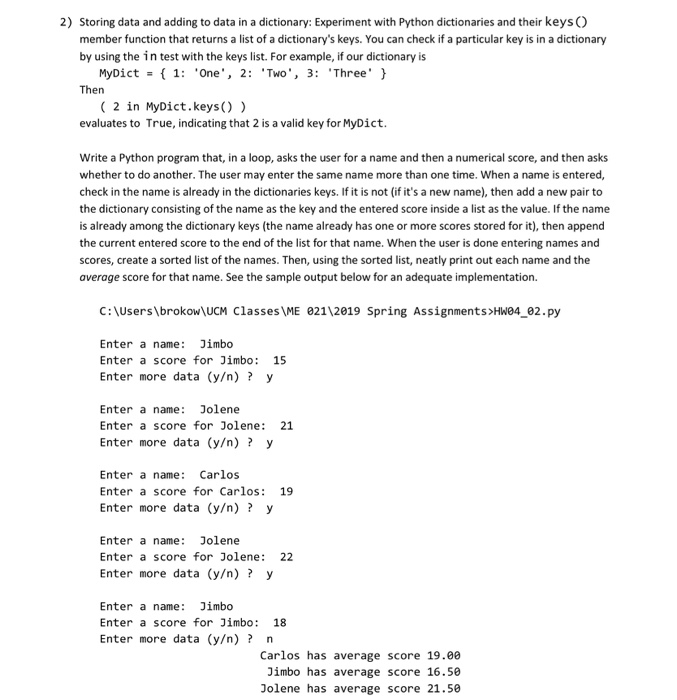
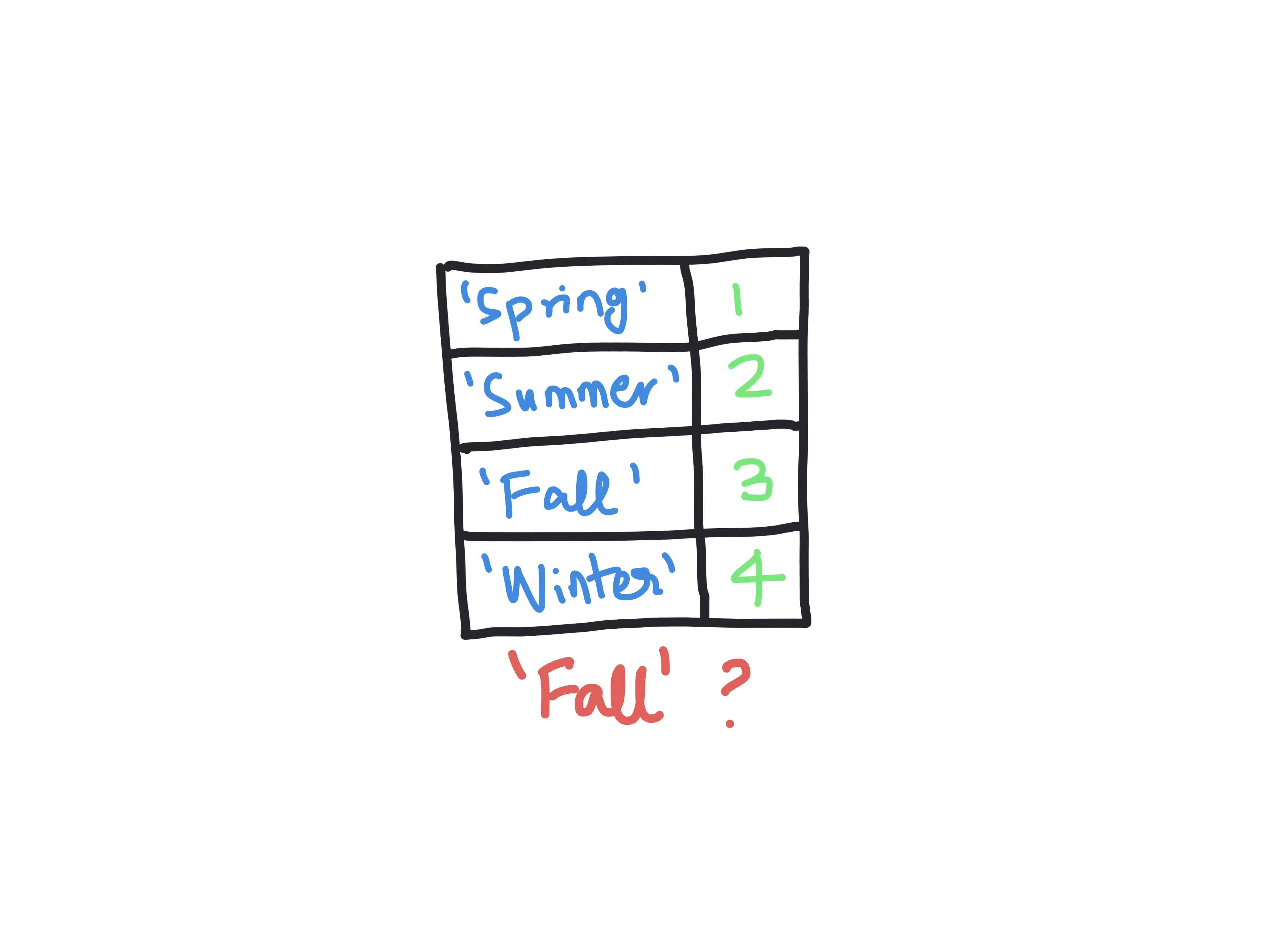


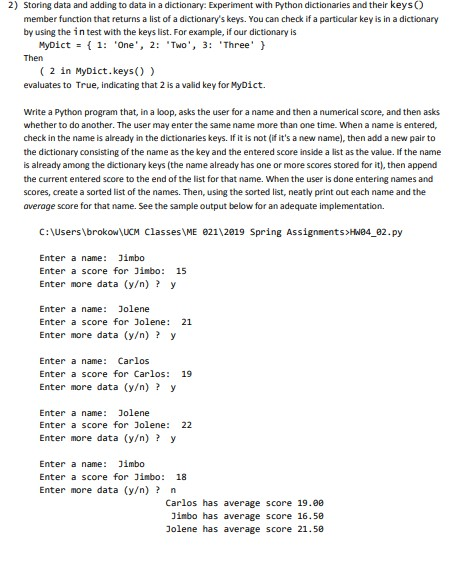
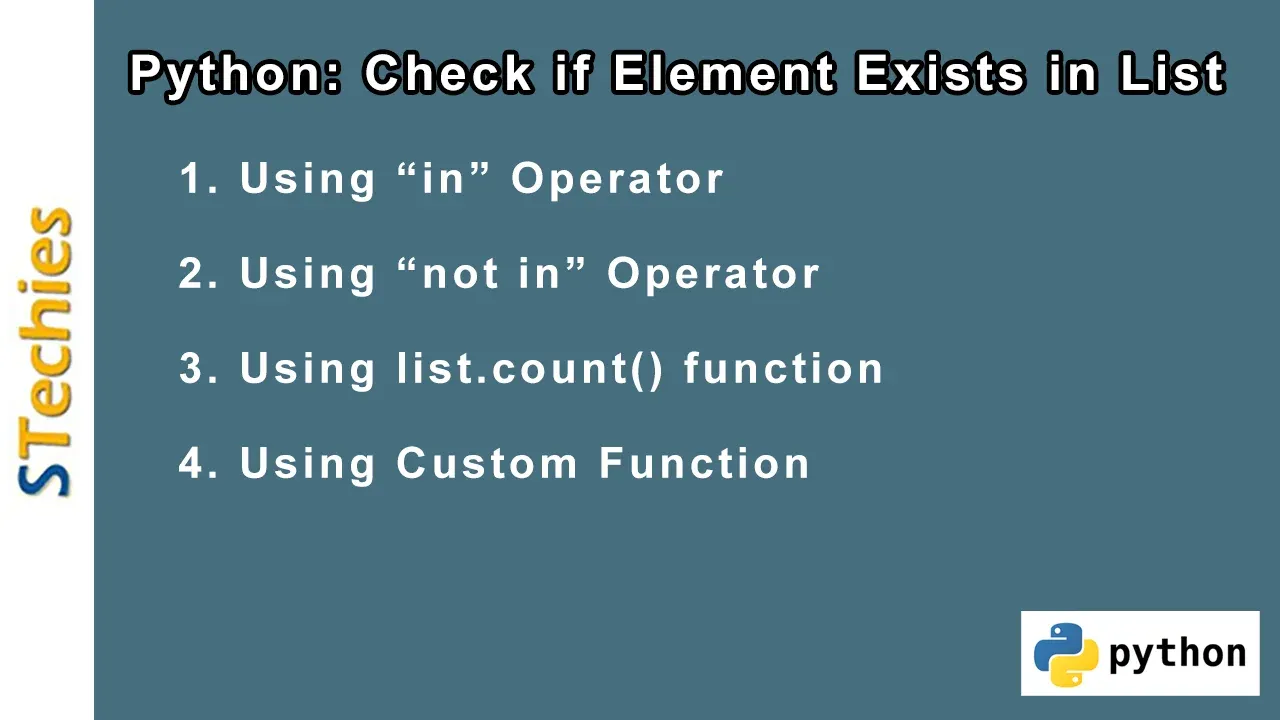
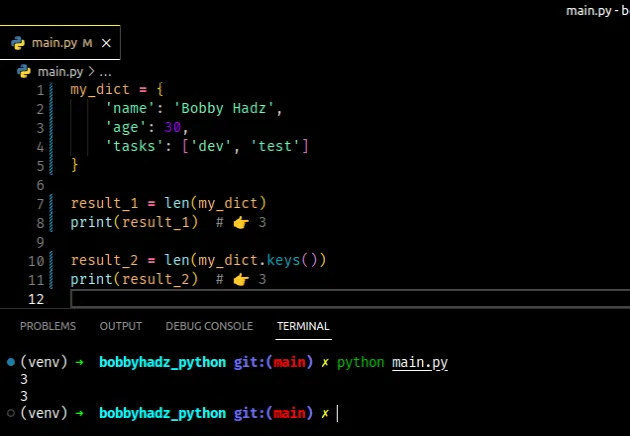
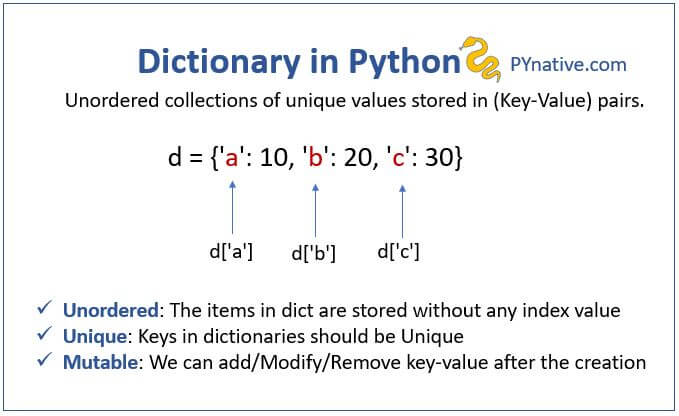
![Class 12] Julie has created a dictionary containing names and marks Class 12] Julie Has Created A Dictionary Containing Names And Marks](https://d1avenlh0i1xmr.cloudfront.net/374bed5d-d4bc-4d9f-b730-4c919131fae6/slide37.jpg)

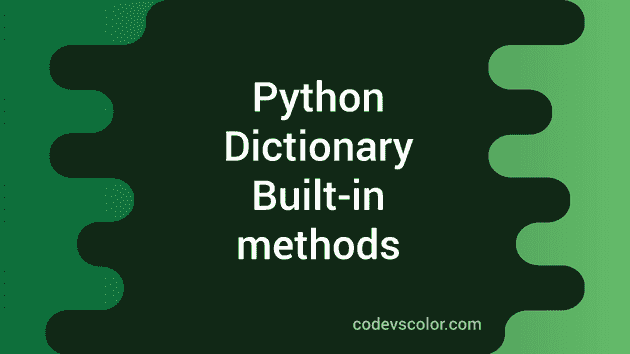
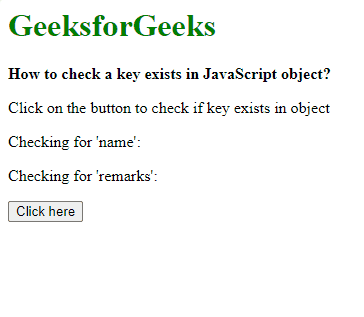
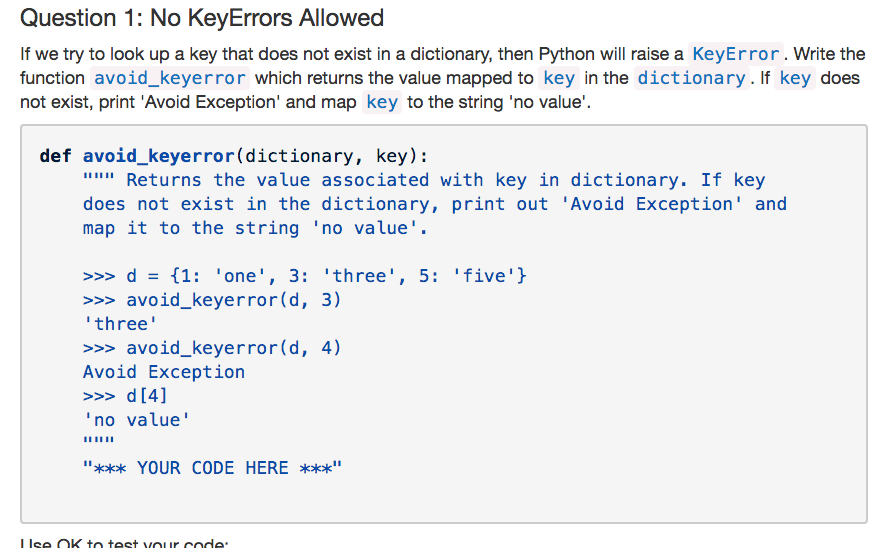
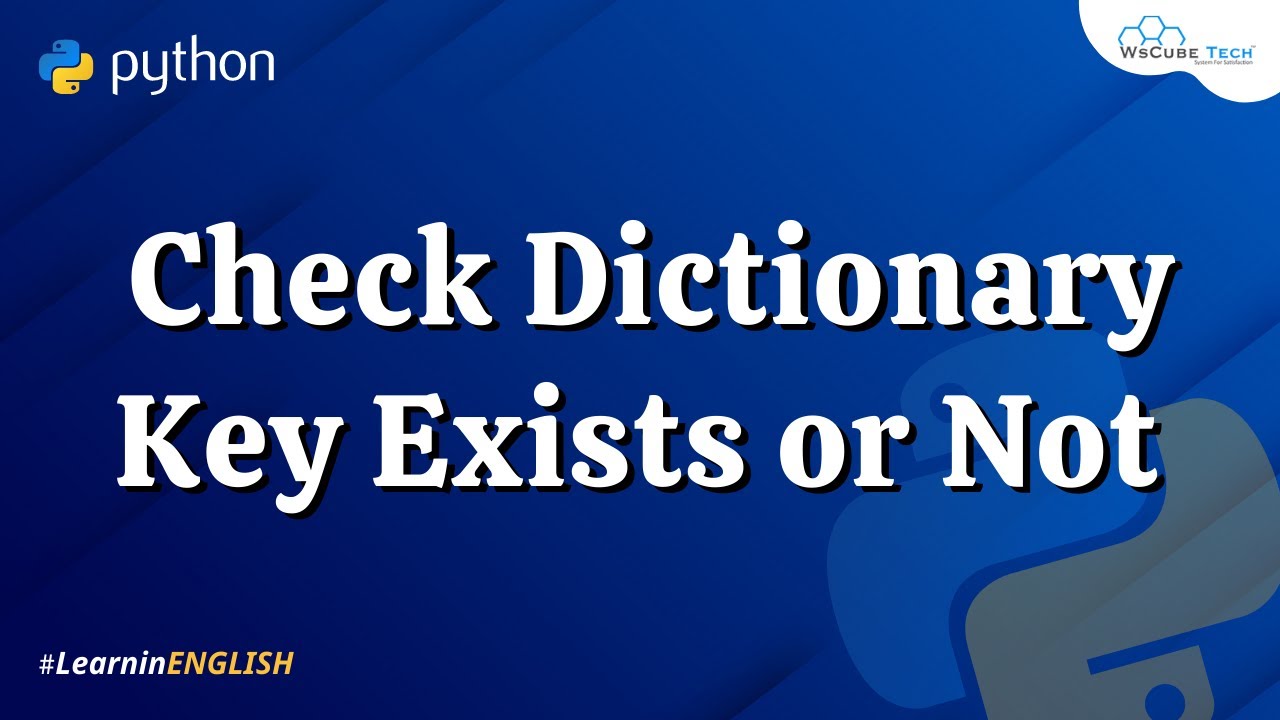


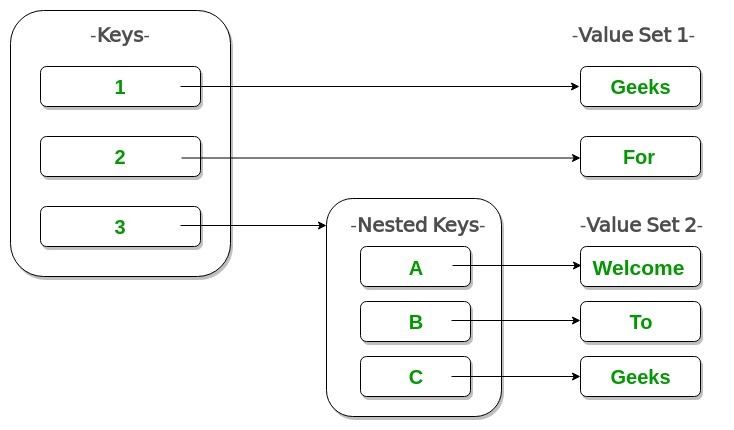
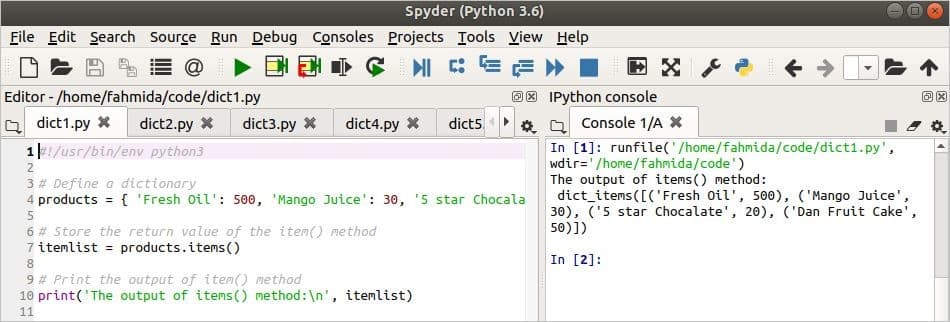
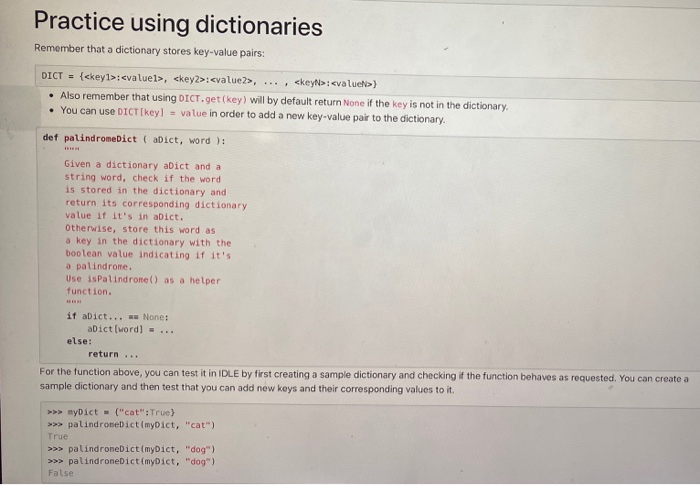
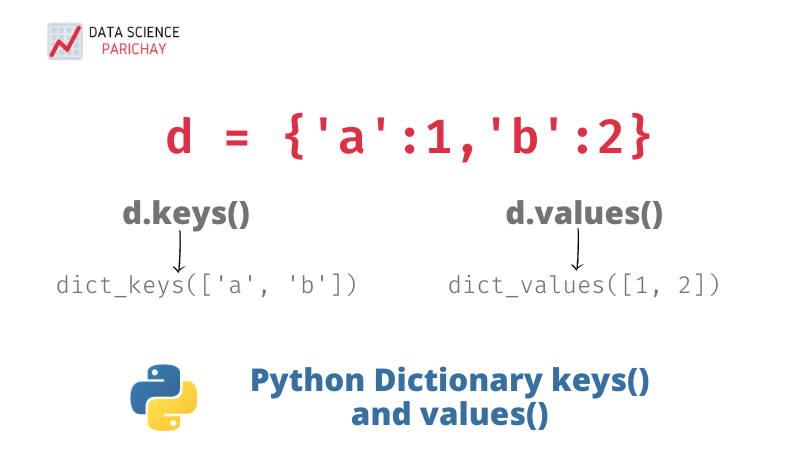
Article link: check if key in dict python.
Learn more about the topic check if key in dict python.
- Check if Key Exists in Dictionary Python – Scaler Topics
- How to check if key exists in a python dictionary? – Flexiple
- Check if a given key already exists in a dictionary
- How to check if a key exists in a Python dictionary – Educative.io
- Check whether given Key already exists in a Python Dictionary
- Python Check if Key Exists in Dictionary – Spark By {Examples}
- Check whether given Key already exists in a Python Dictionary
- How To Check If Key Exists In Dictionary In Python?
- Check if key/value exists in dictionary in Python – nkmk note
- Check if Key exists in Dictionary (or Value) with Python code