Check If Key Exists In Dictionary Python
Different Ways to Check If a Key Exists in a Dictionary:
1. Using the ‘in’ Operator:
The most common and straightforward way to check if a key exists in a dictionary is by using the ‘in’ operator. This operator returns True if the key is present in the dictionary, and False otherwise. Here’s an example:
“`python
my_dict = {‘apple’: 1, ‘banana’: 2, ‘cherry’: 3}
if ‘banana’ in my_dict:
print(“Key ‘banana’ exists in the dictionary!”)
“`
Output:
“`
Key ‘banana’ exists in the dictionary!
“`
2. Using the get() Method:
The get() method allows you to access the value associated with a specific key in a dictionary. If the key exists, it returns the corresponding value. However, if the key does not exist, it returns a default value (None by default). By checking if the returned value is None or not, we can determine if the key exists in the dictionary. Here’s an example:
“`python
my_dict = {‘apple’: 1, ‘banana’: 2, ‘cherry’: 3}
value = my_dict.get(‘banana’)
if value is not None:
print(“Key ‘banana’ exists in the dictionary!”)
“`
Output:
“`
Key ‘banana’ exists in the dictionary!
“`
3. Using the keys() Method:
The keys() method returns a list-like object containing all the keys present in a dictionary. By using the ‘in’ operator with the keys() method, we can effectively check if a key exists in the dictionary. Here’s an example:
“`python
my_dict = {‘apple’: 1, ‘banana’: 2, ‘cherry’: 3}
if ‘banana’ in my_dict.keys():
print(“Key ‘banana’ exists in the dictionary!”)
“`
Output:
“`
Key ‘banana’ exists in the dictionary!
“`
4. Using the has_key() Method (deprecated in Python 3.x):
The has_key() method was used in older versions of Python (2.x) to check if a key exists in a dictionary. However, this method is deprecated in Python 3.x and no longer recommended for use. It’s better to opt for other methods mentioned above or below for compatibility and best practices.
5. Using a try-except Block:
Another way to check if a key exists in a dictionary is by using a try-except block. We can try accessing the value associated with the given key using the dictionary[key] syntax. If the key is present, the code will execute without any errors. Otherwise, a KeyError will be raised, which can be caught using an except block. Here’s an example:
“`python
my_dict = {‘apple’: 1, ‘banana’: 2, ‘cherry’: 3}
try:
value = my_dict[‘banana’]
print(“Key ‘banana’ exists in the dictionary!”)
except KeyError:
print(“Key ‘banana’ does not exist in the dictionary!”)
“`
Output:
“`
Key ‘banana’ exists in the dictionary!
“`
6. Using the __contains__() Method:
Python dictionaries have a built-in __contains__() method that can be used to check whether a key exists in the dictionary or not. It returns True if the key is present and False otherwise. Here’s an example:
“`python
my_dict = {‘apple’: 1, ‘banana’: 2, ‘cherry’: 3}
if my_dict.__contains__(‘banana’):
print(“Key ‘banana’ exists in the dictionary!”)
“`
Output:
“`
Key ‘banana’ exists in the dictionary!
“`
7. Using the isinstance() Function:
The isinstance() function is used to check the type of an object. By checking if a dictionary key is an instance of the string type, we can verify its existence. Here’s an example:
“`python
my_dict = {‘apple’: 1, ‘banana’: 2, ‘cherry’: 3}
key = ‘banana’
if isinstance(key, str) and key in my_dict:
print(“Key ‘banana’ exists in the dictionary!”)
“`
Output:
“`
Key ‘banana’ exists in the dictionary!
“`
8. Using a for Loop and Comparing Keys:
We can iterate through the keys of a dictionary using a for loop and compare each key with the desired key. If a match is found, it means the key exists in the dictionary. Here’s an example:
“`python
my_dict = {‘apple’: 1, ‘banana’: 2, ‘cherry’: 3}
key_to_find = ‘banana’
for key in my_dict:
if key == key_to_find:
print(f”Key ‘{key_to_find}’ exists in the dictionary!”)
break
“`
Output:
“`
Key ‘banana’ exists in the dictionary!
“`
Check if Key Does Not Exist in a Dictionary Python:
To check if a key does not exist in a dictionary, you can use the ‘not in’ operator. It returns True if the key is not present in the dictionary, and False otherwise. Here’s an example:
“`python
my_dict = {‘apple’: 1, ‘banana’: 2, ‘cherry’: 3}
if ‘mango’ not in my_dict:
print(“Key ‘mango’ does not exist in the dictionary!”)
“`
Output:
“`
Key ‘mango’ does not exist in the dictionary!
“`
Check Value in Dictionary Python:
To check if a value exists in a dictionary, you can use the ‘in’ operator with the dictionary.values() method. The values() method returns a list-like object containing all the values present in the dictionary. Here’s an example:
“`python
my_dict = {‘apple’: 1, ‘banana’: 2, ‘cherry’: 3}
if 2 in my_dict.values():
print(“Value 2 exists in the dictionary!”)
“`
Output:
“`
Value 2 exists in the dictionary!
“`
Find Element in Dictionary Python:
To find an element in a dictionary, we can use either the ‘in’ operator with the dictionary.keys() method or the get() method. The ‘in’ operator checks if a key exists, while the get() method returns the associated value if the key exists. Here’s an example using the ‘in’ operator:
“`python
my_dict = {‘apple’: 1, ‘banana’: 2, ‘cherry’: 3}
if ‘banana’ in my_dict:
print(“Element ‘banana’ exists in the dictionary!”)
“`
Output:
“`
Element ‘banana’ exists in the dictionary!
“`
Print Key in Dictionary Python:
To print all the keys in a dictionary, we can use a simple for loop. Here’s an example:
“`python
my_dict = {‘apple’: 1, ‘banana’: 2, ‘cherry’: 3}
for key in my_dict:
print(key)
“`
Output:
“`
apple
banana
cherry
“`
Add Value to Key Dictionary Python:
To add or update a value associated with a key in a dictionary, you can use the dictionary[key] syntax. If the key already exists, the value will be updated. Otherwise, a new key-value pair will be added to the dictionary. Here’s an example:
“`python
my_dict = {‘apple’: 1, ‘banana’: 2, ‘cherry’: 3}
my_dict[‘banana’] = 10
print(my_dict)
“`
Output:
“`
{‘apple’: 1, ‘banana’: 10, ‘cherry’: 3}
“`
How to Check If Value Exists in Python:
To check if a value exists in a Python list, you can use the ‘in’ operator. It returns True if the value is present in the list, and False otherwise. Here’s an example:
“`python
my_list = [‘apple’, ‘banana’, ‘cherry’]
if ‘banana’ in my_list:
print(“Value ‘banana’ exists in the list!”)
“`
Output:
“`
Value ‘banana’ exists in the list!
“`
For Key-Value in Dict Python:
To iterate through a dictionary and access both the keys and values, you can use the items() method. This method returns a list-like object containing tuples with the key-value pairs. Here’s an example:
“`python
my_dict = {‘apple’: 1, ‘banana’: 2, ‘cherry’: 3}
for key, value in my_dict.items():
print(key, value)
“`
Output:
“`
apple 1
banana 2
cherry 3
“`
Change Value in Dictionary Python:
To change the value associated with a key in a dictionary, you can use the dictionary[key] syntax. Here’s an example:
“`python
my_dict = {‘apple’: 1, ‘banana’: 2, ‘cherry’: 3}
my_dict[‘banana’] = 10
print(my_dict)
“`
Output:
“`
{‘apple’: 1, ‘banana’: 10, ‘cherry’: 3}
“`
FAQs:
Q1: What happens if we use the ‘in’ operator on a non-existent key in a dictionary?
A1: If an ‘in’ operator is used on a non-existent key in a dictionary, it will return False.
Q2: What is the difference between using ‘in’ operator and ‘keys()’ method to check if a key exists in a dictionary?
A2: The ‘in’ operator directly checks if a key exists in a dictionary, while the ‘keys()’ method returns a list-like object containing all the keys in the dictionary. By using the ‘in’ operator with the ‘keys()’ method, we perform the existence check indirectly by iterating through the keys.
Q3: Can we check if a value exists in a dictionary directly, without looping through the keys?
A3: Yes, we can use the ‘in’ operator with the ‘values()’ method to check if a value exists in a dictionary.
Q4: Which method is the most recommended and efficient one to check if a key exists in a dictionary?
A4: The ‘in’ operator is generally considered the most recommended and efficient method to check if a key exists in a dictionary due to its simplicity and directness.
Q5: Can we use the ‘in’ operator to check if a key exists in nested dictionaries?
A5: Yes, the ‘in’ operator can be used recursively to check if a key exists in nested dictionaries. You can use multiple ‘in’ operators or a combination with other methods explained above to accomplish this.
In conclusion, we have explored various effective methods to check if a key exists in a Python dictionary. These methods include using the ‘in’ operator, the get() method, the keys() method, the has_key() method (deprecated), a try-except block, the __contains__() method, the isinstance() function, and a for loop. Additionally, we have covered related operations such as checking if a key does not exist, finding elements, printing keys, adding values to keys, changing values, and iterating through key-value pairs. These techniques provide flexibility and versatility in handling dictionary operations efficiently.
How To Check If A Key Exists In A Python Dictionary | Python Beginner Tutorial
How To Check If A List Of Keys Exists In A Dictionary Python?
In Python, dictionaries are widely used data structures that store data in key-value pairs. While working with dictionaries, you may come across a situation where you need to check if a specific list of keys exists in the dictionary. This can be accomplished using different approaches, and in this article, we will explore some of the most commonly used methods to achieve this task in Python.
Method 1: Looping through the keys and checking existence
One simple and straightforward approach is to loop through each key in the list and check if it exists in the dictionary using an ‘in’ operator. Here’s an example:
“`python
def check_keys_exist(dictionary, keys):
for key in keys:
if key not in dictionary:
return False
return True
“`
In this method, we define a function `check_keys_exist` that takes a dictionary and a list of keys as arguments. We loop through each key in the list and use the ‘in’ operator to check if it exists in the dictionary. If any key is not found, we return False. If all keys exist, we return True.
Method 2: Using Set Intersection
Another efficient approach is to convert the list of keys to a set and use the set intersection operation ‘&’. If the length of the resulting intersection is equal to the length of the list of keys, then all the keys exist in the dictionary. This method avoids the need for looping through each key and can perform better for large dictionaries.
“`python
def check_keys_exist(dictionary, keys):
return len(set(keys) & set(dictionary.keys())) == len(keys)
“`
Here, we use the `&` operator to perform set intersection between the set of keys and the set of dictionary keys. If the length of the result is equal to the length of the list of keys, it means all the keys exist in the dictionary, and we return True.
Method 3: Using the all() function
The `all()` function in Python returns True if all elements in an iterable are True. We can use this function along with a list comprehension to check if all the keys exist in the dictionary.
“`python
def check_keys_exist(dictionary, keys):
return all(key in dictionary for key in keys)
“`
In this method, we use a list comprehension to generate a list of boolean values indicating the existence of each key in the dictionary. The `all()` function then checks if all the elements in the generated list are True. If so, it returns True, indicating that all keys exist.
FAQs:
Q1. What happens if one or more keys in the list are not present in the dictionary?
If one or more keys in the list are not present in the dictionary, the mentioned methods will return False, indicating that the keys do not exist. It’s essential to handle this case in your code and perform appropriate actions based on the returned value.
Q2. Are these methods case-sensitive?
Yes, these methods are case-sensitive. For example, if your dictionary contains the key ‘name’, and you check for the key ‘Name’, it will not be considered as existing. Python treats keys as distinct entities, and their capitalization matters.
Q3. Can I check for keys in nested dictionaries?
Yes, the mentioned methods can be used to check for keys in nested dictionaries as well. You can recursively apply the methods to the nested dictionaries until you reach the desired level of depth.
Q4. Is there any difference in performance among these methods?
The performance of these methods may vary depending on the size of the dictionary and the list of keys. The set intersection method (`&`) might have better performance for large dictionaries compared to the looping method, as it avoids the need for iterating through each key.
Q5. Can I use these methods for checking values instead of keys?
No, these methods specifically check for the existence of keys in a dictionary. If you want to check for values, you’ll need to iterate through the dictionary using methods such as `.values()` and compare the values accordingly.
In conclusion, checking if a list of keys exists in a dictionary is a commonly encountered task while working with Python dictionaries. This article covered three different methods to accomplish this task effectively. By selecting the appropriate method based on your specific requirements, you can efficiently check for the existence of keys in Python dictionaries.
How To Check If Key-Value Pair Exists In Dictionary Python?
In Python, dictionaries are a versatile and powerful data type. They store data as key-value pairs, allowing for efficient storage and retrieval of information. However, there may be scenarios where we need to check if a specific key-value pair exists in a dictionary. In this article, we will explore different approaches to accomplish this task in Python and discuss the advantages of each method.
Method 1: Using the ‘in’ operator
The ‘in’ operator is a commonly used approach to check if a key exists in a dictionary. By using this operator, we can easily determine if a specific key is present in the dictionary and, if it is, retrieve its corresponding value.
Here’s an example of how we can use the ‘in’ operator to check for a key-value pair:
“`
# Creating a sample dictionary
sample_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
# Checking if a key-value pair exists
if ‘name’ in sample_dict and sample_dict[‘name’] == ‘John’:
print(“Key-value pair exists in the dictionary”)
else:
print(“Key-value pair does not exist in the dictionary”)
“`
Method 2: Using the ‘get()’ method
The ‘get()’ method in Python dictionaries allows us to retrieve the value associated with a key. However, if the key doesn’t exist, it returns ‘None’ by default. We can leverage this behavior to check if a key-value pair exists without causing any errors.
Let’s see an example of using the ‘get()’ method to check for a key-value pair:
“`
# Creating a sample dictionary
sample_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
# Checking if a key-value pair exists
if sample_dict.get(‘name’) == ‘John’:
print(“Key-value pair exists in the dictionary”)
else:
print(“Key-value pair does not exist in the dictionary”)
“`
Method 3: Using the ‘items()’ method
The ‘items()’ method in Python dictionaries returns a list of key-value pairs. By iterating over this list, we can check each key-value pair and determine if our desired pair exists.
Let’s take a look at an example of using the ‘items()’ method to check for a key-value pair:
“`
# Creating a sample dictionary
sample_dict = {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’}
# Checking if a key-value pair exists
key_value_exists = False
for key, value in sample_dict.items():
if key == ‘name’ and value == ‘John’:
key_value_exists = True
break
if key_value_exists:
print(“Key-value pair exists in the dictionary”)
else:
print(“Key-value pair does not exist in the dictionary”)
“`
FAQs:
Q: Can we determine if only the key or value exists in a dictionary?
A: Yes, absolutely. The methods discussed above can be modified to check for the existence of either a key or a value independently. For example, you can simply use the ‘in’ operator to check if a specific key is present in the dictionary, or iterate over the values using the ‘values()’ method and check if your desired value exists.
Q: What happens if we try to check for a non-existing key-value pair?
A: If you attempt to check for a non-existing key-value pair, the ‘in’ operator and ‘get()’ method will return False or None, respectively. Similarly, when using the ‘items()’ method, the code will not find a matching key-value pair during the iteration.
Q: Which method is the most efficient for checking key-value pair existence?
A: The ‘in’ operator is considered the most efficient way to check for key-value pair existence in a dictionary. It takes advantage of the internal data structure of dictionaries, resulting in faster performance compared to other methods.
Q: What if the dictionary contains duplicate values?
A: The methods discussed in this article will only check for the first occurrence of a specific key and value. If there are duplicates, the existence of the key-value pair will be determined based on the first occurrence.
In conclusion, checking if a key-value pair exists in a dictionary is a common requirement in Python programming. By using the ‘in’ operator, ‘get()’ method, or ‘items()’ method, we can effectively determine the presence of a key-value pair with different levels of simplicity and performance. Understanding these methods will greatly enhance your ability to work with dictionaries in Python.
Keywords searched by users: check if key exists in dictionary python Check if key not exists in dictionary Python, Check value in dictionary Python, Find element in dictionary Python, Print key in dictionary Python, Add value to key dictionary Python, How to check if value exists in python, For key-value in dict Python, Change value in dictionary Python
Categories: Top 20 Check If Key Exists In Dictionary Python
See more here: nhanvietluanvan.com
Check If Key Not Exists In Dictionary Python
In Python, dictionaries are widely used data structures that allow you to store and retrieve data in a key-value pair format. Sometimes, when working with dictionaries, you may need to check if a specific key does not exist. While Python provides several methods and techniques to accomplish this task, it’s essential to understand the most efficient and accurate approach.
In this article, we will explore different ways to check if a key is not present in a dictionary using Python. We will also provide additional insights into the topic and answer some frequently asked questions to ensure your understanding is comprehensive. So let’s dive in!
Method 1: Using the `in` operator
Python allows you to use the `in` operator to check if a specific key exists in a dictionary. By negating this operator using the `not` keyword, you can easily determine if a key is not present. Here’s an example:
“`
my_dict = {“name”: “John”, “age”: 25, “country”: “USA”}
if “height” not in my_dict:
print(“The key ‘height’ does not exist in the dictionary.”)
“`
In this code snippet, we check if the key “height” is not present in the dictionary `my_dict`. If it is not found, we print a corresponding message.
Method 2: Using the `get()` method
Another common way to check if a key does not exist in a dictionary is by utilizing the `get()` method. This method allows you to retrieve the value associated with a given key. However, when the key is not present, it returns a default value, which you can specify. By comparing the default value with the value returned by `get()`, you can determine the absence of the key. Here’s an example:
“`
my_dict = {“name”: “John”, “age”: 25, “country”: “USA”}
if my_dict.get(“height”) is None:
print(“The key ‘height’ does not exist in the dictionary.”)
“`
In this code snippet, we use the `get()` method to retrieve the value associated with the key “height”. If the key is not found, the method returns `None`, indicating its absence.
Method 3: Using the `not` keyword with `dict.get()`
An alternative approach to Method 2 is combining the `not` keyword with `dict.get()`. This method is particularly useful if you want to perform a specific action when the key does not exist. Here’s an example:
“`
my_dict = {“name”: “John”, “age”: 25, “country”: “USA”}
if not my_dict.get(“height”):
print(“The key ‘height’ does not exist in the dictionary.”)
“`
In this code snippet, the `not` keyword negates the truthiness of the returned value by `get()`, which will be `None` if the key does not exist. Hence, the print statement will be executed.
Additional Insights:
1. Unlike lists or tuples, dictionaries do not raise a `KeyError` if the key is not found. Instead, they return the specified default value or `None` if not provided.
2. The mentioned methods are efficient for checking the absence of a key in a dictionary. However, while using them, it’s crucial to ensure that the dictionary itself is not `None`. Checking for `None` before performing these checks will prevent any potential errors.
FAQs:
Q1. What happens if you try to access a non-existent key directly?
A1. If you attempt to access a non-existent key directly using bracket notation (e.g., `my_dict[“height”]`), Python will raise a `KeyError`. To avoid this error, it’s recommended to use the methods discussed above.
Q2. Is there a performance difference between the approaches?
A2. In most cases, the performance difference between the methods is negligible. However, the `in` operator is slightly faster than using `dict.get()` due to direct key lookup.
Q3. Can I use a default value other than `None` with `dict.get()`?
A3. Yes, you can provide a default value as the second argument to `dict.get()`. If the key is not found, the specified default value will be returned.
Q4. How can I check if a key is not present in nested dictionaries?
A4. To check the absence of a key in nested dictionaries, you can use the previously mentioned methods recursively. Iterate through each dictionary level and apply the desired approach until reaching the desired key or the deepest level.
In conclusion, checking if a key does not exist in a dictionary is a common operation when working with Python dictionaries. By using the `in` operator or the `get()` method with appropriate checks, you can easily accomplish this task. Remember to consider the performance implications and handle potential errors gracefully.
Check Value In Dictionary Python
In Python, dictionaries are an incredibly useful data structure for storing and retrieving key-value pairs. They allow you to associate a value with a corresponding key, making it easy to access and manipulate data based on these keys. One common operation when working with dictionaries is to check whether a particular value exists within the dictionary. In this article, we will explore various ways to perform this check and understand the underlying concepts.
Understanding Dictionaries in Python
Before we dive into checking values in a dictionary, let’s first familiarize ourselves with the basic concepts of dictionaries in Python. A dictionary, also known as a hash map or associative array, is an unordered collection of items. Each item in a dictionary has a key-value pair, where the key is used to identify the item and the value is the data associated with that key.
Dictionaries are created using curly braces ({}) and items are separated by commas. For example, let’s say we want to create a dictionary to store the age of different people:
“`python
ages = {‘John’: 25, ‘Alice’: 30, ‘Bob’: 28}
“`
In this dictionary, ‘John’, ‘Alice’, and ‘Bob’ are the keys, and 25, 30, and 28 are the corresponding values. We can access these values by using their respective keys, like `ages[‘John’]`, which would return `25`.
Checking Value Existence in a Dictionary
Now that we have a basic understanding of dictionaries, let’s explore some methods to check the existence of a value in a dictionary.
1. Using the `in` operator:
The simplest and most straightforward way to check if a value exists in a dictionary is by using the `in` operator. By writing `value in dictionary_name`, Python will return `True` if the value exists in the dictionary, and `False` otherwise. Let’s consider the following example:
“`python
ages = {‘John’: 25, ‘Alice’: 30, ‘Bob’: 28}
print(25 in ages) # Output: True
print(35 in ages) # Output: False
“`
2. Using the `values()` method:
Python provides a built-in `values()` method that returns a view object containing all the values in the dictionary. We can convert this view object to a list and then use the `in` operator to check for the presence of a value. Here’s an example:
“`python
ages = {‘John’: 25, ‘Alice’: 30, ‘Bob’: 28}
print(25 in ages.values()) # Output: True
print(35 in ages.values()) # Output: False
“`
3. Using a loop:
Another approach is to iterate over the dictionary’s values using a `for` loop and check if the desired value is encountered during iteration. Here’s an example:
“`python
ages = {‘John’: 25, ‘Alice’: 30, ‘Bob’: 28}
for age in ages.values():
if age == 25:
print(“Value exists!”)
break
else:
print(“Value does not exist!”)
“`
In this example, we loop through each value in the dictionary and compare it with the desired value. If a match is found, we print “Value exists!” and exit the loop using the `break` statement. If the loop doesn’t encounter a matching value, it executes the `else` block and prints “Value does not exist!”.
Frequently Asked Questions (FAQs)
Q1: Can dictionaries have duplicate values?
No, dictionaries cannot have duplicate values. In a dictionary, each key is unique and can only be associated with a single value. However, multiple keys can have the same value.
Q2: How can I check if a key exists in a dictionary?
You can use the `in` operator to check for the existence of a key in a dictionary. For example:
“`python
ages = {‘John’: 25, ‘Alice’: 30, ‘Bob’: 28}
print(‘John’ in ages) # Output: True
print(‘Jane’ in ages) # Output: False
“`
Q3: What happens if I try to access a non-existent key in a dictionary?
If you try to access a key that doesn’t exist in a dictionary, Python will raise a `KeyError`. To avoid this error, you can use the `get()` method, which returns `None` if the key is not found. For example:
“`python
ages = {‘John’: 25, ‘Alice’: 30, ‘Bob’: 28}
print(ages.get(‘John’)) # Output: 25
print(ages.get(‘Jane’)) # Output: None
“`
Q4: How can I get all the keys or values from a dictionary?
You can use the `keys()` and `values()` methods respectively to get a view object containing all the keys or values in a dictionary. To convert them into a list, you can use the `list()` constructor. Example:
“`python
ages = {‘John’: 25, ‘Alice’: 30, ‘Bob’: 28}
all_keys = list(ages.keys())
all_values = list(ages.values())
print(all_keys) # Output: [‘John’, ‘Alice’, ‘Bob’]
print(all_values) # Output: [25, 30, 28]
“`
Conclusion
In this article, we explored different methods to check the existence of a value in a dictionary in Python. We saw how to use the `in` operator, the `values()` method, and loop iteration. Understanding these approaches will empower you to efficiently determine the presence of a value in a dictionary and manipulate the data accordingly. Dictionaries are powerful tools for storing and accessing data, and being able to efficiently check values within them is an essential skill for any Python programmer.
Images related to the topic check if key exists in dictionary python
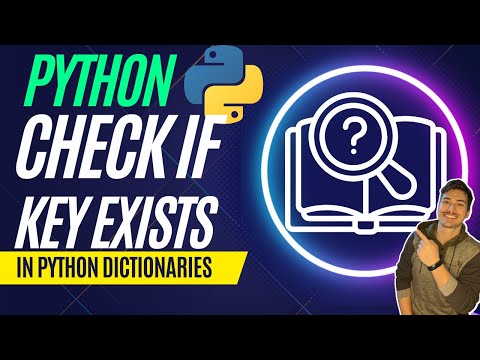
Found 14 images related to check if key exists in dictionary python theme
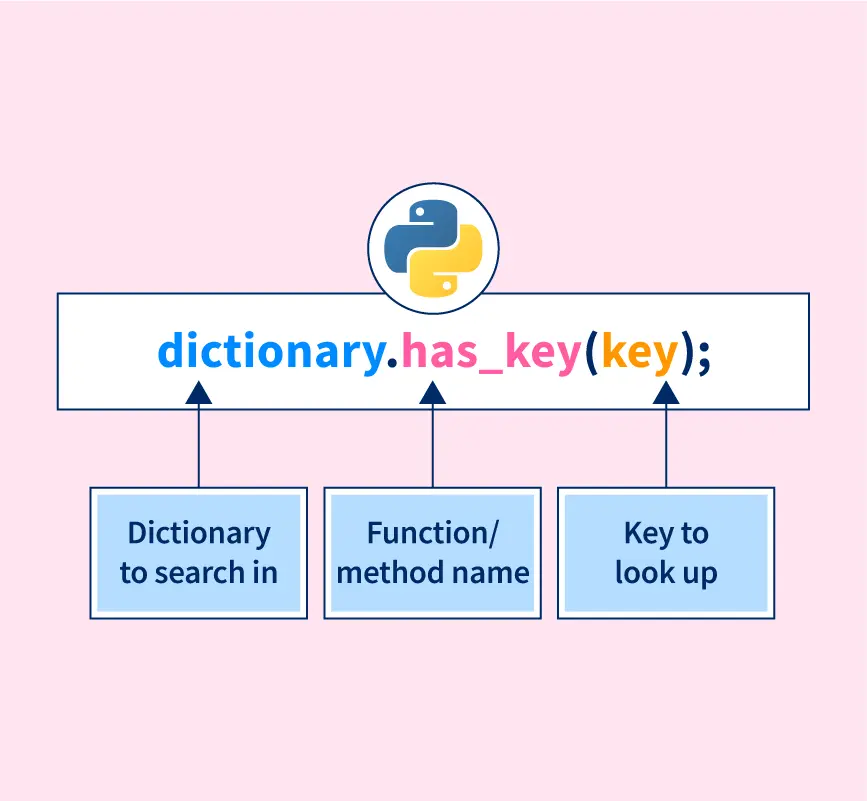
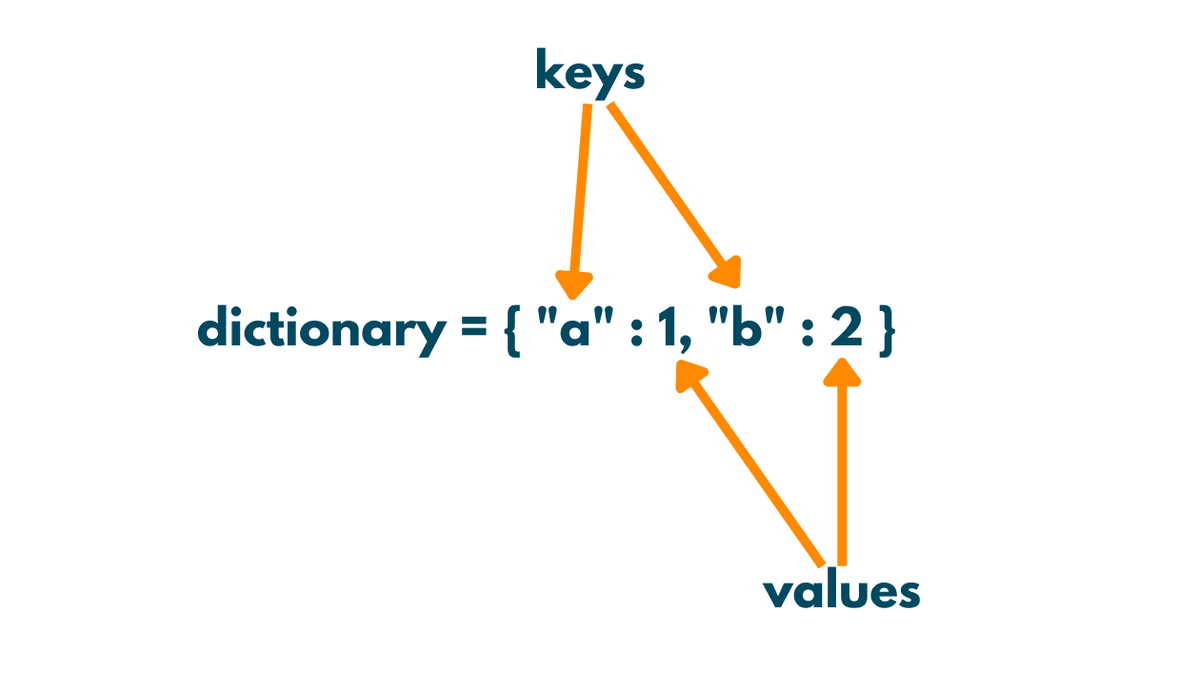
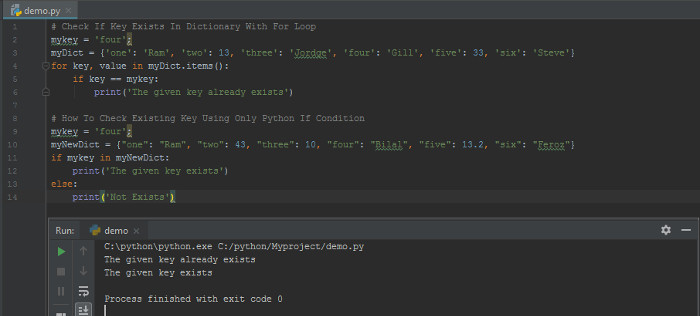

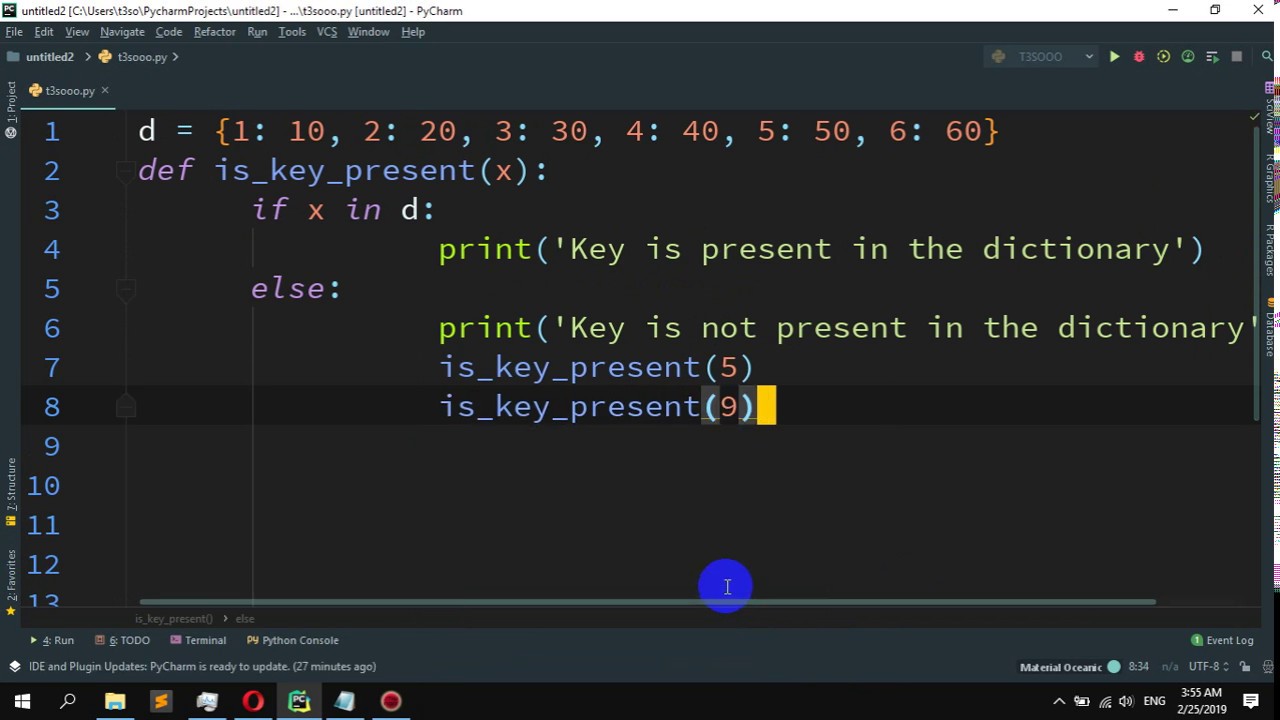
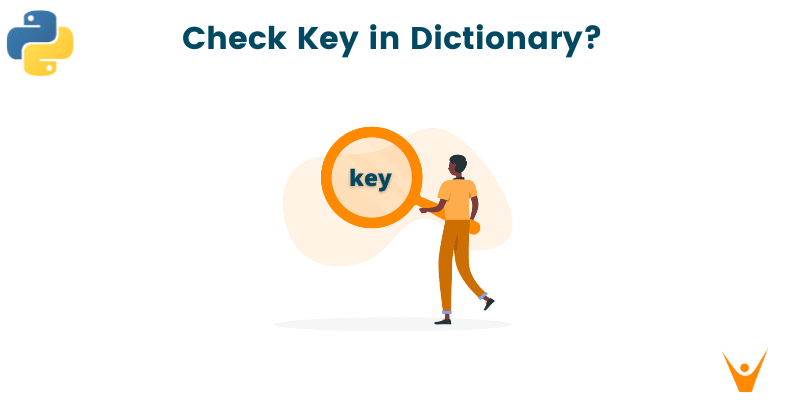
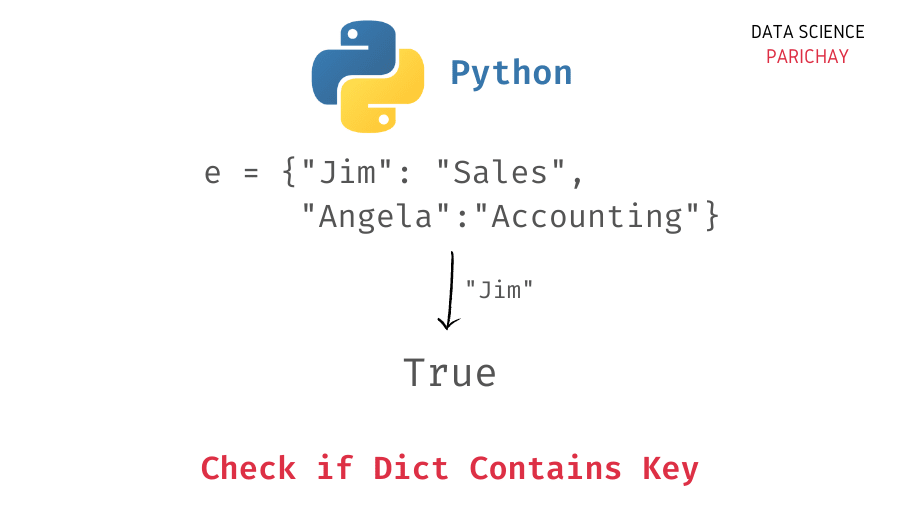
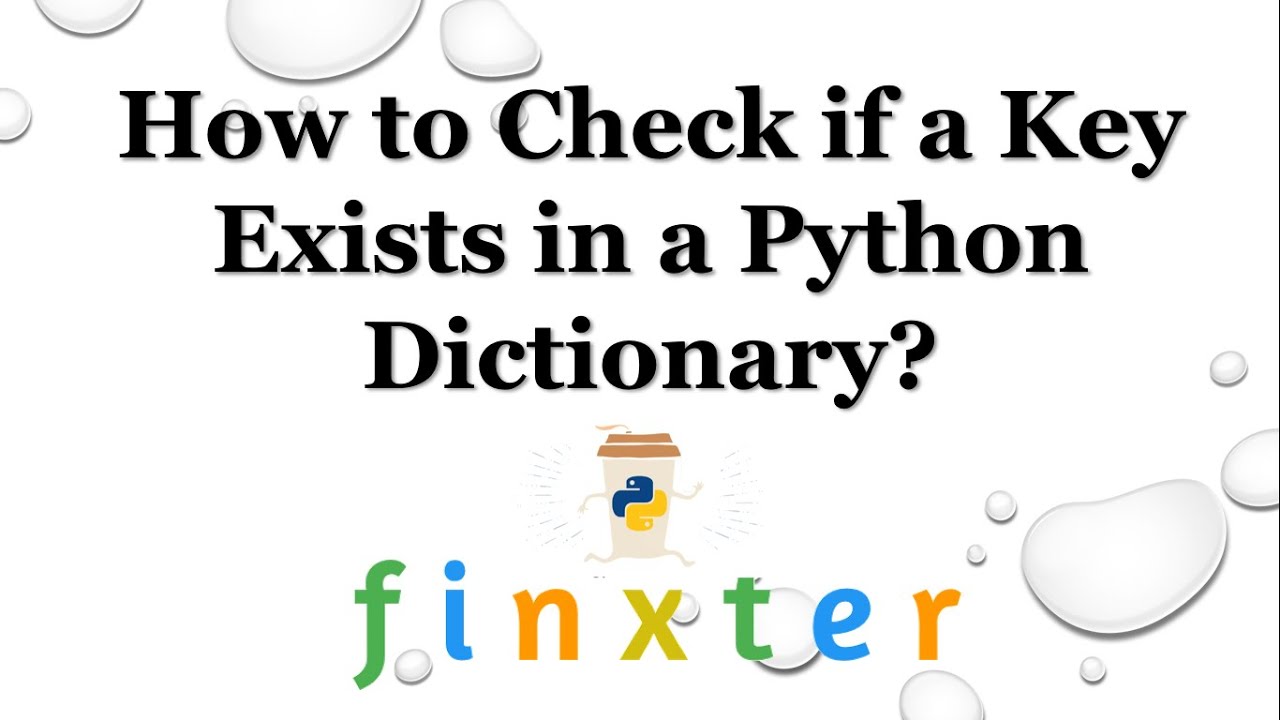
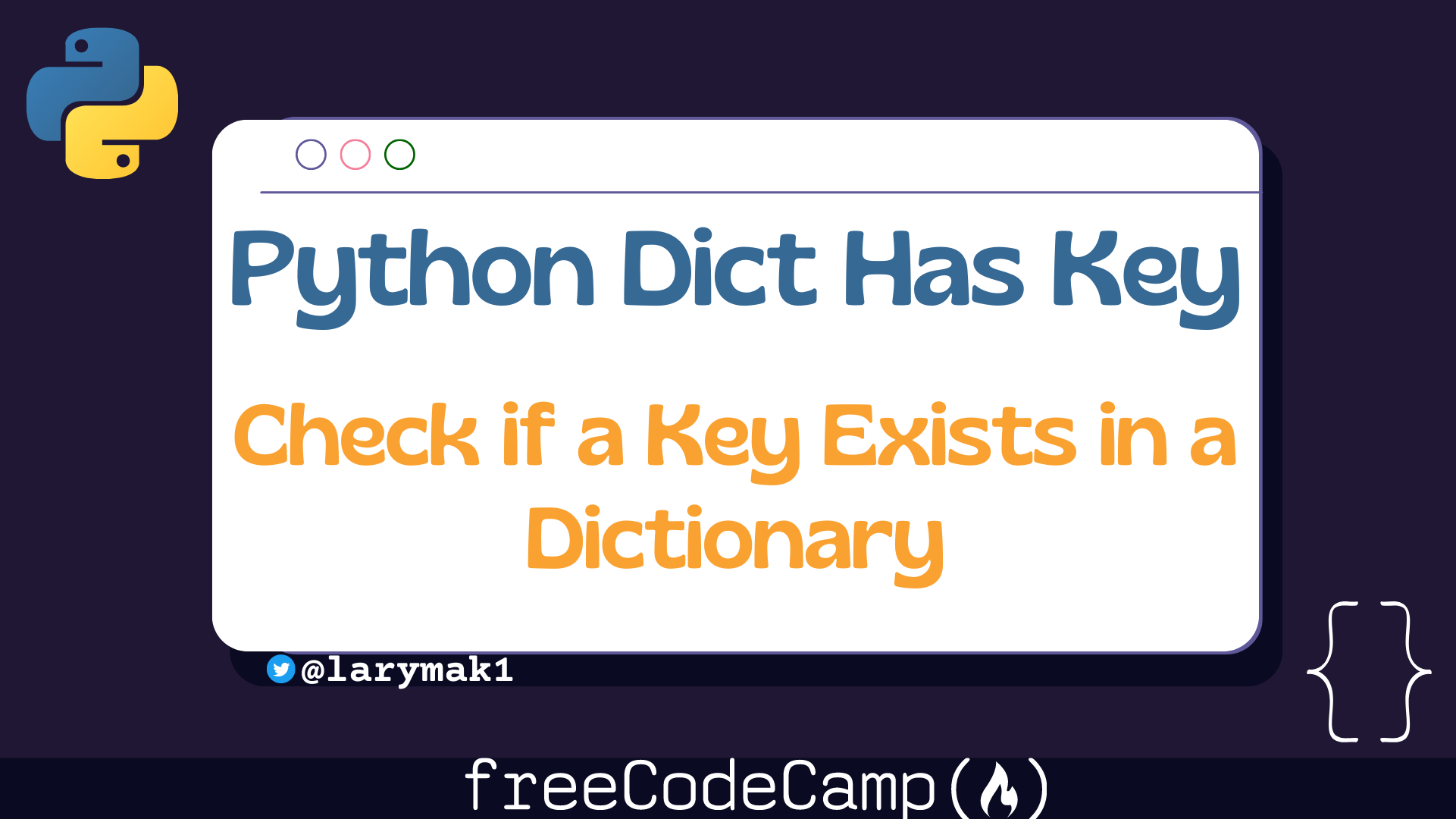

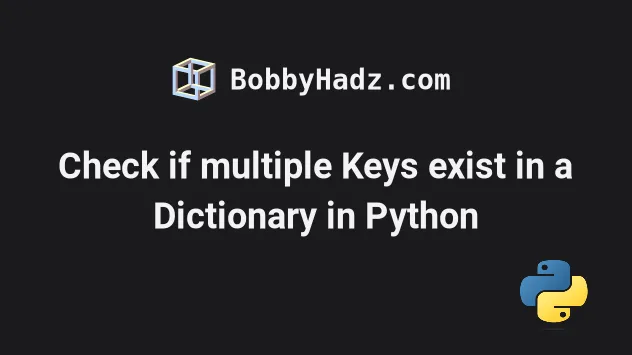
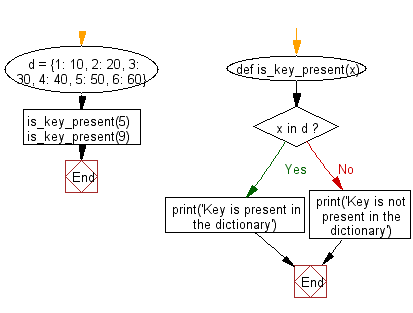


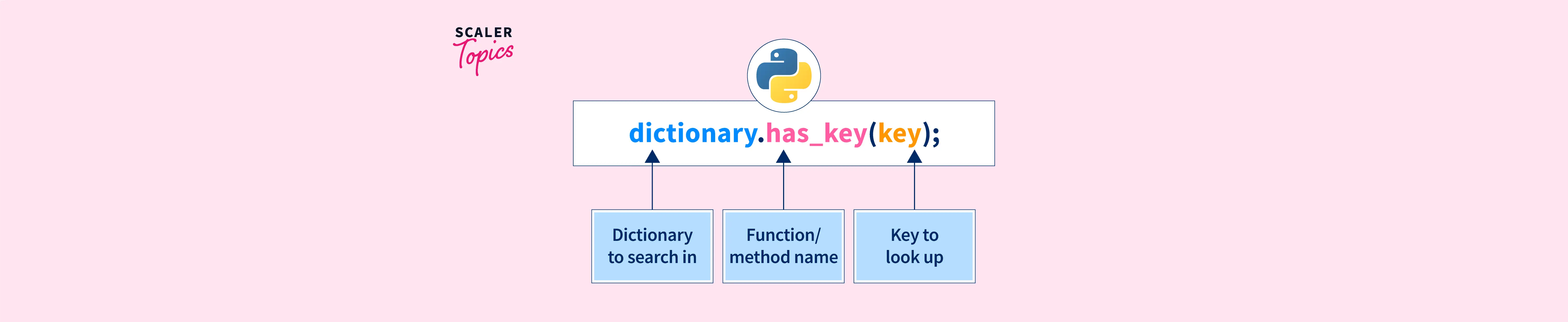
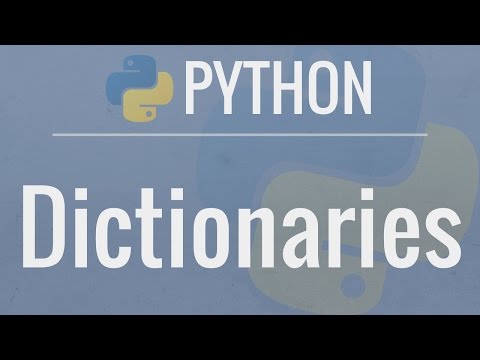
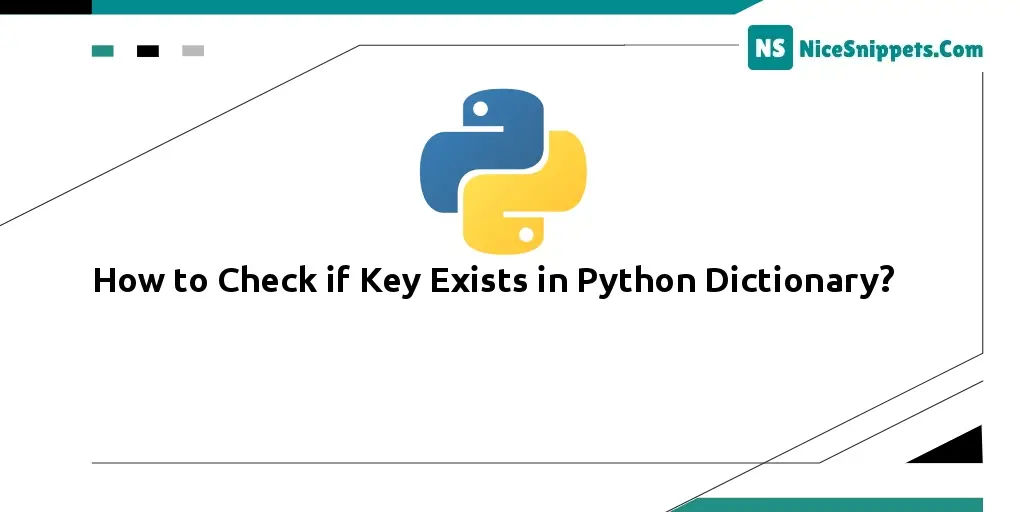

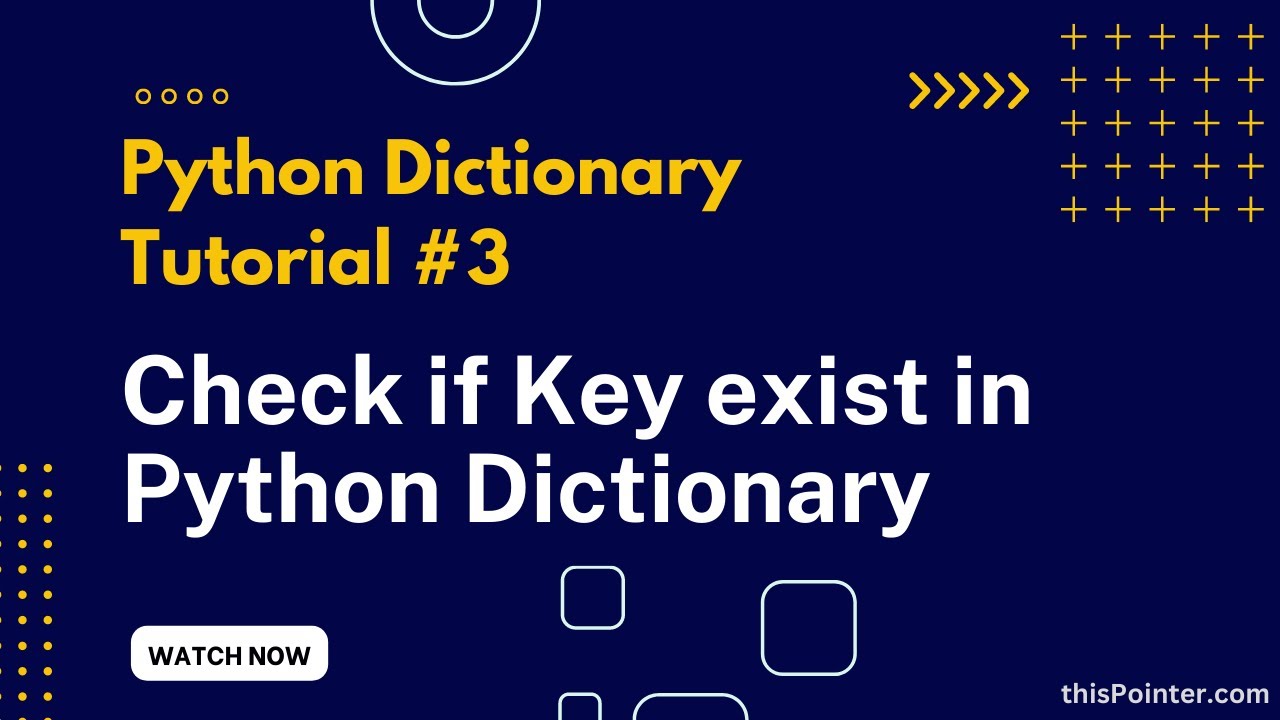
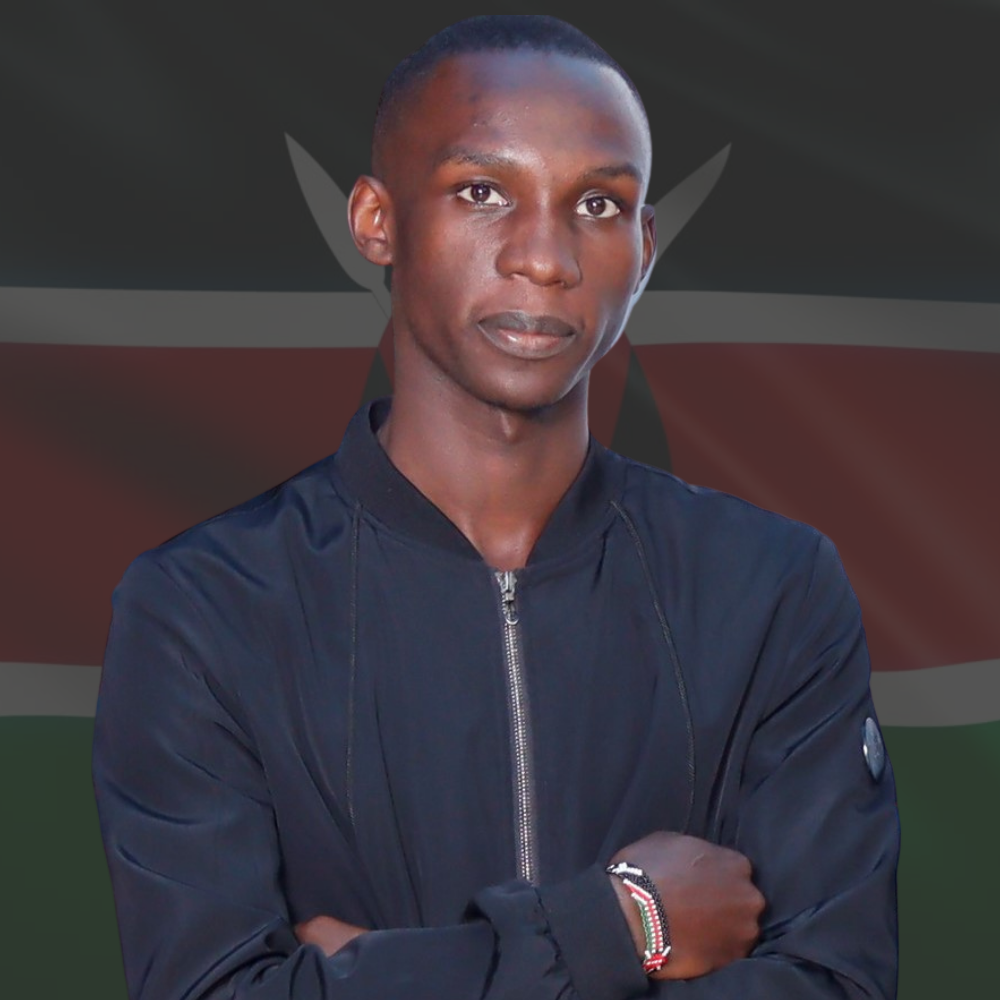
![SOLVED] Check if key exists in object in JS [3 Methods] | GoLinuxCloud Solved] Check If Key Exists In Object In Js [3 Methods] | Golinuxcloud](https://www.golinuxcloud.com/wp-content/uploads/example1.jpg)
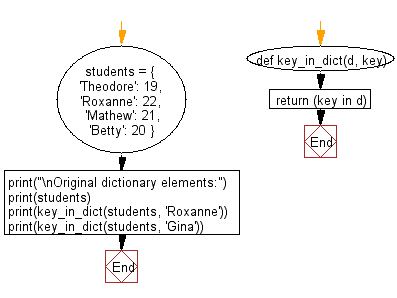
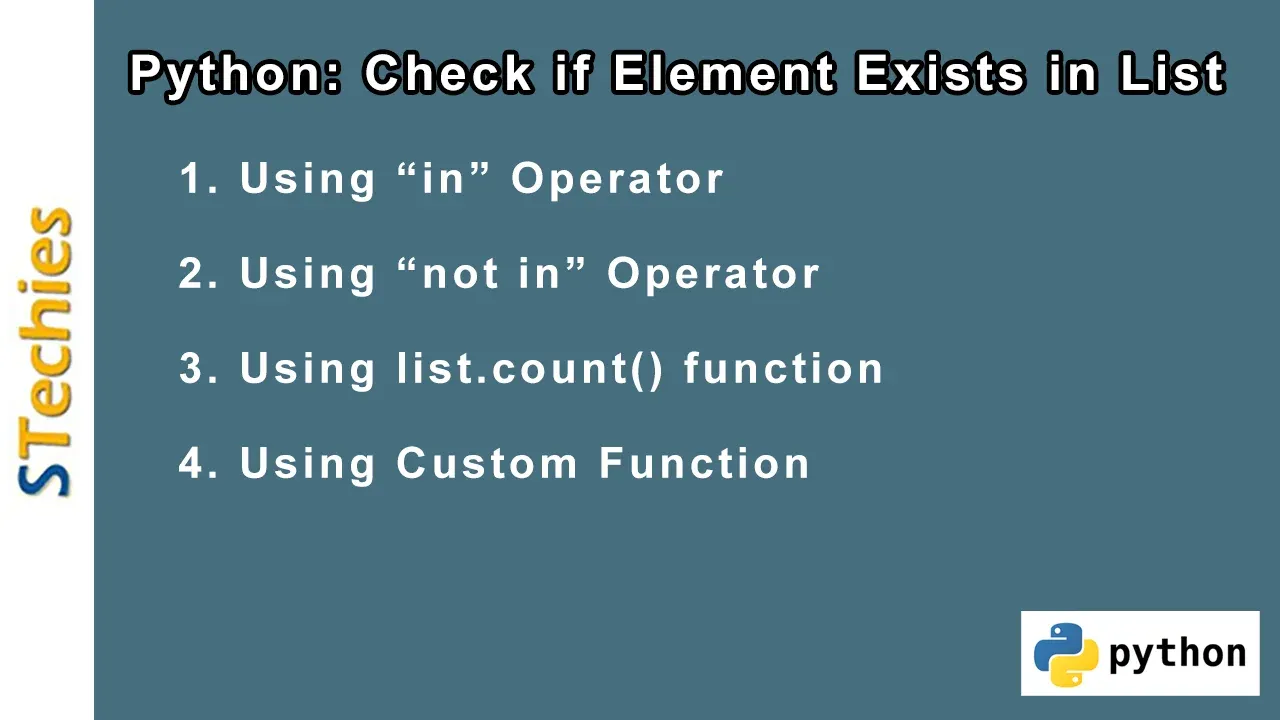
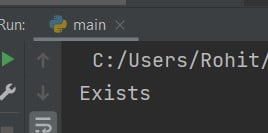
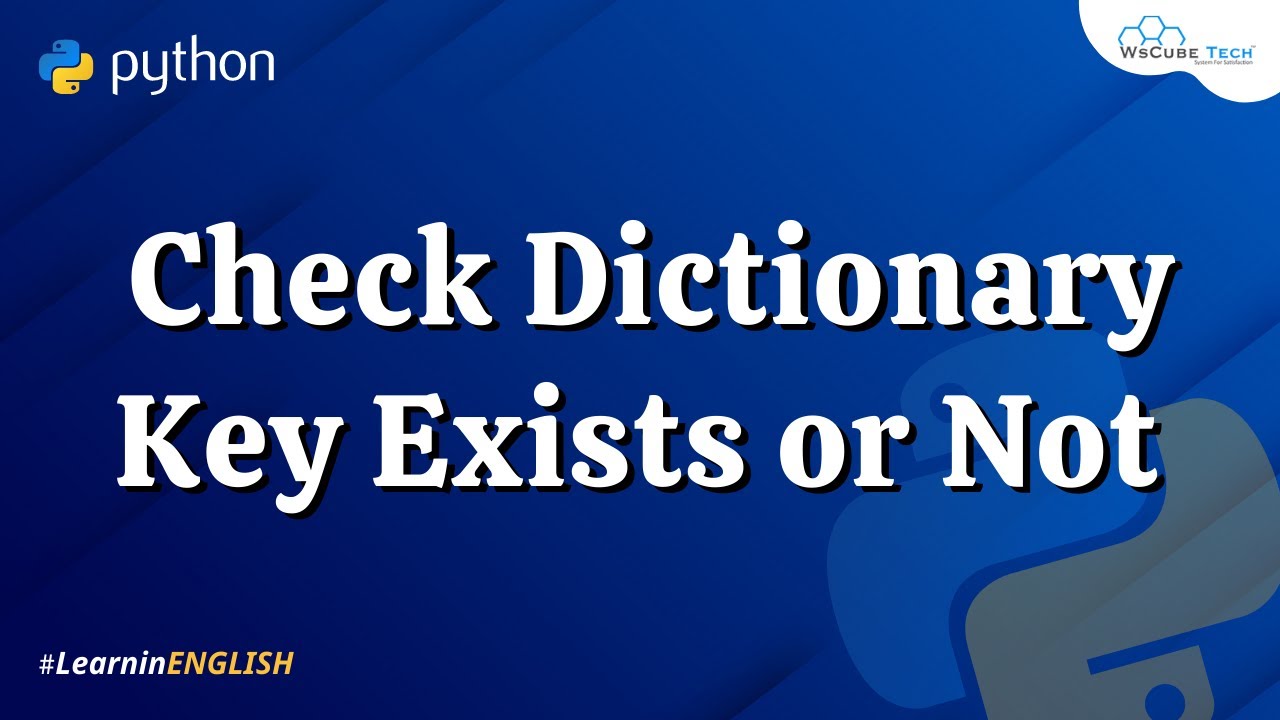
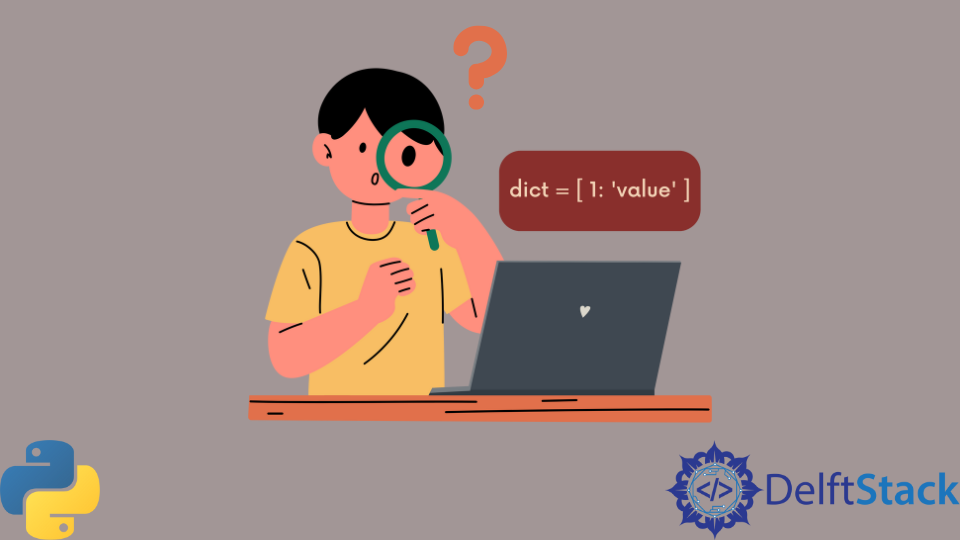

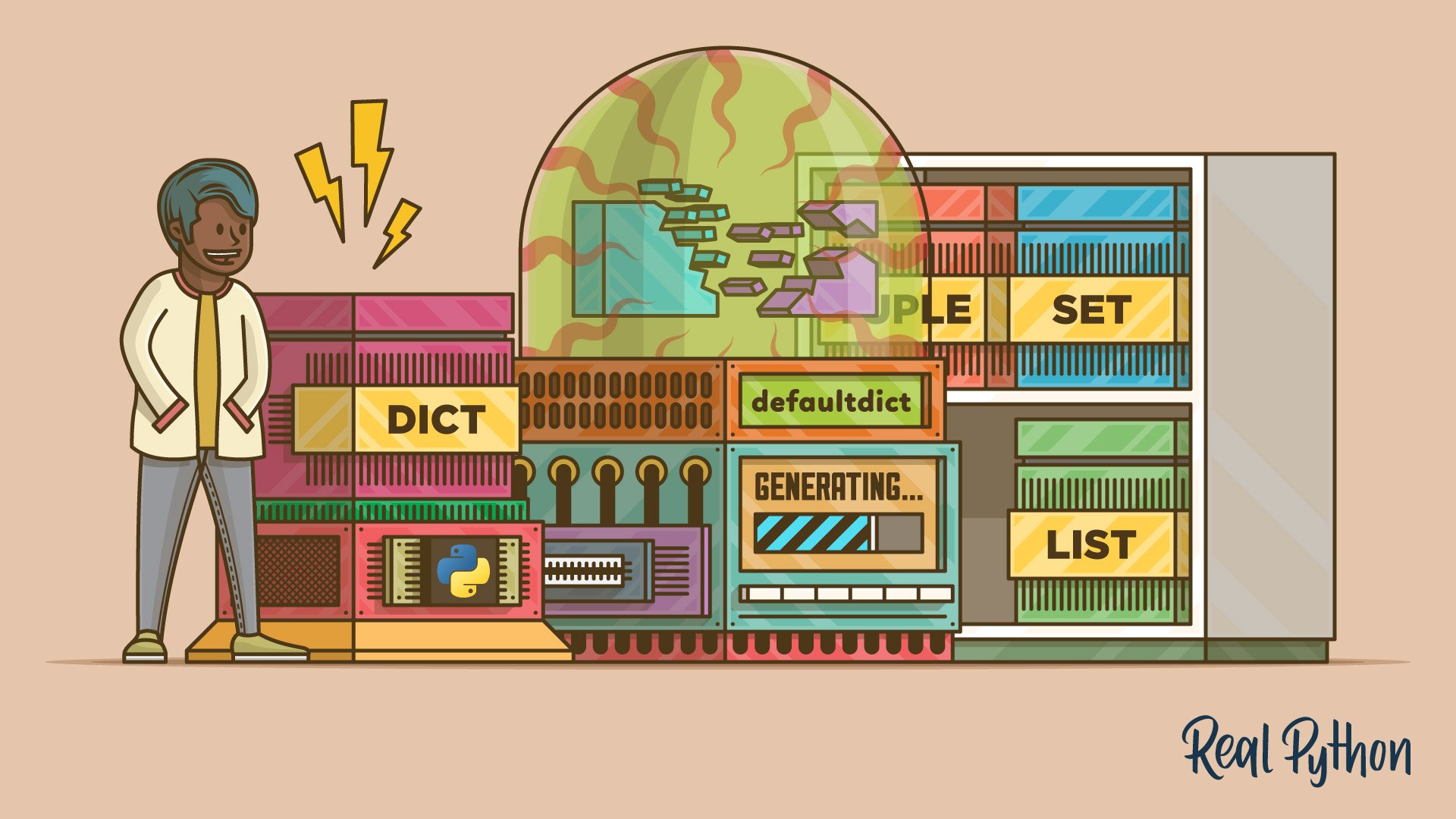
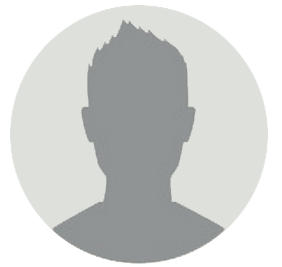

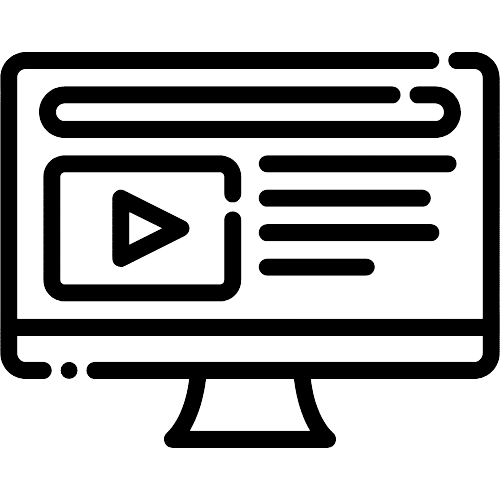

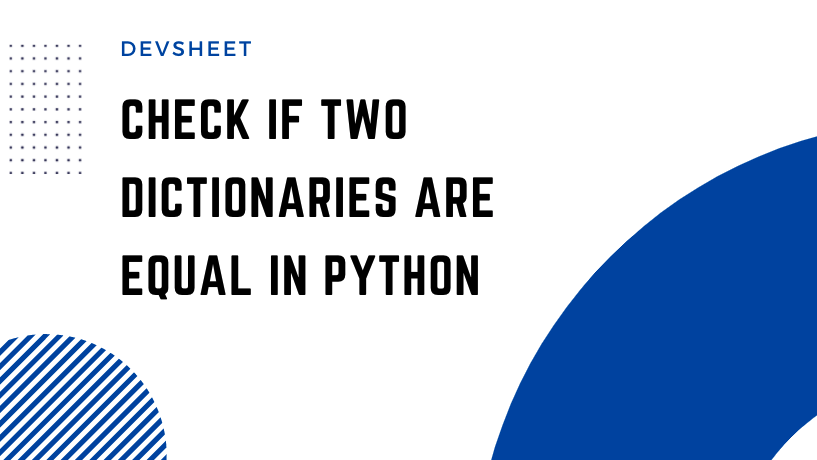

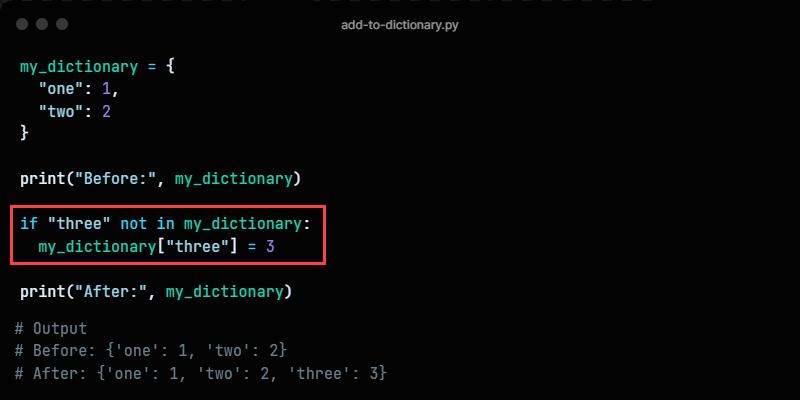
![SOLVED] Check if key exists in object in JS [3 Methods] | GoLinuxCloud Solved] Check If Key Exists In Object In Js [3 Methods] | Golinuxcloud](https://www.golinuxcloud.com/wp-content/uploads/example3.jpg)
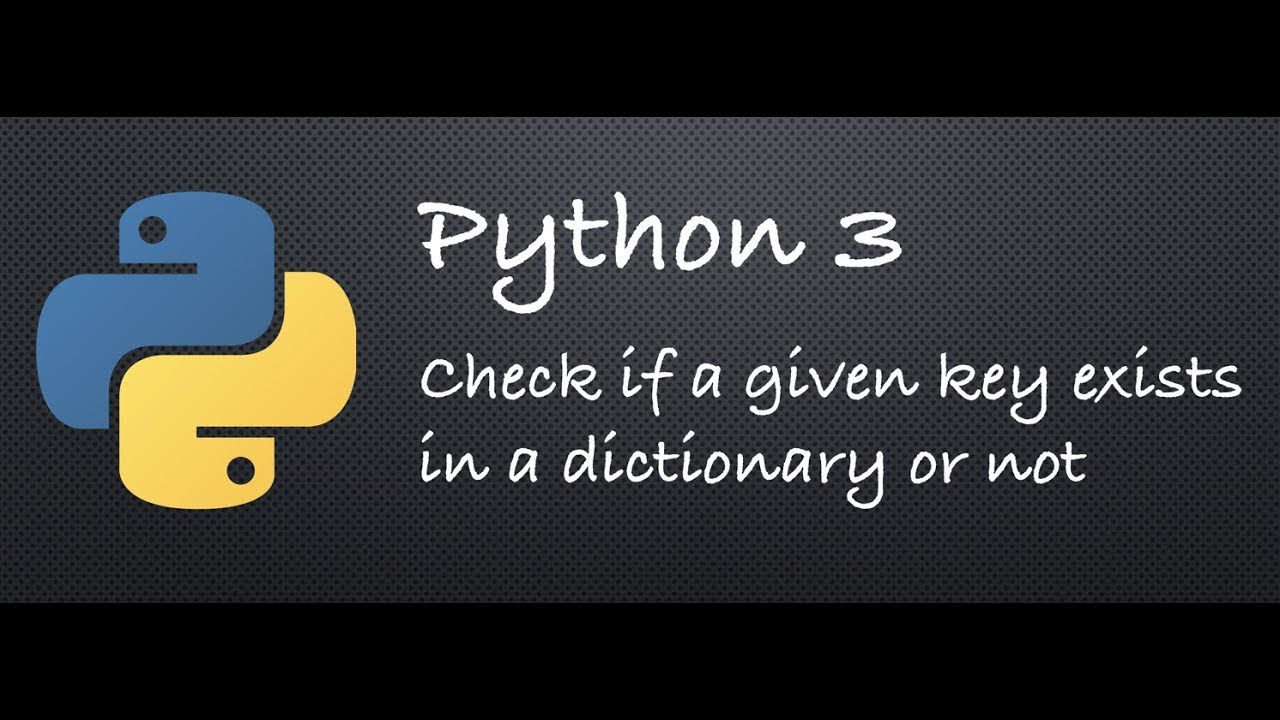
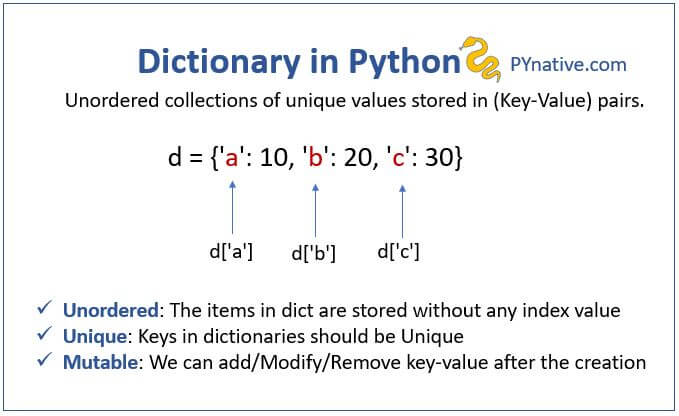
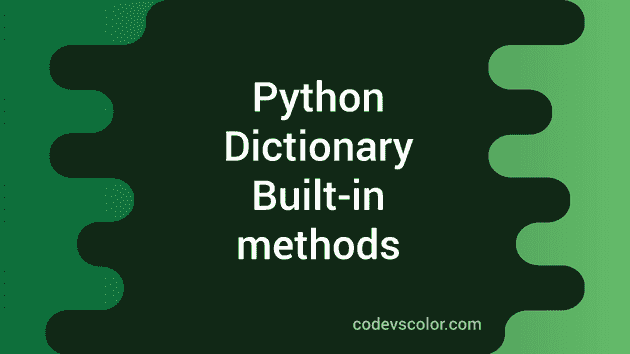
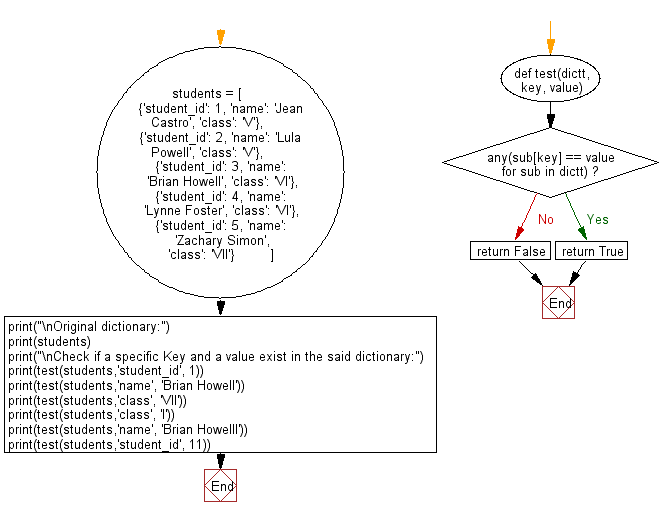
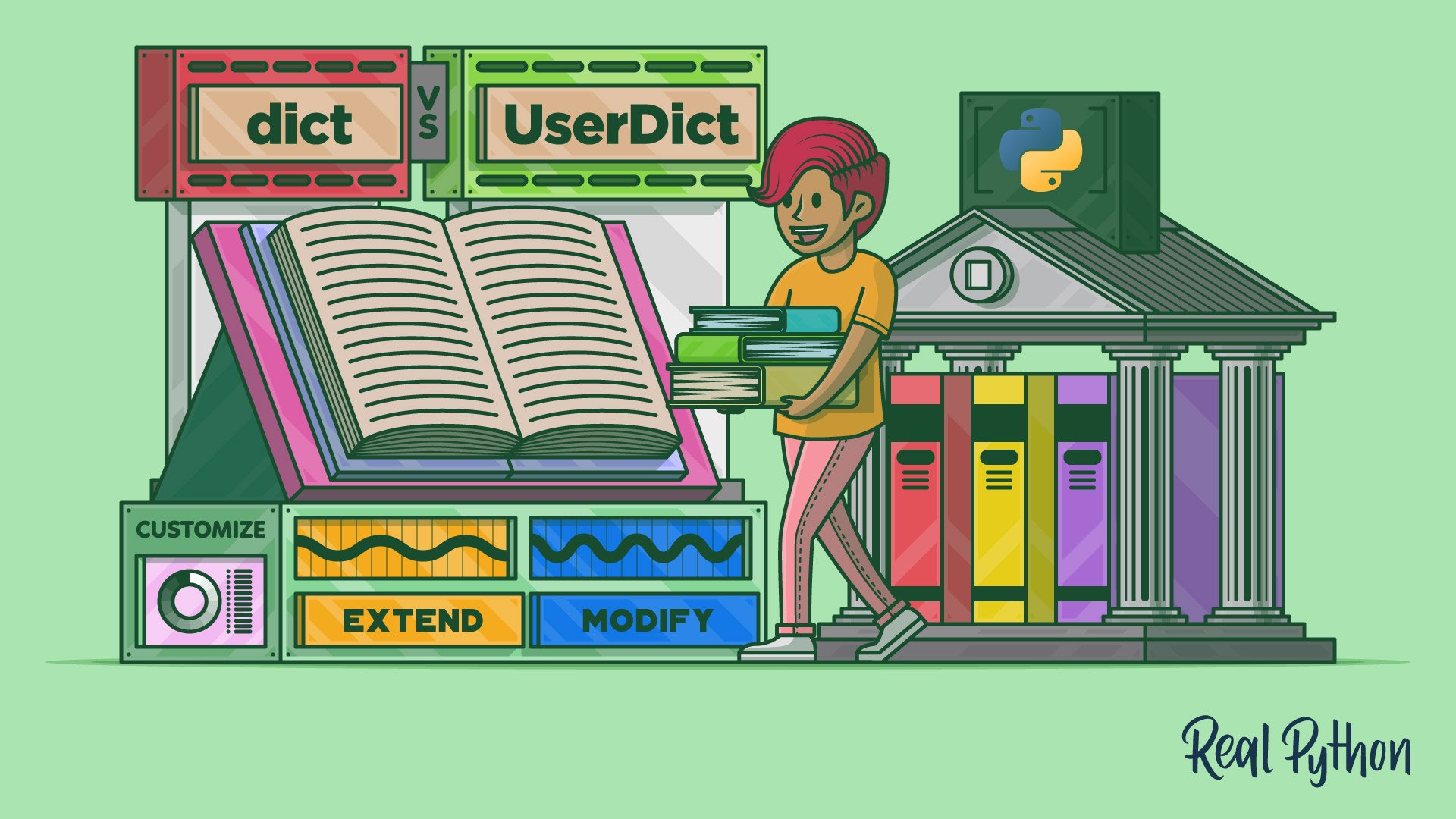
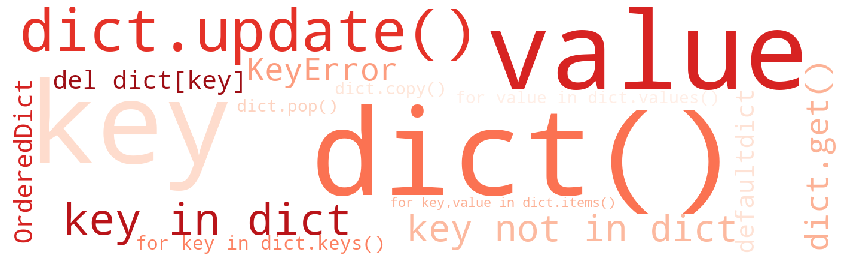
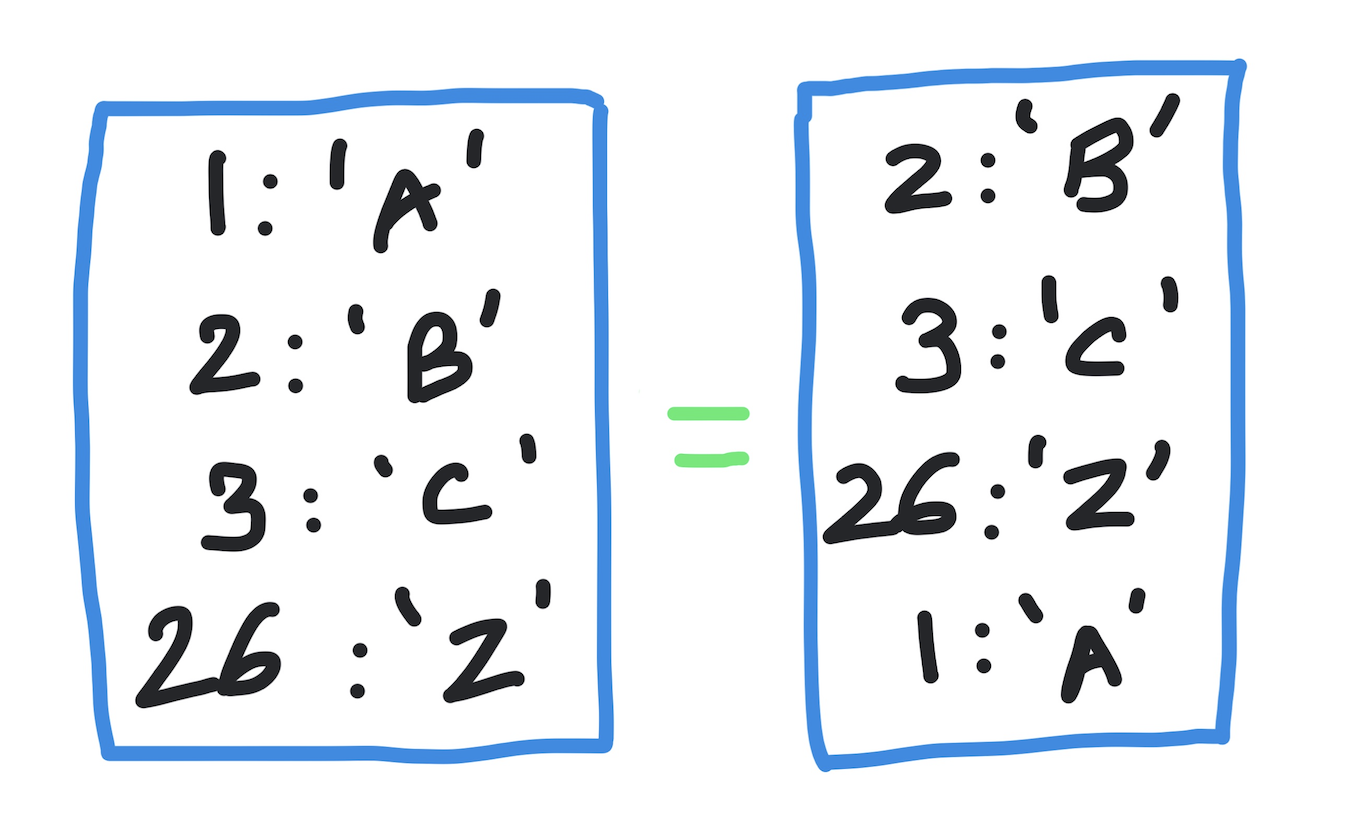
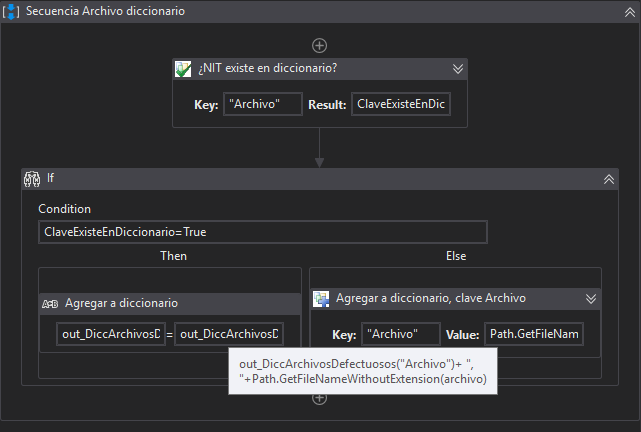
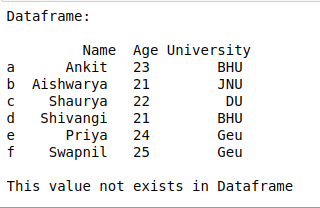
Article link: check if key exists in dictionary python.
Learn more about the topic check if key exists in dictionary python.
- How to check if key exists in a python dictionary? – Flexiple
- Check if Key Exists in Dictionary Python – Scaler Topics
- Check if a given key already exists in a dictionary
- How to check if a key exists in a Python dictionary – Educative.io
- Check whether given Key already exists in a Python Dictionary
- How to Check if a Key Exists in a Python Dictionary – Javatpoint
- Check if key/value exists in dictionary in Python – nkmk note
- Python: Check if Key Exists in Dictionary – Stack Abuse
- Check whether given Key already exists in a Python Dictionary
- Check if Key exists in Dictionary (or Value) with Python code
- How to Check if a Key Exists in a Dictionary in Python
- Python Check if Key Exists in Dictionary – Spark By {Examples}
- How to Check if a Key Exists in a Python Dictionary – Javatpoint