Check If Element In List Python
Python is a powerful programming language that offers various methods to manipulate and operate on lists, one of the most commonly used data structures. One common task while working with lists is checking if a specific element is present in the list. In this article, we will explore different methods to check if an element is in a Python list.
Using the “in” Operator:
The simplest and most common way to check if an element is present in a list is by using the “in” operator. This operator returns a boolean value, True if the element is found in the list, and False otherwise. Let’s see an example:
“`python
my_list = [1, 2, 3, 4, 5]
print(3 in my_list) # Output: True
print(6 in my_list) # Output: False
“`
Using the “not in” Operator:
Similar to the “in” operator, Python provides the “not in” operator to check if an element is not present in a list. It returns True if the element is not found in the list, and False otherwise. Here’s an example:
“`python
my_list = [1, 2, 3, 4, 5]
print(3 not in my_list) # Output: False
print(6 not in my_list) # Output: True
“`
Using the “count()” Method:
The “count()” method allows us to count the occurrences of a specific element in a list. If the count is greater than zero, it means the element is present in the list. Here’s an example:
“`python
my_list = [1, 2, 2, 3, 4, 5]
print(my_list.count(2) > 0) # Output: True
print(my_list.count(6) > 0) # Output: False
“`
Using the “index()” Method:
The “index()” method returns the index of the first occurrence of a given element in a list. If the element is not found, it raises a ValueError. We can utilize this behavior to check if an element exists in a list. If it doesn’t raise an exception, the element is present. Here’s an example:
“`python
my_list = [1, 2, 3, 4, 5]
try:
my_list.index(3)
print(“Element found”)
except ValueError:
print(“Element not found”)
“`
Using the “any()” Function:
The “any()” function takes an iterable as an argument and returns True if at least one element in the iterable is true. We can pass a list as the iterable and utilize the “any()” function to check if an element exists in the list. Here’s an example:
“`python
my_list = [1, 2, 3, 4, 5]
print(any(element == 3 for element in my_list)) # Output: True
print(any(element == 6 for element in my_list)) # Output: False
“`
Using the “all()” Function:
The “all()” function takes an iterable as an argument and returns True if all elements in the iterable are true. Similarly to the “any()” function, we can use the “all()” function to check if an element exists in a list. Here’s an example:
“`python
my_list = [1, 2, 3, 4, 5]
print(all(element == 3 for element in my_list)) # Output: False
print(all(element in my_list for element in [1, 2, 3])) # Output: True
“`
Using the “filter()” Function:
The “filter()” function takes a function and an iterable as arguments, and returns an iterator containing the elements from the iterable for which the function returns True. We can pass a lambda function to filter the list and check if any elements match the desired element. Here’s an example:
“`python
my_list = [1, 2, 3, 4, 5]
filtered_list = filter(lambda x: x == 3, my_list)
print(len(list(filtered_list)) > 0) # Output: True
filtered_list = filter(lambda x: x == 6, my_list)
print(len(list(filtered_list)) > 0) # Output: False
“`
Using the “set()” Function:
Converting the list into a set and then performing membership checking can be an efficient way to check if an element exists in a list. The “set()” function removes duplicate elements from the list and converts it into a set. Here’s an example:
“`python
my_list = [1, 2, 3, 4, 5]
print(3 in set(my_list)) # Output: True
print(6 in set(my_list)) # Output: False
“`
Using the “numpy.isin()” Function:
If you are working with numerical data and have the numpy library imported, you can use the “numpy.isin()” function to check if an element exists in a list. This function returns a boolean array representing whether each element is found in the given list. Here’s an example:
“`python
import numpy as np
my_list = [1, 2, 3, 4, 5]
print(np.isin(3, my_list)) # Output: True
print(np.isin(6, my_list)) # Output: False
“`
These methods provide different ways to check if an element exists in a Python list. Depending on the context and requirements of your code, you can choose the most suitable method for your needs. Experiment with different methods and see which one fits best for your specific situation.
FAQs
Q: How do I check if a list is present in another list in Python?
A: To check if one list is present in another list, you can use the “in” operator or “issubset()” method. The “in” operator checks if every element in the first list is present in the second list, while the “issubset()” method checks if all elements in the first list are in the second list.
Q: How do I check if a string is present in a list in Python?
A: To check if a string is present in a list, you can use the “in” operator. It returns True if the string is found in the list, and False otherwise. For example, `print(“apple” in [“banana”, “apple”, “orange”])` will output True.
Q: How do I get an element from a list in Python?
A: To get an element from a list, you can use indexing. Each element in the list has an index starting from 0. By specifying the index in square brackets, you can access the desired element. For example, `my_list = [1, 2, 3]` and `print(my_list[0])` will output 1.
Q: How do I check if multiple values exist in a list in Python?
A: To check if multiple values exist in a list, you can use the “all()” function with a list comprehension. The list comprehension checks each value against the list and returns True only if all values exist in the list. For example, `my_list = [1, 2, 3, 4, 5]` and `print(all(x in my_list for x in [2, 4]))` will output True.
Q: How do I add a new element to a list in Python?
A: To add a new element to a list, you can use the “append()” method or the “+” operator. The “append()” method adds the element to the end of the list, while the “+” operator concatenates two lists. For example, `my_list = [1, 2]` and `my_list.append(3)` or `my_list = my_list + [3]` will add 3 to the list.
Ways To Check If An Element Is In A Python List
Keywords searched by users: check if element in list python Check list in list Python, Find element in list Python, Python check element in list in another list, Check if string in list Python, Get element in list Python, Check multiple values in list python, Not in list Python, Add new element to python list
Categories: Top 73 Check If Element In List Python
See more here: nhanvietluanvan.com
Check List In List Python
Python is an incredibly versatile programming language that offers numerous useful data structures and functionalities. One of the most commonly used data structures in Python is the list. Lists allow you to store and organize multiple items of different types, making them a powerful tool for various applications. In this article, we will explore the concept of checklist in list Python, how to create them, perform operations, and address some frequently asked questions.
What is a checklist in list Python?
A checklist in list Python refers to a list that contains elements that represent items that need to be checked off or marked as completed. This can be particularly useful when you need to keep track of multiple tasks, to-do items, or any other group of items that need to be managed systematically. With a checklist, you can easily add, remove, and modify items, as well as mark them as complete or incomplete.
Creating a checklist in list Python
To create a checklist in Python, you simply need to initialize an empty list. Here’s an example:
“`python
checklist = []
“`
Now that we have an empty list, we can start adding items to it. Each item can be represented as a string, which makes sense for most use cases. For example, let’s say we want to create a checklist for groceries:
“`python
checklist = [‘Apples’, ‘Milk’, ‘Bread’, ‘Eggs’]
“`
In this case, our checklist contains four items: apples, milk, bread, and eggs. Note that the items are enclosed in single quotes and separated by commas.
Performing operations on a checklist
Once we have a checklist, we often need to perform various operations on it. Let’s explore some common operations:
1. Adding items: To add an item to the checklist, we can use the `append()` function. For example, to add “Butter” to our grocery checklist, we can do:
“`python
checklist.append(‘Butter’)
“`
2. Removing items: To remove an item from the checklist, we can use the `remove()` function. For example, if we want to remove “Milk” from our grocery checklist, we can do:
“`python
checklist.remove(‘Milk’)
“`
3. Marking items as complete: One way to represent completeness is by using a separate list to store completed items. Let’s say we have a separate list called `completed` and we want to mark “Bread” as complete. We can do:
“`python
completed.append(checklist.pop(checklist.index(‘Bread’)))
“`
In this case, the `pop()` function is used to remove “Bread” from the checklist and the `index()` function is used to find its position. The removed item is then appended to the `completed` list.
4. Checking if an item exists: To check if an item exists in the checklist, we can use the `in` operator. For example, to check if “Eggs” is in our grocery checklist, we can do:
“`python
if ‘Eggs’ in checklist:
print(“Eggs is in the checklist!”)
“`
This will print “Eggs is in the checklist!” if the item exists.
5. Checking the length of the checklist: To check how many items are in the checklist, we can use the `len()` function. For example, to get the length of our grocery checklist, we can do:
“`python
print(len(checklist))
“`
This will print the number of items in the checklist.
FAQs
Q: Can a checklist contain items of different types?
A: Yes, a checklist can contain items of different types, including strings, numbers, or even other lists.
Q: How can I sort the items in a checklist?
A: To sort the items in a checklist, you can use the `sort()` function. For example, to sort the grocery checklist alphabetically, you can do:
“`python
checklist.sort()
“`
Q: Can I have duplicate items in a checklist?
A: Yes, Python allows duplicate items in a list. If you want to prevent duplicates, you can use a separate data structure, such as a set, which guarantees uniqueness.
Q: Are there other data structures I can use for checklists?
A: Yes, apart from lists, you can also use other data structures like arrays or dictionaries to represent checklists. Each data structure has its own advantages and disadvantages, so choose the one that best suits your needs.
In conclusion, checklists in list Python are a handy way to manage and organize collections of items. We explored how to create checklists, perform operations, and addressed some frequently asked questions. Armed with this knowledge, you can now efficiently construct and manage your own checklists using the power of Python.
Find Element In List Python
Python provides several built-in functions and methods that can be used to find elements in a list. One of the simplest methods is the use of the ‘in’ operator. This operator returns a boolean value indicating whether a particular element is present in the list or not. It can be used in a simple if statement to validate if an element exists in the list:
“`
my_list = [1, 2, 3, 4, 5]
if 3 in my_list:
print(“Element found!”)
“`
This method works well when you simply need to check the presence of an element. However, if you also require to know the index of the element, you can use the ‘index’ method. The ‘index’ method returns the index of the first occurrence of the specified element in the list:
“`
my_list = [1, 2, 3, 4, 5]
element = 3
index = my_list.index(element)
print(“Element”, element, “found at index”, index)
“`
It’s important to note that if the element is not present in the list, a ‘ValueError’ will be raised. To handle this situation, you can enclose the ‘index’ method call within a try-except block:
“`
my_list = [1, 2, 3, 4, 5]
element = 6
try:
index = my_list.index(element)
print(“Element”, element, “found at index”, index)
except ValueError:
print(“Element not found in the list.”)
“`
In addition to the above methods, there are also other techniques that can be used to find elements in a list based on different criteria. For example, if you want to find all occurrences of a particular element in a list, you can use a list comprehension:
“`
my_list = [1, 2, 3, 4, 5, 2, 3]
element = 2
indices = [i for i, x in enumerate(my_list) if x == element]
print(“Element”, element, “found at indices”, indices)
“`
This method iterates over the list using ‘enumerate’ to get both the index and value of each element. The condition ‘x == element’ is used to filter elements that match the desired element. The resulting indices are stored in the ‘indices’ list.
Another technique involves using the ‘count’ method to determine the number of occurrences of a given element in a list:
“`
my_list = [1, 2, 3, 4, 5, 2, 3]
element = 2
count = my_list.count(element)
print(“Element”, element, “found”, count, “times in the list”)
“`
The ‘count’ method returns the number of occurrences of an element without providing the indices. It can be useful when you only need to know the frequency of a particular element in the list.
Frequently Asked Questions:
Q: What is the most efficient way to find an element in a large list?
A: The most efficient way to find an element in a large list is by using a technique called binary search. However, binary search requires the list to be sorted. If your list is not already sorted, sorting it first using the ‘sort’ method or another sorting algorithm would be necessary before applying binary search.
Q: What should I do if I need to find elements in a list based on complex conditions?
A: In scenarios where complex conditions are involved, such as searching for elements based on multiple criteria, you can use the ‘filter’ function or list comprehensions with more complex filtering conditions. These methods provide flexibility in defining custom conditions to find elements in a list.
Q: Can I find elements in a list using regular expressions?
A: Yes, you can use regular expressions to find elements in a list by iterating over the elements and using the ‘re’ module in Python. You can apply regular expressions to match patterns within the elements, allowing you to filter and find specific elements accordingly.
In conclusion, Python provides several methods and techniques to find elements in a list. The ‘in’ operator, ‘index’ method, list comprehensions, and ‘count’ method are some of the common tools that can be used for this purpose. The choice of method depends on the specific requirements and constraints of your programming task. By understanding these techniques, you can efficiently locate elements within your Python lists and achieve better control over your program’s logic.
Images related to the topic check if element in list python
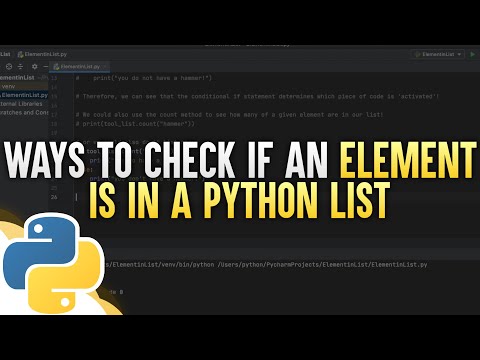
Found 48 images related to check if element in list python theme
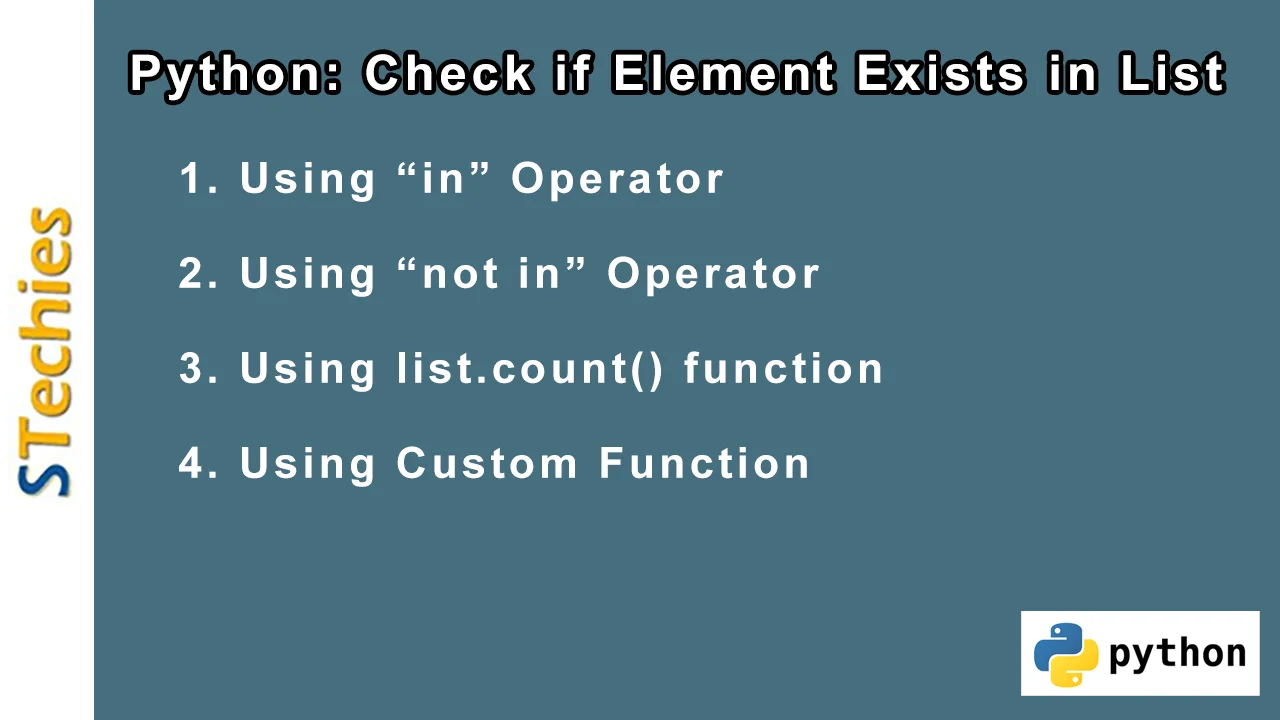
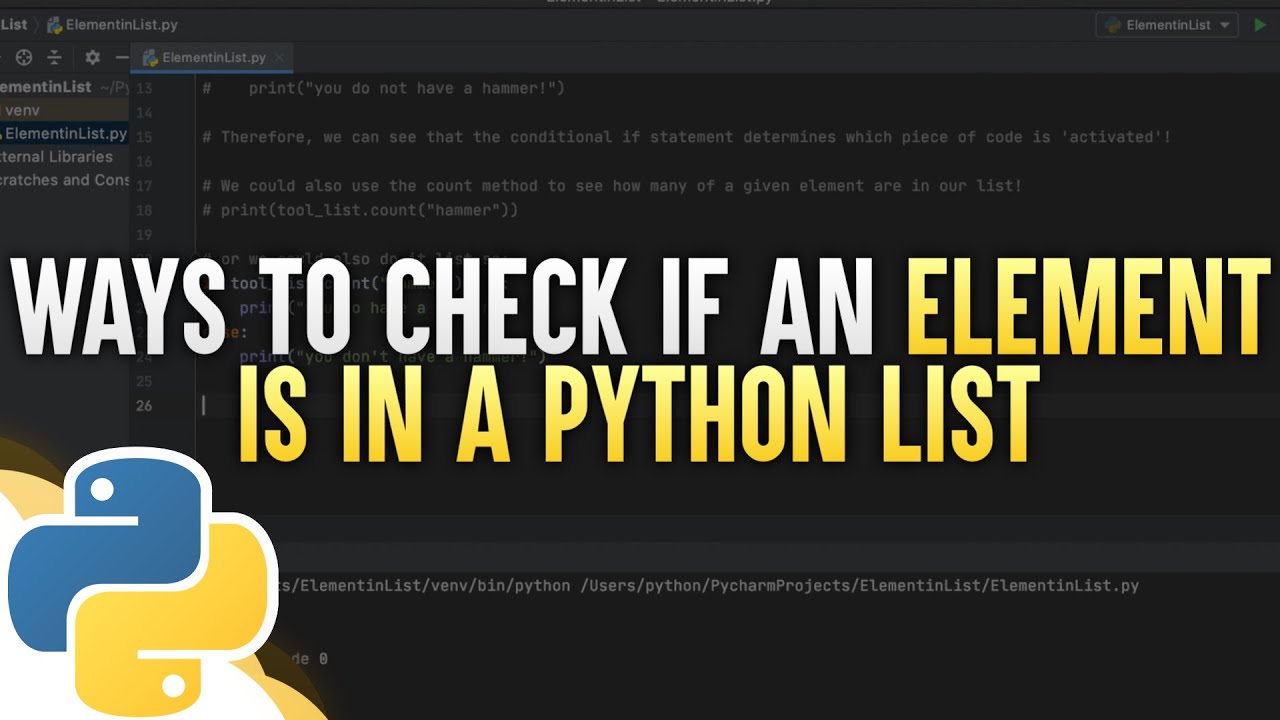


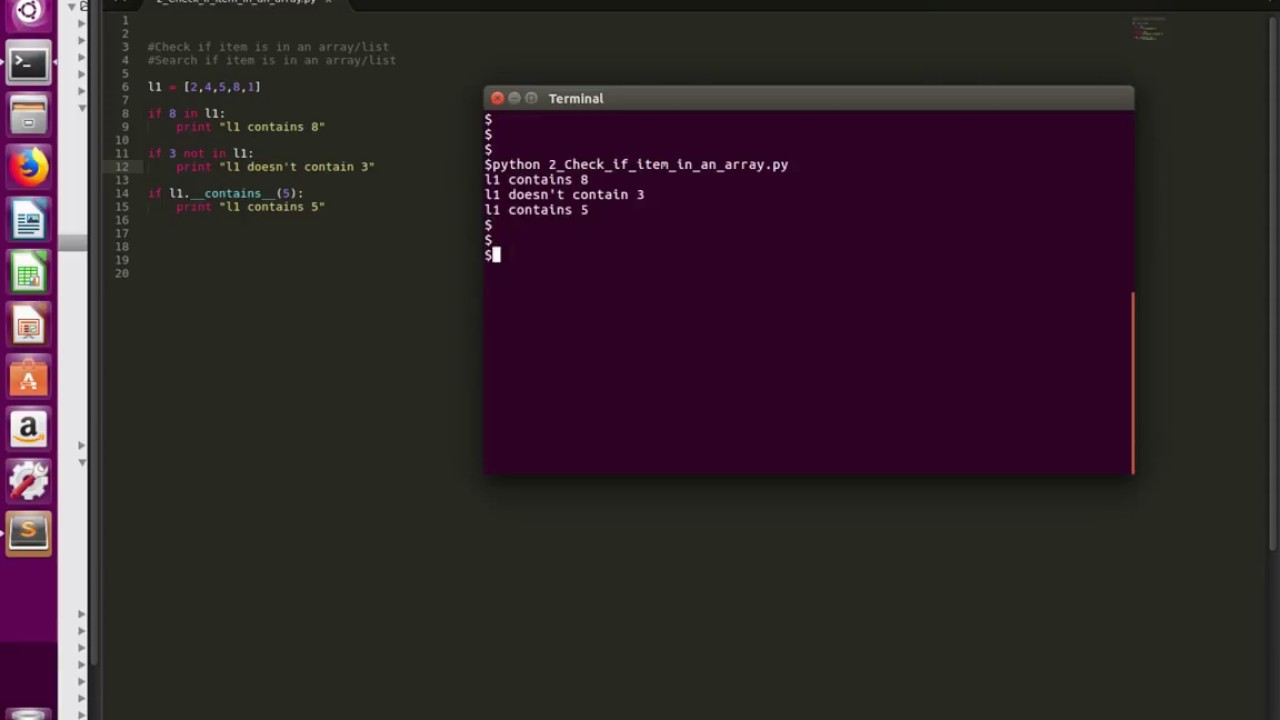

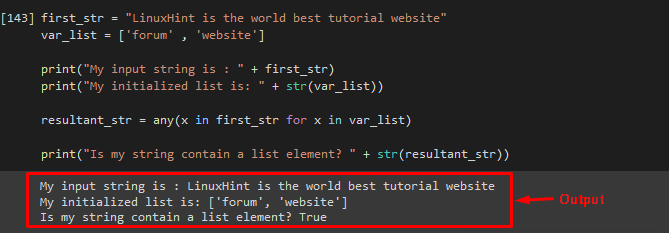
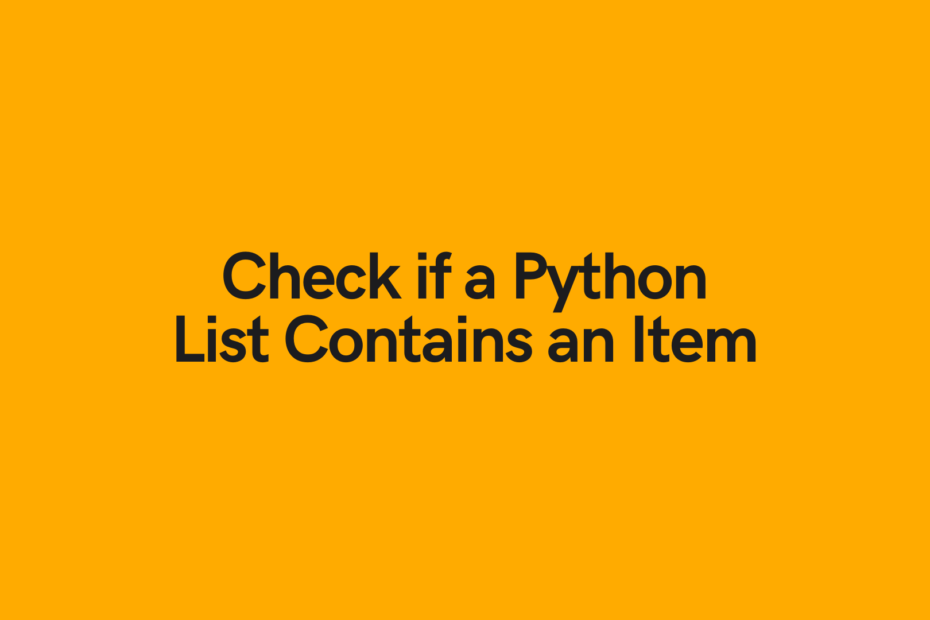
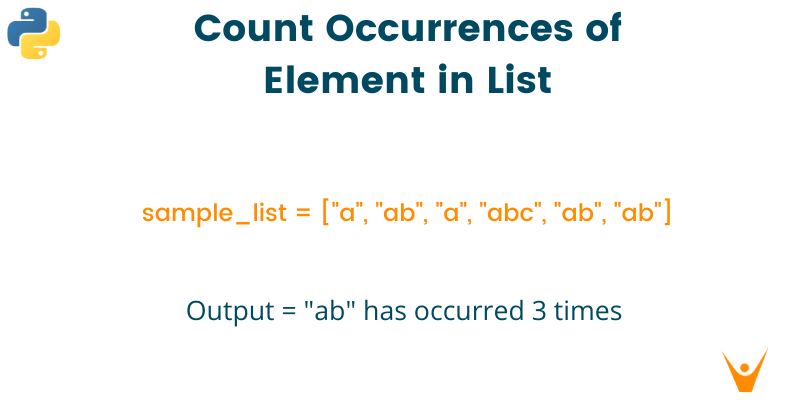
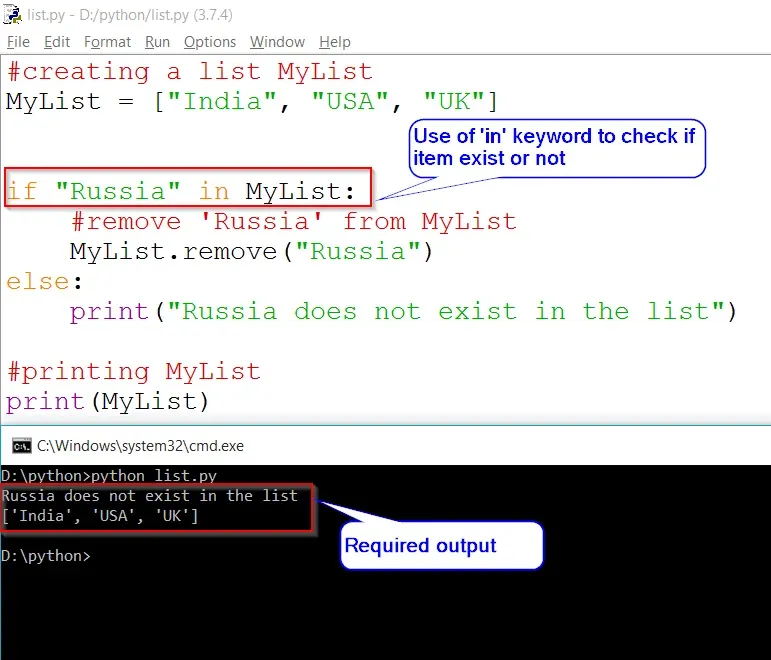

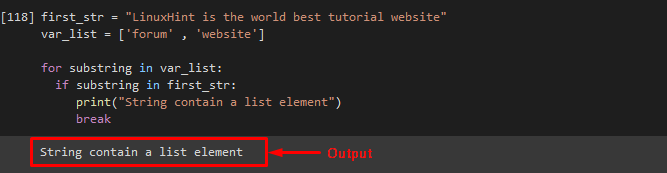
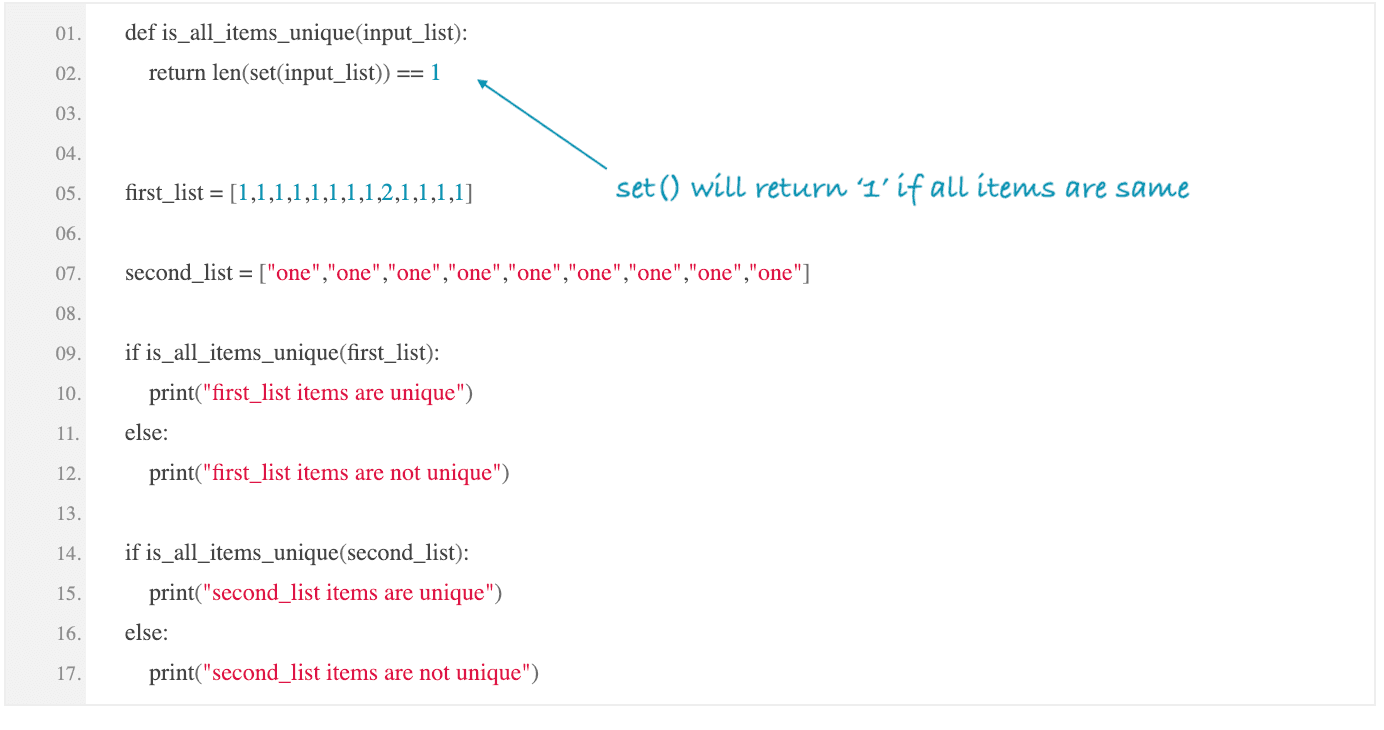

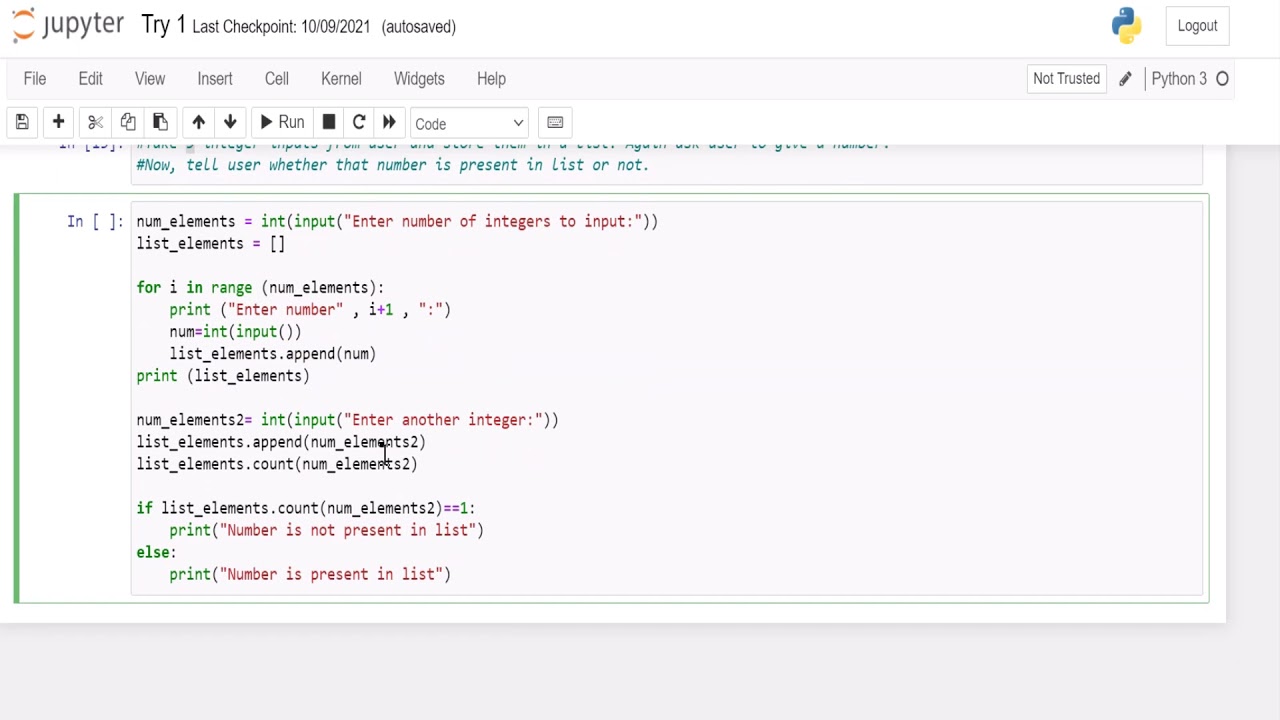
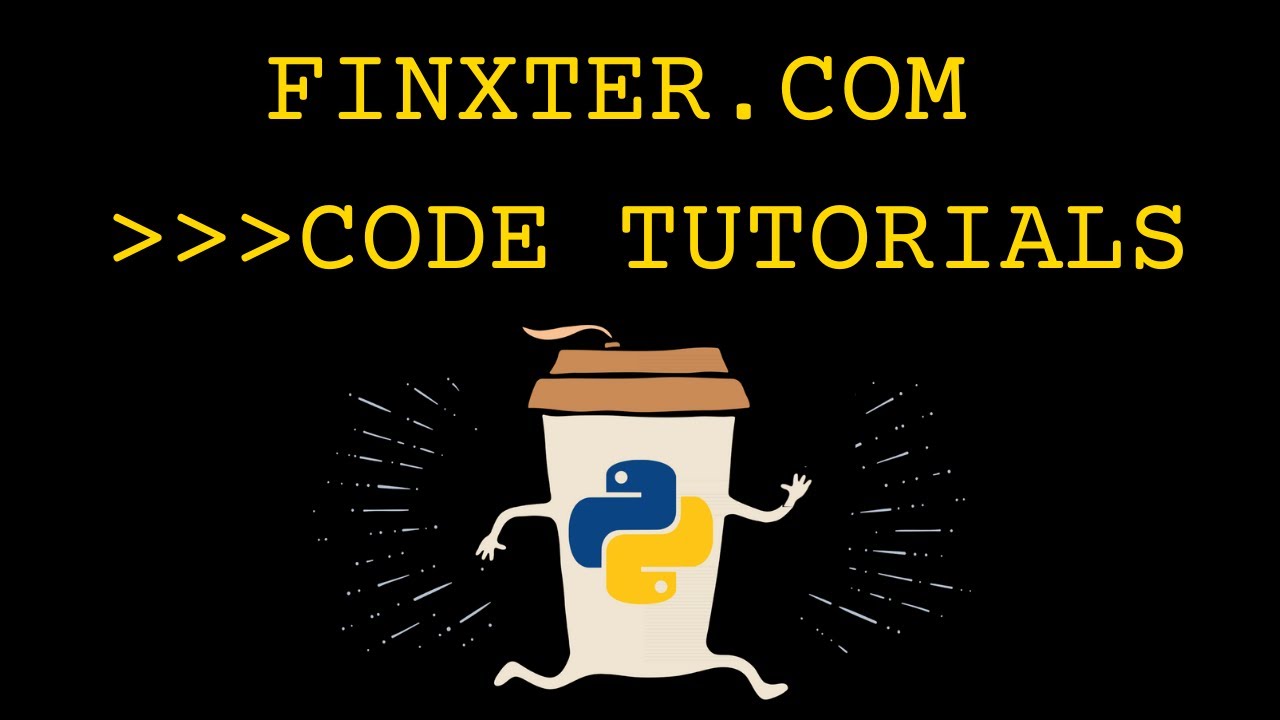

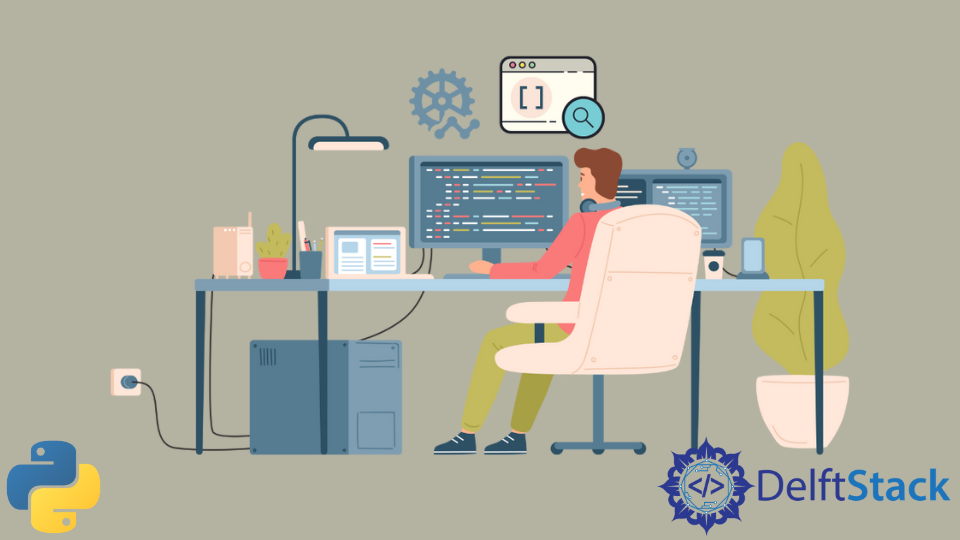
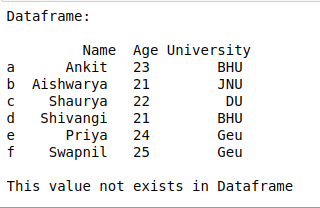
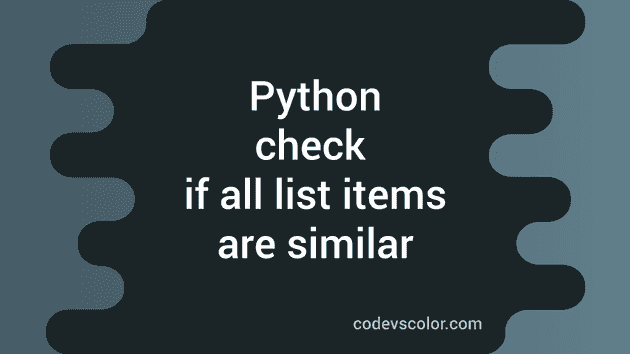
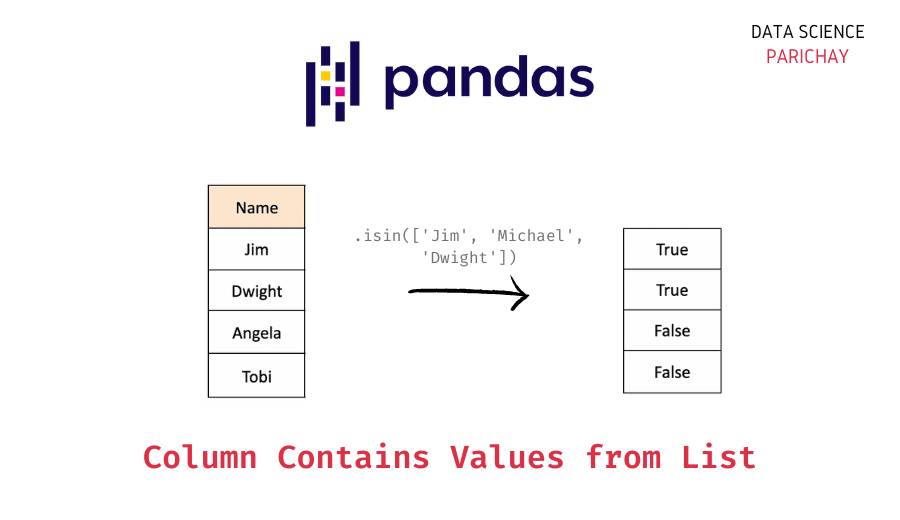
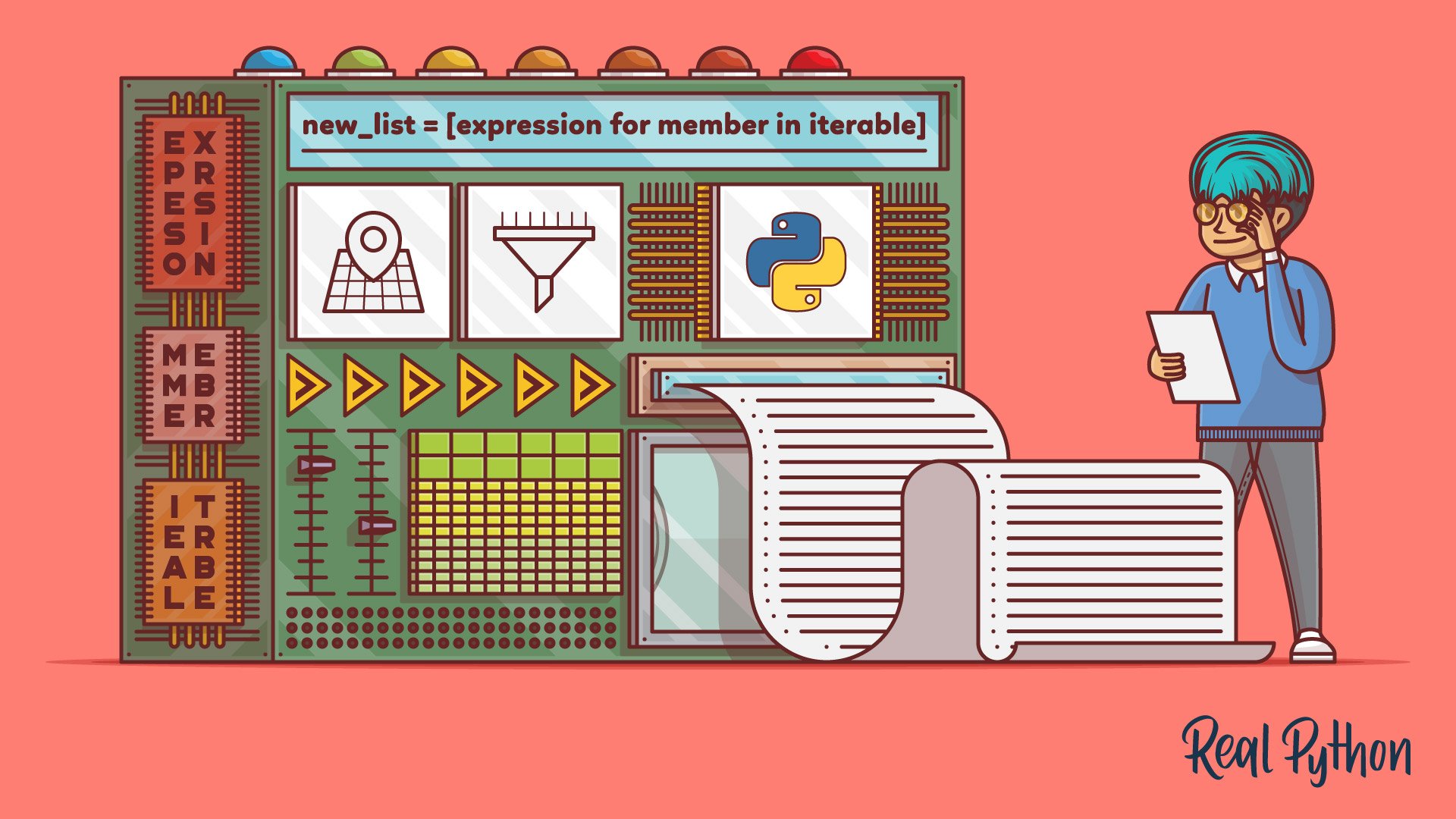

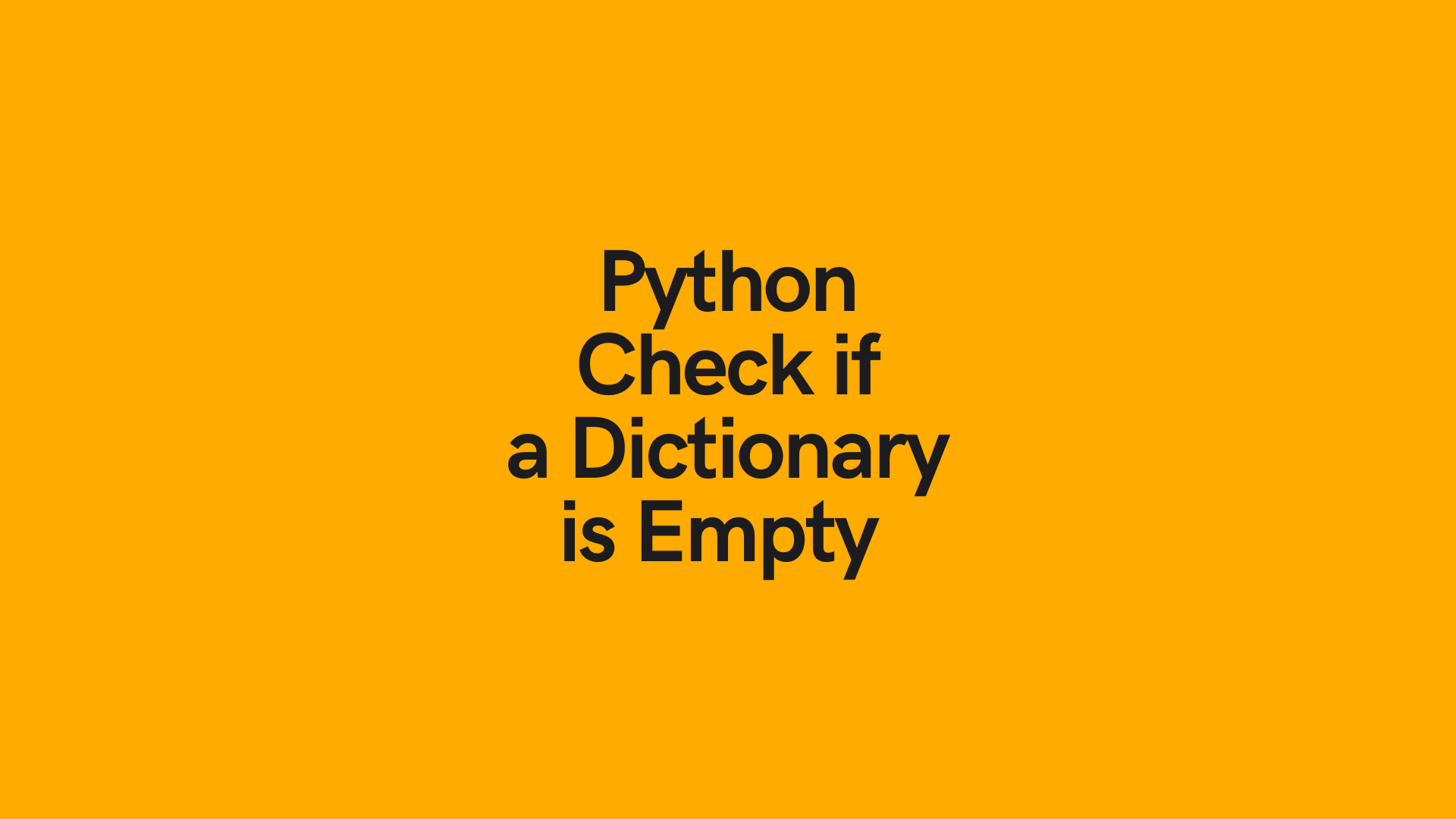

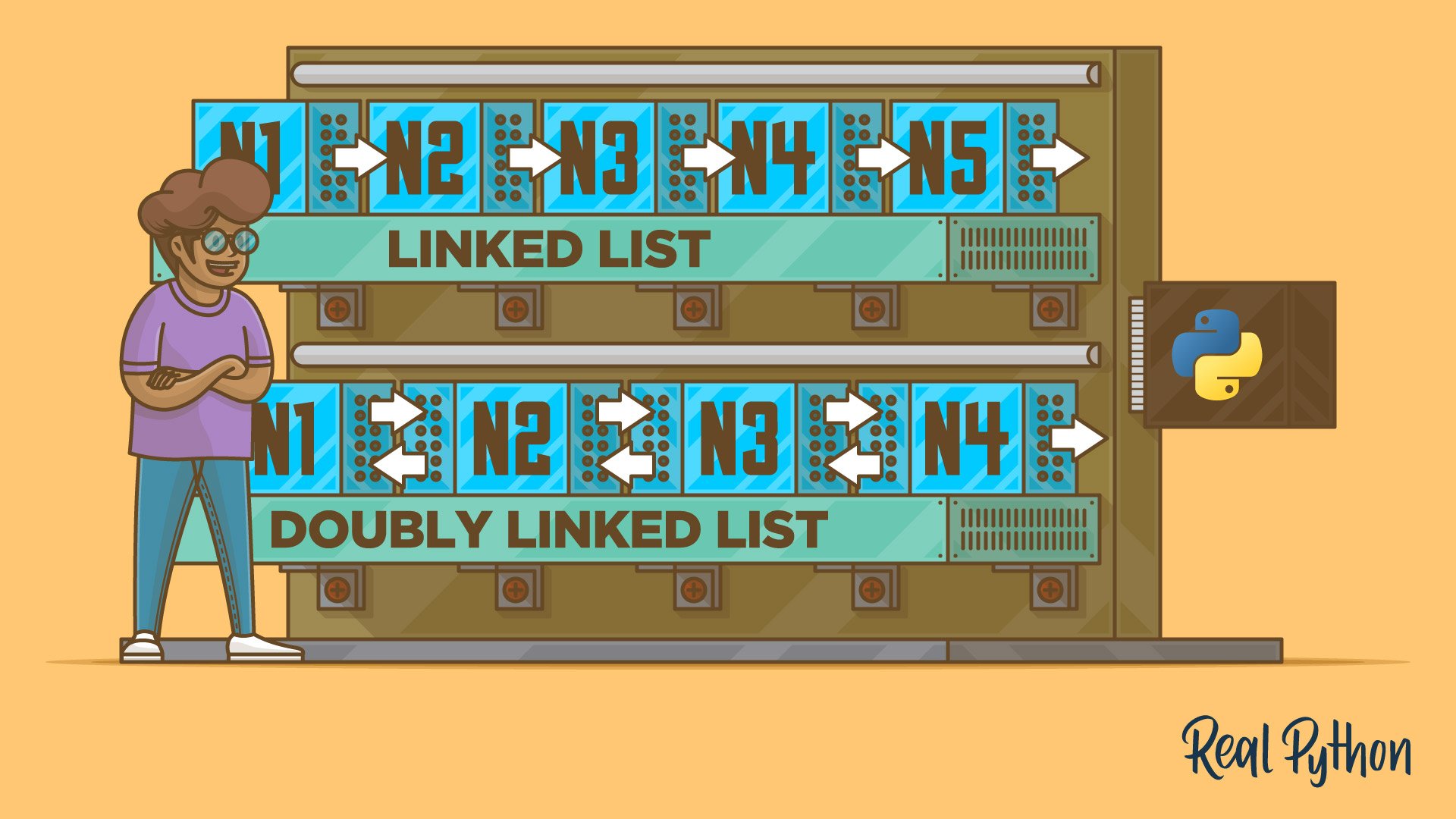
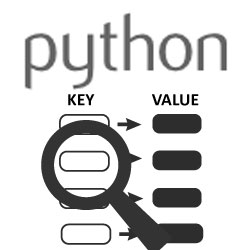
![Python list contains: How to check if an item exists in list? | Flexiple Tutorials | Python Solved: Task 1. [Checking If A List Is Sorted] For This Task, You Will Write A Function To Test Whether A List Is Sorted. You Need To Write The Following Function: Issorted(Alist) ->” style=”width:100%” title=”SOLVED: Task 1. [Checking if a list is sorted] For this task, you will write a function to test whether a list is sorted. You need to write the following function: issorted(alist) ->”><figcaption>Solved: Task 1. [Checking If A List Is Sorted] For This Task, You Will Write A Function To Test Whether A List Is Sorted. You Need To Write The Following Function: Issorted(Alist) -></figcaption></figure>
<figure><img decoding=](https://cdn.numerade.com/ask_images/5d0b21f8e7174c99b52dc05cd1e53ec9.jpg)
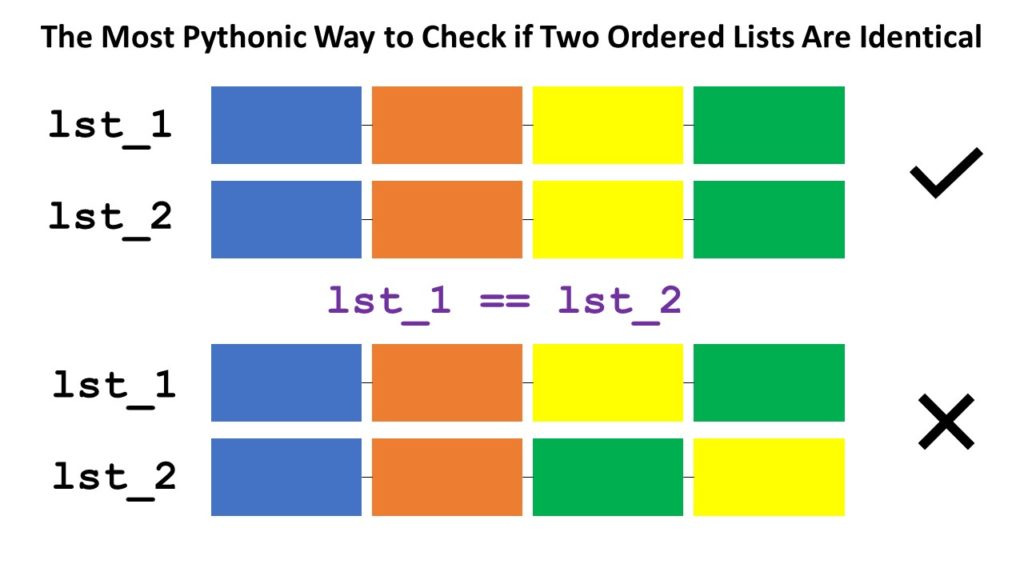

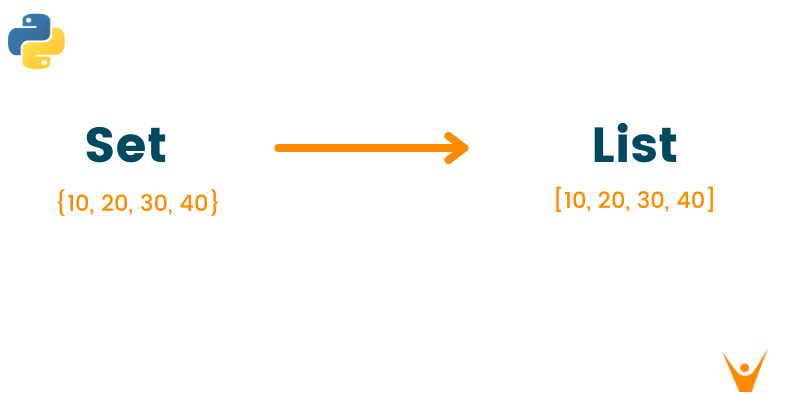

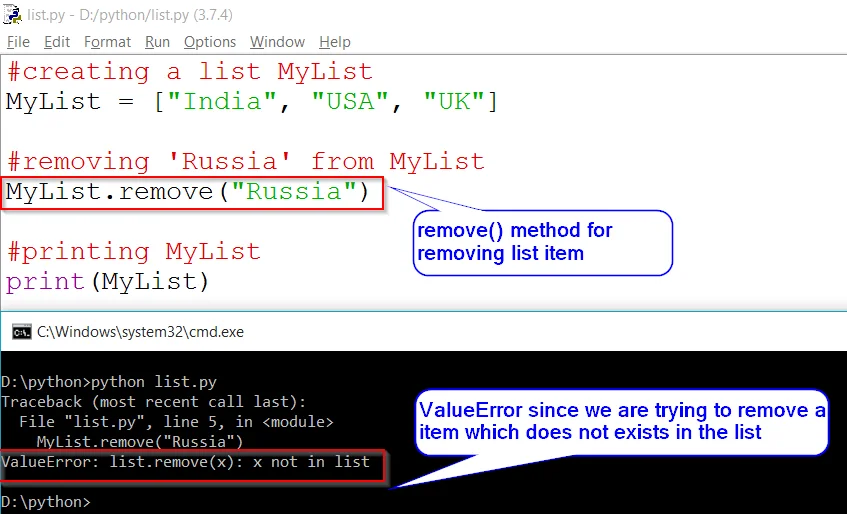

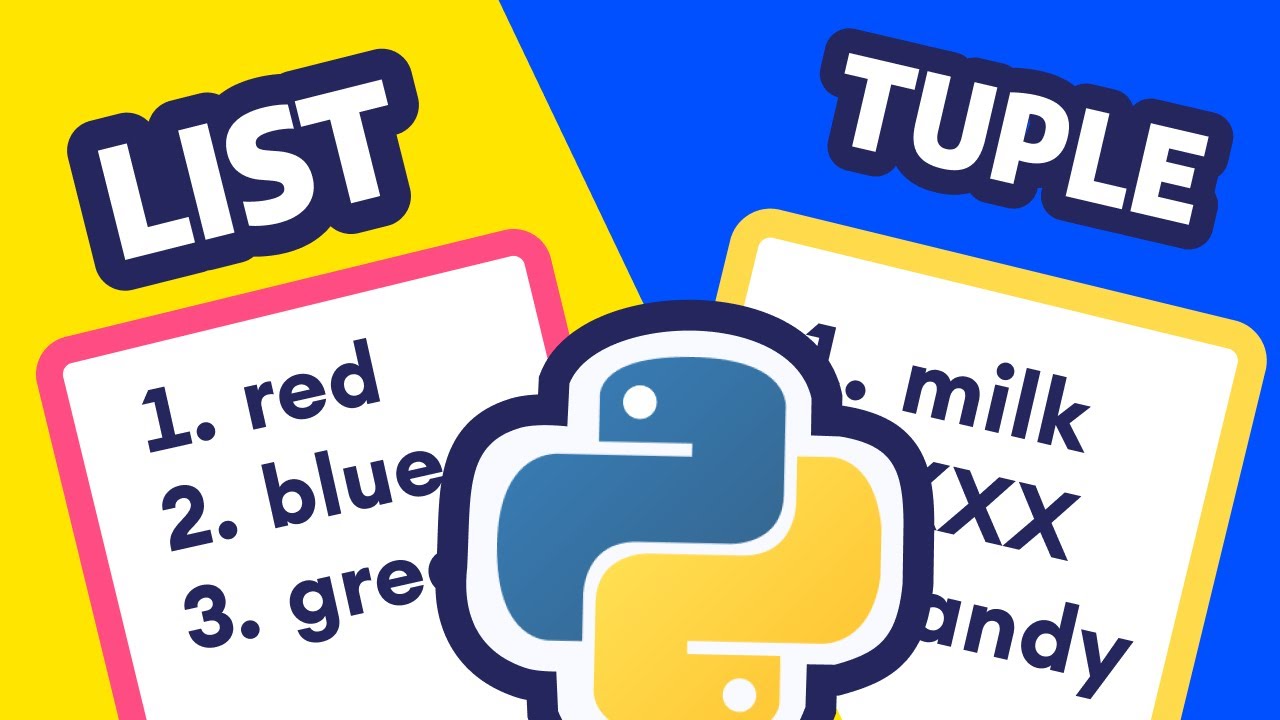


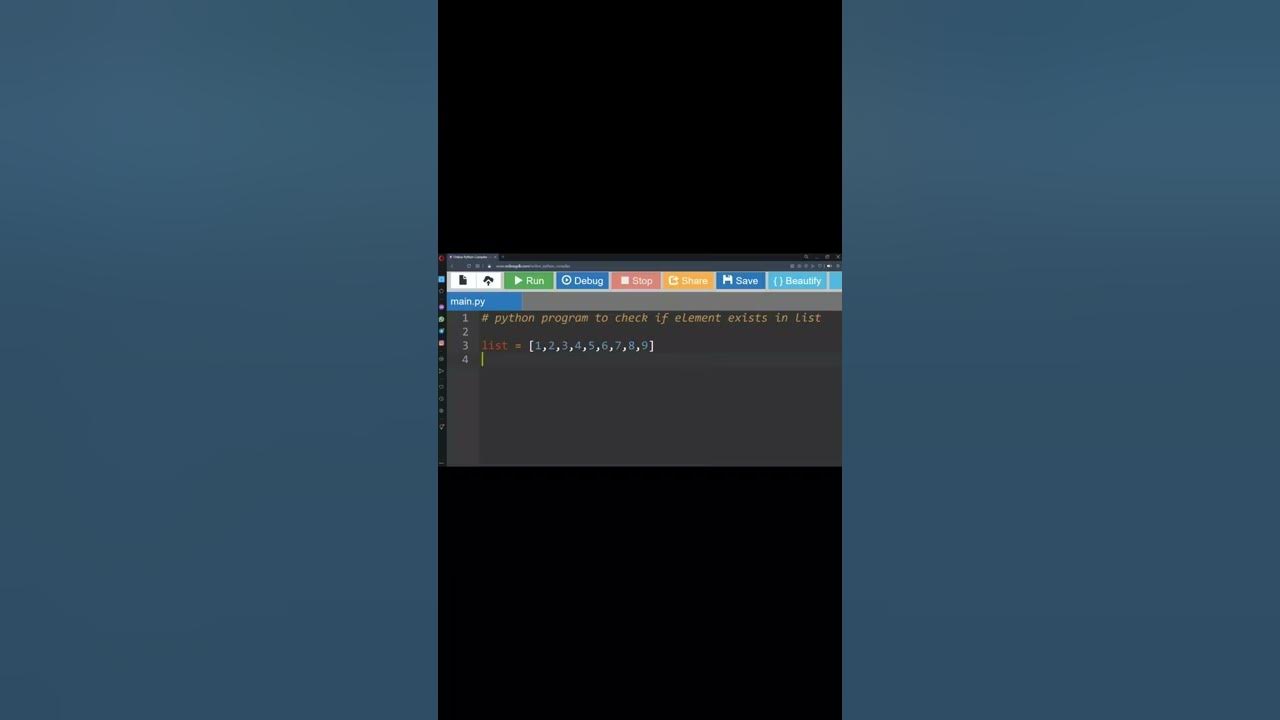
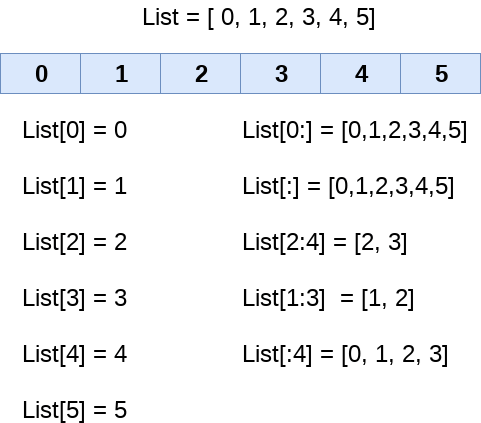
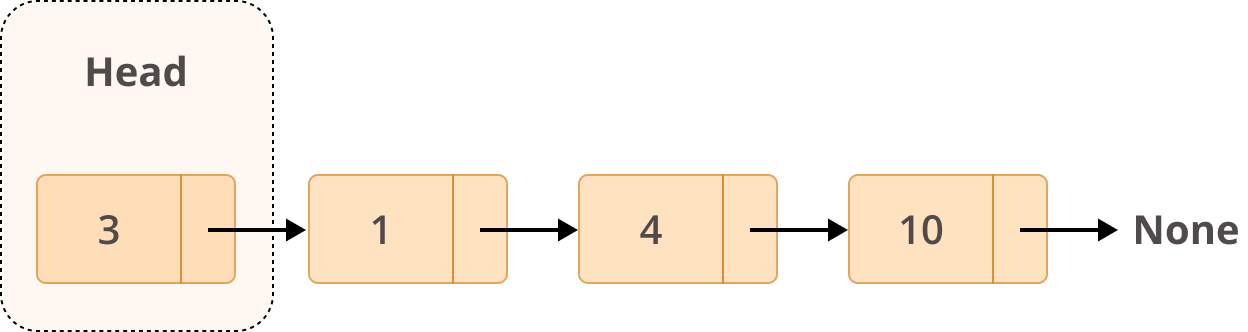

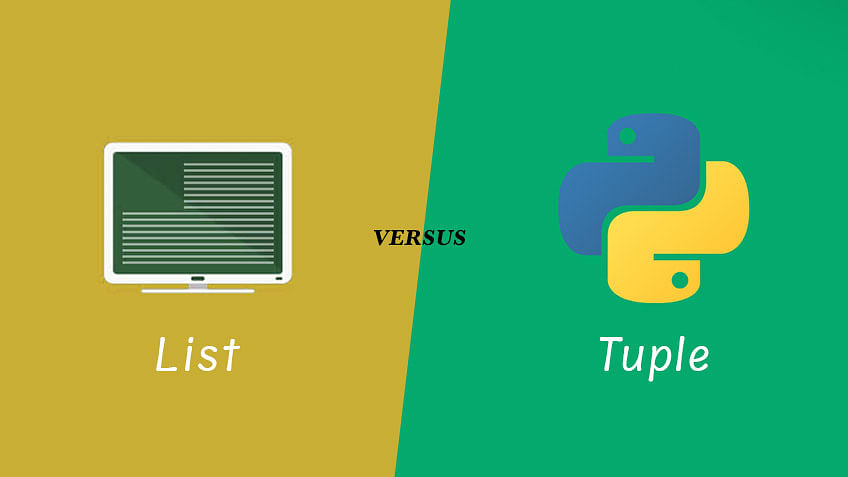

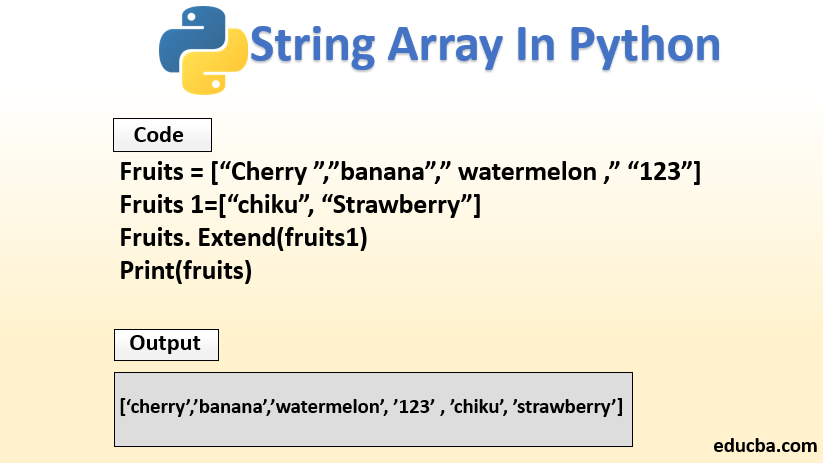
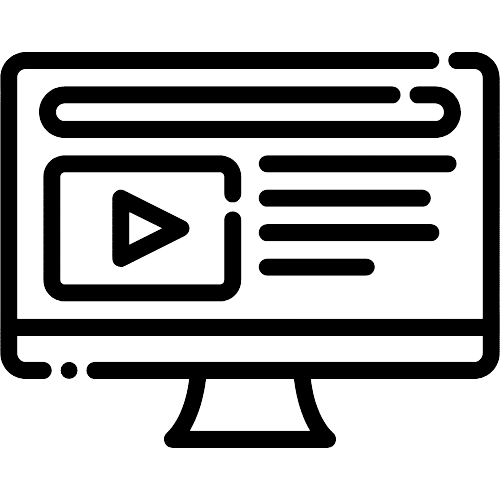
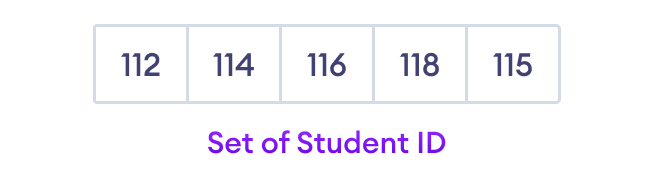
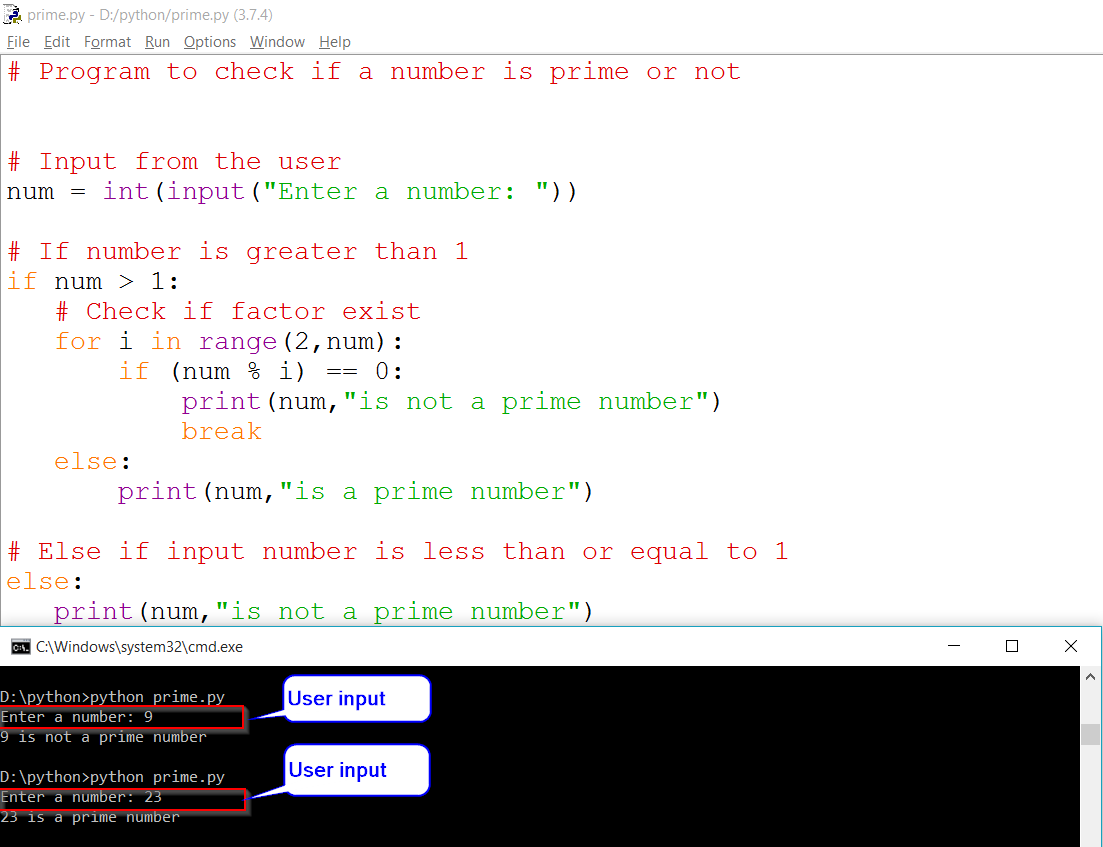
Article link: check if element in list python.
Learn more about the topic check if element in list python.
- Check if element exists in list in Python – GeeksforGeeks
- Python list contains: How to check if an item exists in list?
- Fastest way to check if a value exists in a list – Stack Overflow
- Python List Contains: How to Check If Item Exists in List
- Python : How to Check if an item exists in list – thisPointer
- Python List Contains – Check if Element Exists in List
- How to Check if Element Exists in List in Python – Javatpoint
- Python: Check if Element Exists in List – STechies
- Check if all/any elements in List meet condition in Python
- Check if item exists in a list using python – Devsheet
See more: blog https://nhanvietluanvan.com/luat-hoc