Cannot Make A Static Reference To The Non Static Method
In the world of programming, it is essential to understand the difference between static and non-static methods. These concepts play a significant role in defining how a method can be accessed and utilized within a program. In some instances, you may encounter an error message stating, “cannot make a static reference to a non-static method.” This error occurs when you attempt to access a non-static method from a static method or context. In this article, we will delve into the reasons behind this error, how to address it, and additional best practices for working with static and non-static methods.
What is a Static Method?
To grasp the concept of a static reference to a non-static method, we must first understand what a static method is. In programming, a static method belongs to the class itself rather than an instance (object) of the class. It means that you can access and call a static method directly from the class, without creating an object of that class.
Here’s an example of a static method:
“`java
public class MathUtil {
public static int add(int a, int b) {
return a + b;
}
}
“`
In this example, the method “add” is defined as static within the “MathUtil” class. It can be accessed and called like this:
“`java
int sum = MathUtil.add(5, 7);
System.out.println(sum); // Output: 12
“`
What is a Non-Static Method?
On the other hand, a non-static method belongs to an instance of a class. It can only be accessed and called through an object of that class. Non-static methods can access and modify instance variables and other non-static methods within the class.
Here’s an example of a non-static method:
“`java
public class Circle {
public double calculateArea(double radius) {
return Math.PI * radius * radius;
}
}
“`
In this example, the method “calculateArea” is a non-static method within the “Circle” class. It can be accessed and called only through an object of the “Circle” class.
“`java
Circle myCircle = new Circle();
double area = myCircle.calculateArea(3.5);
System.out.println(area); // Output: 38.48451000647496
“`
What does it mean to make a static reference to a non-static method?
When a method is defined as non-static, it means that its behavior and functionality depend on the specific instance (object) of the class. Non-static methods can access instance variables and other non-static methods associated with the class.
On the other hand, a static method does not rely on any instance of the class and can be accessed and called directly from the class itself, as we mentioned earlier.
Making a static reference to a non-static method means that you are trying to access or call a non-static method directly from a static context, such as a static method or a static block. This is not allowed because a non-static method requires an instance of the class to provide the necessary context for its execution.
Why does making a static reference to a non-static method result in an error?
When you try to make a static reference to a non-static method, the compiler throws an error because it violates the fundamental concept of non-static methods needing an instance (object) to operate correctly. Making a static reference to a non-static method is analogous to trying to drive a car without having a car in the first place.
For example, consider the following code snippet:
“`java
public class Example {
public void nonStaticMethod() {
// Non-static method implementation
}
public static void main(String[] args) {
nonStaticMethod(); // Error: Cannot make a static reference to a non-static method
}
}
“`
In this example, the non-static method “nonStaticMethod” is being called directly from the static `main` method. Since the `main` method is static, it does not have any association with a specific instance of the class. Thus, it cannot directly access non-static methods.
How to fix the “cannot make a static reference to a non-static method” error?
To resolve the “cannot make a static reference to a non-static method” error, you have two primary options:
1. Create an instance of the class: As non-static methods require an instance of the class, you can create an object of the class and then call the non-static method on that object.
“`java
public class Example {
public void nonStaticMethod() {
// Non-static method implementation
}
public static void main(String[] args) {
Example example = new Example();
example.nonStaticMethod(); // Call non-static method on the instance of the class
}
}
“`
In this example, we create an object of the `Example` class named `example` and then call the non-static method `nonStaticMethod` on that object.
2. Make the non-static method static: If making the non-static method static aligns with the logic and requirements of your program, you can change the method’s definition to `static`. This allows the method to be called directly from a static context.
“`java
public class Example {
public static void nonStaticMethod() {
// Non-static method implementation
}
public static void main(String[] args) {
nonStaticMethod(); // Call non-static method directly from the static context
}
}
“`
In this example, we change the `nonStaticMethod` to a static method, enabling it to be called directly from the static `main` method.
Additional considerations and best practices for working with static and non-static methods
1. Understand the purpose of your method: Before deciding whether a method should be static or non-static, think about its purpose and functionality. Does it depend on specific instance variables or non-static methods within the class? If yes, it should be non-static. If not, consider making it static.
2. Favor instance methods over static methods: In object-oriented programming, the primary goal is to encapsulate data and behavior within objects. Instance methods allow you to interact with the encapsulated state of an object, making your code more flexible, maintainable, and modular. Only use static methods when necessary.
3. Avoid unnecessary static references to non-static methods: It is important to avoid unnecessary static references to non-static methods to prevent code confusion and improve readability. Always use the appropriate approach based on the requirements of your program.
4. Utilize static methods for utility functions: Static methods are commonly used for utility functions that do not require access to specific instance variables or non-static methods of a class. These utility functions can be called directly from the class without the need to create an object.
FAQs
Q1. Cannot make a static reference to the non-static method `getClass()` from the type `Object`. Why does this error occur?
A1. The `getClass()` method is inherited from the `Object` class, and it is a non-static method. When you try to call it directly from a static context, such as a static method, it results in the “cannot make a static reference to a non-static method” error. To fix this, create an instance of the class and call the method on that instance.
Q2. Cannot make a static reference to the non-static method `toString()` from the type `Object`. Why does this error occur?
A2. Similar to the previous question, the `toString()` method is also a non-static method inherited from the `Object` class. Attempting to call it directly from a static context yields the mentioned error. Instantiate the class and call the method on the object to resolve the issue.
Q3. Cannot make a static reference to the non-static method `close()` from the type `Scanner`. Why does this error occur?
A3. The `close()` method in the `Scanner` class is a non-static method used to release system resources associated with the scanner. Since it is non-static, calling it directly from a static context raises the error. Create a scanner object and invoke the `close()` method on that object to resolve the issue.
Q4. Cannot make a static reference to the non-static method `save()` from the type `CrudRepository`. Why does this error occur?
A4. The specific implementation of the `CrudRepository` interface may provide a non-static `save()` method. Calling this method directly from a static context leads to the mentioned error. Create an instance of the class implementing the `CrudRepository` interface and call the `save()` method on that object.
Q5. What does “Cannot make a static reference to the non-static type `Node`” mean?
A5. This error occurs when you attempt to access or use a non-static nested class (also known as an inner class) from a static context. Non-static nested classes have an implicit reference to their enclosing instances, and accessing them directly from a static context without a valid instance will result in the mentioned error. Create an instance of the enclosing class and access the nested class through that instance.
How To Resolve Error: Non-Static Variable/Method Cannot Be Referenced From A Static Context
Can We Make Static Reference To Non-Static Method?
In object-oriented programming, the concepts of static and non-static methods are fundamental to understand. A static method is a method that belongs to the class itself, rather than an instance of the class. On the other hand, a non-static method is a method that belongs to an instance of the class and requires an object to be called upon.
One common question that arises in discussions about static and non-static methods is whether it is possible to make a static reference to a non-static method. In simpler terms, a static method cannot directly call a non-static method. However, there are ways to achieve this behavior indirectly, by either creating an instance of the class within the static method or by creating a separate non-static method and calling it from the static method.
To understand why a static method cannot directly reference non-static methods, we need to grasp the underlying concepts of how these methods function. When a method is declared as static, it means that it is not bound to a specific instance of the class and can be accessed directly using the class name. Non-static methods, on the other hand, require an instance of the class to be created before they can be called upon.
When a static method is defined, it exists within the memory independently of instances of the class. Whereas non-static methods are associated with specific instances, which hold their state and data. Attempting to directly reference a non-static method from a static one would lead to undefined behavior since no instance is available to access the non-static method.
Although a static method cannot directly reference non-static methods, there are scenarios where we may need to call a non-static method in a static context. In such cases, the workaround typically involves creating an instance of the class within the static method and then calling the non-static method on that instance.
Let’s consider an example to illustrate this concept. Suppose we have a class called Car, with a non-static method `startEngine()`. If we want to call this method from a static method called `main()`, we could achieve it by creating an instance of the Car class within `main()`, as follows:
“`
public class Car {
public void startEngine() {
// implementation details
}
public static void main(String[] args) {
Car myCar = new Car();
myCar.startEngine();
}
}
“`
In this example, we create an instance of the `Car` class called `myCar`. By doing so, we can access the non-static method `startEngine()` through the object `myCar`. This allows us to indirectly call the non-static method from within the static method.
Alternatively, if we find ourselves frequently needing to call non-static methods within static methods, we can create another non-static method that encapsulates the desired behavior and call that method from the static method. This approach provides a cleaner solution by avoiding repetitive code.
To summarize, a static method cannot directly reference a non-static method due to their contrasting natures. However, we can circumvent this limitation by creating an instance of the class within the static method or by defining a separate non-static method and calling it from the static method.
FAQs:
Q: Why are static methods unable to directly reference non-static methods?
A: Static methods are not tied to any specific instances of the class and exist independently. Non-static methods, on the other hand, require instances of the class to be created. Due to this distinction, static methods cannot directly reference non-static methods.
Q: Can we make a non-static method static?
A: Yes, it is possible to make a non-static method static. However, this decision should be made carefully as it changes the behavior and accessibility of the method. Static methods do not have access to instance variables and can only directly call other static methods or access static variables.
Q: What is the significance of static methods?
A: Static methods are commonly used for utility functions or methods that do not rely on any specific instance of the class. They provide a way to encapsulate behavior without the need for instantiating an object. Examples include mathematical operations or utility functions that perform string manipulations.
Q: Can static methods access instance variables?
A: No, static methods cannot access instance variables directly as they are not associated with any specific instance of the class. However, if necessary, we can pass instance variables as parameters to the static method.
Q: Is it recommended to frequently call non-static methods within static methods?
A: While there may be scenarios where calling non-static methods in static methods is necessary, it is generally advisable to minimize such practices. Directly calling non-static methods within static methods can lead to code complexity and confusion, as it blurs the distinction between class-level and instance-level behavior. It is often better to design methods and classes in a way that avoids this situation as much as possible.
Which Type Cannot Make A Static Reference To The Non-Static Method?
When working with object-oriented programming, particularly in languages like Java, it is essential to understand the difference between static and non-static methods. Static methods belong to the class itself, while non-static methods are specific to each instance or object of that class. This distinction is crucial because it determines how methods can be accessed and called.
A common misconception among beginners is that static methods can access non-static members of a class. However, the opposite is true – static methods cannot directly reference non-static methods or variables. This restriction is put in place because non-static members belong to a particular instance of the class, which may not exist when the static method is called.
To better understand this concept, let’s take a closer look at the nature of static and non-static methods:
Static Methods:
Static methods, also known as class methods, belong to the class itself rather than any specific instance. They can be accessed and invoked without creating an object of that class. Static methods are often used for utility functions, mathematical calculations, or any operation that does not rely on specific object instances.
Non-Static Methods:
Non-static methods, also referred to as instance methods, are defined within a class and require an instance of that class to be accessed or invoked. Each instance of the class has its own set of non-static methods, which can access non-static variables specific to that instance.
Why Can’t Static Methods Refer to Non-Static Methods:
Static methods cannot make direct references to non-static methods because non-static methods are associated with specific instances of a class. When a static method is called, it is not tied to any instance and does not have access to non-static methods or variables. This is because static methods are not aware of any specific instance state.
Attempting to invoke a non-static method from within a static method will result in a compilation error. If a static method needs to interact with non-static methods or variables, an instance of the class must be created first.
For example, consider a class called “Car” with a non-static method “drive()” and a static method “startEngine()”. Inside the “startEngine()” method, we cannot directly call “drive()” since it is a non-static method. We would need to create an instance of the “Car” class, and then call “drive()” on that instance.
Frequently Asked Questions:
Q: Can non-static methods call static methods?
A: Yes, non-static methods can call static methods without any issues. Since static methods belong to the class itself, they can be accessed by any method, whether static or non-static.
Q: Can static methods access static variables?
A: Yes, static methods can access static variables as both belong to the class itself rather than any specific instance. Static variables have a single copy that is shared among all instances of the class.
Q: Can non-static methods access non-static variables?
A: Yes, non-static methods can access non-static variables as they are specific to each instance of the class. Non-static variables have their own copies for each instance of the class.
Q: Can we make a non-static method static?
A: Yes, it is possible to make a non-static method static, but it depends on the requirements of the program. However, by doing so, the non-static method will no longer have access to non-static variables or methods.
Q: Can a class contain both static and non-static methods?
A: Yes, a class can contain both static and non-static methods. This allows the class to have a combination of methods that are globally accessible and methods that rely on specific instances.
In conclusion, static methods cannot make direct references to non-static methods. Static methods belong to the class itself and do not have access to instance-specific variables or methods. It is important to understand this distinction when working with object-oriented programming paradigms, as attempting to invoke a non-static method from within a static method will result in a compilation error. Remember, static methods require a class instance to call non-static methods or access non-static variables.
Keywords searched by users: cannot make a static reference to the non static method Cannot make a static reference to the non static method getClass() from the type Object, cannot make a static reference to the non-static method tostring() from the type object, cannot make a static reference to the non-static method close() from the type scanner, cannot make a static reference to the non-static method save from the type crudrepository, Cannot make a static reference to the non static method, Cannot make a static reference to the non static type node, Using non static variable in static method, static reference to non static method
Categories: Top 98 Cannot Make A Static Reference To The Non Static Method
See more here: nhanvietluanvan.com
Cannot Make A Static Reference To The Non Static Method Getclass() From The Type Object
Understanding the Error:
In Java, static methods belong to the class itself and can be called without creating an instance of the class. Non-static methods, on the other hand, are tied to instances of the class and can only be invoked on those instances. The getClass() method is a non-static method defined in the Object class, which is the root class for all other classes in Java.
This error occurs when a static method attempts to use the getClass() method, as it violates the basic concept of static methods. Since static methods do not have access to instance-specific data, they cannot directly invoke non-static methods like getClass(). As a result, an error is thrown to inform the developer about this violation.
Causes of the Error:
The error “Cannot make a static reference to the non-static method getClass() from the type Object” can occur due to various reasons. Here are a few common causes:
1. Calling getClass() from a static context: When a static method or initializer tries to call the getClass() method, this error is thrown because getClass() is a non-static method and requires an instance of the class.
For example, consider the following code snippet:
“`
public class MyClass {
public static void main(String[] args) {
System.out.println(getClass());
}
}
“`
In this case, the main() method is a static method, and it tries to invoke getClass() method without an instance. This will result in the mentioned error.
2. Accessing non-static member from a static method: Another cause of this error is when a static method attempts to access a non-static member, such as invoking a non-static method or accessing a non-static variable.
Resolution:
To resolve the error “Cannot make a static reference to the non-static method getClass() from the type Object”, you need to ensure that you are not trying to invoke the getClass() method from a static context. Here are a few solutions:
1. Create an instance: One way to resolve this error is by creating an instance of the class and invoking the getClass() method on that instance. For example:
“`java
public class MyClass {
public static void main(String[] args) {
MyClass obj = new MyClass();
System.out.println(obj.getClass());
}
}
“`
By creating an instance of the class, we can access the non-static method getClass() and obtain the class information.
2. Use the class name directly: As an alternative, you can use the class name directly followed by “.class” to obtain the class information. This approach does not require creating an instance. For example:
“`java
public class MyClass {
public static void main(String[] args) {
System.out.println(MyClass.class);
}
}
“`
This will output the class information as expected without invoking the non-static method getClass().
FAQs:
Q1. What is the getClass() method used for?
The getClass() method is defined in the Object class and is used to obtain the runtime class of an object. It returns an instance of the Class class, which provides various methods to retrieve information about the object’s class.
Q2. Why can’t we invoke non-static methods from a static context?
Static methods are not associated with any instance of the class and can be invoked without creating objects. Non-static methods depend on the state of an instance and can only be invoked on instances of the class. Hence, static methods do not have access to non-static members or methods directly.
Q3. Are there any other similar errors related to static and non-static methods?
Yes, there are other errors related to static and non-static methods, such as “Non-static method cannot be referenced from a static context” and “Cannot make a static reference to the non-static field.”
Q4. Can I make a non-static method static to resolve the error?
If the non-static method is dependent on instance-specific data or belongs to the behavior of objects, converting it to a static method may lead to logical issues. Therefore, it is generally not recommended to make a non-static method static to resolve this error.
In conclusion, the error “Cannot make a static reference to the non-static method getClass() from the type Object” occurs when a static method tries to invoke the non-static method getClass(). It violates the concept of static methods and can be resolved by creating an instance or using the class name directly. Understanding the difference between static and non-static methods is crucial in avoiding such errors in Java programming.
Cannot Make A Static Reference To The Non-Static Method Tostring() From The Type Object
In Java, the error message “Cannot make a static reference to the non-static method toString() from the type Object” can be quite confusing for beginners. This error occurs when we try to invoke a non-static method, such as toString(), from a static context. This article will delve into why this error occurs, how to fix it, and answer some frequently asked questions related to this issue.
Understanding Static and Non-Static Methods:
Before we delve into the error itself, let’s clarify the difference between static and non-static methods. In Java, a method is declared as static if it belongs to the class itself, rather than to an instance of the class. These methods can be invoked without creating an object.
On the other hand, non-static methods are associated with specific instances of a class. They can only be called on an object or instance of the class. Non-static methods often access instance variables and are used to operate on specific data associated with an object.
The Error Explained:
The error message “Cannot make a static reference to the non-static method toString() from the type Object” occurs when we try to invoke a non-static method, such as toString(), from a static context. Static contexts include the main method or any other static methods or variables directly associated with the class itself.
The toString() method is a non-static method inherited from the Object class, the root class of all Java classes. It is intended to provide a human-readable string representation of an object. Since it is a non-static method, it cannot be directly accessed or called from a static context.
How to Fix the Error:
To resolve the “Cannot make a static reference to the non-static method toString() from the type Object” error, you have a few options:
1. Create an Instance of the Class:
If you want to call the toString() method, you need to create an instance of the class in which the method is defined. For example, if you have a class named MyClass, you can create an instance and call the toString() method as follows:
MyClass obj = new MyClass();
System.out.println(obj.toString());
2. Make the Method Static:
Another approach is to declare the method as static. This way, the method belongs to the class itself and can be called directly from a static context. However, this might not always be possible or appropriate, especially if the method is intended to operate on instance-specific data.
3. Use the Class name to Call toString():
Alternatively, you can call the toString() method using the name of the class on which it is defined. For example, if you have a class named MyClass, you can call the toString() method like this:
System.out.println(MyClass.toString());
However, keep in mind that this will not give you the desired output if you want to convert an instance of MyClass to a human-readable string.
4. Override the toString() Method:
If you are dealing with a custom class and want to provide a custom string representation, you can override the toString() method. By doing so, you can define how the string should be generated for instances of your class.
To override the toString() method, simply add the following code within your class:
@Override
public String toString() {
// return custom string representation
}
By providing your own implementation, you can ensure that the toString() method is accessible from both static and non-static contexts.
FAQs:
Q: Can I call a non-static method from a static method?
A: No, you cannot directly call a non-static method from a static method. Non-static methods operate on specific instances of a class and require an object to be instantiated.
Q: Why is the toString() method non-static?
A: The toString() method is non-static because it is designed to provide a string representation of an object’s state. Since each object may have different state and data, a non-static method is required to access and provide this information.
Q: Is the toString() method required in all classes?
A: No, the toString() method is not required in all classes. However, it is often a good practice to override this method in custom classes to provide a meaningful string representation for debugging purposes or when printing objects.
Q: Can I directly call toString() on primitive types?
A: No, primitive types do not have a toString() method. However, Java provides wrapper classes such as Integer or Double, which wrap primitive types and provide a toString() method to convert them to a string representation.
In conclusion, the error “Cannot make a static reference to the non-static method toString() from the type Object” occurs when we try to access a non-static method from a static context. It can be resolved by creating an instance of the class, making the method static, calling it using the class name, or overriding the method. Understanding the difference between static and non-static methods is crucial to avoid and fix this error.
Images related to the topic cannot make a static reference to the non static method
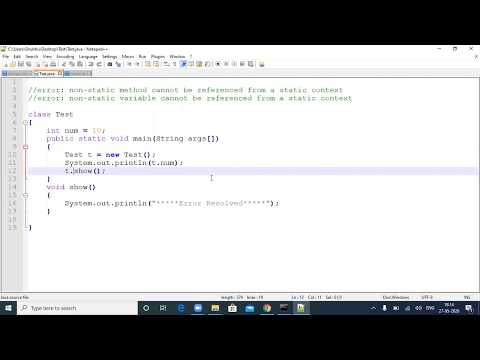
Found 33 images related to cannot make a static reference to the non static method theme
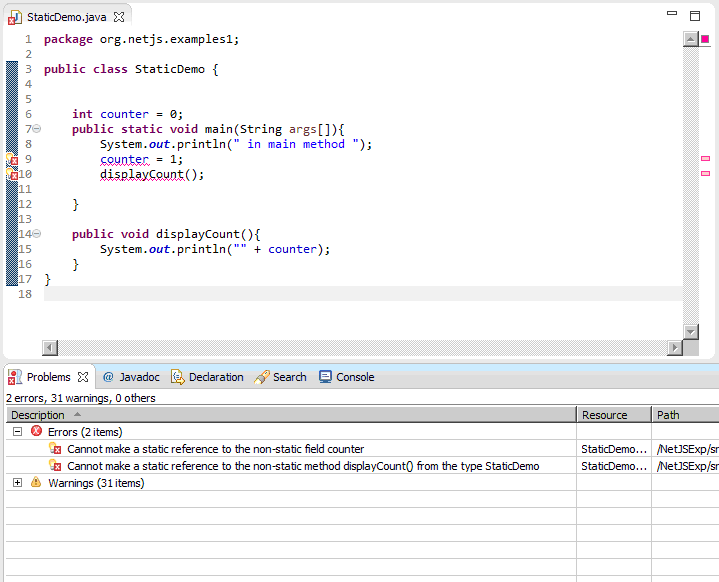
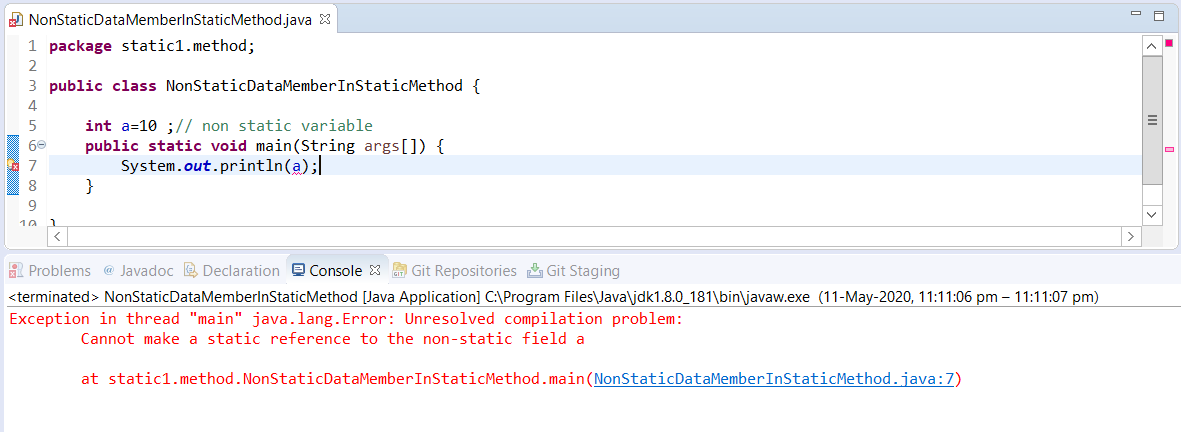
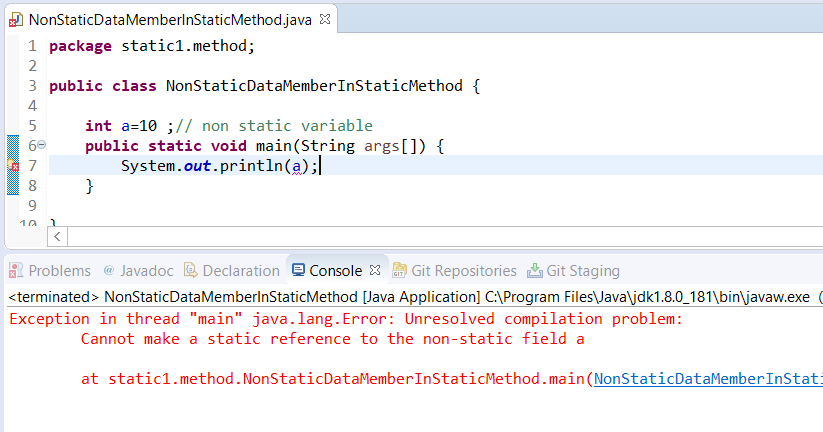
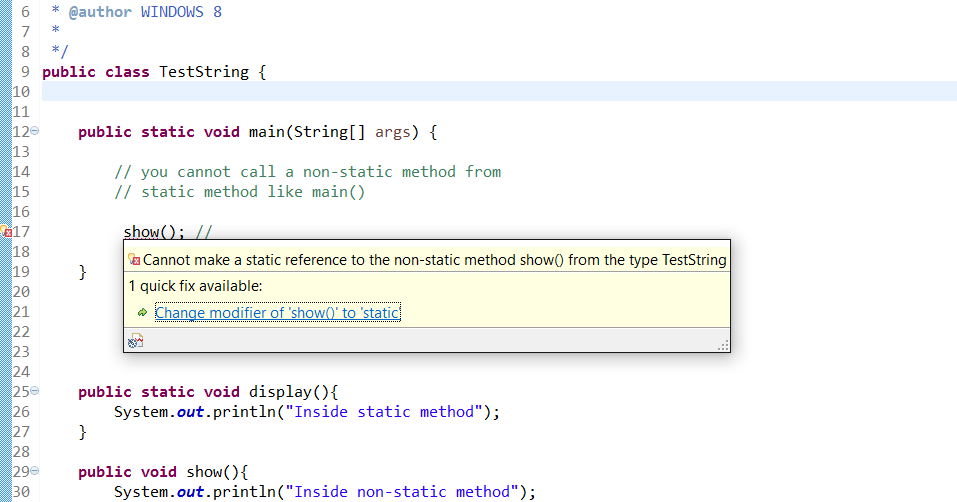
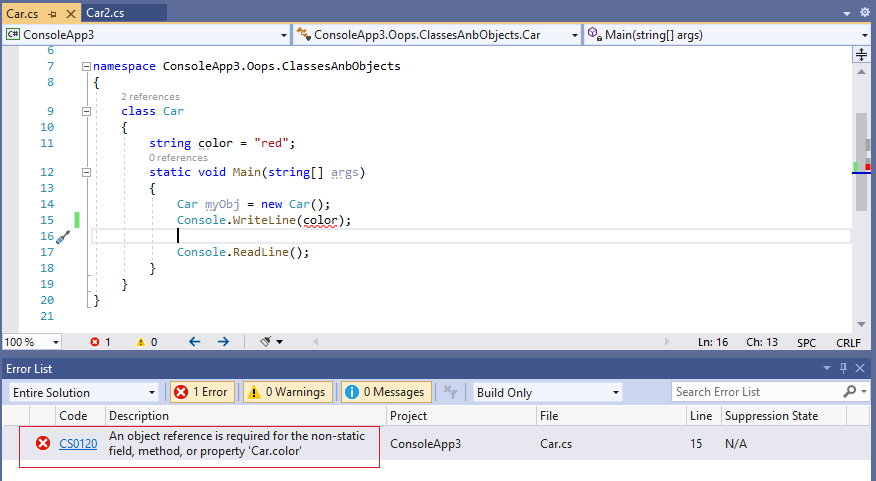
![VS] Cannot make a static reference to the non-static method ..... from the type ... Java(603979977) — greenhelix Vs] Cannot Make A Static Reference To The Non-Static Method ..... From The Type ... Java(603979977) — Greenhelix](https://blog.kakaocdn.net/dn/ZZnDT/btqGvZuihxb/MnuPjBKBT66KAw1HaRgeWK/img.jpg)


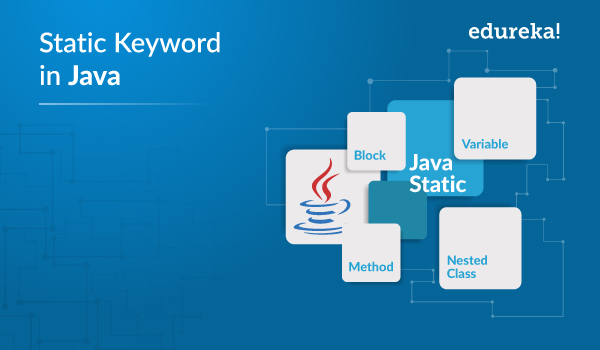
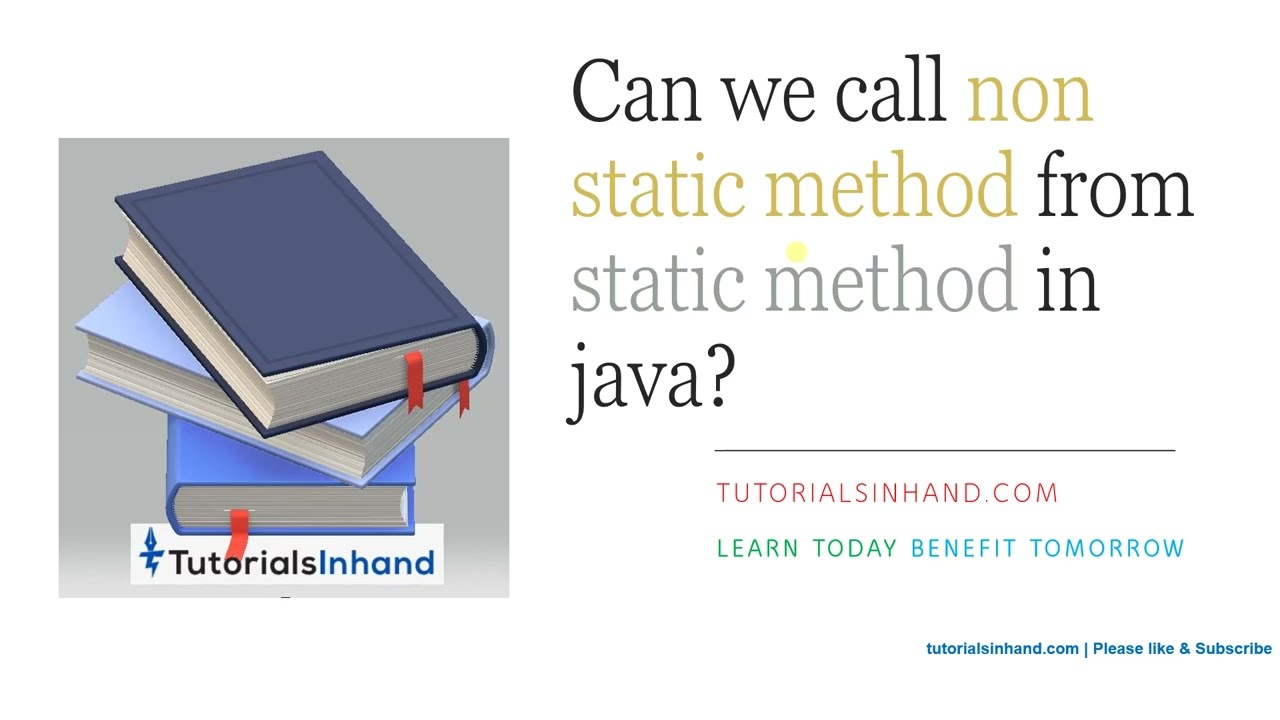

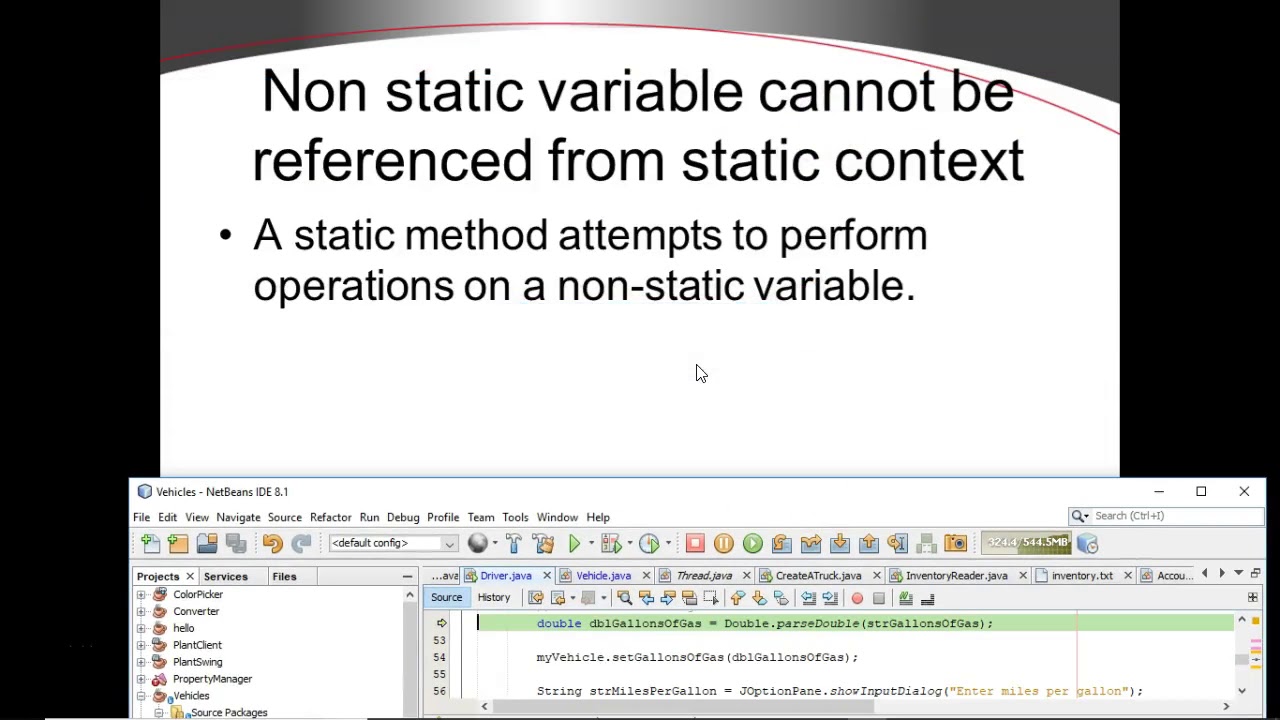
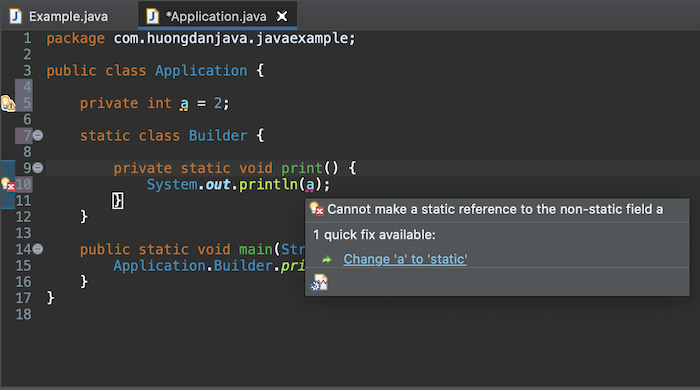

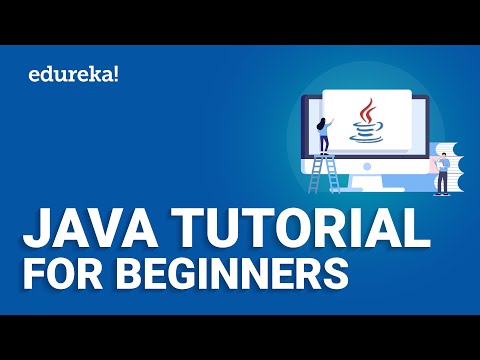
![Static in Java: An Overview of Static Keyword in Java With Examples [2023 Edition] Static In Java: An Overview Of Static Keyword In Java With Examples [2023 Edition]](https://www.simplilearn.com/ice9/free_resources_article_thumb/static_in_java.jpg)
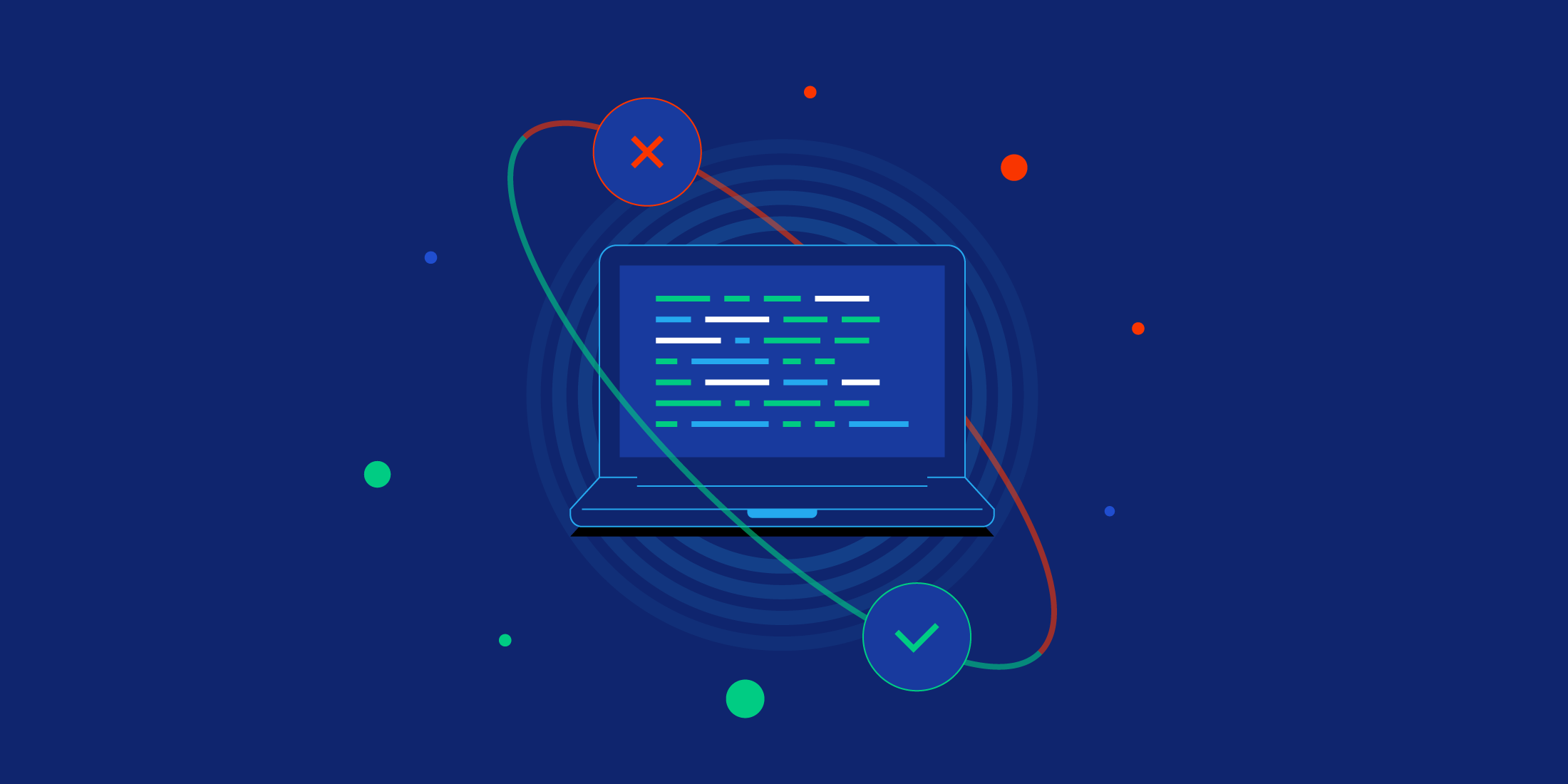
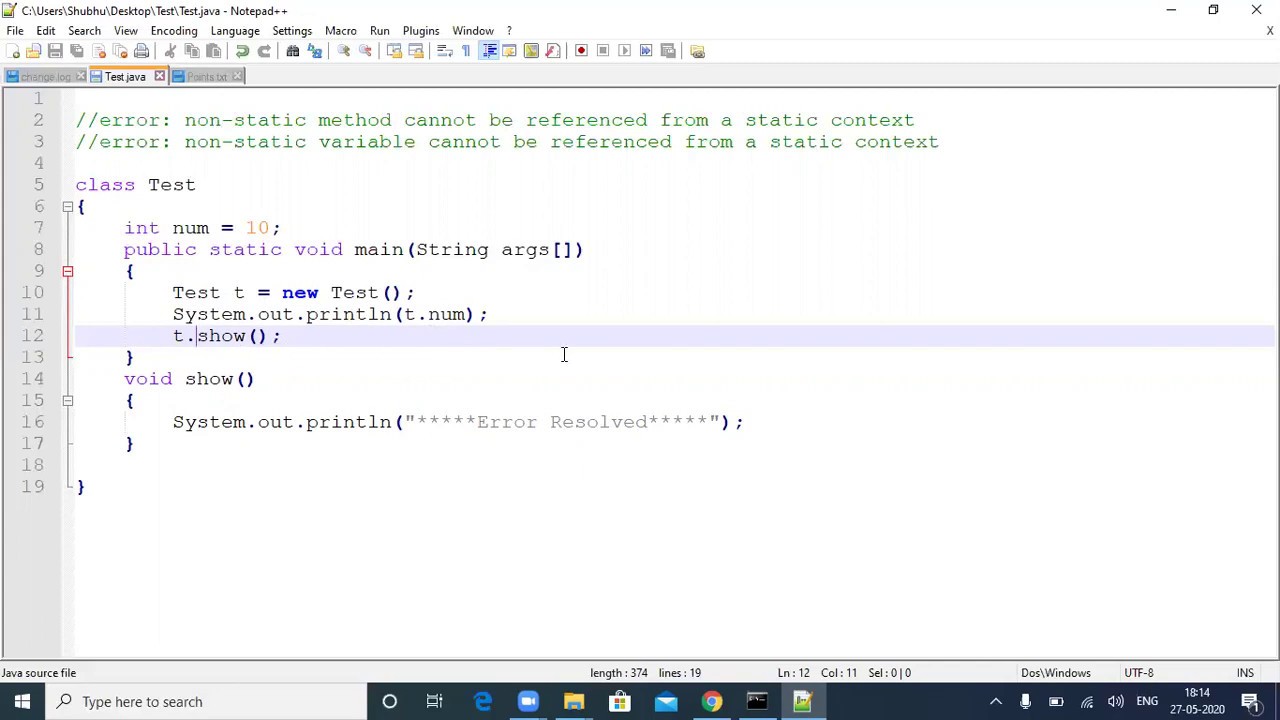
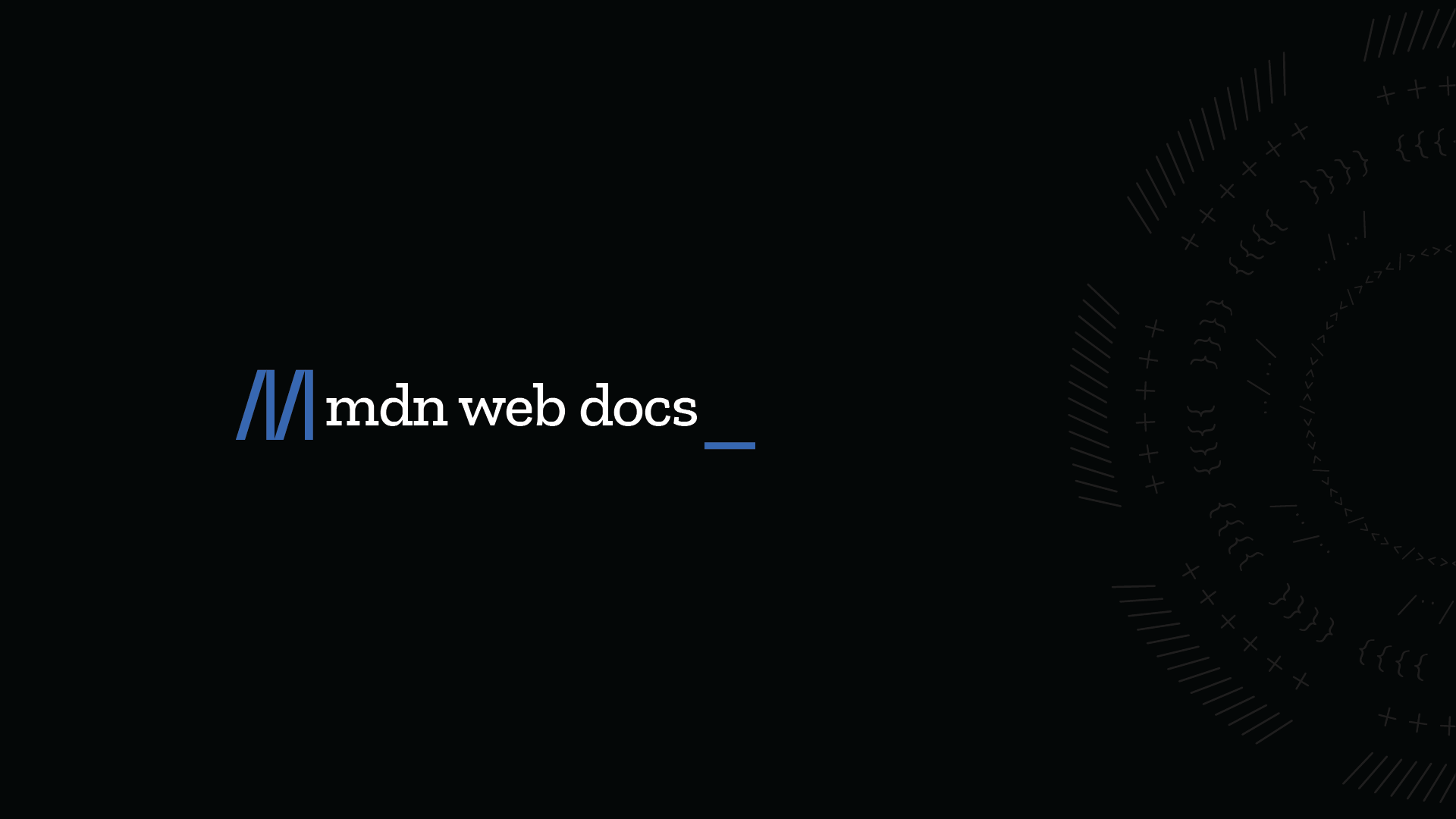
![What is Static Method in Java [+Examples]? What Is Static Method In Java [+Examples]?](https://blog.hubspot.com/hubfs/static-method.jpg)


Article link: cannot make a static reference to the non static method.
Learn more about the topic cannot make a static reference to the non static method.
- Cannot make a static reference to the non-static method
- Fix Cannot make a static Reference to The Non-static Method …
- Can we make static reference to non-static fields in java
- Cannot make a static reference to the non-static method – W3docs
- Difference between static and non-static method in Java
- Restrictions applied to Java static methods – Tutorialspoint
- Cannot make a static reference to the non-static method or fields
- Core Java : Cannot make a static reference to the non-static field
- Can we make static reference to non-static fields in java
- Cannot make a static reference to the non-static method or …
- Cannot make a static reference to the non-static method …
- Cannot make a static reference to the non-static field or method
- RE: Exception:Cannot make a static reference to the non-static …
See more: nhanvietluanvan.com/luat-hoc