Cannot Find Module ‘Glob’
Reasons for the Error:
1. Missing or Incorrect Installation of the glob Module:
One of the main reasons for the “cannot find module ‘glob'” error is the absence or incorrect installation of the glob module. If the module is not installed or installed improperly, Node.js will not be able to locate it, resulting in the error message.
2. Path-related Issues:
Sometimes, the error can occur due to path-related issues. The global or local paths where Node.js searches for modules may not include the necessary paths for the glob module. As a result, Node.js fails to find the module and throws the error.
3. Version Compatibility Problems:
Incompatible versions of Node.js and the glob module can also lead to this error. If the version of the glob module you are trying to use is not compatible with the installed Node.js version, the module may not be recognized, causing the error message.
4. Module Not Listed in package.json File:
The package.json file contains information about the dependencies and modules required for a Node.js project. If the ‘glob’ module is not listed in the package.json file, Node.js may not be able to find it, resulting in the error.
Troubleshooting Steps:
1. Verify glob Module Installation:
Firstly, ensure that the glob module is installed correctly. Open the command line interface and run the following command:
“`
npm install glob
“`
This command will install the glob module and its dependencies. If the installation is successful, the error should be resolved.
2. Check Path Configuration:
Check if the paths set for Node.js include the necessary directories where the glob module is installed. Ensure that the correct paths are set in the environment variables or the project configuration.
3. Update Node.js and glob Versions:
If you encounter version compatibility issues between Node.js and the glob module, try updating either of them. Check the glob module’s latest version compatibility with the Node.js version you have installed and update accordingly. Also, make sure that your Node.js version is up to date to ensure compatibility with the module.
4. Ensure glob Module is Listed in package.json File:
If the glob module is not listed in the package.json file, you need to add it as a dependency manually. Open the package.json file and locate the “dependencies” section. Add the following line:
“`
“glob”: “^x.x.x”
“`
Replace “x.x.x” with the desired version of the glob module. Save the changes and run the command `npm install` to install the module based on the updated package.json file.
FAQs:
Q: What is the most common error message related to the “cannot find module ‘glob'” error?
A: The most common error message related to this issue is “cannot find module ‘glob'”.
Q: How can I resolve the “cannot find module ‘glob'” error?
A: You can resolve this error by verifying the glob module’s installation, checking the path configuration, updating Node.js and glob versions, and ensuring the glob module is listed in the package.json file.
Q: Can I use the glob module without installing it?
A: No, the glob module needs to be installed for it to be accessible to your Node.js application.
Q: Why does the path-related issue cause the “cannot find module ‘glob'” error?
A: If the paths set for Node.js do not include the necessary directories where the glob module is installed, Node.js will not be able to find the module, resulting in the error message.
Q: How can I check the version of Node.js installed on my system?
A: Open the command line interface and run the command `node -v`. This will display the version of Node.js installed on your system.
In conclusion, the “cannot find module ‘glob'” error often occurs due to missing or incorrect installation, path-related issues, version compatibility problems, or the module not being listed in the package.json file. By following the troubleshooting steps mentioned above, you can resolve this error and successfully use the glob module in your Node.js application.
Fix Global Installs Not Working | \”Cannot Find Module\” Error Fix
What Is Glob In Nodejs?
When working with file systems in Node.js, it is often necessary to match files based on patterns or retrieve a list of file paths that meet specific criteria. This is where glob comes into play. Glob is a popular package in the Node.js ecosystem that provides an easy and powerful way to search for files using pattern matching.
Glob supports various wildcard characters, such as `*` and `?`, which allow you to match multiple files or parts of a file name based on specific patterns. It also provides advanced features like recursive search, negation, and extensibility, making it highly flexible for performing file operations.
Here’s an in-depth look at glob and its functionality in Node.js:
Basic Usage:
The basic usage of glob involves using a pattern to match files or directories in a given directory or tree. The pattern can include wildcard characters to represent any number of characters or a single character in a file or directory name. For example, `*.txt` will match all files with a `.txt` extension, while `file??.txt` will match files named “file” followed by any two characters and ending with “.txt”.
The glob module can be easily installed via npm:
“`
npm install glob
“`
Once installed, you can require it in your Node.js application:
“`javascript
const glob = require(‘glob’);
“`
Searching for Files:
To search for files matching a specific pattern, you can use the `glob` function provided by the glob module. The function takes two arguments: the pattern to match and an optional callback function. Here’s an example that searches for all JavaScript files in a directory:
“`javascript
glob(‘*.js’, (err, files) => {
if (err) {
console.error(err);
} else {
console.log(files);
}
});
“`
This code will retrieve an array containing the paths of all JavaScript files in the directory. If an error occurs, it will be logged to the console. Note that glob supports asynchronous operations, allowing you to perform other tasks while the search is in progress.
Advanced Features:
Glob offers several advanced features to enhance file searching and filtering. Some of these features include:
1. Recursive Search: By using the `**` pattern, you can perform a recursive search to match files in subdirectories. For example, `src/**/*.js` will match all JavaScript files within the `src` directory and its subdirectories.
2. Negation: You can negate a pattern by prefixing it with an exclamation mark (`!`). This allows you to match files that do not meet the specified pattern. For instance, `*.!(txt)` will match all files except those with a `.txt` extension.
3. Custom Options: The glob function allows you to customize the search behavior by passing an options object as the second argument. You can define options like the current working directory, case-sensitivity, and whether to follow symbolic links.
4. Sync Operations: If you prefer synchronous operations, glob provides a synchronous counterpart for most functions. Instead of passing a callback, you can directly assign the returned value to a variable.
FAQs:
Q: Is glob limited to file searching or can it also be used for other purposes?
A: Glob is primarily designed for file searching, but it can also be utilized for other tasks like matching directories or filtering lists of strings based on patterns.
Q: Can glob handle large file systems efficiently?
A: While glob can handle large file systems, it may experience performance issues when searching extremely large directories or traversing deeply nested trees. Consider using more specialized tools for such scenarios.
Q: Are glob patterns case-sensitive?
A: By default, glob patterns are case-insensitive on Windows file systems but case-sensitive on Unix-based systems. However, you can override this behavior by setting the `nocase` option to true.
Q: Can glob be used in the browser as well?
A: Although glob is mainly meant for server-side development with Node.js, there are also browser-compatible versions available, such as globby and globbyfs.
In conclusion, glob is a powerful package in the Node.js ecosystem that facilitates efficient file searching based on pattern matching. Whether you need to match files using simple patterns or perform complex searches within an entire directory tree, glob provides a flexible and straightforward solution. Understanding and harnessing the capabilities of glob can significantly improve your file system operations in Node.js.
How To Solve Npm Install Error?
If you are a JavaScript developer, you are most likely familiar with npm (Node Package Manager) and its crucial role in managing project dependencies. However, it is not uncommon to encounter errors when running npm install, which can be frustrating and time-consuming to resolve. In this article, we will guide you through the process of solving npm install errors, providing useful tips and explanations to help you navigate through common issues.
Understanding npm install Errors
Before delving into the solutions, it is important to understand the different types of errors you might encounter during an npm install. These errors can generally be categorized into two types: local and global.
Local Errors:
Local errors occur within a specific project folder. They are often caused by missing or incompatible dependencies, version conflicts, or network connection issues. These errors are isolated to the project you are working on and do not affect other projects.
Global Errors:
Global errors, on the other hand, are not specific to a project but occur at a system-wide level. They might be caused by permission issues, conflicts between globally installed packages, or problems with your local Node.js installation.
Now that you have an understanding of the different types of npm install errors, let’s explore some common issues and their solutions.
1. Clear Cache:
One of the simplest solutions to many npm install errors is to clear the npm cache. This can be done by running the command “npm cache clean –force” in your terminal or command prompt. Once the cache is cleared, try running “npm install” again.
2. Update npm:
Outdated versions of npm can lead to compatibility issues and errors. To update npm to the latest version, use the command “npm install -g npm”.
3. Check Node.js Version Compatibility:
Ensure that the version of Node.js you are using is compatible with the packages you are trying to install. Some packages require a specific Node.js version, so running an outdated version might result in errors. You can check your Node.js version by running “node -v”.
4. Verify Dependencies:
If you encounter an error stating that a specific module or package is missing, double-check your package.json file to make sure the required dependencies are listed. If they are missing, add them to your package.json file and run “npm install” again.
5. Resolve Version Conflicts:
Version conflicts between dependencies are a common cause of npm install errors. To resolve this, manually check the versions of the conflicting packages in your package.json file and attempt to update them to compatible versions. If a specific package version is crucial for your project, consider using a version management tool like yarn or npm shrinkwrap to handle the conflicting versions.
6. Check Network Connectivity:
Network connectivity issues can cause dependencies to fail to download or install properly. Ensure that you have a stable internet connection and try running “npm install” again. If the problem persists, you may need to configure your network proxy settings or switch to a different network.
7. Update Operating System:
If you are using an outdated or unsupported operating system, certain dependencies might fail to install due to incompatibility. Ensure that your operating system is up to date, as some packages require specific system requirements to function properly.
8. Resolve Permissions Issues:
Sometimes, permission issues prevent npm from accessing the necessary directories to install packages. To solve this, run npm install with administrator or root permissions using the command “sudo npm install” (for Unix-like systems) or by opening your terminal/command prompt as an administrator (for Windows systems). However, exercise caution when running commands with elevated permissions, as it poses potential security risks.
9. Remove Global Packages:
If you encounter global errors, conflicting globally installed packages might be the cause. Try uninstalling unnecessary global packages or updating them to resolve any conflicts. Use the command “npm list -g –depth 0” to see a list of globally installed packages and remove or update the relevant ones accordingly.
FAQs:
Q: What is npm install?
A: npm install is a command used in JavaScript projects to install the necessary dependencies listed in the package.json file.
Q: Why does npm install fail?
A: npm install can fail due to various reasons, such as network connection issues, version conflicts between dependencies, permission errors, or missing packages.
Q: How to fix “no such file or directory” error during npm install?
A: This error is often caused by incorrect file paths or missing directories. Double-check the file paths and ensure that the necessary directories exist.
Q: How long does npm install take?
A: The time it takes for npm install to complete depends on the size of the project, the number of dependencies, and the speed of your internet connection. It can range from a few seconds to several minutes.
Q: Should I run npm install with sudo?
A: It is generally recommended to avoid running npm install with sudo unless specifically required. Running with elevated permissions can introduce security risks and potentially damage your system.
In conclusion, encountering errors while running npm install is not uncommon, but armed with the right knowledge and troubleshooting techniques, you can overcome these hurdles. By following the tips and solutions provided in this article, you can efficiently identify and resolve various npm install errors, ensuring a smooth development experience for your JavaScript projects.
Keywords searched by users: cannot find module ‘glob’ Cannot find module nodejs, Glob parent, Glob promise, Usr local lib node_modules serve build main js 4 import path from node path
Categories: Top 63 Cannot Find Module ‘Glob’
See more here: nhanvietluanvan.com
Cannot Find Module Nodejs
When working with Node.js, encountering errors is not uncommon. One such error that developers may come across is the “Cannot find module ‘nodejs'” error. This error message can be frustrating, especially for newcomers to the world of Node.js. In this article, we will dive into the details of this error, understand its causes, and explore possible solutions. Additionally, we will address some common FAQs related to this error to provide a comprehensive guide for resolving the issue.
Understanding the Error:
The “Cannot find module ‘nodejs'” error occurs when Node.js is unable to locate a required module, which is specified in the code, during the execution of a program. When Node.js encounters a line of code that references a module, it attempts to locate and load the module. If the module cannot be found, this error is thrown.
Possible Causes of the Error:
There are several reasons why this error may occur:
1. Module Installation Issues: The module ‘nodejs’ might not be installed or might be installed incorrectly. This error can happen if a required Node.js module is missing or improperly installed.
2. Incorrect Module Name: The specified module name might be incorrect. It is essential to ensure that the module name used in the ‘require’ statement is accurate and matches the module’s actual name.
3. Typo in the Code: A simple typo in the code’s module name or path could also trigger this error. Double-checking the code for any typographical errors is essential for accurate module loading.
Solutions to the “Cannot find module ‘nodejs'” Error:
Now let’s explore some possible solutions to this error:
1. Confirm Module Installation: Begin by verifying that the ‘nodejs’ module is indeed installed. Use the command `npm ls nodejs` in the command line to check if the module is listed as a dependency in the current project. If it is missing, it needs to be installed using `npm install nodejs`.
2. Reinstall the Module: If the module is already installed but the error persists, there might be an issue with the module’s installation. Try reinstalling the module using `npm install nodejs` to ensure its proper installation.
3. Check Module Name: Cross-check the module name used in the ‘require’ statement with the appropriate module’s name. Verify that it is spelled correctly, including any required capitalization or punctuation. A slight mismatch in the module name can lead to this error.
4. Review the Module’s Path: If the module is installed correctly and the name is accurate, check for any inconsistencies or errors in the module’s path. Ensure that the path provided in the ‘require’ statement correctly points to the location of the module.
Frequently Asked Questions (FAQs):
Here are some frequently asked questions related to the “Cannot find module ‘nodejs'” error:
Q1. Why am I getting the “Cannot find module ‘nodejs'” error?
This error typically occurs due to an issue with the installation of the ‘nodejs’ module, an incorrect module name in the code, or a path-related issue.
Q2. How can I verify if the ‘nodejs’ module is installed?
You can check if the ‘nodejs’ module is installed by running the command `npm ls nodejs` in the command line. If it is missing, you can install it using `npm install nodejs`.
Q3. I have checked the module name, and it appears to be correct. What should I do next?
If the module name is correct, double-check the module’s installation. Reinstall it using `npm install nodejs` to ensure proper installation.
Q4. Could a typo in the code be the reason for this error?
Yes, a minor spelling mistake or incorrect case sensitivity in the module name or path mentioned in the code can result in the “Cannot find module ‘nodejs'” error.
Q5. Why is it necessary to review the module’s path?
Reviewing the module’s path is crucial to ensure that the ‘require’ statement points accurately to the module’s location. An incorrect or incomplete path will cause Node.js to be unable to find the module, triggering the error.
In conclusion, the “Cannot find module ‘nodejs'” error can be caused by various factors, such as module installation issues, incorrect module names, or typos in the code. By following the suggested solutions and referring to the FAQs, developers can effectively resolve this error and continue building applications with Node.js seamlessly. Remember to double-check the module installation, module name, and module path to minimize the chances of encountering this error.
Glob Parent
Introduction (103 words):
Parenthood is a lifelong journey filled with joy, challenges, and countless responsibilities. In the digital era, parents are seeking innovative ways to manage their roles effectively. One such groundbreaking solution is Glob Parent, an all-in-one parenting app designed to assist parents in the tech-savvy world. This article provides a comprehensive overview of Glob Parent, delving into its features, benefits, and its impact on modern parenting.
What is Glob Parent? (129 words):
Glob Parent is a cutting-edge parenting app that combines multiple features into an intuitive platform. Developed with the goal of simplifying parenting responsibilities, Glob Parent offers a wide range of functions such as tracking child activities, organizing schedules, monitoring screen time, facilitating communication, providing location services, and offering educational resources for children. By addressing various aspects of modern parenting, this app aims to empower parents with the right tools to ensure their child’s holistic development.
Features and Benefits (441 words):
1. Activity Tracking: Glob Parent offers a detailed activity tracking tool that allows parents to monitor their child’s daily routine comprehensively. From sleep patterns and feeding schedules to exercise and playtime, this feature enables parents to analyze and improve their child’s overall well-being.
2. Schedule Management: With Glob Parent, parents can effortlessly organize their children’s schedules, including activities, appointments, playdates, and school-related events. By efficiently managing their child’s time, parents can ensure a healthy balance between academics, leisure, and extracurricular activities.
3. Screen Time Monitoring: The app enables parents to monitor and manage their child’s screen time, ensuring responsible use of devices. Parents can set limits on device usage, define restricted periods, and receive notifications if their child exceeds the designated screen time, effectively promoting healthy digital habits.
4. Communication Platform: Glob Parent facilitates seamless communication between parents and their children. The app offers secure messaging features, allowing parents to convey important messages, reminders, or words of encouragement. Besides, the app also enables sharing of pictures, videos, and audio files, fostering a meaningful connection even when physically apart.
5. Location Services: The app incorporates location tracking technology, providing parents with real-time information about their child’s whereabouts. This feature ensures parents’ peace of mind by offering a heightened sense of security, especially during outdoor activities or school commutes.
6. Educational Resources: Glob Parent offers an array of age-appropriate educational resources, including interactive games, e-books, and learning materials. This feature promotes early childhood education, making learning fun and engaging for children while providing parents with educational tools to support their child’s development.
Glob Parent FAQs (410 words):
1. Is Glob Parent secure for child data privacy?
Yes, Glob Parent prioritizes data privacy and incorporates robust security measures. It is compliant with strict privacy regulations, ensuring the child’s sensitive information remains secure and inaccessible to unauthorized individuals.
2. How does Glob Parent monitor screen time?
Glob Parent utilizes advanced algorithms to accurately track screen time across various devices. By analyzing app usage patterns and device activity, parents can gain insights into their child’s screen time habits and take appropriate measures if necessary.
3. Can multiple caregivers have access to the same account?
Absolutely. Glob Parent allows multiple caregivers, such as parents, grandparents, or guardians, to access the same account. This feature ensures seamless communication and coordination among caretakers, promoting a united parenting approach.
4. Is there a limit to the number of children that can be managed through Glob Parent?
No, there is no predefined limit to the number of children that can be managed on the app. Whether you have one child or multiple, Glob Parent centralizes all their information, ensuring efficient management for every aspect of their lives.
5. Are there any age restrictions for using Glob Parent?
Glob Parent is suitable for parents with children of all ages. From infancy through adolescence, the app adapts to the changing needs of children and offers age-appropriate features that cater to their developmental stages.
6. Does Glob Parent offer customer support?
Yes, Glob Parent provides dedicated customer support to address any queries or technical issues users may encounter. Their support team strives to promptly resolve customer concerns and ensure a smooth user experience.
Conclusion (100 words):
Glob Parent is revolutionizing modern parenting by leveraging the potential of technology. With its comprehensive features and benefits, this all-in-one app streamlines parenting responsibilities and empowers parents to nurture their child’s growth effectively. From managing schedules to promoting responsible screen time, and enhancing communication, Glob Parent provides an intuitive platform that assists parents in making informed decisions and maintaining a healthy work-life-family balance. Embrace the power of technology, and let Glob Parent make your parenting journey a little easier.
Glob Promise
In today’s rapidly evolving financial landscape, innovation plays a critical role in driving progress and improving the efficiency of existing systems. With the emergence of blockchain technology, the financial industry has witnessed a transformative shift in the way transactions are executed, offering enhanced security, transparency, and global accessibility. Glob Promise, a groundbreaking blockchain-based platform, has emerged as a pioneer in revolutionizing the global financial market, promising to take it to new heights.
What is Glob Promise?
Glob Promise is an advanced blockchain-powered platform that facilitates secure and fast financial transactions on a global scale. Built on the foundations of blockchain technology, the platform aims to eliminate traditional financial intermediaries and offer direct peer-to-peer transactions. By providing a decentralized system, Glob Promise ensures that transactions remain transparent, secure, and cost-effective.
Key Features of Glob Promise
1. Security and Transparency: Glob Promise harnesses the power of blockchain technology to provide secure and transparent transactions. Every transaction conducted on the platform is recorded on an immutable blockchain ledger, ensuring that all financial activities are entirely traceable.
2. Global Accessibility: One of the significant advantages offered by Glob Promise is its ability to connect individuals from different parts of the world in a single financial ecosystem. Regardless of their geographic location, users can seamlessly participate in the global financial market and perform transactions without the need for traditional intermediaries.
3. Elimination of Traditional Intermediaries: By removing intermediaries such as banks and remittance services, Glob Promise empowers individuals to directly transact with each other. This eliminates the lengthy processing times and high fees associated with traditional financial systems, providing users with a seamless and cost-effective solution.
4. Smart Contracts: The platform’s integration of smart contracts automates the execution of transactions based on predefined conditions, eliminating the need for intermediaries. These self-executing contracts ensure that transactions are carried out efficiently while reducing the risks of fraud or human error.
5. Rewards System: Glob Promise recognizes and appreciates its users’ active participation by offering a rewards system. Users are incentivized with tokens for various activities, such as referring new members, making transactions, or contributing to the platform’s growth. These tokens can be utilized for various purposes within the platform or exchanged for other cryptocurrencies.
FAQs
Q: How does Glob Promise ensure the security of transactions?
A: Glob Promise utilizes advanced cryptographic algorithms and blockchain technology to secure every transaction. Each transaction is recorded on an immutable ledger, making it traceable and tamper-proof.
Q: Can anyone participate in the Glob Promise ecosystem?
A: Yes, anyone with an internet connection can participate in the Glob Promise ecosystem. The platform is designed to provide global accessibility and connect individuals worldwide.
Q: How does Glob Promise eliminate traditional intermediaries?
A: By leveraging blockchain technology, Glob Promise facilitates direct peer-to-peer transactions without relying on traditional intermediaries such as banks. Smart contracts automate the process, ensuring secure and efficient transactions.
Q: What advantages does the rewards system offer?
A: The rewards system encourages active user participation by providing incentives in the form of tokens. These tokens can be utilized within the platform or exchanged for other cryptocurrencies, thus facilitating additional financial benefits for users.
Q: Is Glob Promise regulated by any financial authority?
A: As a decentralized platform, Glob Promise does not fall under the jurisdiction of any central authority. However, it adheres to strict security and regulatory frameworks to ensure compliance and security for its users.
Q: Can I use Glob Promise for business transactions?
A: Absolutely! Glob Promise caters to both individual and business users, allowing seamless and secure financial transactions across the globe. The platform’s features, such as smart contracts, facilitate efficient business transactions with reduced costs.
Embracing the Power of Glob Promise
As we embrace the digital age, financial systems continue to evolve, and the need for secure, transparent, and efficient transactions becomes more apparent. Glob Promise stands at the forefront of this new era, harnessing the power of blockchain technology to revolutionize the global financial market. By eliminating traditional intermediaries and offering direct peer-to-peer transactions, Glob Promise opens new doors of opportunity for individuals and businesses alike, empowering them to engage in the global financial ecosystem seamlessly. The future of finance lies within platforms like Glob Promise, driving innovation and transforming the way we access and utilize our financial resources.
Images related to the topic cannot find module ‘glob’
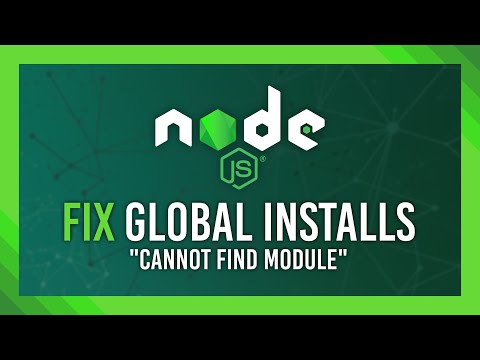
Found 37 images related to cannot find module ‘glob’ theme
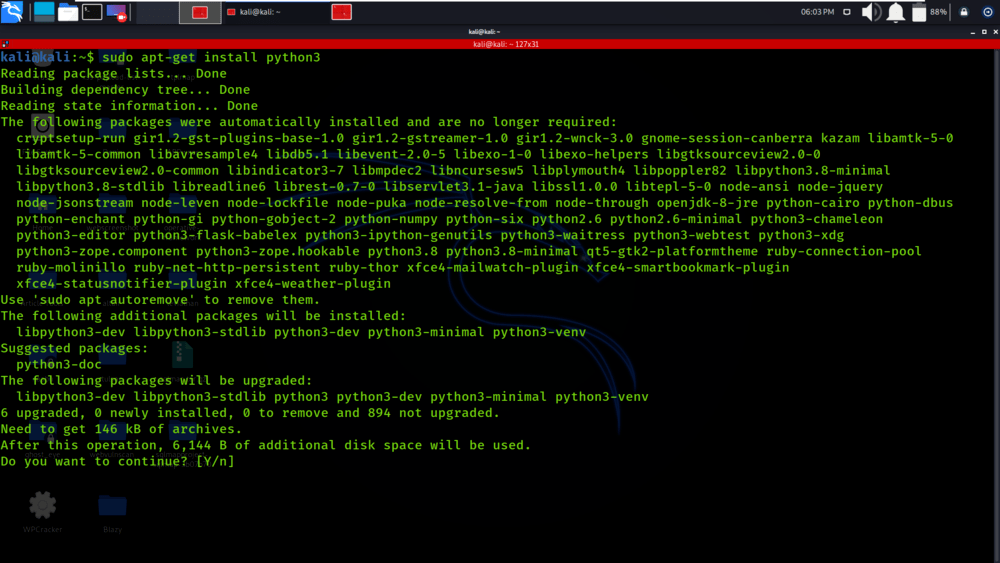
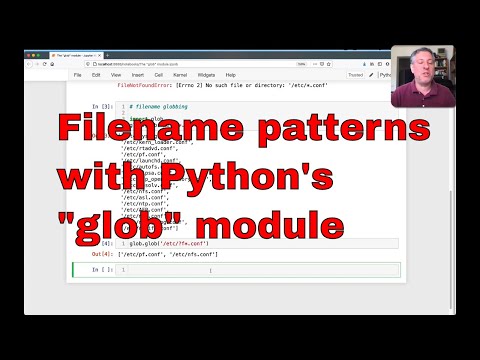
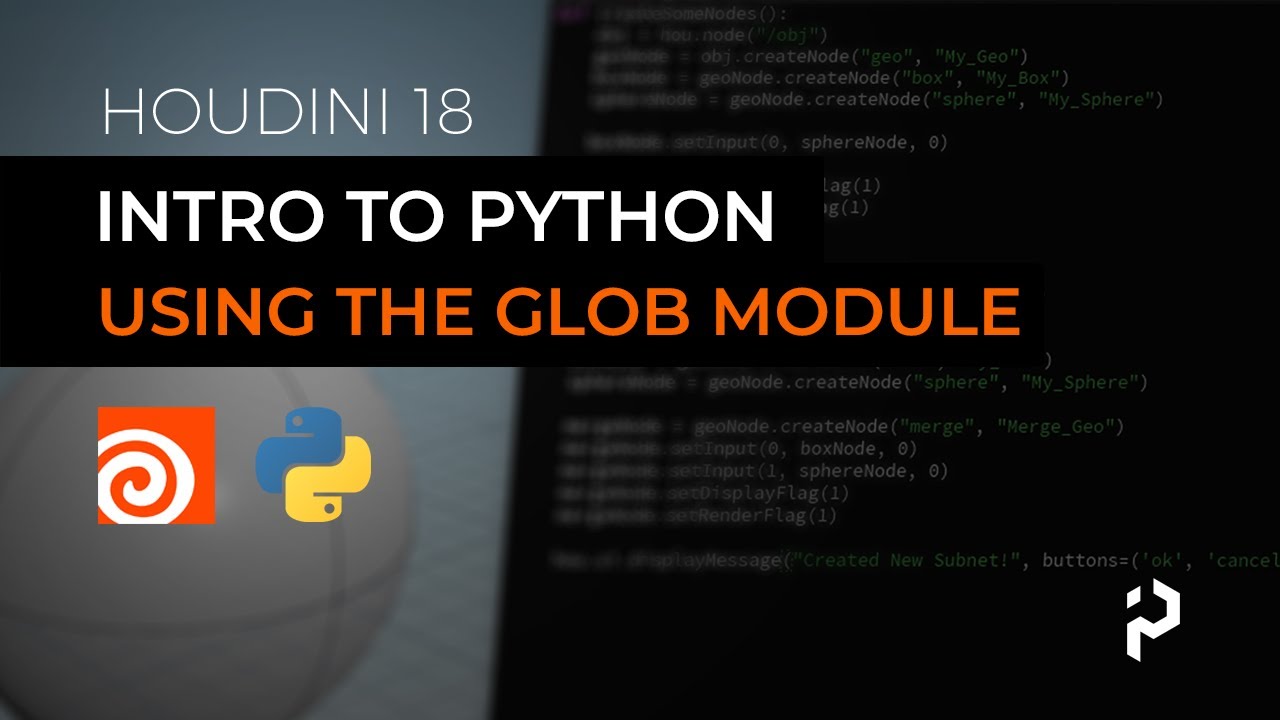

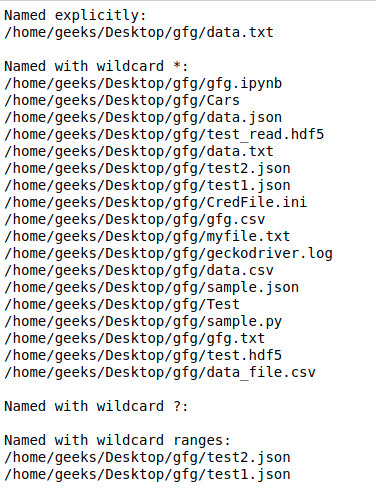
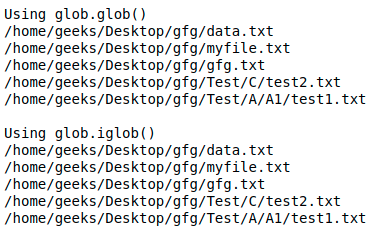
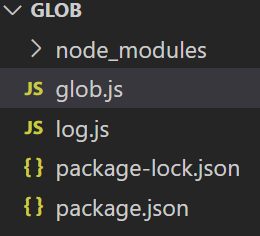
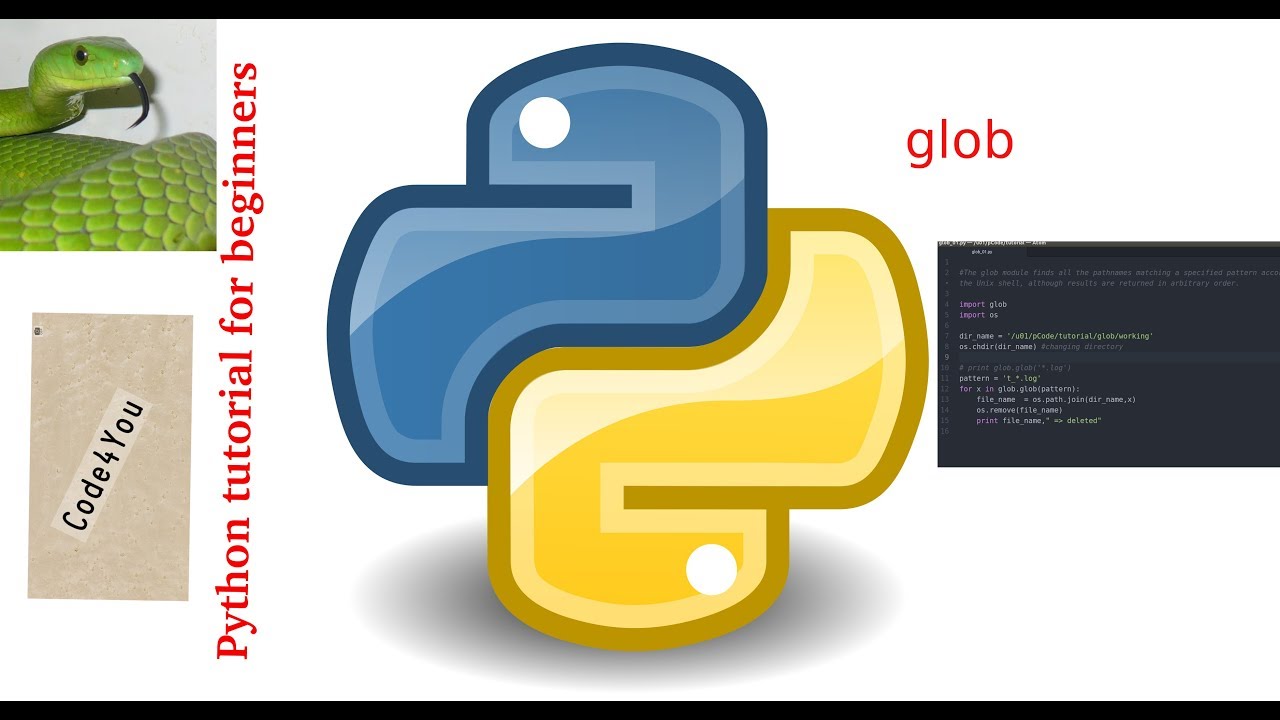

![Python [glob] The Glob Module - YouTube Python [Glob] The Glob Module - Youtube](https://i.ytimg.com/vi/ddylVRjAAco/hqdefault.jpg)
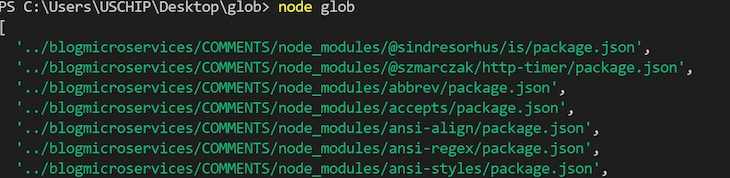

![Error: Cannot find module 'glob' [SOLVED] | GoLinuxCloud Error: Cannot Find Module 'Glob' [Solved] | Golinuxcloud](https://www.golinuxcloud.com/wp-content/uploads/2019/06/new_logo_golinuxcloud_small_progressive-3.jpg)

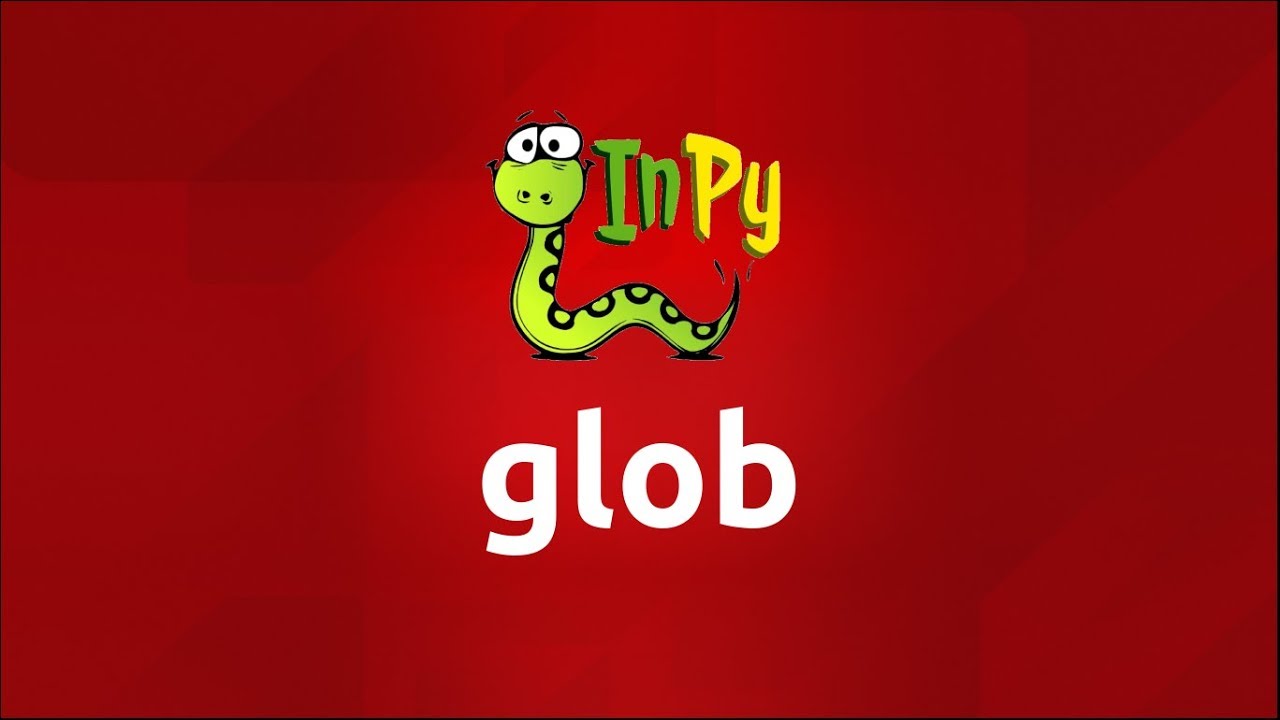
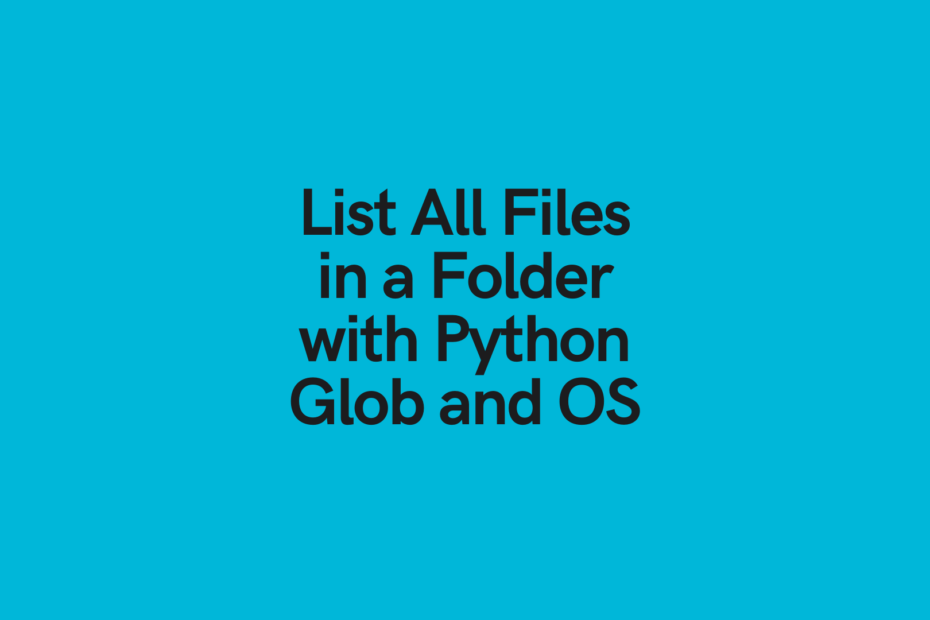
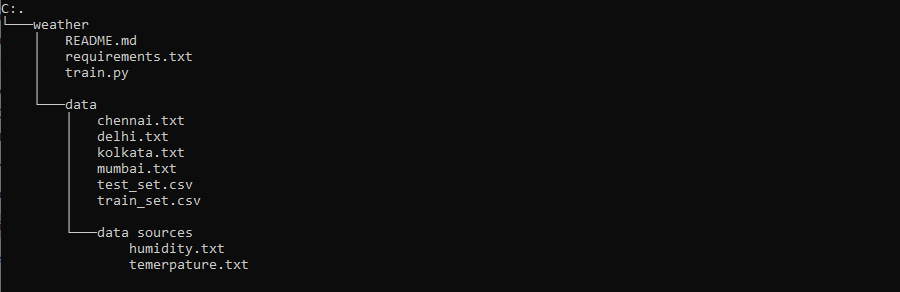

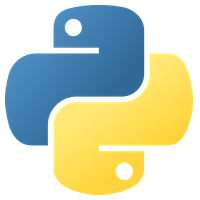
Article link: cannot find module ‘glob’.
Learn more about the topic cannot find module ‘glob’.
- Cannot find module ‘glob’ – npm – Stack Overflow
- glob – npm
- Error: Cannot find module ‘glob’ [SOLVED] – GoLinuxCloud
- Understanding the glob pattern in Node.js – LogRocket Blog
- Error: cannot find module [Node npm Error Solved] – freeCodeCamp
- How to Resolve the ‘module not found’ Error – Vercel
- How To Fix ‘MODULE_NOT_FOUND’ Error in Node.js – Tooabstractive
- Exception when try to install ring-ui Error: Cannot find module …
- Cannot find module ‘glob-all’ [#3166807] | Drupal.org
- npm install fails with “Cannot find module ‘glob'” – 汇智网
- [Solved]-Cannot find module ‘glob’-angular.js
See more: nhanvietluanvan.com/luat-hoc