Cannot Convert Float Nan To Integer
NaN (Not a Number) is a special floating-point value used to represent missing or undefined data. It typically arises as a result of calculations or operations where the data involved is not well-defined or cannot be represented numerically. In Python, NaN is represented by the float type and is available through various libraries such as NumPy and Pandas.
Understanding the limitations of converting NaN to an integer
Converting NaN to an integer can be challenging due to the fundamental differences between these two data types. NaN represents missing or undefined data, whereas integers represent whole numbers without any decimal places. Thus, converting NaN to an integer is not a valid operation as the two concepts are incompatible.
Methods to convert NaN to an integer in Python
Despite the limitations mentioned above, Python provides several methods to handle NaN values. These methods allow you to handle missing or undefined data appropriately, but they do not involve directly converting NaN to an integer. Instead, they focus on handling NaN in a way that is consistent with the intended usage of NaN.
1. Drop NaN values: One common approach is to simply remove rows or columns containing NaN values. This method is often used when the presence of NaN values is undesirable, such as in statistical analyses where missing data can lead to biased results.
2. Replace NaN with a specific value: Another option is to replace NaN values with a predefined value. This can be achieved using functions like fillna() in Pandas or replace() in NumPy. By replacing NaN with a specific numeric value, you can perform subsequent operations that require integers without encountering any errors.
3. Assign a default value: In some cases, you may want to replace NaN with a default value that is specific to your application. For example, if you are working with a dataset where missing data represents a certain condition, you can assign a value that reflects that condition rather than simply replacing it with a generic numeric value.
Dealing with NaN during mathematical operations
NaN values can have a significant impact on mathematical calculations. When NaN is involved, the result of mathematical operations is usually another NaN. However, Python provides functions and methods to handle NaN during mathematical operations in a way that ensures consistency and avoids errors. Some common methods include:
1. Ignoring NaN: Certain mathematical functions in Python have an option to ignore NaN values. For example, NumPy’s nanmean() function calculates the mean of an array, ignoring any NaN values present.
2. Checking for NaN: Another approach is to check for the presence of NaN before performing a mathematical operation. Functions such as isnan() in NumPy and Pandas can be used to identify NaN values and handle them accordingly.
Best practices for handling NaN values
When working with NaN values, it is essential to follow some best practices to ensure your code behaves as expected and produces accurate results:
1. Identify NaN values: Before performing any operations on a dataset, it is crucial to identify and locate NaN values. This can be done using functions like isnull() in Pandas or isnan() in NumPy.
2. Handle NaN consistently: Consistency is key when dealing with NaN values. Make sure to apply the same handling approach consistently throughout your codebase to ensure coherent and predictable results.
3. Consider the context: Before deciding how to handle NaN values, consider the context in which they occur. The appropriate handling approach may vary depending on the specific use case and the desired outcome.
4. Document your approach: When working with datasets that contain NaN values, documenting your approach to handling them is crucial. This helps others understand your code and its behavior in case they need to modify or adapt it.
FAQs
Q: What is “Int nan python”?
A: “Int nan python” refers to the process of converting a NaN value to an integer data type in the Python programming language. However, due to the fundamental differences between NaN and integers, converting NaN to an integer directly is not possible.
Q: How to convert float to int in Pandas?
A: In Pandas, you can use the astype() method to convert a float column to an integer column. However, it is important to note that astype() will raise an error if the column contains NaN values. To convert a float column to an integer column while ignoring NaN values, you can use the fillna() and astype() methods together.
Q: What is “Numpy nan”?
A: “Numpy nan” refers to the representation of NaN (Not a Number) values in the NumPy library. NumPy provides a special floating-point value, nan, to represent missing or undefined data.
Q: How to ignore NaN values in Pandas astype()?
A: To ignore NaN values when converting data types using the astype() method in Pandas, you can first fill the NaN values with a specific value using the fillna() method, and then apply the astype() method to perform the conversion.
Q: What does “Cannot convert non finite values (NA or inf) to integer” mean?
A: This error message occurs when attempting to convert non-finite values (such as NaN or infinity) to an integer data type. Since integers cannot represent non-finite values, the conversion is not possible.
Q: How to handle the “Could not convert string to float” error?
A: The “Could not convert string to float” error typically occurs when attempting to convert a string to a float data type using functions like float() or astype(). To resolve this error, ensure that the string input is a valid float representation and does not contain any non-numeric characters or extra whitespace.
Q: How to convert an array to float in NumPy?
A: To convert an array to a float data type in NumPy, you can use the astype() method with the “float” specifier. This will convert all the elements of the array to floats, including any NaN values present.
In conclusion, converting NaN to an integer in Python is not a valid operation due to the incompatibility between these two data types. However, Python provides various methods to handle NaN values effectively and perform mathematical operations while considering the presence of NaN. By following best practices and understanding the limitations of NaN and integer conversions, you can ensure accurate and reliable data handling in your Python code.
How To Fix Value Error: Cannot Convert Float Nan To Integer
How To Convert Float Nan To Integer?
NaN, which stands for Not a Number, is a special floating-point value commonly used to represent undefined or unrepresentable results in mathematical calculations. While working with numeric data, you may encounter situations where you need to convert a NaN value to an integer in order to perform further operations. In this article, we will explore various methods to convert float NaN to an integer, along with their implications and use cases.
Method 1: Using the math Package
The math package in most programming languages provides various functions to manipulate numeric data. One such function is isnan(), which allows you to check if a given value is NaN. By incorporating this function, you can efficiently convert float NaN to an integer. However, it’s important to note that the result will depend on the implementation of this function and the programming language you are using.
To convert float NaN to integer using the math package, follow these steps:
1. Use the isnan() function to check if the given float number is NaN.
2. If it is NaN, convert it to an integer using the appropriate conversion method according to your programming language.
3. Handle any exceptions or edge cases that may arise during the conversion process.
Method 2: Manual Conversion
In some cases, the math package may not be available or suitable for the task at hand. In these situations, you can manually convert float NaN to an integer using logical statements and control flow structures provided by your programming language.
To manually convert float NaN to integer, follow these steps:
1. Check if the given float number is NaN by comparing it with itself. NaN values are unique in that they never compare equal to any other value, including themselves.
2. If the check returns true, assign the desired integer value to the NaN. Again, this step will depend on how NaN values are represented in your programming language.
3. Handle any possible exceptions or unusual cases that may occur during this process.
FAQs:
Q1: Why would I need to convert float NaN to an integer?
A1: Converting float NaN to an integer is often necessary when dealing with data analysis, statistical calculations, or any situation where undefined or missing values need to be handled in a specific way. By converting NaN to an integer, you can effectively perform mathematical operations and make meaningful comparisons.
Q2: Can I convert any float value to an integer using these methods?
A2: No, these methods primarily serve the purpose of converting NaN to an integer. If you attempt to use these methods on a regular float value, it will simply return that same value without any changes.
Q3: What are the potential risks when converting float NaN to an integer?
A3: The main risk lies in the potential loss of information. NaN values are often used to indicate missing or undefined data, and converting them to integers may result in a loss of that valuable information. Additionally, if the desired integer value is outside the range that can be represented as an integer, you may encounter overflow or underflow exceptions.
Q4: Are there any limitations or considerations specific to certain programming languages?
A4: Yes, each programming language may have its own way of representing and handling NaN values. It’s crucial to refer to the documentation and guidelines provided by the language to ensure accurate conversion.
Q5: Can I convert NaN to a specific integer value?
A5: Yes, in most programming languages, you can assign a specific integer value to NaN during the conversion process. This can be useful when NaN represents a particular missing data category and you want to assign a distinct value to it.
In conclusion, converting float NaN to an integer is a significant task when dealing with numeric data. Understanding how to convert NaN in your chosen programming language is essential to ensure accurate calculations and meaningful comparisons. By following the methods discussed above and considering the FAQs, you can successfully convert float NaN to an integer, leveraging the appropriate techniques provided by your programming language.
What Does Cannot Convert Float Nan To Integer Mean?
Introduction:
Many programmers frequently encounter the error message “Cannot convert float NaN to integer” while working with numerical data in programming languages. This article aims to provide a thorough explanation of what this error means, why it occurs, and how to address it. Additionally, a Frequently Asked Questions (FAQs) section will address common concerns surrounding this issue.
—
Section 1: Understanding the Error Message
When attempting to convert a floating-point number represented as “NaN” (Not a Number) to an integer, programming languages often raise an error. This error typically occurs when trying to manipulate or perform calculations involving undefined or invalid numerical values.
Section 2: Why Does this Error Occur?
The occurrence of the “Cannot convert float NaN to integer” error can be traced back to the IEEE 754 floating-point standard used in most modern programming languages. According to this standard, “NaN” is utilized to represent non-numerical values, such as the result of an invalid mathematical operation, division by zero, or an overflow/underflow condition.
When attempting to convert this invalid numerical representation into an integer, the programming language detects the inconsistency between the floating-point NaN and the requested integer type, prompting an error message.
Section 3: Common Scenarios Triggering the Error
1. Performing arithmetic with invalid inputs:
– Dividing a number by zero
– Taking the square root of a negative number
2. Attempting to apply a mathematical operation to an undefined value:
– Involving a variable that has not been assigned a numerical value yet
3. Handling data from external sources:
– Reading data from a file or database where invalid or missing values are present
Section 4: Handling the “Cannot convert float NaN to integer” Error
When encountering this error, it is crucial to identify and address the root cause. Here are a few approaches to handle the situation:
1. Error avoidance:
– Make sure to check for invalid or missing values before performing any arithmetic operations.
– Use conditional statements or data validation techniques to ensure only valid data is used in numerical calculations.
2. Implement exception handling:
– Use try-catch blocks or error-handling mechanisms provided by the programming language to handle specific exceptions and gracefully address NaN instances.
3. Data preprocessing:
– Check and clean the input data for any NaN values before performing computations.
– Replace NaN values with appropriate placeholders or perform data imputation techniques to fill missing values appropriately.
—
FAQs (Frequently Asked Questions):
Q1: Can NaN be converted to any other type?
A1: NaN can be converted to string or boolean types, as these conversions don’t involve numerical computation.
Q2: Is NaN the same as NULL or undefined in programming?
A2: No, NaN is specific to floating-point numerical computations, while NULL or undefined typically refer to the absence of a valid value in other contexts.
Q3: Can NaN occur in datasets without explicit operations?
A3: Yes, NaN can arise from external data sources, such as importing or parsing data that might contain missing or corrupted values.
Q4: Is it possible to prevent NaN occurrences entirely?
A4: While it may not be entirely preventable, solid data validation techniques, error handling, and pre-processing steps can significantly minimize NaN instances.
—
Conclusion:
Understanding and addressing the “Cannot convert float NaN to integer” error is crucial for effective programming with numerical data. By adopting proper error handling practices and consistently validating data inputs, developers can ensure smooth execution of calculations, minimizing unexpected errors related to NaN values.
Keywords searched by users: cannot convert float nan to integer Int nan python, Convert float to int pandas, Numpy nan, Pandas astype ignore NaN, Astype float, Cannot convert non finite values (NA or inf) to integer, Could not convert string to float, Convert array to float numpy
Categories: Top 46 Cannot Convert Float Nan To Integer
See more here: nhanvietluanvan.com
Int Nan Python
Python is a highly popular programming language known for its simplicity and versatility. It is widely used for web development, scientific computing, data analysis, artificial intelligence, and more. One of the essential functionalities in Python is the ability to perform arithmetic operations with ease. Python provides various built-in data types to handle numbers, including integers (int), floating-point numbers (float), and complex numbers (complex).
In this article, we will explore the int data type in Python, its features, and its applications in programming. We will dive into the details of integers in Python, including their syntax, operations, and common use cases. So, let’s get started!
Understanding Integers in Python
An integer is a whole number, either positive or negative, without any fractional or decimal part. In Python, the int data type represents integers. Integers can be specified directly in code using their numeric value, for instance:
“`python
x = 42
“`
In the above example, we assign the integer value of 42 to the variable x. Python treats this value as an int object. You can perform various numerical operations on integers, including addition, subtraction, multiplication, and division.
Integers support both unary and binary arithmetic operators. Unary operators are applied to a single operand, while binary operators require two operands. Here are some examples:
“`python
x = 5
y = -3
# Unary operator
z = -x # Negation: -5
# Binary operators
add = x + y # Addition: 2
sub = x – y # Subtraction: 8
mul = x * y # Multiplication: -15
div = x / y # Division: -1.6666666666666667
“`
In the above code snippet, we perform various arithmetic operations on the integers x and y. Unary negation is achieved using the `-` operator, while binary operations use the respective arithmetic operators.
Advanced Features and Methods for Integers
Python’s int data type offers some advanced features and methods to manipulate or extract information from integers. Let’s explore a few of them:
1. Integer Division `//`: The double forward slash (`//`) performs integer division, which returns the quotient without the fractional or decimal part. For example:
“`python
result = 9 // 2 # 4
“`
In the above example, integer division of 9 by 2 results in 4.
2. Modulus `%`: The modulus operator (`%`) returns the remainder of the division between two integers. Here is an example:
“`python
remainder = 9 % 2 # 1
“`
The above code calculates the remainder when 9 is divided by 2.
3. Exponentiation `**`: Python supports exponentiation using the double asterisk (`**`). It raises the left operand to the power of the right operand. For instance:
“`python
result = 2 ** 3 # 8
“`
In the above example, 2 raised to the power of 3 results in 8.
4. Conversion Between Different Bases: Python provides built-in functions to convert integers from one base to another. The `bin()` function converts an integer to its binary representation, `oct()` converts to octal, and `hex()` converts to hexadecimal. Here is an example:
“`python
binary = bin(10) # ‘0b1010’
“`
The `bin(10)` call converts the integer 10 to its binary representation ‘0b1010’.
Common Use Cases and FAQs
1. Why use integers in Python programming?
Integers are fundamental data types used for counting, indexing, calculations, and iterations. They enable programmers to work with whole numbers and perform arithmetic operations efficiently.
2. Can integers represent extremely large or small numbers?
Yes, Python supports arbitrary precision integers, which can represent numbers with an unlimited number of digits accurately. These integers offer increased precision compared to fixed-size integers found in some other programming languages.
3. How can I convert a string to an integer in Python?
Python provides the `int()` function to convert a string to an integer. For example:
“`python
number = int(“42”) # 42
“`
The `int(“42”)` call converts the string “42” to the integer value 42.
4. Can integers be used as dictionary keys in Python?
Yes, integers are immutable, and hence, they can be used as dictionary keys. This allows efficient access and retrieval of values based on integer keys.
5. Are there any limitations on the size of integers in Python?
Python’s arbitrary precision integers can grow as large as the computer’s memory allows. However, the operations on extremely large integers might take more time and memory compared to normal-sized integers.
Conclusion
Integers are an essential part of any programming language, including Python. Python’s int data type provides a flexible and powerful way to handle whole numbers in various applications. In this article, we discussed the features, methods, and use cases of integers in Python programming. By leveraging these capabilities, programmers can perform calculations, conversions, and access numerical information efficiently. Understanding the int data type in Python will help you write more sophisticated and accurate algorithms.
Convert Float To Int Pandas
Introduction
Working with numerical data is an integral part of data analysis and machine learning tasks. In many cases, the data we receive may contain floating-point numbers (floats). However, for specific purposes such as indexing or integer-related operations, it may be necessary to convert these float values to integers. Pandas, a powerful data manipulation library in Python, provides us with tools and methods to effortlessly perform this conversion. In this article, we will explore various techniques to convert floats to integers in pandas, providing detailed explanations and examples along the way.
Table of Contents
1. The Need for Converting Floats to Integers in Pandas
2. Working with Series Objects
3. Altering the Data Type using .astype()
4. Truncating Decimal Places using .astype(int)
5. Dealing with NaN (Not a Number)
6. Converting Floats to Integers in DataFrames
7. Handling Data Loss during Conversion
8. Performance Considerations
9. FAQs
9.1. Can I convert a float to an integer without losing any data?
9.2. How can I handle NaN values during the conversion?
9.3. Are there any performance implications when using these conversion methods?
9.4. Can I convert a subset of columns in a DataFrame from float to int?
9.5. Is it possible to convert floats to integers with a certain rounding rule?
1. The Need for Converting Floats to Integers in Pandas
Floats are decimal numbers that can have both whole and fractional parts, while integers represent whole numbers. While floats are useful for precise calculations, integers are often required for indexing, counting, or other integer-oriented operations. Converting floats to integers in pandas allows us to eliminate decimal places and work with whole numbers.
2. Working with Series Objects
In pandas, a Series is a one-dimensional labeled array that can contain data of any type. Converting floats to integers in a Series can be achieved using the .astype() method. Here’s an example:
“`
import pandas as pd
# Create a Series with float values
data = pd.Series([2.5, 4.8, 3.1, 1.9, 7.2])
# Convert float to int
data = data.astype(int)
print(data)
“`
Output:
“`
0 2
1 4
2 3
3 1
4 7
dtype: int64
“`
3. Altering the Data Type using .astype()
The .astype() method allows us to change the data type of a Series explicitly. By passing ‘int’ as an argument, we can convert the float values to integers. Note that this operation does not perform any rounding, it simply removes the decimal places.
4. Truncating Decimal Places using .astype(int)
In some cases, rounding the float values to the nearest integer might be required. To achieve this, we can apply the .astype(int) method with a prior round() operation. Let’s see an example:
“`
import pandas as pd
# Create a Series with float values
data = pd.Series([3.6, 4.2, 9.7])
# Round and convert float to int
data = data.round().astype(int)
print(data)
“`
Output:
“`
0 4
1 4
2 10
dtype: int64
“`
In the above example, each float value is rounded to the nearest integer before being converted to an integer.
5. Dealing with NaN (Not a Number)
In pandas, NaN represents missing or undefined values. During the conversion of floats to integers, pandas automatically converts NaN values to the NaN-int format. This is a special representation of NaN as the integer data type does not have a direct NaN equivalent. For example:
“`
import pandas as pd
import numpy as np
# Create a Series with NaN values
data = pd.Series([2.5, np.nan, 1.8, np.nan])
# Convert float to int
data = data.astype(int)
print(data)
“`
Output:
“`
0 2
1 -9223372036854775808
2 1
3 -9223372036854775808
dtype: int64
“`
In the above output, the NaN values are converted to special integer representations (-9223372036854775808) that signify missing or undefined values.
6. Converting Floats to Integers in DataFrames
A DataFrame is a two-dimensional data structure in pandas that consists of columns with potentially different data types. To convert float values to integers in a DataFrame, we can use the .astype() method along with specifying the column(s) of interest. Here’s an example:
“`
import pandas as pd
# Create a DataFrame with float values
data = pd.DataFrame({‘A’: [1.7, 2.3, 4.8], ‘B’: [2.4, 3.1, 5.9]})
# Convert float to int for column ‘A’
data[‘A’] = data[‘A’].astype(int)
print(data)
“`
Output:
“`
A B
0 1 2.4
1 2 3.1
2 4 5.9
“`
In the above example, only the values in column ‘A’ were converted to integers, leaving column ‘B’ unchanged.
7. Handling Data Loss during Conversion
When converting floats to integers, it’s important to be aware of potential data loss. Integer data types do not support decimal places, so any float value with non-zero decimal components will be truncated. This can lead to precision loss and affect subsequent calculations or analysis. It’s crucial to consider whether converting floats to integers is appropriate for the specific task at hand.
8. Performance Considerations
While pandas provides convenient methods for converting floats to integers, it’s important to consider performance, especially when dealing with large datasets. Converting data types can be a computationally expensive operation, so it’s advisable to only perform the conversion when necessary or to optimize the process by utilizing vectorized operations.
FAQs
9.1. Can I convert a float to an integer without losing any data?
No, converting a float to an integer will always result in potential data loss, as integers cannot represent fractional parts. The decimal places will be truncated, and any non-zero decimal components will be lost.
9.2. How can I handle NaN values during the conversion?
Pandas automatically converts NaN values to the NaN-int format, which represents missing or undefined values. These NaN-int representations can be processed accordingly, depending on the specific analysis or use case.
9.3. Are there any performance implications when using these conversion methods?
Converting data types can be performance-intensive, especially for large datasets. To improve performance, it’s recommended to perform type conversions only when necessary and to utilize vectorized operations whenever possible.
9.4. Can I convert a subset of columns in a DataFrame from float to int?
Yes, you can convert specific columns by accessing them using indexing or column names. By applying the .astype() method individually to the desired columns, you can convert only those columns from float to int.
9.5. Is it possible to convert floats to integers with a certain rounding rule?
Yes, you can implement a rounding rule before converting by using the round() function in combination with the .astype(int) method. This allows you to control the rounding behavior before the actual conversion takes place.
Conclusion
Converting floats to integers is a common task in data analysis, and pandas offers powerful tools to perform this transformation seamlessly. In this article, we have explored various methods to convert float values to integers in pandas, covering Series objects, DataFrames, handling NaN values, performance considerations, and more. By carefully considering the specific requirements of your analysis and data, you can effectively convert floats to integers while minimizing data loss and optimizing performance.
Images related to the topic cannot convert float nan to integer
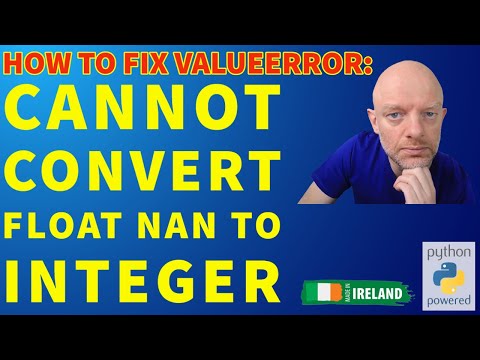
Found 44 images related to cannot convert float nan to integer theme





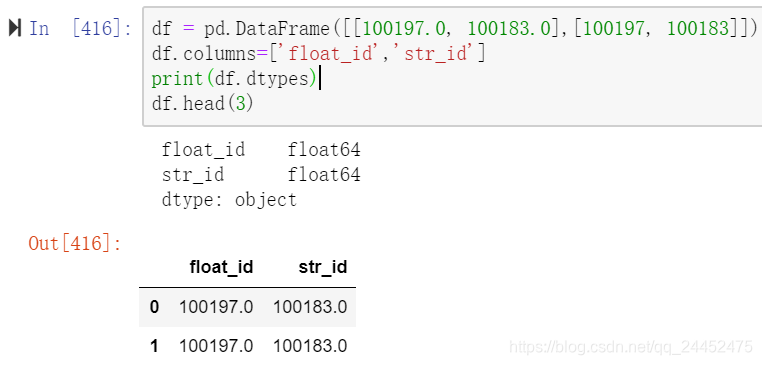
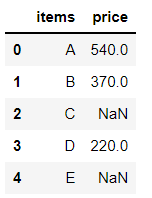


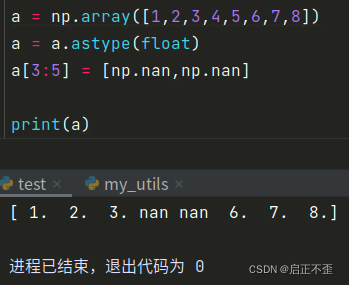


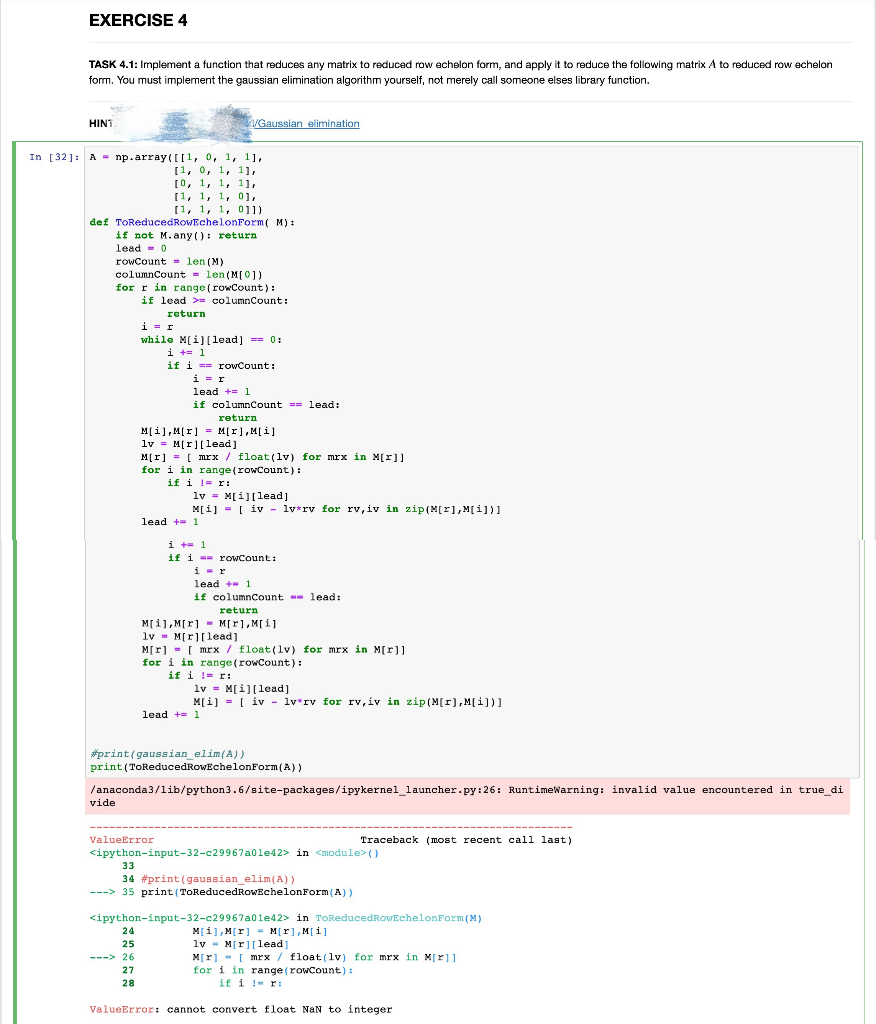


![Fixed] Image Data of Dtype Object Cannot be Converted to Float Fixed] Image Data Of Dtype Object Cannot Be Converted To Float](https://www.pythonpool.com/wp-content/uploads/2023/02/image-data-of-dtype-object-cannot-be-converted-to-float.webp)





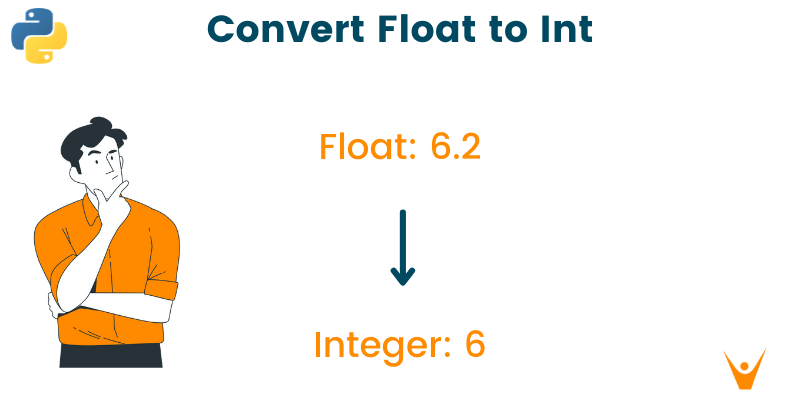

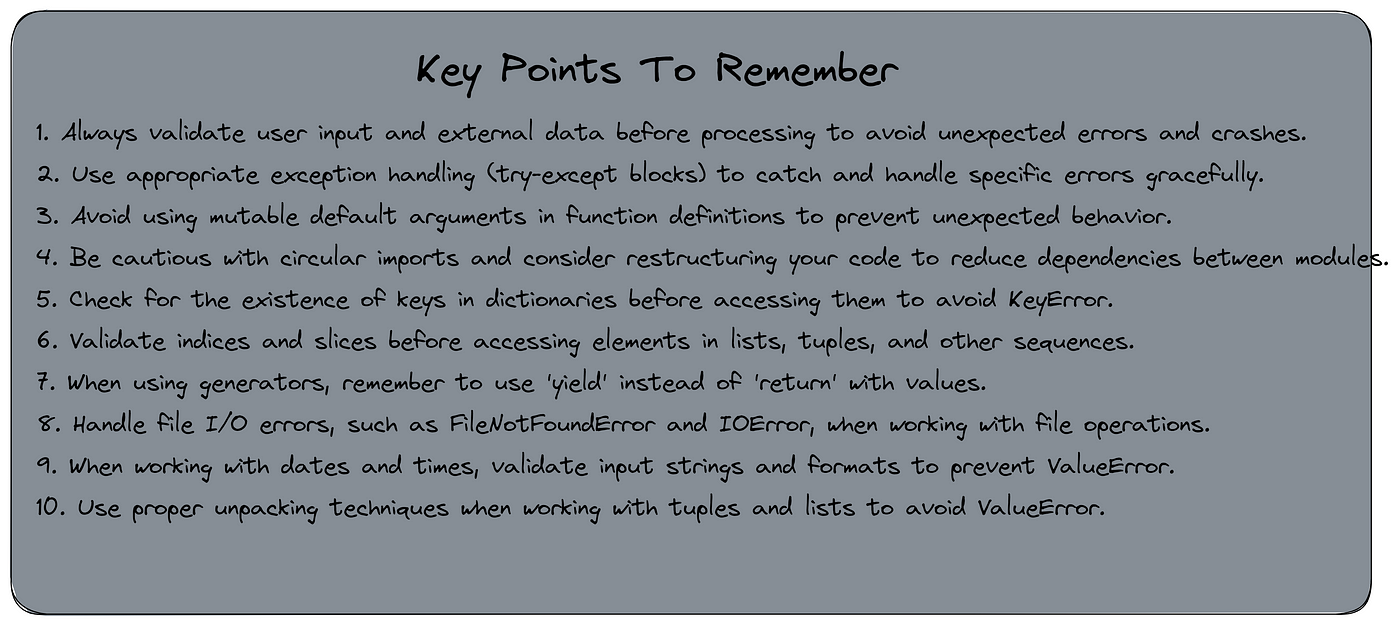

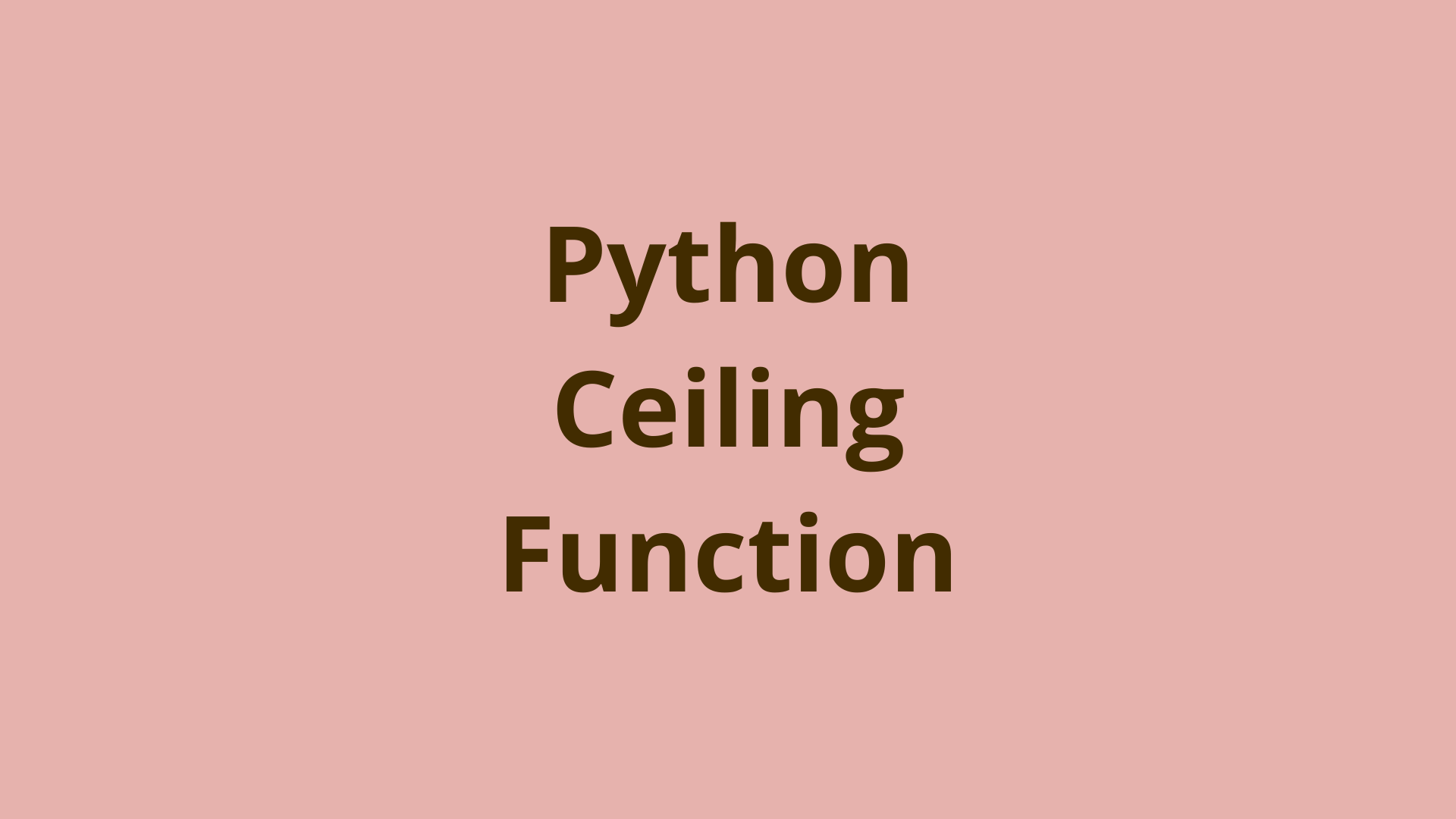

Article link: cannot convert float nan to integer.
Learn more about the topic cannot convert float nan to integer.
- valueerror: cannot convert float nan to integer ( Solved )
- How to Fix: ValueError: cannot convert float NaN to integer
- Pandas: ValueError: cannot convert float NaN to integer
- How to Fix: ValueError: cannot convert float NaN to integer
- ValueError: cannot convert float NaN … – Net-Informations.Com
- How to Fix: ValueError: cannot convert float NaN to integer
- How to convert Float to Int in Python? – GeeksforGeeks
- Pandas Convert Float to Integer in DataFrame – Spark By {Examples}
- ValueError: cannot convert float NaN … – Net-Informations.Com
- Valueerror: cannot convert float nan to integer
- ValueError: Cannot Convert Float NaN to Integer in Python
See more: nhanvietluanvan.com/luat-hoc