Cannot Assign To Function Call
The “cannot assign to function call” error is a common error message encountered by programmers in various programming languages, such as Python. This error occurs when we attempt to assign a value to a function call, which is not allowed because function calls are not mutable.
In programming, assignment statements are used to assign values to variables. A variable is like a container that holds a value, and we can change the value stored in the variable by using assignment statements. On the other hand, function calls are used to execute a function and retrieve a result. When we try to assign a value to a function call, it leads to the “cannot assign to function call” error.
Common Causes of the “Cannot Assign to Function Call” Error
There are several reasons why the “cannot assign to function call” error might occur:
1. Confusion between function calls and assignment statements: One common cause of this error is mistakenly treating a function call as a variable and trying to assign a value to it. It is important to understand the fundamental difference between a function call, which is a statement that executes code, and an assignment statement, which assigns a value to a variable.
2. Immutable objects: In some cases, the error might occur when attempting to assign a value to an immutable object within a function call. Immutable objects are objects whose state cannot be changed once they are created, such as strings or tuples. Since these objects cannot be modified, trying to assign a new value to them will result in the “cannot assign to function call” error.
3. Incorrect usage of assignment operators: Another cause of this error is incorrect usage of assignment operators, such as using the comparison operator (==) instead of the assignment operator (=) to assign a value. The comparison operator checks for equality, whereas the assignment operator is used to assign a value to a variable.
Understanding the Difference between Function Calls and Assignment Statements
To avoid the “cannot assign to function call” error, it is important to understand the difference between function calls and assignment statements.
Function calls are used to execute a function and obtain the result of that function. They are typically written with the function name followed by parentheses, which may contain input parameters if the function requires any. For example:
result = my_function(parameter)
On the other hand, assignment statements are used to assign values to variables. They are typically written with a variable name on the left side of the assignment operator (=) and a value or expression on the right side. For example:
x = 5
Attempting to assign a value to a function call, such as:
my_function(parameter) = result
will result in the “cannot assign to function call” error because function calls are not mutable.
Exploring Examples of the “Cannot Assign to Function Call” Error
Let’s explore some examples to better understand when and how the “cannot assign to function call” error occurs.
Example 1:
“`
def get_total(a, b):
return a + b
get_total(2, 3) = result
“`
In this example, we are attempting to assign the result of the `get_total` function to the variable `result`. However, since function calls are not assignable, this code will produce the “cannot assign to function call” error.
Example 2:
“`
my_string = “Hello, World!”
my_string.upper() = “HELLO”
“`
In this example, we are trying to assign the result of the `upper` method, which converts a string to uppercase, to the original string. However, strings are immutable objects, so we cannot modify them in-place. Therefore, this code will result in the “cannot assign to function call” error.
How to Troubleshoot and Debug the “Cannot Assign to Function Call” Error
When encountering the “cannot assign to function call” error, it is important to troubleshoot and debug the code to identify the root cause. Here are some techniques that can help:
1. Review the error message: Read the error message carefully to understand the specific line of code where the error occurred. This can provide valuable clues about the cause of the error.
2. Check for incorrect assignment statements: Double-check all assignment statements in the code to ensure that they are correctly written and do not attempt to assign a value to a function call.
3. Identify immutable objects: If the error occurs when assigning a value to an object, determine whether that object is immutable. If it is immutable, consider using a different approach or creating a new object if modification is required.
Techniques to Avoid the “Cannot Assign to Function Call” Error
To avoid encountering the “cannot assign to function call” error, there are a few techniques you can employ:
1. Understand the purpose of functions: Functions are meant to execute code and return a result, not to be assigned values. Ensure that you have a clear understanding of the role of functions in your code.
2. Pay attention to data types: Ensure that you are assigning values to variables of the correct data type. For example, make sure you are not trying to assign a value to a function call when you should be assigning it to a variable of the appropriate type.
3. Use temporary variables: If you need to modify the result of a function call or assign it to a variable, use a temporary variable to hold the result. Then, you can perform any necessary modifications or assignments on the temporary variable.
Best Practices for Working with Functions and Assignment Statements
To avoid the “cannot assign to function call” error and write clean, maintainable code, it is important to follow some best practices when working with functions and assignment statements:
1. Use descriptive variable names: Choose meaningful and descriptive names for your variables to improve code readability and reduce the chances of making mistakes.
2. Separate logic into smaller functions: Break down complex tasks into smaller, reusable functions. This improves code organization and makes it easier to identify and fix errors.
3. Test your code incrementally: Test your code regularly and incrementally to catch potential errors early on. This helps in identifying any issues related to function calls and assignment statements.
Tips for Handling Complex Scenarios and Nested Function Calls
In more complex scenarios and when dealing with nested function calls, it is important to keep the following tips in mind:
1. Understand the order of evaluation: When working with nested function calls, ensure that you understand the order in which the functions are evaluated. This will help you avoid errors related to assigning values to function calls.
2. Use intermediate variables: If you have multiple nested function calls, consider using intermediate variables to store the results of each function call. This can help in reducing complexity and makes the code easier to understand and debug.
Resources and Tools for Further Learning about the “Cannot Assign to Function Call” Error
To further expand your knowledge about the “cannot assign to function call” error and related topics, here are some helpful resources and tools:
1. Documentation: Language-specific documentation is a valuable resource for understanding the rules and limitations around function calls and assignment statements.
2. Online forums and communities: Participate in online forums and communities dedicated to your programming language of choice. These platforms often have experienced developers who can provide guidance and solutions to specific issues.
3. Debugging tools: Utilize debugging tools provided by your programming environment to step through your code and identify the point at which the “cannot assign to function call” error occurs.
Conclusion
The “cannot assign to function call” error is a common error that programmers encounter when trying to assign a value to a function call. By understanding the difference between function calls and assignment statements, identifying common causes, and employing troubleshooting techniques, you can effectively handle this error. Remember to follow best practices, handle complex scenarios with care, and use available resources for further learning to become more proficient in avoiding and resolving this error.
\”Debugging Tutorial: How To Fix ‘Syntaxerror: Can’T Assign To Function Call’ \”
What Does Cannot Assign Function To Call Mean?
When working with code, especially in programming languages like JavaScript, you may encounter various error messages that can sometimes be cryptic and challenging to understand. One common error message is “Cannot assign function to call.” In this article, we will explore the meaning of this error message, its possible causes, and how to address it.
Understanding the error message:
The error message “Cannot assign function to call” suggests that you are attempting to assign a function to the call of a function, rather than assigning the function itself. To better comprehend this, let’s break down the error message into its key components.
1. “Cannot assign”: This implies that there is an issue with assigning something.
2. “Function”: Refers to a piece of code that performs a specific task.
3. “To call”: Indicates that the function call is incorrect or incompatible.
Possible causes of the error:
1. Syntax error: The error message may occur due to a syntax error in your code. Make sure that the function call and assignment are written correctly, without any typos or missing characters.
2. Mismatched parentheses: If you are dealing with a function call that has mismatched parentheses, it can cause this error message. Ensure that the opening and closing parentheses for each function call are properly balanced.
3. Incorrect function assignment: The error can also occur if you mistakenly attempt to assign a function call rather than the function itself. This typically happens when assigning the return value of a function to another function call. However, functions should be assigned without parentheses.
4. Incompatible assignments: Sometimes, the error message indicates that you are attempting to assign a function to a variable or assignment that expects a different type of data. This often arises when dealing with incompatible data types or mismatches between function signatures and assignments.
Resolving the issue:
To resolve the “Cannot assign function to call” error, you can follow these steps:
1. Check for syntax errors: Review your code for any syntax errors, such as missing semicolons, incorrect variable names, or improper placement of parentheses. Fixing syntax errors can often resolve this issue.
2. Verify function assignments: Ensure that you are assigning the function itself, not the result of calling the function. Remove any parentheses when assigning a function to a variable or another function call.
3. Debug the function call: If you are assigning a function result to another function call, check the documentation or source code of the function you are calling. Verify the expected return type and argument requirements. Adjust your code accordingly to avoid any incompatible assignments.
4. Double-check data types: If the error persists, review the data types involved in the assignment. Make sure that you are assigning the function to a compatible variable or assignment that expects a function, not any other data type.
FAQs:
Q1: Why am I getting the “Cannot assign function to call” error message?
A1: This error message usually occurs due to incorrect syntax, mismatched parentheses, incorrect function assignment, or incompatible assignments. Review your code for these issues and make appropriate adjustments.
Q2: What is the difference between a function and a function call?
A2: A function is a block of code that performs a specific task, while a function call is the act of invoking or executing that function.
Q3: How can I avoid the “Cannot assign function to call” error?
A3: To avoid this error, ensure that you are assigning the function itself, not the result of calling the function. Pay attention to syntax, balanced parentheses, function signatures, and compatible assignments.
Q4: Can this error message occur in languages other than JavaScript?
A4: Yes, this error message can occur in other programming languages as well, especially those that follow similar function assignment and call patterns.
Q5: Are there any tools that can help me identify and fix this error?
A5: Integrated Development Environments (IDEs) such as Visual Studio Code, Xcode, or JetBrains’ IntelliJ IDEA often provide error highlighting and suggestions to help identify and fix this type of error.
In conclusion, the “Cannot assign function to call” error message can be confusing at first, but understanding its meaning and possible causes can help you debug and fix the issue efficiently. By carefully reviewing your code for syntax errors, verifying function assignments, debugging function calls, and considering data types, you can resolve this error and ensure smooth execution of your code.
What Does Can’T Assign To Operator Mean In Python?
Python is a popular programming language known for its simplicity and readability. However, like any programming language, it can sometimes produce error messages that might confuse beginners or even experienced developers. One such error message is “can’t assign to operator”. In this article, we will explore what this error means, why it occurs, and how to effectively troubleshoot and resolve it.
Understanding the Error Message:
The error message “can’t assign to operator” is raised when you attempt to assign a value to an operator that cannot be assigned to in Python. Operators, such as +, -, *, /, %, and others, are used to perform various operations on variables or values. However, some operators are not allowed to be assigned a new value because they are immutable or have a specific fixed meaning in the language.
For example, consider the following line of code:
x + y = 10
If you try to execute this code, Python will raise the “can’t assign to operator” error because the ‘+’ operator cannot be assigned a new value directly.
Causes of the Error:
The error can occur due to a number of reasons. The most common causes are:
1. Syntax Error: It is important to understand that in Python, the assignment statement follows the pattern: variable = value. If you mistakenly assign a value to an operator instead of a variable, it will result in this error.
2. Operator Immutability: Some operators, such as + and /, have fixed meanings and cannot be assigned new values. Attempting to assign a new value to these operators will result in the “can’t assign to operator” error.
3. Operator Overloading: Python allows operator overloading, which means that the behavior of an operator can be customized for different objects or classes. However, not all operators can be overloaded. If you attempt to assign a new value to an operator that does not support overloading, the error will be raised.
How to Resolve the Error:
To resolve the “can’t assign to operator” error, it is crucial to understand the cause. Here are some steps you can take to troubleshoot and resolve the error:
1. Review the Syntax: Double-check your code for any syntax errors. It is common to mistakenly write an assignment statement with the wrong syntax, such as assigning a value to an operator instead of a variable. Correcting the syntax should resolve the issue.
2. Choose a Different Variable: If you are attempting to assign a value to an operator because you intend to store the result in a variable, choose a different variable name. This will prevent conflicts with the operator and resolve the error.
3. Verify Operator Suitability: Ensure that you are using the correct operator for the desired operation. Some operators may have limitations or requirements specific to the operation being performed. Consulting the Python documentation or relevant resources can help you identify the appropriate operator to use.
4. Check for Operator Overloading Support: If you are attempting to assign a new value to an operator that supports overloading, ensure that the object or class being used supports this behavior. If not, find an alternative way to accomplish the desired functionality without assigning a new value to the operator.
FAQs:
Q: Can I assign a new value to any operator in Python?
A: No, not all operators in Python can be assigned new values. Operators such as +, -, *, /, and others have fixed meanings and cannot be reassigned directly.
Q: Are there any operators in Python that support overloading and can be reassigned?
A: Yes, some operators in Python, such as += and -=, can be overloaded and reassigned to provide custom functionality for different objects or classes.
Q: What is the most common cause of the “can’t assign to operator” error?
A: Syntax errors, such as mistakenly assigning a value to an operator instead of a variable, are a common cause of this error.
Q: How can I avoid encountering this error in my code?
A: Double-checking your code for syntax errors, understanding the purpose and limitations of operators, and following Python’s guidelines for operator usage can help you avoid this error.
In conclusion, the “can’t assign to operator” error in Python occurs when you attempt to assign a value to an operator that cannot be assigned to. By understanding the causes and following the troubleshooting steps provided, you can effectively resolve this error and improve the reliability of your Python programs.
Keywords searched by users: cannot assign to function call Cannot assign to literal
Categories: Top 98 Cannot Assign To Function Call
See more here: nhanvietluanvan.com
Cannot Assign To Literal
In the world of programming languages like Python, there are certain error messages that developers encounter from time to time. One such error message that you may come across is “Cannot assign to literal.” This error can be frustrating, especially if you aren’t familiar with its meaning or how to resolve it. In this article, we will delve into the details of this error message, understand its implications, and explore the steps to resolve it.
What does “Cannot assign to literal” mean?
To grasp the meaning behind the error message, “Cannot assign to literal,” we must first understand the concept of literals in programming. A literal is a value that is directly written into the code, such as a number, a string, or a boolean value (True/False). When you assign a value directly to a literal, you essentially create an immutable object, which means that it cannot be changed.
The error message, “Cannot assign to literal,” indicates that you are attempting to modify an immutable object directly, which is not allowed in most programming languages, including Python. In other words, you are trying to assign a new value to a literal, which is not permissible.
Common scenarios leading to the error:
1. Assignment to a number literal:
For instance:
“`
5 = 10
“`
This assignment is invalid because you are trying to change the value of the literal ‘5’ to ’10’.
2. Assignment to a string literal:
For instance:
“`
“Hello” = “Greetings”
“`
This assignment is invalid because you are trying to change the literal string ‘Hello’ to ‘Greetings’.
3. Assignment to a boolean literal:
For instance:
“`
True = False
“`
This assignment is invalid because you are trying to change the boolean literal ‘True’ to ‘False’.
How to resolve the “Cannot assign to literal” error:
Resolving this error requires understanding the core problem and applying the appropriate solution. Here are a few approaches you can take to fix the error:
1. Use variables instead of literals:
Instead of trying to assign a new value to a literal, assign the value to a variable. Variables can be changed and modified throughout the program execution. For instance:
“`
x = 5
x = 10
“`
Here, by using the variable ‘x’ instead of the literal ‘5’, you can assign a new value to ‘x’ without encountering the error.
2. Modify the code logic:
Review your code for any instances where you are mistakenly trying to change a literal. Ensure that you are making assignments to appropriate variables rather than literals. By modifying your code logic, you can avoid the “Cannot assign to literal” error altogether.
3. Understand the purpose of literals:
As we’ve mentioned earlier, literals are meant to represent fixed values and should not be changed directly. Understanding the role of literals in your code will help you avoid attempting to assign new values to them.
FAQs:
Q: Can I modify the contents of a literal indirectly?
A: Yes, you can modify the contents of a literal indirectly. By using variables or other mutable objects, you can modify the values stored in literals. For example, if you have a variable ‘x’ assigned to a string literal, you can modify the contents of ‘x’ without altering the original literal itself.
Q: Which programming languages exhibit the “Cannot assign to literal” error?
A: The “Cannot assign to literal” error is commonly found in many programming languages, including Python, JavaScript, and Ruby. However, the specific error message may vary depending on the language.
Q: Why are literals immutable?
A: The immutability of literals arises from the need for predictability and consistency in programming. By ensuring that literals are unchangeable, it becomes easier to reason about the behavior of the code and prevent unintended side effects.
Q: Are there any exceptions to the immutability of literals?
A: While literals are generally immutable, some programming languages provide certain mechanisms to allow for limited modifications. For example, Python allows specific types of literals, such as lists and dictionaries, to be modified.
In conclusion, the “Cannot assign to literal” error is a result of attempting to modify an immutable object directly. By understanding the concept of literals and following the provided solutions, you can effectively resolve this error in your code and ensure smooth execution. Remember to use variables instead of literals when assigning new values and review your code logic for any instances of direct assignments to literals.
Images related to the topic cannot assign to function call
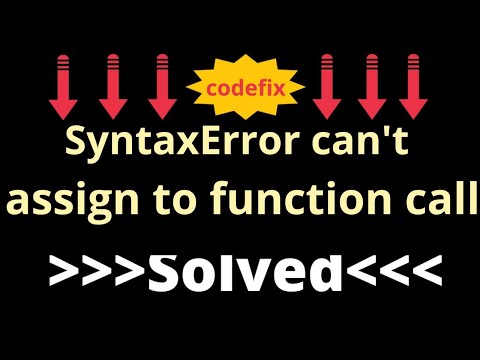
Found 8 images related to cannot assign to function call theme


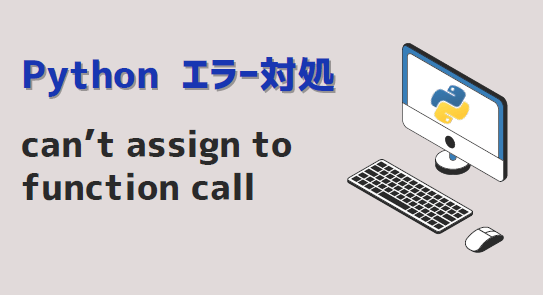
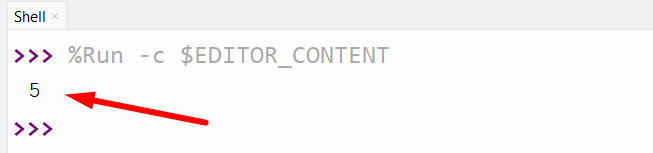


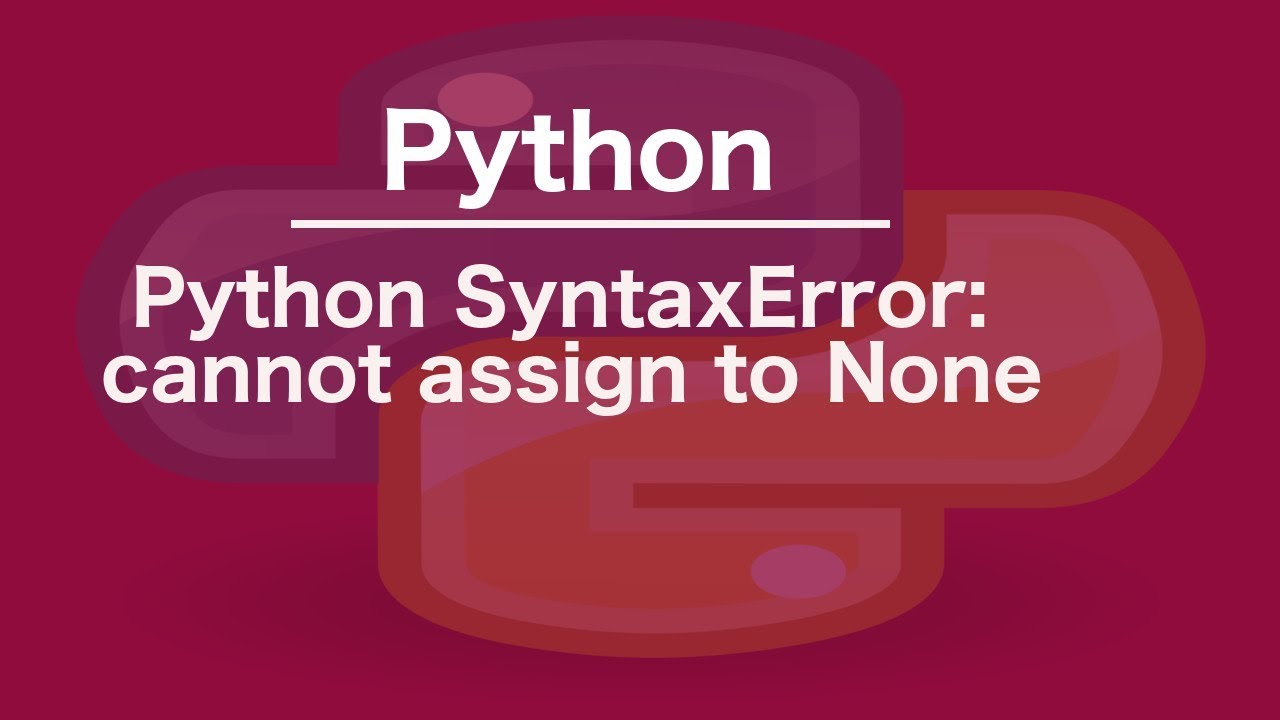
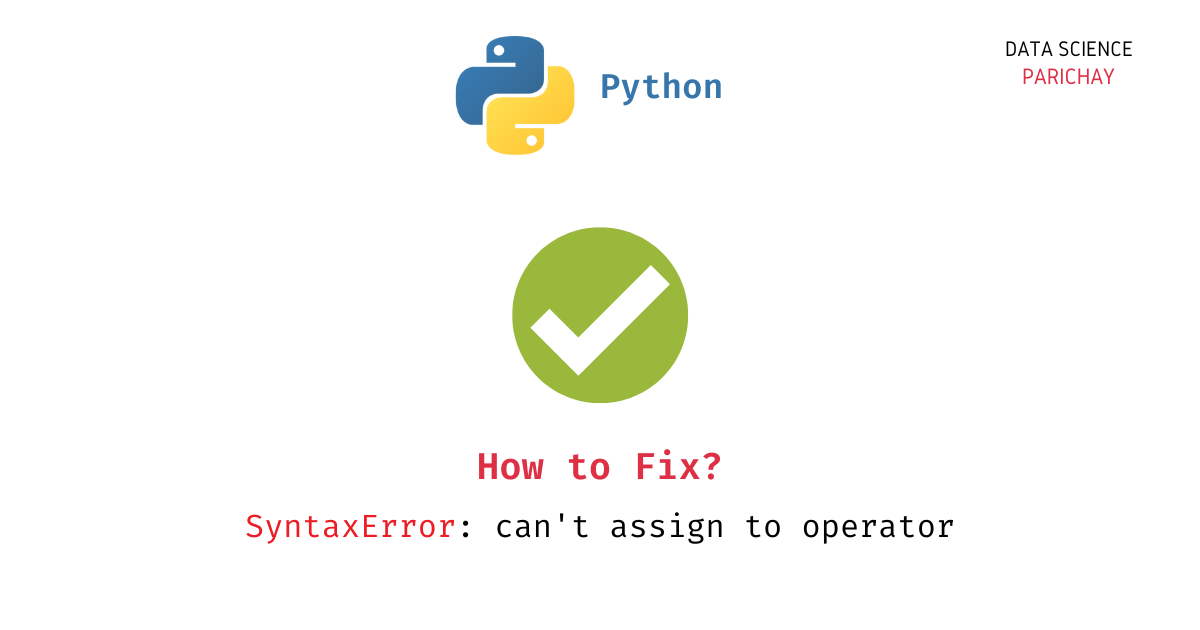
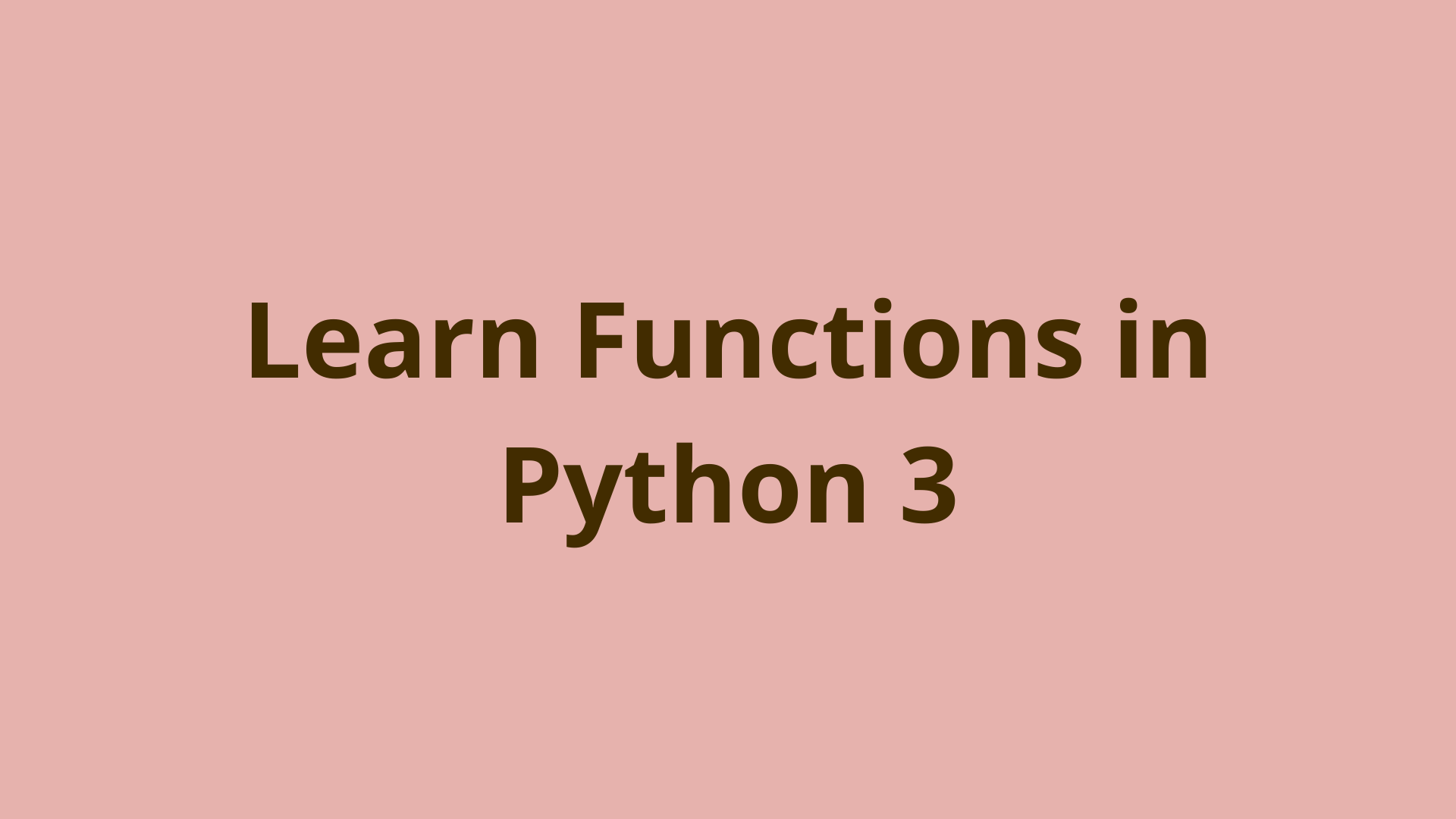
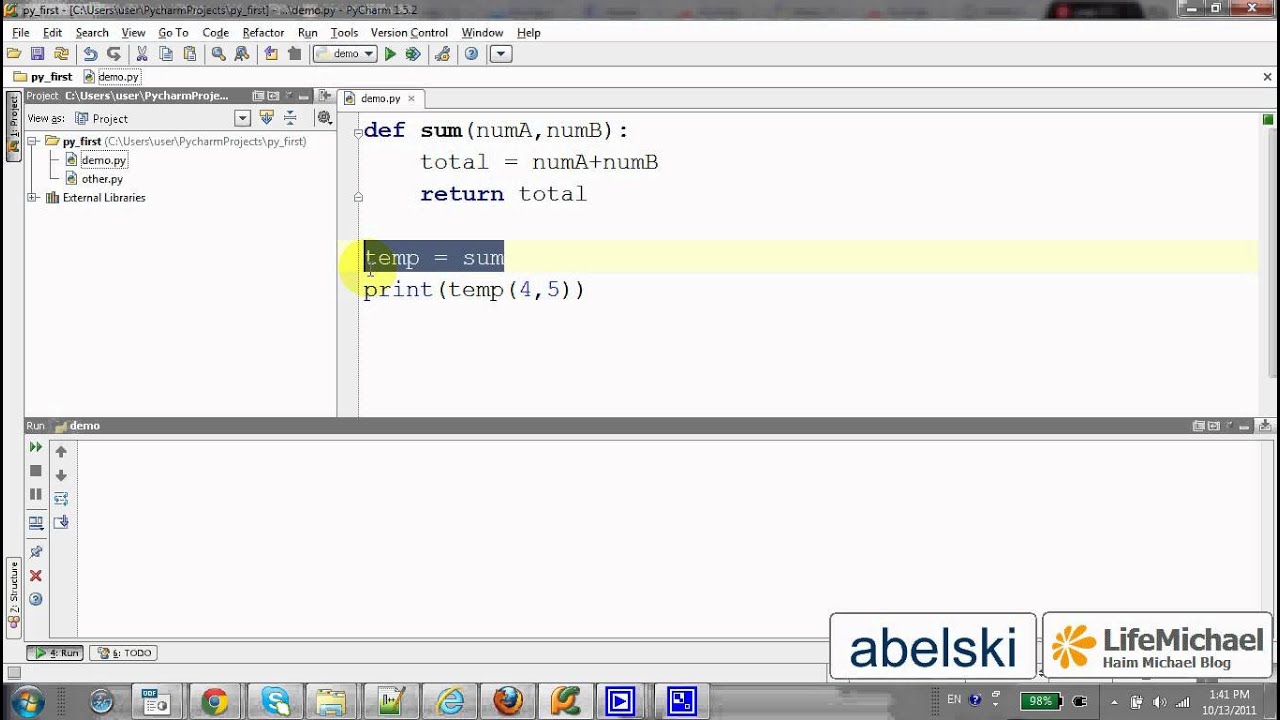

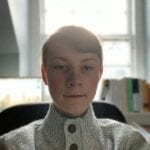
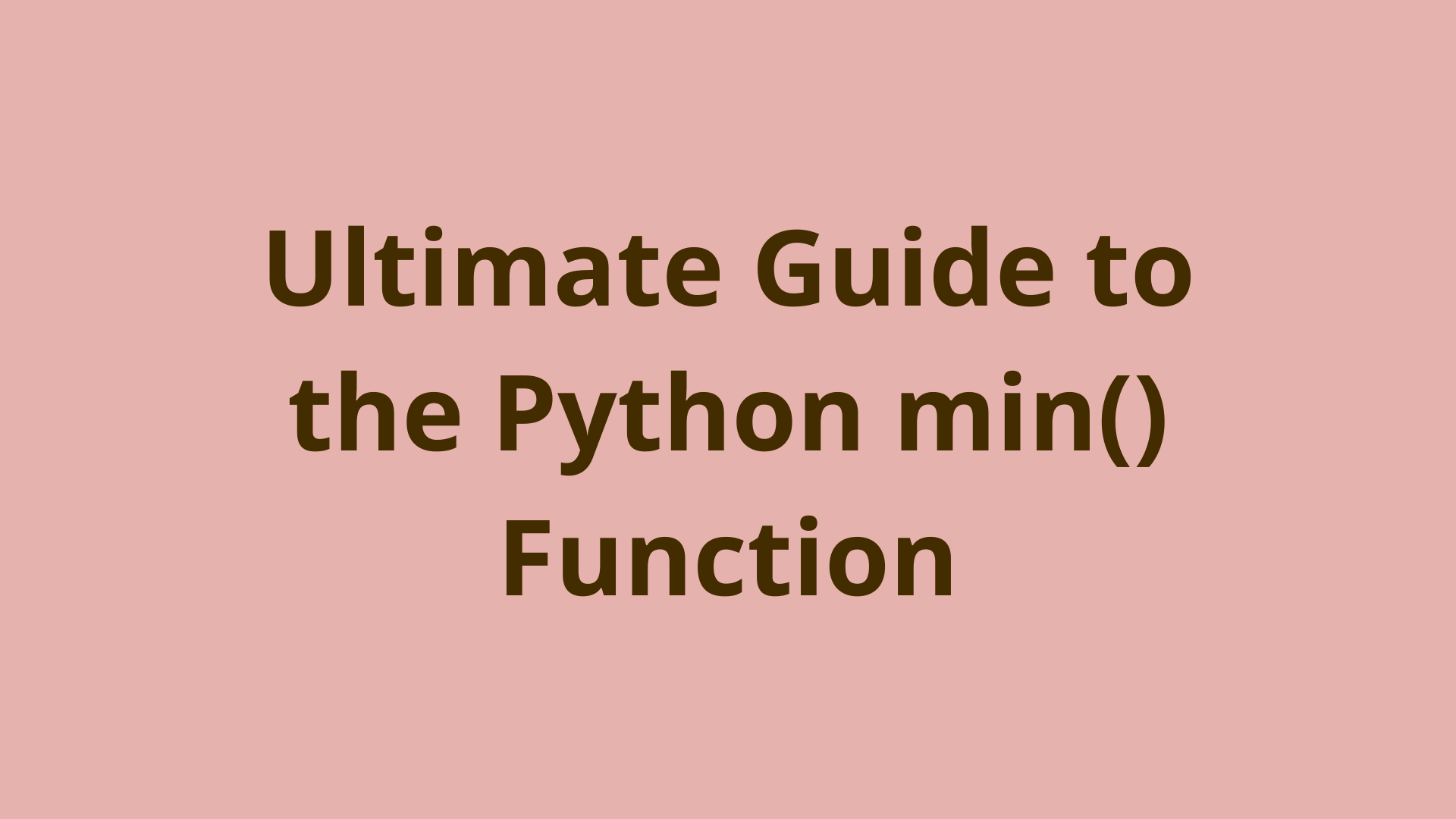
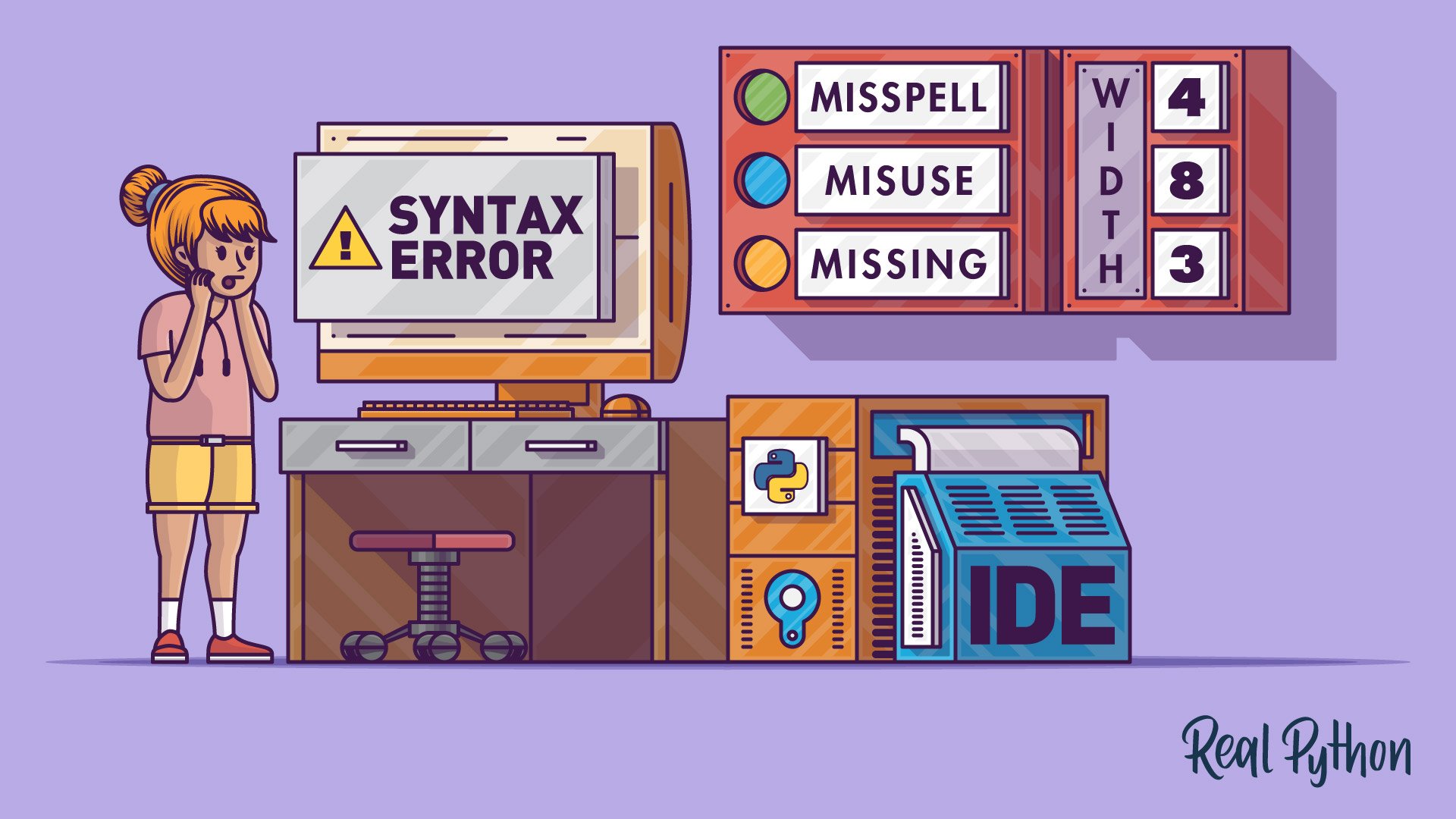
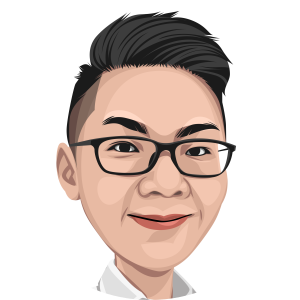
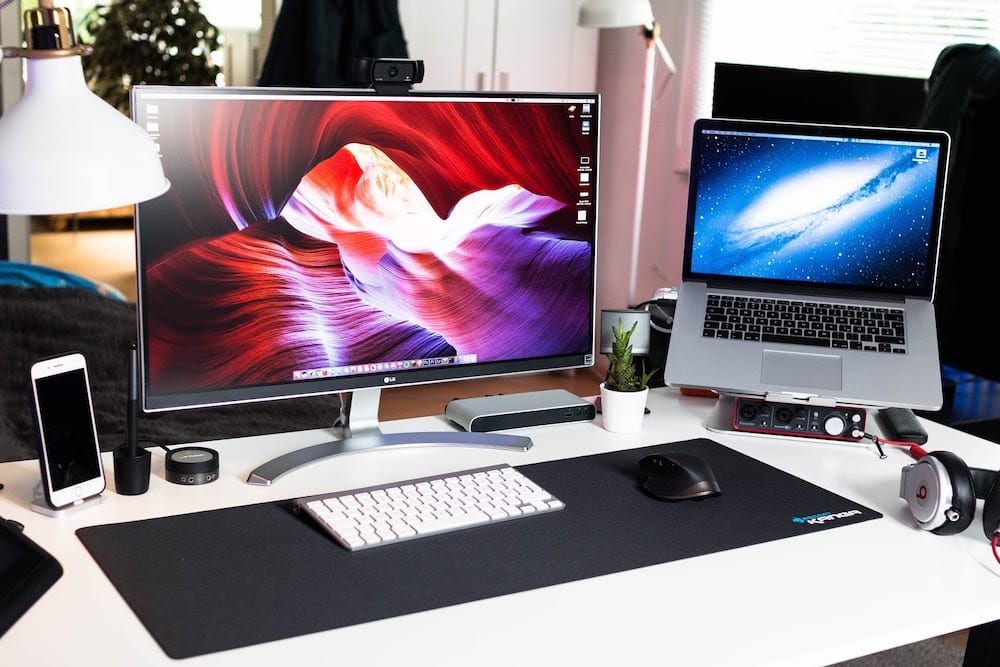
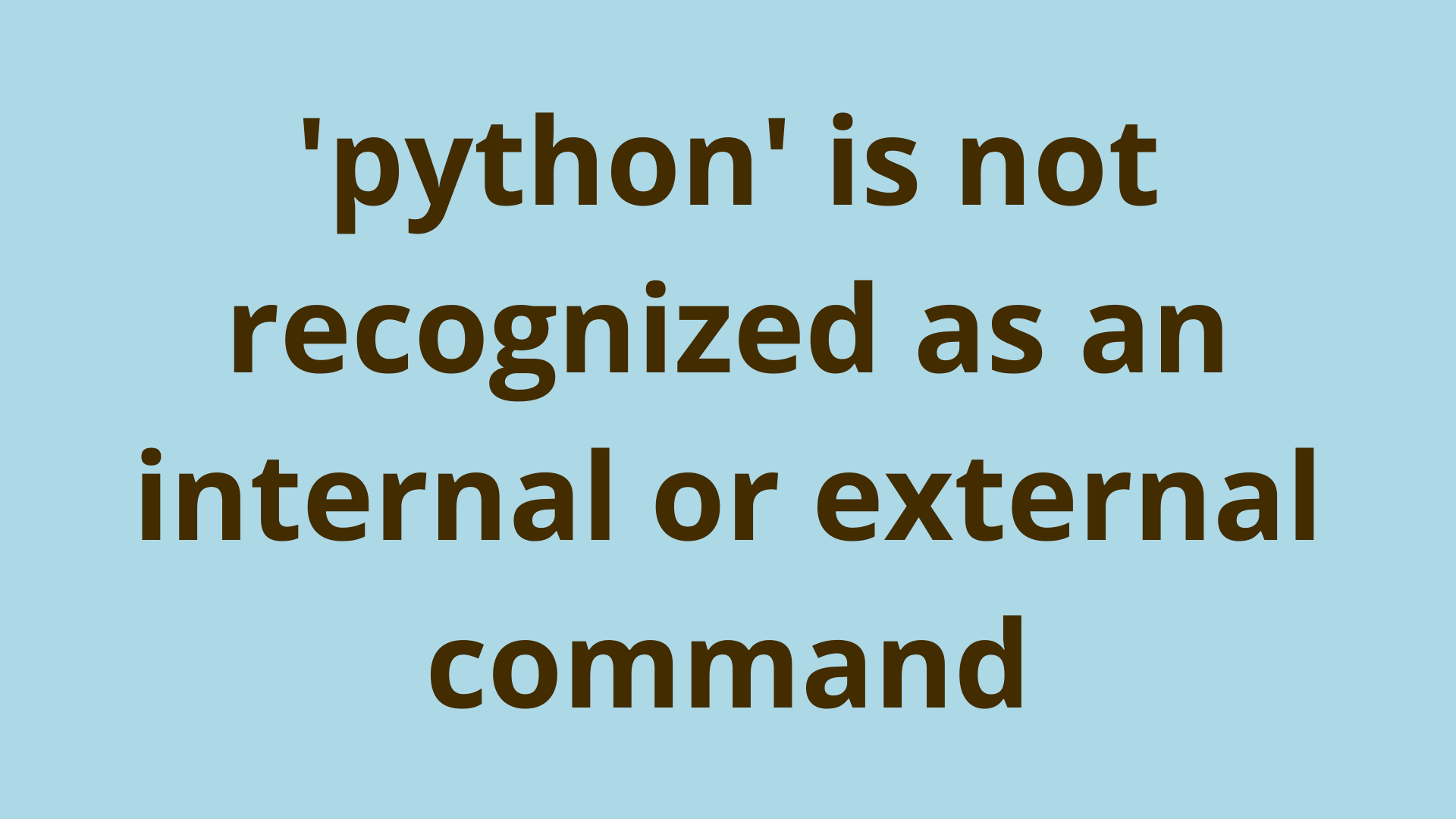



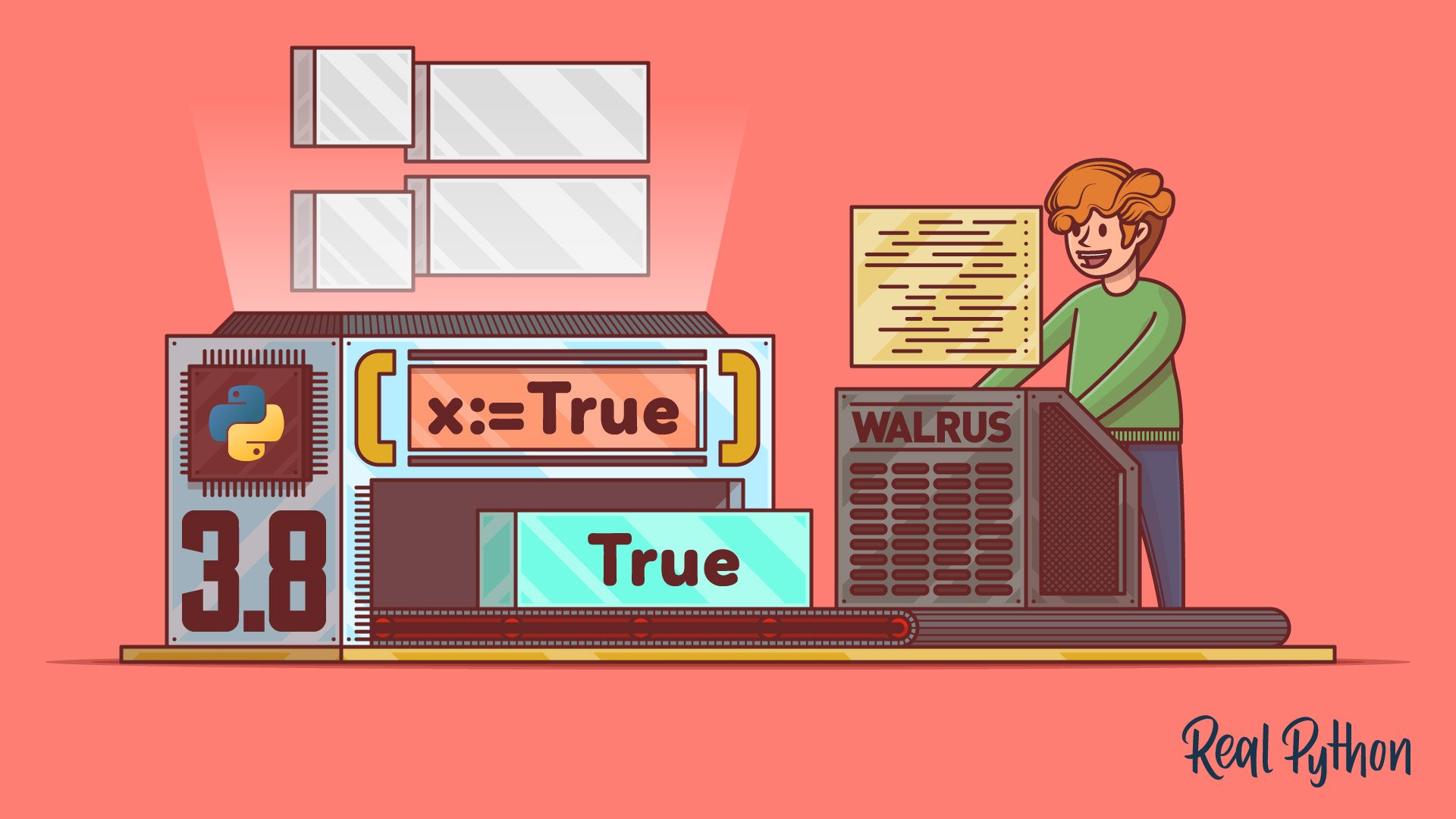
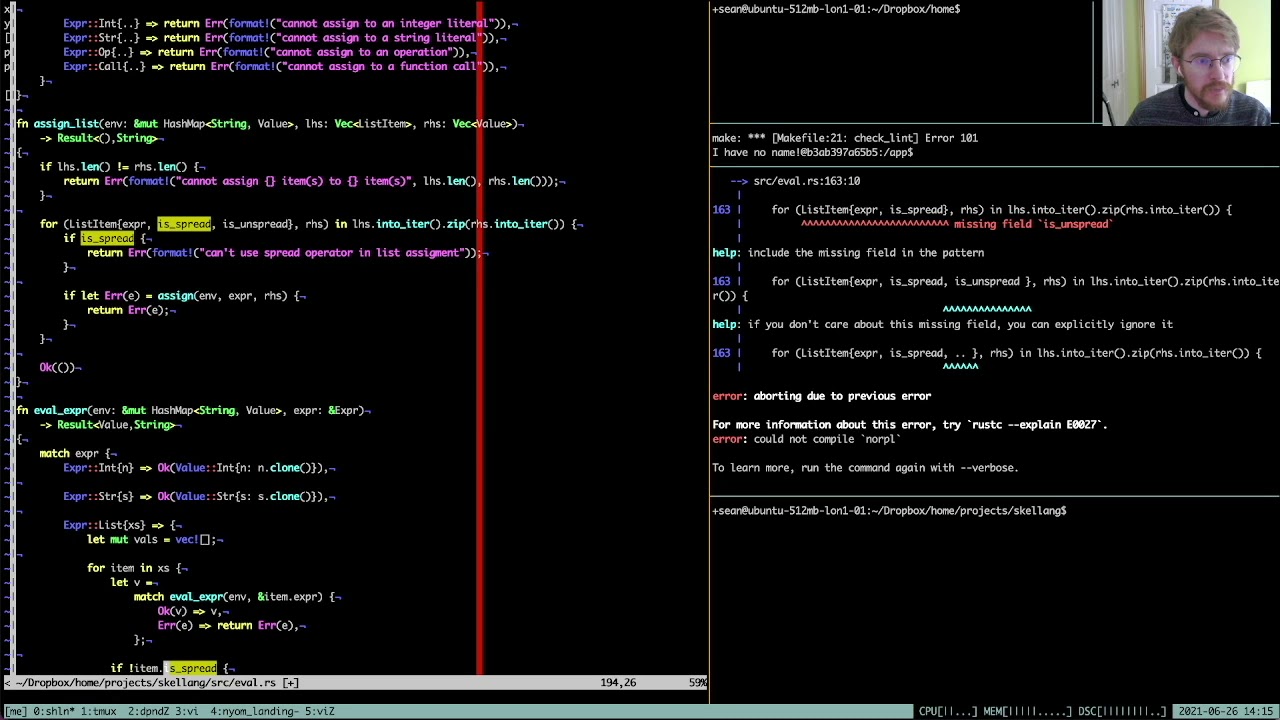

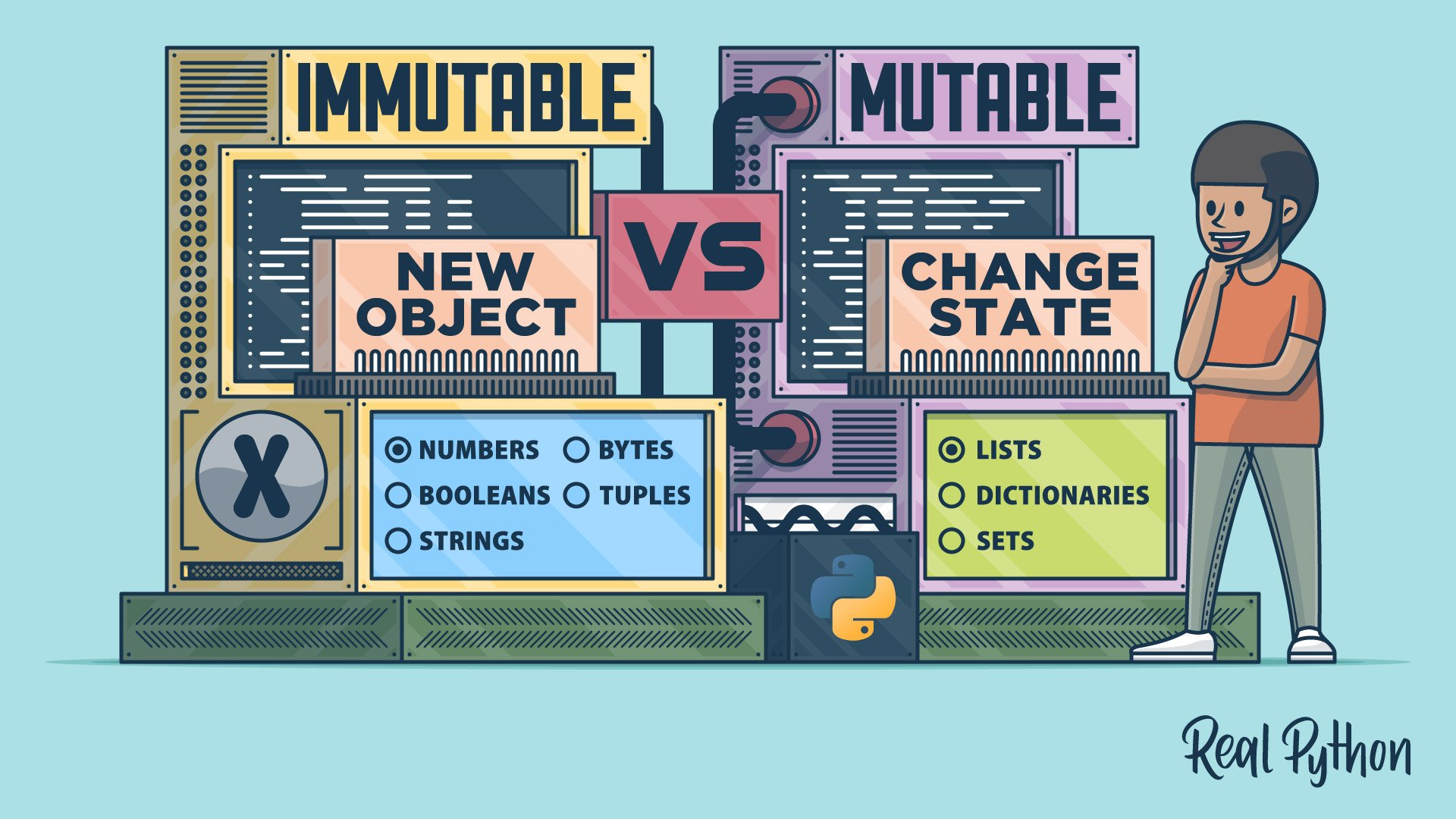
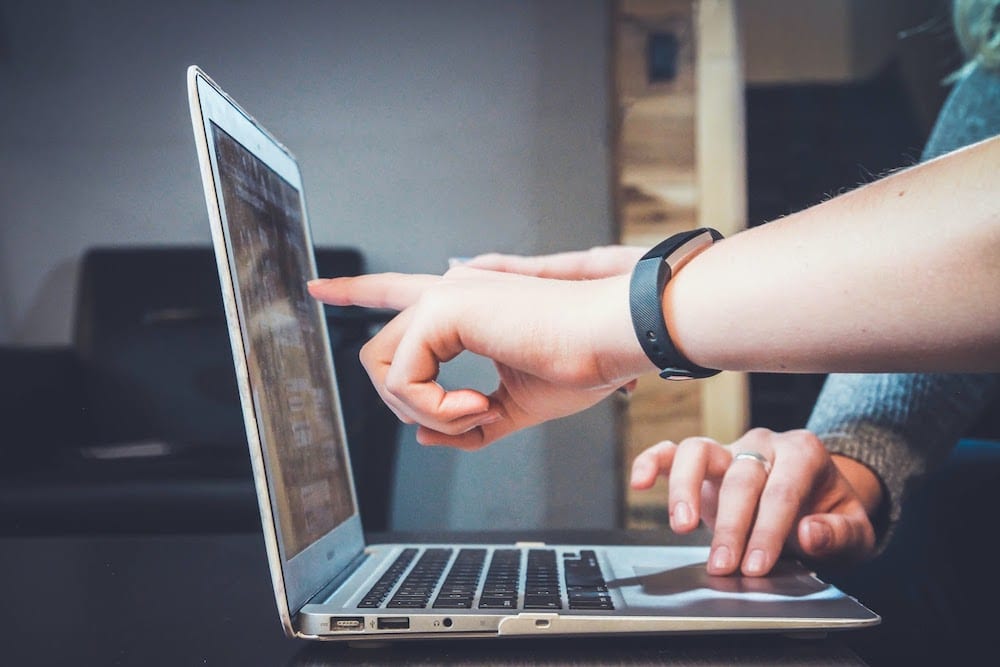


![Syntaxerror: cannot assign to function call [SOLVED] Syntaxerror: Cannot Assign To Function Call [Solved]](https://itsourcecode.com/wp-content/uploads/2021/01/IT-SOURCECODE_ICON-07.jpg)
Article link: cannot assign to function call.
Learn more about the topic cannot assign to function call.
- SyntaxError: cannot assign to function call here in Python
- Python SyntaxError: can’t assign to function call Solution
- SyntaxError: “can’t assign to function call” – Stack Overflow
- Python SyntaxError: can’t assign to function call Solution
- Python SyntaxError: cannot assign to operator Solution – Career Karma
- How to Fix Invalid SyntaxError in Python – Rollbar
- Python Programming/Functions – Wikibooks, open books for an open …
- How to Solve Python SyntaxError Can’t Assign to Function Call
- Python SyntaxError: can’t assign to function call – Initial Commit
- How to fix Python SyntaxError: cannot assign to function call
- SyntaxError: cannot assign to function call here in Python …
- Syntaxerror: cannot assign to function call – Itsourcecode.com
- Lưu trữ Top 22 Syntaxerror: Cannot Assign To Function Call
- 5/9 “can’t assign to function call” – Codecademy
See more: https://nhanvietluanvan.com/luat-hoc