Cannot Access Offset Of Type String On String
The “Cannot access offset of type string on string” error is a common issue that occurs when trying to access or manipulate an array element within a string. It indicates that you are trying to treat a string variable as an array and access an offset, which is not allowed. This error typically occurs in PHP, but it can also occur in other programming languages.
Explanation of the “Cannot access offset of type string on string” Error
In PHP, arrays are mutable and can store multiple values. Each value in an array has a unique index called an offset, which is used to access and manipulate individual elements. However, strings are immutable and can only store a sequence of characters.
When you try to access an offset on a string variable, PHP interprets it as an attempt to treat the string as an array and access a specific character at that offset. However, since strings are not arrays, this operation is not valid and results in the “Cannot access offset of type string on string” error message.
Potential Causes of the Error
1. Mismatched Variable Types: One common cause of this error is assigning a string value to a variable that is expected to be an array. Make sure that you are assigning and using the correct variable types throughout your code.
2. Incorrect Array Manipulation: If you assume a variable is an array and try to modify or access its elements, but it is actually a string, this error can occur. Check your code to ensure you are only performing array operations on valid array variables.
3. Invalid Key Access: Another potential cause is attempting to access or manipulate a specific array key that does not exist. Make sure you are using valid and existing array keys to avoid this error.
Troubleshooting Steps to Resolve the Error
1. Check Variable Assignments: Review your code and verify that you are assigning the correct variable types. Ensure that all array variables are initialized properly and that string variables are not being used as arrays.
2. Validate Array Usage: Double-check your code to ensure that you are only performing array operations on valid array variables. If necessary, add conditionals or checks to ensure that a variable is indeed an array before manipulating it.
3. Verify Array Keys: If you are accessing specific array keys, ensure that they exist in the array and are spelled correctly. Use PHP functions like `array_key_exists()` or `isset()` to validate the existence of the key before accessing it.
Example Solutions for the Error
1. Correct Variable Assignment:
“`
// Incorrect: Assigning a string value to an array variable
$myArray = “Hello World”;
// Correct: Assigning an array value to an array variable
$myArray = [1, 2, 3];
“`
2. Validate Array Usage:
“`
// Incorrect: Performing array operation on a non-array variable
$myString = “Hello World”;
echo $myString[0]; // Results in error
// Correct: Checking variable type before array operation
if (is_array($myArray)) {
echo $myArray[0]; // Accessing array elements if it is an array
}
“`
Best Practices to Avoid the Error in the Future
1. Consistent Variable Usage: Ensure that you use consistent variable types throughout your code. Avoid assigning a string value to an array variable or vice versa.
2. Checking Variable Types: Before performing any array operations, verify that the variable is indeed an array. Use functions like `is_array()` or `gettype()` to check the variable type before manipulating it.
3. Validate Array Keys: Always verify that the array key you are trying to access or manipulate exists in the array. Utilize functions like `array_key_exists()` or `isset()` to check for key existence before operating on it.
FAQs
1. What does the error “Cannot access offset of type string on string” mean?
This error signifies an attempt to treat a string as an array and access an offset. Since strings are not arrays, this operation is not allowed in PHP.
2. How can I resolve the “Cannot access offset of type string on string” error?
You can resolve this error by checking and ensuring that you are using the correct variable types throughout your code, validating array usage, and verifying array keys before accessing or manipulating them.
3. Why am I getting the “Cannot access offset of type string on string 0 php 8” error?
This error typically occurs when you are trying to access the offset 0 on a string variable, but PHP expects it to be an array. Check your code to ensure that you are using the correct variable types and performing array operations appropriately.
4. How do I convert a string to an array in PHP?
To convert a string to an array in PHP, you can use the `str_split()` function. It splits a string into an array of characters. Example: `$myArray = str_split($myString);`
5. How do I convert an array to a string in PHP?
To convert an array to a string in PHP, you can use the `implode()` function. It joins the elements of an array into a single string using a specified delimiter. Example: `$myString = implode(“, “, $myArray);`
6. What does the “Attempt to read property name on string” error mean?
This error occurs when you try to access a property name on a string variable as if it were an object. It usually happens when you mistakenly assume a string to be an object with properties.
7. How can I add a key-value pair to an object in PHP?
In PHP, you can add a key-value pair to an object using the arrow operator (`->`) to access and assign properties. Example: `$myObject->key = “value”;`
Solve ! Php Fatal Error : Array And String Offset Access Syntax Easily !
What Is Illegal String Offset?
When working with PHP, you may come across the term “illegal string offset” at some point. This error message typically occurs when you try to access or modify a string using an invalid offset value. Understanding what an offset is and why it can be illegal is crucial to avoid this error and write efficient and error-free PHP code.
In PHP, an offset refers to the index or position of a character within a string. Each character in a string is assigned a numerical position starting from 0. For example, in the string “Hello”, the character ‘H’ is at offset 0, ‘e’ is at offset 1, ‘l’ is at offset 2, ‘l’ is at offset 3, and ‘o’ is at offset 4.
You can access a specific character in a string by using square brackets and specifying the offset. For instance, to retrieve the character ‘l’ from the string “Hello”, you would write $string[2]. Similarly, you can update a character at a specific position by assigning a new value to that offset, like $string[0] = ‘h’; which would change “Hello” to “hello”.
However, an illegal string offset occurs when you try to use an offset that does not exist within the string. This can happen in various scenarios. Let’s discuss some common causes of this error and how to resolve them.
1. Trying to Access an Offset Beyond the String Length:
The most common cause of illegal string offset errors is attempting to access an index that is greater than or equal to the length of the string. For instance, if you have a string with a length of 5 and you try to access offset 5 or any value higher than 4, you will encounter this error. Ensure that your offset values are within the valid range of the string’s length.
2. Using a Non-Numeric Offset:
PHP only allows numeric offsets within square brackets when working with strings. If you use a non-numeric offset, such as a string or boolean value, you will trigger an illegal string offset error. To avoid this, make sure to use only numeric values as offsets.
3. Attempting to Modify a String:
It is important to note that strings in PHP are immutable, meaning they cannot be modified directly. So, if you try to assign a new value to an offset in a string, you will receive an illegal string offset error. To modify a string, you can use built-in string functions or convert the string into an array, make modifications, and then convert it back into a string.
4. Complex Data Structures:
The illegal string offset error can also occur when trying to access or modify a specific character in multidimensional arrays or objects. In such cases, ensure that you are accessing the correct element and using the appropriate syntax for arrays or objects.
FAQs:
Q: How can I fix the “illegal string offset” error?
A: To fix this error, first confirm that your offset is within the valid range of the string’s length. Make sure you are using a numeric value as an offset. If you are attempting to modify a string, use built-in string functions or convert the string into an array before making any modifications. Lastly, double-check complex data structures such as multi-dimensional arrays or objects for correct syntax and accessing the correct element.
Q: Why can’t I modify a string directly?
A: Strings in PHP are immutable, meaning they cannot be modified directly. Once a string is created, its value cannot be changed. This is why attempting to assign a new value to an offset in a string results in an illegal string offset error. To modify a string, you can use various string manipulation functions provided by PHP or convert the string into an array, modify the array, and then convert it back into a string.
Q: What happens if I continue using illegal string offsets?
A: If you continue using illegal string offsets, PHP will throw a warning or error, depending on your error reporting settings. This can potentially lead to unexpected behavior, incorrect results, or even application crashes. It is crucial to resolve illegal string offset errors to ensure the stability and correctness of your PHP code.
Q: Can functions cause “illegal string offset” errors?
A: Yes, functions can also cause these errors. If a function returns a string and you try to access an illegal string offset within that returned value, you will encounter an illegal string offset error. Check the returned value of the function and ensure that any string offsets you are trying to access are valid.
In conclusion, understanding the concept of illegal string offsets is essential for coding in PHP. By being aware of the causes and resolutions of this error, you can avoid it and write efficient, error-free PHP code. Remember to validate your offsets, use numeric values, and be cautious when working with complex data structures.
What Is Undefined Offset 2 In C?
In the world of programming, errors are an inevitable part of the development process. One such error that programmers often come across when working with C is the “undefined offset 2” error. This error typically occurs when accessing an array at an index that does not exist.
To understand this error further, let’s consider the basics of arrays in C. An array is a collection of elements of the same data type, arranged in a contiguous memory space. Each element in an array is accessed using its index, which starts at 0 and ends at the array size minus 1.
Now, let’s delve into the specifics of the “undefined offset 2” error. When this error is encountered, it means that the program is trying to access the element at index 2 of an array, but either the array itself is not allocated properly, or its size is smaller than the index being accessed. This results in undefined behavior, as the program tries to access memory that it has not reserved for the array.
For example, consider the following code snippet:
“`c
int arr[2] = {1, 2};
int value = arr[2];
“`
In this code, we have declared an array `arr` of size 2, and initialized it with two elements. However, when we try to access `arr[2]`, we encounter the “undefined offset 2” error. This is because `arr` only has indexes 0 and 1, so attempting to access index 2 leads to undefined behavior.
Undefined behavior can manifest itself in different ways, depending on the compiler and the system. Some common outcomes include program crashes, incorrect results, or unexpected behavior that is difficult to debug.
To avoid the “undefined offset 2” error and other similar errors, it is crucial to ensure that array indexes are within the defined bounds. This can be achieved by checking the array’s size and the index being accessed before attempting the access operation. In the above example, we could have avoided the error by either declaring `arr` with a size of 3 or by accessing only the elements within bounds (i.e., indexes 0 and 1).
FAQs about “undefined offset 2” in C:
Q: Is “undefined offset 2” error specific to index 2?
A: No, the error message “undefined offset 2” is just an indication of the specific array index causing the error. It can also occur with other index values, depending on the code and the size of the array.
Q: Why does the “undefined offset 2” error occur?
A: This error occurs when you try to access an array element at an index that is outside the array bounds, causing the program to access memory it has not reserved for the array.
Q: How can I fix the “undefined offset 2” error?
A: To fix this error, you need to ensure that the array index being accessed is within the bounds defined by the array’s size. You can do this by checking the array size before accessing its elements or by dynamically allocating memory to accommodate the required size.
Q: Can the “undefined offset 2” error occur with other programming languages?
A: Yes, similar errors can occur in other programming languages where arrays are used. However, the specific error message may differ depending on the programming language.
Q: How can I prevent “undefined offset 2” errors in my code?
A: To prevent such errors, make sure to verify the array’s size before accessing its elements. Instead of hardcoding the array size, consider using predefined constants or variables to ensure consistency.
Q: Are there any tools available to detect or prevent “undefined offset 2” errors?
A: Yes, various static code analysis tools can help detect potential array index issues before runtime. Additionally, some compilers and integrated development environments (IDEs) provide warnings or suggestions for array bounds checking.
In conclusion, the “undefined offset 2” error in C occurs when trying to access an array at an index that is beyond the array’s bounds. It is crucial to always be mindful of the array’s size and the indexes being accessed to prevent such errors. By ensuring proper array bounds checking, you can enhance the reliability and stability of your C programs.
Keywords searched by users: cannot access offset of type string on string Cannot access offset of type string on string, Cannot access offset of type string on string 0 php 8, Cannot use string offset as an array, Illegal string offset, Convert string to array PHP, Array to string conversion, Attempt to read property name on string, Add key value to object PHP
Categories: Top 89 Cannot Access Offset Of Type String On String
See more here: nhanvietluanvan.com
Cannot Access Offset Of Type String On String
If you’re a programmer or someone who deals with code, you might have come across the error message “Cannot access offset of type string on string.” This error can be frustrating, especially if you’re not sure what it means or how to fix it. In this article, we will delve into the reasons behind this error message, its possible causes, and offer solutions to troubleshoot it effectively.
Understanding the Error:
The error message itself provides some clues as to what might be causing the issue. The term “offset” generally refers to the position or a specific index within a string or an array. Here, the error indicates that the offset you are trying to access is of type string, which is an invalid operation. In simpler terms, you are attempting to treat a string as an array or use array indexing on a string, which is not allowed.
Common Causes:
1. Incorrect Syntax:
The most common reason for encountering this error is using incorrect syntax while trying to access the offset or index of a string. Double-check your code to ensure that you are using the correct syntax for accessing array elements rather than treating the string as an array itself.
2. Mismatched Data Types:
Another possibility is that a value you are trying to access from a string is not of the expected data type. Ensure that you are using appropriate type conversion and that the offset or index you are accessing corresponds to a valid index within the string.
3. Incompatible PHP Version:
It is worth noting that this error can occur due to version compatibility issues with PHP. Older versions of PHP might have different rules regarding string manipulation and array access. Make sure you are using a PHP version that supports the operations you are attempting.
Troubleshooting and Solutions:
Now that we have explored the potential causes of the “Cannot access offset of type string on string” error, let’s look at some troubleshooting steps and possible solutions:
1. Review the Code:
Carefully review the line of code where the error is occurring. Check for any typos or mistakes, such as missing or misplaced brackets, parentheses, or other syntax elements.
2. Verify Data Types:
Ensure that the variable you are trying to access from the string is of the correct data type. If necessary, perform type conversion to match the expected data type.
3. Check Array Access:
Make sure you are accessing arrays correctly and not treating a string as an array itself. If you need to access specific elements within the string, consider using a loop to iterate over the string’s characters instead.
4. Upgrade PHP Version:
If you suspect compatibility issues, ensure that you are using a PHP version that supports the operations you are attempting. Consider updating to a newer version to take advantage of the latest features and bug fixes.
FAQs:
Q1. Can this error occur in languages other than PHP?
A1. The specific error message mentioned in this article is related to PHP. However, similar concepts of accessing offsets of a certain type can be relevant to other languages as well.
Q2. What are some other related error messages?
A2. Some related error messages you might encounter include “Uncaught Error: Cannot use string offset as an array” or “Illegal offset type.”
Q3. Is this error specific to strings, or can it occur with other data types?
A3. While the error message in question is specific to string offsets, similar issues can occur with other data types, such as arrays or objects.
Q4. How can I prevent encountering this error in the first place?
A4. To avoid this error, always ensure that you are using proper syntax and understanding the data types involved. Regularly testing and debugging your code can help identify and fix any potential issues before they become problematic.
Q5. Are there any debugging tools or techniques that can help identify this error?
A5. Yes, utilizing debugging tools like step-by-step execution or print statements can help pinpoint the exact line of code where the error occurs. Additionally, reviewing error logs can provide valuable insights.
In conclusion, encountering the “Cannot access offset of type string on string” error can be confusing, but understanding its causes and applying appropriate solutions can resolve the issue. Remember to review your code, verify data types, and consider PHP version compatibility if necessary. By following these steps, you can effectively troubleshoot and fix this error, allowing your code to function as intended.
Cannot Access Offset Of Type String On String 0 Php 8
PHP is a widely used programming language for web development. It allows developers to create dynamic web pages and applications easily. However, like any other programming language, it has its own share of errors and issues. One of these errors that developers may encounter is the “Cannot access offset of type string on string 0” error, particularly in PHP 8. In this article, we will delve into the causes of this error, how to troubleshoot it, and answer some frequently asked questions.
What does the error message mean?
The error message “Cannot access offset of type string on string 0” indicates that an attempt to access an array element like a string offset has failed because the variable being accessed is not an array. In PHP, you can typically access elements within an array using square brackets and the index of the desired element. However, this error occurs when you try to access an element using a string index instead of an array index.
Causes of the error:
1. Empty variable: One common cause of this error is when the variable being accessed is empty or undefined. For example:
“`php
$myArray = “”;
echo $myArray[0]; // This will cause the error
“`
To avoid this error, you need to ensure that the variable is initialized properly and contains the appropriate value as an array.
2. Incorrect variable type: Another cause of this error is when a variable is incorrectly assigned or converted to a string rather than an array. For instance:
“`php
$myArray = “hello”;
echo $myArray[0]; // This will cause the error
“`
This occurs because the variable `$myArray` is treated as a string and not an array. Make sure you assign the variable correctly as an array and not as a string.
3. Out-of-bounds index: The error can also occur if you try to access an array element with an index that exceeds its length. For example:
“`php
$myArray = [1, 2, 3];
echo $myArray[5]; // This will cause the error
“`
Here, the index 5 does not exist in the array, and hence it throws the error. You should always make sure that the index you are accessing is within the range of the array length.
Troubleshooting the error:
1. Check variable initialization: Ensure that the variable you are trying to access as an array is properly initialized and has the correct value assigned. If the variable is empty or undefined, initialize it as an empty array before accessing its elements.
2. Verify variable type: Double-check the type of the variable you are trying to access. Make sure it is indeed an array and not a string or any other data type.
3. Debugging and error reporting: Enable error reporting in your PHP settings or include appropriate error handling mechanisms to easily identify the line of code causing the error. This will help you locate the exact problem area and resolve it accordingly.
4. Bounds checking: If you are trying to access an array element using an index, ensure that the index is within the bounds of the array. Remember that array indices start from 0, so an array with a length of 3 will have valid indices 0, 1, and 2.
FAQs:
Q: Can I access string characters using an index like an array in PHP?
A: Yes, you can access a string’s characters using an index like an array in PHP. However, ensure that you are treating the variable as a string and not as an array. If you want to access individual characters in a string, you can use the `substr` function.
Q: How can I fix the “Cannot access offset of type string on string 0” error?
A: To fix this error, you need to identify the cause. Check if the variable you are trying to access is properly initialized as an array and contains the expected values. Also, make sure that you are using the correct indices while accessing array elements.
Q: Does the PHP version affect the occurrence of this error?
A: Yes, this error message is specifically associated with PHP 8. In older versions of PHP, a similar error may have a different message. However, the underlying causes and troubleshooting steps remain the same.
Q: Are there any performance implications of this error?
A: The “Cannot access offset of type string on string 0” error does not directly affect the performance of your PHP application or script. It is primarily a syntax or logic error that needs to be resolved for the code to function correctly.
In conclusion, understanding the “Cannot access offset of type string on string 0” error is crucial for PHP developers. By recognizing the causes, troubleshooting methods, and applying the appropriate fixes, you can effectively address this issue and ensure your code runs smoothly without any errors. Remember to validate variable types, initialize variables correctly, and ensure that indices fall within the range of array lengths to prevent encountering this error in your PHP 8 projects.
Cannot Use String Offset As An Array
The root cause of the “Cannot use string offset as an array” error is an attempt to access a character within a string as if it were an array. In PHP, strings are considered a sequence of characters (or an array of characters), but they are treated as immutable, meaning they cannot be modified like arrays can. Therefore, trying to use an array syntax on a string will trigger the error.
One common scenario where this error occurs is when data is fetched from an API or database and stored in a string variable. If the fetched data contains an array within the string, developers might mistakenly try to access this internal array using array syntax. This causes the PHP interpreter to throw the “Cannot use string offset as an array” error, as it recognizes the string variable but cannot treat it as an array.
To better illustrate this, consider the following code snippet:
“`
$response = ‘{“status”: “success”, “data”: [1, 2, 3]}’;
$result = json_decode($response);
// Trying to access the “data” array within the string
$data = $result[‘data’];
“`
In the above example, `$response` contains a JSON-encoded string representing an object with a “data” property, which is an array. After decoding the JSON string using `json_decode()`, `$result` will be an object – not an array. So, attempting to access `$result[‘data’]` using array syntax results in the “Cannot use string offset as an array” error.
To resolve this error, we need to understand the data structure returned by `json_decode()`. By default, `json_decode()` returns an object rather than an associative array. So, to access the “data” property correctly, we need to change the code as follows:
“`
$data = $result->data;
“`
By using object notation (`->`), we can access the “data” property as intended.
FAQs:
Q: Why can’t I treat a string as an array in PHP?
A: In PHP, strings are considered a sequence of characters, but they are treated as immutable. It means that strings cannot be modified using array syntax or treated like mutable arrays.
Q: How can I avoid the “Cannot use string offset as an array” error?
A: To avoid this error, ensure that you understand the data structure returned by the functions you’re using. If a function returns a string, treat it as a string and avoid using array syntax on it. If the data contains internal arrays, access them using object notation or appropriate string manipulation functions.
Q: Can a string ever be treated as an array in PHP?
A: Yes, PHP provides functions like `str_split()` that can convert a string into an array, where each element represents a character of the string. This allows you to access individual characters using array syntax. However, remember that the string itself remains immutable.
Q: Are there any other scenarios where this error can occur?
A: While accessing arrays inside strings is a common cause, this error can also occur in other situations. For example, if you mistakenly use a non-numeric string as an index when accessing an array, PHP will throw the “Cannot use string offset as an array” error.
Q: Can this error be suppressed or handled gracefully?
A: You can handle this error gracefully using error handling mechanisms in PHP, such as `try-catch` blocks. By catching the error, you can display custom error messages or perform alternative actions instead of crashing the application.
In conclusion, the “Cannot use string offset as an array” error in PHP occurs when a string is inappropriately treated as an array. Understanding the data structures returned by functions and using appropriate syntax is crucial to avoid this error. By following the recommended practices and being mindful of the nature of strings in PHP, you can prevent this error and ensure the smooth execution of your code.
Images related to the topic cannot access offset of type string on string
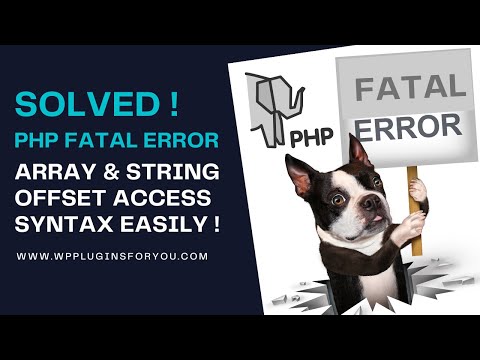
Found 24 images related to cannot access offset of type string on string theme

![php fatal: TypeError: Cannot access offset of type string [#3262205] | Drupal.org Php Fatal: Typeerror: Cannot Access Offset Of Type String [#3262205] | Drupal.Org](https://www.drupal.org/files/issues/2022-02-03/2022-02-03_16-06-36.png)

![php fatal: TypeError: Cannot access offset of type string [#3262205] | Drupal.org Php Fatal: Typeerror: Cannot Access Offset Of Type String [#3262205] | Drupal.Org](https://www.drupal.org/files/issues/2022-02-03/2022-02-03_16-06-59.png)

![php fatal: TypeError: Cannot access offset of type string [#3262205] | Drupal.org Php Fatal: Typeerror: Cannot Access Offset Of Type String [#3262205] | Drupal.Org](https://www.drupal.org/files/issues/2022-02-03/2022-02-03_16-06-10.png)
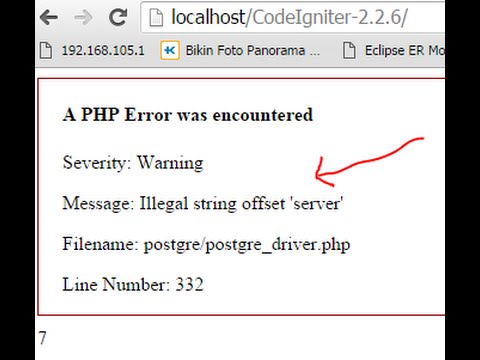

![TypeError: Cannot access offset of type string on string in Drupal\Component\Utility\NestedArray::setValue() [#3299926] | Drupal.org Typeerror: Cannot Access Offset Of Type String On String In Drupal\Component\Utility\Nestedarray::Setvalue() [#3299926] | Drupal.Org](https://www.drupal.org/files/issues/2022-07-26/screen_error.png)


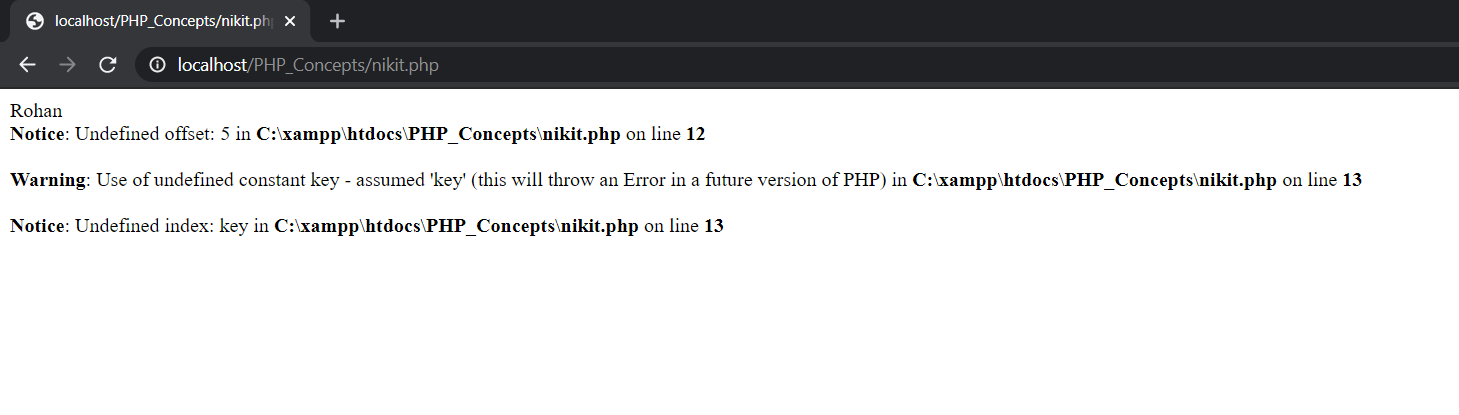


![TypeError: Cannot access offset of type string on string in ctools_content_select_context() [#3296163] | Drupal.org Typeerror: Cannot Access Offset Of Type String On String In Ctools_Content_Select_Context() [#3296163] | Drupal.Org](https://www.drupal.org/files/styles/drupalorg_user_picture/public/user-pictures/picture-3696724-1655188076.jpg?itok=e6f23pCu)
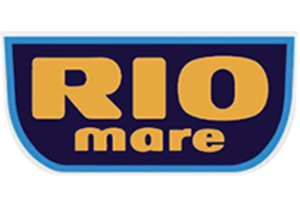
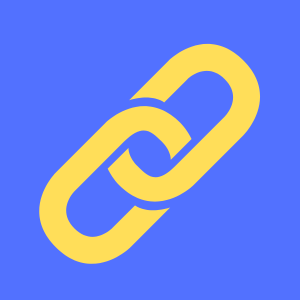
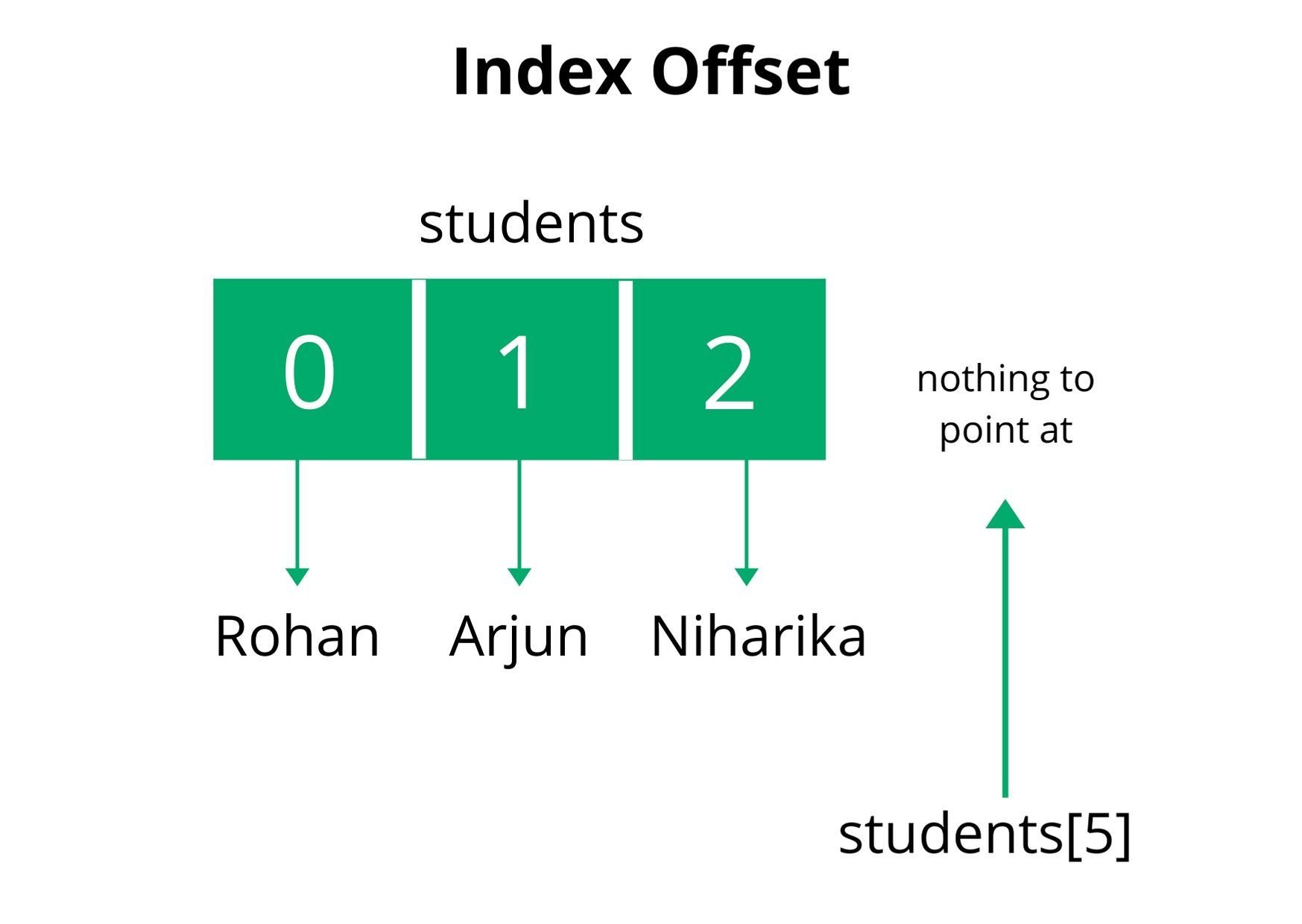

Article link: cannot access offset of type string on string.
Learn more about the topic cannot access offset of type string on string.
- PHP Fatal error: Uncaught TypeError: Cannot access offset of …
- TypeError Cannot access offset of type string on string (View
- Uncaught TypeError: Cannot access offset of type string on …
- How to fix Warning Illegal string offset in PHP – W3docs
- Notice: Undefined Offset : 2 How to Solve? – php – Stack Overflow
- I got an error (Cannot access offset of type string on string)
- Getting error: Cannot access offset of type string on … – Toolset
- Cannot access offset of type string on string – Joomla! Forum
- PHP Fatal error : Cannot access offset of type string on string …
- [theming] TypeError: Cannot access offset of type string on …
See more: nhanvietluanvan.com/luat-hoc