Can’T Compare Offset-Naive And Offset-Aware Datetimes
In the realm of datetime manipulation in Python, there are two distinct concepts: offset-naive and offset-aware datetimes. These two types of datetime objects serve different purposes and have different characteristics. This article will explore the differences between these datetimes, shed light on the limitations of comparing them, and provide guidance on how to handle them in Python.
Offset-Naive Datetimes
Let’s start by understanding offset-naive datetimes. In Python, an offset-naive datetime object doesn’t have any awareness of time zones or daylight saving time adjustments. It is simply a representation of a specific date and time, without considering any particular time zone context. This means that offset-naive datetimes cannot accurately represent time in different regions or handle conversions between time zones.
To create an offset-naive datetime object, you can use the `datetime` module in Python. For example, you can create a new datetime object representing January 1, 2022, at 12:00 PM by using the following code:
“`python
from datetime import datetime
dt = datetime(2022, 1, 1, 12, 0)
“`
Offset-Aware Datetimes
On the other hand, offset-aware datetimes do take into account time zones and daylight saving time adjustments. These datetime objects are aware of the specific timezone in which they exist and can accurately represent time in different regions. Offset-aware datetimes are crucial when dealing with international time conversions or when working with systems that have different time zones.
To work with offset-aware datetimes in Python, you can utilize the `pytz` library, which provides timezone definitions and conversion utilities. Here’s an example of creating an offset-aware datetime object for the same January 1, 2022, at 12:00 PM in the Eastern Standard Time (EST) zone:
“`python
from datetime import datetime
import pytz
est = pytz.timezone(‘US/Eastern’)
dt = est.localize(datetime(2022, 1, 1, 12, 0))
“`
Comparing Offset-Naive and Offset-Aware Datetimes
One crucial limitation is that comparing offset-naive and offset-aware datetimes directly can lead to unexpected results or even errors. This is due to the fundamental difference in the underlying concepts and the lack of synchronization between them.
For example, if you try to compare an offset-naive datetime with an offset-aware datetime using comparison operators like `==`, `<`, or `>` directly, you may encounter a `TypeError` stating that you cannot compare tz-naive and tz-aware datetime-like objects. Here’s an example:
“`python
from datetime import datetime
import pytz
est = pytz.timezone(‘US/Eastern’)
dt_naive = datetime(2022, 1, 1, 12, 0)
dt_aware = est.localize(datetime(2022, 1, 1, 12, 0))
print(dt_naive == dt_aware) # Raises TypeError
“`
To overcome this limitation, you need to ensure that you are comparing either two offset-naive datetimes or two offset-aware datetimes. This involves either converting the offset-aware datetime to an offset-naive one or adding timezone information to the offset-naive datetime. The approach you choose depends on the specific requirements of your application.
FAQs
Q: Can I add or subtract days from a datetime object?
A: Yes, you can manipulate datetime objects by adding or subtracting timedelta objects, which represent time durations. For example, to add one day to a datetime object, you can use the `timedelta` class from the `datetime` module: `new_dt = dt + timedelta(days=1)`.
Q: How can I change the timezone of a datetime object?
A: To change the timezone of a datetime object, you can use the `pytz.timezone()` function to obtain a timezone object for the desired timezone. Then, you can use the `astimezone()` method of the datetime object, passing the timezone object as an argument. Here’s an example: `new_dt = dt.astimezone(pytz.timezone(‘Europe/London’))`.
Q: How can I convert a string date to a datetime object?
A: You can convert a string representing a date to a datetime object using the `strptime()` method from the `datetime` module. This method allows you to specify a format string that matches the structure of your string date. For example, to convert the string ‘2022-01-01’ to a datetime object, you can use `datetime.strptime(‘2022-01-01’, ‘%Y-%m-%d’)`.
Q: Why am I getting an error when accessing the ‘year’ attribute of a datetime object?
A: If you encounter an error like “AttributeError: ‘datetime.date’ object has no attribute ‘year'”, it means that you are trying to access the `year` attribute of a `date` object instead of a `datetime` object. Make sure you are working with the correct type of datetime object or convert the `date` object to a `datetime` object before accessing its attributes.
In conclusion, comparing offset-naive and offset-aware datetimes directly is not supported in Python, as they represent different concepts. When working with these datetime objects, make sure to convert them to the same type before performing comparisons. Take advantage of Python’s datetime functionality and third-party libraries like `pytz` to effectively handle time zone conversions and manipulations.
Python : Django 1.4 – Can’T Compare Offset-Naive And Offset-Aware Datetimes
What Is Offset Naive And Offset Aware Datetimes?
When dealing with datetime values in computer programming, it’s essential to consider the concept of time zones and offsets. A datetime object represents a specific point in time, but without the necessary information about its relation to a time zone or offset, it would be difficult to accurately compare or perform calculations with these values. This is where the concepts of “offset naive” and “offset aware” datetimes come into play.
Offset Naive Datetimes:
An offset-naive datetime object is simply a datetime value that doesn’t have any knowledge of its relationship to a specific time zone or offset. It only contains information about the year, month, day, hour, minute, second, and microsecond. This means that without a time zone or offset, it’s challenging to compare or perform operations on these values accurately.
For instance, let’s consider a scenario where you want to compare two datetime values to determine which one occurred earlier. Without any timezone or offset information, it’s impossible to know if one datetime is in one time zone and the other in a different time zone, leading to inaccurate results.
Offset-naive datetimes are typically used when dealing with local time where the time zone or offset information is not significant. They often represent the local time in the system where the code is being executed, assuming that all datetime values are in the same time zone. However, when sharing or communicating datetime values across different systems or time zones, offset-naive datetimes can lead to ambiguity and confusion.
Offset Aware Datetimes:
Offset-aware datetime objects, on the other hand, are datetime values that are associated with a specific time zone or offset information. In addition to the year, month, day, hour, minute, second, and microsecond, they also include information about the time zone or offset. This provides the necessary context to compare, perform calculations, and standardize datetime values across different time zones accurately.
By having the offset information, you can determine the relative time difference between datetime values correctly. Offset-aware datetime objects allow you to convert a datetime value from one time zone to another, perform arithmetic calculations taking time zones into account, and accurately represent datetime values across different systems or regions.
The most common way to represent offset-aware datetime values is by using the datetime module in Python. This module offers the datetime class that can handle both offset-naive and offset-aware datetimes. When creating an offset-aware datetime object, you can pass the desired time zone or offset information explicitly, ensuring accurate representation and calculations.
It’s worth noting that offset-aware datetime objects are typically stored and manipulated in Coordinated Universal Time (UTC), which is a standardized time reference used worldwide. This allows for consistent time representation and conversion regardless of the specific time zone or offset.
Frequently Asked Questions (FAQs):
Q: Can I convert an offset-naive datetime to an offset-aware datetime?
A: Yes, it is possible to convert an offset-naive datetime to an offset-aware datetime by assigning a specific time zone or offset to the datetime object. However, it’s important to remember that offset-naive datetimes do not inherently contain any information regarding the time zone or offset, so this information must be provided or inferred from external sources.
Q: How do I perform arithmetic calculations with offset-aware datetimes?
A: When performing arithmetic calculations with offset-aware datetimes, it’s crucial to take into account the time zone or offset. The Python datetime module provides functions and methods to handle these calculations accurately. By using these functionalities, you can calculate time differences, add or subtract time intervals, and perform other operations while considering the specific time zone or offset.
Q: Are there any libraries or frameworks that simplify working with offset-aware datetimes?
A: Yes, there are several third-party libraries and frameworks available that can simplify working with offset-aware datetimes. One popular library is pytz, which provides an extensive database of time zone information and allows for easy conversion and manipulation of datetime objects across different time zones. Other libraries like arrow and pendulum also offer additional functionalities and simplifications for working with datetimes.
Q: Are offset-naive datetime values always incorrect or problematic?
A: Offset-naive datetime values are not inherently incorrect or problematic. They are mainly used to represent local time without considering the time zone or offset. In specific cases where datetime values are consistently within one time zone or when time zone information is irrelevant, offset-naive datetimes can be appropriate and more efficient. However, for scenarios involving different time zones, standardized calculations, or sharing datetime values across systems, it’s generally recommended to use offset-aware datetimes.
How To Compare 2 Datetime Objects In Python?
DateTime objects are incredibly useful when dealing with dates and times in Python. They allow you to store information about specific moments in time and perform various operations on them. One of the most common tasks is comparing DateTime objects to check if one is greater, smaller, or equal to another. In this article, we will discuss different methods to compare two DateTime objects in Python.
Method 1: Using Comparison Operators
Python provides several comparison operators, such as less than (<), greater than (>), equal to (==), etc., which can be used to compare DateTime objects. By applying these operators, we can easily determine the relationship between two DateTime objects.
Here’s an example that demonstrates the usage of comparison operators for DateTime objects:
“`python
from datetime import datetime
date1 = datetime(2021, 1, 1)
date2 = datetime(2021, 1, 2)
if date1 < date2:
print("date1 is less than date2")
elif date1 > date2:
print(“date1 is greater than date2”)
else:
print(“date1 and date2 are equal”)
“`
The output of this code will be “date1 is less than date2”, as date1 represents January 1, 2021, which is indeed smaller than date2 (January 2, 2021).
Method 2: Using the compare() Method
Python’s DateTime module provides a built-in compare() method that allows us to compare DateTime objects. This method returns an integer value (-1, 0, or 1) representing the relationship between the two objects.
Here’s an example:
“`python
from datetime import datetime
date1 = datetime(2021, 1, 1)
date2 = datetime(2021, 1, 2)
result = date1.compare(date2)
if result == -1:
print(“date1 is less than date2”)
elif result == 1:
print(“date1 is greater than date2”)
else:
print(“date1 and date2 are equal”)
“`
Again, the output will be “date1 is less than date2”. The compare() method provides a more explicit way to compare DateTime objects and allows for better code readability.
Method 3: Using the timedelta() Function
In some cases, you might need to compare DateTime objects by an interval rather than directly. Python’s DateTime module provides the timedelta() function for this purpose. The function allows you to specify the difference between two DateTime objects in terms of durations like days, hours, minutes, etc.
Let’s consider an example where we want to compare two DateTime objects by a difference of 24 hours:
“`python
from datetime import datetime, timedelta
date1 = datetime(2021, 1, 1)
date2 = datetime(2021, 1, 2)
# Calculate the difference between date1 and date2
difference = date2 – date1
if difference >= timedelta(days=1):
print(“date2 is at least 24 hours after date1”)
else:
print(“date2 is less than 24 hours after date1”)
“`
In this case, the output will be “date2 is at least 24 hours after date1”. This method enables you to compare DateTime objects with a specific time difference rather than directly comparing the objects.
FAQs Section:
Q1: Can I compare DateTime objects with different time zones?
Yes, you can compare DateTime objects with different time zones. Python’s DateTime module takes into account time zone information when comparing DateTime objects. However, you need to ensure that the time zone information is properly set for each DateTime object before making the comparison.
Q2: What happens if I compare DateTime objects with different accuracy (e.g., one has seconds and the other does not)?
When comparing DateTime objects, Python considers the accuracy specified in the objects. If one DateTime object has a higher precision (e.g., includes seconds or microseconds) and the other object does not, the comparison will still produce the correct result. The DateTime module internally handles the comparison by considering the available precision for each object.
Q3: Is it possible to compare DateTime objects within a specific range?
Yes, you can compare DateTime objects within a specific range by using the timedelta() function. By calculating the time difference between the DateTime objects, you can easily check if it falls within a desired range and perform the necessary actions accordingly.
Q4: Are DateTime objects in Python immutable?
Yes, DateTime objects are immutable in Python. Once created, their value cannot be changed. If you need to modify a DateTime object, you can create a new object with the desired changes. This immutability ensures that DateTime objects remain consistent throughout your code and prevents unintended modifications.
In conclusion, comparing DateTime objects in Python is a frequent task when working with dates and times. Whether by using comparison operators, the compare() method, or the timedelta() function, Python provides several ways to compare DateTime objects with ease and precision. By understanding these methods, you can effectively utilize DateTime objects in your Python programs.
Keywords searched by users: can’t compare offset-naive and offset-aware datetimes Cannot compare tz naive and tz aware datetime like objects, Naive and aware datetime, Timezone aware datetime python, Can t compare datetime datetime to datetime date, Python datetime add days, Change timezone datetime python, Python string date to datetime, Can only use dt accessor with datetimelike values
Categories: Top 95 Can’T Compare Offset-Naive And Offset-Aware Datetimes
See more here: nhanvietluanvan.com
Cannot Compare Tz Naive And Tz Aware Datetime Like Objects
When working with date and time in programming, it is crucial to understand the concept of time zones and how they affect datetime objects. In Python, datetime objects can be either time zone naive (tz naive) or time zone aware (tz aware), each with their own set of characteristics and limitations. One common mistake is attempting to compare tz naive and tz aware datetime objects which can lead to unexpected results. In this article, we will delve into the differences between these two types of datetime objects, why they cannot be directly compared, and how to handle them properly in your code.
Understanding Time Zones
Before diving into the specifics, let’s briefly touch upon the concept of time zones, as they play a crucial role in datetime objects. A time zone represents a region on Earth where the same standard time is observed. Time zones are determined by their offset from Coordinated Universal Time (UTC). Different time zones have different offsets, which accounts for variances in time between different regions.
Tz Naive Datetime Objects
Tz naive datetime objects store date and time information without any reference to a specific time zone. These objects are unaware of the offset from UTC and rely on the system’s default time zone. Tz naive datetime objects are created using the datetime module in Python without explicitly specifying a time zone.
Tz Aware Datetime Objects
In contrast to tz naive datetime objects, tz aware datetime objects are aware of the specific time zone they belong to. They store additional information about the offset from UTC and can perform various time zone conversions. Tz aware datetime objects are created using the datetime module along with a timezone info object, such as pytz or the built-in timezone module.
Why They Cannot Be Compared
When attempting to compare tz naive and tz aware datetime objects, Python raises a `TypeError` because these two types are not directly comparable. This error occurs because the two datetime objects represent time in fundamentally different ways. Tz naive objects lack the necessary information about the time zone, while tz aware objects consider time zone offset as a significant factor when comparing.
For example, let’s consider a tz naive datetime object representing the current local time. If we attempt to compare it to a tz aware datetime object representing the same local time but in a different time zone, the comparison would lead to inaccurate results. This is because the tz aware object accounts for the difference in time zones, while the tz naive object remains oblivious to this crucial detail.
Handling Tz Naive and Tz Aware Objects
To properly handle tz naive and tz aware datetime objects, it is important to make them consistently of the same type. The general guideline is to convert all datetime objects to tz aware before comparing or performing any operations involving time zones. Conversely, if time zone information is not necessary, converting tz aware objects to tz naive may be preferred.
To convert a tz naive datetime object to tz aware, various methods are available. One option is to utilize the pytz library, which provides a wide range of time zones. By using the `localize()` method, you can assign the desired time zone to your tz naive datetime object. Another option is to utilize the `timezone` module, which is part of the Python standard library, to create a tz aware object from a tz naive one.
Alternatively, when working with tz aware objects, the `normalize()` method can be utilized to adjust the objects to the specific time zone, if needed. This method ensures that the comparison and time manipulation functions work as expected, even when the time zone offset changes due to daylight saving time or other factors.
FAQs:
Q: Can I directly compare two tz aware datetime objects?
A: Yes, tz aware datetime objects can be directly compared since they contain explicit time zone information.
Q: What happens if I compare a tz naive and a tz aware datetime object?
A: Python throws a `TypeError` when attempting to compare a tz naive and a tz aware datetime object since they represent time in fundamentally different ways.
Q: How can I convert a tz naive datetime object to tz aware?
A: You can use libraries like pytz or the built-in timezone module to convert a tz naive datetime object to tz aware by assigning the desired time zone using the `localize()` or `timezone` methods.
Q: Are tz naive datetime objects aware of the system’s default time zone?
A: Yes, tz naive datetime objects rely on the system’s default time zone to interpret the date and time information.
Q: Can I convert a tz aware datetime object to tz naive?
A: Yes, if time zone information is not necessary, you can convert a tz aware datetime object to tz naive by using the `replace()` method and omitting the `tzinfo` argument.
In conclusion, understanding the differences between tz naive and tz aware datetime objects is crucial for accurate date and time operations. While these two types cannot be directly compared, converting them to the same type and utilizing appropriate methods from libraries like pytz or the timezone module can ensure accurate results in your programming endeavors.
Naive And Aware Datetime
When it comes to dealing with dates and times in programming, there are two important concepts that every developer should be familiar with: naive and aware datetime. These terms refer to how a programming language or framework handles the representation and manipulation of dates and times, and understanding the differences between them is crucial for writing accurately functioning code. In this article, we will explore the definitions of naive and aware datetime, compare their features, and delve into why and when each should be used. So, let’s dive in!
Naive Datetime:
Naive datetime, also known as unaware datetime, refers to a system that does not have information about timezones, daylight saving time, or any other specific time-related attributes. In simple terms, it represents a date and time without any context. Examples of naive datetime systems include Python’s ‘datetime’ module without the ‘pytz’ library and many other programming languages that lack built-in timezone handling capabilities.
Without timezone information, naive datetime systems assume that all datetime values are in the local timezone, which might lead to confusion when working with dates across different regions or when handling daylight saving time transitions. Thus, it is essential to handle timezone-related issues with caution when using naive datetime.
Furthermore, naive datetime objects often lack functionalities for performing timezone conversion, which limits their usability for applications that require accurate cross-timezone calculations or display.
Aware Datetime:
Aware datetime, on the other hand, is a datetime object that includes information about timezones, daylight saving time, and other relevant time-related attributes. It provides a richer representation of dates and times, allowing developers to handle multiple time zones, conversions, and daylight saving time transitions effortlessly.
Python’s ‘datetime’ module, when used in conjunction with the ‘pytz’ library, is an example of a system that supports aware datetime. Other programming languages, such as JavaScript, also offer built-in support for aware datetime representations.
When working with aware datetime, you can easily convert datetime objects from one timezone to another and perform accurate calculations involving different timezones. This flexibility is particularly valuable when developing applications that span multiple regions or when working with international teams.
Differences and Use Cases:
The most significant difference between naive and aware datetime lies in the level of detail and control they provide. Naive datetime can be useful in scenarios where timezone information is not critical, or the application exclusively operates within a single timezone. For example, if you are building a simple appointment tracking system for a local business, you may not need the complexities of timezone handling offered by aware datetime. In such cases, it is more efficient to stick with a naive datetime implementation, as it often involves less overhead.
However, for applications that have global implications and need to accurately handle various timezones, aware datetime is the way to go. Consider a project management tool used by a multinational team, where tasks and deadlines can span across different regions. In this case, you would require aware datetime to convert individual team members’ local times to a centralized timezone and ensure precise coordination.
Frequently Asked Questions (FAQs):
Q: Can I convert a naive datetime object to an aware datetime object?
A: Yes, you can convert a naive datetime object to an aware one by explicitly providing the timezone information. This can be done using the appropriate methods or functions offered by the programming language or library you are utilizing.
Q: What are some common challenges faced when dealing with naive datetime?
A: Naive datetime can pose challenges when working with multiple time zones, daylight saving time transitions, and international applications. It can lead to incorrect calculations, confusion when interpreting local times, and difficulty coordinating events across different regions.
Q: Are there any downsides to using aware datetime?
A: Aware datetime systems sometimes require additional libraries or dependencies to handle different timezones. This can increase the complexity of the codebase and introduce potential compatibility issues. However, the advantages offered by aware datetime generally outweigh these downsides for applications that require precise handling of timezones.
Q: Is aware datetime limited to certain programming languages?
A: No, aware datetime is not limited to specific programming languages. While some languages provide built-in support for aware datetime objects, libraries and frameworks exist for most languages to enable aware datetime functionality.
In conclusion, understanding the differences between naive and aware datetime is vital for accurate date and time handling in programming. Naive datetime, lacking timezone information, is suitable for simple applications operating within a single timezone. However, aware datetime, embracing timezone details, is indispensable when working with global time-related scenarios. By choosing the appropriate datetime representation for your specific use case, you can ensure the accurate representation and manipulation of dates and times, facilitating reliable and consistent programming.
Timezone Aware Datetime Python
What is timezone-aware datetime?
DateTime is a class in Python’s datetime module that represents a date and time. By default, datetime objects are naive, meaning they do not have any information about time zones. However, when working with international applications or systems that handle multiple time zones, it becomes crucial to consider time zone information. A timezone-aware datetime object includes not only the date and time but also the associated time zone information.
Why is timezone-aware datetime important?
If we only work with naive datetime objects, we risk encountering various problems when dealing with different time zones. For instance, if we have a global e-commerce system that allows users to view product listings and make purchases from any part of the world, we need to ensure that the displayed datetime corresponds to the correct time zone of the user.
Without timezone awareness, we could mistakenly display an incorrect time or miscalculate delivery dates based on the user’s location. Furthermore, when working with data across different time zones, we may need to perform operations such as conversions, comparisons, or calculations between different datetime objects. Accurate time zone information is vital in these scenarios.
Working with timezone-aware datetime in Python
Python provides the pytz library to handle time zones and timezone-aware datetime objects. First, we need to install pytz using pip:
“`
pip install pytz
“`
Once installed, we can import the necessary classes from the pytz module:
“`python
from datetime import datetime
import pytz
“`
To create a timezone-aware datetime object, we can use the `datetime` class’ `astimezone()` method in combination with the `pytz` module. Let’s demonstrate an example:
“`python
# Create a datetime object in the UTC time zone
utc_date = datetime(2022, 1, 1, 12, 0, 0, tzinfo=pytz.UTC)
# Convert the UTC datetime to a specific time zone
pacific_date = utc_date.astimezone(pytz.timezone(‘US/Pacific’))
print(pacific_date)
# Output: 2022-01-01 04:00:00-08:00
“`
In the example above, we created a `datetime` object in the UTC time zone and then converted it to the ‘US/Pacific’ time zone using `astimezone()`. The resulting `pacific_date` object is now timezone-aware and represents the same moment in time but in the Pacific time zone.
It’s important to note that the `pytz` library provides a comprehensive IANA time zone database, allowing us to specify numerous time zones with the `timezone()` method.
FAQs about timezone-aware datetime in Python
Q: Can I convert a naive datetime object to a timezone-aware datetime object?
A: Yes, you can convert a naive datetime object to a timezone-aware datetime object using the `astimezone()` method and a `timezone` object from the `pytz` module. The key is to provide the appropriate time zone information when converting.
Q: How can I obtain the current datetime in a specific time zone?
A: You can use the `datetime.now()` function from the `datetime` module combined with `pytz.timezone()` to obtain the current datetime in a particular time zone. Here’s an example:
“`python
import pytz
from datetime import datetime
tz = pytz.timezone(‘US/Pacific’)
current_time = datetime.now(tz)
print(current_time)
“`
Q: How can I compare timezone-aware datetime objects?
A: To compare timezone-aware datetime objects, you can use the standard comparison operators such as `<`, `>`, `<=`, `>=`, `==`. Python will take into account both the date/time values and the associated time zones during the comparison.
Conclusion
Managing time zones and working with timezone-aware datetime objects is crucial when dealing with international applications or systems that handle multiple time zones. By leveraging the pytz library, Python developers can easily convert, compare, and perform operations on datetime objects across different time zones. Understanding timezone-aware datetime empowers developers to ensure accurate and consistent handling of dates and times in their applications.
Images related to the topic can’t compare offset-naive and offset-aware datetimes
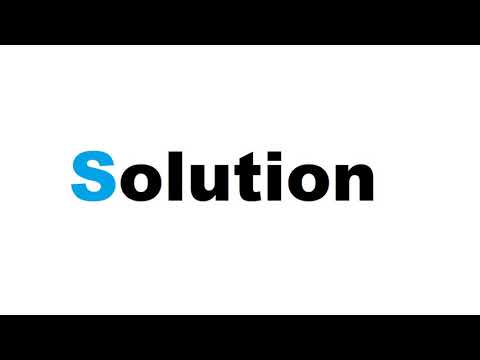
Found 46 images related to can’t compare offset-naive and offset-aware datetimes theme

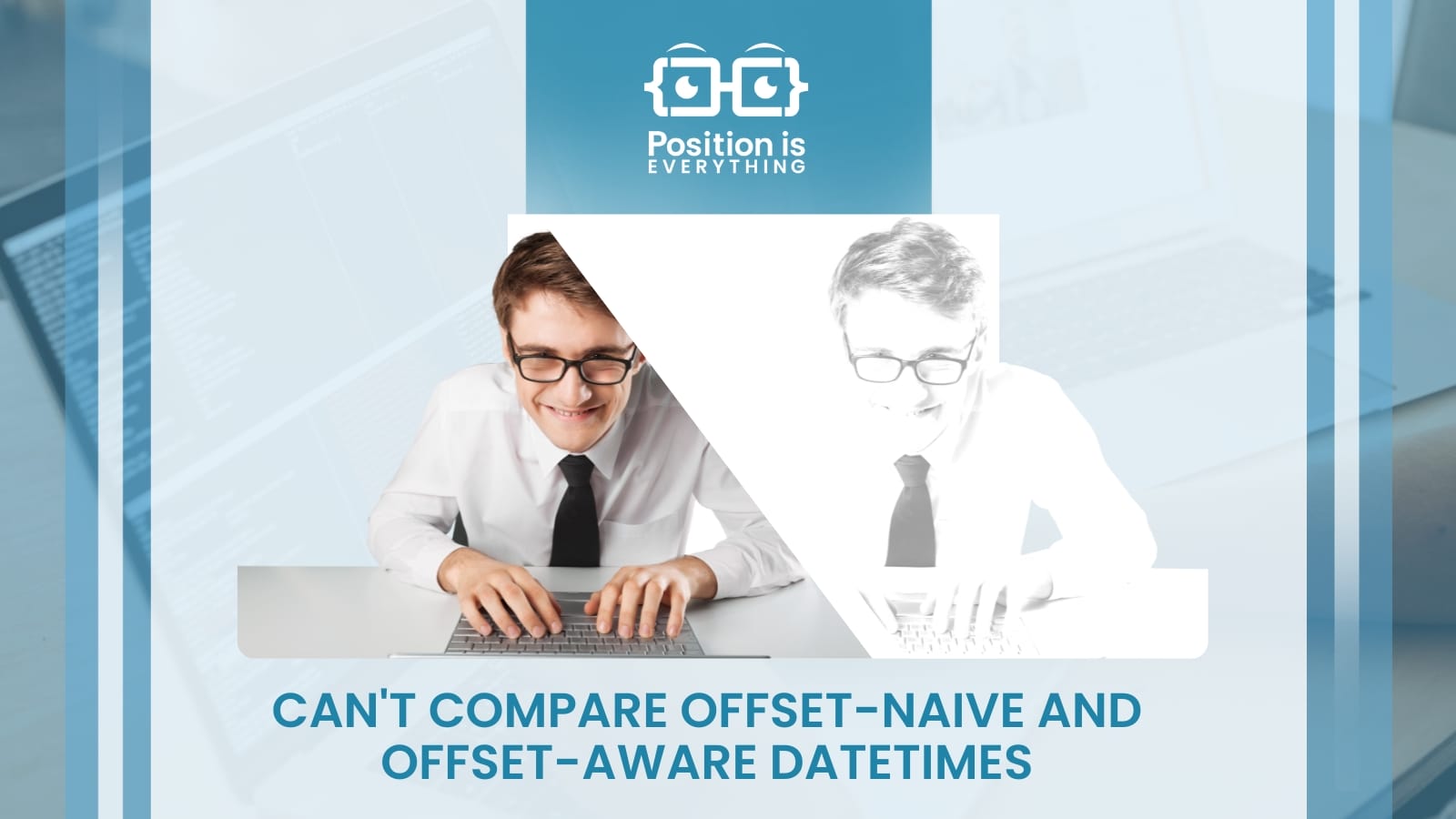

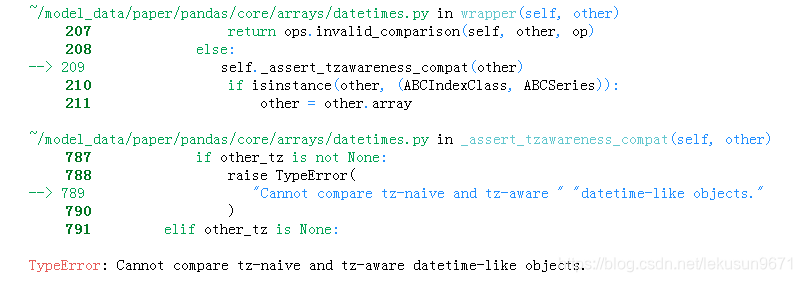
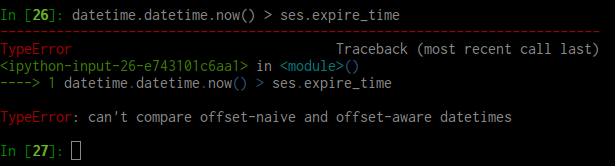
Article link: can’t compare offset-naive and offset-aware datetimes.
Learn more about the topic can’t compare offset-naive and offset-aware datetimes.
- Can’t compare naive and aware datetime.now() <= challenge ...
- 2 Ways to Fix Typeerror: can’t compare offset-naive and offset …
- can’t compare offset-naive and offset-aware datetimes [SOLVED]
- Can’t compare offset-naive and offset-aware datetimes [Fix]
- Can’t compare naive and aware datetime.now() <= challenge ...
- Can’t Compare Offset-naive and Offset-aware Datetimes: Solved!
- Comparing dates in Python – GeeksforGeeks
- Python Tutorial: How to Compare Dates in Python – Pierian Training
- How to remove timezone information from DateTime object in Python
- can’t compare offset-naive and offset-aware datetimes
- can’t compare offset-naive and offset-aware datetimes · Issue …
- Time zones – Django documentation
- can’t compare offset-naive and offset-aware datetimes – Reddit
See more: nhanvietluanvan.com/luat-hoc