Call Async Method From Sync Method C#
In C#, the async and await keywords provide a convenient way to work with asynchronous tasks. Asynchronous programming allows your programs to be more responsive and efficient by avoiding blocking operations. It allows multiple operations to be executed simultaneously, resulting in improved performance.
Understanding synchronous and asynchronous methods
Synchronous methods are those that block the execution until the operation is completed. These methods can be easy to understand and implement, as the execution happens sequentially. However, they can be time-consuming and can lead to inefficient resource utilization.
On the other hand, asynchronous methods are non-blocking and allow the program to continue with other tasks while the async operation is being executed. This improves the responsiveness of the program and allows better utilization of resources. Asynchronous methods typically return a Task or Task
What happens when you call an async method from a sync method?
When you call an async method from a sync method, the execution of the sync method will not wait for the async operation to complete. It will move on to the next line of code, potentially causing unexpected behavior or a race condition.
The drawbacks of calling an async method from a sync method
Calling an async method from a sync method can have several drawbacks. Firstly, it can lead to inefficient resource utilization because the sync method could be wasting time waiting for an async operation to complete. Additionally, it can cause deadlocks if the sync method blocks the calling thread and the async method requires that same thread to complete its operation.
Options for handling the asynchronous behavior from a sync method
There are several options available to handle the asynchronous behavior when calling an async method from a sync method.
1. Using Task.Run:
One option is to use the Task.Run method, which allows you to offload the async operation to a separate thread pool thread. This allows the sync method to continue execution while the async operation is being performed. However, it’s important to note that using Task.Run should be done cautiously, as it can lead to thread pool exhaustion if used indiscriminately.
2. Using Task.Wait/Task.Result:
Another option is to use Task.Wait or Task.Result to block the sync method until the async operation is complete. This can be useful in certain scenarios where you need to wait for the completion of the async operation before proceeding further.
3. Using Thread and ManualResetEvent:
One alternative approach is to use threads and ManualResetEvent to synchronize the async and sync methods. This involves creating a separate thread to execute the async operation, and then using a ManualResetEvent to block the sync method until the async operation is complete.
4. Using TaskCompletionSource:
The TaskCompletionSource class provides a way to create and control the execution of a Task manually. By using TaskCompletionSource, you can create a Task that represents the completion of the async operation and await it in the sync method.
FAQs:
Q: How can I call an async method from a sync method without waiting for its completion?
A: One way to call an async method without waiting for its completion is to use Task.Run to offload the operation to a separate thread.
Q: How do I convert an async method to a sync method?
A: Converting an async method to a sync method can be challenging, as it involves blocking the execution until the async operation completes. It can be done using techniques like Task.Wait or Task.Result, but it is generally not recommended as it can lead to deadlocks.
Q: Can I call an async method without using the await keyword?
A: Yes, you can call an async method without using the await keyword, but doing so will result in the method being executed synchronously.
Q: What are the benefits of using async and await in C#?
A: Using async and await in C# allows for better utilization of resources and improved program responsiveness. It enables parallel execution of tasks, leading to improved performance.
Q: Can I call an async method from a sync method in Python?
A: Yes, you can call an async method from a sync method in Python using the asyncio library. However, the approach may differ slightly from the one used in C#.
In conclusion, calling an async method from a sync method in C# requires careful consideration and understanding of the options available. Using the async and await keywords, along with other techniques like Task.Run or TaskCompletionSource, can help handle the asynchronous behavior effectively. However, it’s important to be cautious and avoid potential pitfalls, such as thread pool exhaustion or deadlocks.
Invoke Async Methods Simultaneously; Call Async Method From Sync Method In Visual Studio 2022 C#
How To Run Asynchronous Method Synchronously C#?
Asynchronous programming has become increasingly important in modern software development, as it allows a program to perform several tasks simultaneously, enhancing performance and responsiveness. However, sometimes there may be a need to run asynchronous methods synchronously, especially when dealing with legacy code or certain specific scenarios. In this article, we will explore different approaches to accomplish this in C#, including their advantages and disadvantages.
1. Understanding Asynchronous Programming in C#
Before diving into the synchronous execution of asynchronous methods, it is crucial to comprehend the basics of asynchronous programming in C#. Asynchronous methods are marked with the `async` modifier and return a `Task` or `Task
When invoking an asynchronous method, a continuation is scheduled to execute once the operation finishes, typically through an `await` keyword. During this time, the calling thread is released and can handle other tasks, significantly improving the overall efficiency and responsiveness of the application.
2. Synchronous Execution using Task.Wait()
The simplest way to run an asynchronous method synchronously is by calling the `Wait()` method on the returned task. The `Wait()` method blocks the current thread until the asynchronous operation completes, allowing us to write code as if it were synchronous.
“`csharp
public void MySynchronousMethod()
{
// Calling an asynchronous method synchronously
Task myTask = MyAsynchronousMethod();
myTask.Wait();
// Code execution continues after the asynchronous task completes
}
“`
While using `Wait()` may seem convenient, it can lead to potential deadlocks in certain situations. If the underlying asynchronous operation depends on a synchronization context, blocking the thread with `Wait()` can prevent the continuation from successfully completing.
3. Synchronous Execution using Task.Result
Another approach to running asynchronous methods synchronously is by using the `Result` property of the returned task. The `Result` property blocks the calling thread and waits for the completion of the asynchronous operation, similar to `Wait()`. However, it throws an exception if the underlying task is faulted or canceled. Therefore, it is recommended to wrap the call within a try-catch block.
“`csharp
public void MySynchronousMethod()
{
try
{
// Calling an asynchronous method synchronously
Task
int result = myTask.Result;
// Code execution continues after the asynchronous task completes
}
catch (AggregateException ex)
{
// Handle exceptions that occurred within the asynchronous task
}
}
“`
4. Synchronous Execution using Task.Run().GetAwaiter().GetResult()
A more robust technique to run asynchronous methods synchronously is by combining `Task.Run()`, `GetAwaiter()`, and `GetResult()`, as it avoids potential deadlocks.
“`csharp
public void MySynchronousMethod()
{
Task
int result = myTask.GetAwaiter().GetResult();
// Code execution continues after the asynchronous task completes
}
“`
Using this approach, we wrap the asynchronous method within `Task.Run()`, which schedules the task on a ThreadPool thread. Then, by invoking `GetAwaiter().GetResult()`, we ensure that the calling thread blocks until the asynchronous operation finishes without causing any deadlocks.
FAQs:
Q1. Why would I need to run asynchronous methods synchronously?
A1. There are several scenarios where running asynchronous methods synchronously may be necessary. This can include working with legacy code that expects synchronous behavior or when compliance with blocking APIs is required, such as if you need to integrate with older libraries that don’t support asynchronous programming.
Q2. Are there any performance implications of running asynchronous methods synchronously?
A2. Yes, running asynchronous methods synchronously can potentially degrade the performance of your application, especially in high-traffic scenarios. Since synchronous execution blocks the calling thread, it ties up system resources that could otherwise be used to handle additional tasks concurrently. Therefore, it is generally recommended to use asynchronous programming wherever possible.
Q3. Can I cancel a synchronous execution of an asynchronous method?
A3. No, once an asynchronous method is running synchronously, it cannot be canceled directly. Since the calling thread is blocked during the synchronous execution, it cannot receive any cancellation tokens or respond to abort requests. Therefore, it is important to consider the potential implications and limitations before opting for synchronous execution.
Q4. How do I handle exceptions when running asynchronous methods synchronously?
A4. When running asynchronous methods synchronously, it is crucial to handle any exceptions that might occur during the asynchronous operation. Using the `await` keyword or the `Task.Result` property within a try-catch block ensures that exceptions are appropriately handled and prevent unexpected application behavior.
In conclusion, while it is generally recommended to embrace asynchronous programming in C#, there are situations where running asynchronous methods synchronously is necessary. By understanding the various approaches discussed in this article, you can make informed decisions and handle such scenarios efficiently while weighing the trade-offs between performance and code simplicity.
What Is Async Method In Sync Code?
In modern programming, the need for asynchronous operations has become increasingly important. Asynchronous methods allow programs to perform tasks without blocking the execution of other tasks. This is particularly useful when dealing with time-consuming operations such as accessing databases or making API calls.
However, when working with synchronous code, which executes tasks in sequence, the concept of asynchronous methods might seem contradictory. So, what exactly is an async method in sync code?
An async method is a function that is designed to be executed asynchronously. It allows the program to continue executing other tasks while the async method is performing its operation. This is achieved through the use of asynchronous programming techniques, such as callbacks, promises, or async/await syntax, depending on the programming language or framework being used.
In sync code, tasks are typically executed one after another, meaning that the program waits for each task to complete before moving on to the next one. However, by utilizing async methods, developers can introduce non-blocking operations within their sync codebase. This enables the program to initiate an operation and continue executing other tasks in the meantime, without waiting for the completion of the async method.
One of the most common use cases for async methods in sync code is making network requests. For example, consider a web application that needs to fetch data from an external API. In a synchronous approach, the application would make the request and wait for the API response before proceeding with any subsequent tasks. This could potentially lead to a poor user experience if the request takes a considerable amount of time to complete.
By using async methods, developers can initiate the network request and continue executing other operations in the meantime. Once the API response is received, the async method can notify the program and handle the response accordingly. This allows the application to remain responsive to user interactions while waiting for the response from the API.
FAQs about async methods in sync code:
Q: Is async method limited to network requests only?
A: No, async methods can be used for any task that could potentially block the execution of other tasks, such as disk I/O operations, database querying, or file operations.
Q: How do async methods improve performance?
A: By utilizing async methods, programs can execute multiple tasks concurrently, improving overall performance by utilizing idle periods and reducing the waiting time for I/O or long-running operations.
Q: Does using async methods complicate the code?
A: While asynchronous programming introduces additional complexity, modern programming languages and frameworks provide powerful tools that simplify the implementation of async methods, such as async/await syntax.
Q: Can async methods be used in any programming language?
A: The availability and syntax of async methods may vary between programming languages and frameworks. However, most modern programming languages, such as JavaScript, Python, C#, and Java, provide support for asynchronous programming.
Q: Are there any potential pitfalls when using async methods?
A: Asynchronous programming introduces challenges, such as managing concurrency, error handling, and ensuring thread-safety. Additionally, excessive usage of async methods can lead to so-called “callback hell” or “promise chaining,” which can make code harder to read and maintain.
In conclusion, async methods in sync code provide a powerful tool for achieving concurrency and responsiveness in modern programming. By allowing tasks to execute concurrently, developers can enhance the performance and user experience of their applications. However, proper understanding and utilization of asynchronous programming techniques are essential to avoid potential pitfalls and maintain code readability.
Keywords searched by users: call async method from sync method c# Call async method in sync method C#, Call async function without await C#, Call async in sync method python, Call function async c#, How to convert async to sync c#, C# async method, Convert synchronous to asynchronous C#, Python call async function from sync
Categories: Top 47 Call Async Method From Sync Method C#
See more here: nhanvietluanvan.com
Call Async Method In Sync Method C#
In modern programming, asynchronous programming has become increasingly important. Asynchronous programming allows us to write code that can execute multiple tasks concurrently, resulting in improved performance and responsiveness of our applications. However, there are times when we need to call an asynchronous method from a synchronous method. In this article, we will explore various methods of calling an async method within a sync method in C# and understand the concepts behind it.
Understanding Asynchronous Programming in C#
Before we delve into calling async methods within sync methods, it’s essential to understand asynchronous programming in C#. In traditional synchronous programming, the execution flow follows a sequential order, where each line of code is executed one after the other. This can lead to performance issues if any task takes a considerable amount of time to complete.
Asynchronous programming, on the other hand, allows us to execute multiple tasks concurrently, releasing the main thread for other work while awaiting the completion of an async task. This enables our applications to be more responsive and scalable.
Calling an Async Method within a Sync Method
To call an async method within a sync method in C#, we have a few options at our disposal. Let’s explore them one by one:
1. Blocking the main thread: One way to call an async method from a sync method is by blocking the main thread. We can use the `.Result` or `.Wait()` methods on the async task to block the main thread until the task completes. However, this approach is not recommended, as it can lead to deadlocks in certain scenarios.
2. Using synchronous wrappers: Another method is to create synchronous wrappers for async methods. This involves creating a new synchronous method that internally calls the async method using the `.GetAwaiter().GetResult()` pattern. While this method is straightforward, it still suffers from a potential deadlock if not used carefully.
3. Creating a Task and synchronously waiting: One effective approach to calling an async method within a sync method is by creating a new `Task` and synchronously waiting for it to complete. We can achieve this by using the `Task.Run` method, which schedules the async method execution on a background thread.
“`
Task.Run(async () =>
{
await MyAsyncMethod();
}).GetAwaiter().GetResult();
“`
This method allows us to call an async method within a sync method without causing any deadlocks.
Frequently Asked Questions (FAQs):
Q: What is the benefit of calling async methods within sync methods?
A: Calling async methods within sync methods can provide better performance and responsiveness to our applications. It allows us to execute multiple tasks concurrently and frees up the main thread for other work.
Q: Is it possible to call an async method directly from a sync method without using any workarounds?
A: Yes, it is possible to call an async method directly from a sync method by blocking the main thread. However, this approach is not recommended as it can result in deadlocks in certain scenarios.
Q: Can we convert an async method into a sync method?
A: While it is possible to convert an async method into a sync method using blocking techniques, it is generally not recommended. The use of async/await pattern provides better scalability and responsiveness to our applications.
Q: What are the potential issues with blocking the main thread when calling async methods?
A: Blocking the main thread can lead to deadlocks in certain scenarios. Deadlocks can occur when the async method needs to continue executing on the main thread to complete but is blocked by the waiting sync method. This can result in unresponsive applications.
Q: Are there any best practices for calling async methods within sync methods?
A: It is generally recommended to avoid calling async methods within sync methods whenever possible. However, if necessary, using the `Task.Run` method to create a new task and synchronously waiting for it can be a safe approach to prevent deadlocks.
Conclusion
Asynchronous programming has become an integral part of modern programming, allowing us to improve the performance and responsiveness of our applications. While calling an async method from a sync method can be challenging, understanding the concepts and utilizing the appropriate methods can help overcome the hurdles. By following the techniques outlined in this article, you can effectively call async methods within sync methods in C# without compromising the scalability and responsiveness of your applications.
Call Async Function Without Await C#
Introduction:
Asynchronous programming is a fundamental concept in modern software development, enabling developers to write more efficient and responsive code. In C#, async/await keywords are widely used to create asynchronous functions. However, there may be circumstances where you want to call an async function without waiting for its completion. In this article, we will dive deep into this scenario, exploring the possibilities and potential use cases.
Understanding Asynchronous Programming in C#:
Before delving into the topic at hand, let’s quickly review the basic concepts of asynchronous programming in C#. In essence, asynchronous programming allows you to initiate a task and continue execution of the program without waiting for the task to complete. This improves the overall responsiveness and scalability of the application, especially in scenarios involving network requests, file operations, or computations that may take longer to complete.
In C#, async/await keywords were introduced in .NET Framework 4.5 to simplify asynchronous programming by providing a more readable and straightforward syntax. By marking a method with the async keyword, the compiler generates asynchronous state machines that allow non-blocking execution of the function. The await keyword then allows you to pause the execution of the method until the awaited task completes.
Calling an Async Function without Await:
By convention, calling an async function without awaiting the result is frowned upon, as it may result in potential issues such as unhandled exceptions and resource leaks. However, there may be situations where you might intentionally choose not to await the completion of an async function.
One possible scenario is when you want to initiate multiple asynchronous operations simultaneously and don’t need to wait for their completion before continuing. In such cases, you can create a List
“`
List
tasks.Add(Task.Run(() => MyAsyncFunction1()));
tasks.Add(Task.Run(() => MyAsyncFunction2()));
await Task.WhenAll(tasks);
“`
In this code snippet, MyAsyncFunction1 and MyAsyncFunction2 will be executed concurrently, and the await Task.WhenAll line ensures that the execution waits for all tasks to complete.
Another situation where calling an async function without await can be useful is when you want to initiate a fire-and-forget operation. This is commonly seen in scenarios where immediate response isn’t required or when you want to execute some operation in the background without affecting the main program flow.
To execute an async function without awaiting its completion, you can take advantage of the fact that calling an async function returns a Task that represents the ongoing execution of the method. By ignoring this Task, you effectively detach the function call from the main program flow, allowing it to continue independently. However, it’s crucial to ensure proper error handling and disposal of resources to prevent potential issues.
FAQs:
1. What happens if you don’t await an async function in C#?
If you call an async function without awaiting its completion, the function will run independently in the background, and the program flow will continue without waiting for it. This can be useful in scenarios where you want to execute a fire-and-forget operation.
2. Are there any potential issues with calling async functions without await?
Calling an async function without await can lead to unhandled exceptions and resource leaks if not properly managed. It’s crucial to handle exceptions by adding a try/catch block within the async function or using a global exception handler. Additionally, you should ensure proper disposal of resources by utilizing the IDisposable pattern or implementing the using statement where necessary.
3. What are the best practices for calling async functions without await?
Although calling async functions without await can have its use cases, it should generally be avoided unless necessary. It’s important to document and communicate the intentional use of fire-and-forget operations. Proper exception handling and resource disposal are crucial to prevent potential issues. If possible, use alternatives like concurrent execution using tasks for better control and clarity in your codebase.
Conclusion:
In conclusion, calling async functions without await in C# can be employed strategically in certain scenarios, such as concurrent execution or fire-and-forget operations. However, it’s essential to exercise caution and follow best practices to avoid potential issues with unhandled exceptions and resource leaks. Understanding the underlying concepts of asynchronous programming and employing the appropriate techniques will ensure a smooth and efficient approach to developing software in C#.
Call Async In Sync Method Python
Python is a versatile programming language with strong support for synchronous and asynchronous programming. Asynchronous programming allows us to write non-blocking code that can run concurrently, making it especially useful when dealing with I/O operations or network requests. However, there are scenarios where we might need to call an asynchronous function from a synchronous function. This is where the concept of “call async in sync method” comes into play.
Understanding Synchronous and Asynchronous Programming
Before diving into the details of calling async in sync methods, it’s important to understand the difference between synchronous and asynchronous programming.
In synchronous programming, code execution occurs sequentially, one line at a time. Each task must complete before moving on to the next. This approach is straightforward to reason about but can lead to inefficiencies when dealing with long-running tasks or waiting for I/O operations.
On the other hand, asynchronous programming allows tasks to run concurrently, reducing the time spent waiting for I/O operations to complete. Asynchronous code doesn’t wait for a task to finish before moving on to the next, making it more efficient when dealing with time-consuming operations.
Calling Asynchronous Functions from Synchronous Code
In Python, calling an asynchronous function from synchronous code requires a bit of extra work. By default, asynchronous functions return a coroutine object, which needs to be scheduled to run on an event loop. However, synchronous code doesn’t have an event loop, so the async function won’t run successfully.
One way to tackle this issue is by using an event loop explicitly. The asyncio module in Python provides an event loop that can be used to run asynchronous functions from synchronous code. The event loop runs concurrently, allowing for the execution of multiple tasks simultaneously.
To call an async function in a sync method, we need to create an event loop, schedule the async function to run on the event loop, and then run the event loop until the async function completes. Here’s an example:
“`python
import asyncio
async def async_function():
# Perform asynchronous tasks here
await asyncio.sleep(1)
return “Async function completed”
def sync_method():
loop = asyncio.get_event_loop()
result = loop.run_until_complete(async_function())
loop.close()
return result
“`
In the above example, the `async_function()` is an asynchronous function that performs some tasks asynchronously using the `await` keyword. The `sync_method()` is a synchronous function that calls the `async_function()` using an event loop. The `run_until_complete()` method schedules the async function to run on the event loop and waits until it completes.
When the event loop encounters the `await asyncio.sleep(1)` line in the `async_function()`, it suspends execution and allows other tasks to run. In this example, we are waiting for one second (simulated with `asyncio.sleep(1)`) before returning a result from the async function.
By using this approach, we can effectively call asynchronous functions from synchronous code and get the desired results.
FAQs:
Q: Why would I need to call an async function from synchronous code?
A: There could be various reasons for this requirement. One common scenario is when you have existing synchronous code and want to take advantage of an async function without disrupting the existing codebase. Another reason could be interacting with libraries or frameworks that provide async functions that you need to use from sync code.
Q: What are the benefits of using async functions?
A: Async functions allow for non-blocking code execution, making your program more efficient when waiting for I/O operations or performing time-consuming tasks. They can significantly improve the performance of applications that rely heavily on network requests or I/O operations.
Q: Can I make all my functions async to make my code more efficient?
A: While async functions offer advantages in certain scenarios, converting all your functions to async may not necessarily make your code more efficient. In fact, it might introduce unnecessary complexity without any noticeable benefit. Use async functions judiciously, especially when dealing with I/O-bound operations.
Q: Are there any alternatives to calling async functions from sync code?
A: Yes, there are other options available such as using callbacks, multiprocessing, or threading. Each approach has its own trade-offs and should be chosen based on the specific use case and requirements of your application.
In conclusion, calling async functions from synchronous code in Python allows us to take advantage of the benefits of asynchronous programming while seamlessly integrating with existing synchronous codebases. By understanding the concepts and techniques involved, you can enhance the efficiency and performance of your applications, especially when dealing with I/O operations or time-consuming tasks.
Images related to the topic call async method from sync method c#
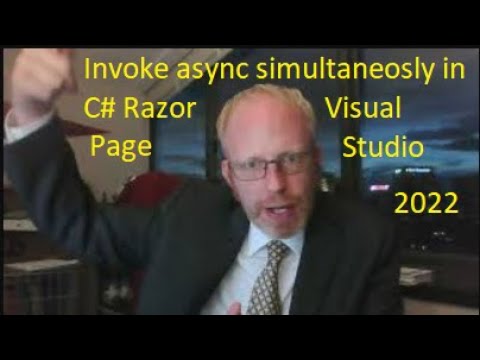
Article link: call async method from sync method c#.
Learn more about the topic call async method from sync method c#.
- How to call asynchronous method from synchronous method …
- How to call an asynchronous method from a synchronous …
- How to Run an Async Method Synchronously in .NET
- How to call asynchronous method from … – venkateswarlu.net
- How to Run an Async Method Synchronously in .NET
- How to call asynchronous method from … – venkateswarlu.net
- Async Main in C# with examples – Dot Net Tutorials
- Asynchronous programming – C# | Microsoft Learn
- What is the best approach to call asynchronous method from …
- How to call asynchronous method from … – Studytonight
- Async and Await in C# – C# Corner
See more: blog https://nhanvietluanvan.com/luat-hoc