C# Wait For Seconds
Introduction:
In C#, developers often need to introduce delays in their code execution. Whether it’s for creating animations, simulating real-time behavior, or executing a task after a certain interval, waiting for a specific amount of time is a common requirement. In this article, we will explore the different ways to achieve this in C# and discuss their pros, cons, and use cases.
What is waiting for seconds in C#?
Waiting for seconds in C# refers to a mechanism where the execution of code pauses for a specified duration expressed in seconds. This pause allows the program to halt temporarily, making the thread idle, and then resume after the specified time interval.
How to use the Thread.Sleep method?
The Thread.Sleep method is a commonly used approach to introduce delays in C# applications. It pauses the current thread’s execution for a specific amount of time. Here’s how you can use it:
“`csharp
// Import the Threading namespace
using System.Threading;
// Pause the execution for 5 seconds
Thread.Sleep(5000);
“`
In this example, the execution will be halted for 5 seconds before proceeding to the next line of code.
What are the alternatives to Thread.Sleep?
While Thread.Sleep is a straightforward and easy-to-use method for waiting in C#, there are alternatives available that offer more flexibility and efficiency. Let’s explore them:
1. Task.Delay:
Task.Delay is a part of the Task Parallel Library (TPL) introduced in .NET 4.5. It allows asynchronous waiting without blocking the executing thread. Here’s how it can be used:
“`csharp
// Import the Threading.Tasks namespace
using System.Threading.Tasks;
// Asynchronously wait for 5 seconds
await Task.Delay(5000);
“`
Unlike Thread.Sleep, Task.Delay allows the thread to be freed up during the waiting period, enabling it to handle other tasks efficiently.
2. async/await:
The async and await keywords were introduced in C# 5.0 and provide a simpler and more readable way to perform asynchronous operations. Here’s how you can use async/await to introduce a delay:
“`csharp
// Mark the method as async
async Task MyMethod()
{
// Asynchronously wait for 5 seconds
await Task.Delay(5000);
}
“`
Using async/await not only allows for waiting but also provides an easier way to handle complex asynchronous operations, making the code more manageable and readable.
What are the drawbacks of Thread.Sleep?
While Thread.Sleep may seem like a convenient option, it has some drawbacks that developers should be aware of:
1. Blocking the thread:
When using Thread.Sleep, the thread is explicitly blocked, which means it cannot be used to perform other tasks during the waiting period. This can lead to inefficient and unresponsive code, especially in multi-threaded scenarios.
2. Inaccurate timing:
Thread.Sleep can be inaccurate when it comes to timing precision. Factors such as thread scheduling, system load, and other processes running on the machine can affect the actual delay duration. Therefore, it may not be suitable for scenarios that require precise timing.
How to use Task.Delay for waiting in C#?
As mentioned earlier, Task.Delay is a more efficient and flexible alternative to Thread.Sleep. Here’s how you can use it:
1. Asynchronous Method:
“`csharp
// Import the Threading.Tasks namespace
using System.Threading.Tasks;
// Asynchronously wait for 5 seconds
await Task.Delay(5000);
“`
2. Synchronous Method:
“`csharp
// Import the Threading.Tasks namespace
using System.Threading.Tasks;
// Synchronously wait for 5 seconds
Task.Delay(5000).Wait();
“`
Task.Delay allows the thread to do other tasks while waiting, making it a better choice in most scenarios.
How to use async and await for waiting in C#?
Using async and await is not only for waiting but also for handling complex asynchronous operations. Here’s an example of how to use async/await to introduce a delay:
“`csharp
// Mark the method as async
async Task MyMethod()
{
// Asynchronously wait for 5 seconds
await Task.Delay(5000);
}
“`
By marking the method as async, we enable the use of the await keyword, which provides a cleaner and more readable way to introduce delays while ensuring the responsiveness of the application.
When should you use Thread.Sleep, Task.Delay, or async/await for waiting?
The choice between Thread.Sleep, Task.Delay, and async/await depends on the specific requirements of your application. Here are some guidelines to help you make the right decision:
1. Thread.Sleep: Use Thread.Sleep when precise timing is not critical, and you don’t mind blocking the thread during the waiting period. It is a simple and straightforward option for short delays.
2. Task.Delay: Use Task.Delay when you want to introduce delays without blocking the executing thread. It is suitable for most scenarios and offers better performance and flexibility compared to Thread.Sleep.
3. async/await: Use async/await when handling complex asynchronous operations is a requirement. It not only allows for waiting but also improves the overall readability and maintainability of the code, especially in scenarios involving multiple asynchronous tasks.
Conclusion:
In this article, we discussed different ways to wait for a specific amount of time in C#. We explored the traditional Thread.Sleep method, its drawbacks, alternatives like Task.Delay, and the benefits of using async/await. It is important for developers to understand the pros and cons of each approach to select the most suitable one based on their application’s requirements. By using the right waiting mechanism, you can ensure efficient, responsive, and maintainable code.
FAQs:
Q: What is the difference between Thread.Sleep and Task.Delay?
A: Thread.Sleep blocks the executing thread, while Task.Delay allows the thread to perform other tasks during the waiting period. Task.Delay is a more flexible and efficient option for introducing delays in C# applications.
Q: Can I use Thread.Sleep and Task.Delay together in the same code?
A: Yes, you can. However, it is not recommended to combine them unless there is a specific requirement. Using one waiting mechanism consistently throughout your codebase ensures consistency and maintainability.
Q: How precise is Task.Delay compared to Thread.Sleep?
A: Task.Delay provides better timing precision compared to Thread.Sleep, as it relies on the underlying timer mechanisms rather than blocking the thread explicitly. However, it is still subject to factors such as thread scheduling and system load.
Q: Is async/await only used for waiting in C#?
A: No, async/await is not limited to waiting. It is a powerful mechanism for handling asynchronous operations in general, allowing for cleaner and more readable code. Waiting is just one of the use cases for async/await.
Ai Ký Lệnh C..Ấ.. M Quay Video. Không Làm Láo Sao Phải C..Â. M.
Keywords searched by users: c# wait for seconds
Categories: Top 71 C# Wait For Seconds
See more here: nhanvietluanvan.com
Images related to the topic c# wait for seconds
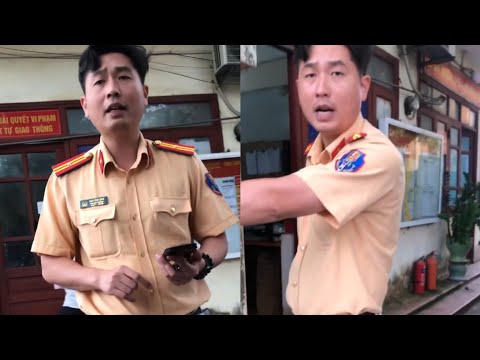
Article link: c# wait for seconds.
Learn more about the topic c# wait for seconds.
- C cơ bản: Giới thiệu ngôn ngữ C – DevIOT
- Ngôn ngữ lập trình C là gì? – Tại sao sử dụng C? – VietTuts.Vn
- Bài 1: Những khái niệm cơ bản về ngôn ngữ C – Tìm ở đây
- Ngôn ngữ lập trình C là gì? Tìm hiểu về ngôn ngữ lập trình C
- Ngôn ngữ lập trình C là gì? Tại Sao Cần Học Lập Trình C
- Trợ giúp > Mã của các nhãn – Cambridge Dictionary
See more: https://nhanvietluanvan.com/luat-hoc