C# Remove Last Character From String
1. Using the Substring method to remove the last character:
The Substring method allows you to extract a portion of a string. To remove the last character, you can utilize this method by specifying the start index as 0 and the length as the original string length minus 1. Here’s an example:
“`csharp
string originalString = “Hello World”;
string modifiedString = originalString.Substring(0, originalString.Length – 1);
// modifiedString will now be “Hello Worl”
“`
2. Utilizing the Remove method to eliminate the last character:
The Remove method allows you to remove a range of characters from a string. By using this method and specifying the start index as the length of the original string minus 1, and the count as 1, you can remove the last character. Here’s an example:
“`csharp
string originalString = “Hello World”;
string modifiedString = originalString.Remove(originalString.Length – 1, 1);
// modifiedString will now be “Hello Worl”
“`
3. Using the TrimEnd method to remove trailing characters:
The TrimEnd method is primarily used to remove specified characters from the end of a string. By passing an array containing the last character as the parameter, you can conveniently remove it. Here’s an example:
“`csharp
string originalString = “Hello World”;
char[] charsToRemove = { originalString[originalString.Length – 1] };
string modifiedString = originalString.TrimEnd(charsToRemove);
// modifiedString will now be “Hello Worl”
“`
4. Using Regular Expressions to remove the last character:
Regular Expressions, also known as regex, provide a flexible and powerful way to manipulate strings. By using a regular expression pattern that matches the last character, you can replace it with an empty string to remove it. Here’s an example:
“`csharp
using System.Text.RegularExpressions;
string originalString = “Hello World”;
string modifiedString = Regex.Replace(originalString, “.$”, string.Empty);
// modifiedString will now be “Hello Worl”
“`
5. Using StringBuilder to remove the last character efficiently:
String concatenation in C# can be an inefficient process, especially when done in a loop. StringBuilder provides a more efficient way to manipulate strings. You can use the Remove method of the StringBuilder class to remove the last character. Here’s an example:
“`csharp
using System.Text;
string originalString = “Hello World”;
StringBuilder stringBuilder = new StringBuilder(originalString);
stringBuilder.Remove(originalString.Length – 1, 1);
string modifiedString = stringBuilder.ToString();
// modifiedString will now be “Hello Worl”
“`
6. Applying the RemoveAt method for removing the last character:
In C#, strings are immutable, meaning they cannot be changed once created. However, you can convert the string to a char array, remove the last element using the RemoveAt method, and then convert it back to a string. Here’s an example:
“`csharp
string originalString = “Hello World”;
char[] charArray = originalString.ToCharArray();
Array.Resize(ref charArray, charArray.Length – 1);
string modifiedString = new string(charArray);
// modifiedString will now be “Hello Worl”
“`
7. Using Array.Resize to remove the last character from a char array:
Similar to the previous approach, you can directly resize the char array using the Array.Resize method, effectively removing the last character. Here’s an example:
“`csharp
string originalString = “Hello World”;
char[] charArray = originalString.ToCharArray();
Array.Resize(ref charArray, charArray.Length – 1);
string modifiedString = new string(charArray);
// modifiedString will now be “Hello Worl”
“`
By employing any of these methods, you can easily remove the last character from a string in C#. Choose the one that suits your specific requirements and coding style.
FAQs:
Q1. Can I remove multiple characters from the end of a string using these methods?
A1. Yes, you can modify the code in each method accordingly to remove multiple characters. For example, you can change the count parameter in the Remove method to the desired number of characters to remove.
Q2. Are there any performance differences between these methods?
A2. Yes, there are performance differences among the methods. Using StringBuilder is generally more efficient when you need to remove characters multiple times, such as in a loop. For a single removal operation, the performance differences are negligible.
Q3. Can I remove characters from the beginning or middle of a string?
A3. Yes, you can modify the parameters of the methods to remove characters from any position in the string. However, note that the RemoveAt method can only be used for removing characters from a char array, not a string.
Q4. Do these methods modify the original string, or do they create a new string?
A4. Most of these methods, such as Substring, Remove, TrimEnd, and StringBuilder’s Remove, create a new string or StringBuilder object with the desired modifications. Only the Remove method using a char array directly modifies the original string.
Q5. Can I use these methods with null or empty strings?
A5. Yes, these methods work with null or empty strings and produce the desired result. However, always handle null strings with proper null checks to avoid handling null reference exceptions.
In conclusion, removing the last character from a string in C# can be achieved by various methods, each with its own advantages. Choose the method that best suits your needs and coding style, considering factors such as performance and readability. With an understanding of these techniques, you can confidently manipulate strings in your C# applications.
Những Ca Khúc Hay Nhất Của Only C
Keywords searched by users: c# remove last character from string
Categories: Top 45 C# Remove Last Character From String
See more here: nhanvietluanvan.com
Images related to the topic c# remove last character from string
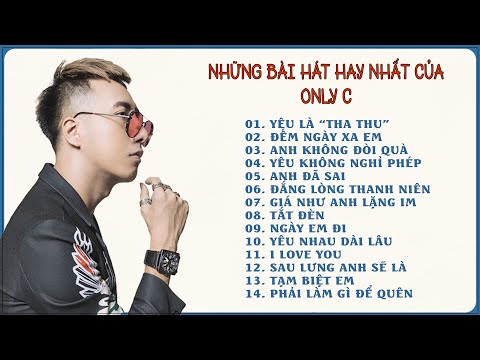
Article link: c# remove last character from string.
Learn more about the topic c# remove last character from string.
- Ngôn ngữ lập trình C là gì? – Tại sao sử dụng C? – VietTuts.Vn
- C cơ bản: Giới thiệu ngôn ngữ C – DevIOT
- Ngôn ngữ lập trình C là gì? Tìm hiểu về ngôn ngữ lập trình C
- C – Wiktionary tiếng Việt
- Miền Bắc tiếp tục nắng nóng gay gắt, nhiệt độ cao nhất 37độ C
- Bài 1: Những khái niệm cơ bản về ngôn ngữ C – Tìm ở đây
- C for Beginners – CodeLearn