C# Remove Characters From String
Introduction
In the world of programming, manipulating strings is a common task. There are various scenarios where we may need to remove specific characters from a string. This article will delve into different methods of removing characters from a string in C#, discussing each technique thoroughly. Whether you need to remove a single character or perform more complex operations, this guide has you covered.
Using the Remove method to delete a specific character
In C#, the Remove method allows us to delete a specific character from a string. This method returns a new string with the desired character removed. The syntax for using the Remove method is as follows:
string result = originalString.Remove(index, 1);
Here, ‘originalString’ represents the input string, ‘index’ refers to the position of the character to be removed, and ‘1’ denotes the number of characters to remove (in this case, it’s just one).
Removing multiple characters using the Replace method
If you need to remove multiple characters from a string, the Replace method is the way to go. This method replaces all occurrences of a specified character or a substring with another string. To remove characters, replace them with an empty string. Here’s an example:
string result = originalString.Replace(“characterToRemove”, “”);
In this code snippet, ‘originalString’ refers to the input string, and ‘characterToRemove’ represents the character(s) you want to remove. By replacing the target characters with an empty string, they effectively get removed from the output string.
Removing characters from the beginning of a string with the Substring method
To remove characters from the beginning of a string, C# provides the Substring method. This method extracts a substring, starting from a specified index to the end of the string. Here’s how you can use it to remove characters from the beginning:
string result = originalString.Substring(numOfCharactersToRemove);
In this example, ‘originalString’ is the input string, and ‘numOfCharactersToRemove’ represents the number of characters to remove from the beginning. The resulting string, stored in ‘result’, will exclude the specified number of characters from the start.
Removing characters from the end of a string with the Substring method
Similar to removing characters from the beginning, the Substring method can also be utilized to remove characters from the end of a string. Here’s an example:
string result = originalString.Substring(0, originalString.Length – numOfCharactersToRemove);
In this code snippet, ‘originalString’ represents the input string, and ‘numOfCharactersToRemove’ refers to the number of characters to be removed from the end. By specifying a range from the beginning of the string (‘0’) to the calculated length, we can exclude the desired characters from the output.
Deleting characters from a specific index position using the Remove method
In addition to removing individual characters, the Remove method can also delete characters based on a specific index position in a string. Here’s an example:
string result = originalString.Remove(startIndex, endIndex – startIndex);
In this example, ‘originalString’ denotes the input string, ‘startIndex’ refers to the index position from which the characters should be removed, and ‘endIndex’ indicates the end index position for the removal operation. By specifying the appropriate range, characters within that range will be deleted from the output string.
Trimming characters from both ends of a string with the Trim method
The Trim method is typically used to remove leading and trailing whitespace from a string. However, it can also be employed to remove specific characters from both ends of a string. In this case, we use the overloaded version of the Trim method, which takes an array of characters to remove. Here’s an example:
char[] charactersToRemove = { ‘a’, ‘b’, ‘c’ };
string result = originalString.Trim(charactersToRemove);
In this example, ‘originalString’ represents the input string, and ‘charactersToRemove’ is an array that contains the characters to be removed. The resulting string, stored in ‘result’, will have all occurrences of the specified characters removed from its beginning and end.
Removing non-alphanumeric characters using Regular Expressions
Regular expressions provide a powerful tool for pattern matching and manipulation of strings. To remove non-alphanumeric characters from a string in C#, we can utilize Regular Expressions. Here’s an example:
using System.Text.RegularExpressions;
string result = Regex.Replace(originalString, “[^a-zA-Z0-9]”, “”);
In this code snippet, ‘originalString’ denotes the input string, and the Regular Expression pattern [^a-zA-Z0-9] matches any character that is not a letter or a number. By replacing such characters with an empty string, we effectively remove the non-alphanumeric characters from the output string.
Removing characters based on custom conditions using Loops and Conditionals
For more complex scenarios, where removal of characters is dependent on specific conditions, we can utilize loops and conditionals. By iterating through the string and checking the desired conditions, we can selectively remove characters. Here’s a simplified example:
string result = “”;
foreach (char c in originalString)
{
if (/* add your condition here */)
{
result += c;
}
}
In this example, each character ‘c’ in the original string is evaluated against a custom condition. If the condition is met, the character is added to the ‘result’ string. By modifying the condition, you can achieve different removal operations based on your requirements.
FAQs
1. Can I remove characters from a string in C# without creating a new string?
No, in C#, strings are immutable, meaning that they cannot be changed after being created. Therefore, any operation that removes characters from a string will result in the creation of a new string.
2. Are these methods case-sensitive in C#?
Yes, both the Remove and Replace methods are case-sensitive. If you need to perform case-insensitive operations, you can use the StringComparison parameter available in these methods.
3. Can I perform multiple removal operations sequentially on a string?
Yes, you can chain multiple removal operations one after another. Each method call will return a new string, which can be further processed using another removal method.
Conclusion
Manipulating strings is an essential skill for every C# developer, and knowing how to remove characters from a string is a fundamental aspect of string manipulation. In this article, we explored various techniques to remove characters from a string in C#. From removing a single character to implementing complex conditions, we covered the essential methods and approaches required for different scenarios. By mastering these techniques, you will have the tools to efficiently remove characters from strings in your C# programs.
Ai Ký Lệnh C..Ấ.. M Quay Video. Không Làm Láo Sao Phải C..Â. M.
Keywords searched by users: c# remove characters from string
Categories: Top 99 C# Remove Characters From String
See more here: nhanvietluanvan.com
Images related to the topic c# remove characters from string
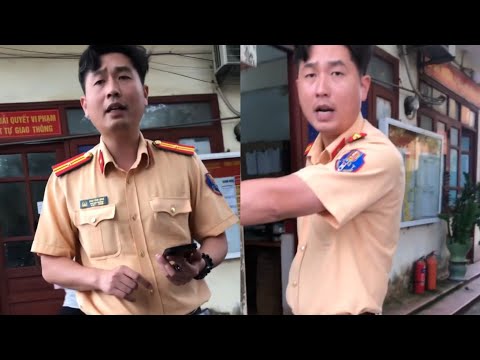
Article link: c# remove characters from string.
Learn more about the topic c# remove characters from string.
- C cơ bản: Giới thiệu ngôn ngữ C – DevIOT
- Ngôn ngữ lập trình C là gì? – Tại sao sử dụng C? – VietTuts.Vn
- Bài 1: Những khái niệm cơ bản về ngôn ngữ C – Tìm ở đây
- Ngôn ngữ lập trình C là gì? Tìm hiểu về ngôn ngữ lập trình C
- Ngôn ngữ lập trình C là gì? Tại Sao Cần Học Lập Trình C
- Trợ giúp > Mã của các nhãn – Cambridge Dictionary
See more: https://nhanvietluanvan.com/luat-hoc