C# Read A Csv File
Basics of CSV files
CSV files are simple text files that store tabular data in plain-text format. Each line of the file represents a row in the table, and the fields within each row are separated by a delimiter, typically a comma (hence the name Comma Separated Values). However, other delimiters such as semicolons or tabs can also be used.
Understanding the structure of a CSV file
CSV files can have a header row that contains the names of the columns, followed by the actual data rows. The header row helps in identifying the fields and their corresponding positions in the file. However, not all CSV files have a header row, and it’s essential to handle such cases as well.
Opening a CSV file in C#
To read a CSV file in C#, you first need to open the file using the appropriate APIs. The most common way to open a CSV file is by using the `StreamReader` class from the `System.IO` namespace.
“`csharp
using System.IO;
string filePath = “path/to/csv/file.csv”;
using (StreamReader reader = new StreamReader(filePath))
{
// Read the CSV file here
}
“`
Handling file exceptions when reading a CSV file
When working with file operations, it’s crucial to handle exceptions that may occur. In the case of reading a CSV file, exceptions such as `FileNotFoundException`, `IOException`, or `UnauthorizedAccessException` may occur. Make sure to use try-catch blocks to handle these exceptions gracefully and provide appropriate error messages to the users.
Reading the CSV file line by line
To read the contents of a CSV file, you need to loop through each line in the file using the `StreamReader.ReadLine()` method until the end of the file is reached.
“`csharp
string line;
while ((line = reader.ReadLine()) != null)
{
// Process each line here
}
“`
Parsing each line of the CSV file
Once you have read a line from the CSV file, you need to parse it into individual fields. The `String.Split()` method can be used to split the line into an array of fields based on the delimiter.
“`csharp
string[] fields = line.Split(‘,’);
// ‘fields’ array now contains the individual fields of the line
“`
Handling special characters and delimiters in the fields
Sometimes, a CSV field may contain special characters or the delimiter itself. In such cases, it’s essential to handle such situations correctly. One approach is to encapsulate the fields within double quotes (“) when writing the CSV file. On the reading side, libraries like the `CsvHelper` package can help parse CSV files with such considerations.
Storing the data from CSV file in appropriate data structures
Once you have parsed the fields, you need to decide on how to store the data for further processing. Depending on the nature of the data and the requirements of your application, you can use data structures like arrays, lists, or custom objects to store the data from the CSV file.
Performing additional processing on the CSV data if required
After reading and storing the data from the CSV file, you can perform additional processing on the data as per your requirements. This could involve calculations, data manipulation, or database operations. The possibilities are endless, and it largely depends on the specific needs of your application.
Now that we have covered the basics of reading a CSV file in C#, let’s address some frequently asked questions related to this topic.
***
**FAQs**
**Q: Can I read a CSV file with a different delimiter other than a comma?**
A: Yes, you can read a CSV file with a different delimiter by specifying the delimiter character when splitting the line using the `String.Split()` method. For example, to read a CSV file with a semicolon delimiter, you can use `string[] fields = line.Split(‘;’)`.
**Q: How can I handle CSV files without a header row?**
A: If a CSV file does not have a header row, you can directly process the data rows without the need for column names. In such cases, you can access the individual fields using their index positions in the `fields` array.
**Q: Is there a library available for reading CSV files in C#?**
A: Yes, several libraries are available for reading CSV files in C#. One popular library is `CsvHelper`, which provides advanced features for handling CSV data, including support for different delimiters, reading and writing using custom objects, and handling special characters in fields.
**Q: How can I handle large CSV files efficiently?**
A: When dealing with large CSV files, it’s recommended to use techniques like streaming and batch processing. Instead of loading the entire file into memory, you can read and process the file line by line to minimize memory usage. Additionally, consider using appropriate data structures and algorithms to optimize performance.
**Q: Can I use LINQ to query CSV data in C#?**
A: Yes, LINQ (Language Integrated Query) can be used to query CSV data in C#. LINQ provides a concise and expressive way to perform various operations on data, such as filtering, sorting, and aggregation. By converting the CSV data into objects or using the `DataTable` class, you can leverage the power of LINQ to query the data more efficiently.
***
In conclusion, reading a CSV file in C# involves understanding the structure of the file, opening the file using the `StreamReader` class, parsing the lines into individual fields, handling special characters and delimiters, and storing the data in appropriate data structures. By following these steps, you can efficiently read and process CSV files using C# and perform further operations on the data as per your application’s requirements.
Ai Ký Lệnh C..Ấ.. M Quay Video. Không Làm Láo Sao Phải C..Â. M.
Keywords searched by users: c# read a csv file
Categories: Top 94 C# Read A Csv File
See more here: nhanvietluanvan.com
Images related to the topic c# read a csv file
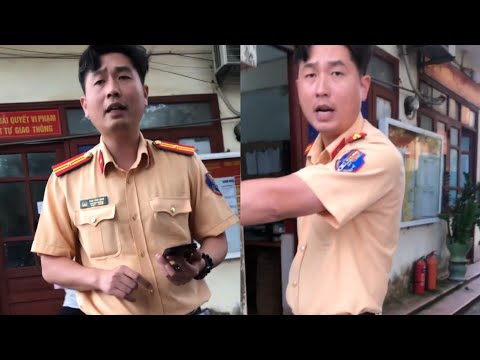
Article link: c# read a csv file.
Learn more about the topic c# read a csv file.
- C cơ bản: Giới thiệu ngôn ngữ C – DevIOT
- Ngôn ngữ lập trình C là gì? – Tại sao sử dụng C? – VietTuts.Vn
- Ngôn ngữ lập trình C là gì? Tìm hiểu về ngôn ngữ lập trình C
- Ngôn ngữ lập trình C là gì? Tại Sao Cần Học Lập Trình C
- C – Wiktionary tiếng Việt
- Bài 1: Những khái niệm cơ bản về ngôn ngữ C – Tìm ở đây
See more: https://nhanvietluanvan.com/luat-hoc