C# Pass Function As Parameter
Basic Syntax for Passing a Function as a Parameter in C#
To pass a function as a parameter in C#, you need to use delegates. A delegate is a reference type that can hold a reference to a method with a specific signature. Here is the basic syntax for passing a function as a parameter in C#:
“`csharp
public delegate returnType DelegateName(parameterType parameterName);
public void FunctionThatTakesDelegate(DelegateName delegateParameter)
{
// Code that uses the delegate
returnType result = delegateParameter(arguments);
}
“`
In this syntax, `DelegateName` is the name of the delegate type, `returnType` is the return type of the delegate, `parameterType` is the type of the parameter(s) the delegate accepts, and `parameterName` is the name of the parameter(s). `FunctionThatTakesDelegate` is the function that takes the delegate as a parameter. Inside this function, you can call the delegate like a regular function, passing the necessary arguments.
Different Ways to Define a Function Delegate in C#
In C#, you can define a function delegate using different approaches based on your requirements and coding style. Here are some of the ways to define a function delegate in C#:
1. Using the `delegate` keyword:
“`csharp
delegate returnType DelegateName(parameterType parameterName);
“`
2. Using the `Action` delegate type:
“`csharp
Action
“`
The `Action` delegate type is a built-in delegate type that represents a method with a void return type and up to sixteen parameters. If you don’t need a return value from the delegate, you can use `Action` to define the delegate.
3. Using the `Func` delegate types:
“`csharp
Func
Func
“`
The `Func` delegate types are a set of built-in delegate types with one to sixteen parameters and a return value. The last type parameter specifies the return type.
Passing a Function as a Parameter to Another Function
Once you’ve defined a function delegate, you can easily pass a function as a parameter to another function. Here is an example that demonstrates this:
“`csharp
public static void PrintMessage(string message)
{
Console.WriteLine(message);
}
public static void ExecuteFunction(Action
{
action(message);
}
static void Main(string[] args)
{
ExecuteFunction(PrintMessage, “Hello, World!”);
}
“`
In this example, `PrintMessage` is a function that takes a string parameter and prints it to the console. The `ExecuteFunction` function takes an `Action
Using Built-in Delegate Types in C#
As mentioned earlier, C# provides several built-in delegate types that you can use as function delegates. Here are a few commonly used ones:
1. `Action`: Represents a method with a void return type and up to sixteen parameters.
2. `Func
3. `Predicate
4. `EventHandler`: Represents a method that handles an event.
Creating Custom Delegate Types in C#
In addition to the built-in delegate types, you can create custom delegate types to meet specific requirements. To define a custom delegate, you can use the `delegate` keyword followed by the delegate signature. Here is an example:
“`csharp
public delegate int Calculate(int a, int b);
“`
In this example, we define a custom delegate type `Calculate` that represents a method with two `int` parameters and an `int` return type. You can then use this delegate type to pass functions with the specified signature as parameters.
Using Lambda Expressions to Pass Functions as Parameters
Lambda expressions provide a concise syntax for representing anonymous functions. They allow you to define a function inline without explicitly declaring a delegate. Here is an example that demonstrates using lambda expressions to pass functions as parameters:
“`csharp
public static int Add(int a, int b)
{
return a + b;
}
public static void PerformOperation(Func
{
int result = operation(2, 3);
Console.WriteLine(result);
}
static void Main(string[] args)
{
PerformOperation((a, b) => a + b);
PerformOperation(Add);
}
“`
In this example, we define an `Add` function that takes two `int` parameters and returns their sum. The `PerformOperation` function takes a `Func
Using Anonymous Methods to Pass Functions as Parameters
Anonymous methods provide another way to define and pass functions as parameters without explicitly declaring a delegate. They are similar to lambda expressions but with a slightly different syntax. Here is an example that demonstrates using anonymous methods:
“`csharp
public static void PrintMessage(string message)
{
Console.WriteLine(message);
}
public static void ExecuteAction(Action
{
action(message);
}
static void Main(string[] args)
{
ExecuteAction(delegate(string message)
{
Console.WriteLine(“Before: ” + message);
PrintMessage(message);
Console.WriteLine(“After: ” + message);
}, “Hello, World!”);
}
“`
In this example, we define a `PrintMessage` function that takes a string parameter and prints it to the console. The `ExecuteAction` function takes an `Action
Benefits and Use Cases of Passing Functions as Parameters in C#
Passing functions as parameters in C# offers several benefits and enables various use cases. Here are some advantages of using this approach:
1. Enhanced reusability: By passing functions as parameters, you can create generic functions that can be customized based on the behavior you want to achieve.
2. Code brevity: Using lambda expressions or anonymous methods, you can define functions directly at the call site, avoiding the need for separate method definitions.
3. Loosely coupled code: Passing functions as parameters promotes loose coupling between different components of an application, making the code more modular and easier to maintain.
4. Callbacks and event handling: Functions can be passed as parameters to handle callbacks or events, allowing for event-driven programming.
Use cases for passing functions as parameters include:
1. Sorting and filtering data: Functions can be passed to sorting or filtering algorithms to define custom sorting order or filtering conditions.
2. Asynchronous processing: Functions can be passed as parameters to asynchronous methods to specify actions to be executed upon completion or failure.
3. Event handling: Functions can be passed as event handlers to handle specific events in an application.
FAQs
Q: Can we pass multiple functions as parameters in C#?
A: Yes, you can pass multiple functions as parameters in C#. To do this, you can define a delegate with multiple function signatures or use multiple delegate parameters.
Q: How do we know the signature of a function that needs to be passed as a parameter?
A: The signature of the function is determined by the delegate it needs to be compatible with. You can refer to the delegate definition or documentation to determine the required signature.
Q: Can we define delegates inside a class?
A: Yes, delegates can be defined inside a class, much like any other member. The visibility and accessibility depend on the access modifiers used.
Q: What is the difference between lambda expressions and anonymous methods?
A: Lambda expressions provide a more concise syntax for defining anonymous functions compared to anonymous methods. Lambdas use the `=>` operator, while anonymous methods use the `delegate` keyword.
Q: What is the purpose of the `Action` and `Func` delegate types?
A: The `Action` delegate type is used when you need to represent a method with no return value. The `Func` delegate types are used when you need to represent methods with varying numbers of parameters and return values.
In conclusion, passing functions as parameters in C# using delegates provides a powerful way to customize code behavior, enhance reusability, and promote modular programming. By understanding the basic syntax, different ways to define function delegates, and utilizing built-in and custom delegate types, developers can harness the full potential of this feature. Whether it’s sorting and filtering data, handling events, or implementing asynchronous processing, passing functions as parameters empowers developers to write more flexible and efficient code in C#.
Ai Ký Lệnh C..Ấ.. M Quay Video. Không Làm Láo Sao Phải C..Â. M.
Keywords searched by users: c# pass function as parameter
Categories: Top 30 C# Pass Function As Parameter
See more here: nhanvietluanvan.com
Images related to the topic c# pass function as parameter
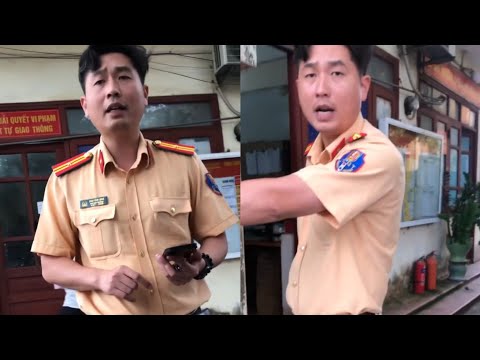
Article link: c# pass function as parameter.
Learn more about the topic c# pass function as parameter.
- C cơ bản: Giới thiệu ngôn ngữ C – DevIOT
- Ngôn ngữ lập trình C là gì? – Tại sao sử dụng C? – VietTuts.Vn
- Ngôn ngữ lập trình C là gì? Tìm hiểu về ngôn ngữ lập trình C
- C – Wiktionary tiếng Việt
- Bài 1: Những khái niệm cơ bản về ngôn ngữ C – Tìm ở đây
- Ngôn ngữ lập trình C là gì? Tại Sao Cần Học Lập Trình C
See more: https://nhanvietluanvan.com/luat-hoc