C# Object To Datetime
In C#, working with DateTime values is a common requirement when developing applications that deal with dates, times, and scheduling. Whether you need to parse a string into a DateTime object, convert an integer or double value to DateTime, format a DateTime object as a string, or handle time zones and conversions, the DateTime class in C# provides a wide range of methods and properties to assist you. In this article, we will explore various techniques to convert and manipulate DateTime values in C#, covering topics like converting a string to DateTime, converting an integer or double to DateTime, formatting DateTime as a string, converting DateTime to Unix timestamp, working with DateTime properties and methods, and handling time zones and conversions.
—
Converting a String to DateTime Using the Parse() Method
The Parse() method in C# allows you to convert a string representation of a date and time into a DateTime object. It can handle a variety of date formats, including standard date strings and custom date formats. The syntax of the Parse() method is as follows:
“`csharp
DateTime.Parse(string input)
“`
Here, the `input` parameter represents the string that you want to convert to a DateTime object. Let’s take a look at an example:
“`csharp
string dateString = “2022-01-01”;
DateTime dateTime = DateTime.Parse(dateString);
“`
In this example, the `dateString` variable contains a string representation of a date (“2022-01-01”). By calling the Parse() method on this string, we are able to convert it into a DateTime object.
However, it’s important to note that the Parse() method may throw a FormatException if the input string is not in a valid date and time format. To handle such exceptions, you can use the TryParse() method, which returns true if the conversion is successful and false if it fails.
—
Converting an Integer or Double to DateTime Using TimeSpan
In some cases, you may need to convert an integer or double value representing a specific time interval to a DateTime object. C# provides the TimeSpan structure, which allows you to represent a time interval or duration. You can use methods like TimeSpan.FromDays(), TimeSpan.FromHours(), TimeSpan.FromSeconds(), etc., to convert an integer or double value to a TimeSpan object. Here’s an example of converting an integer value to TimeSpan:
“`csharp
int hours = 24;
TimeSpan timeSpan = TimeSpan.FromHours(hours);
DateTime dateTime = DateTime.Now.Add(timeSpan);
“`
In this example, we have an integer value `hours` representing the number of hours to add. We use the TimeSpan.FromHours() method to convert the integer value to a TimeSpan object. Then, by calling the Add() method on the current DateTime object (`DateTime.Now`), we add the TimeSpan to get the final DateTime value.
Similarly, you can convert a double value to TimeSpan using methods like TimeSpan.FromMilliseconds(), TimeSpan.FromSeconds(), etc. The process is the same as converting an integer value.
—
Converting a DateTime Object to a String Using the ToString() Method
The ToString() method in C# allows you to convert a DateTime object to its string representation. It converts the date and time value into a formatted string based on the specified format options. The syntax of the ToString() method is as follows:
“`csharp
DateTime.ToString(string format)
“`
Here, the `format` parameter represents the format specifier that determines how the DateTime value will be formatted. C# provides a variety of standard date and time format specifiers that you can use, such as “d” for short date pattern, “t” for short time pattern, “yyyy” for four-digit year, etc. Here’s an example:
“`csharp
DateTime dateTime = DateTime.Now;
string formattedDateTime = dateTime.ToString(“yyyy-MM-dd HH:mm:ss”);
“`
In this example, the `dateTime` object represents the current date and time. By calling the ToString() method with the format specifier “yyyy-MM-dd HH:mm:ss”, we get the formatted string representation of the DateTime object.
You can also use custom date and time format specifiers to display the DateTime value in a specific format. For example, “MMMM” represents the full month name, “hh” represents the two-digit hour in a 12-hour clock, “tt” represents the AM/PM designator, etc. Experiment with different format options to achieve the desired output.
—
Converting a DateTime Object to Unix Timestamp
In certain scenarios, you may need to convert a DateTime object to a Unix timestamp. A Unix timestamp is a numerical representation of the number of seconds or milliseconds that have elapsed since January 1, 1970, at 00:00:00 UTC (Coordinated Universal Time). C# provides two methods, `ToUnixTimeSeconds()` and `ToUnixTimeMilliseconds()`, to convert a DateTime object to Unix timestamp.
Here’s an example of converting a DateTime object to Unix timestamp in seconds:
“`csharp
DateTime dateTime = DateTime.Now;
long unixTimestampSeconds = dateTime.ToUnixTimeSeconds();
“`
In this example, the `dateTime` object represents the current date and time. By calling the `ToUnixTimeSeconds()` method on the DateTime object, we get the Unix timestamp (in seconds) as a `long` value.
Similarly, you can use the `ToUnixTimeMilliseconds()` method to get the Unix timestamp in milliseconds.
—
Working with DateTime Properties and Methods
The DateTime class in C# provides various properties and methods to manipulate and retrieve information from DateTime objects. Here are some commonly used properties and methods:
– `Year`, `Month`, `Day`, `Hour`, `Minute`, `Second`: These properties allow you to access the individual components of a DateTime object. For example:
“`csharp
DateTime dateTime = DateTime.Now;
int year = dateTime.Year;
int month = dateTime.Month;
int day = dateTime.Day;
int hour = dateTime.Hour;
int minute = dateTime.Minute;
int second = dateTime.Second;
“`
– `Add()`, `Subtract()`: These methods allow you to perform arithmetic operations on DateTime objects. For example, to add or subtract a specific time interval from a DateTime object:
“`csharp
DateTime dateTime = DateTime.Now;
TimeSpan timeSpan = TimeSpan.FromDays(7);
DateTime newDateTime = dateTime.Add(timeSpan);
“`
– `CompareTo()`: This method allows you to compare two DateTime objects and determine their relative order. It returns an integer value indicating the relationship between the two DateTime objects.
“`csharp
DateTime dateTime1 = DateTime.Now;
DateTime dateTime2 = DateTime.Now.AddDays(1);
int result = dateTime1.CompareTo(dateTime2);
// result will be -1, indicating that dateTime1 is earlier than dateTime2
“`
Additionally, you can perform various date and time calculations using these properties and methods. For example, calculating the difference between two dates, finding the age based on a birthdate, etc.
—
Handling Time Zones and Converting DateTime Objects
When working with DateTime values, it’s crucial to consider time zones and perform conversions if necessary. In C#, you can handle time zones using the TimeZoneInfo class and the DateTimeOffset structure.
The TimeZoneInfo class provides methods to convert a DateTime object to a specific time zone or retrieve information about time zones. Here’s an example of converting a DateTime object to a specific time zone:
“`csharp
DateTime dateTime = DateTime.Now;
TimeZoneInfo timeZoneInfo = TimeZoneInfo.FindSystemTimeZoneById(“Pacific Standard Time”);
DateTimeOffset dateTimeOffset = TimeZoneInfo.ConvertTime(dateTime, timeZoneInfo);
“`
In this example, the `dateTime` object represents the current date and time. We use the `TimeZoneInfo.FindSystemTimeZoneById()` method to find the time zone information for “Pacific Standard Time”. Then, we call the `TimeZoneInfo.ConvertTime()` method to convert the DateTime object to the specified time zone, resulting in a DateTimeOffset object.
You can also work with UTC time by using the `DateTime.UtcNow` property. UTC (Coordinated Universal Time) is a standard time reference that eliminates time zone variations. Additionally, you can adjust DateTime values for daylight saving time (DST) or convert them to different time zones as per your requirements.
—
FAQs
Q: Can I convert a string in a different format to DateTime using the Parse() method?
A: Yes, the Parse() method attempts to convert the input string based on different standard date and time formats. However, if the input string doesn’t match any of the expected formats, a FormatException will be thrown. In such cases, you may need to use the TryParse() method or provide a custom format specifier.
Q: How can I handle exceptions during string to DateTime conversion?
A: To handle exceptions, you can use a try-catch block and catch the FormatException. Alternatively, you can use the TryParse() method, which returns true or false based on the success of the conversion, without throwing an exception.
Q: Can I format the DateTime value while converting it to a string using the ToString() method?
A: Yes, the ToString() method allows you to specify a format specifier to control how the DateTime value will be formatted. You can use standard date and time format specifiers or custom format specifiers to achieve the desired formatting.
Q: Is it possible to convert a DateTime object to Unix timestamp?
A: Yes, C# provides methods like ToUnixTimeSeconds() and ToUnixTimeMilliseconds() to convert a DateTime object to Unix timestamp. The resulting Unix timestamp represents the number of seconds or milliseconds that have elapsed since January 1, 1970, at 00:00:00 UTC.
Q: How can I perform calculations using DateTime objects?
A: The DateTime class provides properties and methods like Add(), Subtract(), CompareTo(), etc., that allow you to perform various calculations and operations with DateTime objects. You can add or subtract time intervals, compare DateTime values, and access individual components of a DateTime object.
Q: How can I handle time zones and convert DateTime objects?
A: C# provides the TimeZoneInfo class and the DateTimeOffset structure to handle time zones and perform conversions. The TimeZoneInfo class allows you to convert DateTime objects to specific time zones or retrieve time zone information. The DateTimeOffset structure provides better support for time zones by including the time zone offset in addition to the date and time values.
Beach Song + More Nursery Rhymes \U0026 Kids Songs – Cocomelon
Keywords searched by users: c# object to datetime
Categories: Top 63 C# Object To Datetime
See more here: nhanvietluanvan.com
Images related to the topic c# object to datetime
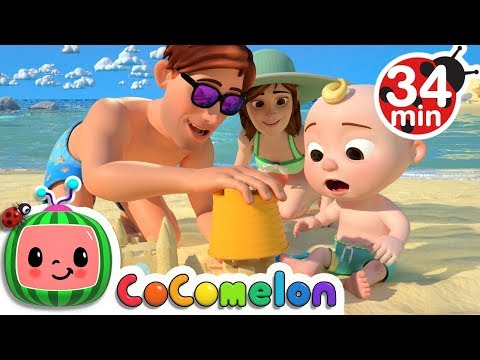
Article link: c# object to datetime.
Learn more about the topic c# object to datetime.
- C cơ bản: Giới thiệu ngôn ngữ C – DevIOT
- Ngôn ngữ lập trình C là gì? – Tại sao sử dụng C? – VietTuts.Vn
- Bài 1: Những khái niệm cơ bản về ngôn ngữ C – Tìm ở đây
- Ngôn ngữ lập trình C là gì? Tìm hiểu về ngôn ngữ lập trình C
- C – Wiktionary tiếng Việt
- C for Beginners – CodeLearn
See more: https://nhanvietluanvan.com/luat-hoc/