C# Move A File
File manipulation is a crucial aspect of software development, as it involves tasks such as checking file existence, moving files, renaming files, copying files, and deleting files. Efficient file management is essential for organizing data and ensuring smooth operation of software applications. In this article, we will explore various file manipulation techniques using the C# programming language.
C# is a versatile and powerful programming language commonly used for developing Windows applications and web services. It provides a robust set of file manipulation methods and classes that enable developers to perform a wide range of file-related tasks with ease. Whether you need to move, rename, copy, or delete a file, C# offers simple and effective ways to accomplish these tasks.
Checking File Existence
Before performing any file manipulation tasks, it is essential to check whether the file exists. In C#, you can use the File.Exists method to determine if a file exists at a given path. This method returns a Boolean value indicating whether the specified file exists.
When checking file existence, you should consider both absolute and relative file paths. An absolute file path specifies the exact location of a file on the system, while a relative file path is relative to the current working directory of the application.
To handle file existence checking in C#, you can use the following code snippet:
“`csharp
string filePath = “C:\\path\\to\\file.txt”;
if (File.Exists(filePath))
{
// File exists
}
else
{
// File does not exist
}
“`
It is important to handle exceptions that may occur during file existence checking, such as invalid file paths or insufficient permissions. By using try-catch blocks, you can gracefully handle these exceptions and provide meaningful error messages to the users of your application.
Moving Files
Moving a file involves transferring it from one location to another. In C#, you can use the File.Move method to move a file. This method takes two parameters – the source file path and the destination file path.
Here is an example of how to move a file using C#:
“`csharp
string sourceFilePath = “C:\\path\\to\\file.txt”;
string destinationFilePath = “C:\\new\\path\\file.txt”;
try
{
File.Move(sourceFilePath, destinationFilePath);
Console.WriteLine(“File moved successfully.”);
}
catch (Exception ex)
{
Console.WriteLine(“An error occurred while moving the file: ” + ex.Message);
}
“`
When moving a file, it is important to handle exceptions that may arise, such as file conflicts or file not found errors. If the destination file already exists, an exception will be thrown. Additionally, if the source file is not found, an exception will be raised. By handling these exceptions, you can ensure that the file move operation is performed smoothly and gracefully.
Renaming Files
Renaming a file involves changing its name while maintaining its file path. In C#, you can use the File.Move method to rename a file. Similar to moving a file, this method requires the source file path and the new file path as parameters.
Here’s an example of how to rename a file using C#:
“`csharp
string sourceFilePath = “C:\\path\\to\\file.txt”;
string newFileName = “newfile.txt”;
try
{
string directory = Path.GetDirectoryName(sourceFilePath);
string newFilePath = Path.Combine(directory, newFileName);
File.Move(sourceFilePath, newFilePath);
Console.WriteLine(“File renamed successfully.”);
}
catch (Exception ex)
{
Console.WriteLine(“An error occurred while renaming the file: ” + ex.Message);
}
“`
When renaming a file, it is essential to handle exceptions that may occur, such as invalid file names or existing file conflicts. If the new file name is invalid or if a file with the same name already exists at the destination, an exception will be thrown. By handling these exceptions, you can ensure that the file gets renamed successfully.
Copying Files
Copying a file involves creating a replica of it in another location. In C#, you can use the File.Copy method to copy a file. This method requires the source file path and the destination file path as parameters.
Here’s an example of how to copy a file using C#:
“`csharp
string sourceFilePath = “C:\\path\\to\\file.txt”;
string destinationFilePath = “C:\\destination\\file.txt”;
try
{
File.Copy(sourceFilePath, destinationFilePath);
Console.WriteLine(“File copied successfully.”);
}
catch (Exception ex)
{
Console.WriteLine(“An error occurred while copying the file: ” + ex.Message);
}
“`
When copying a file, it is important to handle exceptions that may occur, such as file conflicts or insufficient permissions. If a file with the same name already exists at the destination, an exception will be thrown. Additionally, if the destination directory does not have sufficient write permissions, an exception will be raised. By handling these exceptions, you can ensure that the file copy operation is performed smoothly and gracefully.
Deleting Files
Deleting a file involves permanently removing it from the system. In C#, you can use the File.Delete method to delete a file. This method requires the file path as a parameter.
Here’s an example of how to delete a file using C#:
“`csharp
string filePath = “C:\\path\\to\\file.txt”;
try
{
File.Delete(filePath);
if (!File.Exists(filePath))
{
Console.WriteLine(“File deleted successfully.”);
}
else
{
Console.WriteLine(“File deletion failed.”);
}
}
catch (Exception ex)
{
Console.WriteLine(“An error occurred while deleting the file: ” + ex.Message);
}
“`
When deleting a file, it is important to handle exceptions that may occur, such as file not found or insufficient permissions. If the file is not found at the specified path, an exception will be thrown. Additionally, if the file does not have sufficient delete permissions, an exception will be raised. By handling these exceptions, you can ensure that the file deletion operation is performed smoothly and gracefully.
FAQs:
Q: Can I use relative file paths instead of absolute file paths in C#?
A: Yes, C# supports both absolute and relative file paths. The choice depends on your requirements and the context in which your application operates. Relative file paths are useful when you want to refer to files relative to the current working directory of your application.
Q: What happens if the destination file already exists when moving or renaming a file?
A: If the destination file already exists, an exception will be thrown. You need to handle this exception and decide how to deal with the conflict. You may choose to overwrite the existing file, prompt the user for a new name, or take any other appropriate action.
Q: How can I check if a file has been successfully moved, renamed, copied, or deleted?
A: After performing file manipulation operations in C#, you can use the File.Exists method to verify if the file still exists at the specified path. If the method returns false, it indicates that the file has been successfully moved, renamed, copied, or deleted.
In conclusion, file manipulation is an important aspect of software development, and C# provides powerful and flexible methods and classes to handle various file-related tasks. Whether you need to move, rename, copy, or delete a file, C# offers simple yet effective ways to accomplish these tasks. By understanding the techniques discussed in this article and handling exceptions appropriately, you can ensure efficient and error-free file manipulation in your C# applications.
Những Ca Khúc Hay Nhất Của Only C
Keywords searched by users: c# move a file
Categories: Top 51 C# Move A File
See more here: nhanvietluanvan.com
Images related to the topic c# move a file
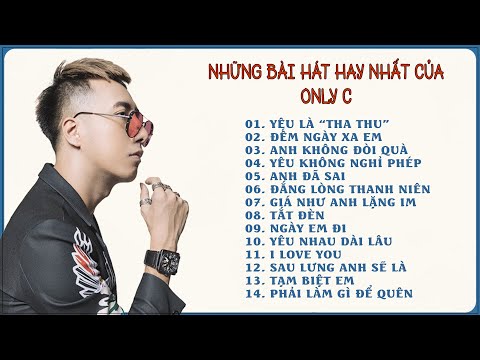
Article link: c# move a file.
Learn more about the topic c# move a file.
- Ngôn ngữ lập trình C là gì? – Tại sao sử dụng C? – VietTuts.Vn
- C cơ bản: Giới thiệu ngôn ngữ C – DevIOT
- Ngôn ngữ lập trình C là gì? Tìm hiểu về ngôn ngữ lập trình C
- C – Wiktionary tiếng Việt
- Miền Bắc tiếp tục nắng nóng gay gắt, nhiệt độ cao nhất 37độ C
- Bài 1: Những khái niệm cơ bản về ngôn ngữ C – Tìm ở đây
- C for Beginners – CodeLearn