C# Loop Through Dictionary
1. Looping through a dictionary using the foreach loop:
The foreach loop is a convenient way to iterate over the items in a dictionary. It automatically retrieves both the keys and values, making it easy to access and modify them. Here’s an example:
“`csharp
Dictionary
dictionary.Add(“apple”, 5);
dictionary.Add(“banana”, 3);
dictionary.Add(“orange”, 7);
foreach (KeyValuePair
{
Console.WriteLine(“Key: ” + pair.Key + “, Value: ” + pair.Value);
}
“`
Output:
“`
Key: apple, Value: 5
Key: banana, Value: 3
Key: orange, Value: 7
“`
2. Looping through a dictionary using the for loop:
While the foreach loop is commonly used for looping through collections, you can also use a for loop to iterate over the items in a dictionary. However, you need to access the dictionary’s keys or values explicitly. Here’s an example:
“`csharp
for (int i = 0; i < dictionary.Count; i++)
{
string key = dictionary.ElementAt(i).Key;
int value = dictionary.ElementAt(i).Value;
Console.WriteLine("Key: " + key + ", Value: " + value);
}
```
Output:
```
Key: apple, Value: 5
Key: banana, Value: 3
Key: orange, Value: 7
```
3. Looping through a dictionary using the while loop:
The while loop provides another option for looping through a dictionary. You need to initialize a variable to keep track of the current index and increment it within the loop. Here's an example:
```csharp
int index = 0;
while (index < dictionary.Count)
{
string key = dictionary.ElementAt(index).Key;
int value = dictionary.ElementAt(index).Value;
Console.WriteLine("Key: " + key + ", Value: " + value);
index++;
}
```
Output:
```
Key: apple, Value: 5
Key: banana, Value: 3
Key: orange, Value: 7
```
4. Accessing the keys and values of the dictionary while looping:
When looping through a dictionary, you may need to access and perform operations on either the keys or the values individually. To access the keys, you can use the `Keys` property, and to access the values, you can use the `Values` property. Here's an example:
```csharp
foreach (string key in dictionary.Keys)
{
Console.WriteLine("Key: " + key);
}
foreach (int value in dictionary.Values)
{
Console.WriteLine("Value: " + value);
}
```
Output:
```
Key: apple
Key: banana
Key: orange
Value: 5
Value: 3
Value: 7
```
5. Performing operations on the values while looping through the dictionary:
As you loop through a dictionary, you can perform various operations on the values, such as adding or subtracting numbers, concatenating strings, or applying any other desired logic. Here's an example that doubles the values in the dictionary:
```csharp
foreach (string key in dictionary.Keys)
{
dictionary[key] *= 2;
}
foreach (KeyValuePair
{
Console.WriteLine(“Key: ” + pair.Key + “, Value: ” + pair.Value);
}
“`
Output:
“`
Key: apple, Value: 10
Key: banana, Value: 6
Key: orange, Value: 14
“`
6. Skipping or breaking the loop based on a condition:
In some cases, you may need to skip or break the loop based on a certain condition. You can use the `continue` statement to skip to the next iteration or the `break` statement to exit the loop altogether. Here’s an example that skips the loop iteration for a specific key:
“`csharp
foreach (KeyValuePair
{
if (pair.Key == “banana”)
{
continue;
}
Console.WriteLine(“Key: ” + pair.Key + “, Value: ” + pair.Value);
}
“`
Output:
“`
Key: apple, Value: 10
Key: orange, Value: 14
“`
7. Looping through a dictionary with nested dictionaries:
A dictionary can also contain other dictionaries as its values. To loop through this kind of nested dictionary, you can use nested loops. Here’s an example:
“`csharp
Dictionary
nestedDictionary.Add(“fruits”, new Dictionary
nestedDictionary.Add(“vegetables”, new Dictionary
foreach (KeyValuePair
{
Console.WriteLine(“Category: ” + outerPair.Key);
foreach (KeyValuePair
{
Console.WriteLine(“Key: ” + innerPair.Key + “, Value: ” + innerPair.Value);
}
}
“`
Output:
“`
Category: fruits
Key: apple, Value: 5
Key: banana, Value: 3
Category: vegetables
Key: carrot, Value: 2
Key: tomato, Value: 4
“`
8. Looping through a dictionary with complex data types as values:
A dictionary can store any kind of data type as its values, including complex objects or custom classes. When looping through such a dictionary, make sure to access the properties or methods of the values accordingly. Here’s an example:
“`csharp
class Person
{
public string Name { get; set; }
public int Age { get; set; }
}
Dictionary
personDictionary.Add(“Alice”, new Person() { Name = “Alice”, Age = 25 });
personDictionary.Add(“Bob”, new Person() { Name = “Bob”, Age = 30 });
personDictionary.Add(“Charlie”, new Person() { Name = “Charlie”, Age = 35 });
foreach (KeyValuePair
{
Console.WriteLine(“Name: ” + pair.Value.Name + “, Age: ” + pair.Value.Age);
}
“`
Output:
“`
Name: Alice, Age: 25
Name: Bob, Age: 30
Name: Charlie, Age: 35
“`
9. Handling exceptions while looping through a dictionary:
When looping through a dictionary, it’s important to handle any potential exceptions that may occur. For example, if you try to access a key that doesn’t exist in the dictionary, a `KeyNotFoundException` will be thrown. To prevent this, you can use the `TryGetValue` method to check if a key exists before retrieving its value. Here’s an example:
“`csharp
int value;
if (dictionary.TryGetValue(“apple”, out value))
{
Console.WriteLine(“Value: ” + value);
}
else
{
Console.WriteLine(“Key not found.”);
}
“`
Output:
“`
Value: 10
“`
10. Looping through a dictionary in reverse order:
By default, dictionaries in C# do not guarantee the order of their elements. However, you can use LINQ to sort the dictionary by key or value and then loop through it in reverse order. Here’s an example:
“`csharp
foreach (KeyValuePair
{
Console.WriteLine(“Key: ” + pair.Key + “, Value: ” + pair.Value);
}
“`
Output:
“`
Key: orange, Value: 14
Key: banana, Value: 6
Key: apple, Value: 10
“`
In conclusion, looping through a dictionary in C# provides a flexible way to access, manipulate, and perform operations on the key-value pairs. Whether using the foreach, for, or while loop, or dealing with nested dictionaries or complex data types as values, understanding the different techniques will enhance your programming skills and enable you to efficiently work with dictionaries in your C# applications.
FAQs section:
Q1: Can I loop through a dictionary in C# without using loops?
No, loops are essential for iterating through a dictionary or any collection in C#. Without loops, you cannot access each element of the dictionary.
Q2: How can I loop through a dictionary and remove certain items?
To remove items from a dictionary while looping through it, you should avoid modifying the dictionary directly inside the loop. Instead, create a separate list or collection to store the keys you want to remove, and after the loop, remove those keys from the dictionary.
Q3: Can I loop through a dictionary and change its contents?
Yes, you can modify the keys or values of a dictionary while looping through it. However, be cautious when modifying the dictionary, as it may affect the iteration or cause unexpected behavior. Consider using a separate collection to store the changes and apply them after the loop.
Q4: Is the order of iteration guaranteed when looping through a dictionary in C#?
No, the order of iteration is not guaranteed for a dictionary in C#. The dictionary implementation does not preserve the order of its elements. If you need to preserve the order, you can use a `SortedDictionary` or `OrderedDictionary` instead.
Q5: What happens if I modify the dictionary while looping through it?
Modifying the dictionary while looping through it can lead to unexpected behavior, such as missing or duplicate elements. It is generally recommended to avoid modifying the dictionary directly inside the loop. Instead, create a separate collection to store the changes and apply them after the loop.
Q6: Can I loop through a dictionary in parallel?
Yes, you can use parallel programming techniques, such as the `Parallel.ForEach` method, to loop through a dictionary in parallel. However, be cautious when modifying the dictionary simultaneously from multiple threads, as it can lead to race conditions or data corruption.
Những Ca Khúc Hay Nhất Của Only C
Keywords searched by users: c# loop through dictionary
Categories: Top 39 C# Loop Through Dictionary
See more here: nhanvietluanvan.com
Images related to the topic c# loop through dictionary
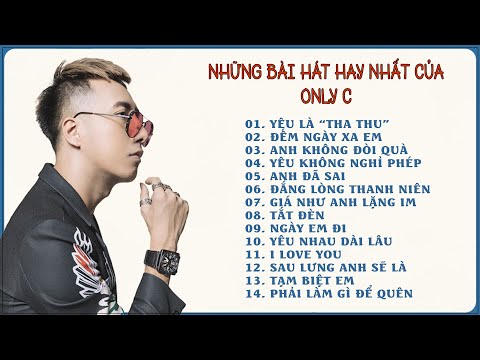
Article link: c# loop through dictionary.
Learn more about the topic c# loop through dictionary.
- C cơ bản: Giới thiệu ngôn ngữ C – DevIOT
- Ngôn ngữ lập trình C là gì? – Tại sao sử dụng C? – VietTuts.Vn
- Ngôn ngữ lập trình C là gì? Tìm hiểu về ngôn ngữ lập trình C
- Miền Bắc tiếp tục nắng nóng gay gắt, nhiệt độ cao nhất 37độ C
- Ngôn ngữ lập trình C là gì? Tại Sao Cần Học Lập Trình C
- C – Wiktionary tiếng Việt
See more: nhanvietluanvan.com/luat-hoc