C# Key Value List
Introduction
In C#, a key value list is a data structure that allows you to store a collection of key-value pairs. This data structure is incredibly useful when you need to associate specific values with unique keys. The keys are used to access and retrieve the corresponding values quickly and efficiently. In this article, we will explore the key value list in C# in depth, covering its creation, access, modification, addition and removal of items, sorting, as well as comparing it with other collection types.
What is a Key Value List in C#?
A key value list, also known as a dictionary or associative array, is a container in C# that stores elements as a collection of key-value pairs. Each key in the list must be unique, and it is used as an identifier to access the corresponding value associated with it. The key-value pairs can be of any valid C# data type, such as integers, strings, objects, etc.
Creating a Key Value List in C#
To create a key value list in C#, you can use the `Dictionary
“`csharp
Dictionary
“`
In the example above, we created a key value list that associates integers with strings as keys. You can replace the data types with any valid C# data types according to your requirements.
Accessing Values in a Key Value List
Accessing values in a key value list is straightforward. You can retrieve the value associated with a particular key by using the key itself. Here’s an example:
“`csharp
int value = keyValueList[“key”];
“`
In the example above, `value` will store the integer value associated with the key “key”. It’s important to note that if the key does not exist in the key value list, a `KeyNotFoundException` will be thrown. Therefore, it is recommended to check if a key exists before retrieving its value using the `ContainsKey` method:
“`csharp
if (keyValueList.ContainsKey(“key”))
{
int value = keyValueList[“key”];
// Do something with the value
}
“`
Modifying Values in a Key Value List
To modify a value in a key value list, you can simply assign a new value to the corresponding key. If the key already exists, its associated value will be updated. Here’s an example:
“`csharp
keyValueList[“key”] = 123;
“`
The value associated with the key “key” has been updated to 123 in the above example.
Adding and Removing Items in a Key Value List
To add items to a key value list, you can use the `Add` method, which takes the key and the corresponding value as parameters. Here’s an example:
“`csharp
keyValueList.Add(“key”, 123);
“`
To remove an item from a key value list, you can use the `Remove` method by specifying the key to be removed. Here’s an example:
“`csharp
keyValueList.Remove(“key”);
“`
Sorting a Key Value List
By default, a key value list does not maintain a specific order of its elements. However, if you need to sort the list based on the keys or values, you can create a sorted key value list using a `SortedDictionary
“`csharp
SortedDictionary
“`
Searching for Values in a Key Value List
To search for values in a key value list, you can use methods like `ContainsKey`, `ContainsValue`, or iterate over the list using a loop. Here’s an example:
“`csharp
if (keyValueList.ContainsValue(123))
{
// Do something
}
“`
Key Value Lists vs. Other Collection Types in C#
Key value lists offer specific advantages and disadvantages compared to other collection types in C#. Here’s a brief comparison:
– Arrays: Key value lists provide more flexibility when it comes to dynamically adding, removing, and modifying elements. Arrays have a fixed size and cannot be easily resized.
– Lists: Key value lists provide a way to associate specific values with unique keys, while lists are mainly used to store a collection of elements without specific associations.
– Sets: Sets ensure unique elements, but they do not allow associating specific values with keys like key value lists do.
– Queues and Stacks: These collection types are designed for specific behaviors (FIFO and LIFO, respectively) and do not provide the key-value association concept.
FAQs (Frequently Asked Questions)
1. Can I use custom objects as keys in a key value list?
Yes, you can use any valid C# data type as keys, including custom objects. However, keep in mind that for custom objects, you need to implement proper equality methods like `GetHashCode` and `Equals` to ensure accurate retrieval of values.
2. What happens if I add a duplicate key to a key value list?
If you attempt to add a duplicate key to a key value list, a `ArgumentException` will be thrown. Remember, keys must be unique.
3. Can I store multiple values with the same key in a key value list?
No, each key in a key value list must be unique. However, you can associate a collection (e.g. a list) with each key if you need to store multiple values.
Conclusion
In this comprehensive guide, we covered the fundamental aspects of a key value list in C#. You now understand how to create, access, modify, add and remove items, sort, and search for values in a key value list. Additionally, you learned about the differences between key value lists and other collection types in C#. By leveraging the power of key value lists, you can efficiently store and retrieve data by associating specific values with unique keys.
Ai Ký Lệnh C..Ấ.. M Quay Video. Không Làm Láo Sao Phải C..Â. M.
Keywords searched by users: c# key value list
Categories: Top 24 C# Key Value List
See more here: nhanvietluanvan.com
Images related to the topic c# key value list
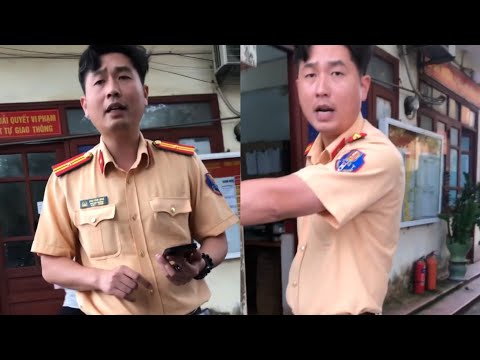
Article link: c# key value list.
Learn more about the topic c# key value list.
- C cơ bản: Giới thiệu ngôn ngữ C – DevIOT
- Ngôn ngữ lập trình C là gì? – Tại sao sử dụng C? – VietTuts.Vn
- Ngôn ngữ lập trình C là gì? Tìm hiểu về ngôn ngữ lập trình C
- C – Wiktionary tiếng Việt
- Bài 1: Những khái niệm cơ bản về ngôn ngữ C – Tìm ở đây
- Ngôn ngữ C là gì? Code ví dụ Hello World – CodeCute
See more: https://nhanvietluanvan.com/luat-hoc/