C# Initialize List With Default Values
C# provides a flexible and convenient way to initialize lists, which are dynamic arrays that can store multiple values of the same type. One common requirement when working with lists is to initialize them with default values. By default, all elements in a list are assigned a default value based on their type. In this article, we will explore various methods to initialize a list with default values in C#.
1. Declaring a List Variable and Assigning Default Values
To declare a list variable and initialize it with default values, you can use the following syntax:
“`csharp
List
“`
In this example, a list variable named “numbers” is declared and initialized with the default values for integers. The list is initially empty, and elements can be added to it later as needed.
2. Initializing a List with Specific Values
If you already know the values you want to include in the list, you can initialize it with specific values at the time of declaration. For example:
“`csharp
List
“`
In this case, a list variable named “fruits” is declared and initialized with three specific string values. You can modify the list as required by adding or removing elements.
3. Initializing a List with a Default Value for Each Element
To initialize a list with a default value for each element, you can use the `Enumerable.Repeat` method from the System.Linq namespace. Here’s an example:
“`csharp
List
“`
In this example, a list variable named “flags” is declared and initialized with five boolean elements, all set to `true`. The `Enumerable.Repeat` method creates an IEnumerable sequence with the specified value repeated a specified number of times, which is then converted into a list using the `ToList` method.
4. Initializing a List with a Default Value Using the Enumerable.Repeat Method
If you want to initialize a list with a single default value for each element, you can use the `Enumerable.Repeat` method again. Here’s an example:
“`csharp
List
“`
In this example, a list variable named “scores” is declared and initialized with ten integer elements, all set to `0`. The `Enumerable.Repeat` method creates an IEnumerable sequence with the specified default value repeated the specified number of times, which is then converted into a list.
5. Initializing a List with Default Values Using Linq and the Enumerable.Range Method
You can also use the `Enumerable.Range` method in combination with Linq to initialize a list with default values. Here’s an example:
“`csharp
List
“`
In this example, a list variable named “numbers” is declared and initialized with ten integer elements, all set to `0`. The `Enumerable.Range` method generates a sequence of integers from the specified start value to the specified end value. Then, the `Select` method is used to assign a default value of `0` to each element, and finally, the sequence is converted into a list.
6. Initializing a List with Default Values Using a For Loop
Another approach to initialize a list with default values is by using a traditional for loop. Here’s an example:
“`csharp
List
for (int i = 0; i < 5; i++)
{
names.Add(string.Empty);
}
```
In this example, a list variable named "names" is declared, and an empty string is added to it five times using a for loop. You can adjust the loop condition depending on the desired number of elements and modify the default value as needed.
7. Initializing a List with Default Values Using a Foreach Loop
You can also utilize a foreach loop to initialize a list with default values. Here's an example:
```csharp
List
foreach (var _ in Enumerable.Range(0, 5))
{
values.Add(0);
}
“`
In this example, a list variable named “values” is declared, and an integer value of `0` is added to it five times using a foreach loop. The `Enumerable.Range` method is used to generate a sequence of integers, and the loop iterates over this range without using the iteration variable (`_`). This approach is useful when you don’t need to access the iteration variable within the loop body.
8. Initializing a List with Default Values Using a Lambda Expression
If you prefer a more compact syntax, you can use a lambda expression in combination with the `Enumerable.Range` method to initialize a list with default values. Here’s an example:
“`csharp
List
“`
In this example, a list variable named “flags” is declared and initialized with five boolean elements, all set to `true`. The `Enumerable.Select` method projects each element of the source sequence to a new value using the provided lambda expression (`_ => true`), and the resulting sequence is then converted into a list.
Best Practices for Initializing Lists with Default Values in C#
– Consider using the `Enumerable.Range` method in combination with other Linq methods (e.g., `Select`) to initialize lists with default values succinctly.
– Use the `Enumerable.Repeat` method when you need to repeat a specific value multiple times.
– If you know the specific values to be included in the list, initialize it directly with those values at the time of declaration.
– Unless you have a specific requirement, prefer initializing lists directly using initialization syntax or Linq methods instead of manually iterating with loops.
FAQs
Q: Can I initialize a list with default values of a complex type?
A: Yes, you can initialize a list with default values of complex types, such as custom classes or structures. The default values will be assigned based on the default constructor or initialization block of the complex type.
Q: How can I initialize a list with default values of a reference type?
A: If you want to initialize a list with default values of a reference type, such as strings or custom objects, you can simply use the respective default constructor or initialization block to set the default values.
Q: Can I initialize a list with default values of a value type other than the default one?
A: Yes, you can specify any valid default value for a value type while initializing a list. You can use the appropriate default constructor of the value type or assign the desired default value explicitly.
Q: What if I want to initialize a list with default values for only a subset of elements?
A: In such cases, you can initialize the list with default values using one of the methods mentioned earlier and then modify specific elements individually afterward.
Q: Is there any performance difference between different methods of initializing a list with default values?
A: The performance difference between different initialization methods is generally negligible for small-sized lists. However, for larger lists, using Linq methods might incur slightly higher overhead due to the extra processing involved. It is recommended to benchmark and profile your specific use cases to determine any significant impact.
In conclusion, initializing a list with default values in C# is a common task that can be accomplished using various approaches. Whether you prefer using Linq methods, for loops, or initialization syntax, you can choose the method that best suits your requirements and coding style. By understanding the basics and applying the best practices mentioned in this article, you can efficiently initialize lists with default values in your C# programs.
Ai Ký Lệnh C..Ấ.. M Quay Video. Không Làm Láo Sao Phải C..Â. M.
Keywords searched by users: c# initialize list with default values
Categories: Top 57 C# Initialize List With Default Values
See more here: nhanvietluanvan.com
Images related to the topic c# initialize list with default values
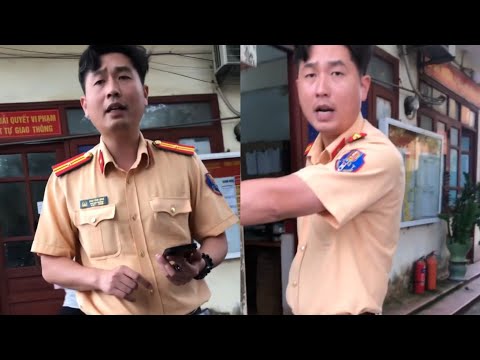
Article link: c# initialize list with default values.
Learn more about the topic c# initialize list with default values.
- C cơ bản: Giới thiệu ngôn ngữ C – DevIOT
- Ngôn ngữ lập trình C là gì? – Tại sao sử dụng C? – VietTuts.Vn
- Ngôn ngữ lập trình C là gì? Tìm hiểu về ngôn ngữ lập trình C
- C – Wiktionary tiếng Việt
- Bài 1: Những khái niệm cơ bản về ngôn ngữ C – Tìm ở đây
- Ngôn ngữ lập trình C là gì? Tại Sao Cần Học Lập Trình C
See more: https://nhanvietluanvan.com/luat-hoc/