C# How To Write To A Text File
In C#, writing data to a text file is a common task that developers often encounter. Whether you need to save configuration settings, log information, or any other type of data, writing to a text file can be a straightforward and efficient solution. This article will guide you through the process of writing to a text file in C#, covering various scenarios and best practices.
Choosing the Appropriate File Access Mode
Before proceeding with writing to a text file, it is essential to choose the appropriate file access mode. There are three main modes available:
1. FileMode.Create: This mode creates a new file. If the file already exists, it will be overwritten.
2. FileMode.Append: This mode appends data to an existing file. If the file does not exist, it will be created.
3. FileMode.OpenOrCreate: This mode either opens an existing file or creates a new one if it doesn’t exist.
Opening a Text File in C#
To open a text file for writing, you need to use the StreamWriter class from the System.IO namespace. Here’s an example of opening a text file in C#:
“`csharp
using System.IO;
string filePath = “path/to/your/file.txt”;
StreamWriter writer = new StreamWriter(filePath);
“`
In the above code snippet, we provided the file path to the constructor of the StreamWriter class to open the file for writing.
Writing Text to a File Using the StreamWriter Class
Once the file is opened, you can write text content to it using the StreamWriter.WriteLine or StreamWriter.Write methods. The WriteLine method writes a line of text to the file and appends a newline character at the end. The Write method, on the other hand, writes text without appending a newline character. Here’s an example:
“`csharp
writer.WriteLine(“Hello, World!”);
writer.Write(“This is a sample text.”);
“`
In the above example, the text “Hello, World!” is written to the file as a line, followed by the text “This is a sample text.” on the same line.
Writing String Arrays to a Text File
If you have an array of strings that you want to write to a text file, you can use the StreamWriter.WriteLine or StreamWriter.Write methods in a loop. Here’s an example:
“`csharp
string[] lines = { “Line 1”, “Line 2”, “Line 3” };
foreach (string line in lines)
{
writer.WriteLine(line);
}
“`
In this example, each element of the `lines` array is written to a separate line in the text file.
Appending Text to an Existing File
To append additional text to an existing file instead of overwriting it, you need to pass FileMode.Append as the second argument to the StreamWriter constructor. Here’s an example:
“`csharp
StreamWriter writer = new StreamWriter(filePath, append: true);
“`
With this modification, any content written to the file will be appended at the end, without erasing the existing content.
Handling Exceptions While Writing to a Text File
When writing to a text file, it’s crucial to handle any exceptions that may occur. Failure to handle exceptions can result in unexpected program crashes or data loss. To handle exceptions, surround your file writing code with a try-catch block. Here’s an example:
“`csharp
try
{
// Code for writing to a file
}
catch (IOException ex)
{
// Handle IO exceptions
Console.WriteLine(“An error occurred while writing to the file: ” + ex.Message);
}
catch (Exception ex)
{
// Handle other exceptions
Console.WriteLine(“An error occurred: ” + ex.Message);
}
finally
{
// Close the file
writer.Close();
}
“`
In this example, the code attempts to write to the file, and if an IO exception occurs, it is caught and handled appropriately. The `finally` block ensures that the file is closed, regardless of whether an exception occurred or not.
Closing the File After Writing is Complete
After finishing writing to a text file, it is important to close the file handle to release system resources properly. You can call the Close method on the StreamWriter object to close the file. Here’s an example:
“`csharp
writer.Close();
“`
Closing the file guarantees that any changes you made are saved, and the file handle is freed up.
Best Practices for Writing to a Text File in C#
Here are some best practices to keep in mind when writing to a text file in C#:
1. Use the using statement: The using statement automatically disposes of the StreamWriter object, ensuring proper resource management. Example:
“`csharp
using (StreamWriter writer = new StreamWriter(filePath))
{
// Code for writing to the file
}
“`
2. Properly handle exceptions: As mentioned earlier, handling exceptions is critical to maintain the stability and reliability of your application.
3. Consider performance: If you’re writing a large amount of data to a file, it might be more efficient to use the FileStream class directly instead of StreamWriter.
4. Use relative file paths: Whenever possible, use relative file paths instead of absolute paths to make your code more portable and flexible.
5. Name your files descriptively: Choose meaningful names for your text files to improve code maintainability and readability.
6. Follow coding standards: Adhere to coding standards and practices to ensure your code is clean, well-organized, and easy for others to understand and maintain.
Frequently Asked Questions (FAQs)
Q: How do I write to a text file in C#?
A: To write to a text file in C#, you can use the StreamWriter class from the System.IO namespace. Open the file using the appropriate file access mode, and then use the StreamWriter.WriteLine or StreamWriter.Write methods to write text content to the file.
Q: How do I append text to an existing text file in C#?
A: To append text to an existing text file in C#, pass FileMode.Append as the second argument to the StreamWriter constructor. This ensures that the new content is added to the end of the file without erasing the existing content.
Q: What happens if an exception occurs while writing to a text file?
A: If an exception occurs while writing to a text file, it is essential to handle the exception appropriately to prevent unexpected crashes or data loss. Surround your file writing code with a try-catch block and handle the exceptions accordingly.
Q: Should I close the file after writing is complete?
A: Yes, it is crucial to close the file after writing is complete to release system resources properly and ensure that any changes you made are saved. Close the file by calling the Close method on the StreamWriter object.
In conclusion, writing to a text file in C# is a fundamental task that developers often encounter. By following the guidelines and best practices outlined in this article, you can efficiently write data to text files and handle potential errors effectively.
Beach Song + More Nursery Rhymes \U0026 Kids Songs – Cocomelon
Keywords searched by users: c# how to write to a text file
Categories: Top 37 C# How To Write To A Text File
See more here: nhanvietluanvan.com
Images related to the topic c# how to write to a text file
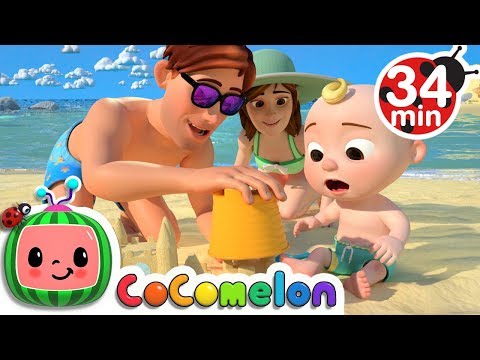
Article link: c# how to write to a text file.
Learn more about the topic c# how to write to a text file.
- C cơ bản: Giới thiệu ngôn ngữ C – DevIOT
- Ngôn ngữ lập trình C là gì? – Tại sao sử dụng C? – VietTuts.Vn
- Bài 1: Những khái niệm cơ bản về ngôn ngữ C – Tìm ở đây
- Ngôn ngữ lập trình C là gì? Tìm hiểu về ngôn ngữ lập trình C
- C – Wiktionary tiếng Việt
- C for Beginners – CodeLearn
See more: https://nhanvietluanvan.com/luat-hoc/