C# How To Read A Text File
Opening the Text File
Reading and processing text files is a common task in programming, and C# provides several methods to accomplish this. The first step is to open the text file using the FileStream class. This class provides methods to create, read, and write to files. To open a text file, you can use the StreamReader class, which is specifically designed to read text from files efficiently.
To open a text file in C#, you can follow these steps:
1. Import the necessary namespace at the beginning of your code:
“`csharp
using System.IO;
“`
2. Create an instance of the StreamReader class and provide the path to your text file as a parameter:
“`csharp
StreamReader reader = new StreamReader(“path/to/your/file.txt”);
“`
3. Once you have opened the file, you can start reading its contents.
Reading the File Line by Line
Reading a text file line by line allows you to process each line individually, which is helpful for tasks such as parsing, filtering, or transforming the text. C# provides a convenient method called ReadLine() in the StreamReader class to read each line sequentially.
To read a text file line by line, use the following code:
“`csharp
string line;
while ((line = reader.ReadLine()) != null)
{
// Process the line here
}
“`
The code above reads each line from the file until there are no more lines, storing each line in the `line` variable. You can replace the comment with your desired actions.
Splitting Each Line into Words
Once you have read a line from the text file, you might need to split it into individual words for further processing. C# provides the Split() method, which can split a string into an array of substrings based on a specified separator.
Here’s an example of splitting each line into words:
“`csharp
string[] words = line.Split(‘ ‘);
“`
In the above code, we use a space character as the separator, assuming that words in the text file are separated by spaces. You can replace the space character with any other separator, such as commas or tabs.
Storing the Words in a List or Array
To store the split words for later use, you can choose between a list (`List
Using a List:
“`csharp
List
“`
Using an Array:
“`csharp
string[] wordArray = words;
“`
Ignoring Empty Lines and Comments
It’s common for text files to contain empty lines or lines starting with comments that need to be ignored during processing. You can use various techniques to handle these scenarios.
Ignoring Empty Lines:
“`csharp
if (string.IsNullOrWhiteSpace(line))
{
continue;
}
“`
Ignoring Comments:
“`csharp
if (line.StartsWith(“//”) || line.StartsWith(“#”))
{
continue;
}
“`
In the above code snippets, the `continue` statement skips the current iteration of the loop, allowing you to move to the next line without processing the empty line or comment.
Removing Leading and Trailing Whitespace from Each Word
Text files often contain leading or trailing whitespace around words. To remove this whitespace, you can use the Trim() method, which eliminates leading and trailing spaces from a string.
Here’s an example of removing whitespace from each word:
“`csharp
for (int i = 0; i < words.Length; i++)
{
words[i] = words[i].Trim();
}
```
The code above iterates through the array of words and trims each word individually.
Dealing with Punctuation Marks and Special Characters
Text files may contain punctuation marks or special characters that you may want to exclude or handle separately. C# provides several methods and libraries to assist in dealing with such characters.
To remove punctuation marks from a word, you can use the Regex class in conjunction with the Replace() method:
```csharp
using System.Text.RegularExpressions;
string wordWithoutPunctuation = Regex.Replace(word, @"[\p{P}-[.]]+", string.Empty);
```
In the above code, we use a regular expression pattern to match any Unicode punctuation character except a period (dot). The Replace() method then replaces the matched characters with an empty string.
Ignoring Case Sensitivity
Sometimes it's necessary to make text processing case-insensitive, treating uppercase and lowercase letters as equal. To achieve this, you can use the ToLower() or ToUpper() methods to convert all characters in a word to lowercase or uppercase, respectively.
Here's an example of making the text processing case-insensitive:
```csharp
string wordLowerCase = word.ToLower();
```
Performing Text Processing Tasks on the Words
After reading and splitting the text file into words, you can perform a variety of text processing tasks on the words, such as counting occurrences or finding patterns. The specific tasks you want to accomplish may influence how you store and manipulate the words.
Counting Occurrences:
```csharp
Dictionary
foreach (string word in words)
{
if (wordOccurrences.ContainsKey(word))
{
wordOccurrences[word]++;
}
else
{
wordOccurrences[word] = 1;
}
}
“`
In this example, we use a dictionary (`Dictionary
Finding Patterns:
“`csharp
foreach (string word in words)
{
if (word.StartsWith(“abc”))
{
// Pattern matched, do something
}
}
“`
Closing the Text File
After you have finished reading and processing the text file, it’s essential to close it properly to release system resources. To close the text file, simply call the Close() method on the StreamReader object:
“`csharp
reader.Close();
“`
By closing the file, you ensure that it can be accessed by other processes and no longer consumes system resources.
FAQs
Q: Can I read a text file using relative paths?
A: Yes, C# supports relative paths to access text files. Simply provide the relative path to the file instead of the absolute path.
Q: How do I handle exceptions when reading a text file?
A: When working with files, reading operations may encounter errors such as a non-existing file or insufficient permissions. You can handle these exceptions using try-catch blocks and display appropriate error messages to the user.
Q: Can I read a large text file in C#?
A: Yes, C# provides methods to read text files of any size. However, for very large files, you may want to consider using techniques like memory mapping or stream reading to process the file efficiently.
Q: How can I read a text file asynchronously in C#?
A: C# provides the async/await pattern to perform asynchronous operations. You can use the StreamReader’s asynchronous methods, such as ReadLineAsync(), to read a text file asynchronously.
Q: Are there any libraries that make text file processing easier in C#?
A: Yes, C# has several libraries, such as CSVHelper and OpenNLP, that provide additional functionalities for reading and processing text files. These libraries can simplify complex tasks like parsing CSV files or performing natural language processing.
In conclusion, reading a text file in C# is a fundamental skill for developers dealing with textual data. By following the steps outlined in this guide, you can efficiently open a text file, read its contents line by line while performing various text processing tasks, and close the file properly to release system resources. Remember to handle different scenarios, such as empty lines, comments, and punctuation marks, based on your specific requirements.
Ai Ký Lệnh C..Ấ.. M Quay Video. Không Làm Láo Sao Phải C..Â. M.
Keywords searched by users: c# how to read a text file
Categories: Top 73 C# How To Read A Text File
See more here: nhanvietluanvan.com
Images related to the topic c# how to read a text file
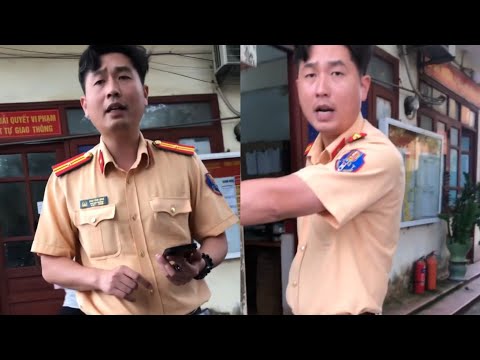
Article link: c# how to read a text file.
Learn more about the topic c# how to read a text file.
- C cơ bản: Giới thiệu ngôn ngữ C – DevIOT
- Ngôn ngữ lập trình C là gì? – Tại sao sử dụng C? – VietTuts.Vn
- Ngôn ngữ lập trình C là gì? Tìm hiểu về ngôn ngữ lập trình C
- C – Wiktionary tiếng Việt
- Bài 1: Những khái niệm cơ bản về ngôn ngữ C – Tìm ở đây
- Ngôn ngữ lập trình C là gì? Tại Sao Cần Học Lập Trình C
See more: https://nhanvietluanvan.com/luat-hoc/