C# Execute Multiple Sql Commands In One Transaction
In a database management system, executing multiple SQL commands in one transaction is a common practice when dealing with complex operations. The ability to group these commands within a single transaction ensures data integrity and allows for easy rollback in case of any errors. In this article, we will explore how to execute multiple SQL commands in one transaction using C#. We will walk through the process step by step and discuss the benefits of this approach.
Create a Connection to the Database
The first step in executing multiple SQL commands in one transaction is establishing a connection to the database. In C#, you can achieve this by using the SqlConnection class from the System.Data.SqlClient namespace. This class provides various properties and methods to interact with the database. Here’s an example of creating a connection:
“`csharp
string connectionString = “Your_Connection_String”;
using (SqlConnection connection = new SqlConnection(connectionString))
{
// Connection logic goes here
}
“`
Replace “Your_Connection_String” with the actual connection string for your database. The ‘using’ statement is used here to ensure that the connection is automatically closed and disposed of when it goes out of scope.
Create a Transaction object
The next step is to create a Transaction object. The Transaction class is part of the System.Data.SqlClient namespace and allows you to group multiple SQL commands as a single unit of work. Here’s how you can create a transaction:
“`csharp
using (SqlConnection connection = new SqlConnection(connectionString))
{
connection.Open();
SqlTransaction transaction = connection.BeginTransaction();
// Transaction logic goes here
}
“`
The ‘BeginTransaction’ method is used to start a new transaction. It takes an optional parameter that allows you to specify the transaction isolation level.
Open the Connection to the Database
Once the transaction is created, the next step is to open the connection to the database using the ‘Open’ method of the SqlConnection object:
“`csharp
using (SqlConnection connection = new SqlConnection(connectionString))
{
connection.Open();
SqlTransaction transaction = connection.BeginTransaction();
// Database logic goes here
}
“`
Begin the Transaction
After the connection is opened, you can begin the transaction by calling the ‘BeginTransaction’ method on the connection object. This starts the transaction and associates it with the current connection. Here’s the updated code:
“`csharp
using (SqlConnection connection = new SqlConnection(connectionString))
{
connection.Open();
SqlTransaction transaction = connection.BeginTransaction();
// Transaction logic goes here
}
“`
Create and Execute First SQL Command
Now that the transaction is in progress, you can create and execute the SQL commands using SqlCommand objects. These commands can include INSERT, UPDATE, DELETE, or any other valid SQL statement. Here’s an example:
“`csharp
using (SqlConnection connection = new SqlConnection(connectionString))
{
connection.Open();
SqlTransaction transaction = connection.BeginTransaction();
try
{
string sql1 = “INSERT INTO Table1 (Column1, Column2) VALUES (@value1, @value2)”;
SqlCommand command1 = new SqlCommand(sql1, connection, transaction);
command1.Parameters.AddWithValue(“@value1”, “Value1”);
command1.Parameters.AddWithValue(“@value2”, “Value2”);
command1.ExecuteNonQuery();
// Execute additional SQL commands
transaction.Commit();
}
catch (Exception ex)
{
transaction.Rollback();
// Handle exception
}
}
“`
Create and Execute Second SQL Command
To include multiple SQL commands, you can repeat the process of creating SqlCommand objects and executing them. Make sure to associate each command with the same connection and transaction objects. Here’s an example of adding a second SQL command:
“`csharp
string sql2 = “UPDATE Table1 SET Column1 = ‘Value3’ WHERE Column2 = ‘Value2′”;
SqlCommand command2 = new SqlCommand(sql2, connection, transaction);
command2.ExecuteNonQuery();
“`
Commit the Transaction
After executing all the necessary SQL commands, you can commit the transaction using the ‘Commit’ method of the SqlTransaction object. This confirms the changes made by the transaction and makes them permanent in the database.
“`csharp
transaction.Commit();
“`
Close the Connection
Once the transaction is committed or rolled back, it is essential to close the connection to release any resources associated with it. You can call the ‘Close’ method on the SqlConnection object to achieve this.
“`csharp
connection.Close();
“`
Handle Exceptions and Rollback the Transaction
In case an exception occurs during the execution of SQL commands, it is crucial to handle it appropriately and roll back the transaction to maintain data integrity. This can be done within a try-catch block, as shown in the previous code snippets. The ‘Rollback’ method of the SqlTransaction object is used to undo the changes made by the transaction.
“`csharp
catch (Exception ex)
{
transaction.Rollback();
// Handle exception
}
“`
Benefits of Executing Multiple SQL Commands in One Transaction
Executing multiple SQL commands in one transaction provides several benefits:
1. Atomicity: By grouping related commands in a single transaction, you ensure that either all the changes are committed together or none of them are. This guarantees that the data remains in a consistent state.
2. Data Integrity: Transactions provide isolation and integrity to the database. When executing multiple SQL commands, it is critical to maintain data integrity across tables and relationships. Using a transaction ensures that these changes are made atomically, preventing partial updates that can lead to data inconsistencies.
3. Performance: When executing multiple SQL commands individually, each command results in a round trip to the database server. By grouping these commands in one transaction, you reduce the number of round trips, leading to improved performance.
4. Rollback Capability: In case of any error during the execution of SQL commands, you can easily roll back the entire transaction, undoing all the changes made. This helps maintain data consistency and allows for error handling and recovery.
In conclusion, executing multiple SQL commands in one transaction using C# is a powerful technique for ensuring data integrity and facilitating error handling. By following the steps outlined in this article, you can effectively group related SQL commands and manage them as a single unit of work. Remember to open and close the connection, begin the transaction, execute the commands, and handle exceptions to maintain a reliable database system.
FAQs:
1. Can I execute different types of SQL commands within the same transaction?
Yes, you can execute any valid SQL command within the same transaction. Whether it’s INSERT, UPDATE, DELETE, or any other SQL statement, as long as it is supported by your database server, you can include it in the transaction.
2. Can I include multiple transactions within a single transaction?
No, a transaction cannot be nested within another transaction. Each transaction forms an independent unit of work. However, you can create a savepoint within a transaction to roll back to a specific point if needed.
3. Can I execute multiple transactions simultaneously?
While it is possible to execute multiple transactions simultaneously, it can lead to concurrency issues and impact database performance. It is generally recommended to execute transactions sequentially to maintain data integrity and avoid conflicts.
4. Should I always use transactions for executing multiple SQL commands?
Transactions are best suited for scenarios where data integrity is critical, such as inserting, updating, or deleting related records across multiple tables. If you’re executing isolated statements that don’t rely on each other, using transactions may not be necessary.
5. Can I use C# to execute multiple SQL commands in one transaction across different databases?
Yes, you can use C# to execute multiple SQL commands in one transaction, even if they target different databases. Simply create separate connections to each database and associate them with the same transaction object to ensure atomicity.
Remember that when executing multiple SQL commands in one transaction, always aim for a balance between data integrity, performance, and the specific requirements of your application.
Ai Ký Lệnh C..Ấ.. M Quay Video. Không Làm Láo Sao Phải C..Â. M.
Keywords searched by users: c# execute multiple sql commands in one transaction
Categories: Top 29 C# Execute Multiple Sql Commands In One Transaction
See more here: nhanvietluanvan.com
Images related to the topic c# execute multiple sql commands in one transaction
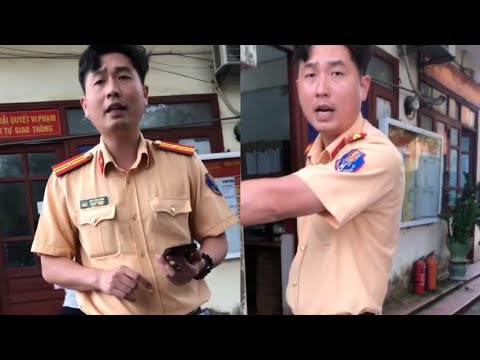
Article link: c# execute multiple sql commands in one transaction.
Learn more about the topic c# execute multiple sql commands in one transaction.
- C cơ bản: Giới thiệu ngôn ngữ C – DevIOT
- Ngôn ngữ lập trình C là gì? – Tại sao sử dụng C? – VietTuts.Vn
- Ngôn ngữ lập trình C là gì? Tìm hiểu về ngôn ngữ lập trình C
- C – Wiktionary tiếng Việt
- Bài 1: Những khái niệm cơ bản về ngôn ngữ C – Tìm ở đây
- Ngôn ngữ lập trình C là gì? Tại Sao Cần Học Lập Trình C
See more: https://nhanvietluanvan.com/luat-hoc/