C# Enum To Int
Introduction:
In the world of programming, enumerations (enums) and integers are essential data types used to represent values. Enums are user-defined types that allow developers to declare a set of named constants. On the other hand, integers are numeric data types used to store whole numbers. In this article, we will explore the concept of converting enums to their corresponding integer representations in C#.
Creating an Enumeration in C#:
To begin our discussion, let’s first understand how to create an enumeration in C#. Enumerations provide a way to define a discrete set of values that a variable can hold. Here’s the syntax for creating an enum:
“`csharp
enum Days
{
Monday,
Tuesday,
Wednesday,
Thursday,
Friday,
Saturday,
Sunday
}
“`
In the example above, we declare an enum called “Days” and list the possible values it can have. By default, the values are assigned consecutive integer numbers starting from zero. For instance, Monday is assigned the value 0, Tuesday 1, and so on.
Assigning Integer Values to Enum Members in C#:
In certain cases, you may want to assign specific integer values to enum members. This can be accomplished by explicitly specifying the values during enum declaration. Consider the following example:
“`csharp
enum Colors
{
Red = 1,
Green = 2,
Blue = 4
}
“`
In the example above, we create an enum called “Colors” and assign values 1, 2, and 4 respectively to the members Red, Green, and Blue. It’s important to note that the assigned values don’t have to be consecutive or start from zero. This flexibility allows developers to assign meaningful or specific values to enum members.
Converting an Enum Value to Its Corresponding Integer Representation in C#:
At times, you may need to convert an enum value to its corresponding integer representation. This can be achieved using the explicit casting operator or the Convert class provided by C#. Here’s an example of converting an enum value to an integer using explicit casting:
“`csharp
Days today = Days.Wednesday;
int todayValue = (int)today;
Console.WriteLine(todayValue); // Output: 2
“`
In the example above, we assign the enum value Wednesday to the variable “today” and then explicitly cast it to an integer using `(int)today`. The result, 2, is stored in the variable “todayValue.” By casting an enum to an integer, we can easily perform various operations on the enum value, such as arithmetic calculations or conditional checks.
Using the Convert Class to Convert an Enum to an Integer in C#:
C# provides the Convert class, which offers a range of methods for converting data types. To convert an enum to an integer using the Convert class, we can utilize the `Convert.ToInt32` method. Here’s an example:
“`csharp
Days today = Days.Wednesday;
int todayValue = Convert.ToInt32(today);
Console.WriteLine(todayValue); // Output: 2
“`
In this example, we assign the enum value Wednesday to the variable “today” and then use `Convert.ToInt32(today)` to convert it to an integer. The result is stored in the variable “todayValue.” By employing the Convert class, we can achieve the same outcome as explicit casting, providing developers with flexibility in choosing their preferred conversion method.
Converting an Integer to Its Equivalent Enum Value in C#:
Now that we’ve explored how to convert an enum to an integer, let’s discuss the process of converting an integer to its equivalent enum value. In C#, this conversion can be performed using the `Enum.ToObject` method followed by casting to the desired enum type. Here’s an example:
“`csharp
int todayValue = 2;
Days today = (Days)Enum.ToObject(typeof(Days), todayValue);
Console.WriteLine(today); // Output: Wednesday
“`
In the example above, we have an integer value, 2, which represents Wednesday. Using `Enum.ToObject(typeof(Days), todayValue)`, we convert the integer to an enum object of the “Days” type. Finally, we cast the enum object to the “Days” enum, which assigns the correct enum value to the variable “today.” This way, we can easily convert an integer to its corresponding enum value.
Handling Invalid Enum Conversions in C#:
While converting enums to integers and vice versa is a common practice in programming, it’s important to handle cases when the conversion may not be valid. For example, converting an integer value that does not correspond to any enum member can result in unexpected behavior or even cause runtime errors.
To handle invalid enum conversions, you can use the `Enum.IsDefined` method to check whether a given value is a valid member of the enum. Here’s an example:
“`csharp
int invalidValue = 10;
if (Enum.IsDefined(typeof(Days), invalidValue))
{
Days invalidDay = (Days)invalidValue;
Console.WriteLine(invalidDay);
}
else
{
Console.WriteLine(“Invalid enum value.”);
}
// Output: Invalid enum value.
“`
In the example above, we try to convert the integer value 10 to an enum value using `(Days)invalidValue`. However, since 10 is not a valid member of the enum, the `Enum.IsDefined(typeof(Days), invalidValue)` check fails, and we print the message “Invalid enum value.”
FAQs:
Q1. Why do we need to convert an enum to an integer?
Converting an enum to an integer is often required to perform arithmetic calculations, store enum values in databases or files, compare enum values, or pass enum values between different parts of a program.
Q2. Can we implicitly convert an enum to an integer?
No, enum to integer conversions require explicit casting or using the Convert class. This prevents potential data loss or unexpected behavior that could arise from implicit conversions.
Q3. Are there any limitations on assigning values to enum members?
Enum members can be assigned any integer value as long as it is within the range of the underlying data type, which is usually an integer. However, it’s recommended to choose values that have logical or meaningful significance to simplify code readability.
Q4. Can we convert an integer value directly to an enum without checking for validity?
While it’s possible to directly cast an integer to an enum without checking validity, it’s considered good practice to validate the value first using the `Enum.IsDefined` method to avoid runtime errors or unintended behavior.
Conclusion:
In this article, we have explored the concept of converting enums to their corresponding integer representations in C#. We learned how to create enumerations, assign integer values to enum members, convert enums to integers, and vice versa. Additionally, we discussed handling invalid enum conversions. Understanding the conversion mechanisms between enums and integers is crucial for effective programming and allows for greater flexibility in manipulating data.
Ai Ký Lệnh C..Ấ.. M Quay Video. Không Làm Láo Sao Phải C..Â. M.
Keywords searched by users: c# enum to int
Categories: Top 100 C# Enum To Int
See more here: nhanvietluanvan.com
Images related to the topic c# enum to int
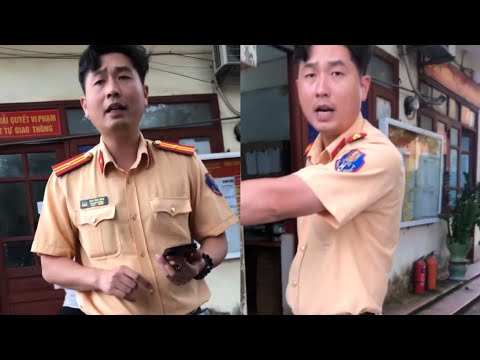
Article link: c# enum to int.
Learn more about the topic c# enum to int.
- C cơ bản: Giới thiệu ngôn ngữ C – DevIOT
- Ngôn ngữ lập trình C là gì? – Tại sao sử dụng C? – VietTuts.Vn
- Ngôn ngữ lập trình C là gì? Tìm hiểu về ngôn ngữ lập trình C
- C – Wiktionary tiếng Việt
- Ngôn ngữ lập trình C là gì? Tại Sao Cần Học Lập Trình C
- Bài 1: Những khái niệm cơ bản về ngôn ngữ C – Tìm ở đây
See more: https://nhanvietluanvan.com/luat-hoc/