C# Dictionary Get Key From Value
Introduction:
C# is a powerful programming language that provides various data structures to store and manipulate data efficiently. One such data structure is the dictionary, which allows developers to store key-value pairs. While retrieving the value of a key is straightforward, getting the key from a value in a C# dictionary can be a bit more challenging. In this article, we will delve into the various methods and techniques available to accomplish this task, along with examples and FAQs to provide a comprehensive understanding of the topic.
Overview of C# Dictionary and Key-Value Pairs:
In C#, a dictionary is a collection type that stores a set of unique keys and their corresponding values. It provides fast retrieval of values based on their associated keys, making it an efficient choice for many programming scenarios. Each key-value pair stored in a dictionary is unique, ensuring that duplicate keys cannot exist. The dictionary itself uses hashing algorithms to organize data, enabling quick access and retrieval.
Understanding the Key-Value Pair Concept in C# Dictionaries:
Before we dive into retrieving a key from a value in a C# dictionary, it’s important to grasp the concept of key-value pairs. A key is a unique identifier associated with a value, allowing us to locate and retrieve the value quickly. The value, on the other hand, represents the data or information associated with the given key.
Accessing the Value of a Key in a C# Dictionary:
To access the value of a key in a C# dictionary, we can use the square bracket notation ([]). For example:
“`csharp
Dictionary
myDictionary.Add(“apple”, 10);
myDictionary.Add(“banana”, 5);
int appleValue = myDictionary[“apple”]; // Accessing the value of the key “apple”
Console.WriteLine(appleValue); // Output: 10
“`
Retrieving a Key from a Value in a C# Dictionary:
Retrieving a key from a value in a C# dictionary is not as straightforward as getting the value from a key. By design, dictionaries are optimized for value retrieval based on keys rather than the other way around. Despite this, there are several techniques available to achieve this goal.
Using LINQ to Extract the Key from a Value in a C# Dictionary:
One of the common methods to retrieve a key from a value in a C# dictionary is by using LINQ (Language-Integrated Query). With LINQ, we can query the dictionary and filter based on the desired value. Here’s an example:
“`csharp
Dictionary
myDictionary.Add(“apple”, 10);
myDictionary.Add(“banana”, 5);
string key = myDictionary.FirstOrDefault(x => x.Value == 5).Key; // Filtering based on value 5
Console.WriteLine(key); // Output: banana
“`
Handling Multiple Keys with the Same Value in a C# Dictionary:
If a dictionary contains multiple keys with the same value, the LINQ approach mentioned above will only return the first key found. To retrieve all keys associated with a specific value, we can iterate over the key-value pairs and store the keys that match the desired value. Here’s an example:
“`csharp
Dictionary
myDictionary.Add(“apple”, 10);
myDictionary.Add(“banana”, 5);
myDictionary.Add(“cherry”, 5);
List
foreach (var pair in myDictionary)
{
if (pair.Value == 5)
keys.Add(pair.Key);
}
foreach (var key in keys)
{
Console.WriteLine(key);
}
// Output:
// banana
// cherry
“`
Iterating over Key-Value Pairs to Find a Value’s Key in a C# Dictionary:
Another approach to finding the key associated with a particular value is to iterate over all the key-value pairs in the dictionary. By comparing the values, we can determine the corresponding key. Here’s an example:
“`csharp
Dictionary
myDictionary.Add(“apple”, 10);
myDictionary.Add(“banana”, 5);
string searchValue = “5”;
string resultKey = null;
foreach (var pair in myDictionary)
{
if (pair.Value.ToString() == searchValue)
{
resultKey = pair.Key;
break; // Exit the loop once the key is found
}
}
Console.WriteLine(resultKey); // Output: banana (if search value is “5”)
“`
FAQs:
Q1: Can a C# dictionary have duplicate values?
A1: No, a C# dictionary ensures that each key is unique and maps to a single value. Duplicate keys are not allowed.
Q2: What happens if we try to retrieve a value using a non-existent key?
A2: If we attempt to access a non-existent key, a KeyNotFoundException will be thrown. It is recommended to check for key existence using the ContainsKey method before accessing the value.
Q3: Can values be of any data type in a C# dictionary?
A3: Yes, values in a C# dictionary can be of any data type. Dictionaries are generic, allowing for flexibility in storing different types of values based on the provided type parameters.
Conclusion:
Retrieving the key from a value in a C# dictionary opens up new possibilities and facilitates efficient programming. Although C# dictionaries are primarily optimized for value retrieval based on keys, the techniques outlined in this article enable developers to achieve the desired functionality. By utilizing LINQ, iterating over key-value pairs, and handling scenarios with multiple keys having the same value, developers can access keys associated with specific values within a C# dictionary effectively.
Ai Ký Lệnh C..Ấ.. M Quay Video. Không Làm Láo Sao Phải C..Â. M.
Keywords searched by users: c# dictionary get key from value
Categories: Top 86 C# Dictionary Get Key From Value
See more here: nhanvietluanvan.com
Images related to the topic c# dictionary get key from value
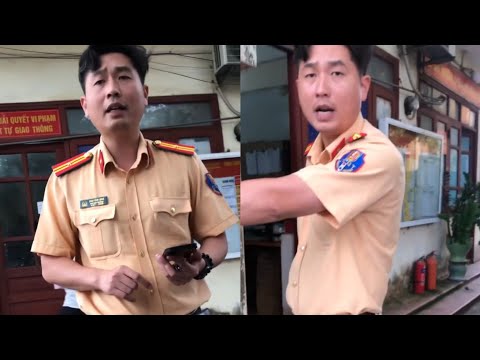
Article link: c# dictionary get key from value.
Learn more about the topic c# dictionary get key from value.
- C cơ bản: Giới thiệu ngôn ngữ C – DevIOT
- Ngôn ngữ lập trình C là gì? – Tại sao sử dụng C? – VietTuts.Vn
- Ngôn ngữ lập trình C là gì? Tìm hiểu về ngôn ngữ lập trình C
- C – Wiktionary tiếng Việt
- Bài 1: Những khái niệm cơ bản về ngôn ngữ C – Tìm ở đây
- Ngôn ngữ lập trình C là gì? Tại Sao Cần Học Lập Trình C
See more: https://nhanvietluanvan.com/luat-hoc/