C# Dereference Of A Possibly Null Reference
In C#, a null reference is a value that represents the absence of an object. It means that a reference variable is not pointing to any valid memory location. In other words, when a variable is assigned a null value, it indicates that it has no value or object associated with it.
Importance of Handling Null References to Avoid Runtime Errors
Handling null references is crucial in C# because dereferencing a null reference can lead to runtime errors, such as NullReferenceException. This exception occurs when code tries to access a member (property, method, or field) of an object that is currently null. Null reference errors can cause program crashes and unexpected behavior, making code unreliable.
Dereferencing a Null Reference in C#
Dereferencing refers to accessing or using the data or behavior of an object through a reference variable. When a null reference is dereferenced in C#, it results in a NullReferenceException. This exception occurs at runtime and must be handled to prevent program termination.
Consequences of Dereferencing a Null Reference in C#
Dereferencing a null reference can lead to unpredictable behavior and runtime errors. When null references are accessed, the program will throw a NullReferenceException, causing the program to crash or halt. This can be particularly harmful if the null reference is used to access important data or perform critical operations.
Potential Exceptions Thrown when Dereferencing a Null Reference
The main exception thrown when dereferencing a null reference in C# is the NullReferenceException. This exception indicates that an object reference variable is null, and an attempt is made to access a member or invoke a method on it.
Checking for Null References Before Dereferencing
To avoid null reference exceptions, it is essential to check for null references before attempting to dereference them. This helps ensure that the reference variable is pointing to a valid object before accessing its members.
Importance of Null Checks in C#
Null checks play a vital role in C# code to prevent null reference exceptions. By checking for null references before dereferencing them, developers can handle potential null scenarios gracefully, improving the reliability and stability of the program.
Different Ways to Check for Null References
There are several ways to check for null references in C#. One common approach is to use an if statement to check if the reference variable is null before accessing its members. For example:
“`csharp
if (myObject != null)
{
// …access members of myObject…
}
“`
Another method is to use the null conditional operator (?.) introduced in C# 6.0, which will be discussed in more detail later.
Conditional Null Checks Using the Null Conditional Operator
The null conditional operator (?.) is a concise way to check for null references before accessing members. It allows for conditional method invocations or property access, only if the reference variable is not null.
This operator simplifies code and reduces the chances of null reference exceptions. For example:
“`csharp
myObject?.SomeMethod(); // Invokes SomeMethod only if myObject is not null
“`
Using the Null Coalescing Operator to Handle Null References
The null coalescing operator (??) is another useful tool for handling null references in C#. It provides a default value to assign if the reference variable is null.
Understanding the Null Coalescing Operator (??)
The null coalescing operator (??) allows for assigning a default value when a reference variable is null. It is useful when you want to provide a fallback value in case the reference variable does not point to a valid object.
Applying the Null Coalescing Operator to Assign Default Values
The null coalescing operator can be applied to assign default values to variables when they are null. For example:
“`csharp
string name = myObject?.Name ?? “Default”;
“`
If myObject or its Name property is null, the variable name will be assigned the value “Default”.
Cascading Null Coalescing to Handle Multiple Null References
The null coalescing operator can be cascaded to handle multiple null references within a single expression. This allows for providing fallback values at each step in case any of the references are null.
Handling Null References with the Null Conditional Operator
The null conditional operator (?.) is a powerful feature introduced in C# 6.0 that simplifies null reference checks when accessing members.
Introduction to the Null Conditional Operator (?.)
The null conditional operator (?.) allows for performing method invocations, property access, or indexing on potentially null objects without causing null reference exceptions.
Performing Method Invocations on Potentially Null Objects
The null conditional operator can be used to safely call methods on potentially null objects. If the object is null, the method will not be called, avoiding a NullReferenceException.
“`csharp
string result = myObject?.ToString(); // Returns null if myObject is null
“`
Using the Null Conditional Operator with Properties and Indexers
The null conditional operator is not limited to method invocations; it can also be used with properties and indexers. It allows for accessing properties or indexing an object without causing a null reference exception.
“`csharp
int count = myObject?.Items.Count; // Returns null if myObject or Items is null
“`
Best Practices for Dealing with Null References in C#
To effectively handle null references in C#, following best practices can improve code reliability and maintainability.
Initializing Variables to Avoid Null References
Initializing variables when declaring them can help prevent null references. By assigning an initial value, the variable will not be null by default, reducing the chances of encountering null reference exceptions.
Using the Null Conditional Operator to Simplify Code
Utilizing the null conditional operator (?.) can simplify code by reducing the number of null reference checks. It provides a concise and readable way to handle null references when accessing members of objects.
Employing Defensive Programming Techniques
Defensive programming techniques involve validating inputs, checking for null references, and implementing exception handling strategies. By taking a defensive approach, developers can minimize the impact of null reference issues and create robust and reliable code.
Exceptions Related to Null References and How to Handle Them
Common Exceptions Thrown When Dealing with Null References
The most common exception thrown when dealing with null references is the NullReferenceException. This exception typically occurs when null references are dereferenced without checking for null.
Appropriate Exception Handling Strategies
To handle null reference exceptions, it is recommended to implement appropriate exception handling strategies. This includes using try-catch blocks to catch and handle the exceptions gracefully, providing meaningful error messages to the users, and gracefully recovering or terminating the program if necessary.
Application of Try-Catch Blocks to Catch Null Reference Exceptions
Try-catch blocks can be used to catch null reference exceptions and handle them accordingly. By wrapping code that might throw a NullReferenceException in a try block, developers can catch the exception in a catch block, allowing for appropriate error handling or recovery.
Preventing Null References Through Proper Design and Coding
Writing Defensive Code to Minimize Null References
Writing defensive code involves anticipating potential null references and implementing checks to validate inputs or object states. By diligently implementing null checks and ensuring that the program can handle null scenarios, developers can minimize null reference issues.
Utilizing the Null Object Pattern to Avoid Null References
The null object pattern is a design pattern that replaces null references with objects that implement the same interface but provide default behavior. By using the null object pattern, developers can eliminate null references and provide consistent behavior when dealing with missing objects.
Implementing Null Checking Mechanisms in Object-Oriented Design
In object-oriented design, null checking mechanisms can be implemented to safeguard against null references. This can include using interfaces or base classes that enforce non-null objects and implementing validation methods or constructors that ensure objects are instantiated correctly.
Additional Tips and Tricks for Working with Null References in C#
Leveraging Debugging Tools and Techniques
When dealing with null reference issues, leveraging debugging tools and techniques can be helpful. Tools like the debugger in Integrated Development Environments (IDEs) allow developers to step through the code, inspect values, and identify null reference errors.
Utilizing Code Analysis to Detect Potential Null Reference Issues
Code analysis tools can also be used to detect potential null reference issues. These tools examine code statically and provide warnings or suggestions for potential null reference problems. By utilizing code analysis tools, developers can catch null reference issues early in the development process.
Real-World Scenarios and Examples Demonstrating Null Reference Handling
To provide practical insights into handling null references, real-world scenarios and examples can be helpful. Demonstrating how to handle null reference issues in various situations, such as accessing external resources or dealing with user input, can enhance understanding and application of null reference handling techniques.
In conclusion, understanding and effectively handling null references in C# is crucial to prevent runtime errors and ensure the reliability of software. By utilizing null checks, null conditional operators, and null coalescing operators, developers can minimize null reference exceptions and create robust and maintainable code. Following best practices, implementing exception handling strategies, and considering proper design can further enhance null reference handling in C#.
Những Ca Khúc Hay Nhất Của Only C
Keywords searched by users: c# dereference of a possibly null reference
Categories: Top 37 C# Dereference Of A Possibly Null Reference
See more here: nhanvietluanvan.com
Images related to the topic c# dereference of a possibly null reference
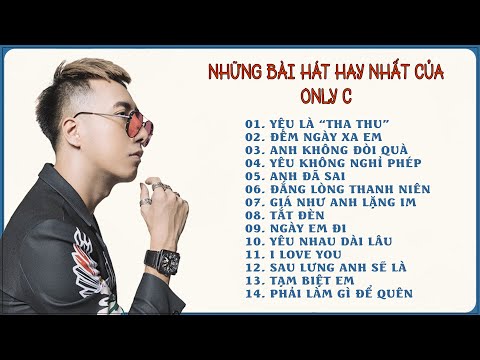
Article link: c# dereference of a possibly null reference.
Learn more about the topic c# dereference of a possibly null reference.
- C cơ bản: Giới thiệu ngôn ngữ C – DevIOT
- Ngôn ngữ lập trình C là gì? – Tại sao sử dụng C? – VietTuts.Vn
- Ngôn ngữ lập trình C là gì? Tìm hiểu về ngôn ngữ lập trình C
- C – Wiktionary tiếng Việt
- Miền Bắc tiếp tục nắng nóng gay gắt, nhiệt độ cao nhất 37độ C
- Ngôn ngữ lập trình C là gì? Tại Sao Cần Học Lập Trình C
See more: nhanvietluanvan.com/luat-hoc