C# Delete A File
Introduction to Deleting Files in C#
Deleting files is a common task in programming and can be easily accomplished in C#. This article will guide you through various methods to delete a file using C#. We will cover different scenarios, including checking if a file exists before deletion, handling exceptions, deleting a read-only file, using wildcard characters, deleting from a specific directory, and deleting multiple files at once.
Methods for Deleting a File in C#
1. Check if a file exists before deletion:
Before deleting a file, it is always a good practice to check if the file exists to prevent any exceptions. The File.Exists() method helps you determine if a file exists or not. You can use it as follows:
“`csharp
string filePath = “path/to/file.txt”;
if (File.Exists(filePath))
{
// File exists, proceed with deletion
File.Delete(filePath);
}
else
{
// File does not exist
Console.WriteLine(“File is not found.”);
}
“`
2. Using the File.Delete() method:
The File.Delete() method allows you to delete a file directly without checking the file’s existence first. However, it throws an exception if the file does not exist. Here’s an example of using this method:
“`csharp
string filePath = “path/to/file.txt”;
try
{
File.Delete(filePath);
Console.WriteLine(“File deleted successfully.”);
}
catch (IOException ex)
{
Console.WriteLine(“An error occurred while deleting the file: ” + ex.Message);
}
“`
3. Handling exceptions when deleting a file:
When deleting a file using the File.Delete() method, you should handle potential exceptions that might occur. Common exceptions include IOException when the file is in use or does not exist, and UnauthorizedAccessException when you don’t have permission to delete the file. Here’s an example of handling these exceptions:
“`csharp
string filePath = “path/to/file.txt”;
try
{
File.Delete(filePath);
Console.WriteLine(“File deleted successfully.”);
}
catch (IOException ex)
{
Console.WriteLine(“An error occurred while deleting the file: ” + ex.Message);
}
catch (UnauthorizedAccessException ex)
{
Console.WriteLine(“You don’t have permission to delete the file: ” + ex.Message);
}
“`
4. Deleting a file using the FileInfo class:
The FileInfo class provides additional methods and properties to work with files. To delete a file using the FileInfo class, you can simply create an instance of it and call the Delete() method. Here’s an example:
“`csharp
string filePath = “path/to/file.txt”;
FileInfo fileInfo = new FileInfo(filePath);
if (fileInfo.Exists)
{
fileInfo.Delete();
Console.WriteLine(“File deleted successfully.”);
}
“`
5. Deleting a file with wildcard characters:
Sometimes, you may need to delete files that match a specific pattern using wildcard characters. The Directory.GetFiles() method can be used along with the File.Delete() method to achieve this. Here’s an example:
“`csharp
string directoryPath = “path/to”;
string searchPattern = “*.txt”;
string[] files = Directory.GetFiles(directoryPath, searchPattern);
foreach (string file in files)
{
File.Delete(file);
}
Console.WriteLine(“Files matching the pattern were successfully deleted.”);
“`
6. Using the Directory class to delete a file:
If you know the directory where the file is located, you can use the Directory class along with the File.Delete() method directly. Here’s an example:
“`csharp
string directoryPath = “path/to”;
string fileName = “file.txt”;
string filePath = Path.Combine(directoryPath, fileName);
File.Delete(filePath);
Console.WriteLine(“File deleted successfully.”);
“`
7. Deleting a read-only file in C#:
By default, files may have a read-only attribute set, which prevents them from being deleted. To delete a read-only file, you can remove the read-only attribute using the File.SetAttributes() method before deletion. Here’s an example:
“`csharp
string filePath = “path/to/file.txt”;
File.SetAttributes(filePath, FileAttributes.Normal);
File.Delete(filePath);
Console.WriteLine(“File deleted successfully.”);
“`
8. Deleting a file from a specific directory:
If you want to delete a file from a specific directory and you know the file’s name, you can directly provide the file’s full path to the File.Delete() method. Here’s an example:
“`csharp
string filePath = “path/to/file.txt”;
File.Delete(filePath);
Console.WriteLine(“File deleted successfully.”);
“`
9. Deleting multiple files at once in C#:
To delete multiple files in one go, you can loop through a collection of file paths and call the File.Delete() method for each file. Here’s an example:
“`csharp
string[] filePaths = { “path/to/file1.txt”, “path/to/file2.txt”, “path/to/file3.txt” };
foreach (string filePath in filePaths)
{
File.Delete(filePath);
}
Console.WriteLine(“Multiple files deleted successfully.”);
“`
FAQs
Q1. Can I delete a file permanently without moving it to the Recycle Bin?
In C#, the File.Delete() method permanently deletes a file without moving it to the Recycle Bin. However, please note that this action cannot be undone, so use it with caution.
Q2. How can I secure the deletion process to prevent accidental file deletion?
To minimize the risk of accidentally deleting important files, you should always double-check the file’s path and inform users about the deletion process. Additionally, you can implement access controls and limit the privileges of the accounts responsible for file deletion.
Q3. What happens if I try to delete a file that is already open or in use?
If you try to delete a file that is already open or in use by another process, the File.Delete() method will throw an IOException. You should handle this exception and provide adequate feedback to the user.
Q4. Are there any alternatives to deleting files using C#?
Yes, apart from using C# to delete files, you can also use command-line utilities, such as the del or rm command in the Windows or Unix-like operating systems, respectively.
Conclusion
In this article, we covered various methods to delete a file in C#. We discussed checking file existence, using the File.Delete() method, handling exceptions, deleting files with wildcard characters, using the FileInfo and Directory classes, deleting read-only files, deleting files from specific directories, and deleting multiple files at once. By applying these methods appropriately, you can confidently and efficiently manage file deletion in your C# applications.
Những Ca Khúc Hay Nhất Của Only C
Keywords searched by users: c# delete a file
Categories: Top 95 C# Delete A File
See more here: nhanvietluanvan.com
Images related to the topic c# delete a file
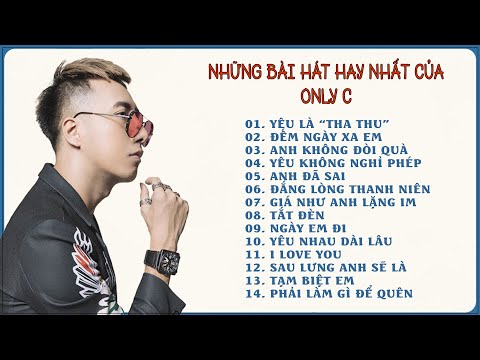
Article link: c# delete a file.
Learn more about the topic c# delete a file.
- C cơ bản: Giới thiệu ngôn ngữ C – DevIOT
- Ngôn ngữ lập trình C là gì? – Tại sao sử dụng C? – VietTuts.Vn
- Ngôn ngữ lập trình C là gì? Tìm hiểu về ngôn ngữ lập trình C
- Ngôn ngữ lập trình C là gì? Tại Sao Cần Học Lập Trình C
- C – Wiktionary tiếng Việt
- Bài 1: Những khái niệm cơ bản về ngôn ngữ C – Tìm ở đây
See more: blog https://nhanvietluanvan.com/luat-hoc