C# Array Of Objects
In C#, an array is a collection of elements of the same type. However, with the use of objects, we can create arrays that can store multiple objects of different types. This provides flexibility and allows us to store and access different objects in a single array.
Declaring and Initializing an Array of Objects
To declare and initialize an array of objects in C#, we need to specify the type of objects that the array will contain. Here is the syntax:
“`csharp
// Declare and initialize an array of objects
Object[] myArray = new Object[5];
“`
In the above example, we declared and initialized an array named “myArray” of type “Object” with a size of 5. This means that our array can store 5 objects.
Accessing the Elements of an Array of Objects
To access the elements of an array of objects, we can use the index operator. The index of an array starts from 0. Here is an example:
“`csharp
// Accessing the elements of an array of objects
Object firstObject = myArray[0];
“`
In the above example, we accessed the first element of the array “myArray” and stored it in the variable “firstObject”.
Modifying the Elements of an Array of Objects
To modify the elements of an array of objects, we can use the index operator along with the assignment operator. Here is an example:
“`csharp
// Modifying the elements of an array of objects
myArray[1] = new MyClass();
“`
In the above example, we created a new instance of the class “MyClass” and assigned it to the second element of the array “myArray”.
Iterating through an Array of Objects
To iterate through an array of objects, we can use a loop statement such as the “for” loop. Here is an example:
“`csharp
// Iterating through an array of objects
for (int i = 0; i < myArray.Length; i++)
{
Console.WriteLine(myArray[i].ToString());
}
```
In the above example, we used a "for" loop to iterate through the array "myArray" and printed each element using the "ToString()" method.
Sorting an Array of Objects
To sort an array of objects in a specific order, we can use the "Array.Sort()" method. However, this method requires that the objects in the array implement the "IComparable" interface. Here is an example:
```csharp
// Sorting an array of objects
Array.Sort(myArray);
```
In the above example, we sorted the array "myArray" in ascending order based on the default comparison of the objects.
Searching for an Object in an Array
To search for a specific object in an array of objects, we can use the "Array.IndexOf()" method. This method returns the index of the first occurrence of the object in the array. Here is an example:
```csharp
// Searching for an object in an array
int index = Array.IndexOf(myArray, myObject);
```
In the above example, we searched for the object "myObject" in the array "myArray" and stored the index of its first occurrence in the variable "index".
FAQs about C# Array of Objects
Q: Can an array of objects store objects of different types?
A: Yes, it is possible to store objects of different types in an array of objects. Since the type "Object" is the base class for all types in C#, it can be used to store any objects.
Q: How can I access the properties and methods of an object in an array?
A: To access the properties and methods of an object in an array, you need to first access the object itself using the index operator and then use the member access operator. For example: `myArray[0].PropertyName` or `myArray[0].MethodName()`.
Q: Can I resize an array of objects in C#?
A: No, the size of an array in C# is fixed once it is created. If you need to resize an array, you will need to create a new array with the desired size and copy the elements from the old array to the new array.
Q: Can I use the "foreach" loop to iterate through an array of objects?
A: Yes, you can use the "foreach" loop to iterate through an array of objects. Here is an example:
```csharp
foreach (Object obj in myArray)
{
Console.WriteLine(obj.ToString());
}
```
Q: Can I sort an array of objects based on a custom comparison?
A: Yes, you can implement the "IComparable" interface in your objects and provide a custom comparison logic. This allows you to sort the array of objects based on the custom comparison.
In conclusion, an array of objects in C# provides a powerful way to store and manipulate multiple objects of different types. By understanding how to create, access, modify, iterate through, sort, and search for objects in an array, you can utilize this feature effectively in your C# programs.
Những Ca Khúc Hay Nhất Của Only C
Keywords searched by users: c# array of objects
Categories: Top 100 C# Array Of Objects
See more here: nhanvietluanvan.com
Images related to the topic c# array of objects
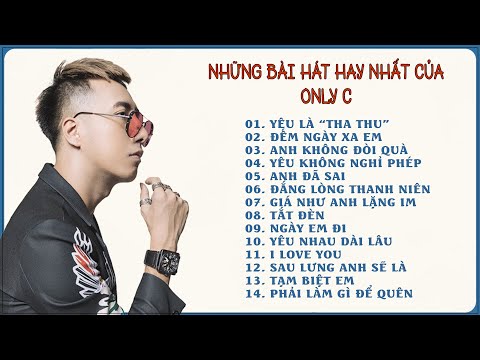
Article link: c# array of objects.
Learn more about the topic c# array of objects.
- Ngôn ngữ lập trình C là gì? – Tại sao sử dụng C? – VietTuts.Vn
- C cơ bản: Giới thiệu ngôn ngữ C – DevIOT
- Ngôn ngữ lập trình C là gì? Tìm hiểu về ngôn ngữ lập trình C
- C – Wiktionary tiếng Việt
- Miền Bắc tiếp tục nắng nóng gay gắt, nhiệt độ cao nhất 37độ C
- Bài 1: Những khái niệm cơ bản về ngôn ngữ C – Tìm ở đây
- C for Beginners – CodeLearn