C# Add To Dictionary
In C#, a Dictionary is a collection that allows you to store data in key-value pairs. Each key in a Dictionary must be unique, and the key-value pairs are not sorted in any particular order. A Dictionary is defined using the Dictionary class in C#, which is part of the System.Collections.Generic namespace.
To define a Dictionary in C#, you need to specify the data types of both the key and the value. For example, to create a Dictionary that stores string keys and integer values, you would use the following syntax:
“`csharp
Dictionary
“`
Using the Dictionary Class in C#
The Dictionary class in C# provides a set of methods and properties that allow you to perform various operations on a Dictionary. One of the most common operations is adding a key-value pair to the Dictionary.
Adding a Key-Value Pair to a Dictionary in C#
To add a key-value pair to a Dictionary in C#, you can use either the Add() method or the indexer syntax.
Using the Add() Method to Add a Key-Value Pair in C#
The Add() method is a member of the Dictionary class and allows you to add a new key-value pair to the Dictionary. Here’s an example:
“`csharp
myDictionary.Add(“Key1”, 1);
“`
In the example above, we are adding a key-value pair with the key “Key1” and the value 1 to the Dictionary called myDictionary.
Using Indexer Syntax to Add a Key-Value Pair in C#
In addition to the Add() method, you can also use indexer syntax to add a key-value pair to a Dictionary. Here’s an example:
“`csharp
myDictionary[“Key2”] = 2;
“`
In the example above, we are adding a key-value pair with the key “Key2” and the value 2 to the Dictionary called myDictionary. This syntax is more concise and is often preferred when adding or updating values in a Dictionary.
Using the AddOrUpdate() Method to Add or Update a Key-Value Pair in C#
Sometimes, you may need to add a key-value pair to a Dictionary, but if the key already exists, you want to update its value instead. The AddOrUpdate() method can be used for this purpose. Here’s an example:
“`csharp
myDictionary.AddOrUpdate(“Key3”, 3, (key, value) => value + 1);
“`
In the example above, we are adding a key-value pair with the key “Key3” and the value 3 to the Dictionary called myDictionary. If the key already exists, the lambda expression `(key, value) => value + 1` is used to determine the updated value. In this case, the existing value is incremented by 1.
Adding Multiple Key-Value Pairs to a Dictionary at Once in C#
If you have multiple key-value pairs that you want to add to a Dictionary at once, you can use the AddRange() method. Here’s an example:
“`csharp
Dictionary
{
{ “Key4”, 4 },
{ “Key5”, 5 },
{ “Key6″, 6 }
};
myDictionary.AddRange(multiplePairs);
“`
In the example above, we create a new Dictionary called multiplePairs with three key-value pairs. We then use the AddRange() method to add all these key-value pairs to the existing Dictionary myDictionary.
Adding a Range of Key-Value Pairs to a Dictionary in C#
In addition to adding multiple key-value pairs at once, you can also add a range of key-value pairs to a Dictionary using the AddRange() method. Here’s an example:
“`csharp
myDictionary.AddRange(Enumerable.Range(1, 10).ToDictionary(x => $”Key{x}”, x => x));
“`
In the example above, we use the Range() method from the Enumerable class to generate a range of numbers from 1 to 10. We then use the ToDictionary() method to convert this range into a Dictionary. Finally, we use the AddRange() method to add all the key-value pairs from this range to the existing Dictionary myDictionary.
Handling Exceptions when Adding to a Dictionary in C#
When adding key-value pairs to a Dictionary in C#, you need to be careful about handling exceptions. The Add() method, in particular, can throw an ArgumentException if the key already exists in the Dictionary. One way to handle this exception is by using a try-catch block. Here’s an example:
“`csharp
try
{
myDictionary.Add(“Key1”, 1);
}
catch (ArgumentException)
{
// Handle the exception
}
“`
In the example above, we attempt to add a key-value pair with the key “Key1” and the value 1 to the Dictionary. If the key already exists, an ArgumentException is thrown, and we can handle it accordingly within the catch block.
FAQs:
Q: Can I add different data types as values in a Dictionary?
A: Yes, you can add values of different data types in a Dictionary. The key must be of a specific data type, but the values can be of any data type.
Q: How can I check if a key already exists in a Dictionary before adding it?
A: You can use the ContainsKey() method to check if a key already exists in a Dictionary. It returns a Boolean value indicating whether the key is present or not.
Q: Can I add null as a key or value in a Dictionary?
A: Yes, you can add null as a key or value in a Dictionary. However, you need to be careful when accessing or manipulating the Dictionary, as null values can cause null reference exceptions.
Q: Is the order of key-value pairs maintained in a Dictionary?
A: No, the key-value pairs in a Dictionary are not maintained in any particular order. If you need to retrieve the key-value pairs in a specific order, you can sort them or use a different data structure, such as a SortedDictionary.
Q: How can I remove a key-value pair from a Dictionary?
A: You can use the Remove() method to remove a specific key-value pair from a Dictionary. Alternatively, you can also use the Clear() method to remove all key-value pairs from a Dictionary.
Q: What happens if I add a key-value pair to a Dictionary with the same key multiple times?
A: If you add a key-value pair to a Dictionary with the same key multiple times using the Add() method, an ArgumentException will be thrown. However, if you use the indexer syntax, the value for the existing key will be updated instead.
Ai Ký Lệnh C..Ấ.. M Quay Video. Không Làm Láo Sao Phải C..Â. M.
Keywords searched by users: c# add to dictionary
Categories: Top 56 C# Add To Dictionary
See more here: nhanvietluanvan.com
Images related to the topic c# add to dictionary
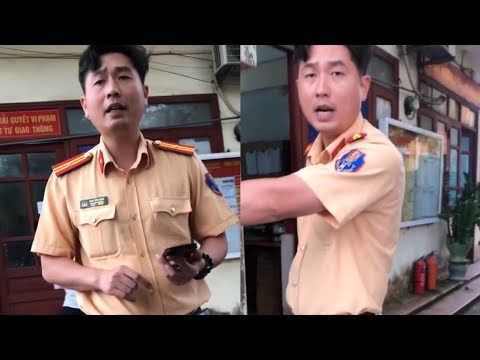
Article link: c# add to dictionary.
Learn more about the topic c# add to dictionary.
- C cơ bản: Giới thiệu ngôn ngữ C – DevIOT
- Ngôn ngữ lập trình C là gì? – Tại sao sử dụng C? – VietTuts.Vn
- Bài 1: Những khái niệm cơ bản về ngôn ngữ C – Tìm ở đây
- Ngôn ngữ lập trình C là gì? Tìm hiểu về ngôn ngữ lập trình C
- Ngôn ngữ lập trình C là gì? Tại Sao Cần Học Lập Trình C
- Trợ giúp > Mã của các nhãn – Cambridge Dictionary
See more: https://nhanvietluanvan.com/luat-hoc/