Byte To Hex String C#
In the field of programming, bytes and hex strings are common terms that developers encounter frequently. Understanding how to convert bytes to hex strings is crucial for various programming tasks. In this article, we will dive deep into the concept of byte to hex string conversion in C#.
What is a Byte in C#?
In C#, a byte is a fundamental data type that represents an 8-bit unsigned integer. It can store values in the range of 0 to 255. Bytes are widely used in various programming scenarios, such as handling binary data, encryption algorithms, and network communication protocols. Generally, a byte is considered as the smallest addressable unit of memory in computers.
Representation of Bytes in Computer Memory
In computer memory, bytes are stored sequentially as a series of 8 bits. Each bit can have a value of either 0 or 1, representing the binary system. For example, the decimal value 187 is represented in binary as 10111011. This binary representation is how the byte is stored in memory.
Importance of Bytes in Programming
Bytes play a vital role in programming due to their versatility and compactness. They are used extensively in handling low-level operations, such as reading and writing data from files, creating network packets, or interacting with hardware devices. Additionally, bytes are also used in encryption algorithms, image processing, and manipulating binary data efficiently.
What is a Hex String in C#?
A hex string, short for hexadecimal string, is a representation of binary data in base-16 format. It uses a combination of digits from 0 to 9 and the letters A to F to denote values from 0 to 15. For example, the decimal value 187 is represented in hex string as BB.
Representation of Hex Strings in Memory
In memory, hex strings are stored as sequences of characters, where each character corresponds to one nibble (half byte) of data. For example, the hex string “ABCDEF” is stored as six individual characters in memory.
Usage and Importance of Hex Strings in Programming
Hex strings find usage in various programming scenarios, such as representing binary data in a human-readable format, comparing data patterns, debugging, and cryptographic operations. They provide an easy and concise representation of binary data, allowing developers to visualize and manipulate it efficiently.
Converting Byte to Hex String in C#
In C#, there are multiple methods available to convert bytes to hex strings. One of the widely used approaches is through the BitConverter class, which provides convenient methods to convert different data types, including bytes, to hex strings.
Exploring the BitConverter Class for Byte to Hex String Conversion
The BitConverter class in C# exposes static methods that allow converting bytes to hex strings and vice versa. The method `ToString` of the BitConverter class is commonly used to convert a byte array to a hex string.
Converting Individual Bytes to Hex Strings
To convert individual bytes to hex strings, you can use the `ToString` method with the appropriate format specifier. For example, `byteValue.ToString(“X2”)` will convert a single byte to a 2-digit uppercase hex string.
Benefits and Limitations of Converting Bytes to Hex Strings
Advantages of Using Hex Strings for Byte Representation
Using hex strings for byte representation offers several advantages. First, it provides a compact and readable representation of binary data. Second, hex strings can be easily transmitted over various communication protocols, as they only use printable characters. Third, hex strings are commonly used for debugging purposes as they facilitate identifying patterns or inspecting data at a granular level.
Limitations and Potential Issues with Using Hex Strings
While hex strings have their advantages, they also have some limitations. First, hex strings occupy more memory compared to the original byte representation. Second, converting large amounts of data to hex strings can have a performance impact, especially if done inefficiently. Lastly, hex strings may not be suitable for all scenarios, such as when working with highly resource-constrained systems or optimizing for speed.
Performance Considerations in Byte to Hex String Conversion
When converting bytes to hex strings, it is crucial to consider performance. To improve performance, it is recommended to use optimized algorithms, such as using lookup tables or bitwise operations, instead of using slow string concatenation operations. Additionally, minimizing unnecessary string allocations and utilizing efficient memory handling techniques can significantly improve the overall performance.
Examples of Byte to Hex String Conversion in C#
Let’s explore some examples of byte to hex string conversion in C#.
1. Converting Byte Arrays to Hex Strings:
“`csharp
byte[] byteArray = { 187, 128, 255 };
string hexString = BitConverter.ToString(byteArray).Replace(“-“, “”);
Console.WriteLine(hexString); // Output: BB80FF
“`
2. Handling Different Data Types During Conversion:
“`csharp
int integerValue = 255;
byte byteValue = (byte)integerValue;
string hexString = byteValue.ToString(“X2”);
Console.WriteLine(hexString); // Output: FF
“`
Best Practices for Byte to Hex String Conversion in C#
To ensure accuracy and reliability in byte to hex string conversion, it is essential to follow these best practices:
1. Handle data types correctly: Ensure that the input data type is compatible with the conversion method being used. Cast or transform the data appropriately before conversion.
2. Implement error handling: Handle potential exceptions that may occur during byte to hex string conversion, such as invalid input or out-of-range values. Employ try-catch blocks to handle and report any errors gracefully.
3. Optimize code for efficiency and readability: Use optimized algorithms and techniques to improve the performance of the conversion process. Consider readability and maintainability to make the code easier to understand and modify in the future.
In conclusion, understanding how to convert bytes to hex strings is crucial for various programming tasks in C#. Bytes serve as essential components for handling binary data, encryption algorithms, and network communication protocols. Hex strings provide a human-readable representation of binary data and are widely used for debugging and cryptographic operations. By following best practices and optimizing for efficiency, developers can successfully convert bytes to hex strings in their C# programs.
How Do You Convert Byte Array To Hexadecimal String C#?
How To Print Bytes As Hex In C?
Printing bytes as hex is a common task in C programming, especially when working with binary data or low-level file operations. In this article, we will explore different approaches to print bytes as hex in C and provide insights on when and how to use them effectively.
Table of Contents:
1. Printing Bytes as Hex in C
2. Exploring Possible Implementations
3. Code Examples
4. Frequently Asked Questions (FAQs)
1. Printing Bytes as Hex in C:
To print bytes as hex in C, we need to convert each byte to its corresponding hex representation. Hexadecimal (hex) uses a base-16 numbering system, making it ideal for representing binary data. In C, we can achieve this by utilizing the `printf` function from the standard library with format specifiers.
2. Exploring Possible Implementations:
There are multiple ways to print bytes as hex in C. Some common implementation methods include using loops, bitwise operations, or predefined libraries. Each method has its advantages and disadvantages, depending on the specific requirements of your project.
– Loop Implementation:
One simple approach is to use a for loop to iterate through the bytes and print their hex values individually. This method involves using the `%02X` format specifier in each iteration to print the bytes in uppercase hex format with leading zeros.
– Bitwise Operations:
Another efficient way to print bytes as hex is by utilizing bitwise operations. By using logical AND and right-shift operations, we can extract each nibble (half-byte) and convert it into its respective hex digit. This method is particularly useful when dealing with large amounts of binary data.
– Predefined Libraries:
If you prefer a more convenient and versatile approach, you can utilize predefined libraries like `stdio.h` and `inttypes.h`. These libraries provide functions specifically designed for printing bytes as hex, such as `fprintf`, `sprintf`, and `printf` with format specifiers like `%02hhX`.
3. Code Examples:
To illustrate the implementation methods discussed above, let’s consider some code examples:
– Loop Implementation:
“`c
void printBytesAsHexLoop(const unsigned char* data, size_t size) {
for (size_t i = 0; i < size; i++) {
printf("%02X ", data[i]);
}
printf("\n");
}
```
- Bitwise Operations:
```c
void printBytesAsHexBitwise(const unsigned char* data, size_t size) {
for (size_t i = 0; i < size; i++) {
printf("%X%X ", (data[i] & 0xF0) >> 4, data[i] & 0x0F);
}
printf(“\n”);
}
“`
– Predefined Libraries:
“`c
#include
#include
void printBytesAsHexLibrary(const unsigned char* data, size_t size) {
for (size_t i = 0; i < size; i++) {
printf("%02hhX ", data[i]);
}
printf("\n");
}
```
4. Frequently Asked Questions (FAQs):
Q1. Why should I print bytes as hex in C?
A1. Printing bytes as hex in C is helpful for debugging and visualizing binary data or manipulating low-level file operations. Hexadecimal representation provides a concise and more readable format than raw binary data.
Q2. Can I print bytes as hex in lowercase format?
A2. Yes, you can modify the format specifiers to print hex values in lowercase. For example, the `%02x` format specifier will print lowercase hex values with leading zeros.
Q3. Which implementation method is the most efficient?
A3. The efficiency of each implementation method depends on the specific use case and the size of data being processed. Generally, bitwise operations tend to be more efficient for large sets of data, while loop implementations are suitable for smaller data sizes.
Q4. How can I handle endianness issues when printing bytes as hex?
A4. Endianness refers to the byte order of multibyte data types. To handle endianness issues, you may need to convert the byte order before printing the data. Techniques like byte swapping or using platform-specific functions/library calls can be employed.
Q5. Are there any libraries specifically designed for hex printing in C?
A5. While there isn't a dedicated library solely for hex printing, various standard libraries like `stdio.h` and `inttypes.h` provide functions and format specifiers suitable for printing bytes as hex.
In conclusion, printing bytes as hex in C is a useful technique when working with binary data or low-level operations. This article has explored different implementation methods, including loop, bitwise operations, and utilizing predefined libraries. By following the provided code examples and understanding the nuances discussed, you can efficiently print bytes as hex in your C programs.
How To Convert Byte To String In C?
Converting data types in programming is a common task that developers encounter on a regular basis. In C programming, converting a byte to a string is often needed when dealing with binary data or when manipulating character-oriented operations. Converting bytes to strings allows for easier data handling and communication between systems. In this article, we will dive into the process of converting a byte to a string in C and explore various methods to achieve this conversion.
Before we delve into the conversion methods, it’s important to understand the fundamental differences between bytes and strings. A byte, in simple terms, is the unit of measurement for digital information and can represent a single character. On the other hand, a string is a sequence of characters that are collectively treated as a single entity. The conversion from a byte to a string involves encoding the byte value into a character representation.
Method 1: Using the Standard Library Functions
The C programming language provides several standard library functions that make byte to string conversion straightforward. One such function is `sprintf`, which converts byte data to a string and stores it in a character array. The following code snippet demonstrates the usage of `sprintf`:
“`c
unsigned char byteValue = 0xAB;
char stringValue[3];
sprintf(stringValue, “%02X”, byteValue);
“`
In this example, the `%02X` format specifier specifies that the byte `0xAB` should be converted to a two-character hexadecimal string. The resulting string is then stored in the `stringValue` array.
Method 2: Bit Manipulation
Another way to convert a byte to a string is by using bit manipulation techniques. This method involves extracting individual bits from the byte and converting them to their corresponding ASCII representation. Here’s an example code snippet:
“`c
unsigned char byteValue = 0xAB;
char stringValue[9];
for (int i = 0; i < 8; i++) {
stringValue[7 - i] = '0' + ((byteValue >> i) & 1);
}
stringValue[8] = ‘\0’;
“`
In this method, the byte is iterated bitwise from the least significant bit to the most significant bit. Each bit is converted to either ‘0’ or ‘1’ and stored in the `stringValue` array.
Method 3: Using a Look-Up Table
When the range of possible byte values is limited, a look-up table can be utilized for efficient byte to string conversion. This method involves mapping each byte value to its equivalent string representation. Here’s an example implementation:
“`c
unsigned char byteValue = 0xAB;
const char* lookupTable[256] = {
“00”, “01”, “02”, … , “FE”, “FF”
};
const char* stringValue = lookupTable[byteValue];
“`
In this method, an array of string literals is created, where each index corresponds to its hexadecimal value. By accessing the array using the byte value as an index, the corresponding string representation can be obtained.
FAQs:
Q1. When is byte to string conversion necessary in C?
Byte to string conversion becomes necessary in situations like encoding binary data for transmission over a network, displaying binary values in a readable format, or when dealing with character-oriented operations.
Q2. Can I convert a byte to a string using libraries other than the standard C library?
Yes, depending on the specific development environment or platform, there may be alternative libraries or functions available that offer byte to string conversion capabilities. However, the methods mentioned in this article using the standard C library are widely applicable.
Q3. How do I convert a string back to a byte in C?
To convert a string representation back to a byte, you can use the `strtol` function provided by the standard library. This function allows you to parse a string and obtain its numeric value.
Q4. Does the byte to string conversion method affect the original byte value?
No, the byte to string conversion methods explained here do not alter the original byte value. They only produce a string representation of the byte.
Q5. How can I handle bytes with leading zeros in the converted string?
Depending on the desired format, you can use format modifiers like `%02X` in the `sprintf` function to handle leading zeros. This ensures that the resulting string representation contains leading zeros if the byte value is less than `0x10`.
In conclusion, converting a byte to a string in C allows for efficient handling of binary data and interoperability with character-based operations. The methods presented in this article offer different approaches to achieve this conversion, ranging from utilizing standard library functions to bit manipulation and look-up tables. By understanding these techniques, developers can easily convert bytes to strings and manipulate them as needed.
Keywords searched by users: byte to hex string c# Byte array to hex string c, Convert byte to string C++, Byte to hex string arduino, Convert hex to string c, Byte array to string C#, Convert byte to hex c++, Hex string to byte array, String to byte c
Categories: Top 21 Byte To Hex String C#
See more here: nhanvietluanvan.com
Byte Array To Hex String C
In C programming, byte arrays are commonly used to store sequences of bytes or binary data. Sometimes, it becomes necessary to convert a byte array into a hexadecimal string representation for various purposes like printing, saving, or transmission.
Converting a byte array to a hex string in C involves a systematic procedure that transforms each byte into its hexadecimal representation and concatenates them to form the final string. Let’s explore this process in detail.
Procedure to Convert Byte Array to Hex String:
1. Declare a byte array:
Start by declaring a byte array containing the binary data that needs to be converted.
2. Calculate the size of the byte array:
Determine the number of bytes present in the array using the sizeof() operator.
3. Iterate through the byte array:
Use a loop to iterate through each byte in the array.
4. Convert each byte to hexadecimal:
For every byte, apply a conversion process that converts it into its corresponding hexadecimal representation. This can be achieved using bitwise operations and masks.
5. Append hexadecimal representation to a string:
Concatenate the converted hexadecimal representation of each byte to a string.
6. Repeat until all bytes are processed:
Keep repeating steps 3 to 5 until all bytes in the byte array have been processed.
7. Null-terminate the string:
Add a null character at the end of the hexadecimal string to mark its termination.
8. The resulting hex string:
The final result will be a null-terminated string representing the byte array in hexadecimal format.
Let’s consider an example to understand the conversion process better:
“`c
void ByteArrayToHexString(const unsigned char* byteArray, int size, char* hexString)
{
for (int i = 0; i < size; i++)
sprintf(hexString + (i * 2), "%02x", byteArray[i]);
hexString[size * 2] = '\0';
}
int main()
{
unsigned char byteArray[] = { 0xAA, 0xBB, 0xCC, 0xDD, 0xEE };
int size = sizeof(byteArray);
char hexString[size * 2 + 1];
ByteArrayToHexString(byteArray, size, hexString);
printf("Hex String: %s\n", hexString);
return 0;
}
```
In this example, we have a byte array `byteArray` with a size of 5 bytes. The `ByteArrayToHexString` function takes the byte array, its size, and a character array `hexString` as parameters. Within the function, a loop iterates through each byte in the array and converts it to its corresponding hexadecimal representation using the `sprintf` function. The result is stored in the `hexString` array, which is then printed in the `main` function using `printf`.
FAQs:
Q1. What is the purpose of converting a byte array to a hex string in C?
- Converting a byte array to a hex string is often required when dealing with binary data that needs to be represented in a human-readable format, such as when printing, saving, or transmitting data.
Q2. Can the conversion process be simplified?
- The conversion process can vary depending on the requirements or constraints. However, the outlined procedure provides a general approach. Different methods, libraries, or custom implementations might provide alternative ways to achieve the same result.
Q3. How can the converted hex string be converted back to a byte array?
- To convert the hex string back to a byte array, the reverse process must be followed. Each pair of characters in the hex string is converted to its corresponding byte value using the `sscanf` or `strtoul` functions, and the resulting bytes are stored in a byte array.
Q4. What are some common applications where byte array to hex string conversion is required?
- Byte array to hex string conversion is often used in cryptographic algorithms, network protocols, file formats, and various low-level programming tasks.
Q5. Are there any built-in functions or libraries available in C to perform this conversion?
- C does not provide built-in functions to directly convert a byte array to a hex string. However, certain libraries or frameworks may offer such functionality that you can incorporate into your project.
In conclusion, converting a byte array to a hex string in C follows a systematic procedure involving iterative conversion of each byte to its hexadecimal representation. Understanding this process enables developers to manipulate, represent, and transmit binary data in a human-readable format.
Convert Byte To String C++
Introduction (100 words):
In C++, converting a byte to a string is a common requirement in various programming scenarios. Whether it be for sending data over a network, storing binary data, or simply displaying byte values as human-readable output, understanding how to convert bytes to strings is essential. In this comprehensive guide, we will delve into different methods available in C++ to perform this conversion. We will explore various techniques, best practices, and provide code examples to help you accomplish this task effortlessly.
I. Converting Byte to String in C++ (500 words)
1. Using the std::stringstream class:
The std::stringstream class provides facilities for formatting data as strings in a similar way to how the C library functions printf and sprintf work. By using this class, we can convert a byte to a string representation easily.
Example code snippet:
“`cpp
#include
#include
std::string byteToString(byte value) {
std::stringstream ss;
ss << static_cast
return ss.str();
}
“`
Explanation:
Firstly, we include the necessary headers for working with stringstream and the string class. Next, we define a function `byteToString()` that takes a byte value as input. Inside the function, we create an instance of stringstream and insert the byte value into it using the `<<` operator. Finally, we convert the stringstream object to a string using the `str()` member function and return the result.
2. Using a character array (c-string) approach:
By treating the byte as a character and forming a string from it, we can effectively convert a byte into its string representation.
Example code snippet:
```cpp
#include
std::string byteToString(byte value) {
char str[4];
snprintf(str, sizeof(str), “%d”, value);
return str;
}
“`
Explanation:
In this approach, we define a character array `str` with a fixed size of 4 (including space for the null-terminating character). The `snprintf` function is then used to store the byte value as a string in `str`. The `sizeof` operator ensures that we don’t exceed the allocated space while converting. Finally, we return the character array as a string.
3. Utilizing the std::to_string() function:
C++11 introduced the `std::to_string()` function, which greatly simplifies the conversion of fundamental types to string format.
Example code snippet:
“`cpp
#include
std::string byteToString(byte value) {
return std::to_string(value);
}
“`
Explanation:
With the help of `std::to_string()`, we can directly convert a byte value to its string representation. The function takes the byte value as its argument and returns the string representation.
II. Frequently Asked Questions (FAQs) (400 words)
1. Why is it important to convert a byte to a string?
Converting a byte to a string is essential in many applications. It allows us to handle binary data, transmit data over networks, manipulate file contents, and display byte values in a human-readable format.
2. Which approach is the most efficient for byte-to-string conversion?
In terms of efficiency, using the `std::to_string()` function is generally the most optimized approach. Since it is available starting from C++11, it leverages modern functionalities and takes care of the conversion process internally.
3. Are there any limitations to consider when using std::stringstream?
While std::stringstream provides a versatile approach, it may introduce some overhead due to the underlying formatting mechanism. In performance-critical scenarios, it is recommended to perform benchmarking and consider alternate approaches accordingly.
4. Can I convert multiple bytes to a string simultaneously?
Yes, if you have a sequence of bytes that you want to convert to a single string, you can iterate over the bytes and concatenate their string representations using any of the discussed methods.
5. How can I handle non-printable byte values during conversion?
If you encounter non-printable bytes or bytes with special characters, you can implement custom logic within the conversion function to handle such cases. For instance, you can replace non-printable values with a placeholder symbol or escape special characters accordingly.
Conclusion (70 words):
In this guide, we have explored various methods to convert a byte to a string in C++. By leveraging libraries such as std::stringstream and functions like std::to_string(), developers can effortlessly perform this conversion. It is crucial to understand the different approaches and choose the one that best suits your requirements while considering factors like performance, readability, and complexity.
Images related to the topic byte to hex string c#
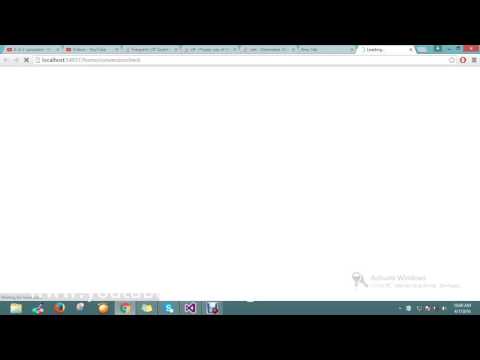
Article link: byte to hex string c#.
Learn more about the topic byte to hex string c#.
- How do you convert a byte array to a hexadecimal string in C?
- Working with Hexadecimal values in C programming language
- How to convert byte array to string in C – Tutorialspoint
- Functions of Hexadecimal in C Programming Language – eduCBA
- Convert a string to hexadecimal ASCII values – GeeksforGeeks
- Program for converting an array of bytes to hexadecimal
- How to convert a byte array to a hexadecimal string – Quora
- Converting Between Byte Arrays and Hexadecimal Strings in …
- Hex string to byte array, in C – Programming Idioms
- C# byte array to hex string – ZetCode
- Bytes To Hex String (2) : HEX « Data Types « C# / C Sharp
- How to convert between hexadecimal strings and numeric types
See more: https://nhanvietluanvan.com/luat-hoc/