Break From Foreach Javascript
Introduction:
JavaScript provides several methods for iterating over arrays, and one widely used method is the forEach loop. While this loop is effective for most scenarios, there may be instances where developers need to break out of the loop prematurely. In this article, we will explore how to break from a forEach loop in JavaScript, discuss alternative approaches, and address common questions and considerations.
What is a forEach Loop in JavaScript?
A forEach loop is a higher-order function in JavaScript that allows you to iterate over each element in an array. It provides a simpler and more readable syntax compared to traditional for loops, especially when it comes to performing operations on every item in the array.
Syntax and Working of a forEach Loop:
The syntax of a forEach loop is as follows:
“`
array.forEach(function(currentValue, index, array) {
// code to execute
});
“`
The “currentValue” parameter represents the value of the current element being processed, “index” refers to the index of the element, and “array” represents the entire array.
Advantages of using a forEach Loop:
1. Simplicity: The forEach loop simplifies the process of iterating over an array and executing operations on each element.
2. Readability: The code written within a forEach loop is concise and self-explanatory, making it easy to understand and maintain.
3. Avoiding side effects: forEach loops are “side-effect free,” meaning they do not change the original array or return a new one, promoting safer coding practices.
Breaking from a forEach Loop:
Unlike traditional for loops, a forEach loop does not provide a built-in mechanism to break out of the loop prematurely. The forEach loop will always iterate over the entire array, regardless of the conditions specified within the loop’s body. However, there are alternative approaches that can be used to break from a forEach loop.
Alternative Approaches to Breaking from a forEach Loop:
1. Using a normal for loop: The simplest way to break out of a loop is to use a regular for loop instead of a forEach loop. By using a for loop, you have more control over the loop’s flow and can include break statements within conditional statements to exit the loop at any desired point.
2. Using a for…of loop: Introduced in ES6, the for…of loop provides a concise alternative to the forEach loop. It allows for early breaks using the standard break statement, providing the flexibility necessary to exit the loop prematurely.
3. Using Array.prototype.every() method: The every() method is another option for breaking from a forEach loop-like iteration. The every() method tests whether all elements in the array pass a given condition and stops iterating as soon as any element fails the condition. By implementing a condition that always returns false after the desired break point, you can effectively terminate the loop.
Considerations and Best Practices:
1. Maintain readability: It is crucial to ensure that the code remains clear and understandable, especially when employing alternative approaches. Annotate the code with meaningful comments to explain the intention and purpose of the break.
2. Evaluate performance implications: While breaking from a forEach loop may be necessary in specific cases, it is essential to evaluate its impact on performance. In some instances, refactoring the code to remove break requirements or exploring other iteration methods might result in better performance.
3. Understand functional programming concepts: forEach loops align with functional programming paradigms that prioritize immutability and avoiding side effects. Ensure that breaking from the loop is necessary and adheres to functional programming principles.
FAQs:
Q1: Can I use the break statement within a forEach loop?
A1: No, the break statement cannot be used within a forEach loop. Iterating over the entire array is an inherent characteristic of forEach loops.
Q2: What alternatives can I use to break out of a forEach loop?
A2: You can leverage for loops, for…of loops, or the every() method to achieve early termination.
Q3: Will breaking from a forEach loop affect performance?
A3: Breaking from a loop might introduce minor performance implications, particularly when compared to the inherent efficiency of the forEach loop. However, evaluating the specific use case is crucial to determine the exact impact.
Q4: Are there limitations when it comes to breaking from a forEach loop in TypeScript?
A4: TypeScript follows JavaScript’s behavior, making it impossible to break from a forEach loop using the traditional break statement. However, alternative approaches mentioned earlier can be utilized.
Conclusion:
While the forEach loop in JavaScript does not include a built-in mechanism for breaking out prematurely, alternative methods and approaches can be employed to achieve this behavior. By understanding these options, developers gain more flexibility in controlling the flow of their code. It is essential to carefully analyze specific use cases and consider performance implications before implementing any of these approaches.
How To Use The Break Statement Inside The Array Foreach Method
Keywords searched by users: break from foreach javascript Break forEach JavaScript, Continue forEach js, Foreach break c#, Return in forEach js, Break for js, Every js, Jump target cannot cross function boundary, TypeScript forEach break
Categories: Top 47 Break From Foreach Javascript
See more here: nhanvietluanvan.com
Break Foreach Javascript
JavaScript is a powerful programming language widely used for developing interactive web pages and web applications. Among the numerous features it offers, the forEach method is an essential one for working with arrays. It allows developers to easily iterate through each element of an array and perform actions or apply functions to them. However, there are scenarios where breaking out of a forEach loop is necessary. In this article, we will explore the concept of breaking out of a forEach loop in JavaScript and provide a detailed explanation of various techniques and their implications.
Understanding forEach and Its Limitations:
The forEach method in JavaScript was introduced in ECMAScript 5. It provides a convenient way to iterate through each element of an array without the need for explicit loop control. By passing a callback function to forEach, developers can define the action to be performed on each element. However, the crucial limitation of forEach is that it does not support breaking out of the loop prematurely, like traditional for or while loops.
Why Breaking Out of a forEach Loop?
While forEach simplifies iterating over an array, there are cases where developers need to break out of the loop before finishing iteration. For instance, in scenarios where a specific condition is met, or when the desired element is found, developers may want to stop further iteration to avoid unnecessary computations or improve performance.
Breaking forEach Loop Using Exceptions:
One technique to break a forEach loop is using exceptions. By throwing an exception from within the callback function, the loop can be exited prematurely. However, this approach is not recommended as it goes against the principle of using exceptions solely for exceptional conditions. Additionally, it can lead to code complexity and reduced readability.
Using Array.prototype.some() or Array.prototype.every():
Another approach is to utilize the Array.prototype.some() or Array.prototype.every() methods as alternatives to forEach. These methods can act as a loop with the capability to break upon a specific condition. The some() method iterates through the array until the callback function returns true. If the callback returns false for each element, the iteration continues. On the other hand, the every() method stops when the callback returns false. By carefully crafting the callback function, developers can achieve the desired break behavior.
Implementing a Custom Loop:
If breaking out of a forEach loop is frequently required in a certain codebase, implementing a custom loop might be a more efficient solution. By creating a custom function, developers can introduce the break functionality while retaining the benefits of forEach. This approach provides greater control and readability, allowing the code to be easily understood by other developers.
FAQs:
1. Can we break out of a forEach loop in JavaScript?
While forEach does not offer built-in support for breaking out of the loop, there are alternative techniques available to achieve this behavior, such as using exceptions, Array.prototype.some(), or Array.prototype.every().
2. Is breaking out of a forEach loop good practice?
Breaking out of a forEach loop is generally discouraged, as forEach is designed to perform actions on each element without explicit control flow. Breaking out of the loop can lead to code complexity and decreased readability. It is advisable to leverage other looping constructs if frequent breaks are required.
3. Are there alternatives to forEach that support breaking out of a loop?
Yes, the Array.prototype.some() and Array.prototype.every() methods are alternatives to forEach that allow breaking out of a loop based on specific conditions. These methods can be utilized to achieve similar iteration patterns with break-like functionality.
4. What is the performance impact of breaking out of a forEach loop?
Breaking out of a forEach loop using exceptions or custom loops introduces additional computational overhead compared to the native forEach method. However, the impact depends on the specific implementation and the number of iterations involved. Consider benchmarking and profiling your code to evaluate the performance implications.
In conclusion, while the forEach method in JavaScript does not directly support breaking out of a loop, there are various techniques available to achieve this behavior. Developers must carefully consider the use case, code readability, and performance implications before utilizing alternative approaches. By understanding these techniques, developers can efficiently tackle scenarios where breaking out of a forEach loop is necessary, without compromising code quality.
Continue Foreach Js
In JavaScript, the forEach function is a powerful tool that allows you to iterate over elements in an array. It provides an easier and more elegant way to loop through arrays compared to traditional for loops or while loops. One particular scenario where this function becomes handy is when you want to skip or continue to the next iteration based on a certain condition. In this article, we will explore how to achieve this behavior using the continue statement in conjunction with the forEach function.
Understanding the forEach Function
Before we delve into using the continue statement, let’s review the basic functionality of the forEach function. The forEach function is a built-in method available on arrays in JavaScript. It takes in a callback function as an argument and executes that function once for each element in the array.
The callback function takes three arguments: the current element, the current index, and the array that forEach was called upon. With the forEach function, we can perform various operations on each element of an array without having to explicitly write a loop. It greatly simplifies the code and improves code readability.
Syntax of the forEach function:
“`js
array.forEach(function(currentValue, index, array) {
// Perform operation on currentValue
});
“`
Using continue with forEach
The continue statement is not supported natively in forEach loops. However, we can achieve similar behavior by using a return statement instead. When the return statement is encountered within the callback function, it immediately exits that iteration and moves on to the next element.
Let’s consider an example where we have an array of numbers and we want to iterate through the array to find and print only odd numbers. We can achieve this using the continue concept with the forEach function as follows:
“`js
const numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
numbers.forEach(function(number) {
if (number % 2 === 0) {
return; // Skip even numbers
}
console.log(number); // Print odd numbers
});
“`
In the code above, the callback function checks if the number is even using the modulo operator `%`. If the number is even, the return statement is encountered, causing the current iteration to be skipped. Consequently, only odd numbers are printed to the console.
FAQs
Q: Can we achieve the same outcome using traditional for loops instead of forEach?
A: Yes, it is possible to achieve similar behavior using traditional for loops. However, forEach provides a more concise and elegant way to achieve the same result, especially when working with arrays.
Q: Are there any performance differences between using continue in forEach and traditional for loops?
A: In most cases, the performance difference between continue in forEach and for loops is negligible. Modern JavaScript engines optimize forEach loops, making them perform competitively with for loops.
Q: Can we use continue with other looping constructs, such as map or reduce?
A: No, the continue statement is specific to loop constructs that support it, such as for and while. To skip iterations in map or reduce, you would need to use different techniques, such as returning null or undefined within the callback function.
Q: Is it possible to break out of a forEach loop prematurely?
A: The forEach loop does not support breaking out prematurely. If you need to stop the loop before all elements are processed, you would need to switch to a traditional for loop or use another looping construct like while or for…of.
Q: Can I modify array elements using continue with forEach?
A: Yes, you can modify array elements within the forEach loop by directly manipulating the elements or assigning new values to them. However, it’s important to exercise caution and ensure the modifications do not affect the loop’s behavior unintentionally.
Conclusion
The continue statement is a powerful tool for controlling the flow within loops. Although not directly supported in forEach, we explored how we can achieve similar behavior by using a return statement instead. By skipping specific iterations within a forEach loop, we can elegantly and efficiently perform operations on array elements based on various conditions. The forEach function, combined with continue-like behavior, simplifies code and enhances readability. Keep in mind the specific syntactical differences between continue and return when applying this concept.
Images related to the topic break from foreach javascript
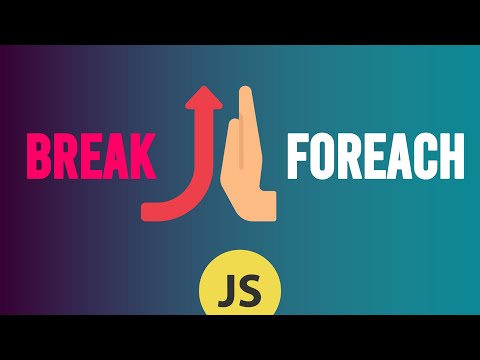
Found 7 images related to break from foreach javascript theme

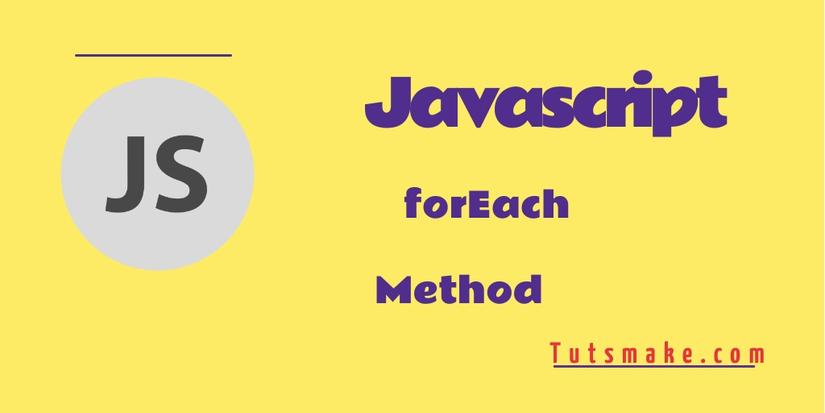
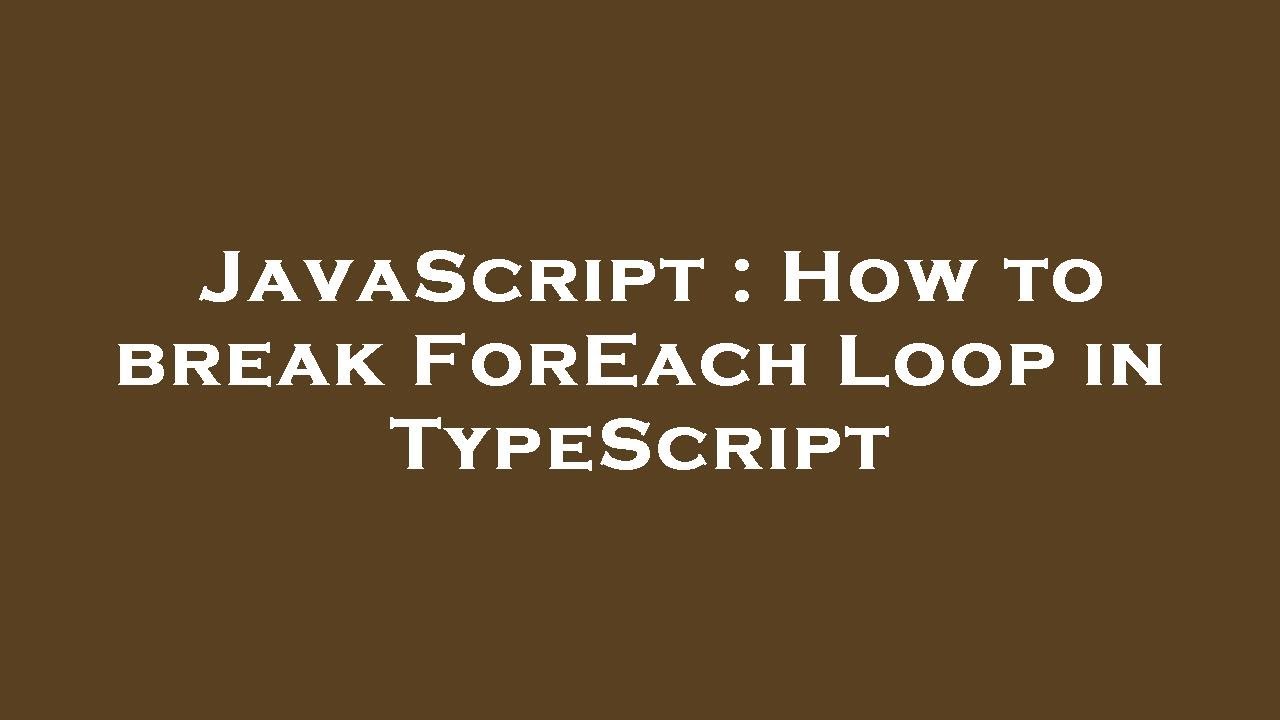



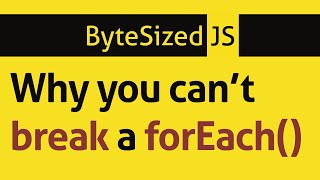
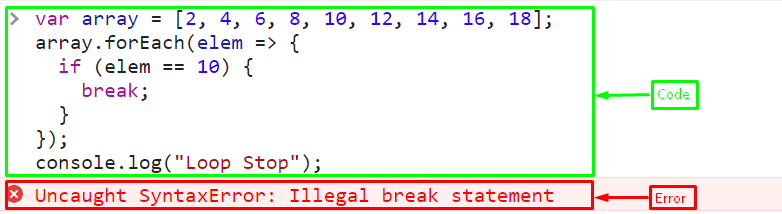


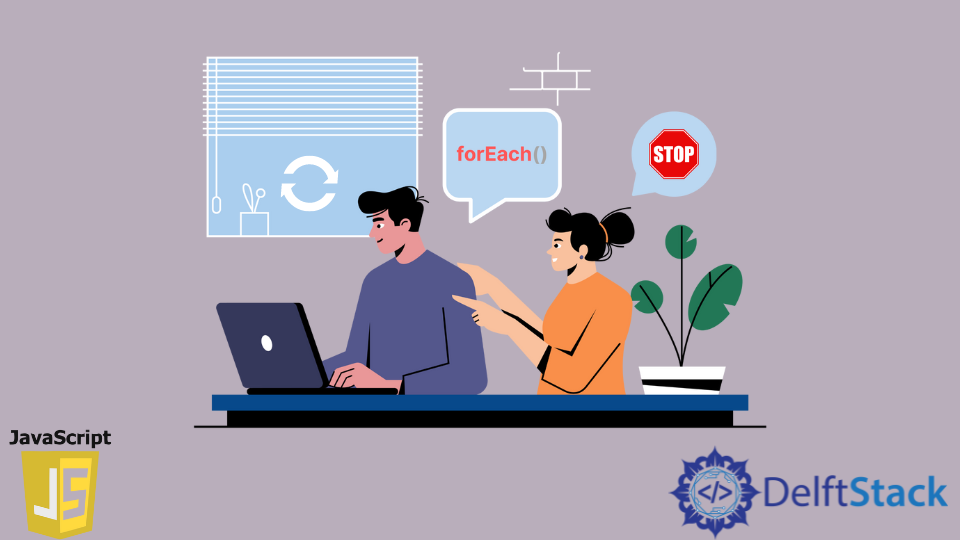
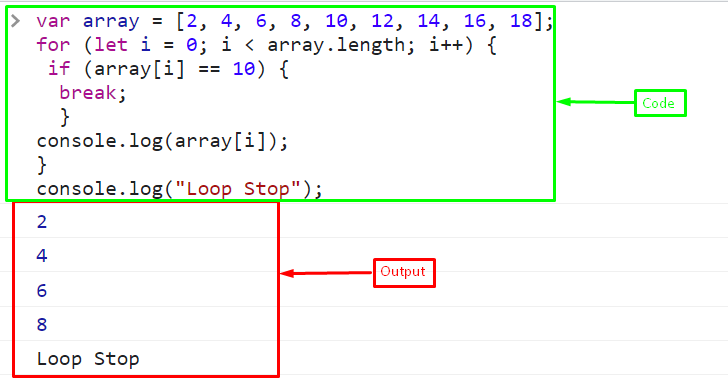

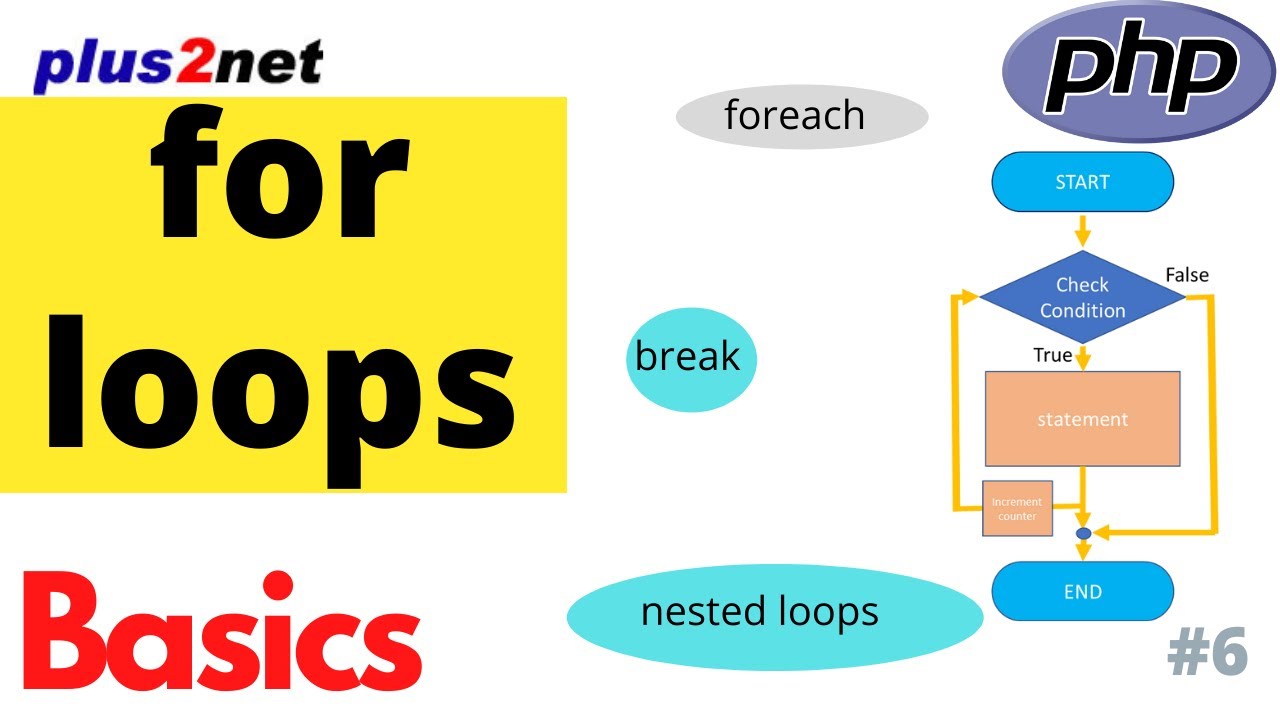




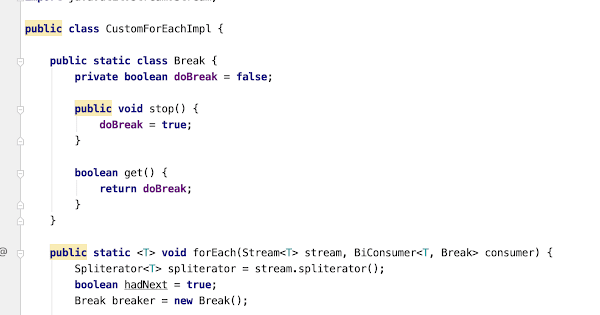


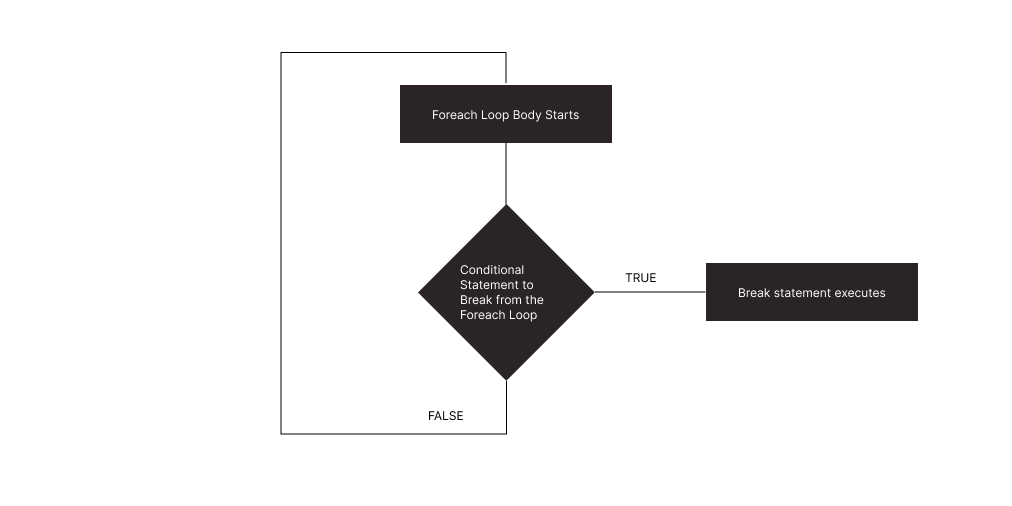

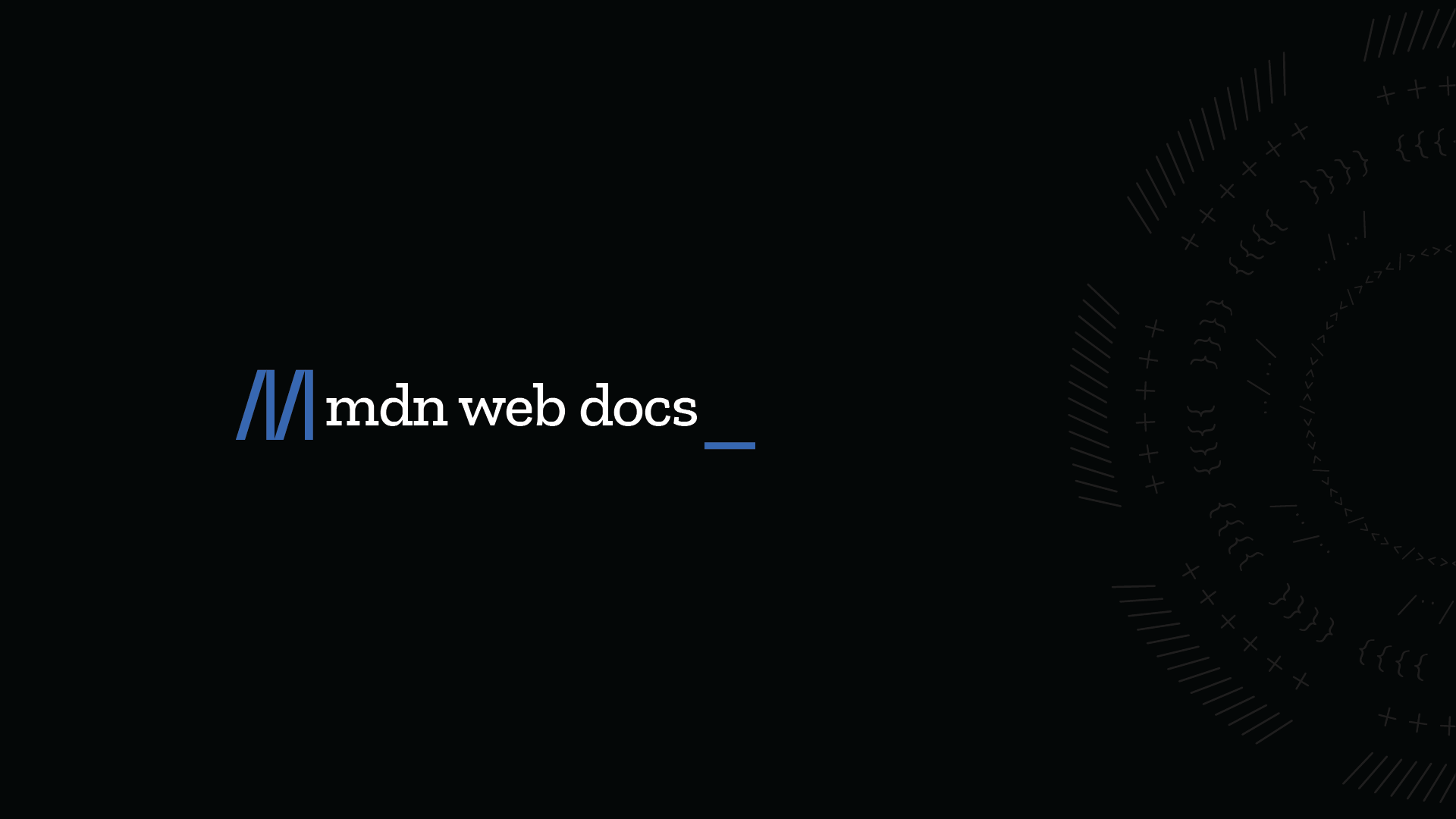
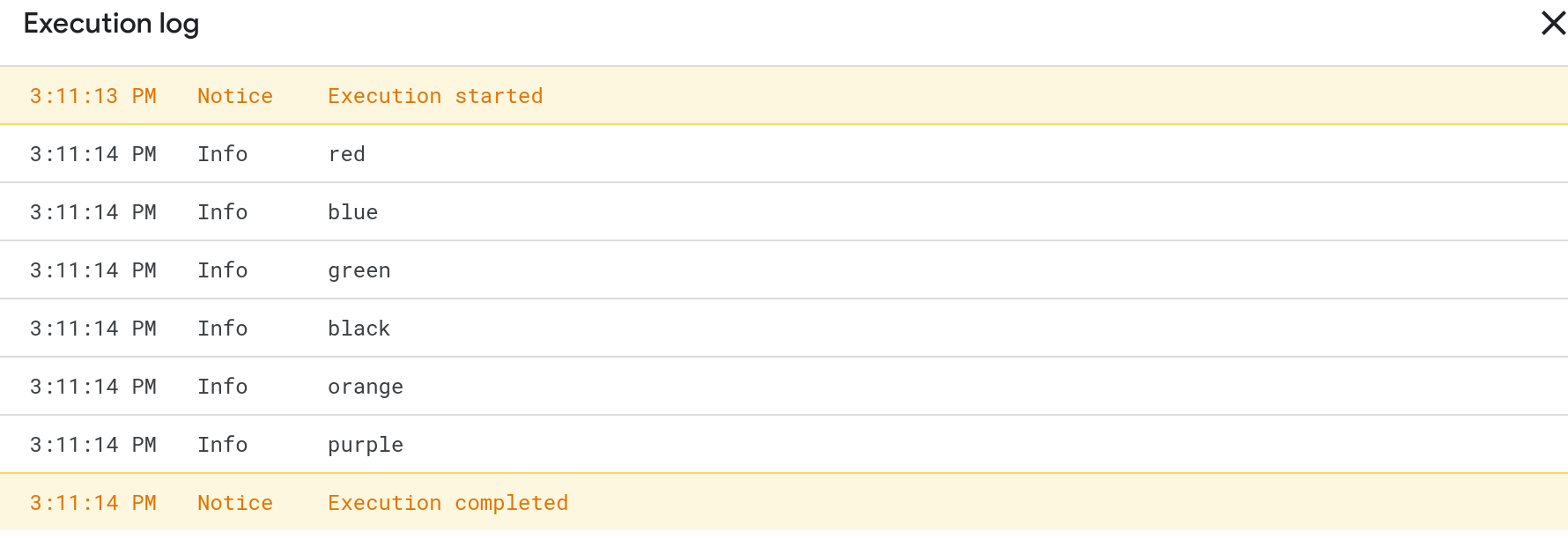
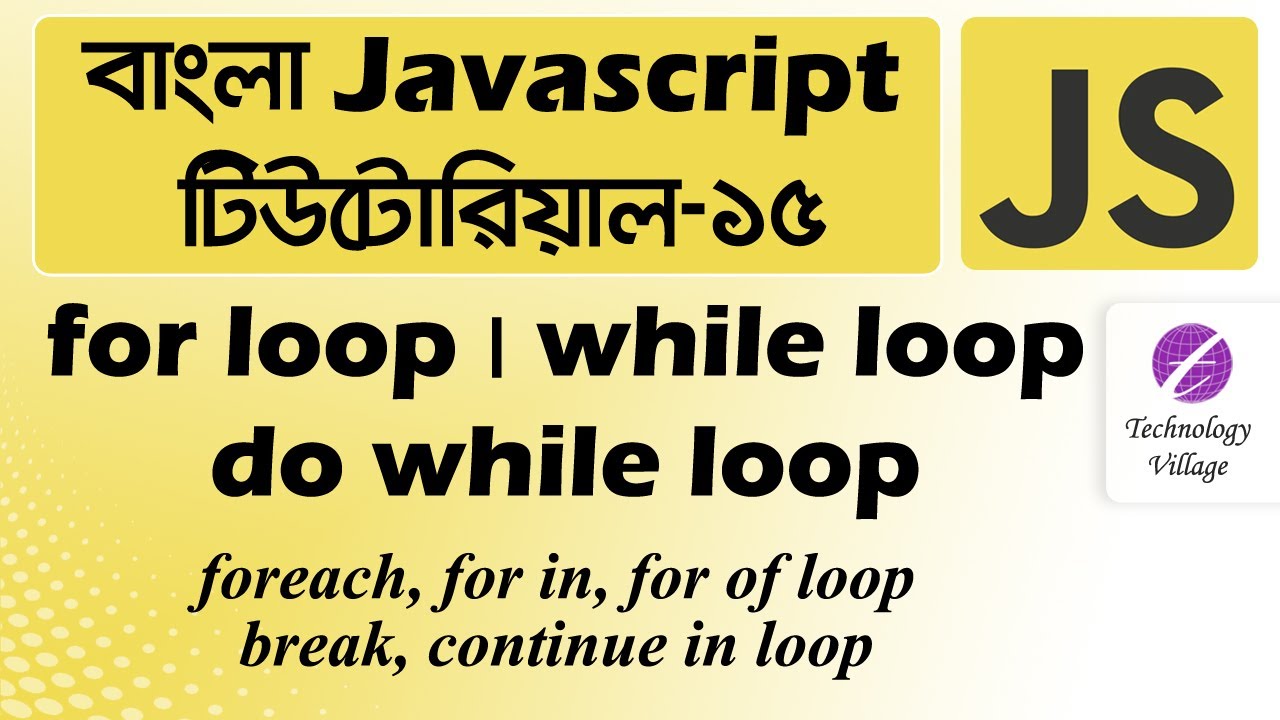

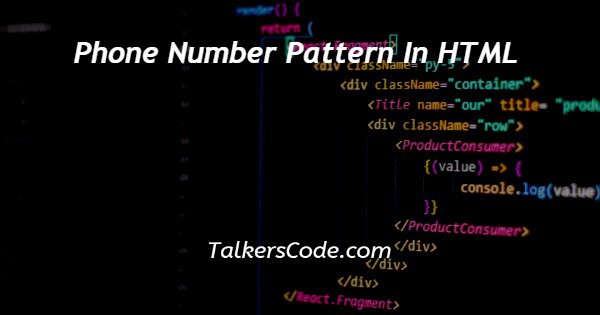

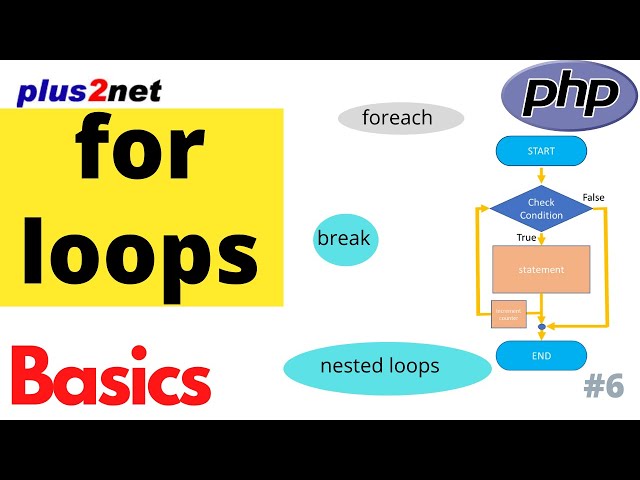
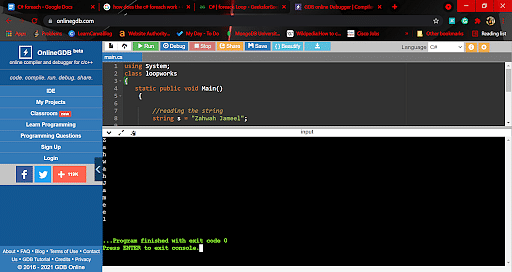
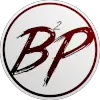

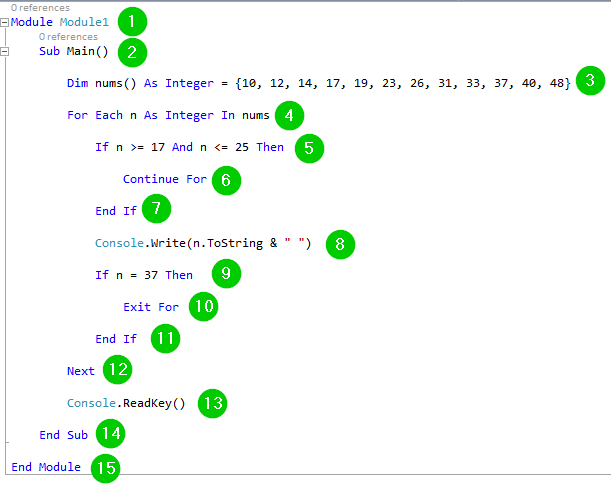
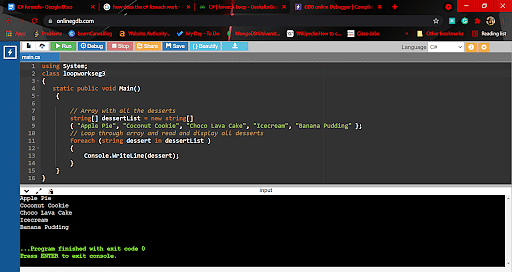
Article link: break from foreach javascript.
Learn more about the topic break from foreach javascript.
- How to Break Out of a JavaScript forEach() Loop – Mastering JS
- how to stop Javascript forEach? [duplicate] – Stack Overflow
- The Right Way to Break from forEach in JavaScript – Webtips
- How to stop forEach() method in JavaScript – Tutorialspoint
- How to exit a forEach Loop in Javascript – Techozu
- Tutorials How to Exit, Stop, or Break an Array#forEach Loop in …
- 3 things you didn’t know about the forEach loop in JS – Medium
- How to Stop JavaScript forEach? – Linux Hint
- How to stop forEach() method in JavaScript ? – GeeksforGeeks
- How to break out of forEach in JavaScript – Sabe.io
See more: https://nhanvietluanvan.com/luat-hoc/