Because The Type Requires A Json Object
JSON (JavaScript Object Notation) is a lightweight data-interchange format that is easy for humans to read and write and easy for machines to parse and generate. It is commonly used for transmitting data between a server and a web application as an alternative to XML. JSON objects are at the heart of this data format and understanding their structure and usage is essential. In this article, we will explore JSON objects in detail, covering their structure, supported data types, creation, manipulation, validation, and examples of practical usage.
Exploring the Structure of a JSON Object
A JSON object is represented by a collection of key-value pairs enclosed within curly braces {}. The keys are strings, and the values can be of various data types such as strings, numbers, booleans, arrays, or even nested JSON objects. Here’s an example of a simple JSON object:
{
“name”: “John Doe”,
“age”: 25,
“isEmployed”: true
}
In this example, “name,” “age,” and “isEmployed” are the keys, and their corresponding values are “John Doe,” 25, and true, respectively. The keys and values are separated by a colon, and each key-value pair is separated by a comma.
Data Types Supported in JSON Objects
JSON objects support several data types, including:
1. Strings: Represented by double quotes, e.g., “Hello, World!”
2. Numbers: Integer or floating-point values, e.g., 42 or 3.14
3. Booleans: True or false
4. Arrays: Ordered collections of values enclosed within square brackets [], e.g., [1, 2, 3]
5. Nested JSON Objects: JSON objects can be nested within other JSON objects, allowing for hierarchical structures
Creating a JSON Object
There are several ways to create a JSON object. One common approach is to build it manually using string concatenation or interpolation. However, in most programming languages, libraries or built-in functions are available to simplify the process.
For example, in C#, you can use the Newtonsoft.Json library to create JSON objects programmatically. Here’s an example of creating the same JSON object mentioned earlier using Newtonsoft.Json:
“`csharp
JObject jsonObject = new JObject();
jsonObject[“name”] = “John Doe”;
jsonObject[“age”] = 25;
jsonObject[“isEmployed”] = true;
“`
Accessing and Manipulating JSON Objects
Once you have a JSON object, you can access its values by referencing the corresponding keys. In C#, you can use the GetValue or GetValue
“`csharp
string name = (string)jsonObject.GetValue(“name”);
int age = (int)jsonObject.GetValue(“age”);
bool isEmployed = (bool)jsonObject.GetValue(“isEmployed”);
“`
You can also modify the values of a JSON object by assigning new values to its keys:
“`csharp
jsonObject[“name”] = “Jane Smith”;
“`
Validating JSON Objects
Validating JSON objects involves ensuring that they adhere to the expected structure and data types. One way to validate a JSON object is by using JSON schema validation. A JSON schema defines the expected structure and properties of a JSON object.
Various tools and libraries, such as Ajv for JavaScript or Newtonsoft.Json.Schema for C#, provide capabilities to validate JSON objects against a JSON schema.
Examples of Using JSON Objects in Practice
JSON objects find extensive usage in various applications and APIs. They are commonly used for data exchange between client-server communication or transmitting structured data over HTTP.
For example, a weather API might return weather information as a JSON object:
“`json
{
“temperature”: 25,
“humidity”: 70,
“conditions”: “Sunny”
}
“`
A web application can retrieve this JSON object and display the weather information to the user.
FAQs:
Q: What is the meaning of “Cannot deserialize the current JSON object” error?
A: This error typically occurs when trying to deserialize a JSON array into an object or vice versa. It indicates a mismatch between the expected JSON structure and the actual JSON data.
Q: How can I force deserialization from a JSON object using JsonPropertyAttribute?
A: You can use the JsonPropertyAttribute from the Newtonsoft.Json library to explicitly specify the property name during deserialization. By applying this attribute to the corresponding property, you can ensure that it is deserialized correctly from the JSON object.
Q: How can I deserialize JSON to a C# object?
A: In C#, you can use libraries like Newtonsoft.Json to deserialize JSON into C# objects. By defining a class with properties corresponding to the JSON structure, you can use the library’s deserialization methods to easily convert JSON into C# objects.
Q: What is the ValueKind = object in C# JSON?
A: ValueKind = object is a property of the System.Text.Json.JsonElement class in C#. It represents that the JSON value is an object and can be accessed and manipulated accordingly.
Q: How can I deserialize JSON to a dynamic object in C#?
A: In C#, you can use the Newtonsoft.Json library to deserialize JSON into a dynamic object. By using the dynamic keyword, you can assign the JSON object to a variable and access its properties without defining a specific class structure. However, this approach sacrifices compile-time type checking.
Learn Json In 10 Minutes
How To Deserialize The Json Object In C#?
JSON (JavaScript Object Notation) is a lightweight data interchange format that has gained popularity due to its simplicity and compatibility with a wide range of programming languages. In C#, deserialization refers to the process of converting a JSON string into a .NET object. This article will guide you through the steps to deserialize a JSON object in C# and provide some insights into best practices and common questions regarding this process.
1. Understand the JSON Structure
Before diving into the deserialization process, it is important to understand the structure of the JSON object you will be working with. JSON objects consist of key-value pairs enclosed within curly braces. The values can be simple data types (strings, numbers, booleans, null) or complex objects and arrays. Familiarize yourself with the JSON structure to effectively deserialize it into a .NET object.
2. Import Required Libraries
To deserialize a JSON object in C#, you need to import the System.Text.Json namespace, which is included in the .NET Core runtime. By using this library, you have access to various classes and methods to perform efficient JSON deserialization.
“`csharp
using System.Text.Json;
“`
3. Create a Class Structure
To deserialize a JSON object, you need to create a class structure that matches the JSON structure. Each property in the class should have the same name and data type as the corresponding key in the JSON object. Consider the following JSON object as an example:
“`json
{
“name”: “John Doe”,
“age”: 30,
“city”: “New York”
}
“`
To deserialize this object, create a class as follows:
“`csharp
public class Person
{
public string Name { get; set; }
public int Age { get; set; }
public string City { get; set; }
}
“`
4. Deserialize the JSON Object
Now that you have the class structure ready, you can proceed with the deserialization process. The System.Text.Json namespace provides a JsonSerializer class that you can use to deserialize the JSON object.
“`csharp
string jsonString = “{\”name\”:\”John Doe\”,\”age\”:30,\”city\”:\”New York\”}”;
Person person = JsonSerializer.Deserialize
“`
In this example, the `jsonString` variable contains the JSON object in string format. By calling `JsonSerializer.Deserialize
5. Access the Deserialized Object
Once the deserialization is complete, you can access the properties of the deserialized object as you would with any other object. For example, to access the name property of the Person object, use `person.Name`.
“`csharp
Console.WriteLine(person.Name);
“`
This will output “John Doe” in the console.
FAQs:
Q1. Can I deserialize JSON arrays using the same approach?
A1. Yes, JSON arrays can be deserialized in C# using the same approach. You need to create a class structure that represents the elements of the JSON array and deserialize it accordingly.
Q2. How can I handle optional properties in the JSON object?
A2. To handle optional properties in the JSON object, you can mark the corresponding properties in your class with the `[JsonPropertyName(“propertyName”)]` attribute and set a default value. This way, if the property is missing in the JSON object, the deserializer will assign the default value.
Q3. How can I deserialize JSON objects with nested structures?
A3. When dealing with nested JSON objects, create separate classes for each nested structure and define the appropriate relationships between them. The deserialization process will automatically map the nested properties based on the class structure.
Q4. Can I customize the deserialization process?
A4. Yes, you can customize the deserialization process by implementing the `JsonConverter
Q5. Are there any performance considerations when deserializing large JSON objects?
A5. When deserializing large JSON objects, it is recommended to use the `JsonDocument` class instead of direct deserialization into a .NET object. This allows you to parse and access specific parts of the JSON object without fully deserializing the entire object into memory, which can improve performance and reduce memory usage.
In conclusion, deserializing JSON objects in C# is a straightforward process using the System.Text.Json namespace. By understanding the structure of the JSON object, creating a class structure, and utilizing the JsonSerializer class, you can easily convert JSON strings into .NET objects. Keep in mind the best practices and consider any special cases or customization requirements to handle various scenarios effectively.
How To Convert String To Json In C#?
JSON (JavaScript Object Notation) is a widely used data-interchange format that is easy to read and write. It is commonly used to transfer data between a server and a web application, as well as for storing configuration data. In C#, converting a string to JSON can be accomplished using various methods provided in the .NET framework. In this article, we will explore these methods and discuss how to convert a string to JSON in C#.
Methods to Convert String to JSON in C#
1. Using the Newtonsoft.Json Package:
The Newtonsoft.Json package (also known as Json.NET) is a popular and widely used library for working with JSON data in .NET applications. It provides various methods to deserialize JSON data from a string. To start, you need to ensure that the Newtonsoft.Json NuGet package is added to your project.
Here is an example of how to convert a string to JSON using the Newtonsoft.Json package:
“`csharp
using Newtonsoft.Json;
string jsonString = “{\”name\”:\”John\”, \”age\”:30, \”city\”:\”New York\”}”;
dynamic jsonObj = JsonConvert.DeserializeObject(jsonString);
“`
In the above code snippet, we first define a JSON string. By calling the `JsonConvert.DeserializeObject` method and passing the JSON string as a parameter, we can convert it to a dynamic object (`dynamic jsonObj`). The Newtonsoft.Json package automatically maps the JSON string’s properties to corresponding dynamic object properties.
2. Using the System.Text.Json Namespace:
Starting from .NET Core 3.0, the `System.Text.Json` namespace is introduced as the primary JSON library for working with JSON data. Using this namespace, you can serialize and deserialize JSON without any external dependency on third-party packages. Here is an example of how to convert a string to JSON using the `System.Text.Json` namespace:
“`csharp
using System.Text.Json;
string jsonString = “{\”name\”:\”John\”, \”age\”:30, \”city\”:\”New York\”}”;
JsonDocument jsonDoc = JsonDocument.Parse(jsonString);
dynamic jsonObj = null;
if (jsonDoc.RootElement.ValueKind == JsonValueKind.Object)
{
jsonObj = jsonDoc.RootElement;
}
“`
In the above example, we first parse the JSON string using the `JsonDocument.Parse` method provided by the `System.Text.Json` namespace. If the parsed JSON is an object, we assign it to the dynamic object (`dynamic jsonObj`). The `JsonValueKind` property allows us to determine the type of JSON value being parsed.
Frequently Asked Questions (FAQs):
Q1. What is the difference between Newtonsoft.Json and the System.Text.Json namespace?
The Newtonsoft.Json package (Json.NET) has been around for a longer time and is widely used in existing .NET applications. It provides extensive features, flexibility, and customization options. On the other hand, the `System.Text.Json` namespace is a built-in JSON library introduced in .NET Core 3.0, offering improved performance and reduced memory allocations. It is recommended to use `System.Text.Json` for new projects unless specific requirements or backward compatibility necessitate using Newtonsoft.Json.
Q2. Can I convert a JSON string to a strongly typed object in C#?
Yes, you can convert a JSON string to a strongly typed object in C#. Both the Newtonsoft.Json and the `System.Text.Json` namespaces provide methods to deserialize JSON into strongly typed objects. These methods automatically map the JSON properties to corresponding object properties, which simplifies working with JSON data in a type-safe manner.
Q3. How can I handle JSON parsing errors while converting a string to JSON?
Both Newtonsoft.Json and `System.Text.Json` provide error handling mechanisms for handling JSON parsing errors. They raise exceptions when the JSON data or format is invalid. You can use `try-catch` blocks to catch these exceptions and handle them accordingly. Additionally, you can use the error detection methods provided by these libraries to detect and handle JSON parsing errors programmatically.
Q4. Is JSON data only used in web applications?
While JSON data is commonly used in web applications, it is not limited to just web-related scenarios. JSON can be utilized in any system or application where lightweight and human-readable data interchange is desired. It is extensively used in both client-side and server-side applications, mobile apps, and microservices because of its simplicity and compatibility with multiple programming languages.
In conclusion, converting a string to JSON in C# is a straightforward process, and you have multiple choices available depending on your requirements and the .NET framework version you are working with. The Newtonsoft.Json package and the `System.Text.Json` namespace provide robust methods to deserialize a JSON string into dynamic or strongly typed objects. By understanding these methods and using proper error handling techniques, you can efficiently work with JSON data in your C# applications.
Keywords searched by users: because the type requires a json object Cannot deserialize the current JSON object, Cannot deserialize the current JSON array, Jsonobjectattribute can also be added to the type to force it to deserialize from a JSON object, Newtonsoft JSON jsonserializationexception Cannot deserialize the current JSON array, C# JSON to object, JSON to C# sharp class, ValueKind = object C#, JSON to dynamic object C#
Categories: Top 84 Because The Type Requires A Json Object
See more here: nhanvietluanvan.com
Cannot Deserialize The Current Json Object
#### Understanding JSON
Before we delve into the concept of deserialization and the associated error, let’s briefly discuss JSON (JavaScript Object Notation). JSON is a lightweight data-interchange format that is widely used to transmit data between a server and a web application. It is human-readable and easy for both humans and machines to understand.
JSON consists of key-value pairs, where keys are always in double quotes, and values can be of various types such as strings, numbers, booleans, arrays, or even other JSON objects. Here’s an example of a JSON object:
“`json
{
“name”: “John Doe”,
“age”: 30,
“isStudent”: true,
“courses”: [
“Math”,
“Science”,
“History”
]
}
“`
#### Deserialization and Its Purpose
Deserialization is the process of converting a JSON string into an object of a specific class or data type. This transformation allows developers to easily work with the data in a structured manner, rather than treating it as a raw string. Deserialization is often used when retrieving data from an external source, such as a web API, and mapping it to the corresponding object model.
#### The Error: Cannot deserialize the current JSON object
The error message “Cannot deserialize the current JSON object” typically occurs when the JSON string being deserialized does not match the expected format or structure of the target object. This can happen due to various reasons:
1. Incorrect JSON Structure: The JSON string may have missing or mismatched key-value pairs, or there may be extra elements that the deserialization process does not expect. Ensure that the JSON string adheres to the expected structure defined by the target object.
2. Incompatible Data Types: If the data types of the JSON values do not match the corresponding properties of the object being deserialized, the deserialization process will fail. For example, if a JSON string contains a string value for a property that expects an integer, deserialization will raise an error.
3. Missing or Invalid Deserialization Attributes: Some programming languages require special annotations or attributes to be added to the target class or properties to inform the deserialization process. If these attributes are missing or incorrectly specified, the deserialization may fail.
4. Nested Objects or Arrays: If the JSON string contains nested objects or arrays, it is essential to ensure that the structure and data types of these nested elements match the expected format defined by the target object.
#### Handling the Error
When encountering the “Cannot deserialize the current JSON object” error, several steps can be taken to fix the issue:
1. Validate JSON Structure: Carefully examine the JSON string being deserialized and compare it with the expected structure. Ensure that all key-value pairs are correctly formatted, and all necessary elements are present.
2. Check Data Types: Verify that the data types of JSON values match the corresponding properties of the target object. Make sure that integer values are not passed as strings, and vice versa.
3. Verify Serialization Attributes: In some programming languages, such as C#, specific attributes like `[JsonProperty]` or `[DataMember]` need to be added to the target class or properties. Ensure that these attributes are present and correctly configured.
4. Handle Nested Objects or Arrays: If the JSON string contains nested objects or arrays, ensure that their structure and data types align with the expected format. Consider creating appropriate classes or data models for these nested elements and adjusting the target object’s structure accordingly.
#### FAQs
**Q: Can the “Cannot deserialize the current JSON object” error occur when serializing data?**
A: No, this error specifically relates to deserialization. When serializing data, the error message might be different if there are any issues.
**Q: How can I verify the expected JSON structure for deserialization?**
A: The expected JSON structure can typically be obtained from the API documentation or by examining the object model used for deserialization in your code.
**Q: Are there any automated tools available to help with JSON deserialization?**
A: Yes, some programming languages provide libraries or frameworks that handle JSON deserialization automatically. For instance, in Java, libraries like Jackson or Gson provide convenient methods for deserializing JSON strings.
**Q: How can I troubleshoot the “Cannot deserialize the current JSON object” error?**
A: Review your code carefully, double-check the JSON structure, data types, attributes, and any nested objects or arrays. Debugging tools and examining the error message stack trace can also provide valuable insights.
**Q: Are there any performance implications with JSON deserialization?**
A: Deserialization can be a computationally expensive task, especially when dealing with large volumes of data. It’s important to consider performance optimizations, such as using streaming deserialization methods or selectively deserializing only the required data.
In conclusion, the “Cannot deserialize the current JSON object” error often arises due to inconsistencies between the expected JSON structure and the actual JSON string being deserialized. By carefully examining the JSON structure, validating data types, and reviewing deserialization attributes, developers can effectively troubleshoot and resolve this common error.
Cannot Deserialize The Current Json Array
JSON (JavaScript Object Notation) has become the go-to data format for many web-based applications due to its simplicity and flexibility. It allows data to be exchanged between a client and a server in a structured and easily readable manner. However, when working with JSON, you may encounter an error that reads: “Cannot deserialize the current JSON array.”
In this article, we will delve into the possible causes of this error and provide a comprehensive understanding of how to tackle the issue. We will explore various scenarios, potential solutions, and common FAQS to help you troubleshoot effectively.
Understanding the Error:
The error message “Cannot deserialize the current JSON array” typically occurs when attempting to convert a JSON array into an object. Deserialization is the process of converting JSON data into a structured object in the programming language being used.
When a JSON array is expected to be deserialized into a single object, the error occurs if the JSON data consists of an actual array instead. This mismatch causes the deserialization process to fail, resulting in the error message.
Potential Causes of the Error:
1. Invalid JSON structure: Check if the JSON being passed is indeed an array and not a single object. The deserialization process expects a single object, so double-check the JSON structure.
2. Typo or inconsistency: Ensure the appropriate property names and keys are used in both the JSON and the class/object that is being deserialized. Any mismatch can lead to the error.
3. Array field within an object: If you have a JSON object that contains an array as one of its fields, make sure the appropriate object structure and data types are defined to handle the array during deserialization.
4. Mixed data types: Verify that the data types of the JSON array’s elements match the expected types during deserialization. If there is a mismatch, the deserialization process will fail.
5. Null values: Null values within the JSON array can also cause the error. Make sure your code can handle and interpret null values appropriately during deserialization.
Possible Solutions:
1. Check the JSON data: Review the JSON data being passed to ensure it adheres to the expected structure. Validate the JSON using an online JSON validator to identify any syntax errors or inconsistencies.
2. Verify object structure: Compare the structure of the JSON data with the class/object you are trying to deserialize into, ensuring they match precisely. Pay close attention to property names, keys, and data types.
3. Handle array fields: If dealing with an object that contains an array field, ensure that both the object and the array are properly defined in the code. Utilize collections or lists in the appropriate object property to handle the expected array during deserialization.
4. Handle mixed data types: If your JSON array contains mixed data types, you may need to consider using a more flexible data type, such as `object` or `JToken`, to handle the variability during deserialization.
5. Null value handling: Modify your code to handle null values within the JSON array. Use conditional statements or converters to handle null cases explicitly.
FAQs:
Q1: Can’t I simply convert the JSON array into a JSON object to avoid the error?
A1: Converting a JSON array into a JSON object would result in a different data structure altogether. You would need to evaluate the requirements and, if necessary, adjust both the JSON structure and the deserialization process accordingly.
Q2: Can this error be caused by server-side issues?
A2: While the “Cannot deserialize the current JSON array” error is generally related to deserialization on the client side, server-side issues can indirectly contribute to the error. Ensure the server is returning the expected JSON structure and that it aligns with what your client-side code expects.
Q3: How can I debug this error in my code?
A3: Debugging the error involves carefully inspecting the JSON data, checking for any inconsistencies, examining class/object structures, and verifying data types. You can start by printing the JSON data, inspecting any parsing or deserialization methods, and running through the code step by step.
In conclusion, the “Cannot deserialize the current JSON array” error occurs when attempting to convert a JSON array into an object during deserialization. By understanding the potential causes and employing the appropriate solutions, you can resolve this error and ensure smooth JSON handling in your applications. Always double-check the JSON structure, verify object structures, handle array fields, account for mixed data types, and appropriately handle null values during deserialization to avoid encountering this error.
Images related to the topic because the type requires a json object
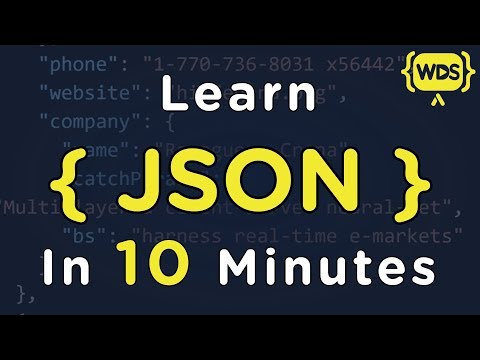
Found 28 images related to because the type requires a json object theme
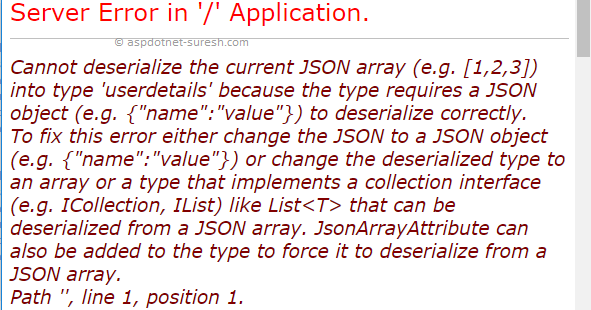





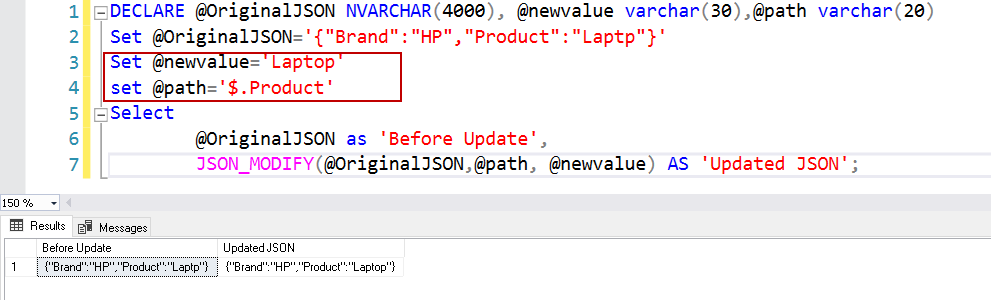
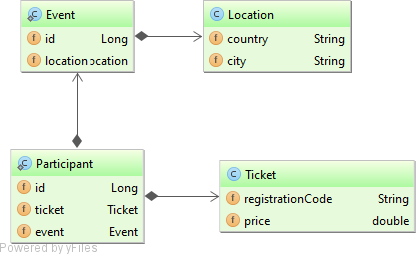
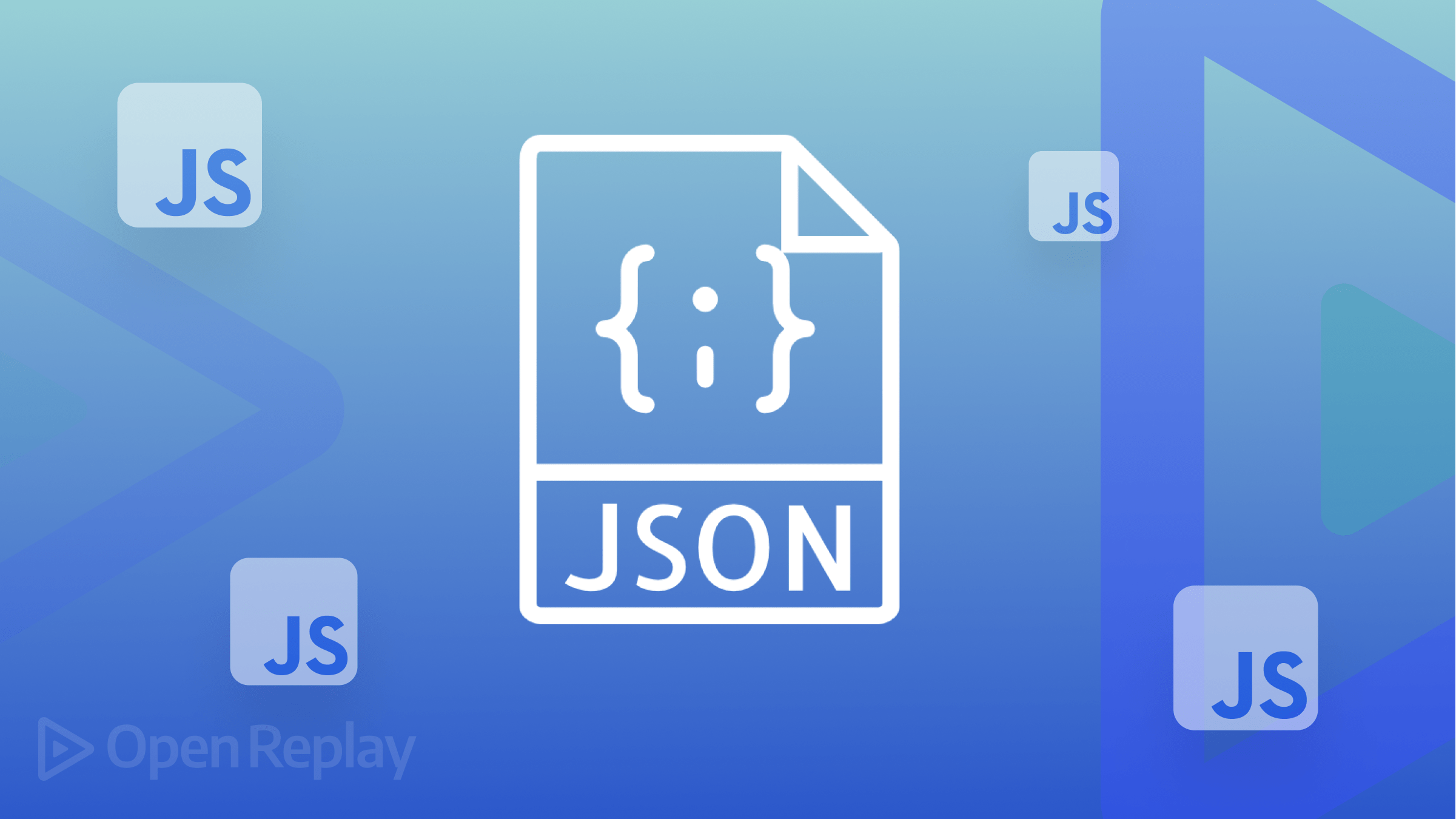

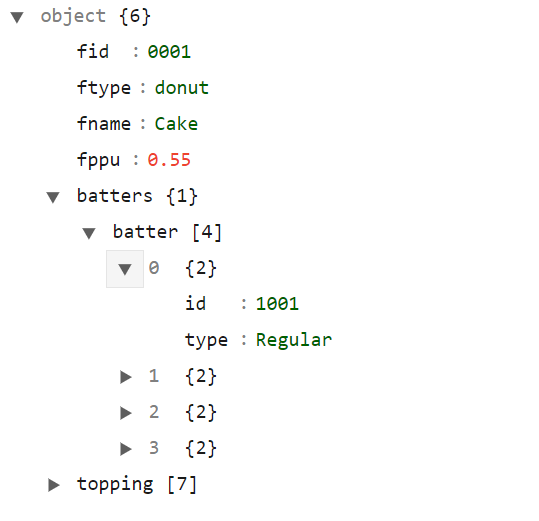


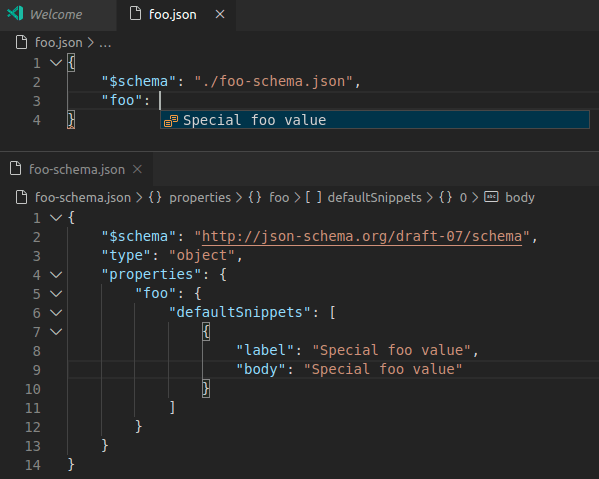
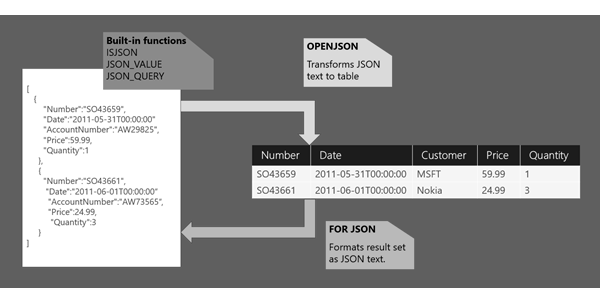
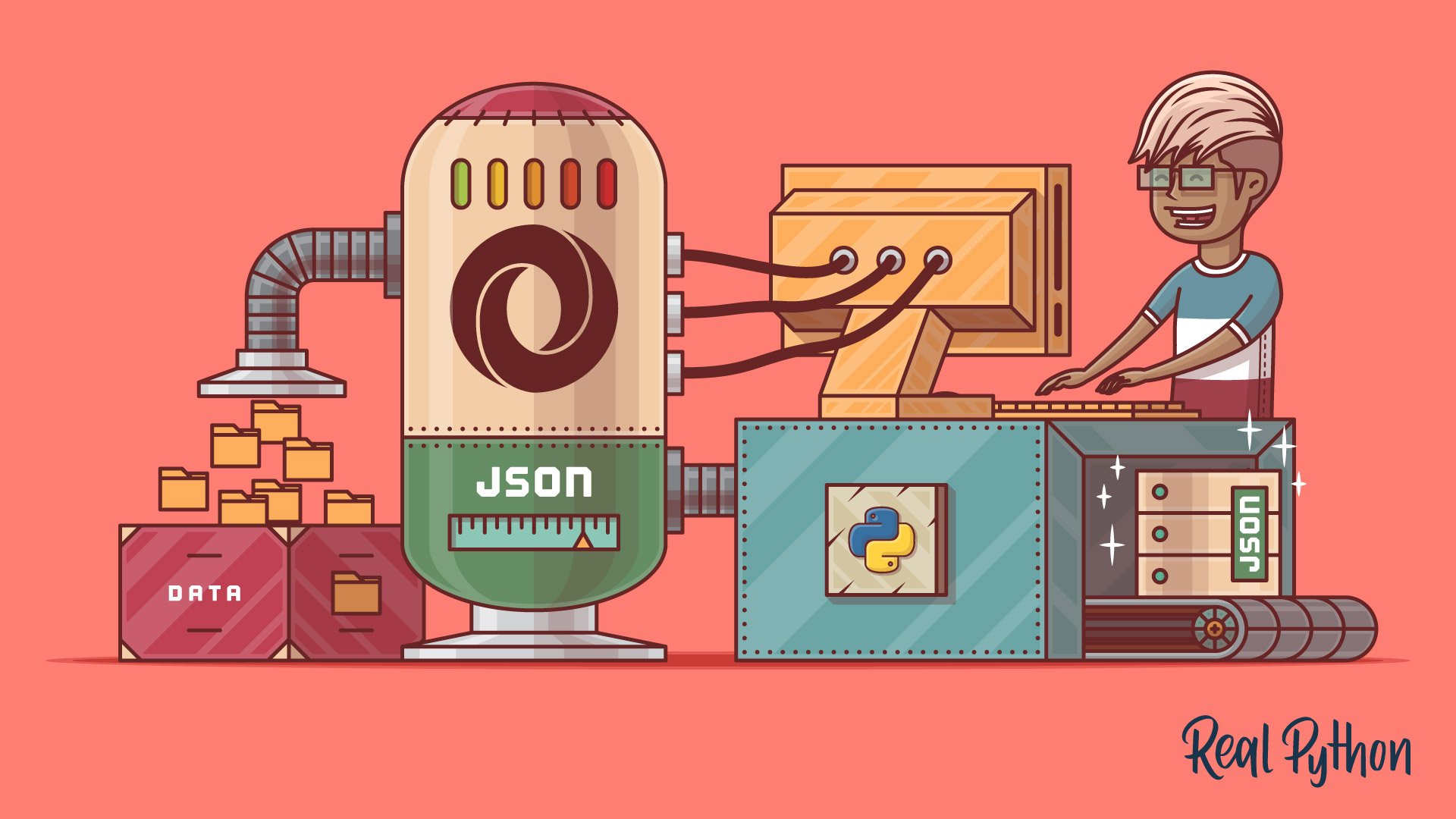

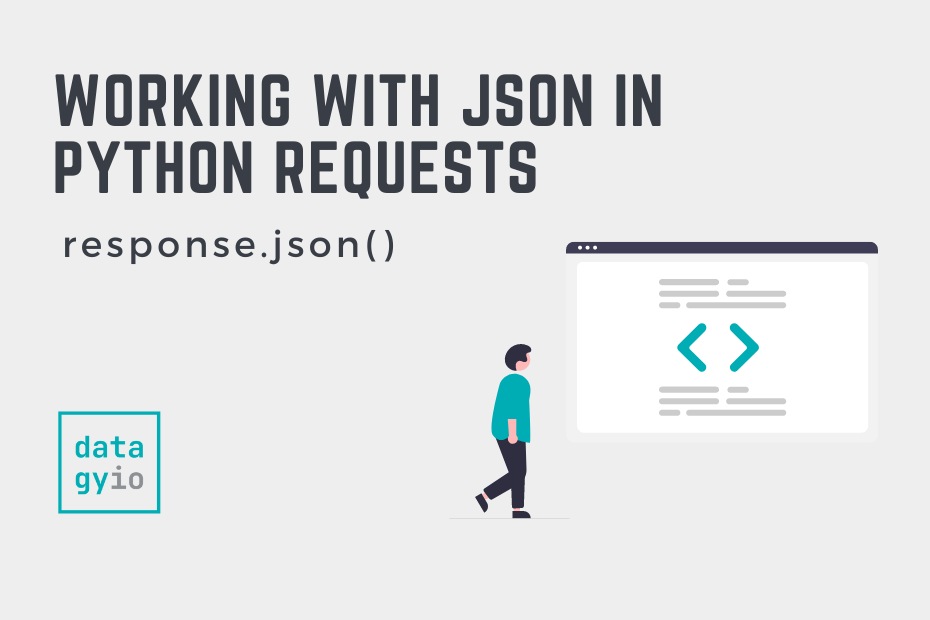





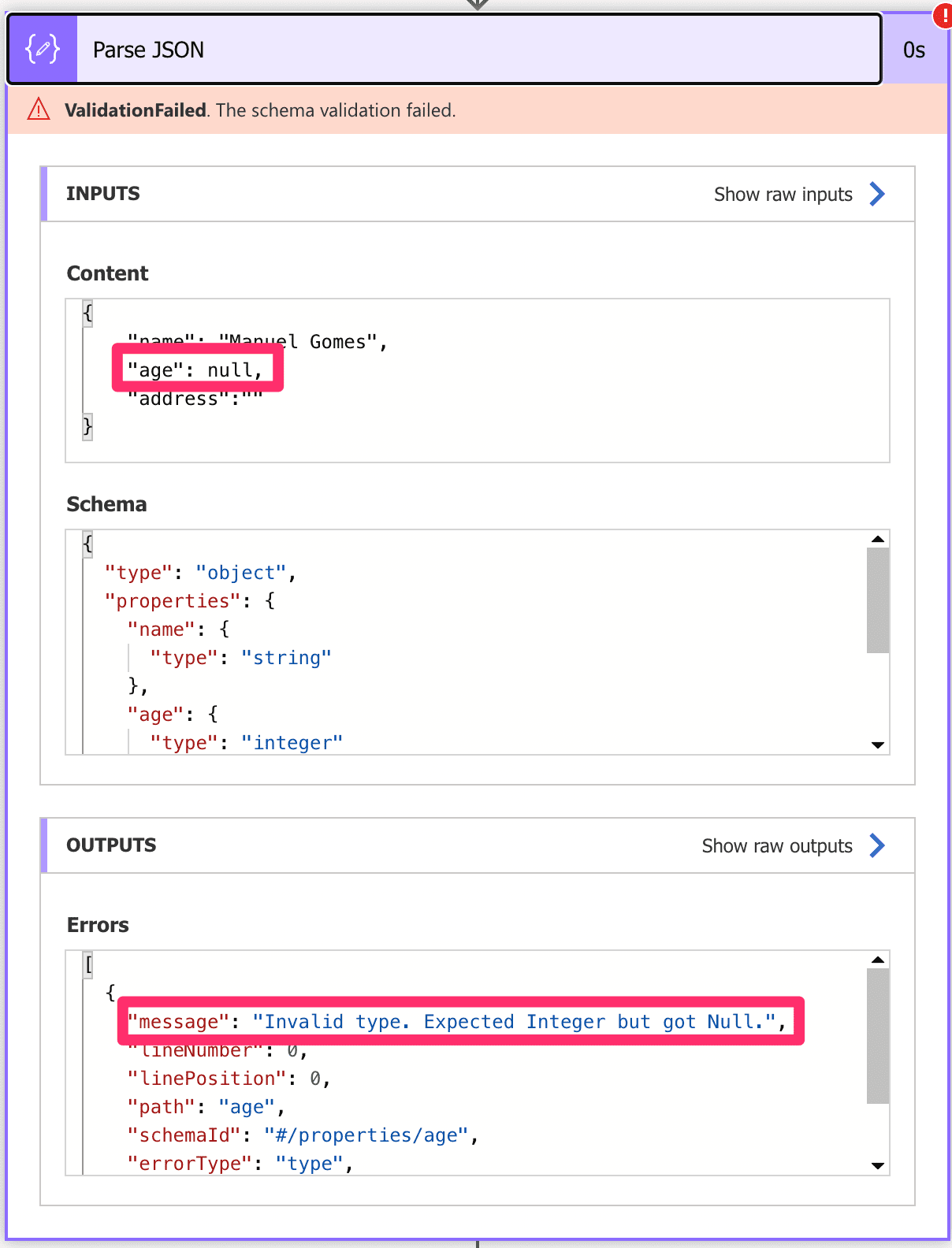
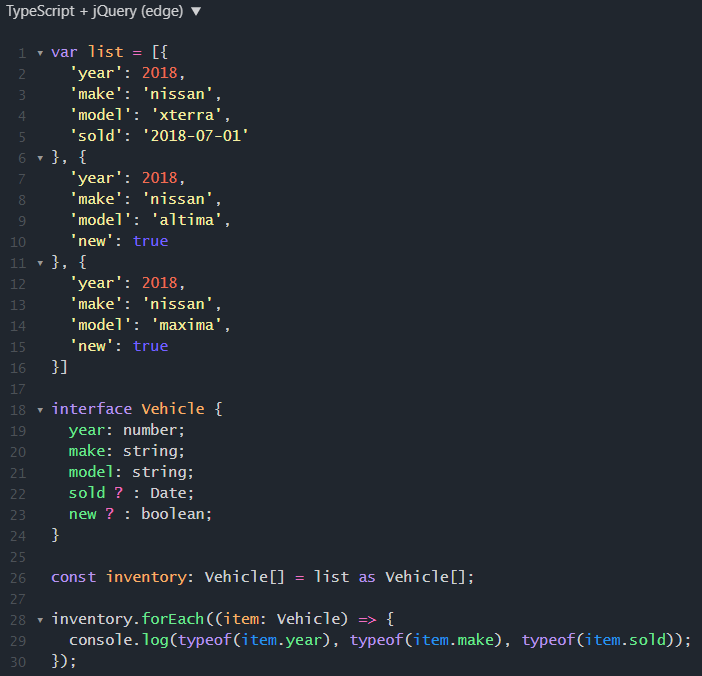

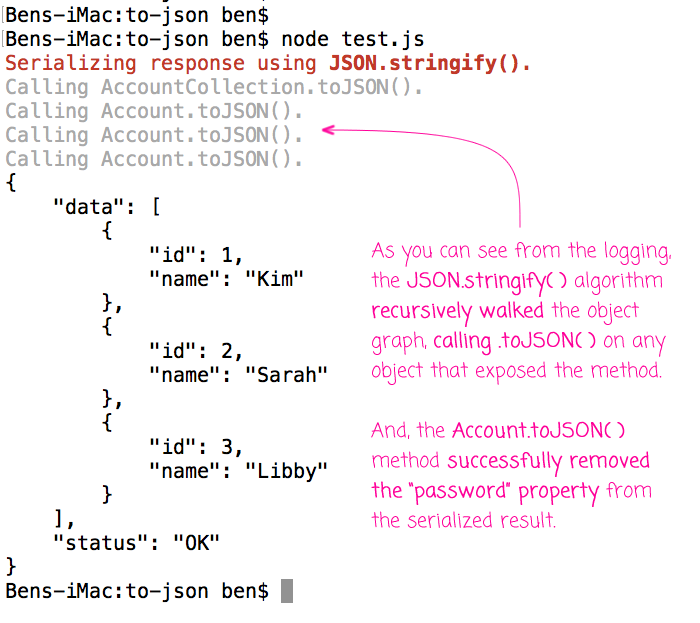




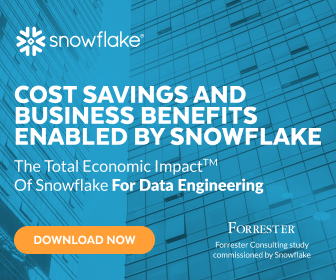
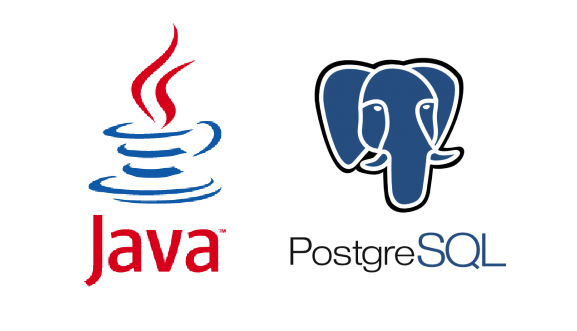
Article link: because the type requires a json object.
Learn more about the topic because the type requires a json object.
- Cannot deserialize the JSON array (e.g. [1,2,3]) into type …
- Cannot deserialize the current json object because(e.g.{“name”:”
- Cannot deserialize the current JSON array into type because …
- Cannot deserialize because the type requires a json object
- Cannot deserialize the current JSON array – Experts Exchange
- How to serialize and deserialize JSON using C# – .NET | Microsoft Learn
- Convert string to JSON in C# – C# Corner
- Working with JSON – Learn web development | MDN
- How to create a JSON string in C# – Educative.io
- Cannot deserialize the current JSON object (e.g. {“name …
- Can’t Deserialize JSON Response | OutSystems
- JSON object deserialize | Blazor Forums – Syncfusion
See more: nhanvietluanvan.com/luat-hoc